The command `git pull upstream` is used to fetch and integrate changes from the upstream repository into your current branch.
git pull upstream main
What is `git pull`?
The `git pull` command is a critical tool in Git version control systems, designed to update your local repository with changes from a remote repository. It fundamentally serves two purposes: fetching the data from the remote repository and merging that data into your current branch. Understanding this command is essential for maintaining synchronization within team projects, especially when multiple collaborators are involved.
It’s crucial to differentiate between `git pull`, `git fetch`, and `git merge`. While `git fetch` retrieves updates from a remote without merging them into your working directory, `git pull` performs both actions in one step. It's a convenient shortcut, but it can also lead to complications if changes conflict with your local version.
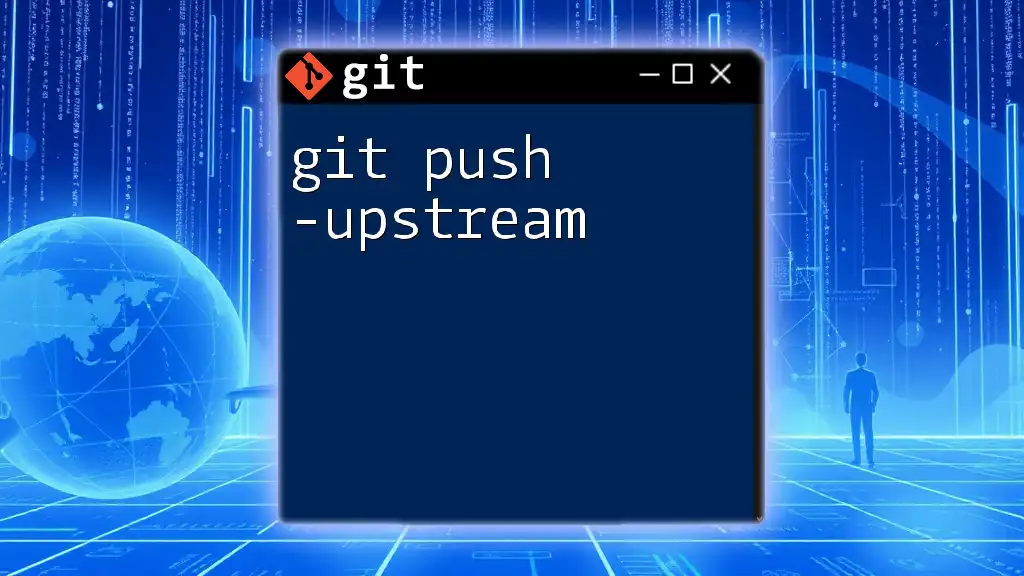
Understanding Upstream Repositories
In Git, an "upstream" repository refers to the original version of a project from which your local version (or fork) derives. This is especially relevant in collaborative environments. When a forked repository is created, it is connected to the upstream repository, allowing developers to pull the latest changes and stay updated.
Understanding the role of upstream is vital because it helps maintain the integrity of the codebase and ensures that all contributors are on the same page when it comes to project evolution. The term "origin" usually refers to the remote repository from which your local repository was cloned, which may differ from the upstream repository, especially in open-source projects.
