The `git reset --hard` command resets the current branch's HEAD to a specified commit and discards all changes in the working directory and index, effectively reverting to that point in the repository's history.
git reset --hard <commit-hash>
Understanding Git and Its Purpose
What is Git?
Git is a version control system that empowers developers to track changes in their code over time. It enables collaborative work by allowing multiple developers to work on a project simultaneously without overwriting each other’s changes. With its branching and merging features, Git has become the go-to tool for modern software development, ensuring that all contributions are recorded and can be reviewed or reverted as necessary.
Why Use Git Reset?
In Git, changes are recorded in commits. As projects evolve, developers sometimes need to undo changes or revert to a previous state. The `reset` command is central to this management process, allowing developers to manipulate the history and state of their Git repositories. Understanding the reset commands is crucial for maintaining a clean and functional project history.
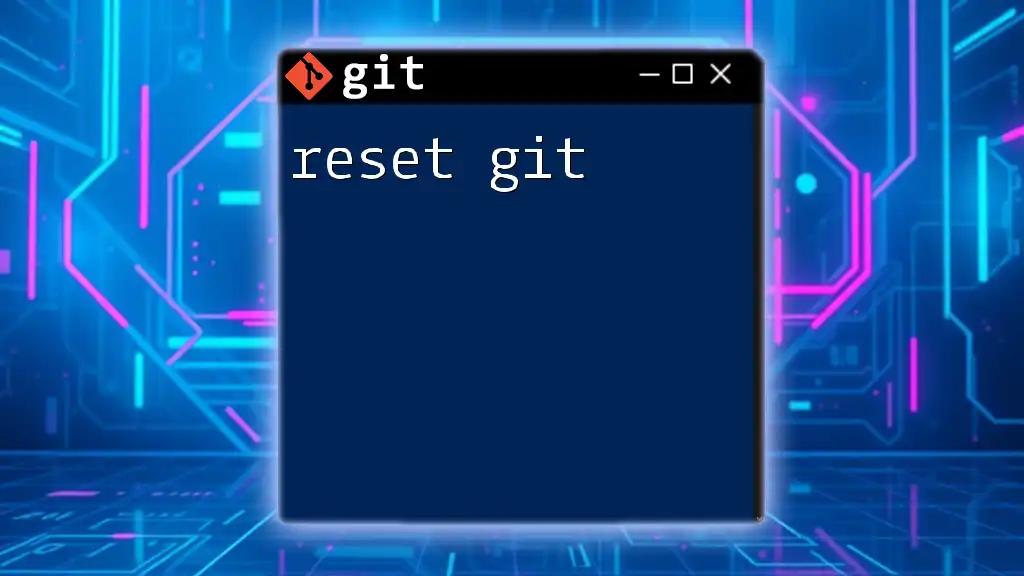
Overview of the `git reset` Command
What is `git reset`?
The `git reset` command is used to undo changes in your Git repository by moving the current branch to a specified commit. It can adjust the state of the staging area and working directory, depending on the options used. This flexibility makes it a powerful tool in the version control arsenal.
Types of Reset: Soft, Mixed, and Hard
Git provides three main types of reset:
-
Soft (`git reset --soft`): Moves the HEAD to a specified commit, but keeps changes in the staging area (index). This is ideal when you want to adjust your last commits but retain the associated changes.
-
Mixed (default, `git reset`): Moves the HEAD to a specified commit and resets the staging area, but keeps working directory files unchanged. This is useful for modifying the index while preserving local changes in your files.
-
Hard (`git reset --hard`): Resets everything – the HEAD, staging area, and working directory – to the specified commit. This option is the most destructive and should be used with caution.
