The command `git pull origin master` fetches the latest changes from the remote repository's master branch and merges them into your current local branch.
git pull origin master
What is `git pull`?
The `git pull` command is a fundamental part of using Git for version control. It serves as a convenient way to update your local repository with changes from a remote repository. Essentially, `git pull` is a command that combines two actions: it first fetches the latest changes from the specified remote repository, and then it merges those changes into your current branch. This two-step process ensures that your local copy is in sync with the latest updates on the server.
Common Use Cases
You would typically use `git pull` in the following scenarios:
- Regularly Syncing: If you're working with a team, using `git pull` frequently keeps your local copy up to date.
- Before Feature Development: Always pull from your main branch before starting a new feature to ensure you’re building on the latest code.
- To Review Changes: Pull changes to examine updates made by others before merging.
However, it’s essential to avoid using `git pull` when:
- You have uncommitted changes in your working directory, as merging can lead to conflicts.
- You’re in the middle of complex changes that aren’t ready to be shared.
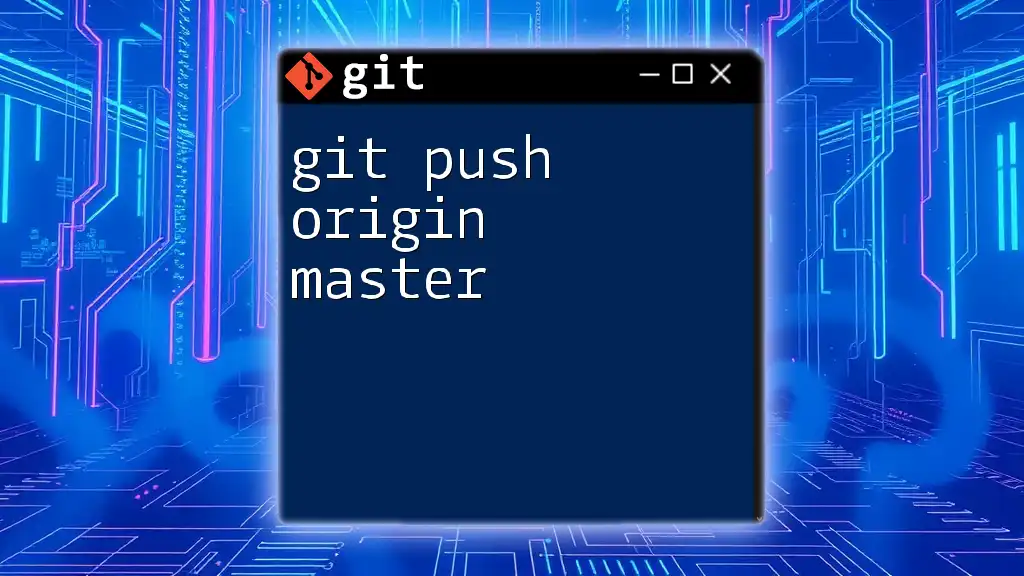
Understanding `origin` in Git
What is an Origin?
In Git, `origin` typically refers to the default remote repository where your local repository was cloned from. This name is created automatically when you clone a repo, and it serves as a shorthand for the full URL of that repository.
