The "python git" refers to the process of using Git for version control in Python projects to manage source code efficiently and collaborate with others seamlessly.
Here's a basic example of how to initialize a new Git repository in a Python project:
git init my-python-project
cd my-python-project
This command initializes a new Git repository in a directory called `my-python-project` and navigates into that directory.
Understanding Git Basics
What is Git?
Git is a powerful Version Control System (VCS) that allows developers to track changes in code, collaborate efficiently, and manage project history. By using Git, Python developers can ensure that their code is reliably maintained over time, providing the ability to revert to previous versions if necessary. This functionality is particularly useful in team environments where multiple developers contribute to the same codebase.
Key Git Concepts
In the world of Git, certain terms are fundamental to understanding how it works:
-
Repository: This is where your project’s files and its entire revision history are stored. A repository can be local (on your machine) or remote (on a platform like GitHub).
-
Commit: A commit represents a snapshot of your project at a specific point in time. Each commit includes a message that describes what changes were made, making it easier to track progress.
-
Branches: Branches allow developers to work on different features or bug fixes in isolation without affecting the main codebase. This enables flexible experimentation without the risk of breaking the main application.
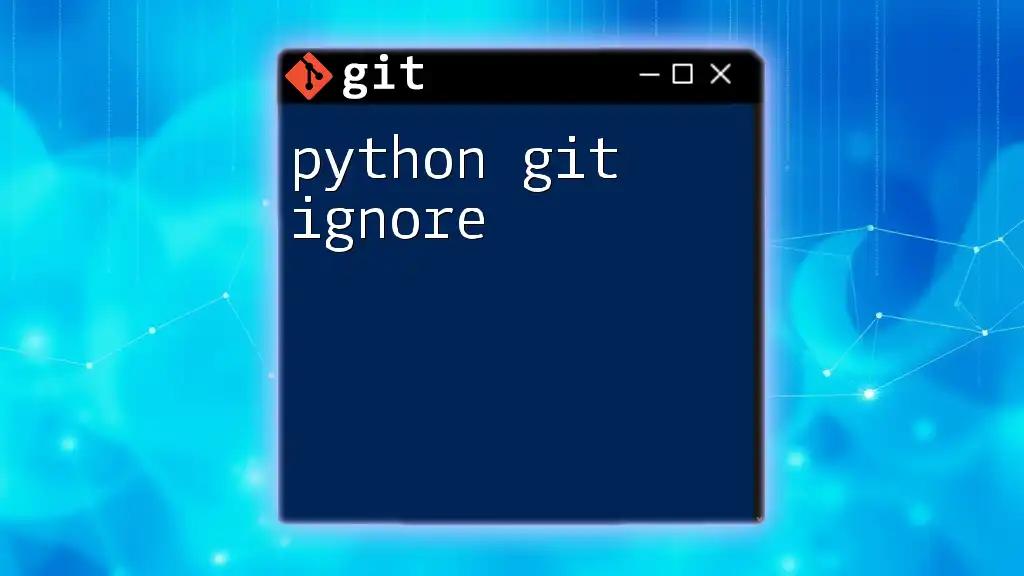
Setting Up Git for Your Python Project
Installing Git
To get started, you’ll first need to install Git. The installation process varies depending on your operating system:
-
Windows: Download the Git installer from the official Git website. Follow the installation instructions.
-
macOS: You can install Git via Homebrew with the command:
brew install git
-
Linux: Use your package manager. For instance, on Ubuntu, you can run:
sudo apt-get install git
After installation, verify it by running:
git --version
This will display the installed Git version, confirming that Git is ready for use.
Creating Your First Repository
Creating a new repository for your Python project is straightforward. Here’s how:
-
Create a directory for your project:
mkdir my_python_project cd my_python_project
-
Initialize the repository:
git init
Upon running this command, Git creates a hidden `.git` folder within your project directory. This folder contains all the metadata and history of your project. Understanding what’s inside can help you troubleshoot issues or track changes at a granular level.
