To push changes to a remote Git repository without using SSH, you can solely rely on HTTPS by specifying the remote URL and authenticating using your GitHub username and personal access token.
git remote set-url origin https://<username>:<token>@github.com/<username>/<repository>.git
git push origin main
Understanding Git Push
What is Git Push?
The git push command is a fundamental component of version control with Git, allowing developers to upload their local repository changes to a remote repository. Unlike git commit, which captures changes in your local repository, git push effectively updates a remote repository with your local changes, making them accessible to other collaborators.
Importance of Pushing Changes
Pushing changes to a remote repository is crucial for a few reasons:
- Collaboration: Multiple team members can contribute and access the latest updates in a project.
- Version Control: Ensures that the history of changes is maintained, providing a clear audit trail and facilitating rollback if needed.
- Continuous Integration: Allows automated systems to deploy or test the latest changes, ensuring software quality.
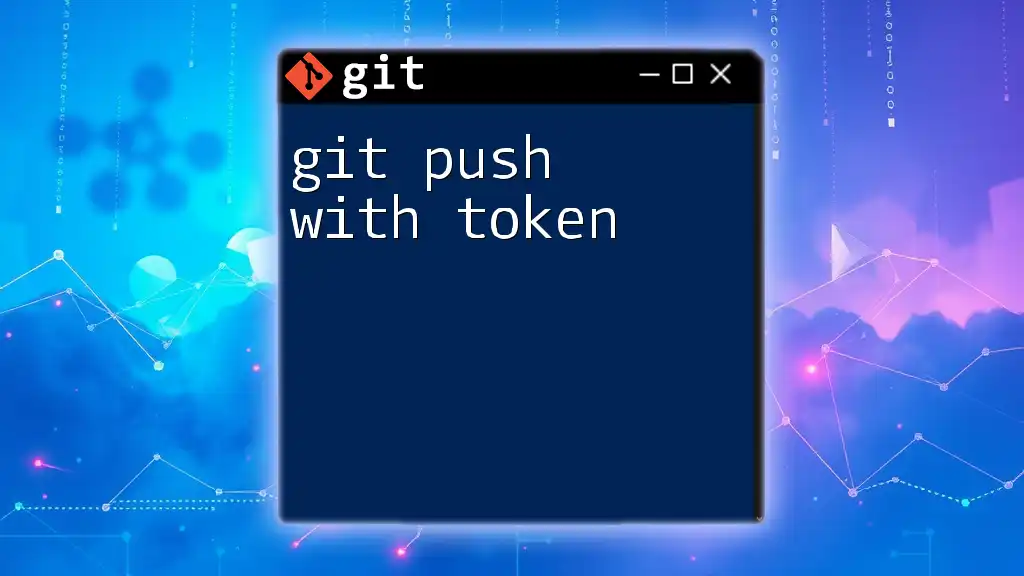
Why Use HTTPS Instead of SSH?
Benefits of Using HTTPS
Using HTTPS to push changes instead of SSH provides several advantages:
- Simplicity: Setting up HTTPS requires minimal configuration—there's no need to generate and manage SSH keys.
- Compatibility: HTTPS is more likely to work in restrictive network environments, such as enterprise firewalls, which may block SSH traffic.
- Accessibility: New users or those unfamiliar with SSH can quickly get started without delving into complex setups.
Drawbacks of SSH
While SSH is secure, it comes with its own challenges:
- Complex Setup: Users must understand key generation and management, which can be daunting for beginners.
- Key Issues: Problems with SSH keys, like permissions and configuration errors, can result in access issues.
