JupyterHub Git enables users to seamlessly integrate Git version control within JupyterHub environments, allowing collaborative coding and easy project management among multiple users.
Here’s a simple command to clone a Git repository in a JupyterHub terminal:
git clone https://github.com/username/repository.git
Setting Up JupyterHub with Git
Installing Git on JupyterHub
Prerequisites
Before installing Git, ensure that you have administrative permissions and that your server meets the necessary system requirements. This typically involves having an up-to-date Linux operating system.
Step-by-Step Installation Guide
To install Git on JupyterHub, use the following commands in the terminal:
sudo apt-get update
sudo apt-get install git
After running this command, confirm the installation by checking the installed Git version:
git --version
This should display the installed version, indicating a successful installation.
Configuring Git in JupyterHub
Setting Up User Information
Once Git is installed, it’s crucial to configure it with your identity. This information will be recorded in every commit you make. Set your user name and email address with these commands:
git config --global user.name "Your Name"
git config --global user.email "you@example.com"
Make sure to replace "Your Name" and "you@example.com" with your actual name and email address.
Global vs Local Configuration
Understanding the difference between global and local configurations is essential. The `--global` option applies your IAM settings across all repositories in your user account while local configurations can be set per repository using:
git config user.name "Local User"
git config user.email "local@example.com"
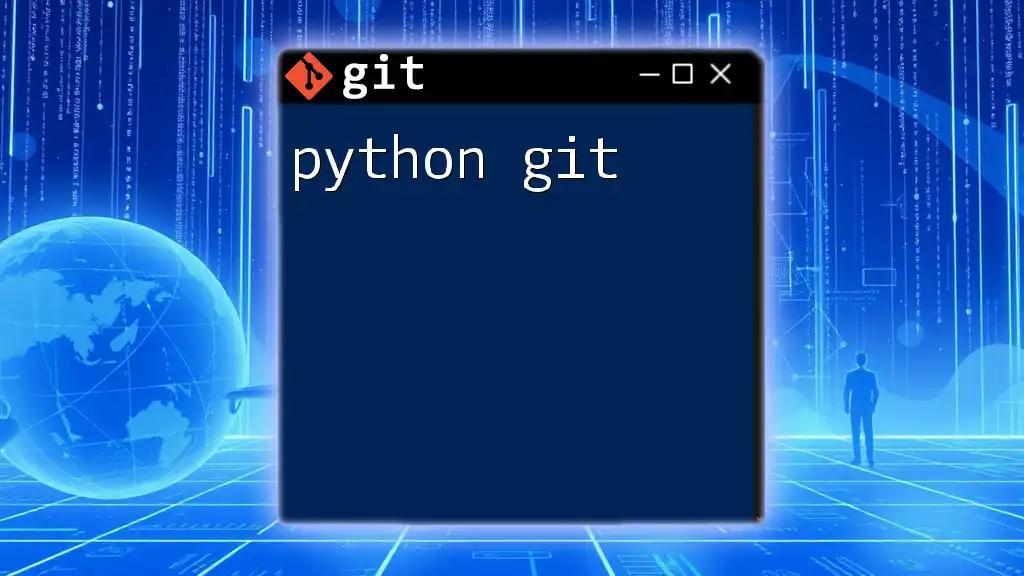
Creating and Cloning Repositories
Creating a New Git Repository
Initial Setup
To start a new project in JupyterHub, create a Git repository using the `git init` command within your desired directory. For example:
git init my_project
This command sets up a new directory named `my_project` with a `.git` directory that contains all the necessary metadata for version control.
Understanding the Directory Structure
Inside your project directory, the `.git` folder is central to Git's operations. It holds all your commit history, branches, and configuration files. Familiarity with this structure will help you troubleshoot any issues you encounter as you work.
Cloning an Existing Repository
How to Clone
If you wish to work with an existing Git repository, you can clone it to your local JupyterHub environment using:
git clone https://github.com/username/repo.git
This command will copy all files, branches, and history from the specified repository to your current directory.
Understanding Cloning Behavior
Cloning a repository means getting a complete copy of the project along with its entire history. This is particularly useful in collaborative projects where you want to contribute without starting from scratch.
