The command `git push -u origin master` pushes your local `master` branch changes to the remote repository named `origin` and sets upstream tracking for future pushes and pulls.
git push -u origin master
Understanding Git Basics
What is Git?
Git is a distributed version control system that allows multiple developers to collaborate on projects efficiently. It enables users to track changes, revert to previous versions, and create branches to manage different lines of development. Key features of Git include:
- Version Tracking: Git logs changes, allowing you to easily revert to previous versions of your project.
- Branching and Merging: Create isolated environments for feature development, bug fixes, and testing.
- Collaboration: Multiple users can work on the same project simultaneously without interfering with each other's changes.
Repositories
Local vs Remote Repositories
A local repository resides on your personal machine, where you make changes and commits. A remote repository, such as GitHub or GitLab, serves as a shared storage for your code.
The Git workflow often involves the following steps:
- Commit changes locally.
- Push those changes to the remote repository.
This process ensures that your local changes are safely stored and accessible to your collaborators.
Branching
What is a Branch?
A branch in Git represents an independent line of development. When you create a branch, you're making a copy of the code at a specific point, allowing you to experiment with new features or fixes without impacting the main codebase.
Master Branch
Traditionally, the `master` branch was the default branch where production-ready code resides. However, many projects are now adopting renaming conventions such as `main` for inclusivity. Regardless, the principles remain the same.
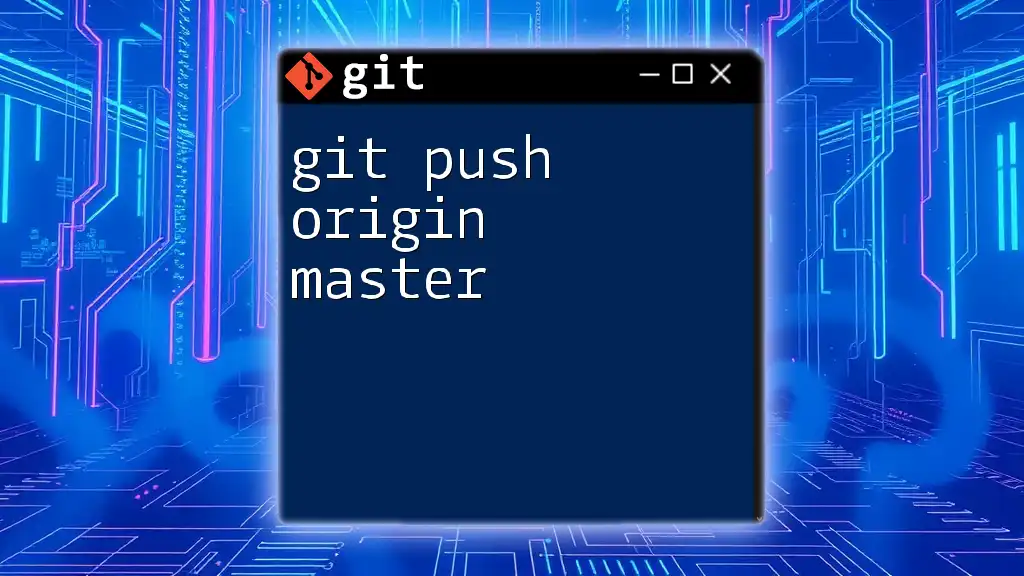
The `git push` Command
What is `git push`?
The command `git push` is used to upload your local repository changes to a remote repository. When you execute a push operation, Git transfers all committed changes in your specified branch to the remote repository.
Syntax of `git push`
The general syntax for the `git push` command is:
git push [options] [<repository>] [<refspec>]
This syntax allows for flexibility, as you can specify various options, the target repository, and the branch you want to push.
