The `git pull` command is used to fetch changes from a remote repository and merge them into the current branch, and it can be customized using configuration settings for tracking branches.
git config --global pull.rebase true
Understanding `git pull`
What is `git pull`?
`git pull` is a command that allows you to fetch and merge changes from a remote repository into your current branch in one step. It acts as a combination of two commands: `git fetch`, which retrieves the changes from the remote, and `git merge`, which integrates those changes into your local branch.
To fully grasp the functionality of `git pull`, it’s essential to recognize its differences from `git fetch`. While `git fetch` only updates your local repository with the latest changes from the remote, `git pull` applies those changes to your current working branch immediately.
Basic Syntax of `git pull`
The basic syntax for the `git pull` command is structured as follows:
git pull [<repository> [<refspec>...]]
Here’s a breakdown of each component:
-
`<repository>`: This represents the remote repository from which you want to pull updates. If omitted, Git uses the default remote, usually named `origin`.
-
`<refspec>`: This defines the branches you want to retrieve. If not specified, Git pulls the changes from the corresponding branch of the remote repository.
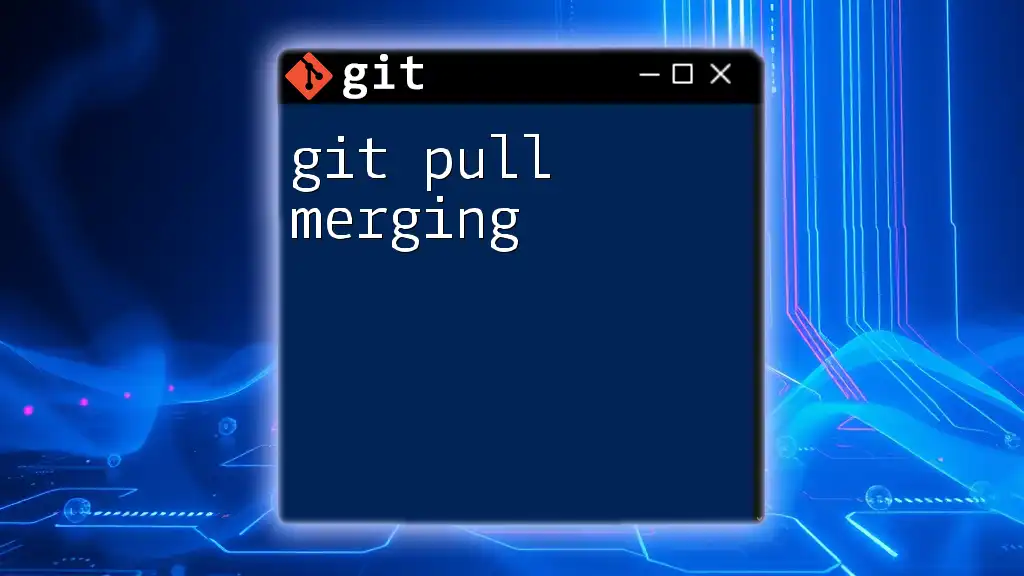
The Default Behavior of `git pull`
Working with Remote Repositories
Git needs to know where to pull the updates from, and this is managed through "remotes." Typically, the default remote for a repository is established when cloning, often referred to as `origin`. To check your current remotes, you can use:
git remote -v
To set a default remote for a local branch, use the following command:
git branch --set-upstream-to=origin/<branch-name> <local-branch-name>
Merge vs. Rebase
When executing a `git pull`, Git uses a merging strategy by default. This means it will generate a new merge commit if there are changes in both your local and remote branches. For example:
git pull origin main
In this case, any new commits from the main branch on the remote will be merged into your local branch.
Alternatively, you can choose to rebase instead of merging by using:
git pull --rebase origin main
With this approach, Git will apply your local commits on top of the commits fetched from the remote, creating a cleaner linear history.
