In this post, we'll explore how to efficiently use Git commands within Neovim (nvim) to enhance your workflow and version control.
Here's a quick example of how to stage and commit changes in a Git repository using nvim's integrated command mode:
git add .
git commit -m "Your commit message"
Understanding nvim
Neovim, often abbreviated as nvim, is an extended fork of Vim that aims to improve its usability and provide better performance. By incorporating modern programming concepts, it offers advanced features such as built-in terminal support, improved plugin architecture, and a powerful API. This makes nvim not only suitable for seasoned developers but also for anyone looking to enhance their coding experience.
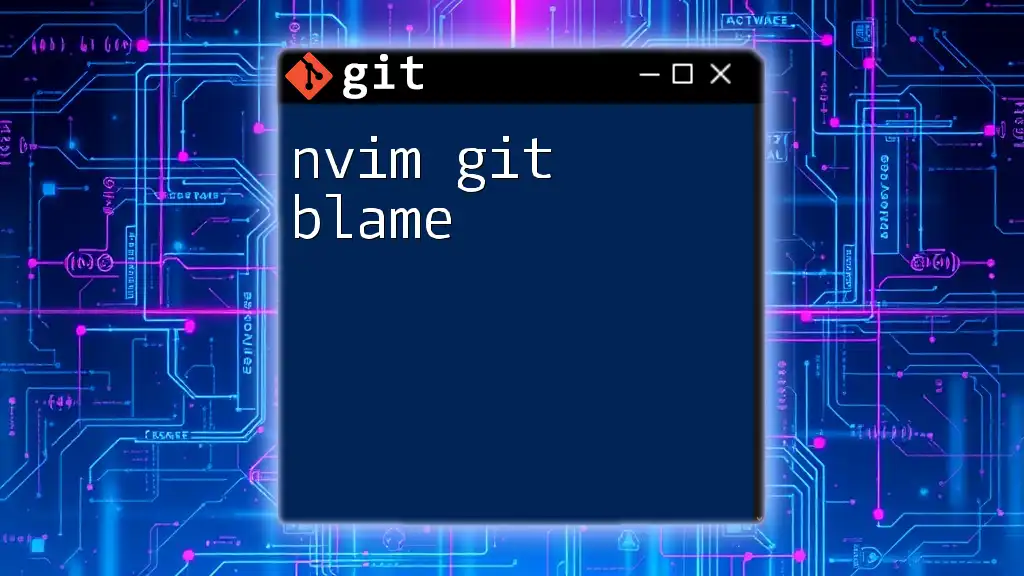
Why Use Git with nvim?
Integrating Git with nvim allows users to harness the power of version control directly within their coding environment. This integration can streamline workflows, minimizing context switches and boosting productivity. With Git commands accessible right from your text editor, you can manage and track your code changes without leaving your development environment, making it an efficient solution for both solo and team projects.
