The `git push` command is used to upload local repository changes to a remote repository, and can be customized using various options to control its behavior.
Here’s an example of the syntax:
git push origin main
Understanding the Basics of `git push`
What is `git push`?
`git push` is a command used to upload your local repository content to a remote repository. When you make changes to files and commit them locally, `git push` allows you to share those changes with other developers by sending your commits to the corresponding remote branch.
Importance of `git push` in Collaboration
The execution of `git push` is critical in collaborative environments. Whenever you push your updates to a remote repository, you ensure that your team members see the progress and modifications you've made. This seamless sharing helps to maintain synchronization across different team members, reducing the potential for conflicts down the line.
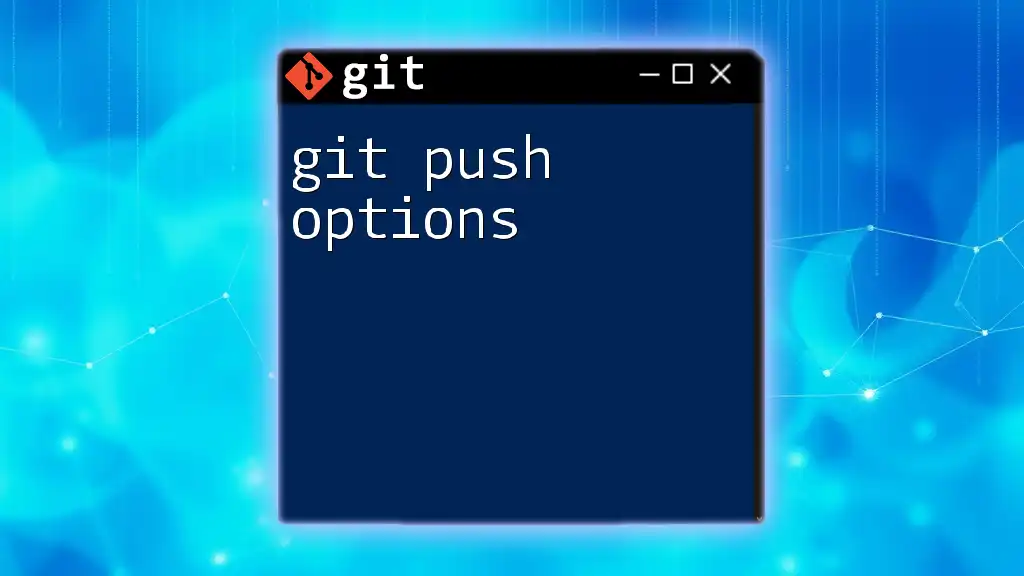
The Syntax of `git push`
General Syntax
The general syntax for the `git push` command is straightforward:
git push <remote> <branch>
- Remote: A remote repository is a version of your project that is hosted on the internet or network. Commonly used remotes are named `origin`, which typically points to the primary repository.
- Branch: Branches in Git serve as independent lines of development. Each branch allows you to work on different features or fixes without interfering with the main project code.
Example of Basic Push
An example command might look like this:
git push origin main
In this example, you are pushing all committed changes from your local `main` branch to the `main` branch on the remote repository named `origin`. After executing this command, others can see your changes.
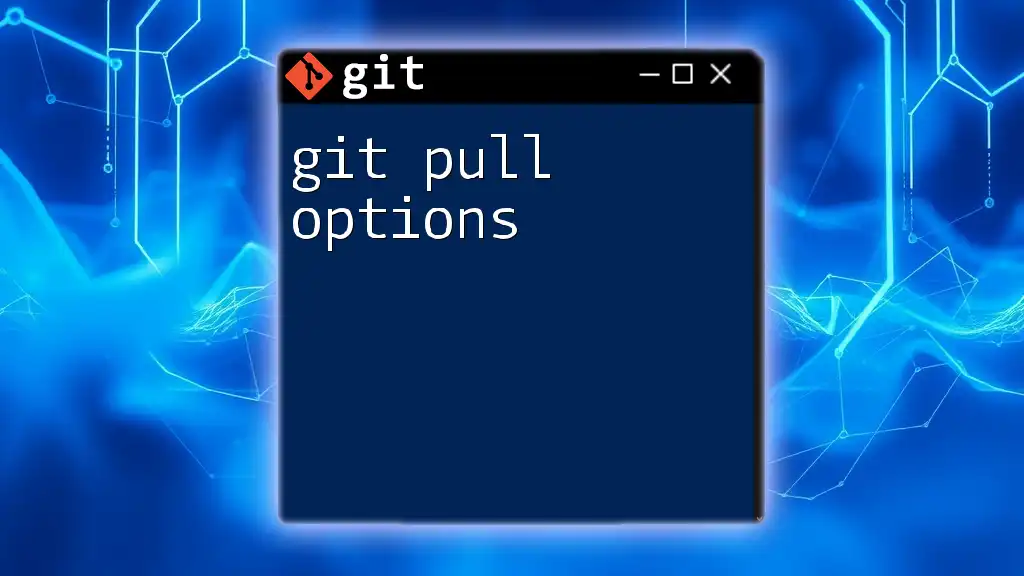
Common `git push` Options
`-u` or `--set-upstream`
The `-u` or `--set-upstream` option is used to link your local branch to a remote branch with one command. This simplifies future pushes, as you won’t need to specify the remote and branch each time.