Changing Remote Names
If you need to rename your remote repository, you can do so with the following command:
git remote rename old-name new-name
For example, if you want to rename `origin` to `upstream`, the command would look like:
git remote rename origin upstream
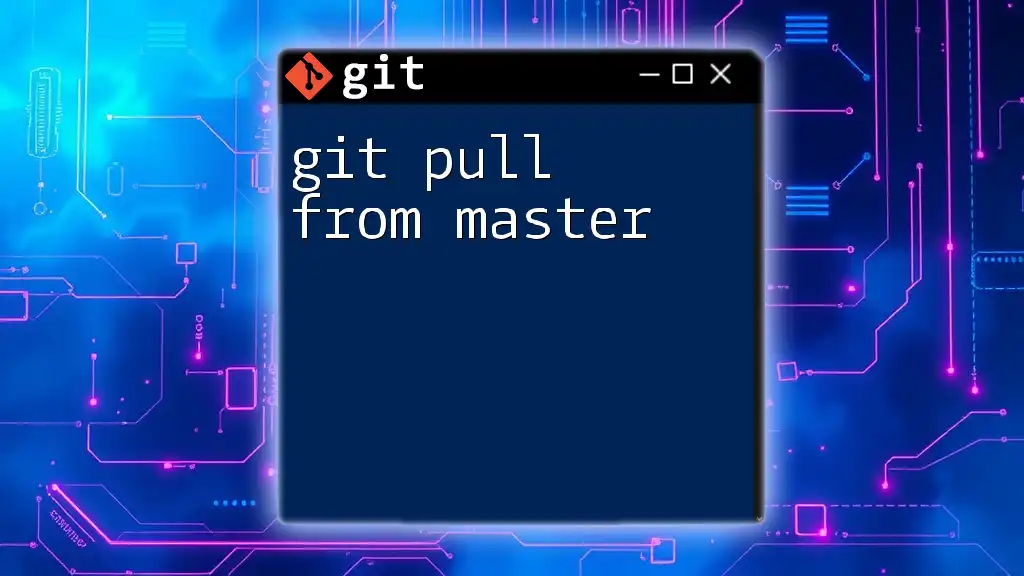
The Significance of `master`
What is the Master Branch?
Historically, the master branch has been the default primary branch for many Git repositories. This branch often contains the stable or production-ready code. Understanding how to interact with the master branch is essential for effective Git usage.
Branching Strategies
While the master branch has been the default for many years, some projects are shifting towards using `main` as their primary branch name. When working with `git pull`, it's crucial to ensure you’re pulling from the correct branch. If your project uses `main`, you would run:
git pull origin main
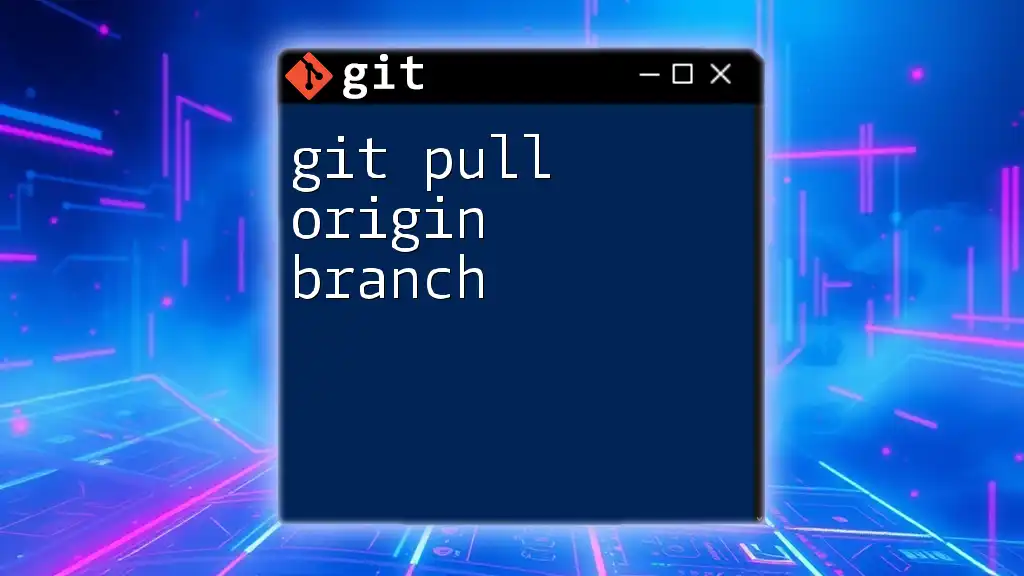
How to Execute `git pull origin master`
Basic Command Syntax
To pull the latest changes from the master branch of your remote repository, the command you'll use is:
git pull origin master
Step-by-Step Execution
-
Connect to Your Repository: Open your terminal and navigate to your local repository folder.
-
Check Your Current Branch: Ensure you are on the correct branch where you want to merge the updates. You can check your current branch with:
git branch
-
Run the Command: Execute `git pull origin master`.
Upon execution, Git fetches the changes from the `origin` repository and attempts to merge them into your current branch.