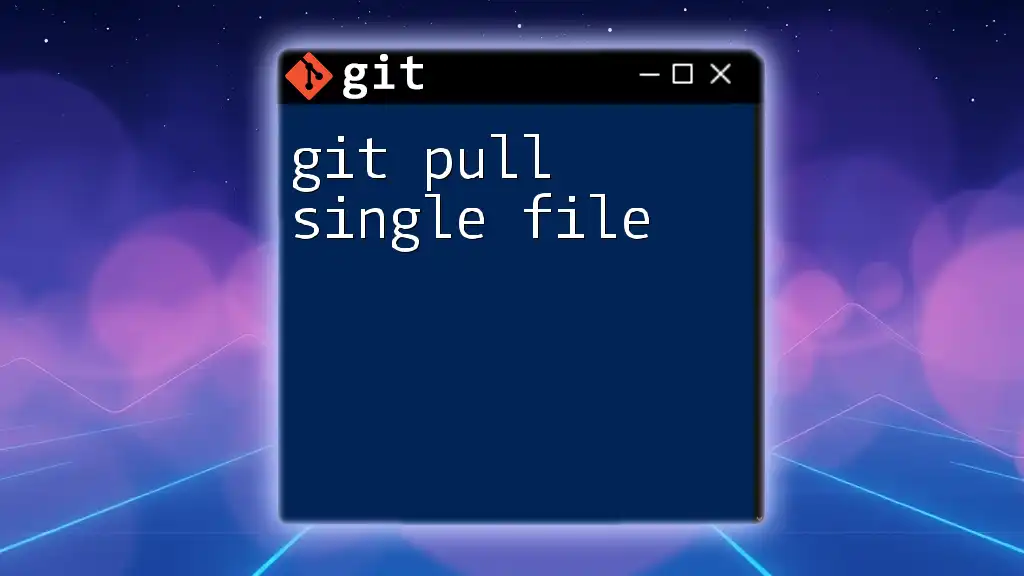
Configuring `git pull` Settings
Global Configuration
To streamline your workflow, you may want to set global defaults for how `git pull` handles updates. This can be done by configuring your Git environment with the following command:
git config --global pull.rebase true
Additionally, you can choose other options, such as `false` for merge or `interactive` if you want to manage each commit in an interactive rebase.
Per-Repository Configuration
If you need specific settings for a lone repository, you can configure it with:
git config pull.rebase false
Each repository can have its custom rules, allowing flexibility in team environments where different branches might require various update strategies.
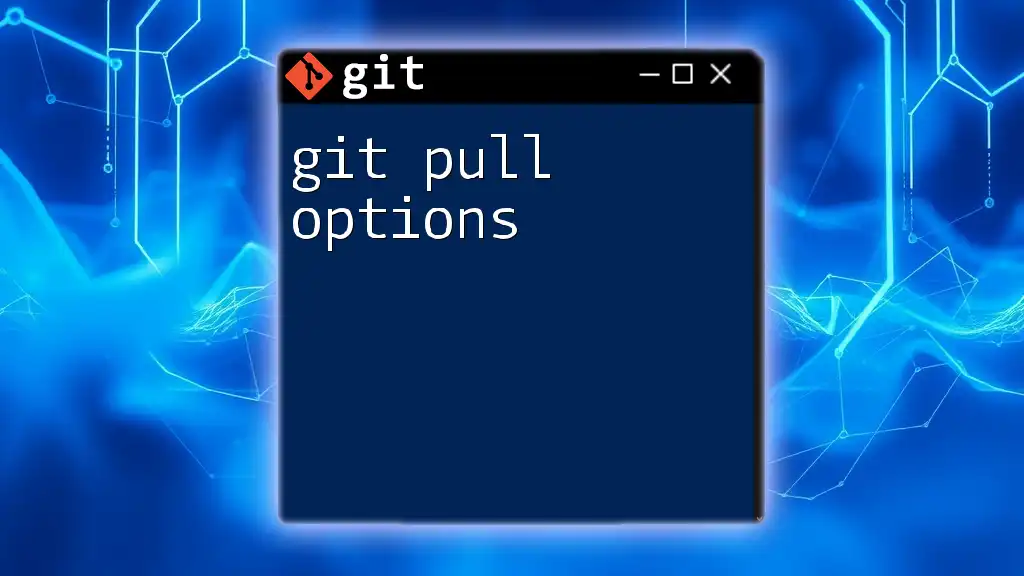
Customizing `git pull` Behavior
Interactive Pulls
Sometimes, pulling changes interactively can be beneficial. This allows you to select which changes to merge or rebase into your current branch, promoting better control over your commit history.