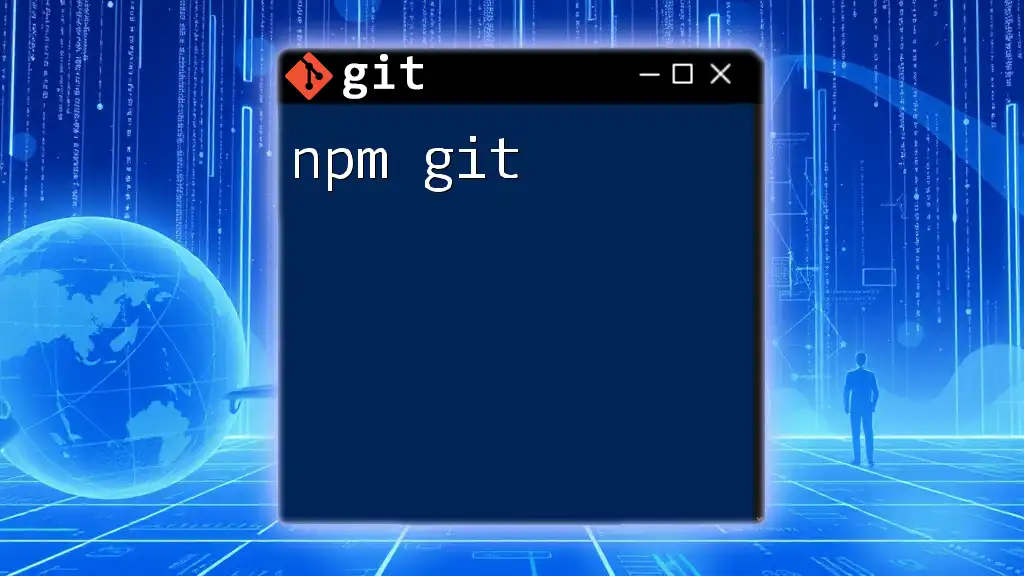
Setting Up Your Environment
Installing Neovim
To start using nvim, you'll first need to install it. Below are the steps for various operating systems:
-
Windows: You can download the latest release from [the Neovim GitHub Releases page](https://github.com/neovim/neovim/releases). Alternatively, use a package manager like Scoop or Chocolatey:
scoop install neovim
-
macOS: Use Homebrew for a quick installation:
brew install neovim
-
Linux: Installation methods may vary by distribution. For Ubuntu, you can use:
sudo apt install neovim
Installing Git
Git can be installed across different platforms as follows:
-
Windows: Download the Git installer from [the official Git website](https://git-scm.com/download/win) and follow the setup instructions.
-
macOS: Use Homebrew:
brew install git
-
Linux: For Ubuntu, use:
sudo apt install git
Configuring Neovim
To leverage the full potential of nvim with Git, you'll want to create or modify your configuration file, typically named `init.vim` or `init.lua`. This file can be found in `~/.config/nvim/`.
Here’s how to set up basic configurations:
" init.vim configuration example
set number " Show line numbers
set relativenumber " Show relative line numbers
syntax on " Turn on syntax highlighting
filetype plugin indent on " Enable file type detection
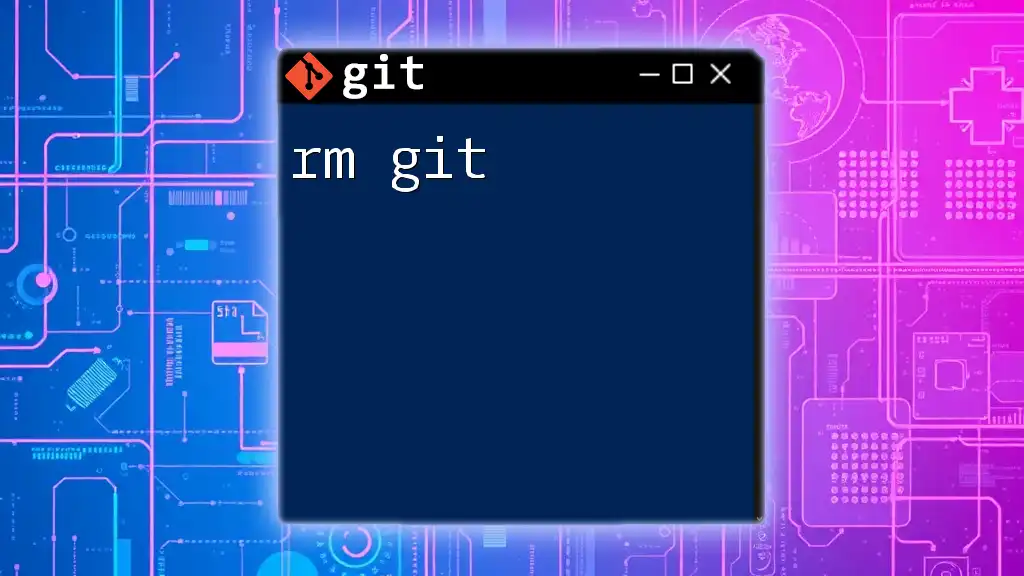
Essential Plugins for Git Integration
Why Use Plugins?
