To fetch all the remote tags from a Git repository, you can use the following command:
git fetch --tags
Understanding Git Tags
What are Git Tags?
Git tags are a powerful feature in Git that allow you to mark specific points in your repository's history as important. This is often utilized for marking release versions (e.g., v1.0, v2.1). There are two types of tags: lightweight tags, which are essentially pointers to specific commits, and annotated tags, which include metadata such as the tagger's name, email, date, and a message, much like a commit.
Why Use Tags in Git?
Tags provide a clear way to manage versions of your code. Unlike branches, which indicate ongoing development, tags denote a specific snapshot of your repository. This simplicity makes tags invaluable for:
- Versioning and releases: Clearly marking stable points in your project timeline.
- Easier access to specific commits: Quickly tagging significant achievements or changes for easy retrieval.
- Collaboration and deployment: Facilitating more manageable collaboration among team members and smoother deployments.
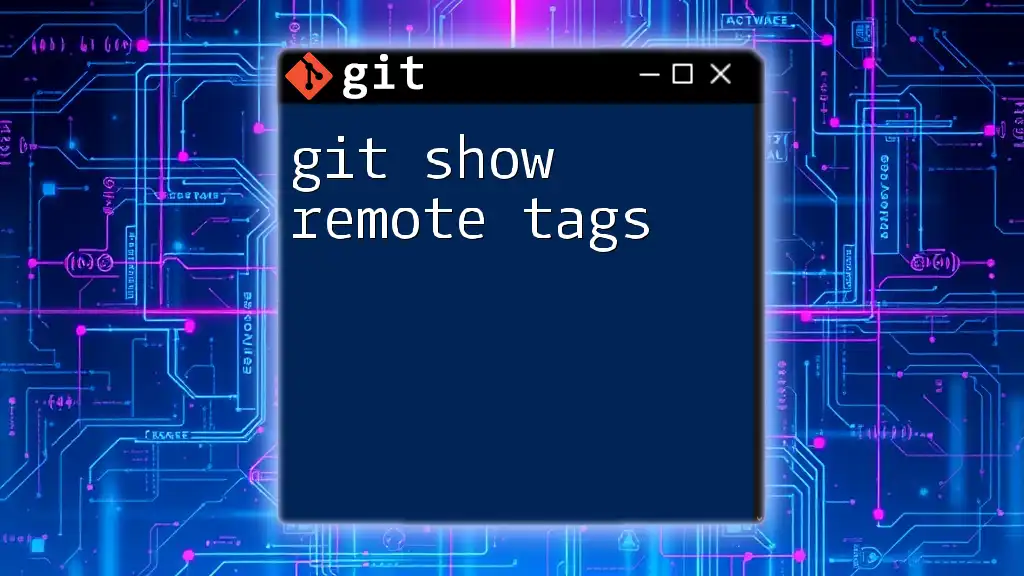
Setting Up Your Environment
Installing Git
To take advantage of `git pull remote tags`, you first need to have Git installed on your machine. Here’s how you can install Git across various operating systems:
- Windows: Download the installer from the official Git website and follow the setup instructions.
