To fetch updates from the upstream repository in Git, use the following command to download the latest changes without merging them into your local branch:
git fetch upstream
Understanding Upstream Repositories
What is an Upstream Repository?
An upstream repository is essentially the main source of a project that your local copy interacts with, often referred to as the original repository from which you forked or cloned. This is crucial in collaborative environments where multiple contributors are working on the same codebase, allowing them to stay updated with changes made by others.
To clarify, an upstream repository typically refers to the project or repository that you want to pull updates from. In contrast, your local repository represents your own working copy, while a downstream repository refers to those repositories that are forked from your own.
How to Define Upstream in Your Repository
Setting an upstream repository allows your local Git configuration to know from where to fetch updates. You can set an upstream repository using the following command:
git remote add upstream <repository-url>
Replace `<repository-url>` with the actual URL of the repository. This command configures your local repository to recognize the specified upstream repository.
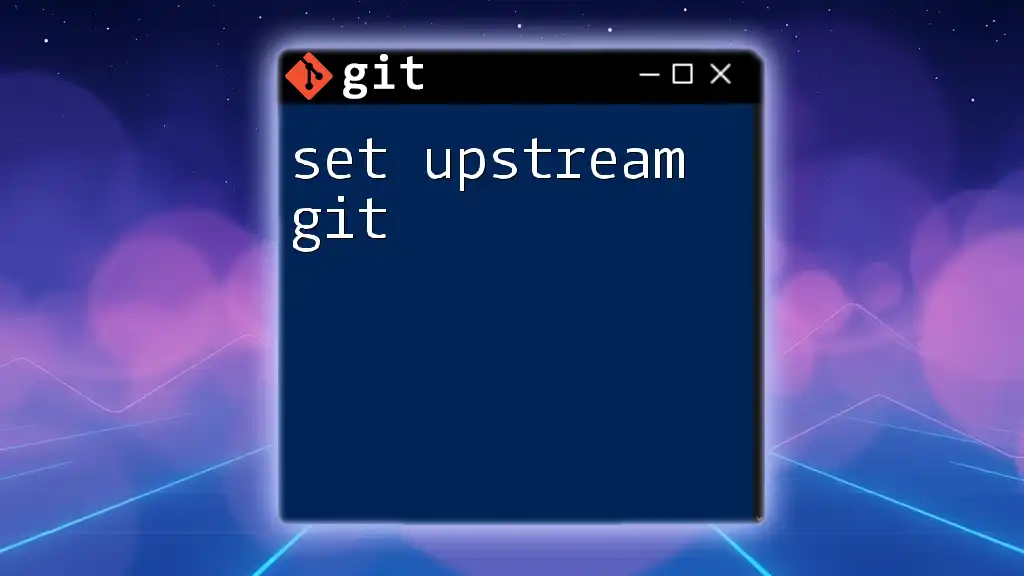
The Basics of Git Fetch
What Does `git fetch` Do?
The `git fetch` command is a fundamental part of Git's workflow. This command retrieves updates from the upstream repository without altering your working directory. It downloads new commits, files, and references from the upstream repository, storing them in your local repository.
Unlike `git pull`, which fetches the changes and immediately merges them into your working branch, `git fetch` allows you to review the fetched updates before integrating them.
