The command `$ git push -u origin main` uploads your local `main` branch to the `origin` remote repository and sets it as the default upstream branch for future pushes and pulls.
git push -u origin main
Understanding the Basics of Git
What is Git?
Git is a distributed version control system that enables multiple people to work on a project concurrently. It tracks changes to files and allows for easy collaboration by maintaining a history of revisions. The power of Git lies in its ability to manage different versions of a project, making it invaluable for both individual developers and large teams.
Key Concepts in Git
-
Repository: A repository (or repo) is where all the files, including the historical data of changes made to the project, are stored. It serves as the core of your project.
-
Branches: Branching allows you to diverge from the main line of development and isolate changes related to specific features or fixes. This enables multiple features to be developed simultaneously without affecting the stable version.
-
Commits: A commit represents a snapshot of your files at a particular point in time, along with a message that describes the changes you've made. Each commit is identified by a unique hash, which makes it easy to track changes back in time.
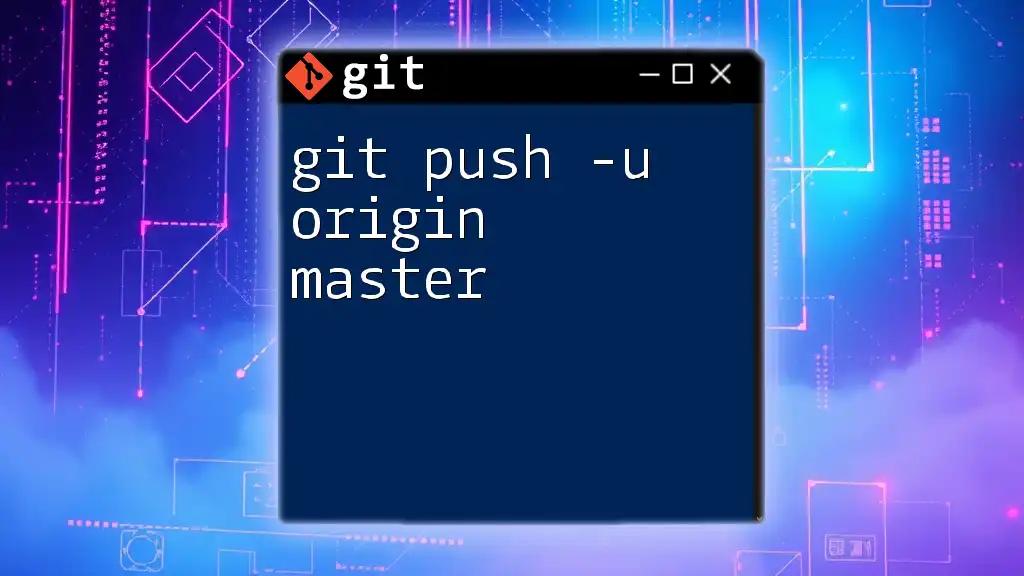
Breaking Down the Command `$ git push -u origin main`
The Purpose of the Command
The command `$ git push -u origin main` is essential for uploading your local commits to a remote repository, typically housed on platforms like GitHub or GitLab. This command syncs your changes so that others can see them and contribute further, ensuring that everyone is on the same page regarding the project's status.
Detailed Breakdown of Each Component
`$ git push`
The `push` command is used to upload your local commits to a remote repository. It essentially transfers your changes from your local branch to a corresponding branch on the remote. This is vital for sharing your progress with teammates.
`-u` (or `--set-upstream`)
The `-u` flag, short for `--set-upstream`, is crucial for establishing a tracking relationship between the local branch you're working on and the remote branch you're pushing to. Using this flag means that future `git push` and `git pull` commands will automatically refer to that remote branch, making your workflow smoother and more efficient.
`origin`
In Git, `origin` is the default name given to the main remote repository from which you cloned your project. It's a shorthand reference, allowing you to avoid typing the full URL of the remote repository every time you need to interact with it. Recognizing the use of `origin` simplifies the command structure, enhancing productivity.
`main`
The term `main` refers to the primary branch in your project. Historically, this was often called `master`, but many communities have shifted to `main` to avoid any insensitive connotations. The `main` branch is typically the stable branch where production-ready code resides, and it serves as the basis for creating new features.