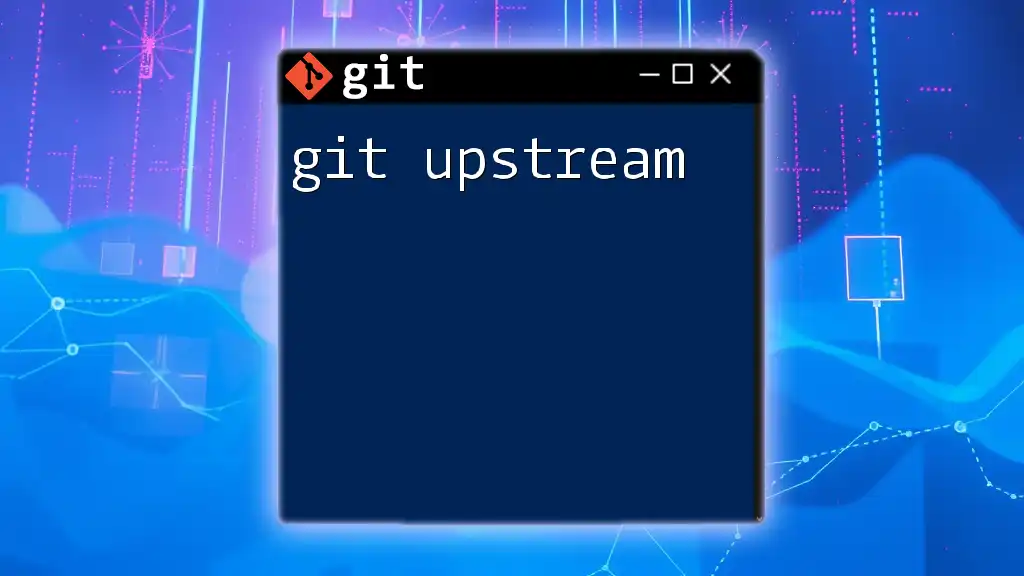
Setting Upstream Remote
What is a Remote?
In Git, a "remote" is a version of your repository that is hosted on the internet or another network. Remotes facilitate collaboration by allowing team members to access shared codebases easily. Understanding how to manage remotes is crucial for successful team dynamics, especially in distributed workflows.
Adding an Upstream Remote
To interact with an upstream repository, you need to add it as a remote to your local Git configuration. Here’s how to add an upstream remote, which typically happens in forking situations:
To add an upstream repository, run the following command in your terminal, replacing the URL with the actual URL of the original repository:
git remote add upstream https://github.com/OriginalOwner/OriginalRepo.git
This command connects your local repository to the upstream repo, allowing you to pull the latest changes when necessary. By specifying the remote name as upstream, you clearly distinguish between your origin and the source repository, facilitating workflow.
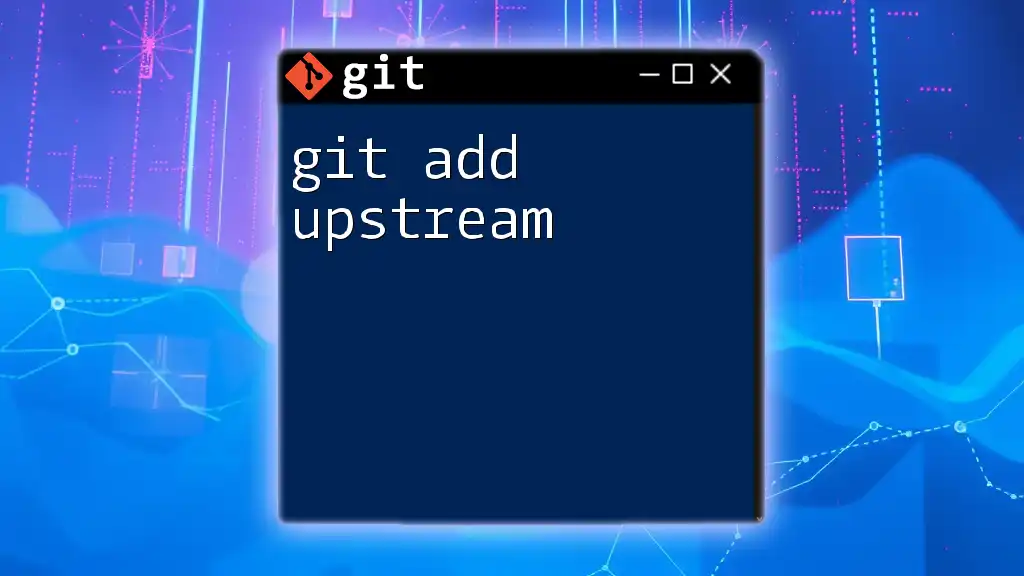
Using `git pull upstream`
The Command Breakdown
The `git pull upstream` command is employed to integrate changes from the upstream repository into your current branch. The typical syntax of this command is:
git pull [options] [remote] [branch]
When you execute `git pull upstream`, Git looks for the specified branch on the upstream repository and merges its content into your current branch.