Plugins add extra functionality to Neovim, enabling features that enhance the development experience. Git integration plugins can simplify common tasks, making it easier to navigate and manage repositories.
Must-Have Git Plugins
-
Fugitive.vim: One of the most popular Git plugins for Vim/nvim, Fugitive provides an easy-to-use interface for performing Git commands.
To install Fugitive, add the following to your plugin manager configuration. For example, if you’re using `vim-plug`:
Plug 'tpope/vim-fugitive'
After installing, you can use various commands:
:Gstatus " Show the current status of the git repository :Gcommit " Commit changes in the buffer
-
Gina.vim: Another great Git plugin that offers advanced functionality like asynchronous operations and a more streamlined interface.
Install using:
Plug 'lambdalisue/gina.vim'
Some commands include:
:Gina status " View the git status :Gina commit " Commit changes with a message
-
Other Recommended Plugins: Consider plugins like `vim-gitgutter`, which provides visual indicators for git changes in the gutter, or `vim-signify` for similar functionality.
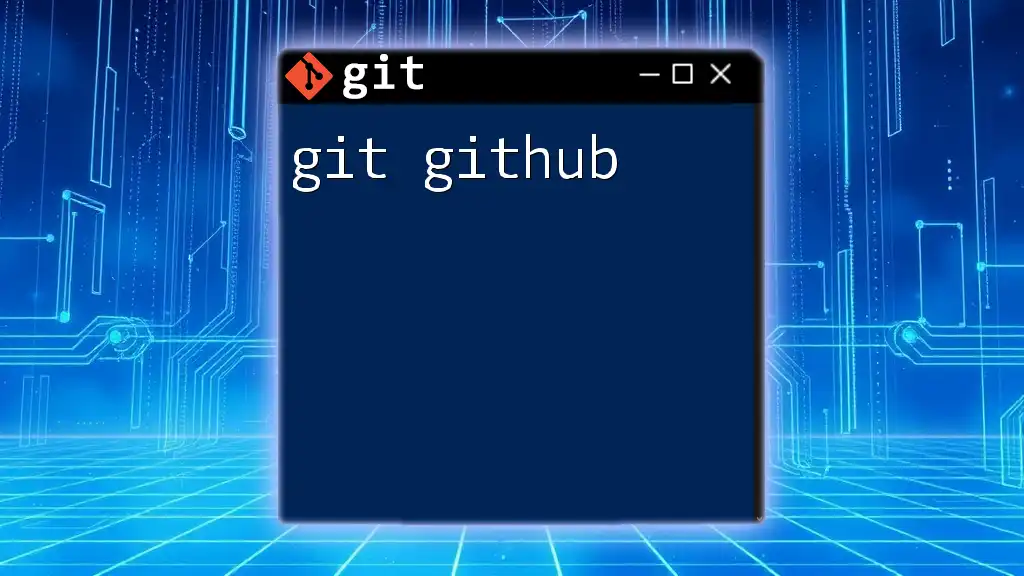
Basic Git Commands in Neovim
Viewing Your Repo Status
To check the status of your repository, simply use:
:Gstatus
This command will show you modified files, staged changes, and untracked files, all accessible within the nvim interface.
Committing Changes
To commit your changes, use:
:Gcommit
This launches a commit buffer where you can type your commit message. Save and exit the buffer to apply the commit.
Branching and Merging
Creating a new branch can be done with:
:!git checkout -b my-branch
To switch branches, use:
:!git checkout other-branch
When merging branches, typically you would want to switch to the branch you want to merge into first and then run:
:!git merge my-branch
Conflict Resolution
When conflicts occur, you can utilize nvim's built-in diffing capabilities. Start by running:
:!git mergetool
This will open the conflicting files, allowing you to resolve conflicts directly in the editor using the diff view.