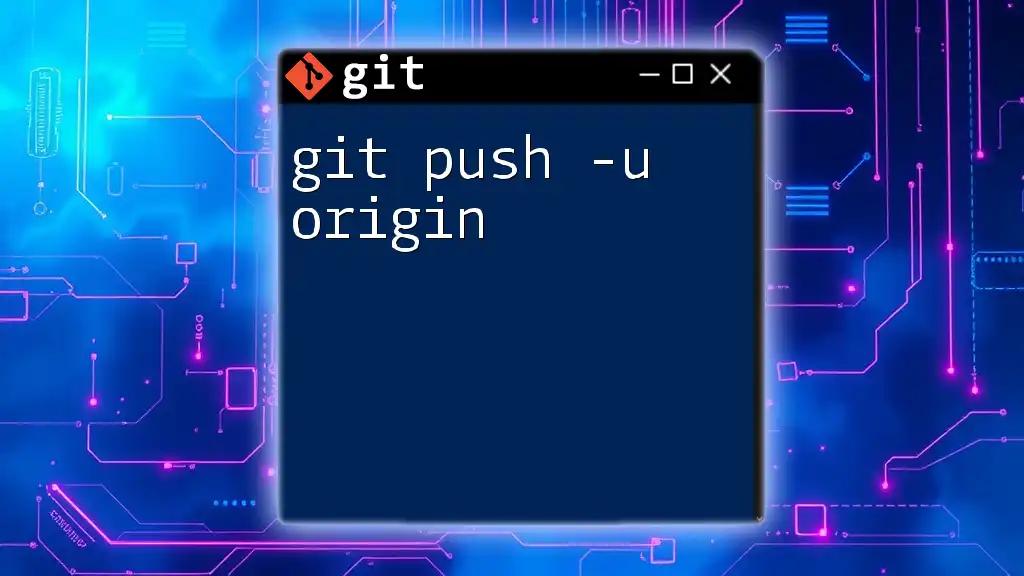
How to Use `$ git push -u origin main` in Your Workflow
Setting Up Your Repository
-
Initializing a Git Repository: Start by creating a new directory for your project and navigating into it. Then initialize the Git repository using:
git init
-
Creating a Branch and Making Your First Commit: Once your repository is initialized, create a branch, make changes, and commit them:
git checkout -b feature-branch // Make your changes to files git add . git commit -m "First commit on feature-branch"
Using the Command in Practice
After committing your changes, you're ready to push them to the remote repository. Assuming you've already set up the remote repository, you would execute:
git push -u origin main
Running this command uploads your local commits on the `main` branch to your remote repository (origin). If the `main` branch does not exist on the remote, it will be created. After this push, your local `main` branch is set to track the remote `main` branch, making future pushes and pulls convenient.
Best Practices
- Utilize the command `git push -u origin <branch>` when pushing a new branch to keep your workflow organized. Future pushes will require just `git push` or `git pull`.
- Maintain a clean commit history by ensuring you test your code before pushing to the `main` branch. This avoids introducing errors into the primary codebase.