Common Use Cases
Using `git pull upstream` is essential in various scenarios, particularly:
- Keeping a Fork Up to Date: If you've forked a repository, regularly pulling from the upstream ensures that you are working with the latest code modifications.
- Collaborating on Feature Branches: While working on a feature branch, pulling changes helps avoid integration conflicts and keeps you updated on the main line of development.
Example Workflow
Let’s walk through a typical workflow for pulling changes from an upstream repository.
-
Navigate to your Project Directory: First, make sure you’re in your local repository directory:
cd my-forked-repo
-
Fetch Changes from Upstream: Before pulling changes, you can optionally fetch updates:
git fetch upstream
This command retrieves the latest changes without merging, allowing you to inspect what’s new.
-
Merge Changes with Your Local Branch: To incorporate changes from the upstream branch (e.g., `main`), run:
git pull upstream main
Here, you pull the updates from the main branch of the upstream remote directly into your active branch.
Resolving Conflicts
When merging changes, conflicts may arise if the same lines were modified in both branches. Understanding how to resolve these conflicts is crucial. Here are the steps to handle a merge conflict after using `git pull upstream`:
- Git will indicate conflicted files. Inspect these files to understand the nature of the conflicts.
- Open the file and look for conflict markers (`<<<<<<`, `======`, and `>>>>>>`). Manually edit the file to resolve the conflicts.
- Once resolved, add the modified file to the staging area:
git add conflicted-file.txt
- Finally, commit the merge:
git commit -m "Resolved conflicts after pulling upstream"
By diligently resolving conflicts, you maintain project coherence and contribute effectively to collaborative activities.
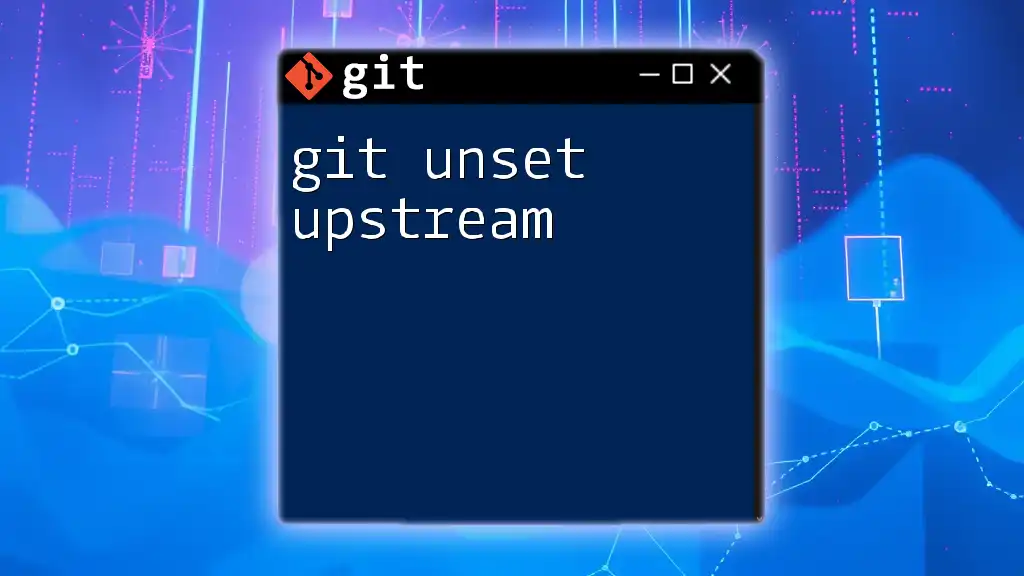
Best Practices for Using `git pull upstream`
To maximize your efficiency and minimize issues while using `git pull upstream`, consider the following best practices:
- Regularly Pull Changes: This minimizes the possibility of conflicts by keeping your work synced with the upstream repository. Avoid waiting long periods before integrating changes.
