The command `git pull --rebase` updates your current branch with changes from the remote repository while preserving your local commits, and if you want to delete all local changes before doing so, you can use `git reset --hard` beforehand.
Here's how you can combine these commands:
git reset --hard && git pull --rebase
Understanding Git Pull
What is `git pull`?
`git pull` is a command that allows you to fetch changes from a remote repository and merge them into your current branch. It is a simple way to synchronize your local repository with a remote one, ensuring you have the latest updates from other collaborators. It's essential to note that `git pull` is essentially a combination of two commands: `git fetch` (which downloads the changes) and `git merge` (which integrates them).
Syntax of `git pull`
The general syntax for `git pull` is:
git pull <remote> <branch>
Where `<remote>` is typically `origin`, the default name for your remote repository, and `<branch>` is the branch you wish to pull changes from.
When to Use `git pull`
You should use `git pull` whenever you want to incorporate changes made by others in a collaborative environment. However, caution is advised. If multiple developers are working on the same branch, you might encounter merge conflicts. To reduce the chances of conflicts, it’s often advisable to commit your local changes before pulling. Always ensure that your working directory is clean (no uncommitted changes) before executing this command to avoid unexpected results.
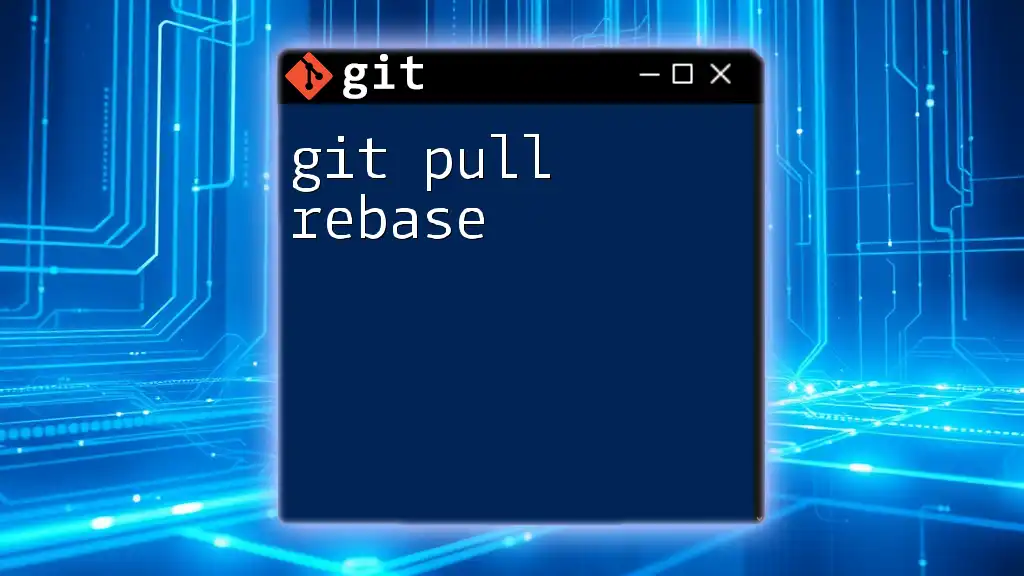
The Rebase Concept
What is Rebasing?
Rebasing is a powerful feature in Git that allows you to integrate changes from one branch into another. Instead of creating a merge commit (which can clutter your history), rebasing rewrites commit history linearly, making it easier to follow. When you rebase, you are effectively taking all the changes from one branch and reapplying them onto another.
Syntax of Rebase
The general syntax for executing a rebase is as follows:
git rebase <branch>
In this case, `<branch>` is the branch that you want to rebase onto your current branch.
Using Git Pull with Rebase
To pull changes while rebasing, you can combine the `git pull` command with the `--rebase` flag. The syntax looks as follows:
git pull --rebase <remote> <branch>
This command will first fetch the changes from the specified remote branch, and then reapply your local commits on top of those changes. This keeps your commit history cleaner and much easier to read.
Pros and Cons of Rebasing
Benefits of using rebase include:
- A cleaner project history.
- Easier navigation with tools like `git log` and `git bisect`.
Risks and downfalls involve:
- Potential complications if you’ve already shared your commits with others. Rebasing alters commit history, leading to confusion.