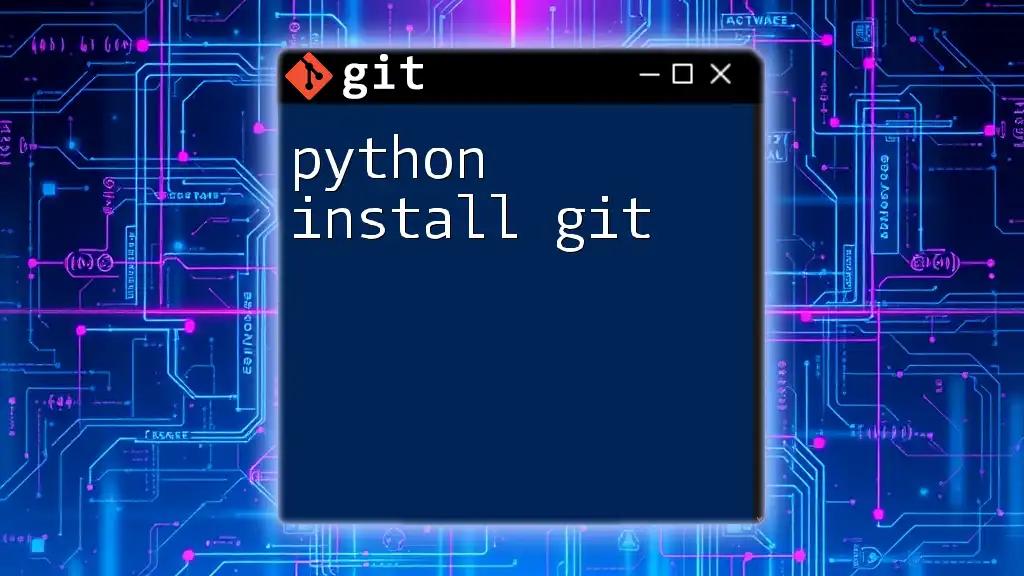
Basic Git Commands for Python Developers
Checking Git Status
To see the current state of your repository, you can use:
git status
This command provides information on which files have been modified, staged for commit, or remain untracked. This is crucial for understanding and managing your workflow.
Adding Changes
When you're ready to include changes in the next commit, use:
git add .
This command stages all modified and new files. By selectively adding files, you can maintain control over what gets included in your commits.
Committing Changes
Once you’ve staged your changes, commit them with a descriptive message:
git commit -m "Your commit message"
Having a clear and concise commit message is critical. It facilitates understanding of what changes were made and why. For instance:
git commit -m "Fix bug in user authentication"
Such practices lead to better-maintained project histories, especially when revisiting the code months later.
Viewing Commit History
To view the history of commits in your repository, run:
git log
This command outputs a detailed history of commits, showing the commit ID, author, date, and message. You can navigate this log to track changes over time or to revert to specific versions of your code.
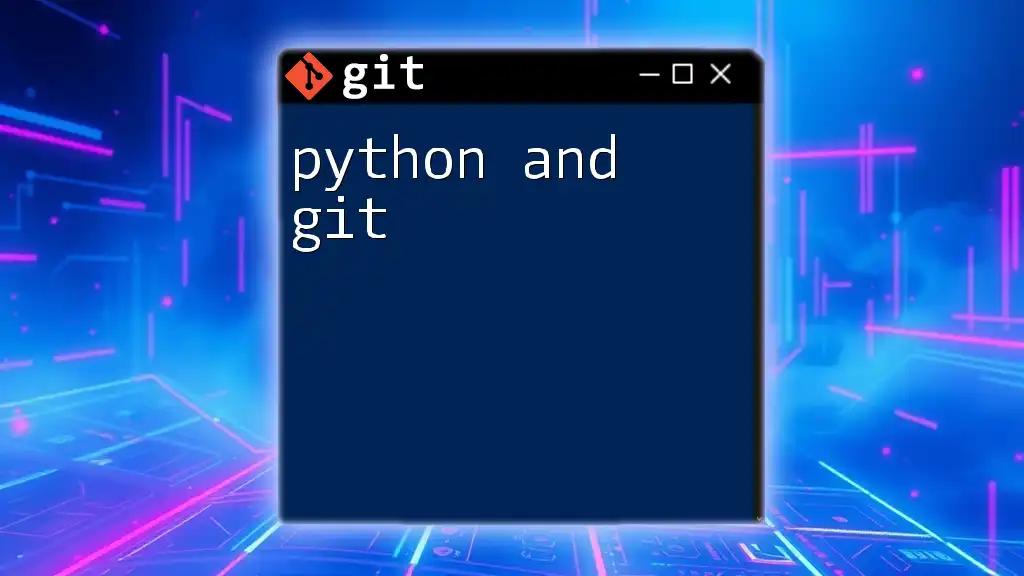
Branching and Merging in Git
Creating and Switching Branches
Branches allow you to work on new features without affecting the main codebase. To create a new branch, use:
git branch feature_branch
To switch to this branch, run:
git checkout feature_branch
You can also combine these two commands:
git checkout -b feature_branch
This command creates and switches to a new branch in one go.