- macOS: Use Homebrew by running the command:
brew install git
- Linux: Use your distro's package manager, such as:
sudo apt-get install git
After installation, verify that Git is installed properly by typing:
git --version
Configuring Git
Before diving into commands, configure Git by setting your user name and email. You can do this globally:
git config --global user.name "Your Name"
git config --global user.email "your.email@example.com"
Additionally, setting up SSH keys will ensure secure communication with remote repositories. Generate an SSH key using:
ssh-keygen -t rsa -b 4096 -C "your.email@example.com"
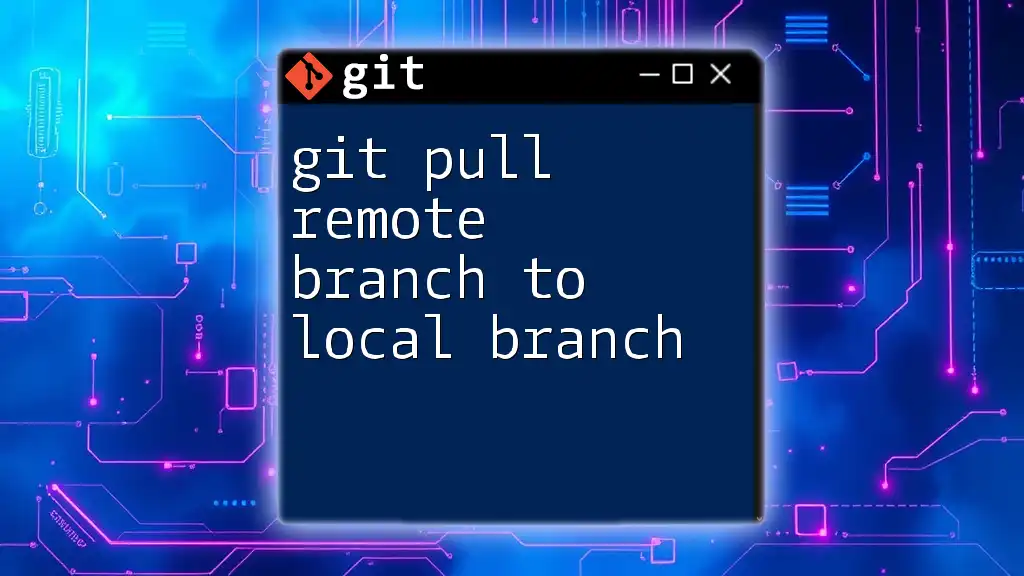
Pulling Remote Tags
Understanding the Command
When working with remote repositories, you might wonder about the difference between `git fetch` and `git pull`. While `git pull` updates your local branch with changes from the remote, `git fetch` merely downloads updates from the remote without merging them. To specifically fetch tags, you would use:
git fetch --tags
Basic Command Syntax
The command `git fetch --tags` downloads all the tags from the remote repository into your local repository. This does not merge them but ensures you have access to them.
Detailed Steps to Pull Remote Tags
Step 1: Navigate to Your Local Repository
Before pulling tags, ensure you navigate to the directory of your local repository. Use the command:
cd path/to/your/local/repo
Step 2: Pulling Tags
Once you are in the correct directory, execute the command to pull the tags:
git fetch --tags
This command communicates with the remote repository and downloads all tags, helping you stay current with important markers in your project's life cycle.
Verifying Pulled Tags
Listing Available Tags
After pulling tags, you can view them with the command:
git tag
The terminal will list all available tags for easy reference.
Checking Out a Tag
To work with a specific tag, you can switch your working directory to this tagged state using:
git checkout <tag-name>
This command will update your repository to reflect the state at that specific tag, allowing you to explore or even build on that point in time.
