To set an upstream branch in Git, use the following command, which establishes a tracking relationship between your local branch and a remote branch:
git push --set-upstream origin <branch-name>
Replace `<branch-name>` with the name of your local branch that you want to link to the remote repository.
Understanding Upstream Branches
What Does Upstream Mean?
In Git, the term upstream refers to the remote repository branch that your local branch is tracking. By default, when you clone a repository, Git sets the local master branch to track the remote master branch from the default remote, typically named `origin`.
Understanding the difference between local and remote branches is crucial for effective version control. Local branches are branches that exist on your machine, while remote branches exist on the server. When you set an upstream branch, you allow the local branch to communicate seamlessly with the corresponding branch in the remote repository.
Why Set an Upstream Branch?
Setting an upstream branch offers several benefits:
- Simplified Workflow: Once you have an upstream branch set, you can easily pull changes from the remote repository or push your changes with fewer commands.
- Automatic Tracking: This allows Git to automatically know which remote branch your local branch corresponds to, making it easier to synchronize changes.
- Collaboration: In a team environment, maintaining correct upstream references helps avoid conflicts and fosters smoother collaboration. Understanding and setting upstream branches ensures everyone is on the same page regarding the latest changes.
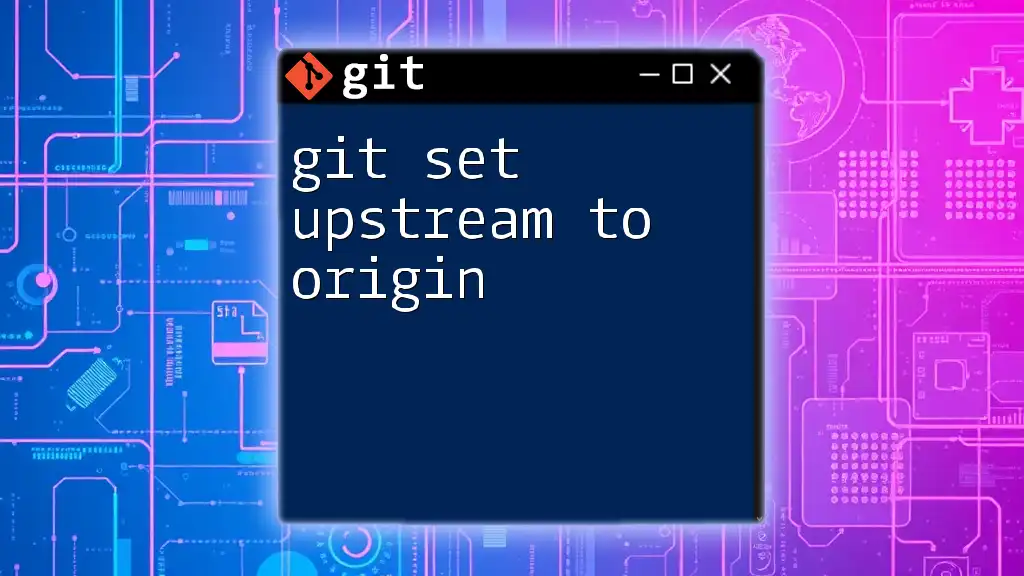
How to Set Upstream Branches
The Basic Command
To set an upstream branch for your local branch, you use the following command:
git push --set-upstream <remote> <branch>
- `<remote>`: This is usually `origin`, the default name for your remote repository.
- `<branch>`: This refers to the local branch you wish to set upstream, such as `master` or any feature branch.
This command pushes your changes to the remote repository and sets the specified branch as the upstream branch for your local branch.
Setting Upstream on New Branches
When creating a new branch, you can associate it with an upstream branch in one go. Here’s a step-by-step guide:
-
First, create and switch to your new branch.
git checkout -b <branch_name>
-
Then, push the branch and set the upstream reference.
git push --set-upstream origin <branch_name>
For example, if you want to create a new feature branch named `feature-login`, you would run:
git checkout -b feature-login
git push --set-upstream origin feature-login
This step creates your branch and sets it to track the remote `feature-login` branch on `origin`.