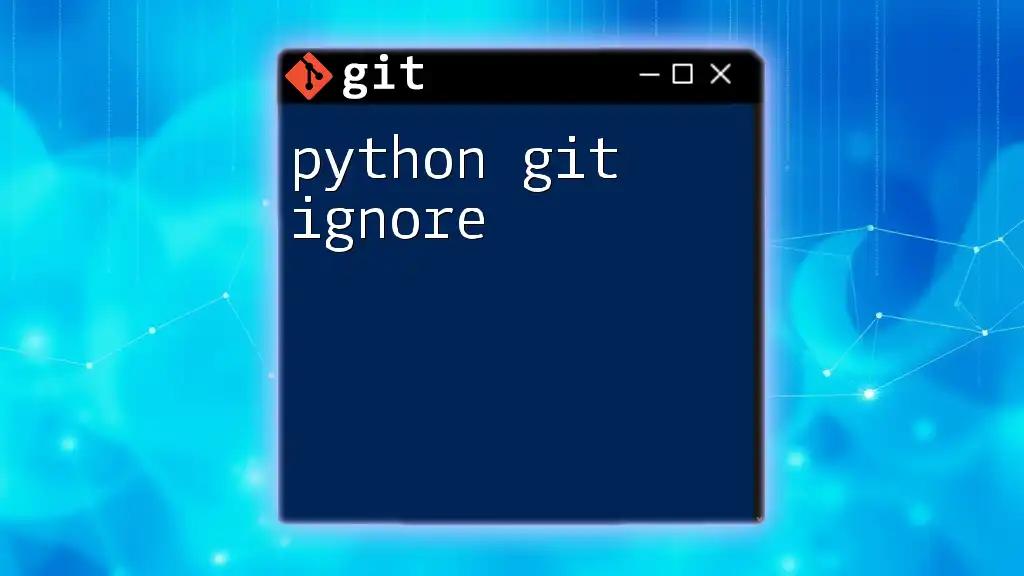
Basic Git Commands for JupyterHub Users
Essential Commands Overview
Stage and Commit Changes
Once you create or modify files in your repository, you need to stage and commit these changes. First, stage your changes using:
git add .
This command will stage all modified and new files. After staging, commit your changes with an appropriate message:
git commit -m "Initial commit"
Clear and concise commit messages are vital for understanding the history of your project, making it easier for collaborators to follow along.
Viewing Changes and History
Checking the Status
To check the current status of your Git repository, use:
git status
This command will show you any untracked files, modifications, and other relevant details, helping you assess what changes are ready to be committed.
Viewing Commit History
To review your project’s history, utilize:
git log --oneline
This concise version of the log displays each commit along with its unique identifier and message. It’s a quick way to understand the evolution of the project.
Branch Management
Creating and Switching Branches
Branches allow you to develop features or fix bugs in isolation. You can create a new branch using:
git branch feature-xyz
Then switch to this new branch with:
git checkout feature-xyz
Utilizing branches helps keep your main codebase clean while you experiment with new features.
Merging Changes
After completing work on a branch, you'll want to integrate your changes back into the main branch. To do this, first switch to the main branch:
git checkout main
Then merge your feature branch:
git merge feature-xyz
This combines the changes and keeps your project history intact.
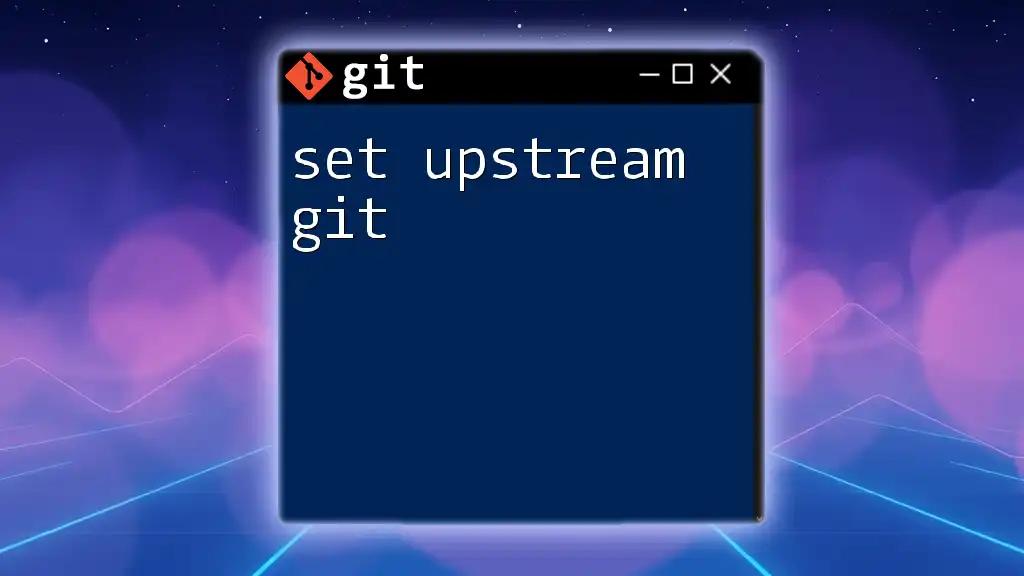
Working with Remotes
Adding Remote Repositories
Understanding Remotes
Remotes are versions of your repository hosted elsewhere, typically on platforms like GitHub. Working with remotes allows you to collaborate with others seamlessly.
