Ansible can be used to automate the management of Git repositories by executing git commands within playbooks, enabling seamless version control integration in your deployments.
Here's a simple example of how to use Ansible to clone a Git repository:
- name: Clone a Git repository
git:
repo: 'https://github.com/yourusername/yourrepository.git'
dest: '/path/to/destination'
Benefits of Integrating Git with Ansible
Integrating Ansible with Git offers numerous advantages that enhance your automation processes. This combination ensures that your infrastructure as code remains organized, collaborative, and resilient.
Version Control for Playbooks
With Git as your version control system, managing playbook versions becomes much easier. Each modification is tracked, allowing you to refer back to prior versions easily. This is invaluable for teams working on complex infrastructure setups, as you can ensure that updates are deliberate and reversible.
Collaboration Among Teams
Using Git alongside Ansible promotes team collaboration. Multiple users can work on different features simultaneously, and Git handles conflicts when code changes overlap. Instead of wrestling with conflicting versions, teams can focus on achieving their automation goals.
Rollback Capabilities
One of the most compelling reasons to utilize Git with Ansible is its rollback capability. If a deployment goes awry, you can quickly revert to the last stable version of your playbook. This ensures that disruptions are minimized, and your systems remain operational.
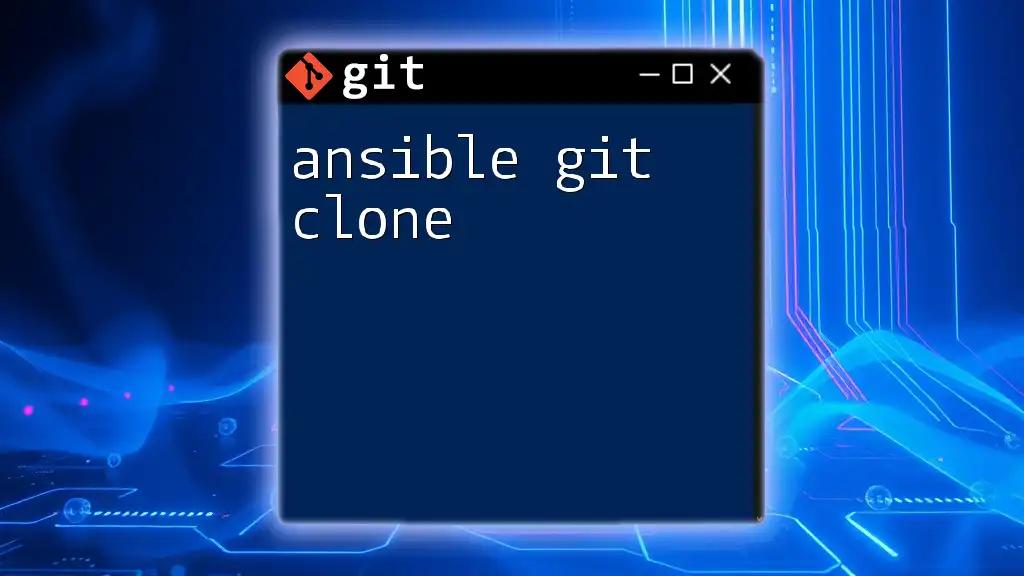
Setting Up Your Environment
Prerequisites
Before diving into Ansible and Git, ensure you have the required software. You need both tools installed on your local machine or server. Installing Essentials can usually be done via package managers, like `apt` for Ubuntu or `brew` for macOS.
Creating a Git Repository
Creating a Git repository for your Ansible projects is straightforward. Here are the commands to get started:
git init my_ansible_repo
cd my_ansible_repo
This initializes a new Git repository, enabling you to start tracking changes right away!