Merging Branches
Once you have made changes in your feature branch, you may want to merge them back into the main branch (often named `main` or `master`). First, ensure you’re on the main branch:
git checkout main
Then merge the changes:
git merge feature_branch
If there are conflicts, Git will notify you, and you'll need to resolve them manually by editing the files in conflict before committing the merge.
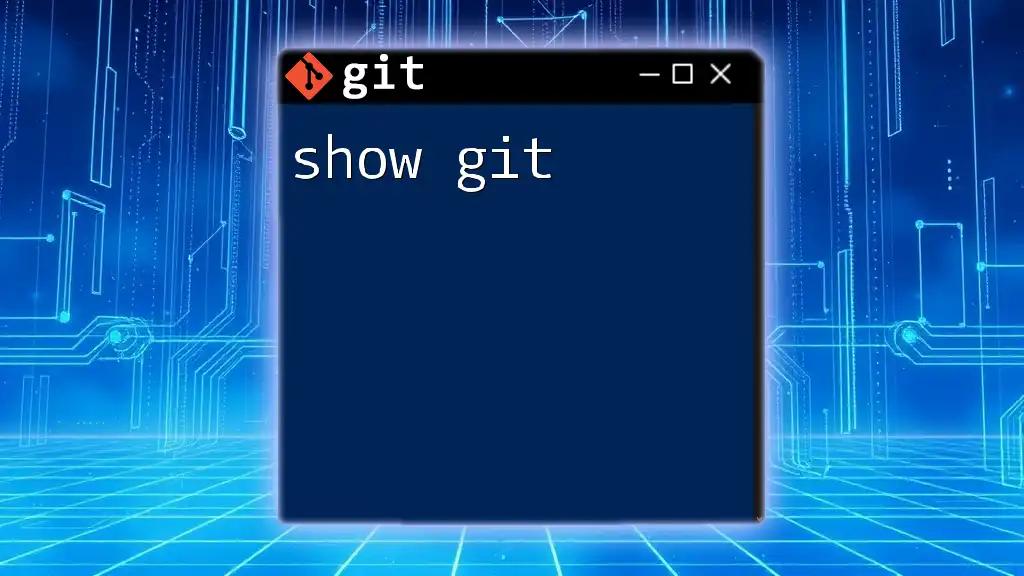
Using Git with Remote Repositories
Setting Up Remote Repositories
Remote repositories are hosted versions of your Git projects, often used for collaboration. GitHub is a popular choice. You can create a new repository there by following the prompts on their website.
Adding a Remote Repository
Once your repository is set up on GitHub, you can link it to your local repository with:
git remote add origin <repository-url>
This command connects your local repository to the remote one, allowing you to push and pull changes back and forth.
Pushing and Pulling Changes
To share your commits with the remote repository, use:
git push origin main
This uploads your local changes to the remote `main` branch, making them accessible to others.
To sync your local repository with changes made by others, run:
git pull origin main
This command fetches and merges updates from the remote repository into your local branch.