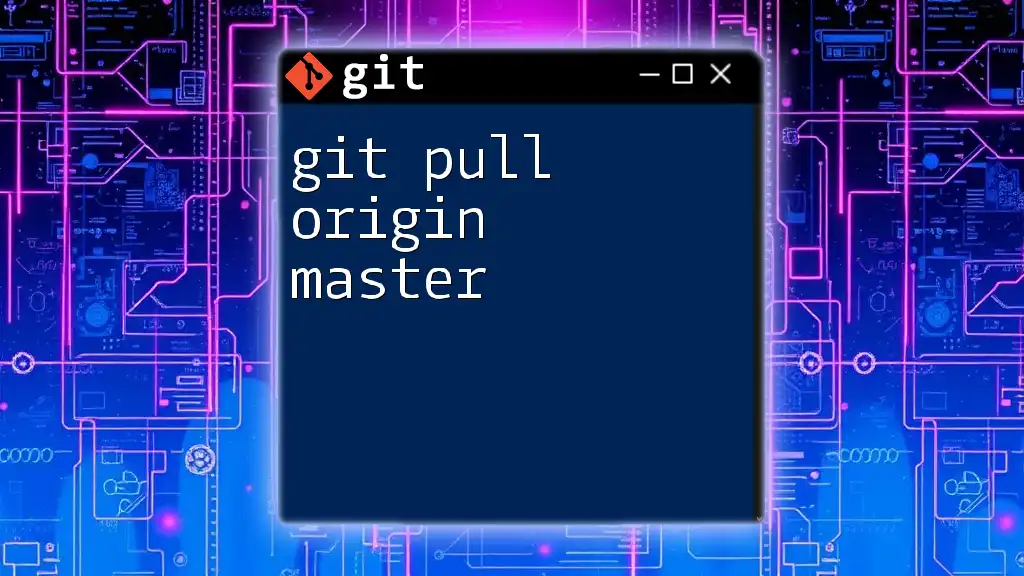
The `-u` Option Explained
What Does `-u` Stand For?
The `-u` flag stands for `--set-upstream`. This option establishes a tracking relationship between your local branch and the corresponding branch in the remote repository.
How `-u` Affects Your Workflow
By using the `-u` flag, you simplify your future push and pull operations. Once you set the upstream branch, you can simply use `git push` or `git pull` without specifying the remote and branch name each time.
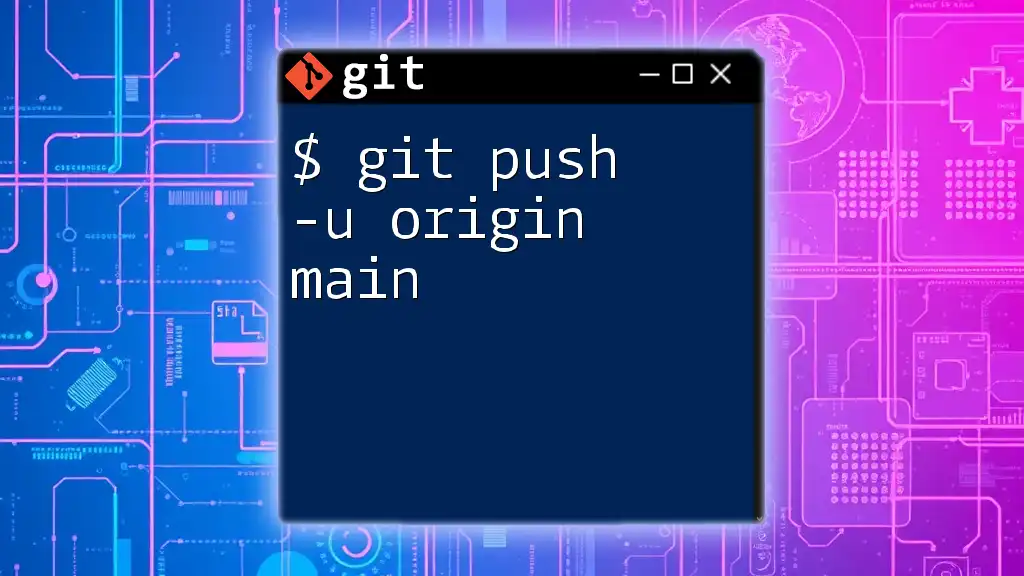
The `origin` Keyword
Understanding `origin`
In Git terminology, `origin` is the default name for your remote repository. By convention, when you clone a repository, Git automatically names the remote repository as `origin`.