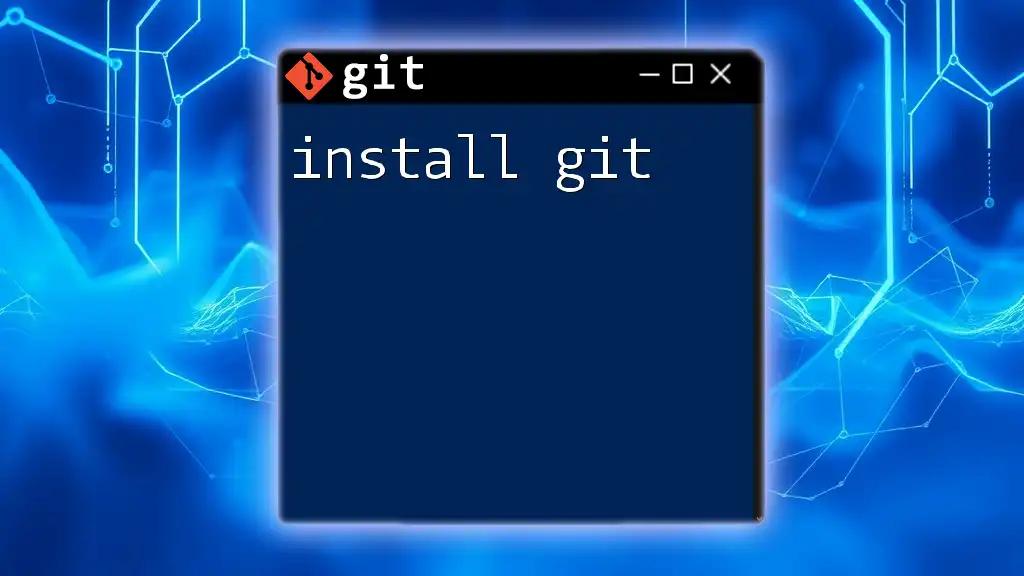
Basic Ansible Git Commands
Using Git within Ansible
Cloning a Repository
To start using playbooks that someone else has created, you need to clone a Git repository:
git clone https://github.com/yourusername/your_ansible_playbook.git
This command downloads a copy of the specified repository to your local environment, where you can begin modifying it as needed.
Committing Changes
Tracking changes to your playbooks is crucial. After you've made changes, stage and commit them as follows:
git add .
git commit -m "Updated playbooks for deployment"
Here, `git add .` stages all modified files, and `git commit` saves your changes with a descriptive message. This process keeps your repository organized and enables collaboration among team members.
Advanced Git Commands with Ansible
Branching Strategies
When working on new features or troubleshooting, it's best to use branches. You can create a new branch with:
git checkout -b feature/new-playbook
This command creates and checks out a new branch where you can freely make changes without affecting the main or production-active playbooks.
Merging Branches
Once your feature is complete, merging it back into the main branch is necessary. After switching to the main branch, use the following command:
git checkout main
git merge feature/new-playbook
Merging facilitates sharing your updates and ensures that your entire team has access to the latest code.
Resolving Merge Conflicts
Despite the convenience of Git, merge conflicts can arise—especially in collaborative projects. Common strategies for conflict resolution include:
- Understanding the conflicting changes highlighted by Git.
- Selecting which changes to keep or merging divergent changes logically.
- Utilizing `git status` and `git diff` to gain insights into conflicts.
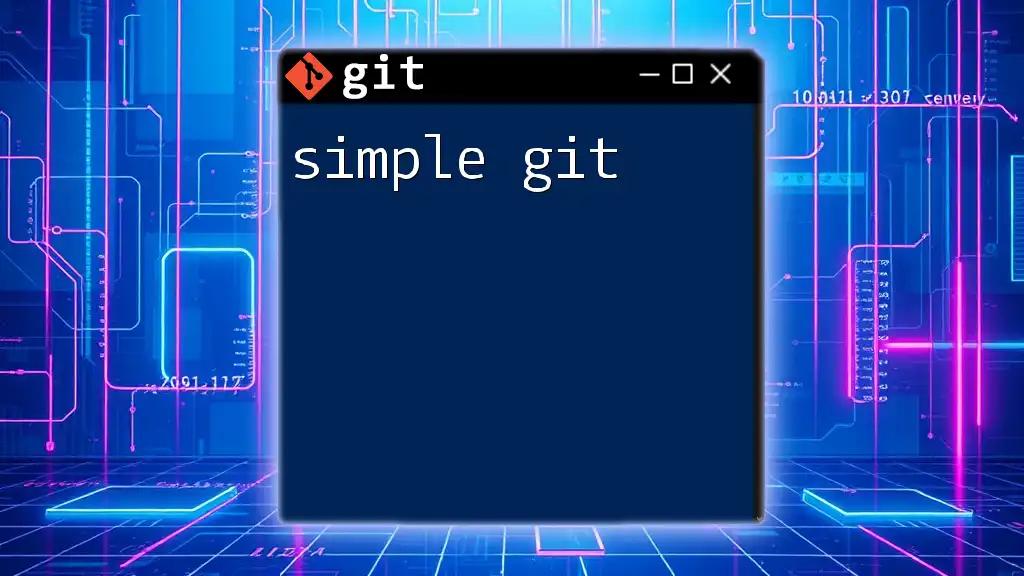
Automating Deployments with Git and Ansible
CI/CD Integration
Effective automation transcends local development; incorporating Continuous Integration (CI) and Continuous Deployment (CD) practices maximizes your deployment efficiency. Integrating CI/CD pipelines with Git and Ansible dramatically enhances deployment speeds while maintaining quality.