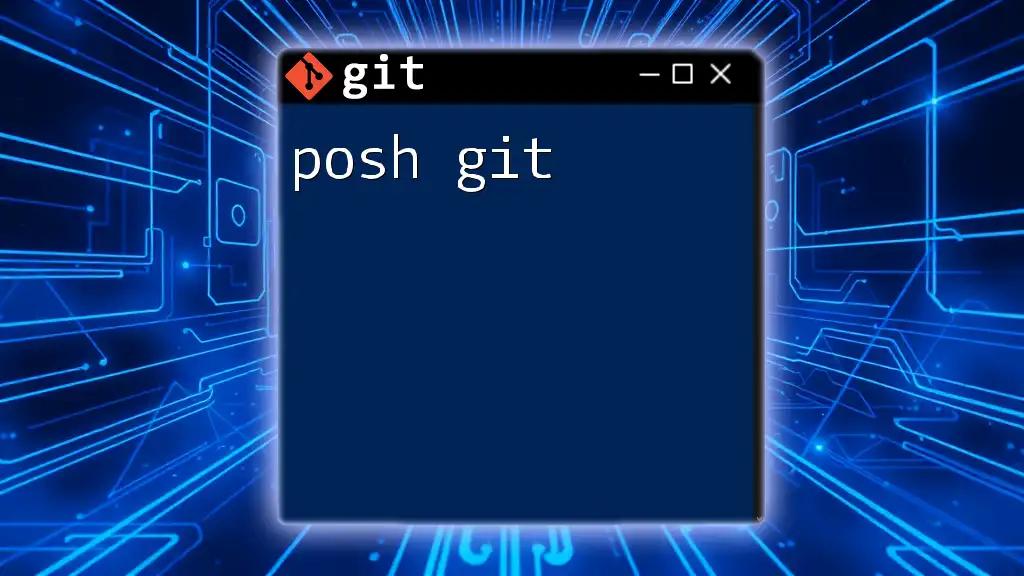
Python-specific Git Workflows
Maintaining Python Environments
In Python projects, it’s vital to keep your environment clean. Use a `.gitignore` file to specify which files and directories Git should ignore. Here’s an example `.gitignore` that excludes common Python artifacts:
# .gitignore example
venv/
__pycache__/
*.pyc
This setup ensures that unnecessary files, such as virtual environments or compiled Python files, don’t clutter your repository.
Collaborating on Python Projects
Collaboration often requires using pull requests. This practice fosters code reviews where teammates can suggest changes or improvements. Using Git effectively in these situations can streamline development and enhance code quality.
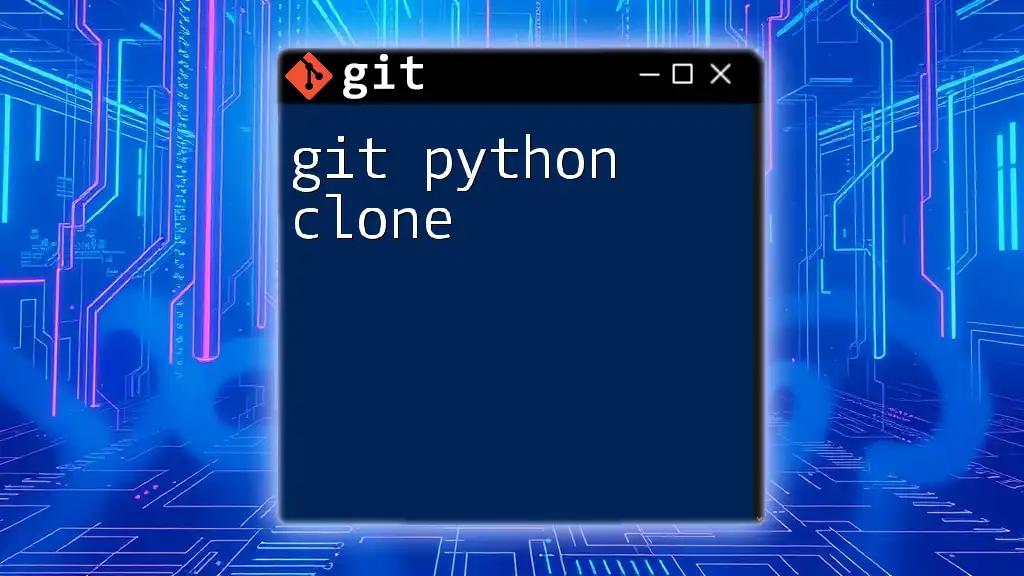
Advanced Git Features for Python Developers
Tags and Releases
Tags are essential for marking specific commits as noteworthy, such as releases. To create a tag, use:
git tag -a v1.0 -m "Release version 1.0"
Tags help other developers identify stable points in the project history, making version tracking clear.
