The `git push ssh` command securely uploads your local repository changes to a remote repository over SSH, ensuring that your data is encrypted during transmission.
Here's a code snippet demonstrating a typical command:
git push ssh://username@host:/path/to/repo.git master
Understanding `git push`
What Does `git push` Do?
The `git push` command is an essential part of the Git workflow, allowing you to transfer your local repository changes to a remote repository. When you commit changes locally, those changes are saved only in your local repository. Pushing is the process that updates the remote repository with those local changes, making them visible and accessible to other collaborators. This synchronization fosters collaboration and ensures that everyone is working with the most recent version of the code.
Common Use Cases for `git push`
- Collaborating with Teams: When working in development teams, pushing changes is crucial. It allows team members to see updates, provide feedback, and build on each other’s work.
- Deploying Code: Many teams set up their repositories in such a way that when code is pushed to a specific branch, automated processes deploy that code to production.
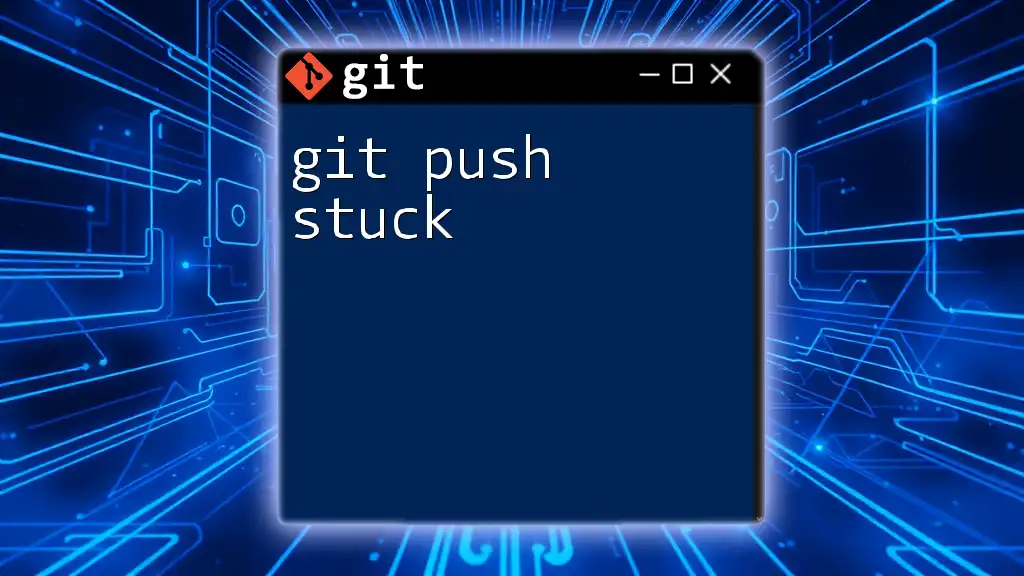
Setting Up SSH for Git
Generating SSH Keys
Using SSH keys to authenticate with your remote repository is one of the best practices in Git. It provides a more secure and efficient way to transmit commands than using HTTPS, as it does not require you to enter your password every time you push changes.
To generate your SSH key, open your terminal and run:
ssh-keygen -t rsa -b 4096 -C "your_email@example.com"
In this command:
- `-t rsa` specifies the type of key to create, which is RSA in this case.
- `-b 4096` specifies the number of bits in the key. A value of 4096 ensures a strong key.
- `-C` allows you to add a comment (commonly your email) to identify the key.
Adding Your SSH Key to the SSH Agent
After generating the SSH key, you should add it to the SSH agent, which manages your SSH keys for secure, passwordless connections.
First, start the SSH agent:
eval "$(ssh-agent -s)"
Next, add your SSH key:
ssh-add ~/.ssh/id_rsa
Here, `~/.ssh/id_rsa` is the default path where your SSH key is stored. Once added, the key can be used to authenticate your Git operations without repeatedly entering your credentials.
Adding Your SSH Key to Your Git Hosting Service
Now that your SSH key is set up, it’s time to add it to your version control service (like GitHub, GitLab, or Bitbucket). Begin by copying your public key to the clipboard:
cat ~/.ssh/id_rsa.pub
The output will be your public key starting with `ssh-rsa`.
Next, navigate to your account settings on the hosting service and find the section for SSH keys. Here, paste your public key. This step authorizes your local machine to communicate securely with your remote Git repository.