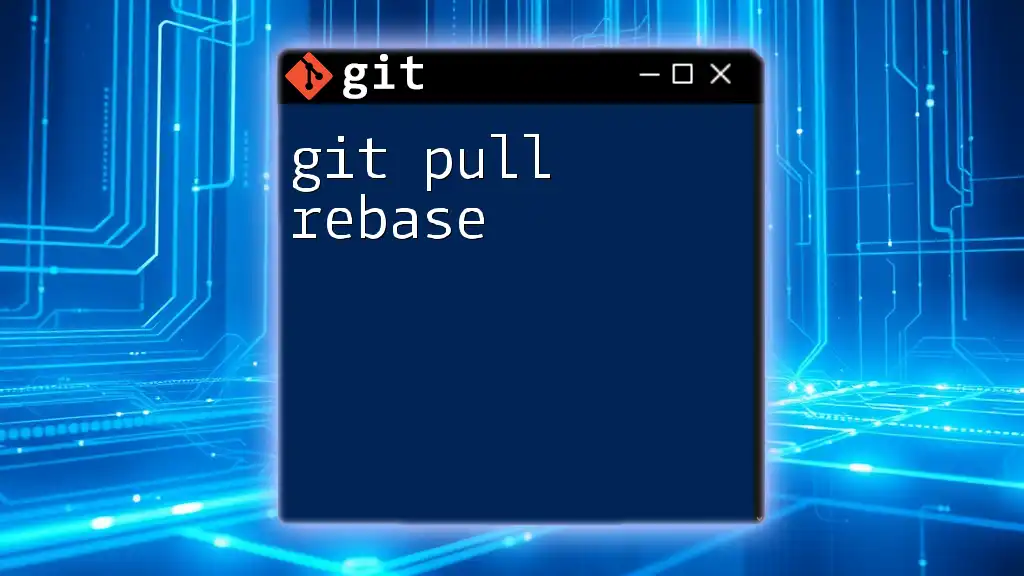
Troubleshooting Common Issues
Unable to Fetch Tags
If you run into issues while fetching tags, error messages could indicate problems like network connectivity or incorrect remote URLs. Ensure your network is stable and that your remote URL is correctly set using:
git remote -v
Understanding Detached HEAD state
When you check out a tag, you might enter a detached HEAD state. This means you’re no longer working on a branch, and any new commits made will not belong to a branch unless explicitly created. To prevent losing changes, create a new branch from the tag like this:
git checkout -b new-branch-name
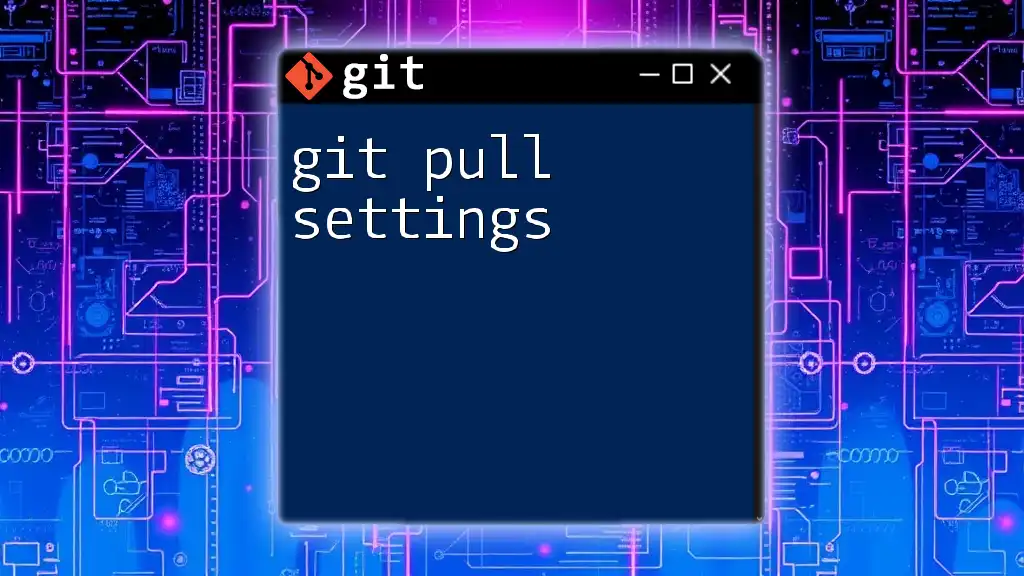
Conclusion
Pulling remote tags is an essential aspect of maintaining an organized workflow in Git. By grasping the concept of tags, knowing how to pull them, and understanding the underlying commands, you equip yourself with vital skills for effortless version control. Tag management keeps your project organized and accessible, allowing you to focus on what matters most—development.