Rebasing vs. Merging
While merging integrates changes from different branches, rebasing provides a cleaner commit history by moving the entire branch to the tip of another branch. When merging with:
git merge feature_branch
you create a merge commit, while rebasing with:
git rebase main
repositions your feature branch onto the main branch, offering a linear history. Understanding when to use each method is crucial for maintaining a tidy project timeline.
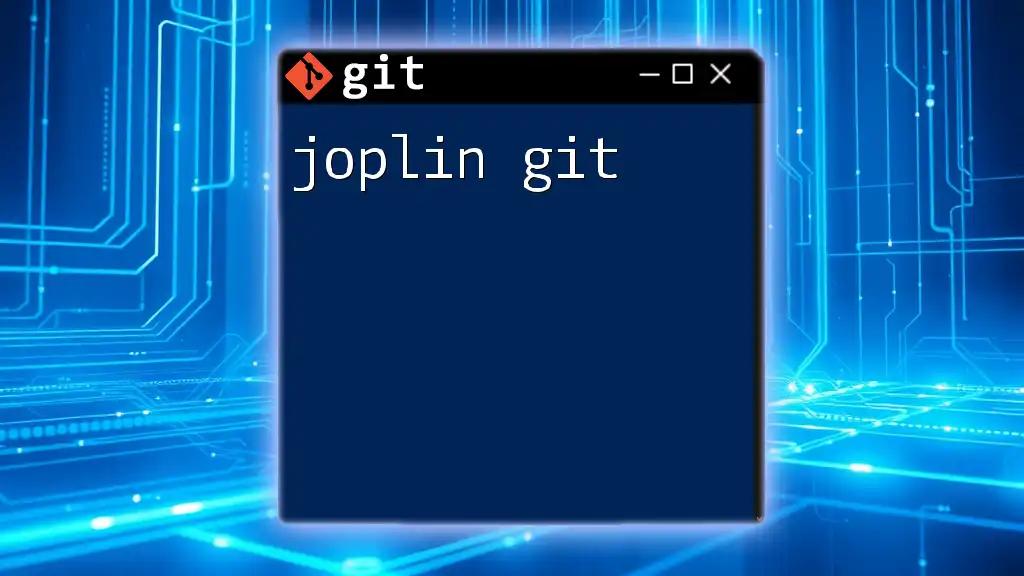
Troubleshooting Common Git Issues
Fixing Merge Conflicts
Merge conflicts arise when two branches modify the same part of a file differently. Git will highlight these conflicts during a merge. Open the file, resolve the conflicts by choosing which changes to keep, and then stage and commit the resolution.
Example of a conflict in Python code:
def example_function():
print("This is the new feature")
Compare and choose the necessary lines, resolving any discrepancies before finalizing the merge.
Undoing Changes
If you need to undo changes, you have several options. To discard modifications in a tracked file:
git checkout -- <file>
Alternatively, if you want to unstage a file before committing:
git reset <file>
These commands can safeguard your project from unintended modifications.