Understanding Output Messages
Following the command, you might see various output messages indicating the success or failure of the operation. Typical messages include:
- Fast-forwarded: This means Git successfully merged the changes without any conflicts.
- Merge Commit: A merge was completed with a new commit created.
- CONFLICT: This indicates that there are conflicts that need your resolution.
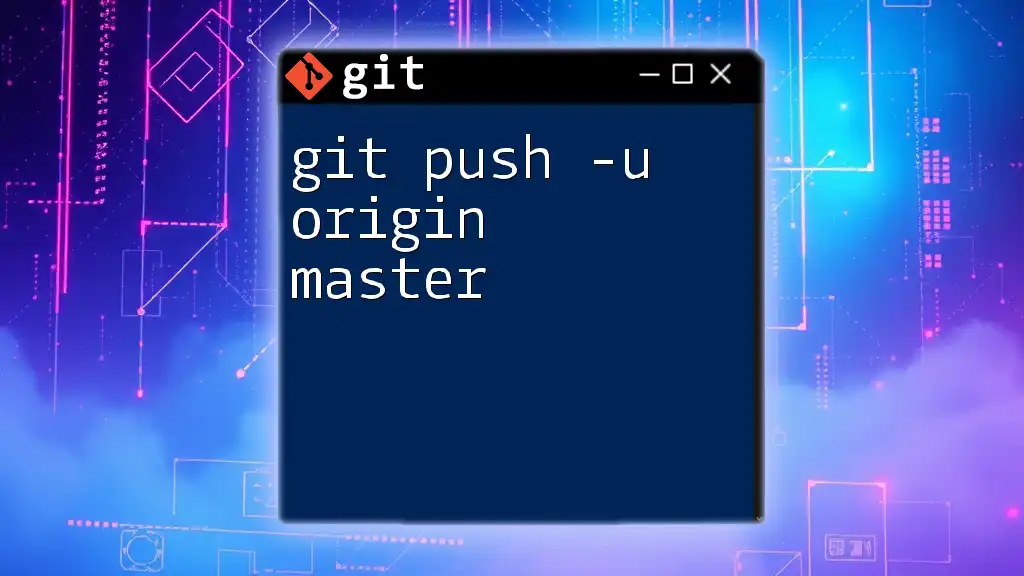
Troubleshooting Common Issues
Merge Conflicts
One common issue encountered when executing `git pull origin master` is merge conflicts. This occurs when changes made in your local branch conflict with changes pulled from the remote branch.
To resolve a merge conflict:
-
Identify the conflicted files marked in your terminal.
-
Open the files and look for the conflict markers (`<<<<<<`, `======`, `>>>>>>`).
Edit the file to resolve the conflicts manually.
-
After resolving conflicts, stage the changes using:
git add <file-name>
- Finally, commit the changes:
git commit -m "Resolved merge conflict in <file-name>"
Detached HEAD State
Sometimes, you may end up in a detached HEAD state. This can happen if you check out a specific commit. To resolve this, you can navigate back to a stable branch, usually using:
git checkout master
Now you can run `git pull origin master` again.