- Use Descriptive Commit Messages: When merging changes, clear commit messages enhance collaboration and make it easier for other developers to understand the history of modifications.
- Communicate with Team Members: Regular communication can prevent overlaps in work and clarify which branches or features each collaborator is focusing on.
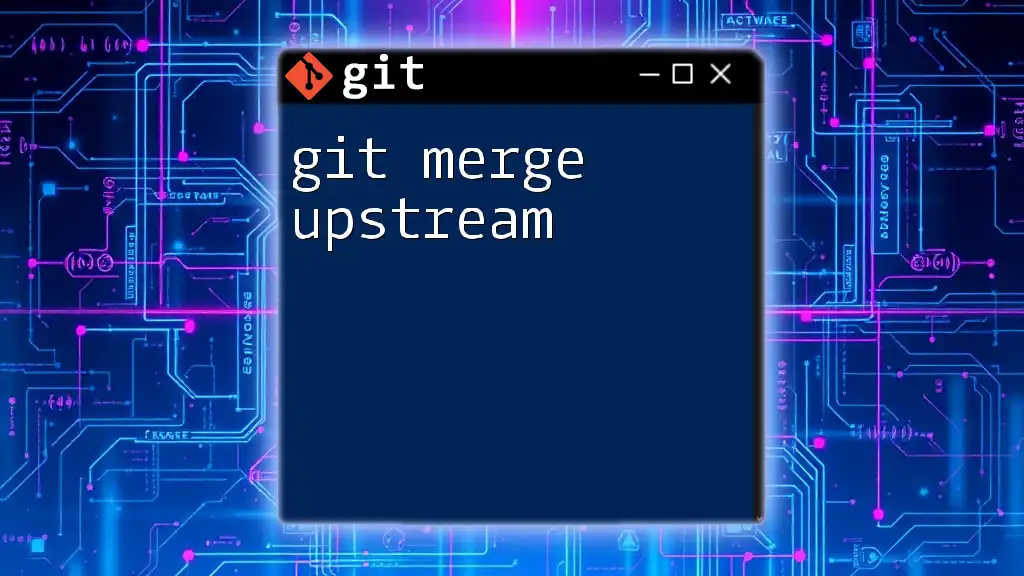
Troubleshooting Common Issues
While working with `git pull upstream`, you may encounter several challenges. Being prepared to troubleshoot these common issues is essential:
- Failed Merges: If you see a message indicating a merge failure, carefully inspect the conflicts and follow the resolution process.