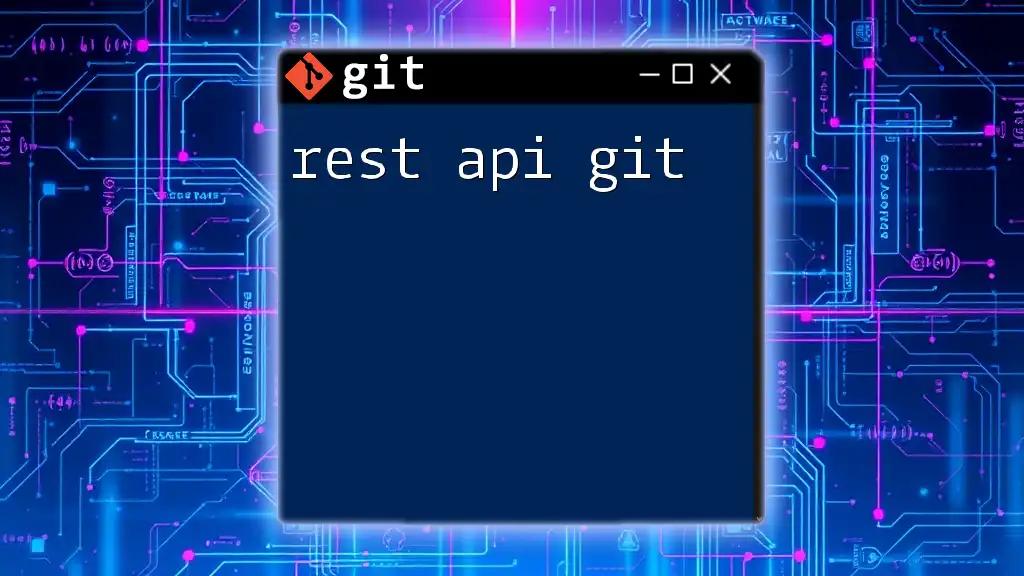
The Hard Reset Explained
What Does `git reset --hard` Do?
The command `git reset --hard` is a powerful tool that discards all changes made in the working directory and staging area, reverting everything back to the specified commit. This means any uncommitted changes will be lost. It's essential to ensure that you have backed up any important modifications before executing this command.
When to Use `git reset --hard`
A hard reset is typically used in scenarios where significant changes have been made that you want to discard entirely. For example, after experimenting with code that you ultimately decide not to keep, or when you want to return a repository to a clean, stable state.
Important Considerations
While `git reset --hard` can be beneficial, it carries inherent risks. If used carelessly, it can lead to data loss since uncommitted changes cannot be recovered after executing this command. Always assess whether a hard reset is your best choice and consider alternatives first.
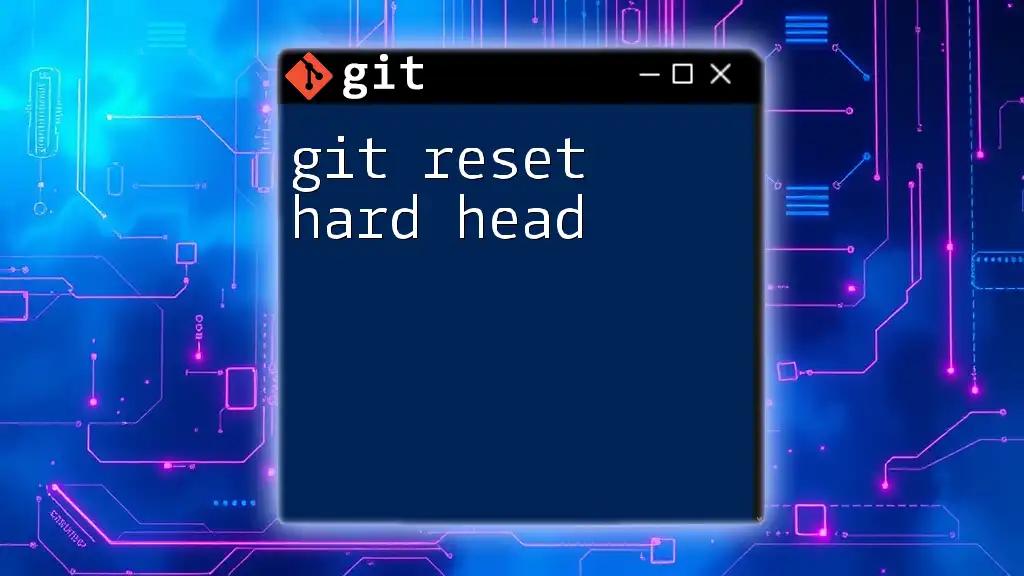
How to Use `git reset --hard`
Syntax and Basic Usage
The syntax for `git reset --hard` is as follows:
git reset --hard <commit>
Where `<commit>` represents the commit hash (or reference) to which you want to reset your repository. If you don’t specify a commit, Git uses the current `HEAD`.