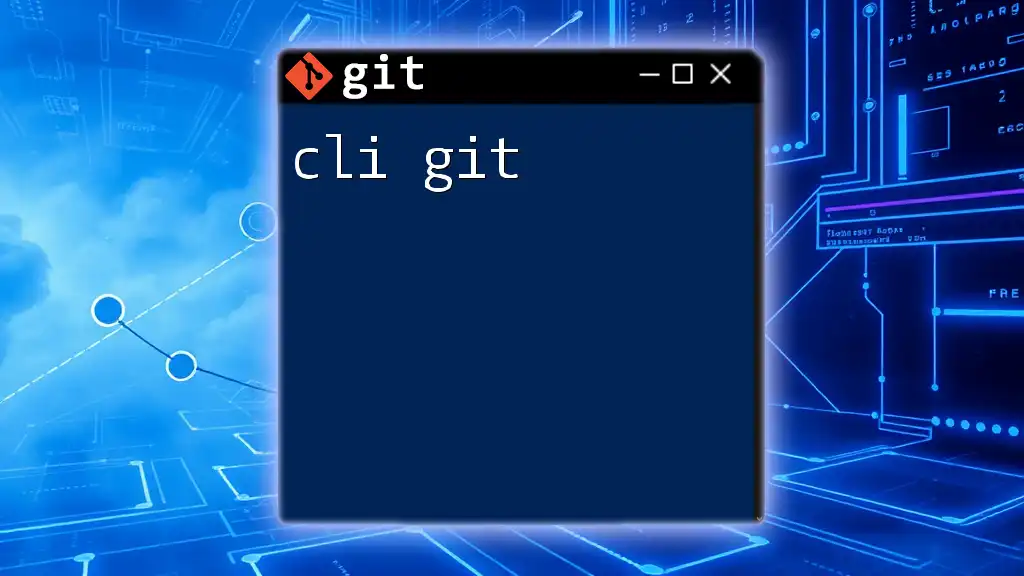
Advanced Git Operations in Neovim
Interactive Rebase
To edit multiple commits, an interactive rebase is invaluable, done by issuing:
:!git rebase -i HEAD~3
This will allow you to specify how to edit the last three commits—perfect for cleaning up history before pushing changes.
Stashing Changes
If you need to temporarily shelve uncommitted changes, you can stash them with:
:!git stash
And to apply a stashed change later:
:!git stash pop
Using Git Logs
To view your commit history, use:
:Glog
This command helps you browse your commit history without leaving nvim, allowing for easy searching and navigation through past messages.
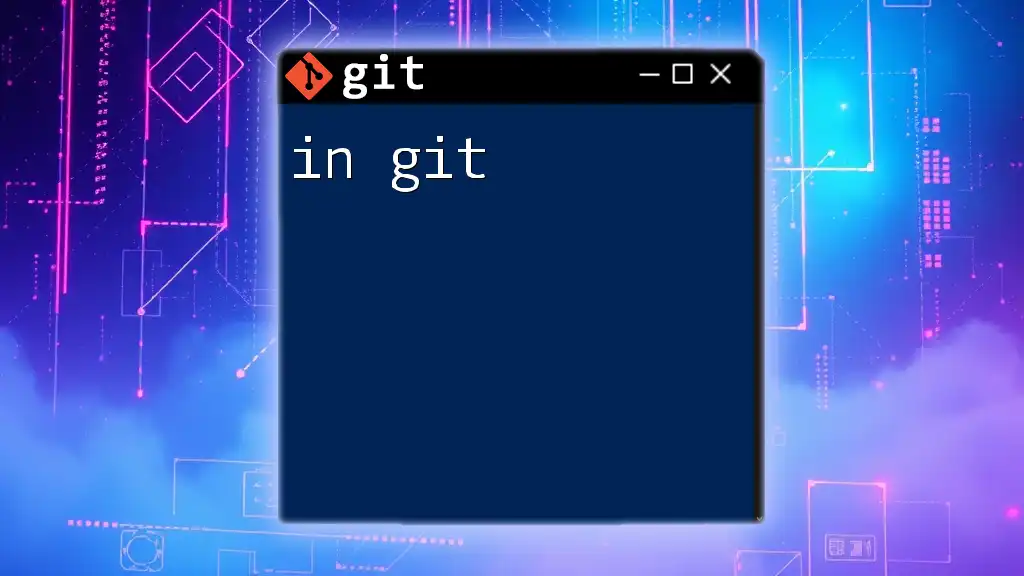
Tips and Tricks for Efficient Workflow
Keyboard Shortcuts
Customizing key mappings can vastly improve your efficiency. To create shortcuts for Git commands, you could add something like this to your `init.vim`:
nnoremap <leader>gs :Gstatus<CR> " Press 'space' + 'gs' to run :Gstatus
nnoremap <leader>gc :Gcommit<CR> " Press 'space' + 'gc' to run :Gcommit
Integrating with Other Tools
Using `Tmux` alongside Neovim can facilitate a powerful development environment. A setup with split panes for your editor and terminal can streamline tasks, allowing you to run Git commands while coding.