Setting Upstream for Existing Local Branches
If you have a branch that already exists locally but does not yet track a remote branch, you can set it easily using the following command:
git branch --set-upstream-to=<remote>/<branch>
For example, if you have a local branch named `bugfix` and wish to set it to track the remote `bugfix` branch, you would execute:
git branch --set-upstream-to=origin/bugfix
This informs Git that the local `bugfix` branch should now track the remote `bugfix` branch, facilitating easier updates between the two.
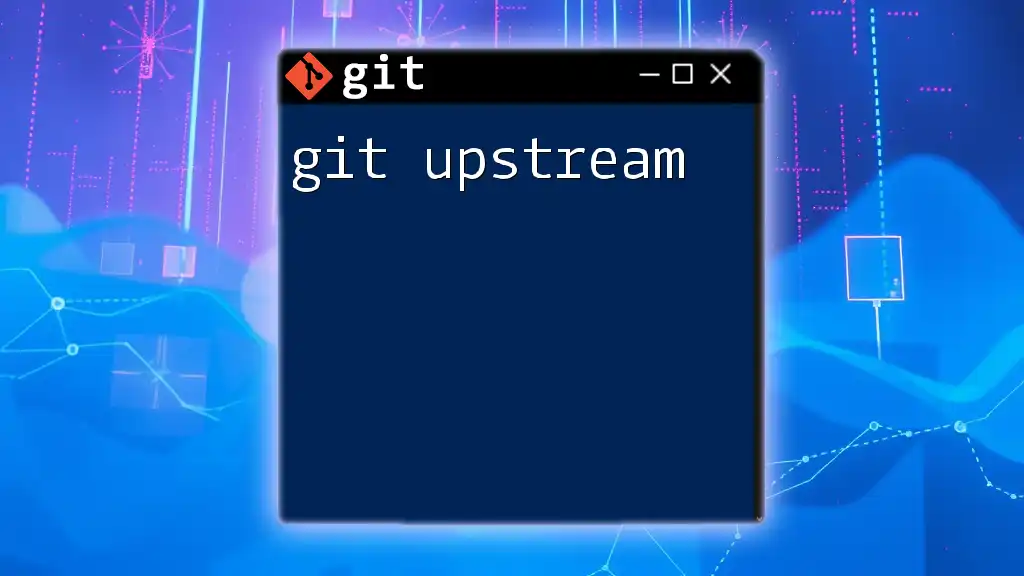
Managing Upstream Branches
Viewing Your Upstream Configuration
To check the upstream tracking configuration of your branches, use:
git branch -vv
This command displays all your local branches along with their tracking information. You’ll see both the current branch and its corresponding upstream branch listed, making it easy to identify if everything is set up correctly.
Understanding this output can alert you to any potential issues, such as branches that are not tracking any upstream, which could cause confusion during collaboration.
Changing the Upstream Branch
In some cases, you may need to change the upstream branch for an existing local branch. This can be done with:
git branch --set-upstream-to=<new_remote>/<new_branch>
Suppose you want to change the upstream branch of your local `feature-gallery` branch to track a different remote branch. You could use a command like this:
git branch --set-upstream-to=origin/feature-gallery-updated
This command will update the tracking information. It’s crucial when branches are renamed or if your team restructures the remote repository.
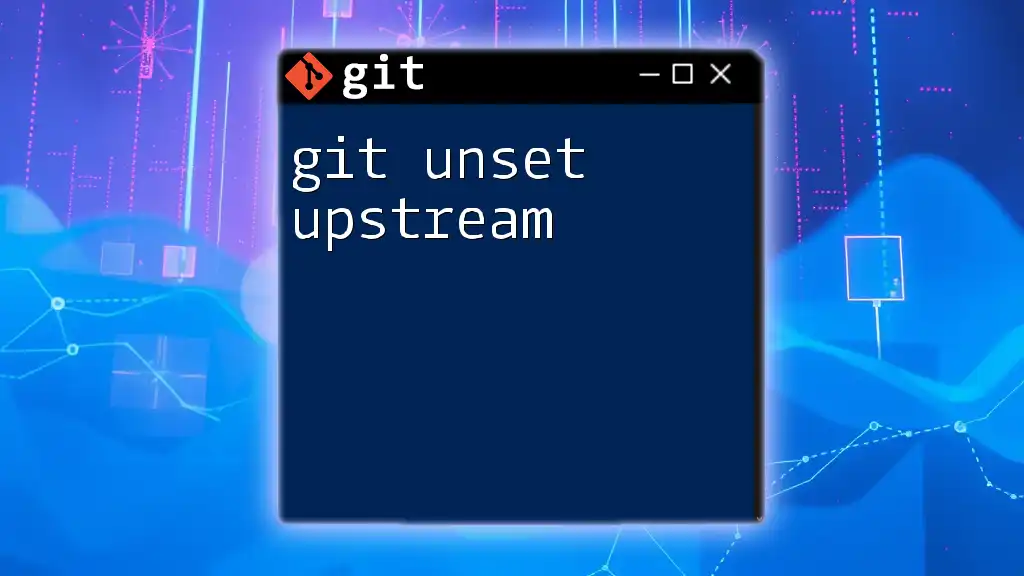
Common Issues and Troubleshooting
Conflicts When Setting Upstream
When setting an upstream branch, you might encounter conflicts, especially if the branch has diverged from its remote counterpart. Common error messages might indicate that the remote branch has changes that conflict with your local changes.