- Increased complexity during conflict resolution, as you may encounter multiple conflicts when applying each commit.
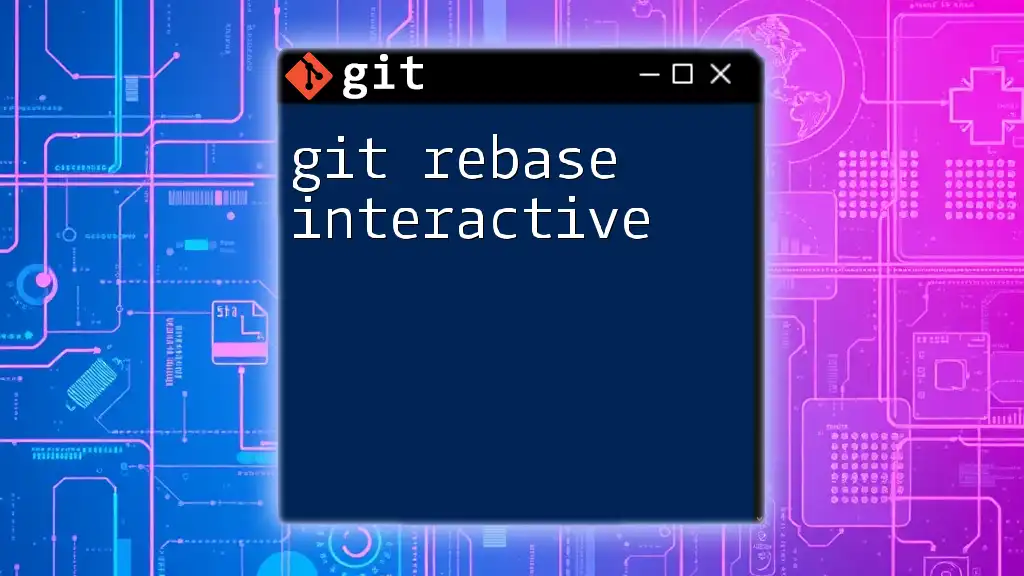
Deleting Branches
Branch Deletion Basics
Branches are used to manage features, fixes, and experiments in isolation. Once these tasks are complete, deleting branches prevents clutter in your repository. It's essential to know the difference between deleting local branches (your working copy) and remote branches (on the shared repository).
Deleting a Local Branch
To delete a local branch that is no longer needed, use the following syntax:
git branch -d <branch_name>
Here, `<branch_name>` refers to the branch you wish to delete. The `-d` option will only delete the branch if it has been fully merged. If it hasn't been merged, Git will prevent the deletion to avoid losing work.
Forcing Deletion of a Local Branch
If you’re certain about the deletion and you want to bypass the check for merged branches, you can use the `-D` option instead:
git branch -D <branch_name>
This command will forcibly delete the branch, regardless of its merged status. Use with caution!
Deleting a Remote Branch
To delete a branch on the remote repository, employ the following syntax:
git push <remote> --delete <branch_name>
In this case, `<remote>` usually refers to `origin`, and `<branch_name>` is the branch that you want to remove. This operation is essential for cleaning up branches that have been merged or are no longer in use.