Workflows for Teams
When collaborating on projects, it’s crucial to establish a consistent branching strategy and commit message guidelines. Using tools like pull requests and reviews in conjunction with Git will result in a more fluid team workflow.
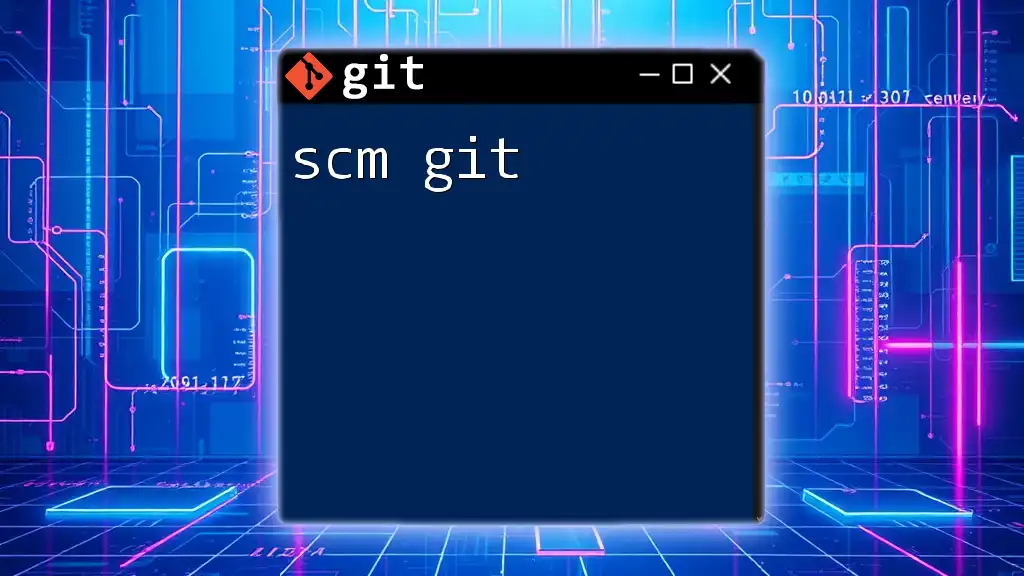
Troubleshooting Common Issues
Common Setup Problems
If you encounter problems during installation, ensure that you’ve added your PATH correctly to your shell profile. This is a common issue in both Git and Neovim setups.
Debugging Plugins
If a plugin fails to work, check your configuration for typos or errors. You can run Neovim in verbose mode to gather more information about what might be going wrong:
nvim -V3logfile
This command will output detailed logs to help you debug issues.