To resolve such issues, make sure to perform a fetch followed by a merge or rebase:
git fetch origin
git merge origin/<branch> # or git rebase origin/<branch>
This process will integrate the latest changes from the remote branch before you try to set it as upstream.
Deleting an Upstream Branch
If you need to remove the upstream reference for a branch, you can do so with:
git branch --unset-upstream
This command is useful if you're done with a branch or want to switch to tracking a different one. For example:
git checkout feature-login
git branch --unset-upstream
This will clear the upstream association for `feature-login`, allowing you to modify the tracking branch as needed.
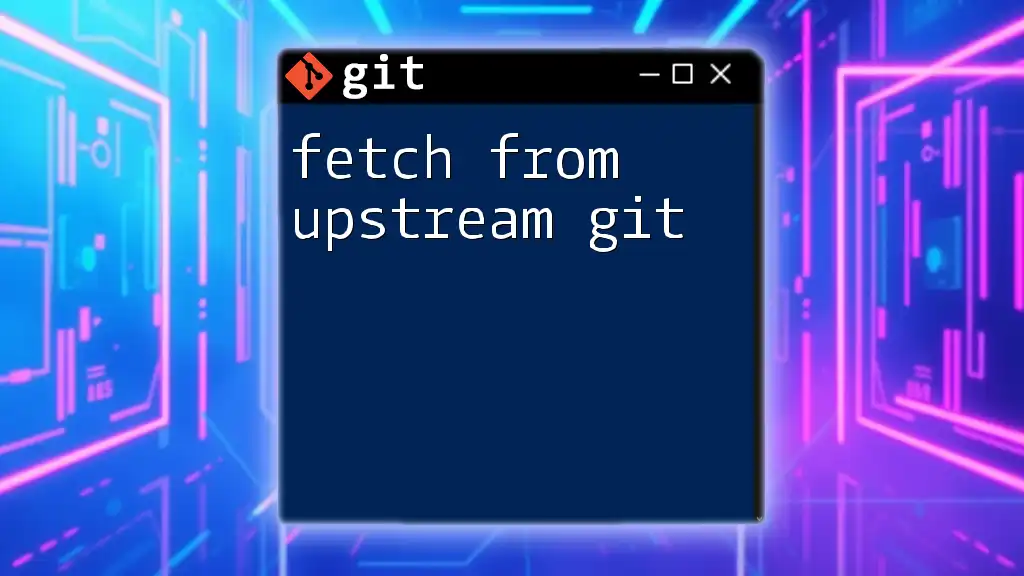
Best Practices for Using Upstream Branches
Naming Conventions
Standardizing naming conventions for your branches is essential. Choices such as using prefixes like `feature/`, `bugfix/`, or `hotfix/` help convey the purpose of each branch. This consistency makes it easier for all team members to understand the repository’s structure and purpose of each branch.
Keeping Upstream in Mind During Collaboration
Regularly pulling from upstream branches helps ensure your local work is based on the latest codebase. The command:
git pull
will automatically pull changes from your set upstream branch, allowing you to work with the latest updates smoothly. Communicating regularly with your team about branch usage helps prevent confusion and merge conflicts.