Fetching with `git pull`
You can enforce a fast-forward merge only, preventing automatic merges when it's unnecessary. Use:
git pull --ff-only
This command ensures that the local branch is updated without creating a merge commit if the update won’t result in a fast-forward.
Handling Conflicts
Conflicts can arise if both your local and remote changes affect the same lines of code. In such cases, Git will pause the pull process, allowing you to resolve the conflicts manually.
When facing a merge conflict, identify the files with issues and edit them. Git will mark the conflicting areas, which will look something like this:
<<<<<<< HEAD
// Your changes
=======
// Changes from the remote
>>>>>>> main
After editing, use:
git add <conflicted-file>
To stage resolved files, and then finalize the merge with:
git commit
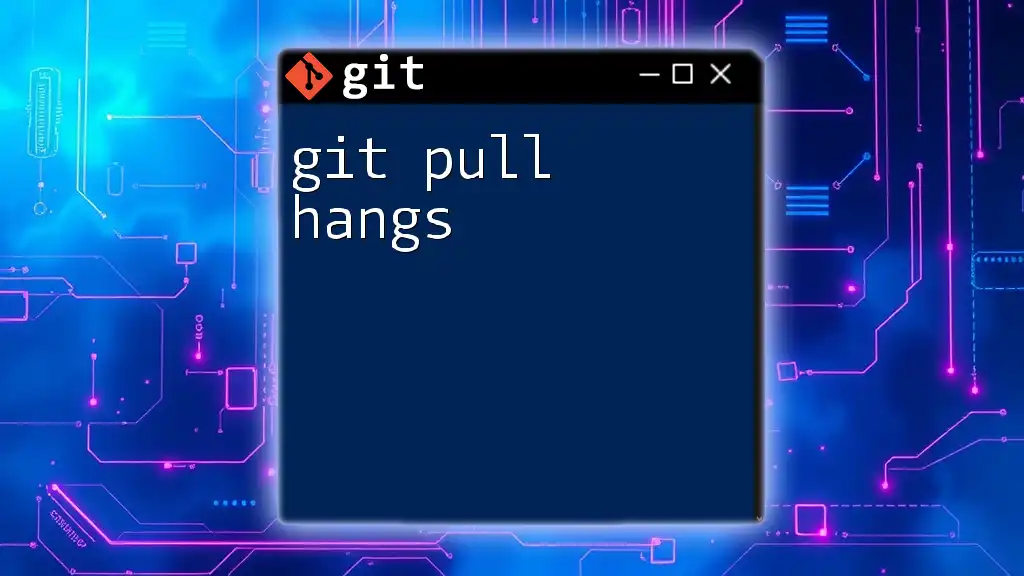
Best Practices for Using `git pull`
Frequent Pulls
Making a habit of pulling changes regularly is encouraged. Frequent pulls minimize the risk of substantial conflicts and ensure that you always have the latest updates from your collaborators.
Using Branches Effectively
Git branches are powerful tools for managing work on features or bug fixes independently. When pulling, it’s crucial to ensure you're on the appropriate branch to avoid merging changes into the wrong context.