Examples
Example 1: Resetting to a Specific Commit
To revert your repository to a specific commit identified by the hash `abc1234`, you would use:
git reset --hard abc1234
Executing this command will change the HEAD to the specified commit while wiping out all changes since that point, both from the index and working directory.
Example 2: Resetting to the Latest Commit
If you want to ensure that your working directory is in sync with the latest commit, the following command will do just that:
git reset --hard HEAD
This command effectively discards any changes made since the last commit, ensuring your workspace is clean and up-to-date.
Example 3: After Unwanted Changes
Let's say you've made several changes that you no longer want to keep. The command below will revert your working directory to the last committed state:
git reset --hard
Remember that this will delete all uncommitted changes, so it is crucial to use this command with care.
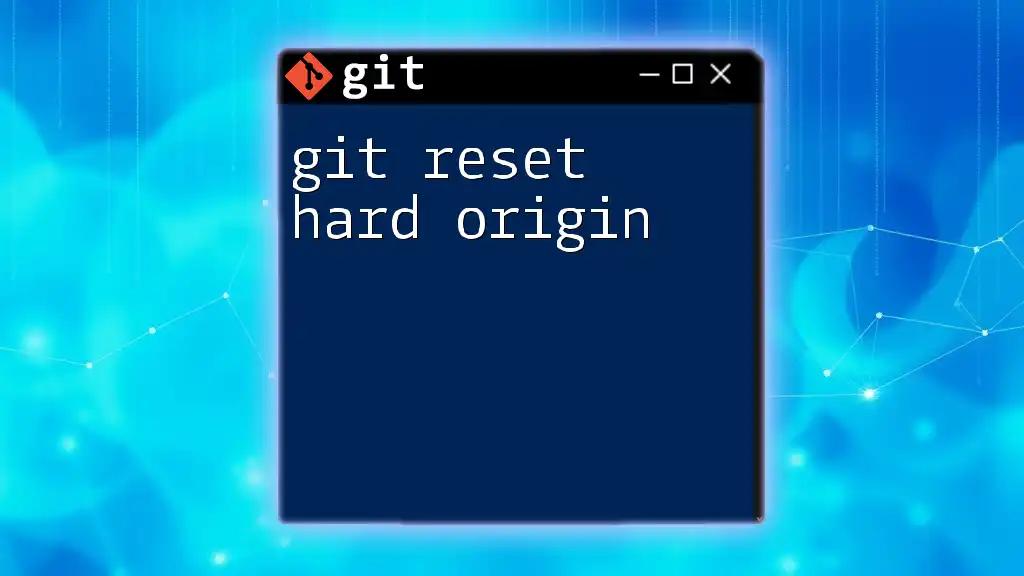
Effects of Using `git reset --hard`
Impact on Commit History
When you perform a hard reset, the commit history will reflect the change. The commits made after the specified commit will be effectively "lost" from the current branch history unless they are referenced from another branch or tag. This can significantly affect collaborative projects if other team members rely on those commits.