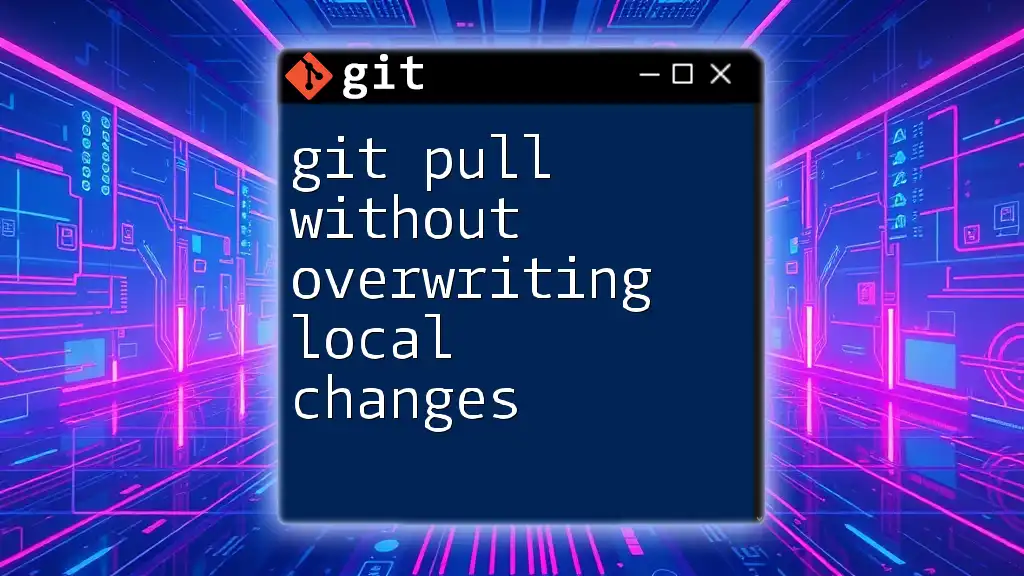
Setting Up Your Repository for HTTPS
Creating a New Repository on GitHub (or similar platform)
To set up a new repository, follow these steps:
- Navigate to GitHub (or your chosen platform).
- Click on the "+" icon to create a new repository.
- Enter the repository name, description, and choose visibility (public or private).
Once your repository is created, initialize it locally with:
git init your-repository-name
git remote add origin https://github.com/yourusername/your-repository-name.git
This sets the remote URL for your local repository.
Changing Remote URL to HTTPS
If you already have a repository set up with SSH and want to switch to HTTPS, you can do so easily. Use this command to update the remote URL:
git remote set-url origin https://github.com/yourusername/your-repository-name.git
By doing this, you ensure that all future pushes and pulls are conducted over HTTPS.
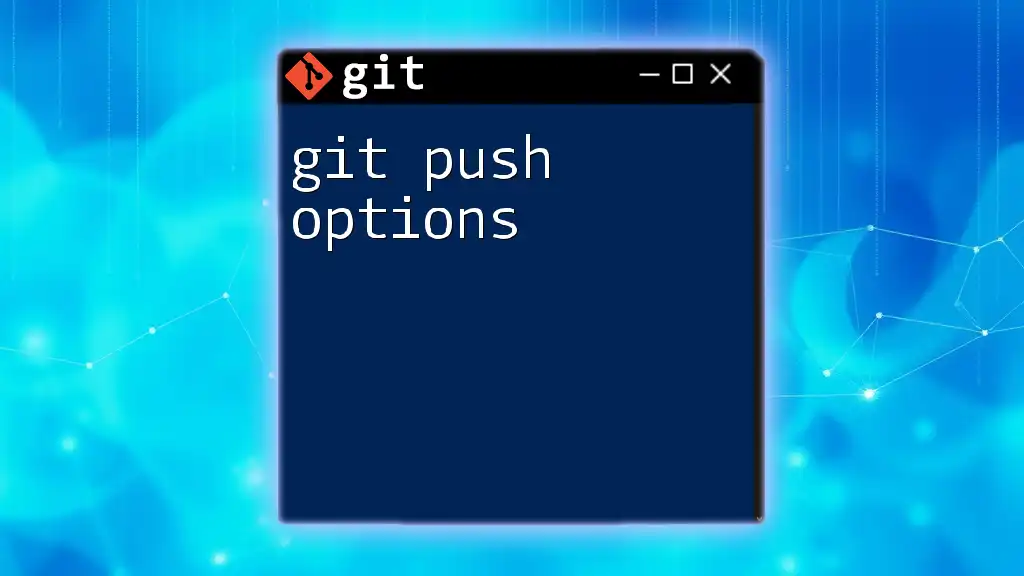
Authenticating with HTTPS
Using Username and Password
When using HTTPS, Git will prompt you to enter your username and password each time you push changes. It's important to use a strong password to maintain the security of your account.