Triggering Ansible Playbooks with Git Hooks
Git hooks are scripts that run automatically at various stages of the Git lifecycle. For example, a `post-receive` hook can trigger an Ansible playbook deployment after code is pushed to the repository. Here's a snippet of what this might look like:
#!/bin/sh
git --work-tree=/path/to/your/app --git-dir=/path/to/your/repo checkout -f
ansible-playbook /path/to/playbook.yml
With this setup, your playbook executes upon every successful Git push, providing automated infrastructure updates with minimal manual intervention.
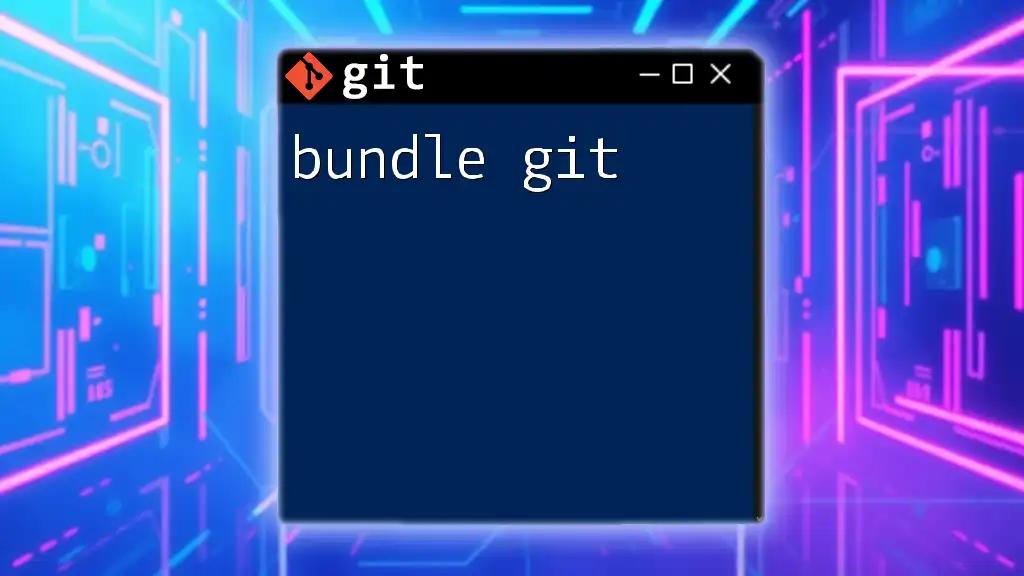
Best Practices
Structuring Your Ansible Repositories
Organizing your Ansible repository is crucial for long-term success. Group related playbooks, roles, and inventories clearly. A recommended hierarchy includes directories for `roles`, `inventory`, and `playbooks`. This structure not only enhances readability but also promotes reusability.
Using Semantic Versioning
Using semantic versioning ensures that everyone understands the significance of each version of your playbooks. Semantic versioning typically follows the format `MAJOR.MINOR.PATCH` to indicate the nature of changes made.
Documentation Within Your Git Repo
Good documentation should accompany every repository. Include `README.md` files that explain the purpose of your playbooks, along with usage instructions. This practice enhances onboarding for new team members and provides clarity on the project for all contributors.