How to Check Your Remote
To verify your remote settings, use the command:
git remote -v
This command will return a list of your remotes along with their fetch and push URLs. If needed, you can rename your remotes to something more descriptive.
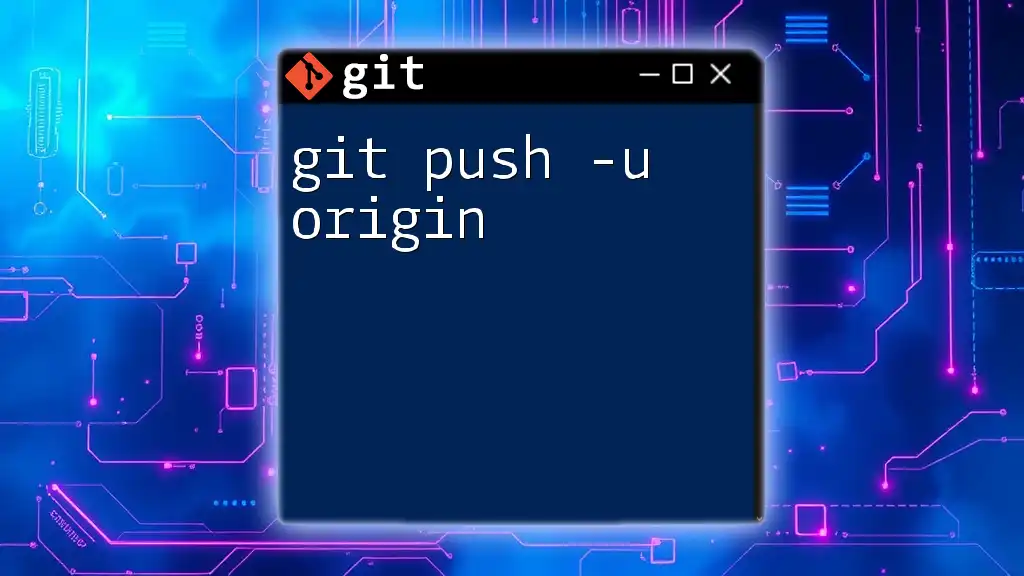
The `master` Branch in Context
Importance of Branching Strategies
Maintaining a clean and functional `master` branch is vital for a successful collaborative environment. Pushing changes to `master` often signifies that those changes are stable and ready for production.
Common Practices for the Master Branch
Before pushing changes to `master`, consider these best practices:
- Merge Before You Push: Always merge changes from your feature branch into `master` to ensure you're working with the latest code.
- Resolve Conflicts: Address any conflicts beforehand to ensure a clean commit history.
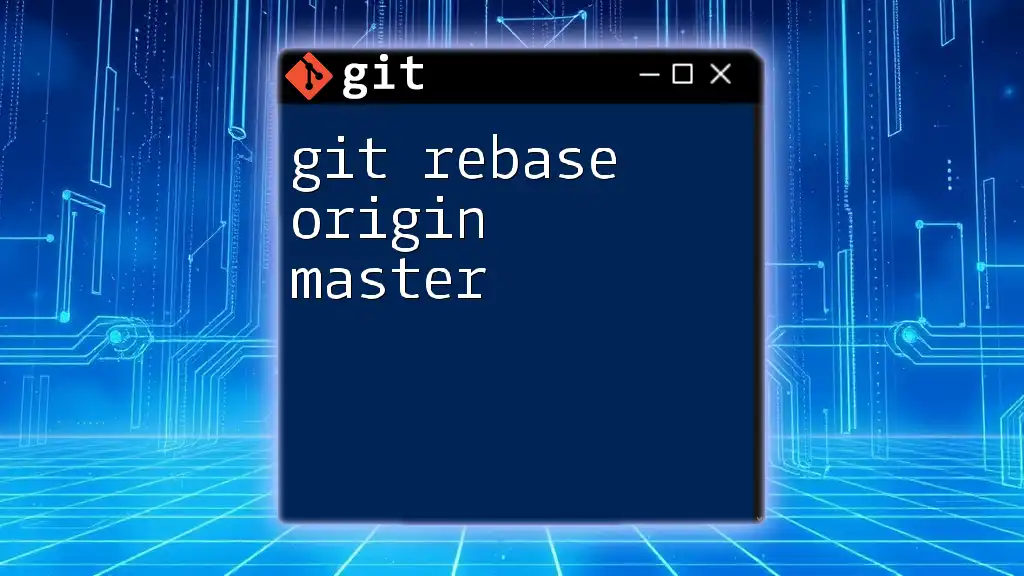
Walkthrough Example
Setting Up Your Project
To illustrate the use of `git push -u origin master`, let us start from scratch. Open your terminal and follow these steps:
- Initialize a new Git repository:
git init my-project
cd my-project
- Create a new file and add some content:
echo "Hello, Git!" > README.md
- Stage the changes:
git add README.md
- Commit the changes:
git commit -m "Initial commit"
Executing the Command
Now let’s run the command `git push -u origin master` to push your changes to the remote repository:
git push -u origin master
During this operation, Git will establish a link between your local `master` branch and the `origin` master branch. After running this command, the expected output will confirm that your changes have been pushed successfully.
Troubleshooting Common Issues
When using `git push -u origin master`, you may encounter common errors, such as:
- Authentication Issues: Ensure that you have the necessary permissions for the repository.
- Rejected Pushes: If someone else pushed changes to `master` since your last pull, resolve conflicts before trying again.
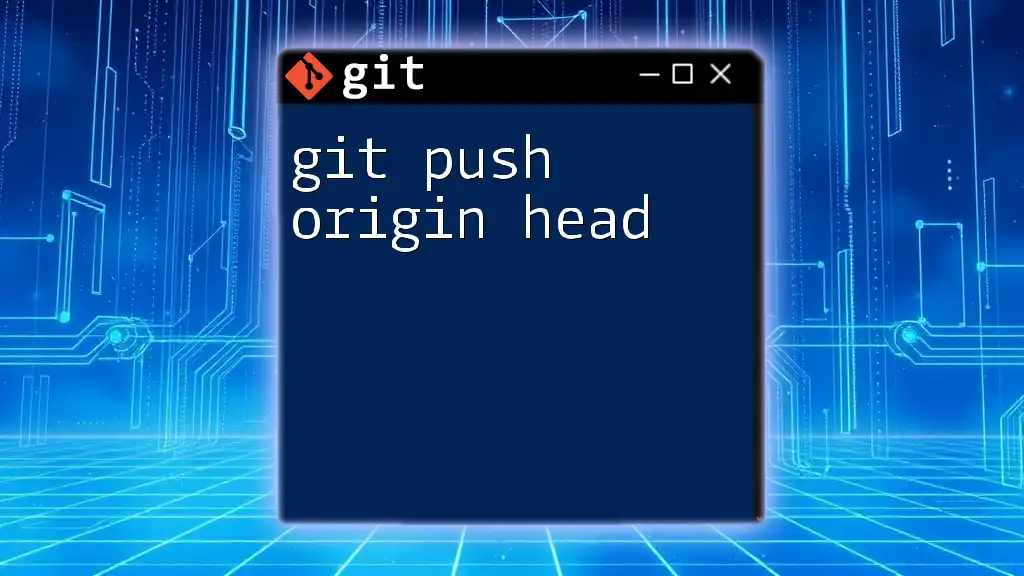
Conclusion
Understanding the command `git push -u origin master` is crucial for effective collaboration in software development. By mastering this command, you can ensure your workflows are efficient and error-free. Remember to practice with real repositories and foster your Git skills for a smoother coding experience.