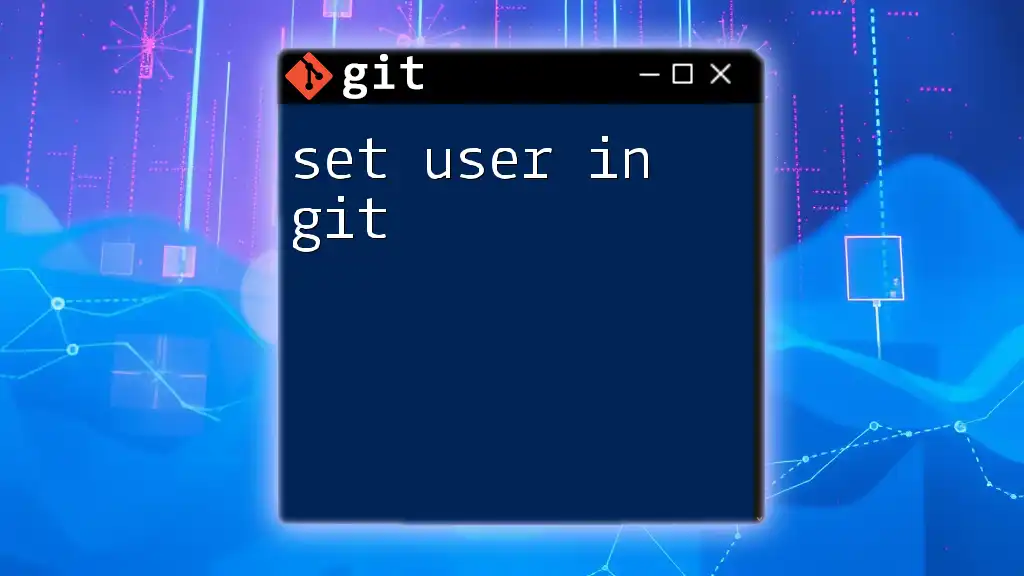
Conclusion
Setting upstream branches in Git is critical for smooth collaboration and a streamlined workflow. By understanding the concepts and commands outlined in this guide, you empower your team to effectively manage changes, resolve conflicts, and maintain synchronization with remote repositories. As you become comfortable with these practices, you’ll find that using Git becomes an integral and efficient part of your development process.
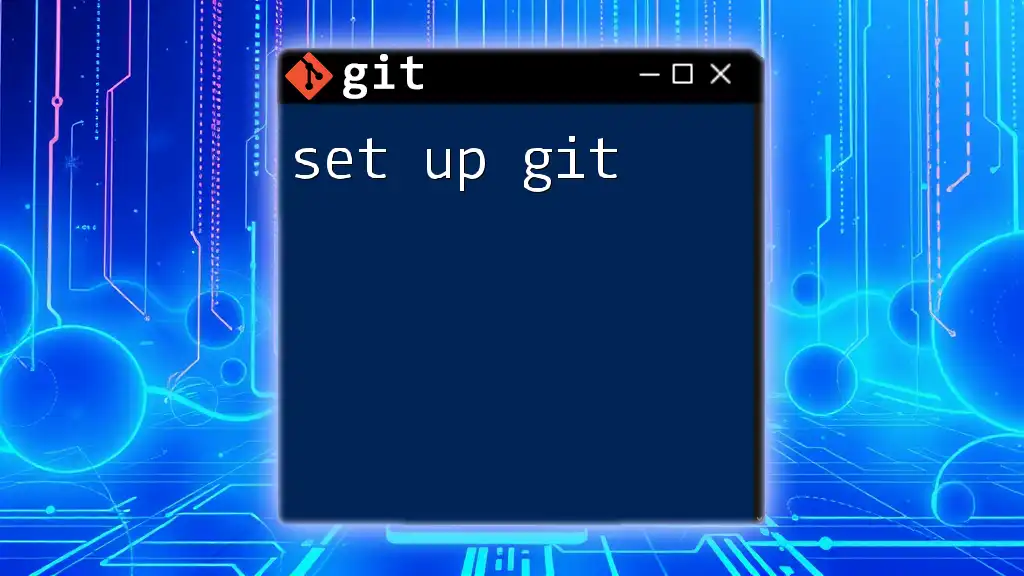
Additional Resources
For further reading, consider exploring the official Git documentation or enrolling in specialized courses on Git and version control. We encourage you to stay engaged with our content to deepen your understanding of Git commands and improve your workflow.