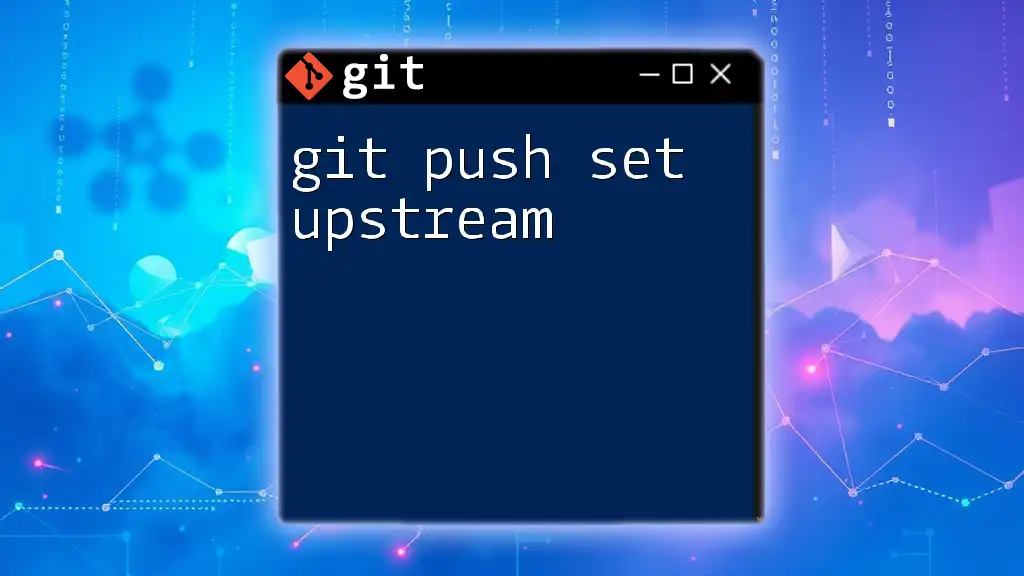
Using `git push` with SSH
Basic Syntax of `git push`
The standard syntax for using the `git push` command is:
git push [remote] [branch]
- remote: This typically signifies the name of the remote repository, with `origin` being the default.
- branch: The branch you want to push. If omitted, Git will push the current branch to its corresponding upstream branch.
Pushing Changes to a Remote Repository
After making changes in your local repository, commit these changes:
git commit -m "Your commit message"
Then push your changes to the remote repository like this:
git push origin main
In this command:
- `origin` refers to the remote repository's name.
- `main` is the branch you are pushing to. Be sure to replace this with the correct branch name if necessary.
Handling Errors During `git push`
Errors are a part of working with Git, especially during the pushing process. Common errors include authentication issues or "no upstream branch" messages.
To troubleshoot:
- Verify that you have added the correct SSH key to your Git hosting service.
- Ensure that the repository URL is using the SSH format, which looks like `git@github.com:username/repo.git`.
- If you encounter "failed to push some refs" errors, consider running `git pull` first to merge recent changes from the remote repository.
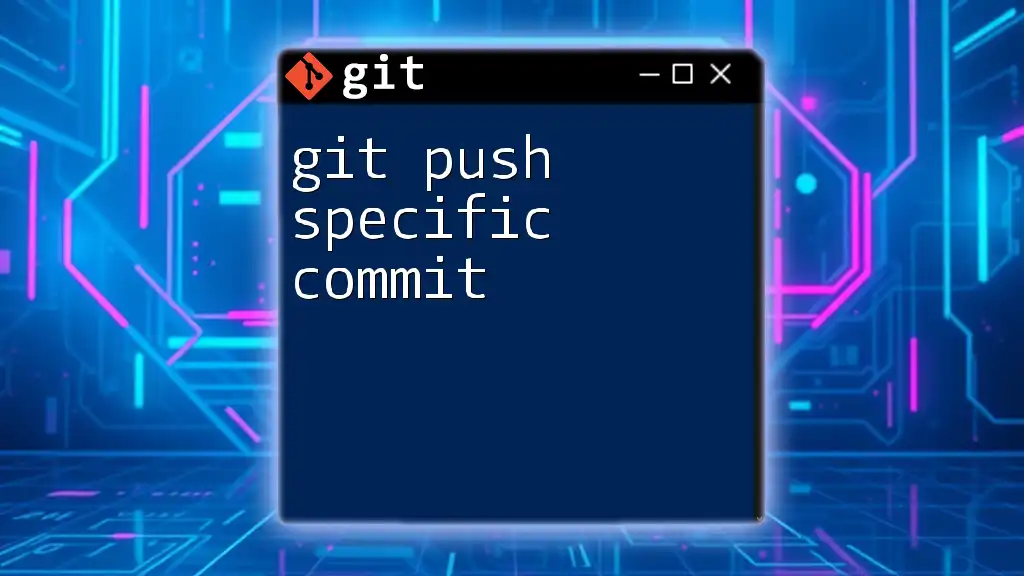
Advanced `git push` Usage
Force Pushing
Force pushing can be a double-edged sword. While it lets you overwrite changes in a remote repository, it can disrupt your team’s workflow. Use it cautiously, only when you're sure that you want to replace the remote branch with your local version. The command looks like:
git push origin main --force
Important: Always communicate with your team before force pushing, as this action can lead to lost commits if others have made changes to the same branch.
Pushing Tags
Tags are useful for marking specific points in history as important, like version releases. To create and push a tag, follow these steps:
git tag v1.0
git push origin v1.0
The first command creates a tag called `v1.0`, while the second command pushes that tag to the `origin` repository. Tags serve as a reference for versions, making it easier to track specific code milestones.