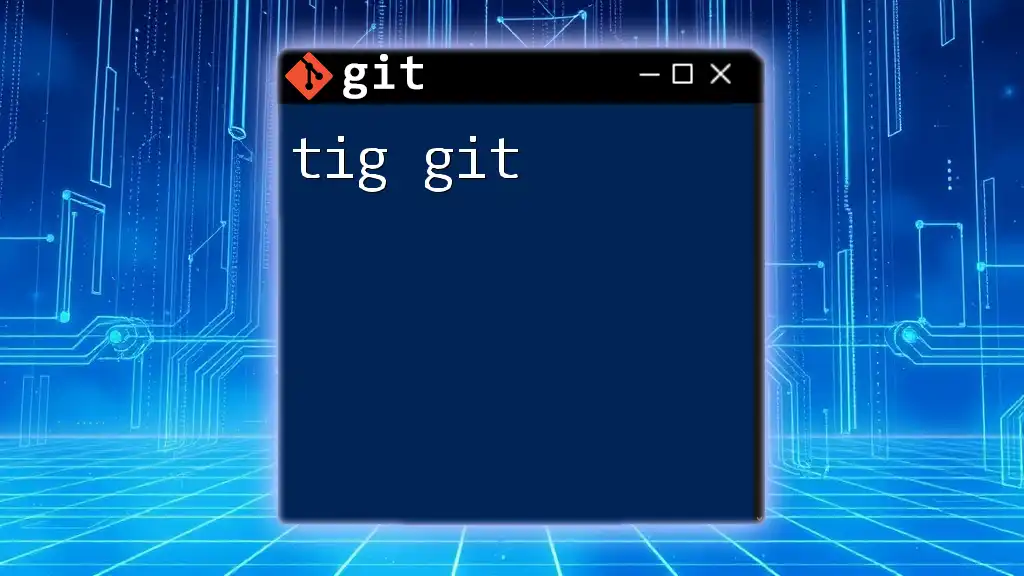
Conclusion
Integrating Git with nvim significantly enhances your coding efficiency, allowing you to manage version control alongside your development work seamlessly. By setting up your environment, mastering essential commands, and utilizing plugins, you can leverage the full power of both nvim and Git to elevate your programming experience.
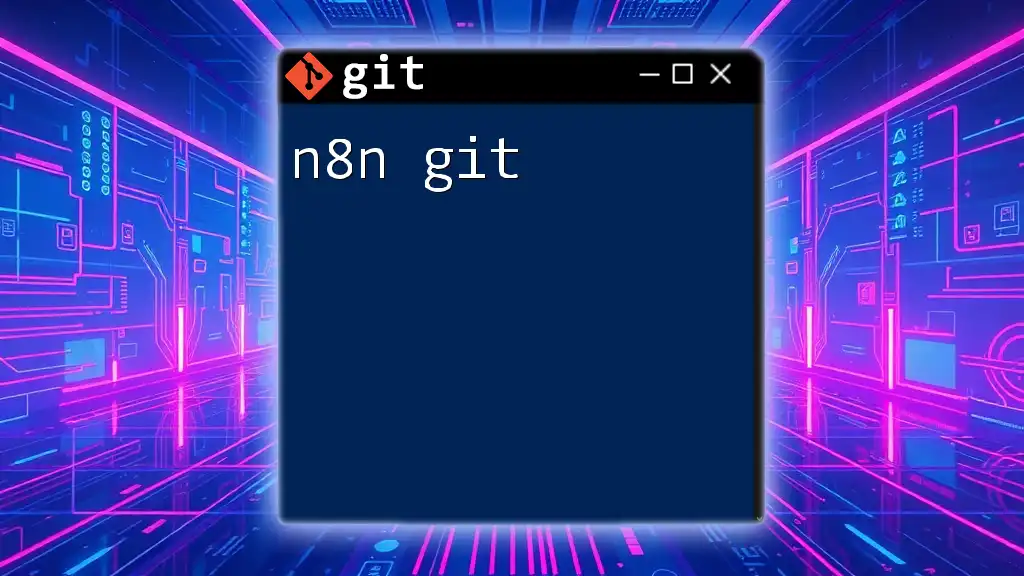
Additional Resources
For those looking to delve deeper into utilizing nvim and Git effectively, consider reading books on Git best practices or exploring community forums like Stack Overflow and Reddit. Engaging with other users will enhance your skills and understanding further.