Syntax of the `git fetch` Command
The basic syntax for fetching from an upstream repository is simple:
git fetch <remote-name>
For example, if your upstream repository is named `upstream`, you would use:
git fetch upstream
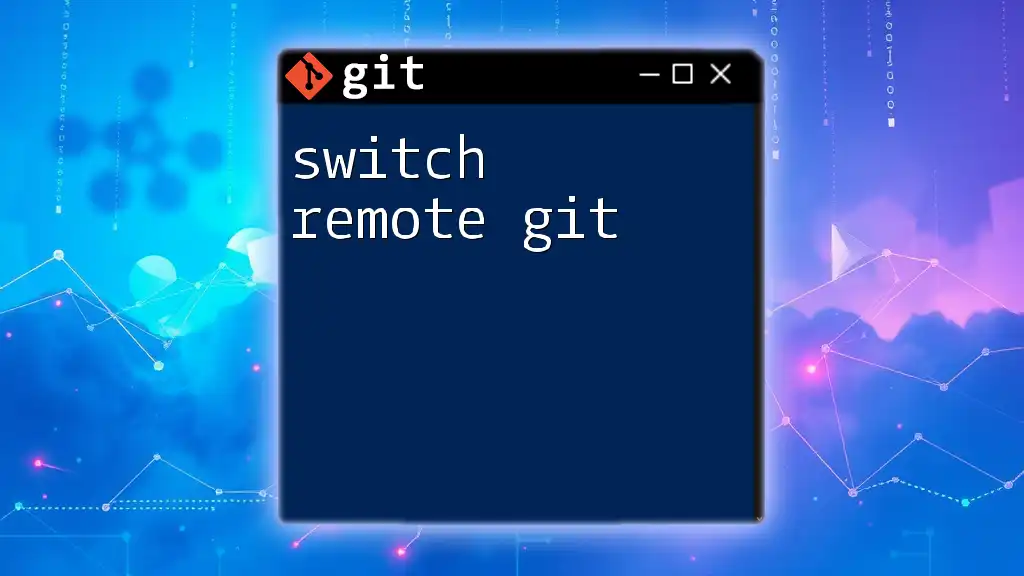
How to Fetch from an Upstream Repository
Step-by-Step Guide to Fetching
Step 1: Verify Your Current Remotes
Before performing a fetch, it's good practice to check what remotes you currently have set up. To do this, use the following command:
git remote -v
This command lists all remotes associated with your local repository, displaying the names and the URLs of each remote. Make sure that your upstream remote exists in this list.
Step 2: Fetch Changes from the Upstream
To fetch updates from your upstream repository, execute:
git fetch upstream
Upon execution, you will not see visible changes in your files just yet; instead, Git retrieves the new commits and stores them for you to review.
Step 3: Review Fetched Changes
After fetching, it's important to examine what changes were made in the upstream repository. You can check the log of the upstream branch with:
git log upstream/main --oneline
This command displays a concise list of commits from the upstream `main` branch, allowing you to see what updates are available for merging.
Handling Merged Changes After Fetching
Once you've reviewed the changes, you have two primary options for integrating those changes into your local branch: merging or rebasing.
-
Merge:
If you choose to merge, use the following command:
git merge upstream/main
This merges the fetched changes into your current branch, effectively combining the codebases.
-
Rebase:
If you prefer rebasing (which can keep a cleaner project history), use:
git rebase upstream/main
Rebasing applies your local changes on top of the fetched commits, making it as if your work started from the latest state of the upstream branch.
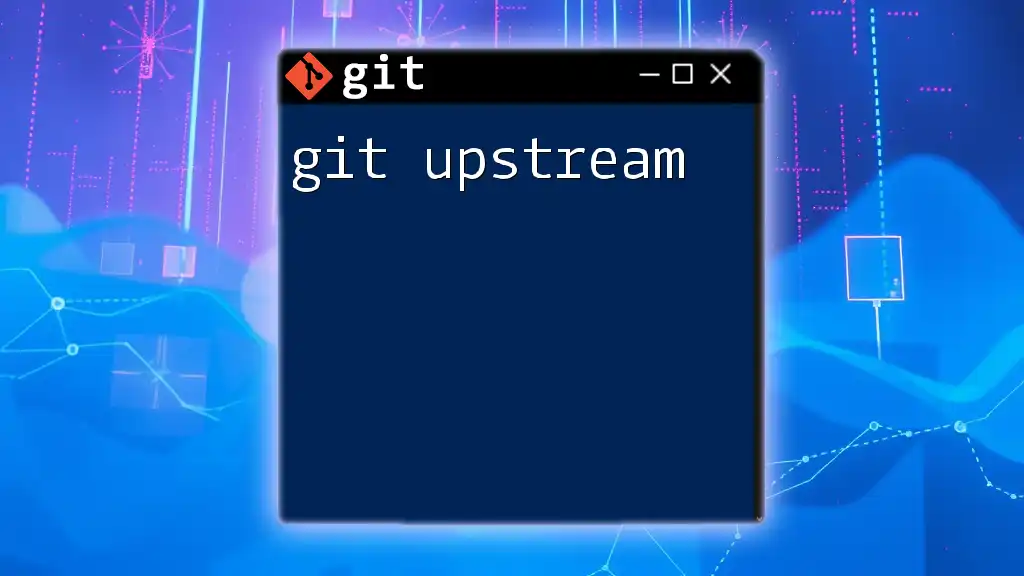
Best Practices for Using Git Fetch
When to Use `git fetch`?