For example:
git push -u origin main
Using this command means anytime you run `git push` afterward, Git knows to push your local `main` branch to `origin/main`. This is particularly useful for new branches or when starting a fresh collaboration, as it creates a clear line of continuity.
`--force` and `--force-with-lease`
Both of these options allow you to push changes that might otherwise be rejected by Git due to conflicts between the remote and local repositories.
- `--force`: This option forces the push without considering remote changes. While useful in certain contingencies, it can overwrite work done by others, which may lead to data loss.
Example:
git push --force origin main
- `--force-with-lease`: This alternative is safer; it checks if the remote branch has changed since the last fetch. If there are updates from other contributors, the command will fail, preventing accidental overwriting of their work.
Example:
git push --force-with-lease origin main
You should prefer `--force-with-lease` to safeguard against unintended consequences.
`--tags`
Tags are often used to mark specific points in your repository’s history, such as releases. When you want to share these tags, you can do so with the `--tags` option.
Example:
git push origin --tags
This command pushes all tags from your local repository to the remote, which is especially handy when you have a release version prepared.
`--all`
If you want to push all local branches to the remote repository simultaneously, the `--all` option is your best choice.
Example:
git push origin --all
This is useful in a scenario where multiple developers have been working on their respective branches and you want to update the remote repository with all their progress.
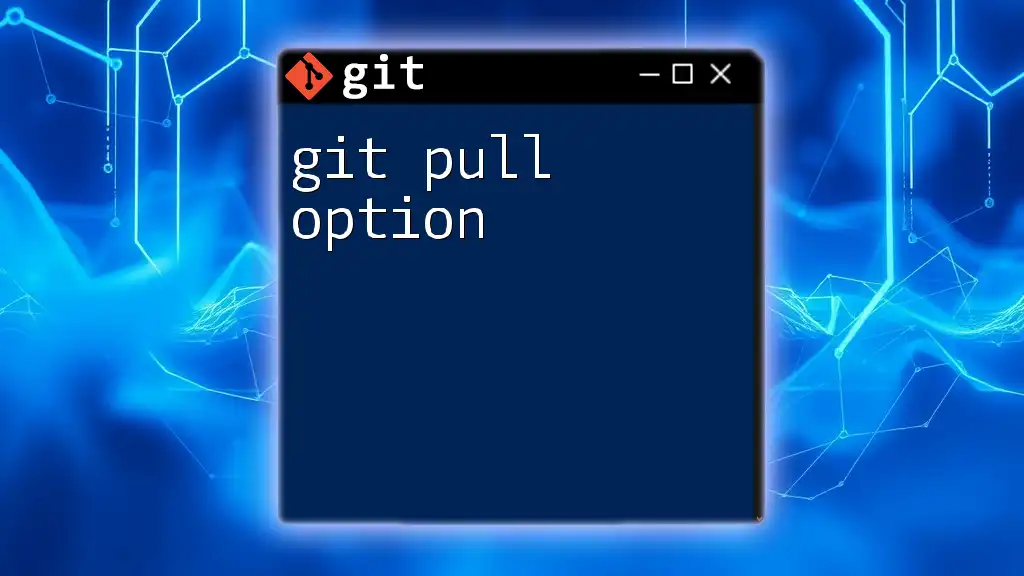
Dealing with Errors During `git push`
Common Errors Explained
Git presents users with various error messages when a push fails, often due to conflicting changes.
- "Updates were rejected because the remote contains work that you do not have locally": This indicates that someone has pushed changes to the remote that you haven't integrated. To resolve this, pull the latest changes first:
git pull origin main
Resolve any conflicts locally, commit the result, then attempt to push again.
- "Failed to push some refs": This error generally relates to trying to push changes that conflict with updates on the remote. As in the previous paragraph, ensure your local repository is updated before pushing.
Resolving Conflicts
Conflicts can be a common hurdle when multiple developers are working on the same files. To maintain harmony within the team, always resolve conflicts locally before attempting to push your changes. Using tools like `git merge`, `git rebase`, or even graphical interfaces can help streamline the resolution process.