Adding a Remote Repository
To connect your local repository to a remote one, you’ll use:
git remote add origin https://github.com/username/repo.git
This command establishes a link between your local repository and the remote repository you specify.
Pushing and Pulling Changes
Pushing Local Changes
Once your changes are ready to be shared, push them to the remote repository with:
git push origin main
This command sends your commits from the local main branch to the corresponding branch on the remote repository.
Pulling Updates from Remote
To incorporate changes made by collaborators into your local copy, use:
git pull origin main
This command fetches changes from the remote and automatically merges them with your local main branch.
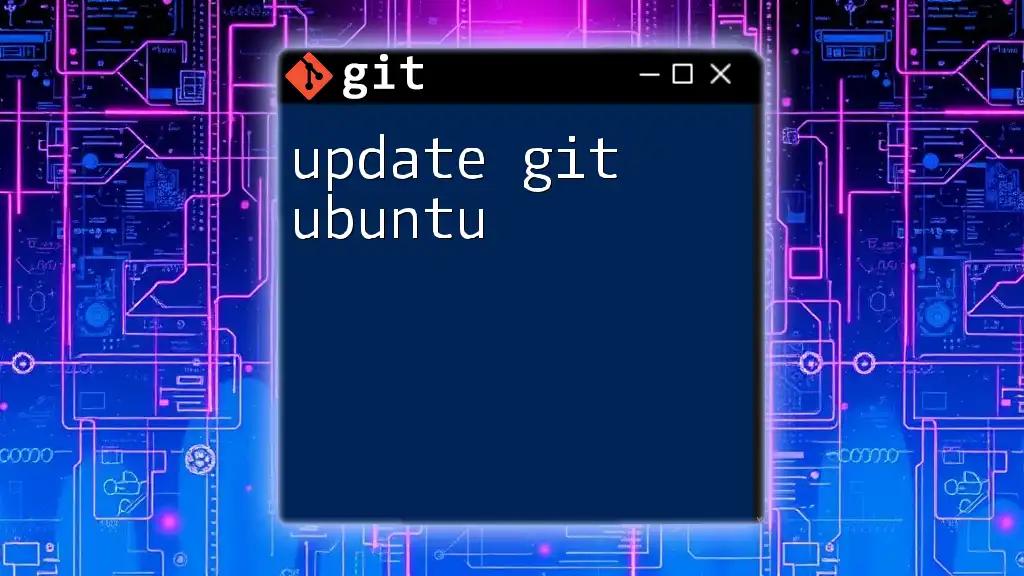
Best Practices for Using Git within JupyterHub
Commit Regularly
Establish a habit of committing often. Regular commits allow you to track progress and simplify the process of identifying changes made over time.
Write Clear Commit Messages
Effective commit messages provide context for the changes made. Aim for clarity and brevity. Start with what was changed, followed by why it was changed.
Branching Strategy
Adopt a branching strategy that suits your project’s needs. For example, consider using the "feature" branching model, where each new feature is developed in its own branch and merged back into main upon completion. This keeps your code organized and manageable.