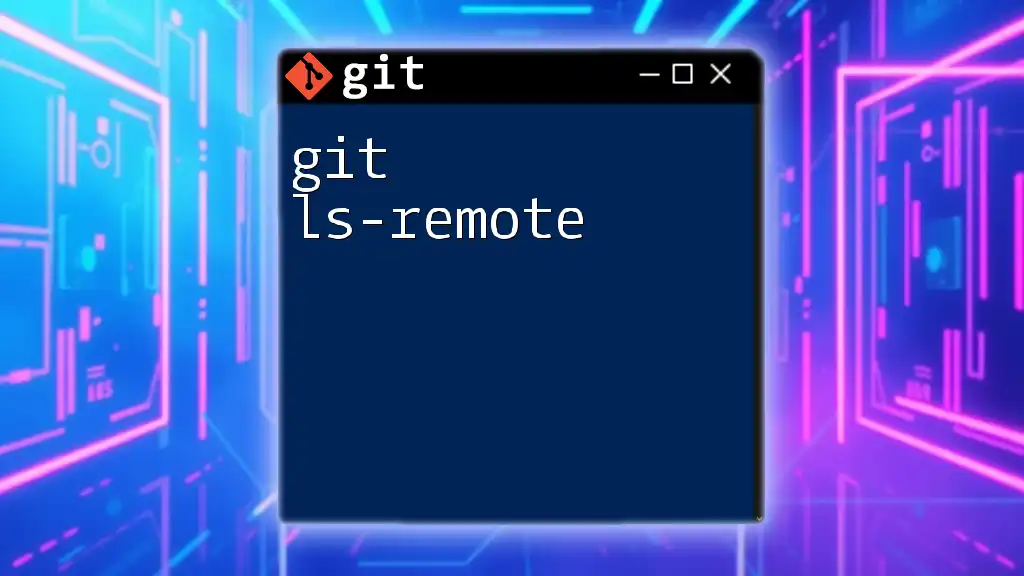
Additional Resources
Useful Git Commands
Become familiar with other Git commands that enhance your workflow, such as `git commit`, `git branch`, and `git merge`.
References
For more in-depth learning, refer to the official [Git documentation](https://git-scm.com/doc) and explore various tutorials and books available online.
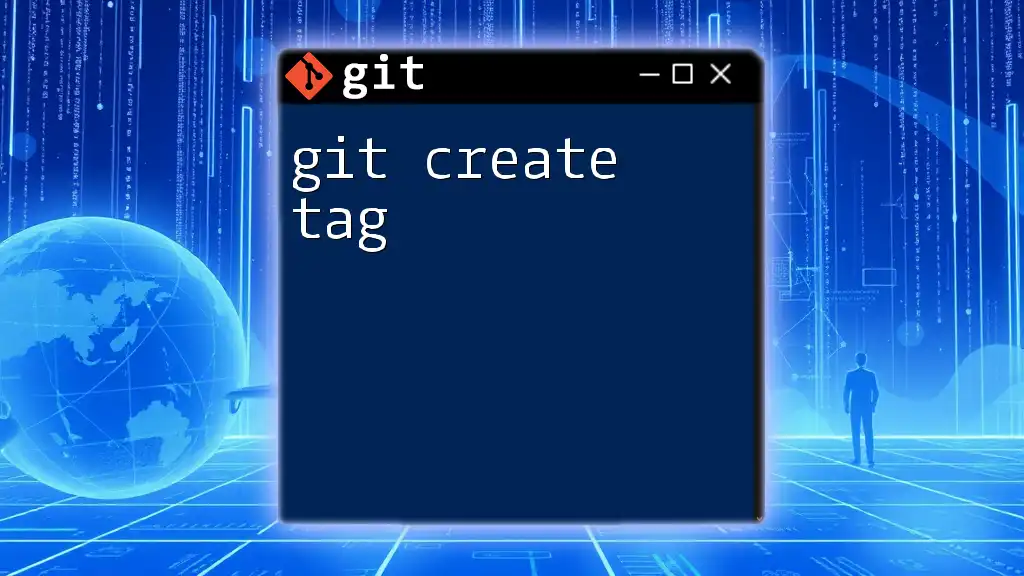
Call to Action
If you wish to master Git and become a proficient user, don’t hesitate to sign up for our Git training course. Share this article with your peers or on social media to spread the knowledge of Git best practices!