Setting Upstream Branches
When working with a new branch, you may want to set an upstream branch. This allows you to push without specifying the remote and branch every time. Use:
git push -u origin feature-branch
The `-u` option sets the branch you are pushing as its upstream. Now, you can simply use `git push` in future commands without additional parameters.
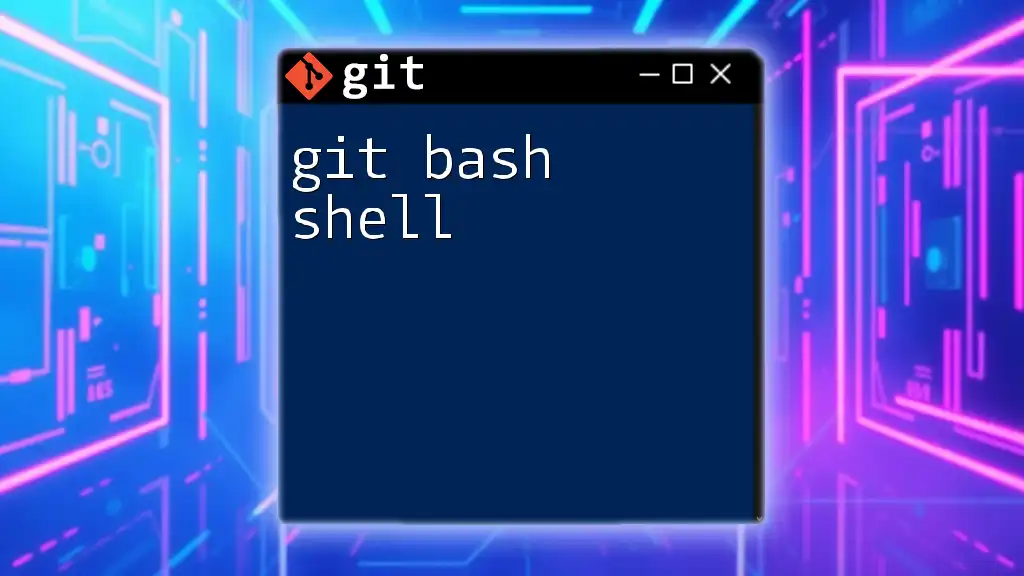
Conclusion
In summary, using `git push ssh` enhances your productivity with Git by providing a secure and efficient method to transfer updates to remote repositories. By understanding how to properly set up SSH keys and employing the `git push` command effectively, you can streamline your workflow and collaborate seamlessly with your team.
As you become more comfortable with `git push` and its various features, consider delving deeper into advanced Git commands to further optimize your development process.
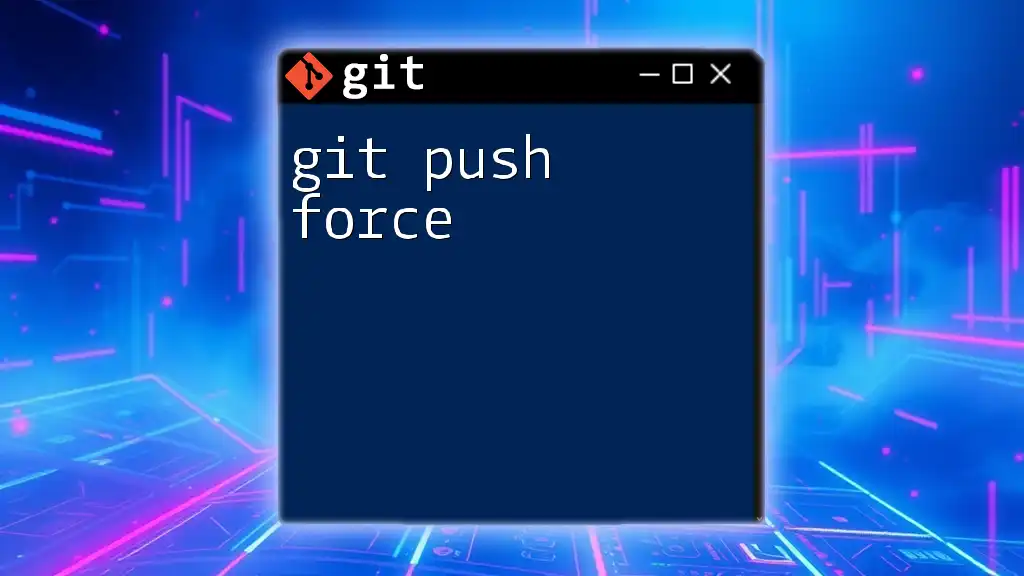
FAQs
Common Questions About `git push ssh`
-
Why should I use SSH over HTTPS?
SSH offers enhanced security and convenience, as it does not require you to enter your credentials each time you interact with the remote repository. -
How can I check if my SSH key is set up correctly?
Run `ssh -T git@github.com` (or your respective service) in your terminal. If set up correctly, you will receive a success message indicating that authentication is successful.