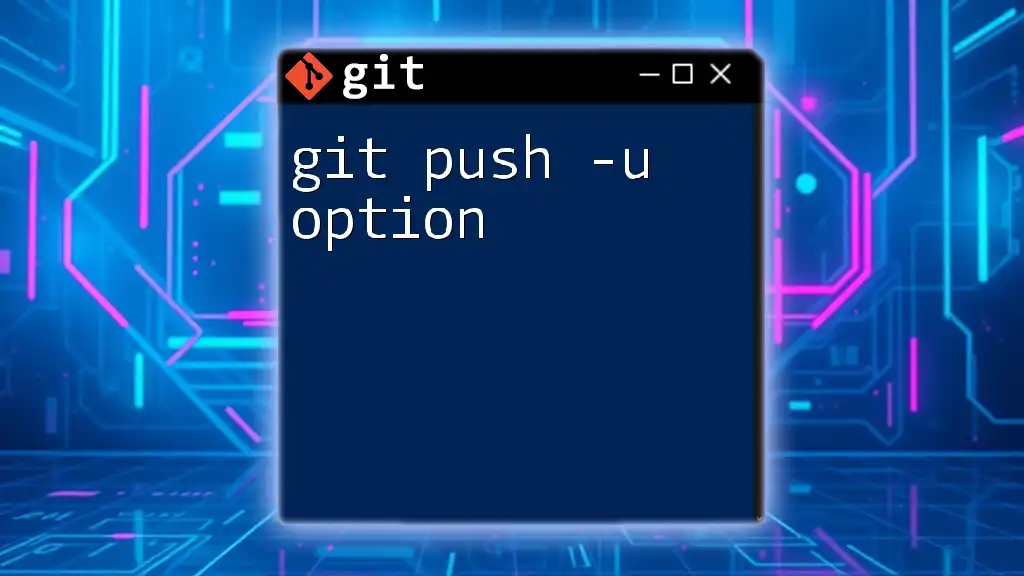
Best Practices for Using `git push`
Commit Often Before Pushing
Aim to commit your work frequently with meaningful commit messages. This practice maintains a clean project history and makes it easier for team members to understand changes you are pushing to the remote.
Reviewing Changes Before Pushing
Before executing a push command, use:
git status
and
git diff
These commands help you ensure that only intended changes are sent to the remote repository, which minimizes the risk of accidental pushes that could disrupt the workflow.
Creating a Consistent Branching Model
Having a structured branching model helps your team understand where to push changes and where to look for features or fixes. Models like Git Flow or Trunk-Based Development encourage clarity in collaboration and maintainability.
Testing Changes Locally
Always test your changes locally before pushing to the remote. This can prevent issues that may arise from untested code affecting other team members. Use testing frameworks or write unit tests to verify new features or modifications.
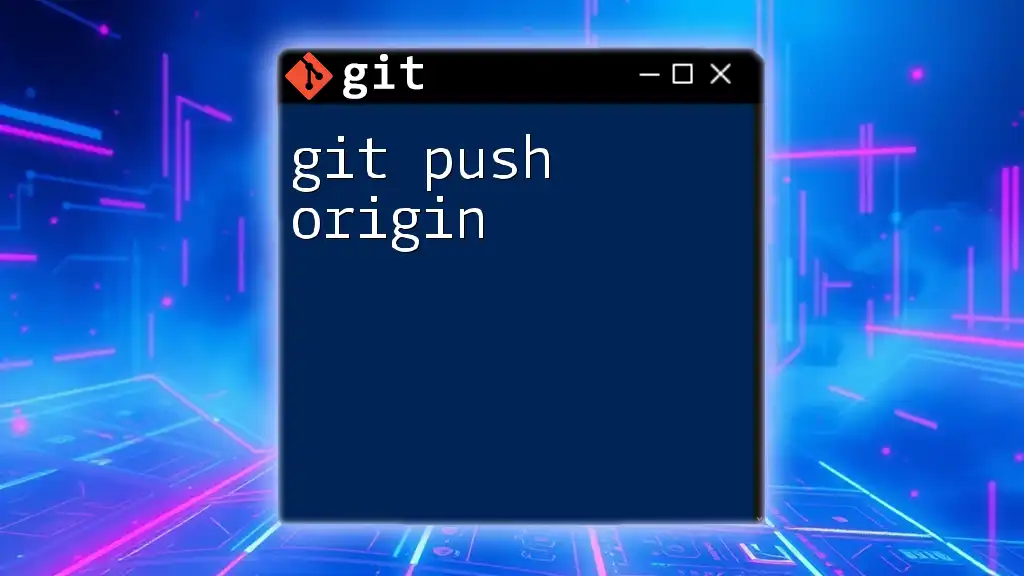
Conclusion
Understanding the various `git push` options is essential for effective version control using Git. The ability to push changes reliably not only ensures your own work is saved but also fosters collaboration with your team. By adopting best practices and leveraging `git push` options properly, you will enhance both your productivity and the quality of your team's codebase.