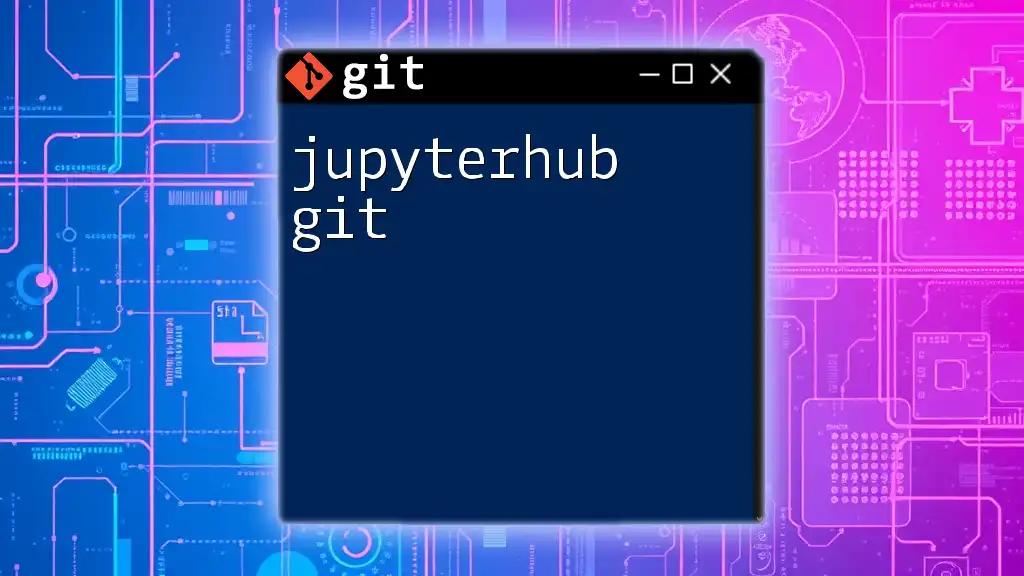
Conclusion
Integrating Git into your Python development significantly enhances your workflow, providing tools for version control, collaboration, and project management. Embracing Python Git will empower you to maintain clean, organized code with ease, enabling you to focus on building great software.
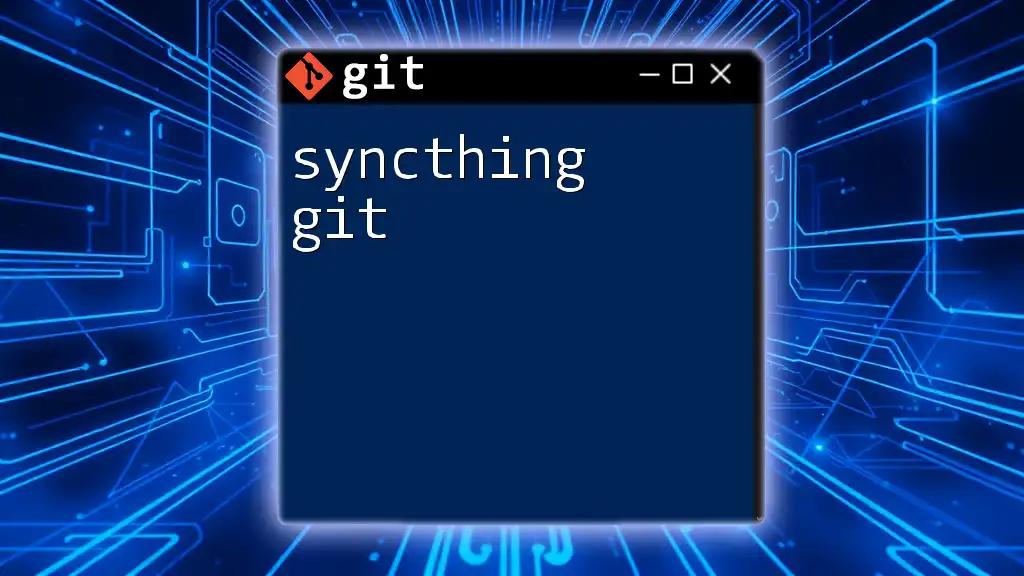
Additional Resources
For further learning, consult the official Git documentation, engage in community forums, and explore online courses to deepen your understanding of Git within the context of Python development. Dive in and start mastering Python Git today!