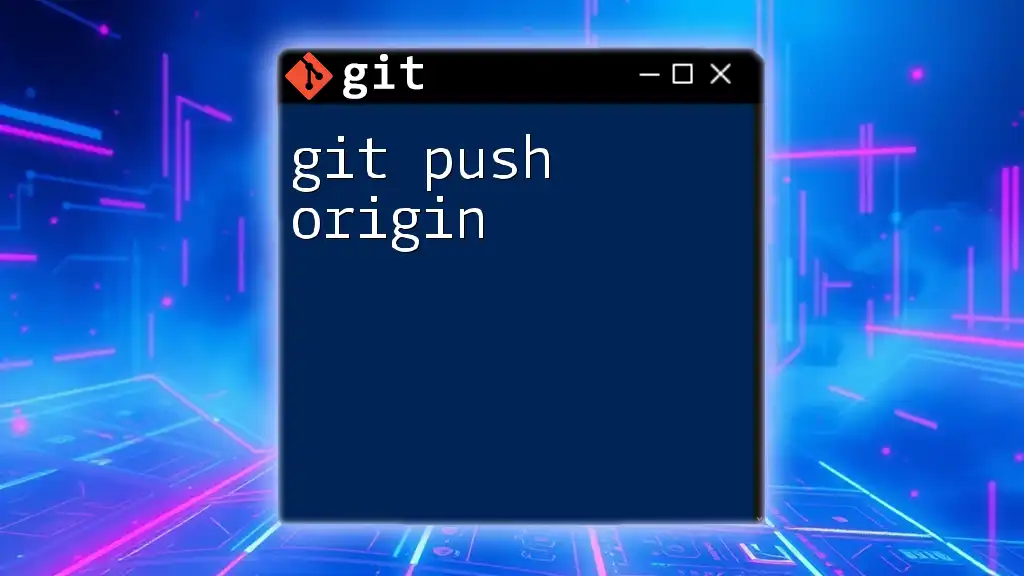
Additional Resources
For further reading, refer to the official Git documentation and explore online tutorials and interactive coding platforms that focus on Git. These will help you reinforce your command-line skills and deepen your understanding of version control.
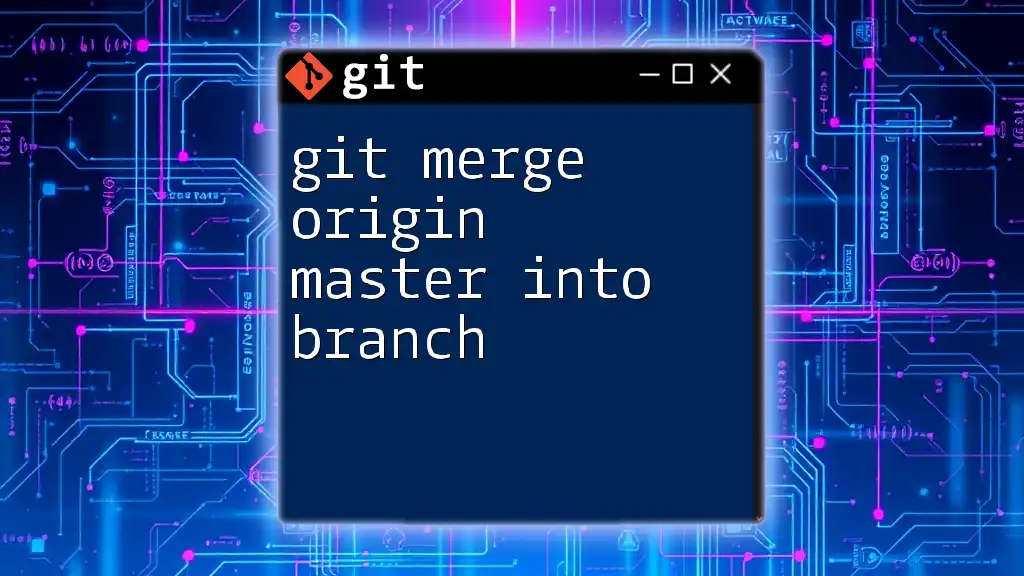
FAQ Section
You may still have questions regarding `git push -u origin master`. Here are some common inquiries and quick answers to enhance your understanding and confidence in using this command effectively.