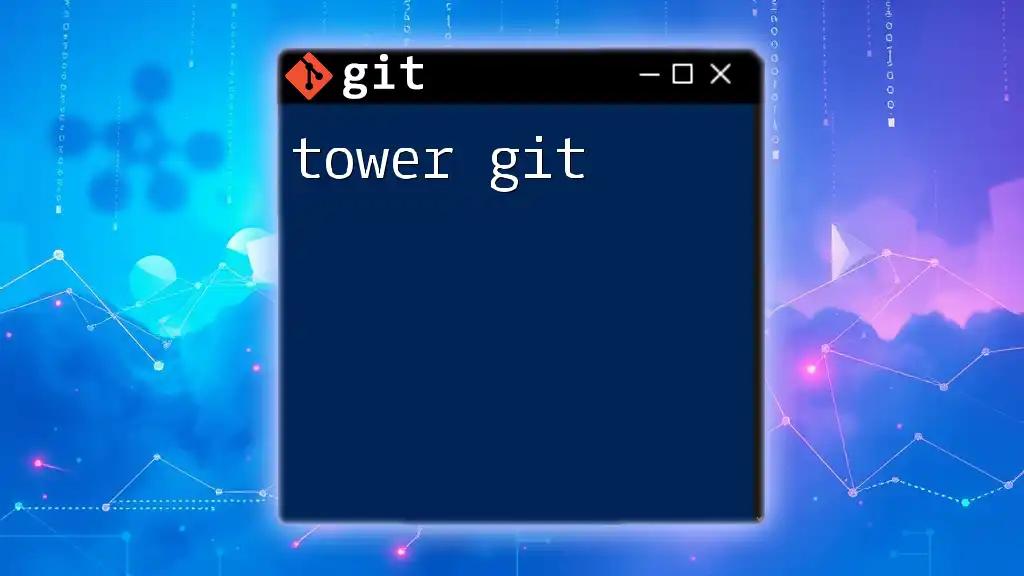
Troubleshooting Common Issues
Resolving Merge Conflicts
Conflicts can arise when merging branches, especially if changes diverge significantly. When this occurs, you’ll receive a message prompting you to resolve conflicts in affected files. Once resolved, stage the files and commit the changes:
git add conflicted-file
git commit
Undoing Changes
Mistakes happen, and knowing how to undo changes is crucial. You can revert uncommitted changes using:
git checkout -- file.txt
For rolling back committed changes, use `git revert` to safely create a new commit that undoes the changes from a previous commit.
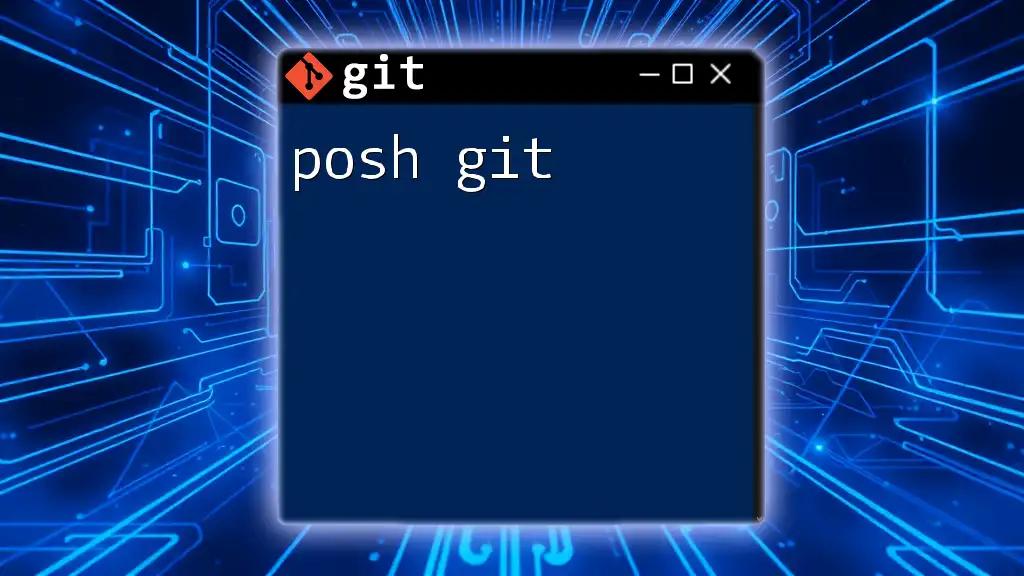
Conclusion
As you navigate through JupyterHub Git, remember that consistency and clarity are key. By establishing good habits now, you’ll enhance not only your workflow but also your collaboration with others. This guide equips you with the foundational tools needed to effectively manage projects in a collaborative JupyterHub environment. For continued learning, explore additional resources and documentation to deepen your Git expertise.