It's advisable to use `git fetch` frequently—especially in collaborative environments. Regularly fetching ensures that you remain updated with the latest developments contributed by your team members. This practice minimizes the risk of conflicts when you eventually merge or pull changes into your local branch.
Common Pitfalls to Avoid
While using `git fetch`, it's crucial to avoid errors that might arise from merging incorrect branches or failing to resolve conflicts. Some common mistakes include:
- Forgetting to fetch before trying to merge or pull changes, leading to outdated local branches.
- Not reviewing fetched changes before merging, resulting in potential merge conflicts.
- Failing to set the upstream correctly, which can cause confusion about your intended source of updates.
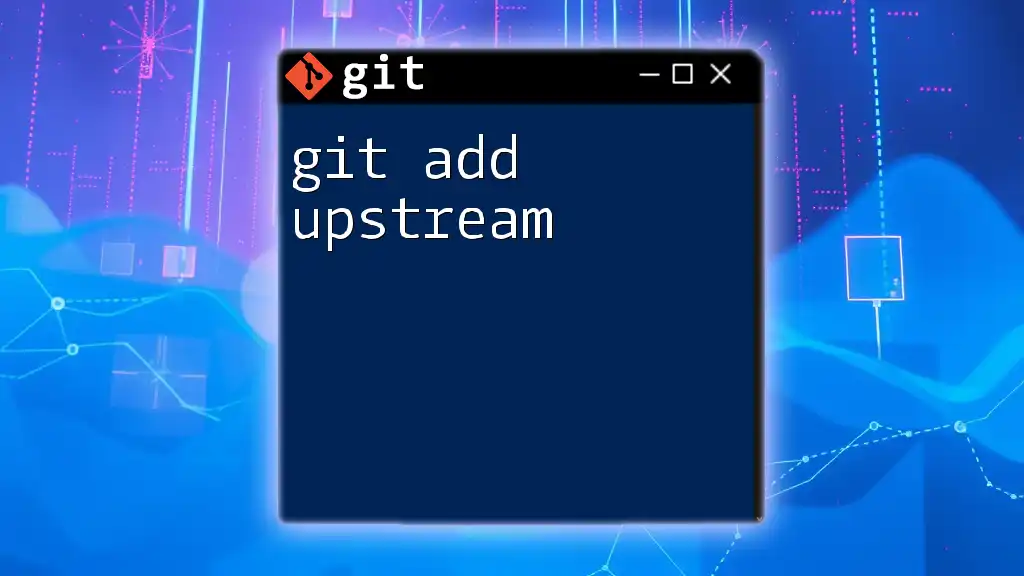
Conclusion
Using `git fetch` from upstream repositories is a vital practice that helps developers maintain an effective workflow in collaborative projects. Regular fetching keeps your local environment up-to-date and prepares you for a smooth integration of changes.
By understanding how to set an upstream repository, fetch updates, and handle those changes appropriately, you can improve your version control process and minimize issues in your development cycle.