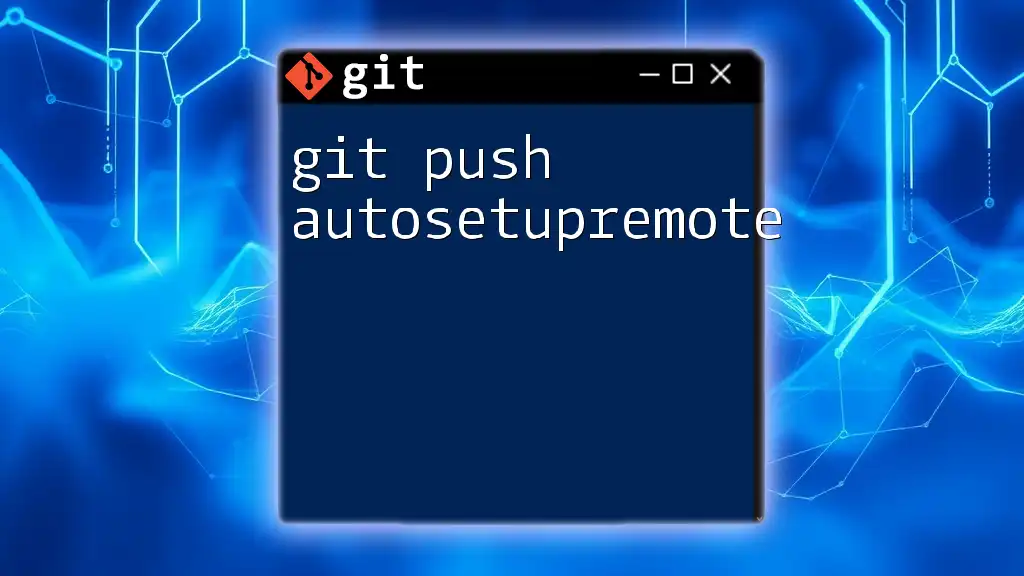
Additional Resources
For further reading, consider exploring the official Git documentation, online tutorials, or community forums and resources where the Git user community shares insights on advanced usage and troubleshooting techniques.
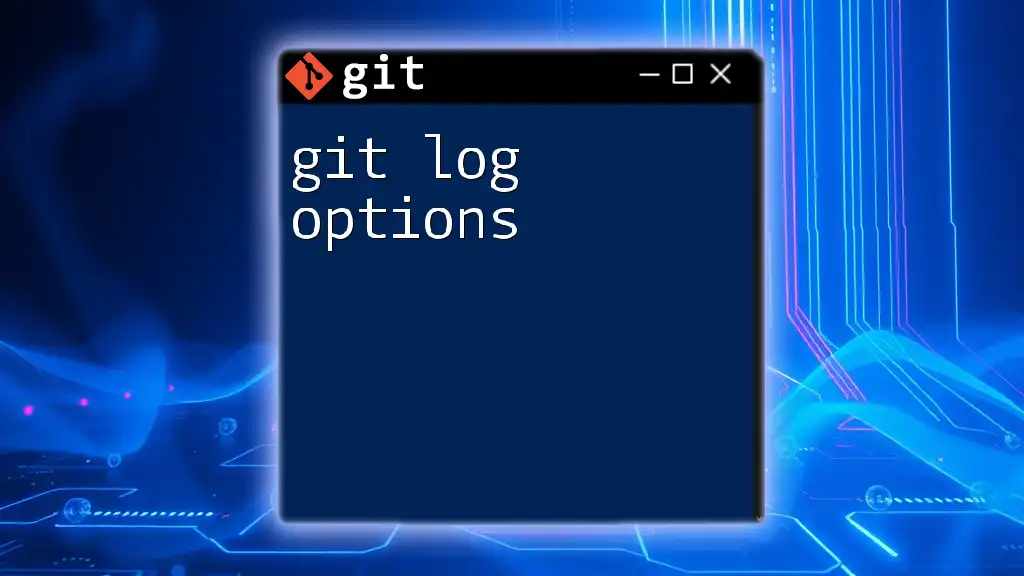
Call to Action
Start applying what you've learned about the `git push` option in your projects today! If you're interested in mastering Git, consider exploring our training services for in-depth learning and hands-on practice opportunities.