Local Changes vs. Remote Changes
If you have uncommitted local changes, you could face challenges when trying to pull new updates. To safely pull changes, use the `git stash` command to temporarily store your changes:
- Stash your changes:
git stash
- After stashing, run the `git pull` command:
git pull origin master
- Once the pull is complete, reapply your stashed changes:
git stash pop
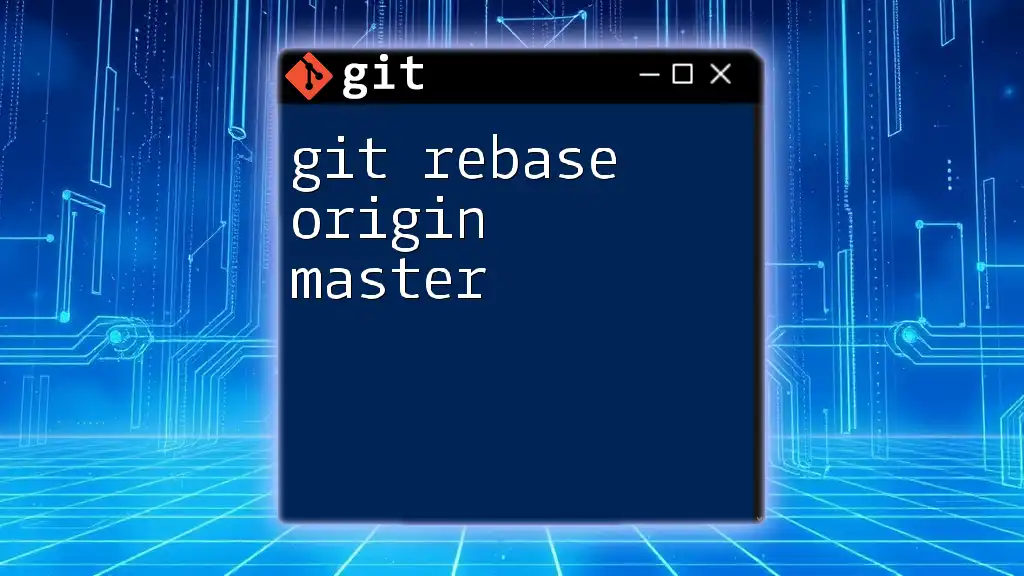
Best Practices for Using `git pull origin master`
Pulling Frequently
Frequently using `git pull` allows you to stay updated and minimizes the chance of large merge conflicts later on. By integrating updates regularly, your local branch remains aligned with the team's work.
Synchronizing with Team Members
Communicate with your team about when you’re pulling changes. This helps coordinate efforts and ensures everyone is on the same page. Consider scheduling regular pulls and merges, especially in larger teams.
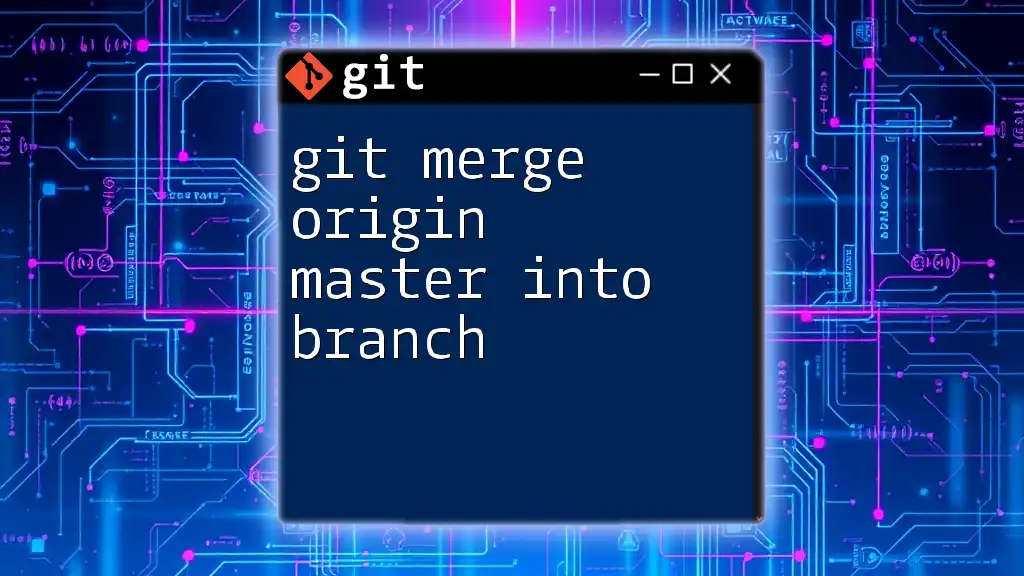
Alternatives to `git pull origin master`
Using `git fetch` and `git merge`
If you prefer more control over the updating process, consider using `git fetch` followed by `git merge`. This method allows you to see incoming changes before merging them. The commands would look like this:
git fetch origin
git merge origin/master
Pulling from Other Branches
If you need to pull changes from a different branch (say `feature-branch`), simply specify that branch:
git pull origin feature-branch
This approach can come in handy when collaborating on multiple features.