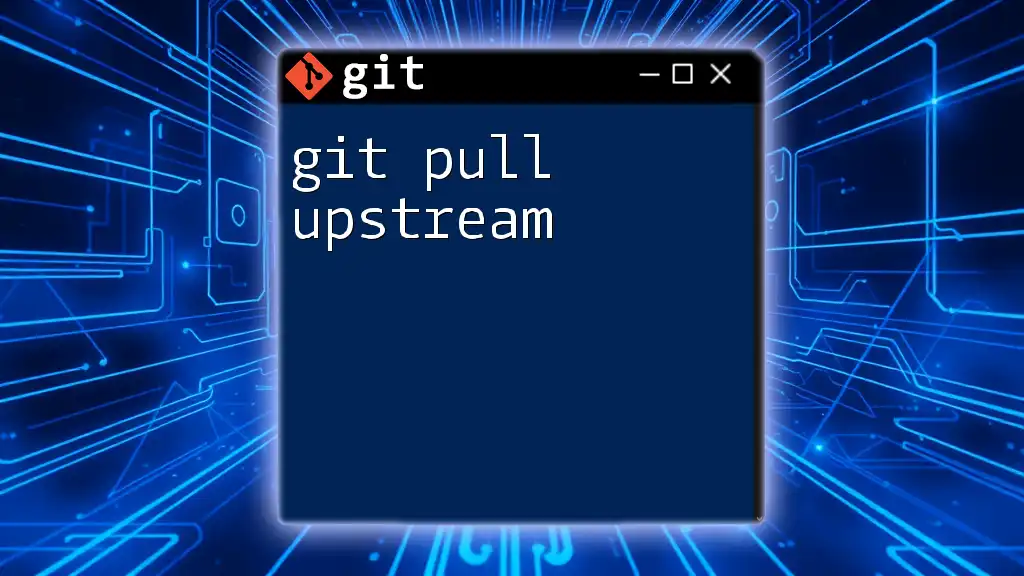
Additional Resources
For further learning, consult the official Git documentation or consider using tools and GUI clients that can assist in visualizing and managing your Git repositories more easily.
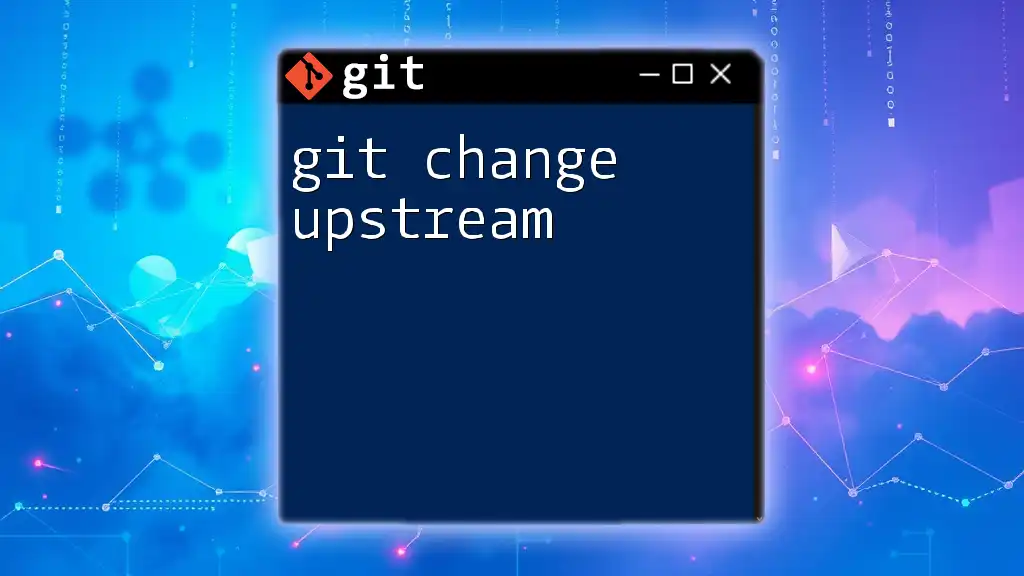
FAQs
What is the difference between `git fetch` and `git pull`?
The key difference lies in their functions. `git fetch` downloads changes from the upstream repository without altering your local working state, while `git pull` performs a fetch followed by an automatic merge of changes into your current branch.
Can I fetch changes from multiple upstream repositories?
Yes, you can manage multiple upstream repositories by adding them as different remotes, such as `upstream`, `origin`, etc. Be sure to specify the correct remote when performing a fetch.
Do I need permissions to fetch from an upstream repository?
Permissions depend on the repository's configuration. If the upstream repository is public, you can fetch without special permissions. However, private repositories may require appropriate access rights for you to fetch updates.