Impact on Working Directory and Staging Area
The working directory will mirror the state of the repository at the commit you have reset to. Any files modified or added since that commit will be erased. The index or staging area will also reflect this reset, as nothing will remain in that area.
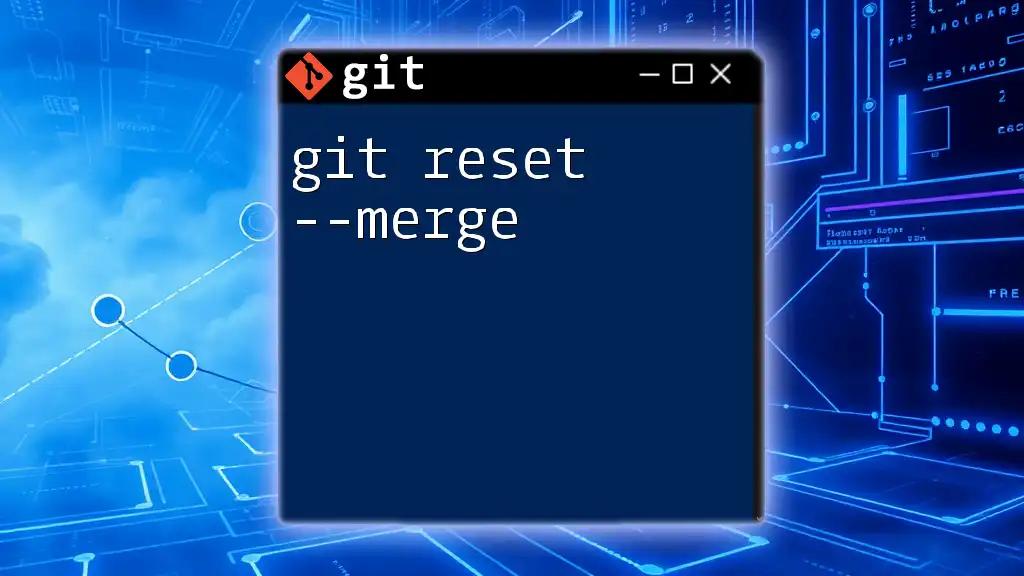
Recovery Options
Handling Uncommitted Changes
To protect against losing valuable uncommitted changes before executing a hard reset, use `git stash`. This command temporarily shelves changes so they can be reapplied later. For example:
git stash
git reset --hard HEAD
git stash pop
In this sequence, you save your changes, reset your working directory, then restore them afterward.
Exploring Alternative Commands
Using `git revert`
If you wish to undo changes without erasing history, consider using `git revert`. This command creates a new commit that undoes changes made by previous commits, allowing you to maintain project integrity and log.
Using `git checkout` for File Reversion
For selectively reverting changes in specific files rather than the entire commit history, `git checkout` can be employed. For instance, to revert a specific file to the last committed state, you would use:
git checkout -- filename
This option provides a more granular approach than a hard reset.