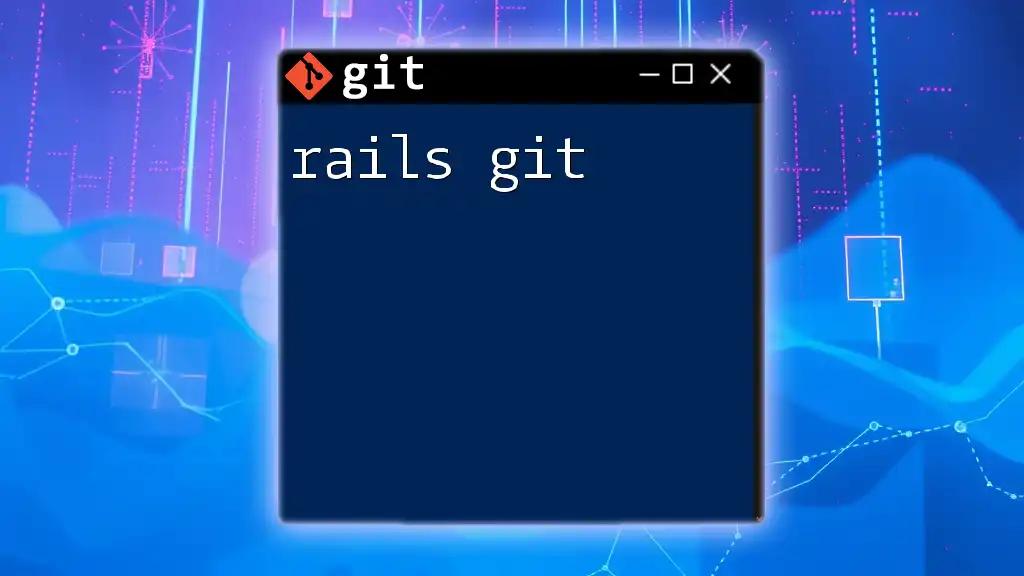
Troubleshooting Common Issues
Common Git Issues
Familiarize yourself with typical problems when using Git, like user-permission issues, conflicts when merging, or problems with submodules. Knowledge of these pitfalls helps in smooth collaboration and effective problem-solving.
Diagnosing Ansible-Focused Problems
When playbooks fail to execute correctly, debugging steps include checking output logs, using `ansible-playbook` flags like `-vvv` for verbose output, and reviewing the code for issues. Understanding common error messages allows for quicker resolutions.
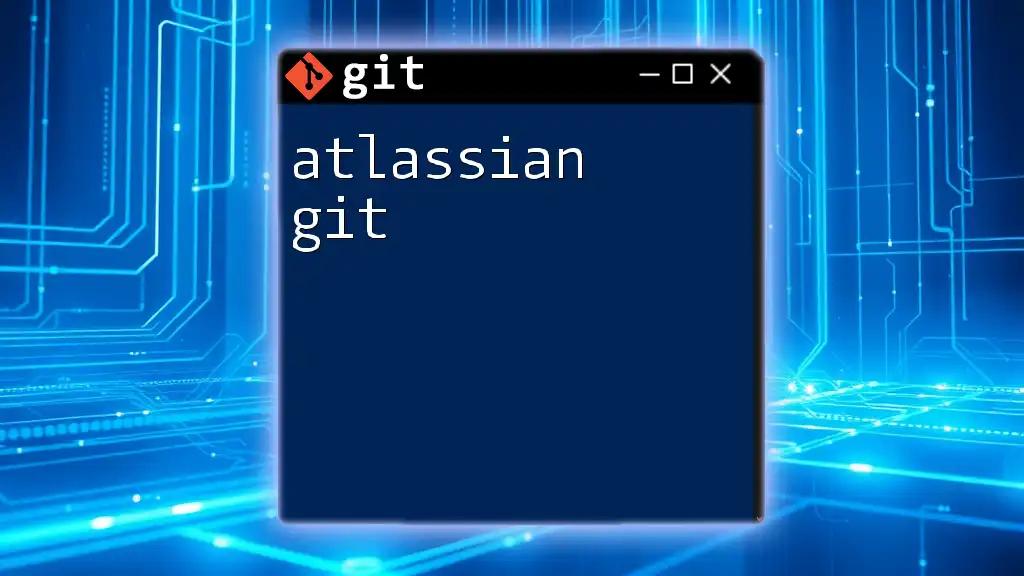
Conclusion
The integration of Ansible and Git is a powerful strategy for managing infrastructure as code. The benefits of version control, team collaboration, and rollback capabilities far outweigh the initial setup efforts. By embracing these tools, you're setting the stage for streamlined deployments, improved management, and a more collaborative development environment.
Additional Resources
For continued learning, explore additional resources such as blogs, videos, and courses focused on mastering Ansible and Git. These resources can deepen your understanding and enhance your skills.
Useful Commands Reference
Keep a cheat sheet of essential Git commands at hand for quick reference, especially for operations related to branching, merging, and viewing logs. Using these commands effectively streamlines your workflow and results in more efficient operations.