Using Personal Access Tokens
With recent changes in security measures, many platforms now recommend using personal access tokens instead of passwords. These tokens allow you to authenticate more securely. Here’s how to generate a token on GitHub:
- Go to your GitHub settings.
- Navigate to "Developer settings" and then "Personal access tokens."
- Click on "Generate new token," set the necessary permissions, and generate the token.
Once you have your token, authenticate your pushes like this:
git push https://<access_token>@github.com/yourusername/your-repository-name.git
This way, your token replaces your password in the URL, providing a secure method to push changes.
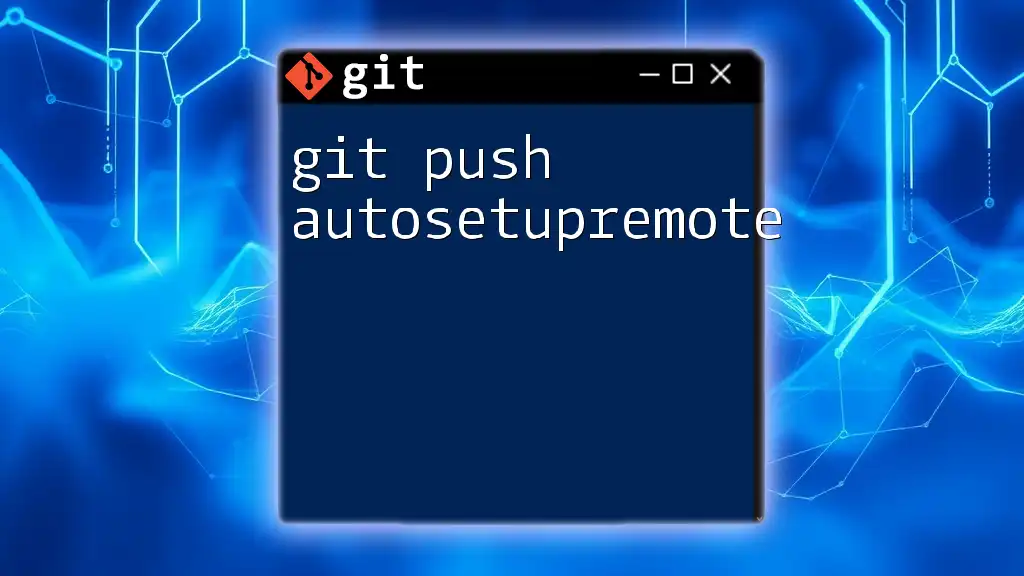
Performing a Git Push Using HTTPS
Step-by-Step Guide to Pushing Changes
To push your changes to the remote repository, follow these steps:
- Stage your changes using the following command:
git add .
- Commit your changes with a descriptive message:
git commit -m "Your commit message"
- Push your changes to the remote repository on the desired branch (e.g., main):
git push origin main
This command syncs your local changes with the remote repository, making them available for others to see and utilize.
Troubleshooting Push Issues
Common Errors
When pushing with HTTPS, some common errors may occur:
- Authentication Failed: This often happens due to incorrect username/password or token issues. Double-check your credentials and ensure that they have the necessary permissions.
- Repository Not Found: This could indicate an incorrect remote URL. Use `git remote -v` to verify the remote repository's URL and make sure it matches your platform.
Fixing Push Problems
If you encounter issues, consider the following steps:
- Check Credentials: Ensure your username and personal access token are entered correctly.
- Update Your Git Configuration: If necessary, reconfigure your remote URL or authentication methods.