You can switch branches using:
git checkout <branch-name>
And double-check the current branch with:
git branch
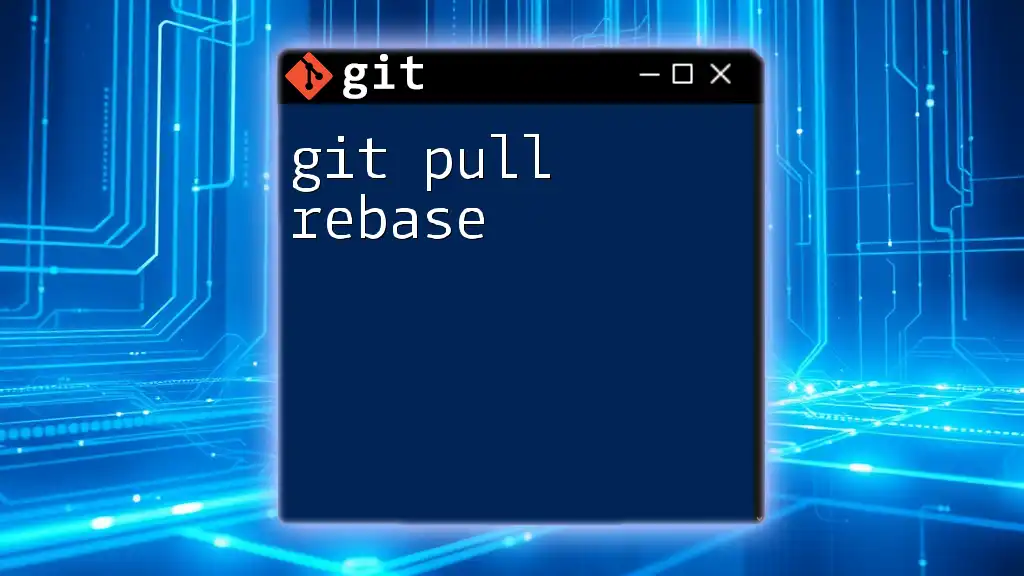
Troubleshooting Common Issues
Authentication Problems
Often, issues can arise from improper authentication credentials when attempting to pull from a remote repository. If you encounter an error stating a lack of permission, confirm your SSH setup or verify that your user has the correct permissions for the repository.
Conflicts Beyond Resolution
Sometimes, a pull can lead to more significant issues such as diverging branches or unintended project states. If you find yourself in a complex situation after a pull, you can roll back recent changes with:
git reset --hard HEAD~1
This command removes the last commit and all its changes, letting you return to a stable state.
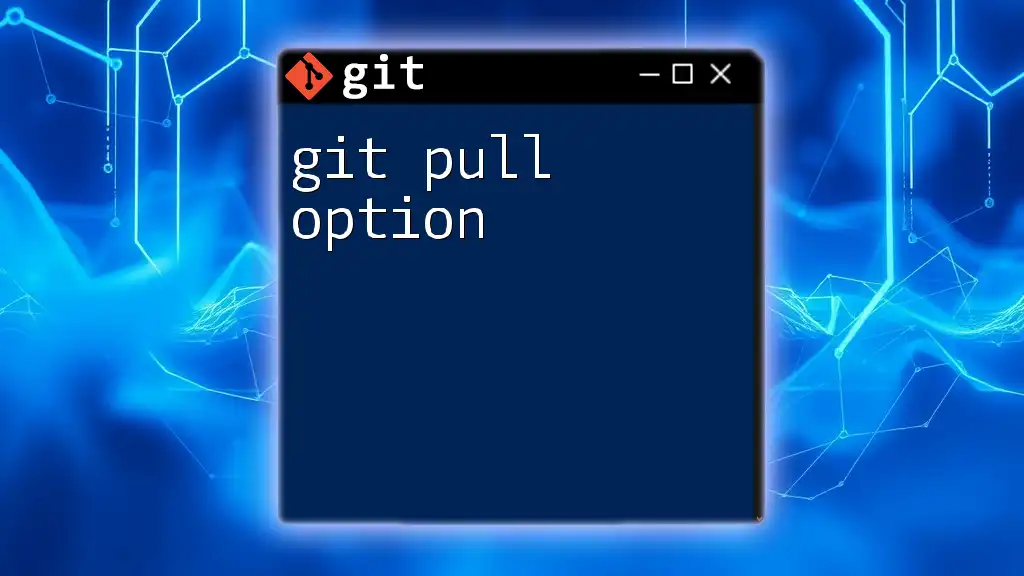
Conclusion
Understanding and mastering `git pull settings` can vastly improve your workflow and enhance collaboration among your team. By configuring `git pull` to suit your needs, you can ensure a seamless integration of changes, reduce conflicts, and maintain a cleaner project history. Don’t hesitate to explore various configurations and strategies to find what works best for you!