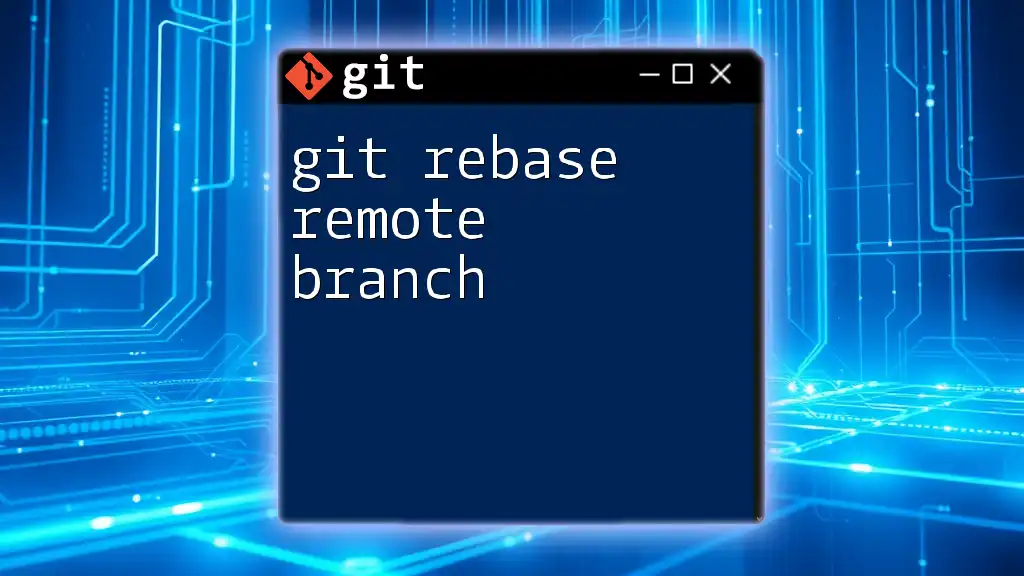
Combining `git pull`, `rebase`, and Branch Deletion
Scenarios Requiring All Three Commands
In collaborative environments, you may often find yourself needing to use `git pull`, rebase, and branch deletion together. For instance, if you’re finishing a feature and want to integrate the latest changes from the main branch while keeping a clean history, you would first pull the latest changes, rebase your feature branch to include those updates, and finally, delete your feature branch once it’s been merged.
Step-by-Step Example
Here’s a practical scenario:
-
Start by ensuring you are on your feature branch:
git checkout feature/my-feature
-
Pull the latest changes from the main branch and rebase:
git pull --rebase origin main
-
After resolving any possible conflicts and ensuring everything is working as expected, and you've merged your feature back to the main branch, it's time to clean up:
git branch -d feature/my-feature
This sequence of commands will help keep your workflow streamlined and your Git repository clutter-free.
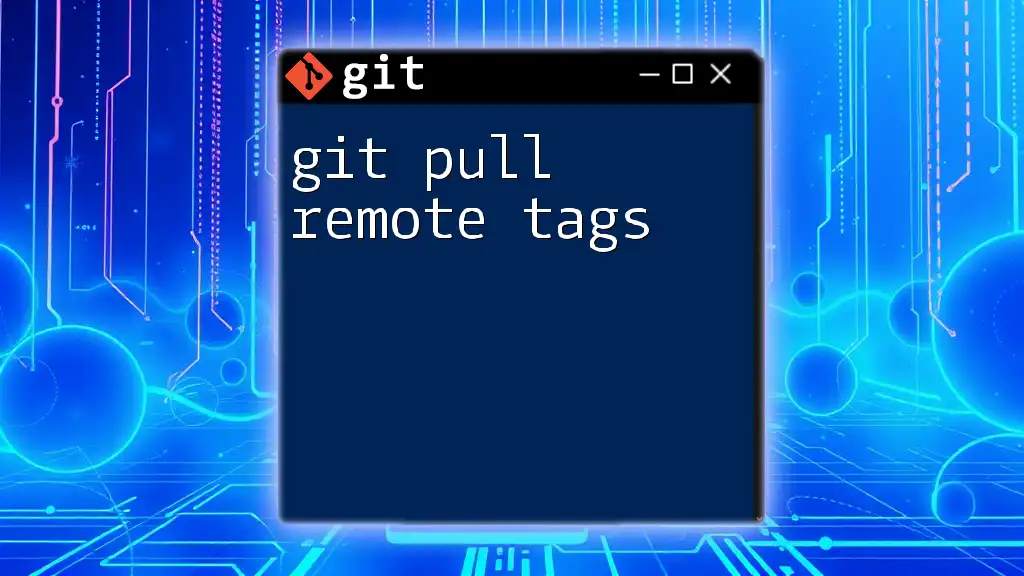
Conclusion
Mastering the commands `git pull`, `rebase`, and deleting branches is crucial in today's collaborative coding environments. Understanding when and how to use these commands will not only streamline your workflow but will also enhance your project management skills. By practicing these commands regularly, you'll find yourself navigating Git with confidence and ease.