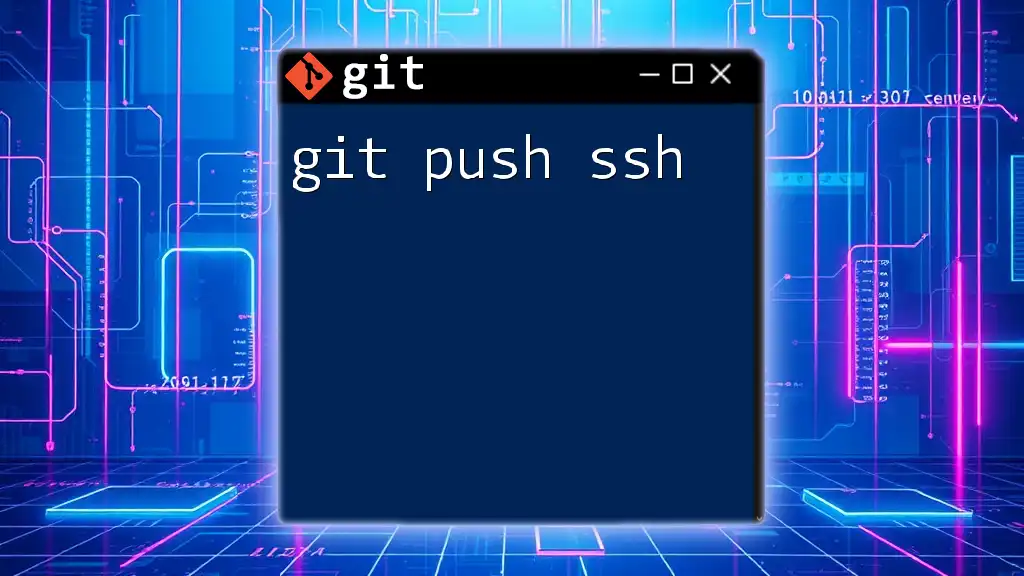
Best Practices for Git Push Using HTTPS
Managing Credentials Securely
To enhance security when working with HTTPS, consider using credential helpers. This feature can cache your credentials, so you don't have to enter them every time.
git config --global credential.helper cache
This command instructs Git to remember your credentials in memory for use in future commands, streamlining the workflow.
Regularly Updating Tokens
For improved security, it’s wise to cycle through your personal access tokens periodically. Revoking old tokens and generating new ones helps prevent unauthorized access and keeps your account secure.
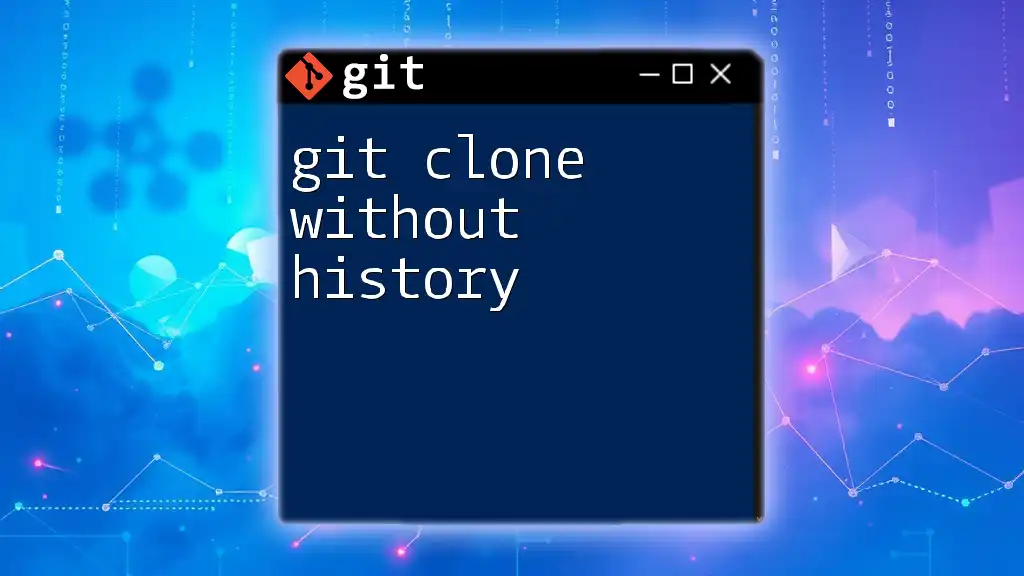
Conclusion
Understanding how to use git push without SSH is consistently valuable for Git users, especially those working in environments where SSH may not be feasible. By utilizing HTTPS, you ensure easy access while maintaining robust security practices.
Experiment with these commands and features, and don't hesitate to explore the vast capabilities of Git. As you become more comfortable, you'll find that Git can greatly enhance your collaborative programming efforts.