- Mistaken Commits: You might accidentally merge unwanted changes. In this case, use:
This will cancel the ongoing merge and allow you to reevaluate your next steps.git merge --abort
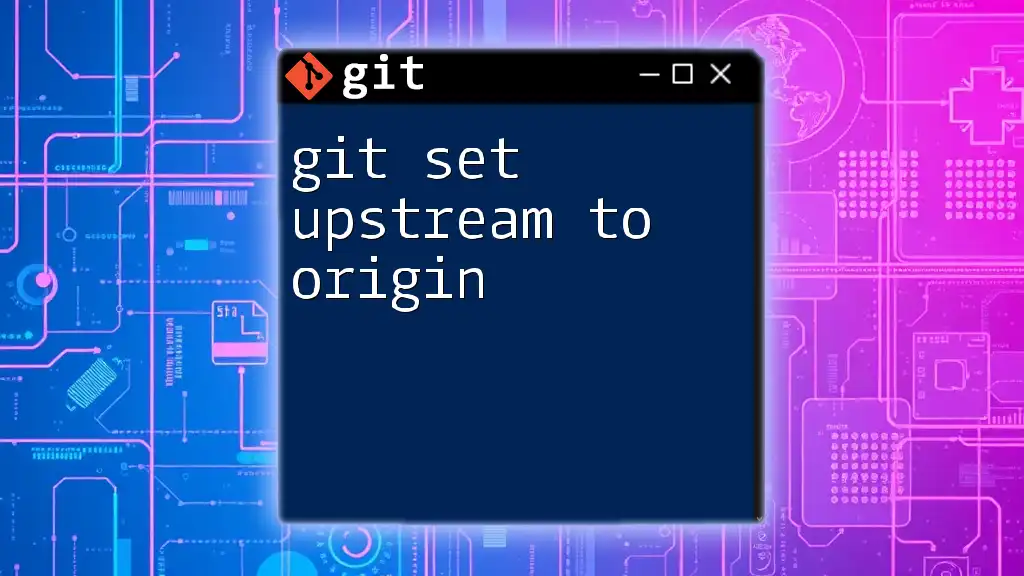
Conclusion
Mastering the `git pull upstream` command is paramount for effective collaboration in software development. It ensures that you stay updated with the latest changes from the original project, facilitates smoother integration, and minimizes the chances of conflicting changes. Practice using this command to become proficient and confident in managing your repository alongside your collaborators.
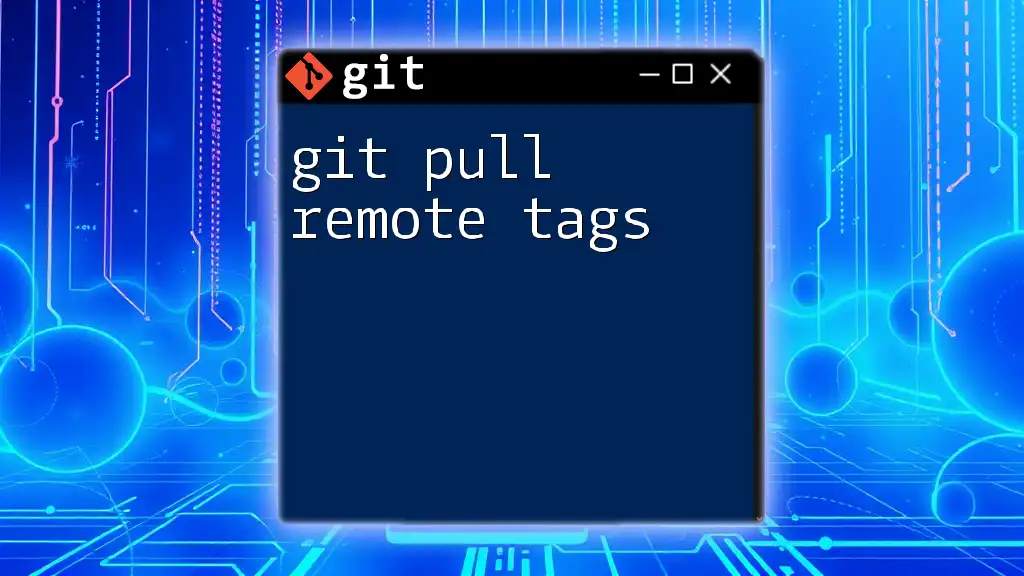
Additional Resources
To further deepen your understanding and skills in Git, consider exploring the following resources:
- Git Documentation: [Git - Documentation](https://git-scm.com/doc)
- Online Courses: Platforms like Udemy, Coursera, or freeCodeCamp offer comprehensive Git tutorials.
- Community Forums: Engage with fellow Git users on sites like Stack Overflow or GitHub Discussions to seek help and share experiences.
By continuously learning and practicing, you’ll ensure a robust grasp of Git operations, tailored to modern collaborative software development.