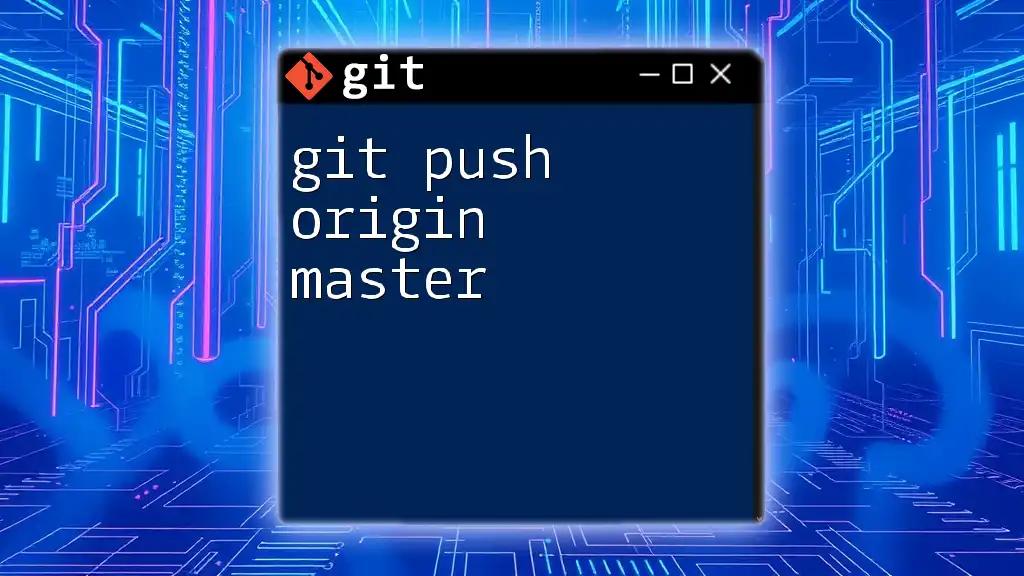
Common Errors and Troubleshooting
Possible Errors When Pushing
-
"failed to push some refs to ...": This error usually occurs when someone else has pushed changes to the remote branch that you do not have in your local version. To resolve this, you should first pull the latest changes and then attempt to push again:
git pull origin main git push
-
"error: src refspec main does not match any": This typically indicates that the `main` branch does not exist in your local repository yet. Ensure you have created the branch and made at least one commit before pushing.
Solutions to Common Problems
- Always check your branch status using `git status` before performing a push to confirm you are on the intended branch.
- If you encounter issues related to permissions, ensure you have the necessary access rights to push to the remote repository.
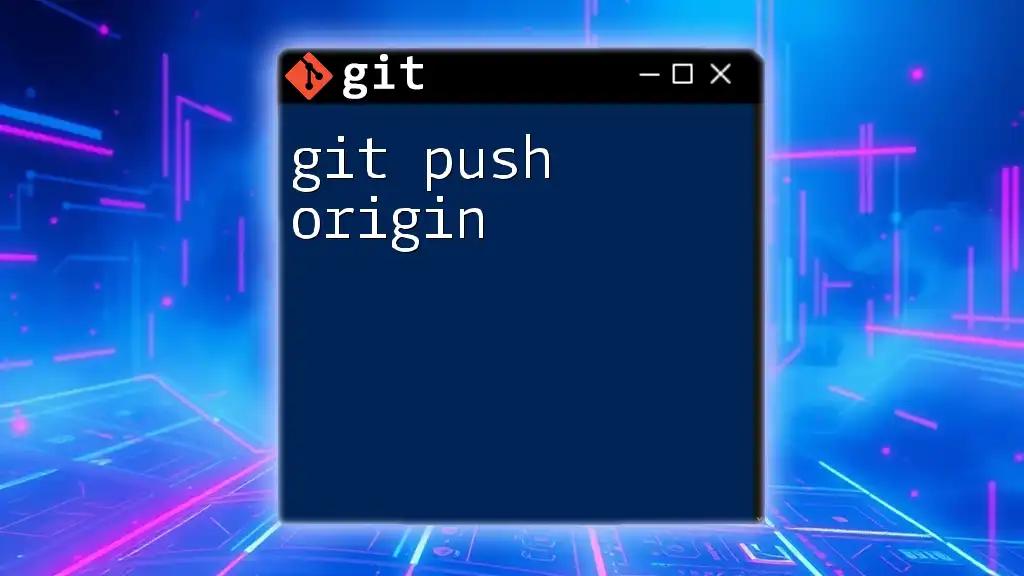
Conclusion
The command `$ git push -u origin main` is a pivotal part of the Git workflow, facilitating collaboration and version control. By understanding its components and practicing its use, you can streamline your development process, ensuring that your team stays up-to-date with the latest changes.