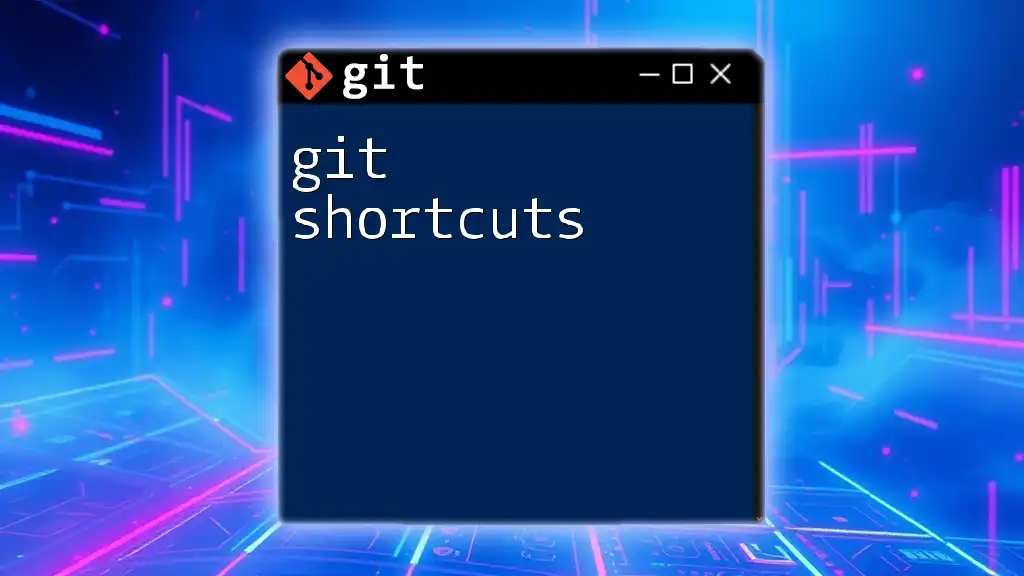
Additional Resources
For further exploration, consult the official Git documentation or refer to various online tutorials and books dedicated to mastering Git.
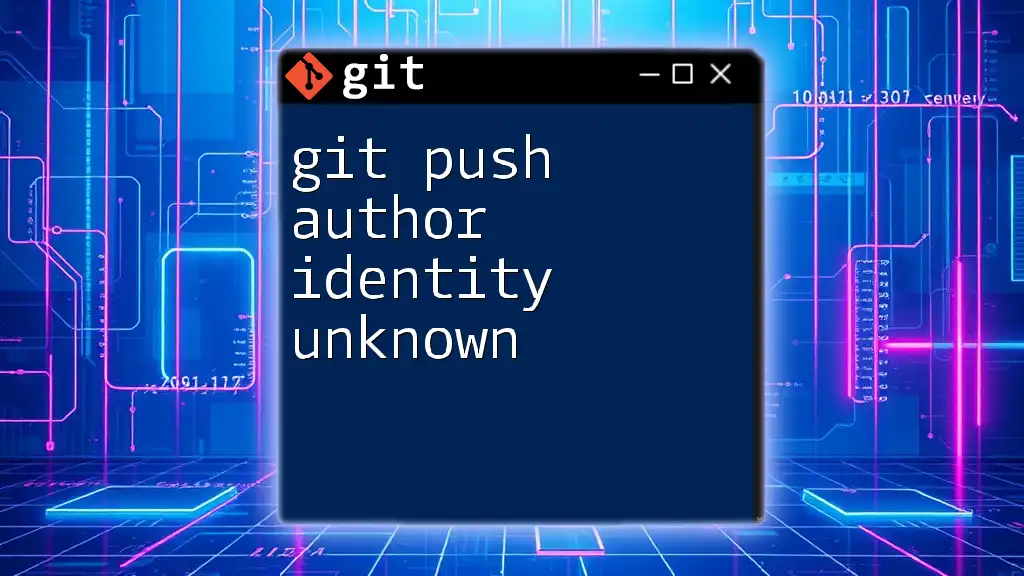
Call to Action
If you found this guide helpful, consider signing up for more quick and concise tutorials on Git commands. We’d love to hear your feedback and answer any questions you may have in the comments below!