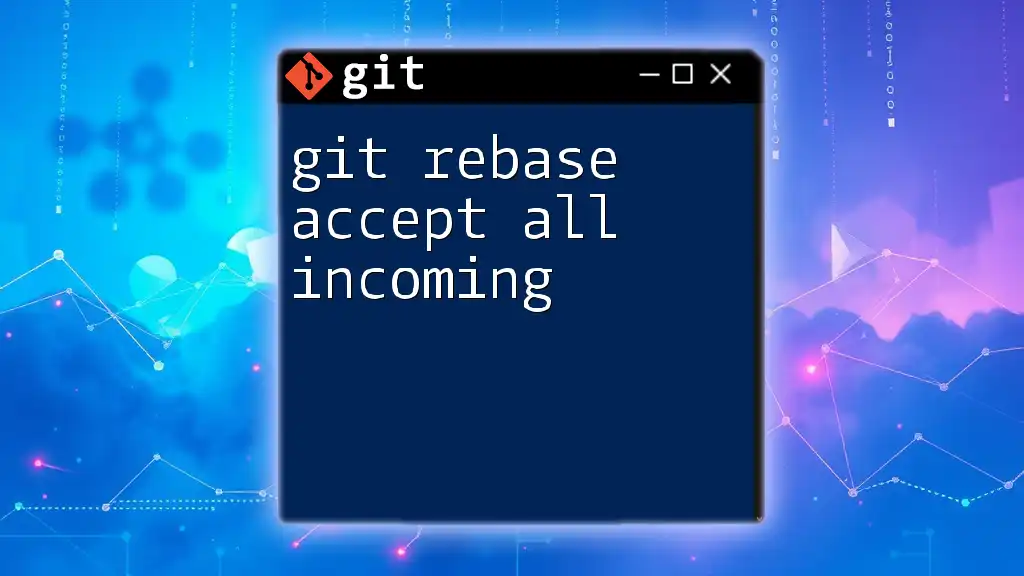
Additional Resources
For further learning, consider checking the official Git documentation, exploring recommended books, and engaging in community forums where you can share experiences, ask questions, and deepen your understanding of Git.
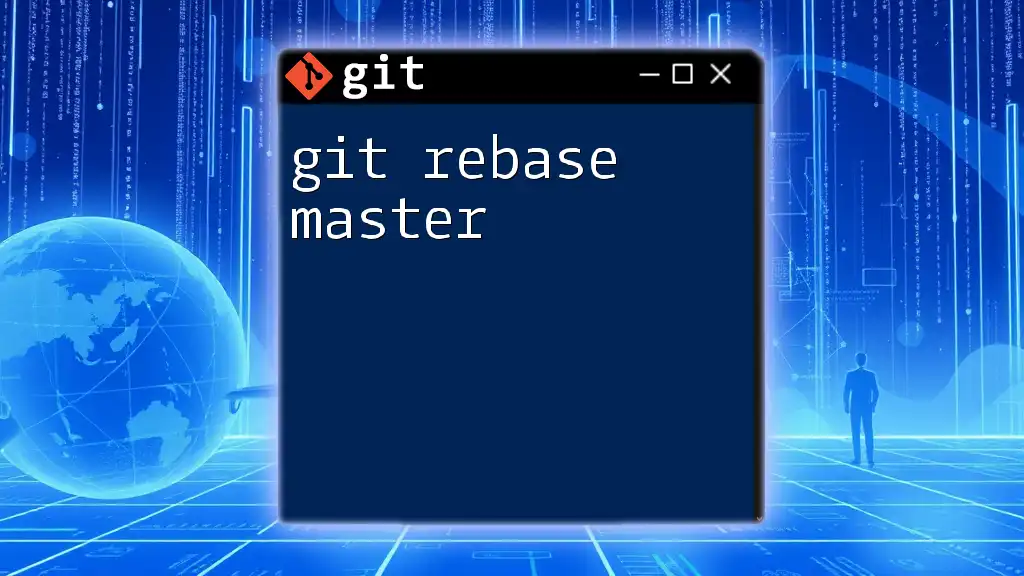
Call to Action
Stay tuned for more tips and strategies to enhance your Git skills. Feel free to leave feedback, comments, or questions regarding this article, and let’s build a robust Git learning community together!