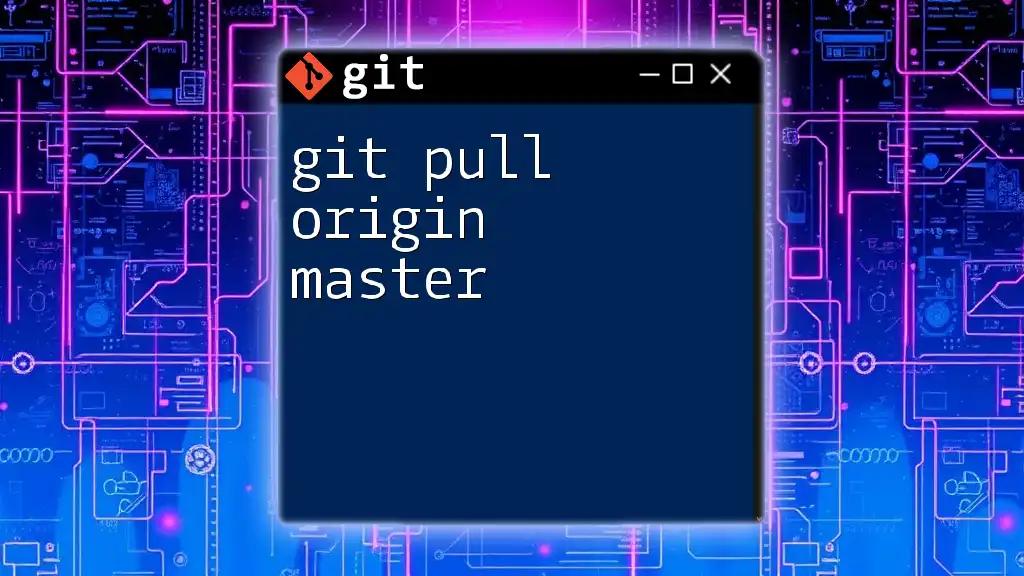
Additional Resources
To deepen your Git knowledge, refer to the official Git documentation and explore online tutorials that offer hands-on experience with Git commands and workflows. Crafting practical exercises around pushing and pulling can solidify your understanding and build confidence in using Git effectively.
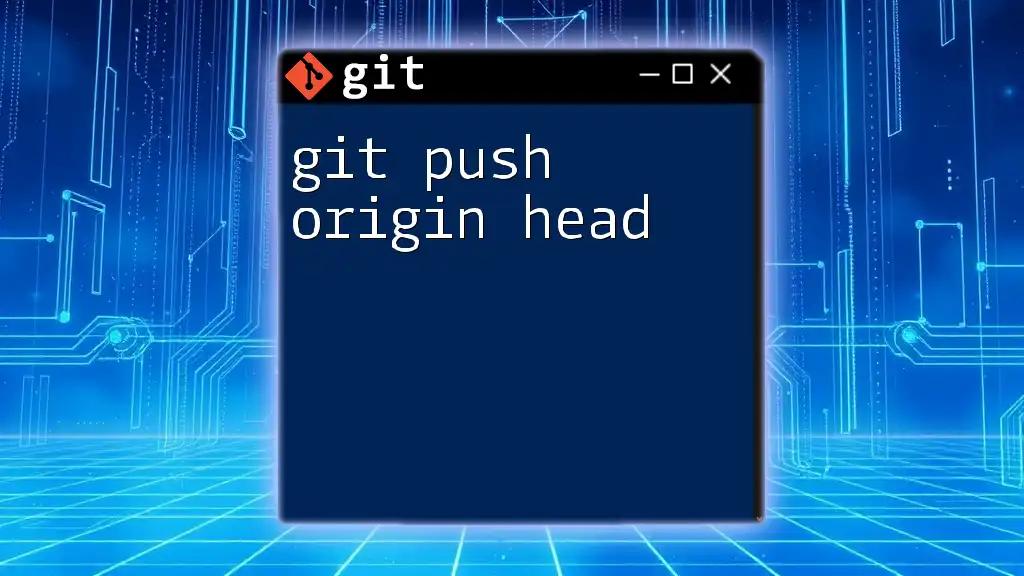
FAQ Section
What does `set upstream` mean?
Setting upstream establishes a tracking relationship between your local branch and the remote branch. This means that any subsequent push or pull will automatically reference the specified remote branch, greatly simplifying your workflow.
Can I push to a different branch without `-u`?
Yes, you can push changes to a different branch without using `-u`, but you will need to specify the full command each time. For example, to push to a branch called `dev`, you could run:
git push origin dev
How does this command differ in other Git workflows?
In advanced workflows like Git Flow or feature branching, the fundamental command remains the same. However, the context in which you push may differ, as integration conditions and branch selection might vary greatly depending on your team's guidelines.