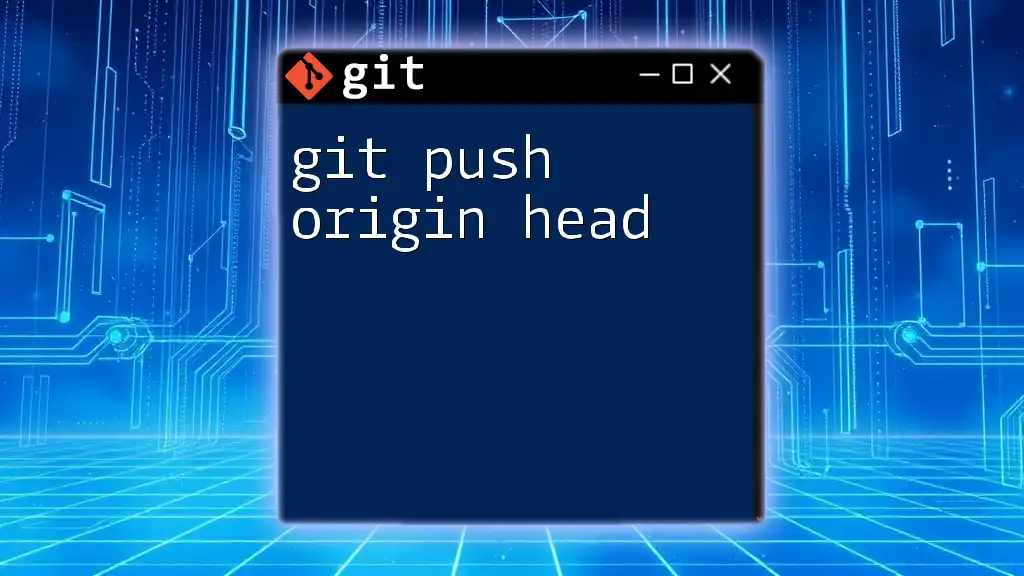
Conclusion
Understanding how to effectively use `git pull origin master` is crucial for anyone involved in collaborative code development. This command enables you to keep your work in line with ongoing changes from your team or project repositories. By practicing good habits like frequent pulls, managing conflicts, and communicating effectively, you’ll foster a smoother workflow and more efficient collaboration.
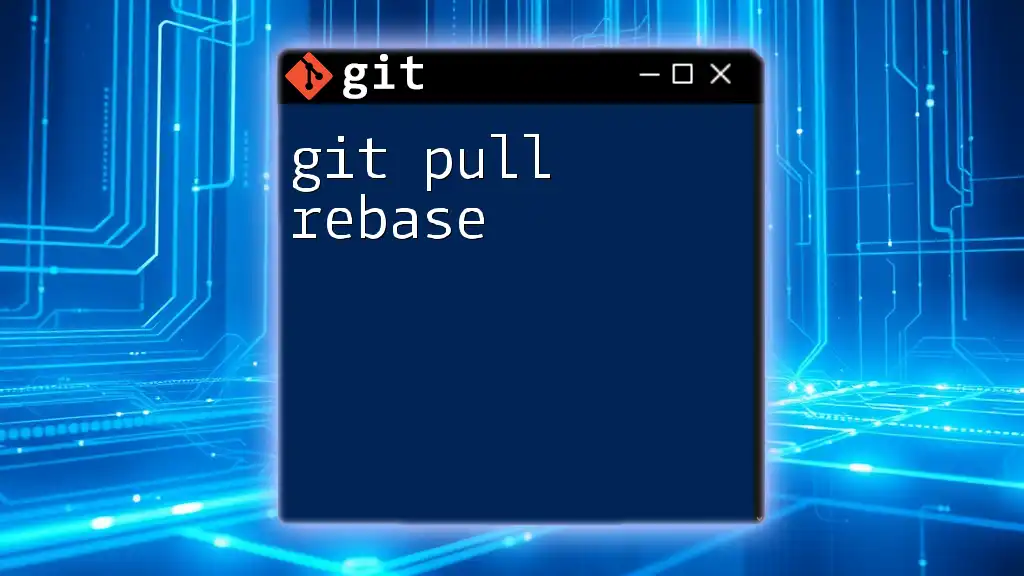
Additional Resources
To further enhance your understanding of Git, explore official documentation, online courses, or cheat sheets focused on Git commands. Continuously learning will empower you to handle version control with confidence and proficiency.