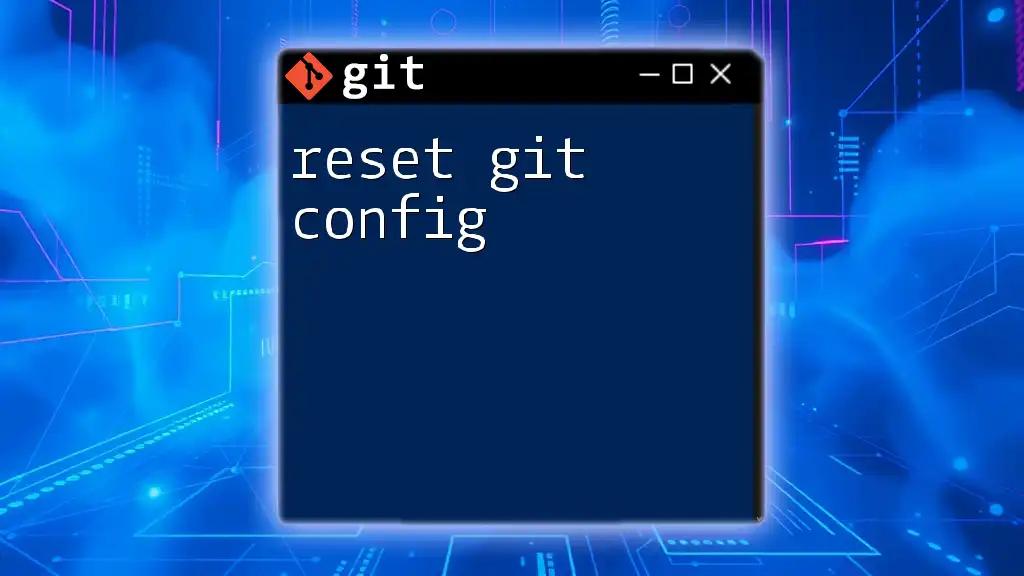
Common Scenarios and Use Cases
Collaboration Scenarios in Teams
In team environments, a hard reset might be necessary when an unstable feature branch contains experimental code that everyone agrees to discard. However, communication is crucial; everyone must be aware of the reset to avoid confusion or loss of important changes.
Solo Developer Workflows
For individual developers, hard resets can help maintain a cleaner workflow, especially during debugging or when exploring new ideas that ultimately don't lead to valuable changes.
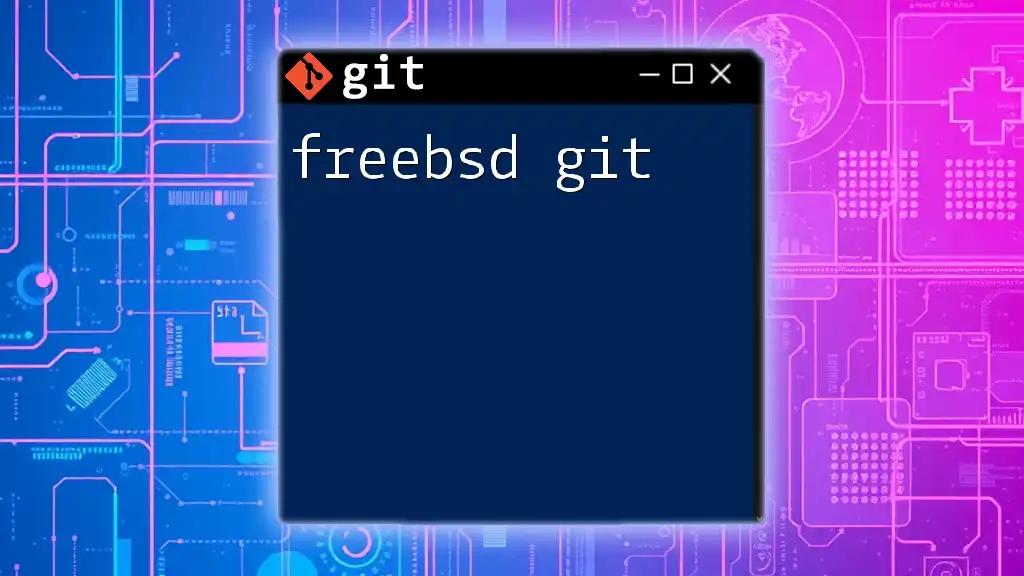
Conclusion
Recap of `git reset --hard`
The `git reset --hard` command is a powerful lever in your Git toolkit that allows you to discard changes completely, but it must be used with caution. Understanding its effects on your commit history, working directory, and staging area will prepare you to wield it effectively.
Final Thoughts and Recommendations
Mastering Git commands, especially ones as impactful as `reset --hard`, is crucial for any developer. Utilize it wisely, and always consider backing up changes you might need in the future. With practice, you'll be able to use Git confidently and efficiently, safeguarding your projects and collaborating effectively.