When a `git push` gets stuck, it may be due to network issues or conflicting changes, and you can troubleshoot it by checking your connection or by using the following command to forcefully push your changes.
git push origin branch-name --force
What is `git push`?
`git push` is a command that allows you to upload your local repository changes to a remote repository. This is essential for collaborating with others, as it ensures that everyone has access to the latest version of the project. When you execute `git push`, you are essentially synchronizing your work with a central repository, which is often hosted on platforms like GitHub, GitLab, or Bitbucket.
Understanding how `git push` interacts with remote repositories is crucial. When you push, Git takes your commits from your local branch and applies them to the corresponding branch in the remote repository, making your changes available to others. However, a failure during this process—often described as a "git push stuck"—can be frustrating.
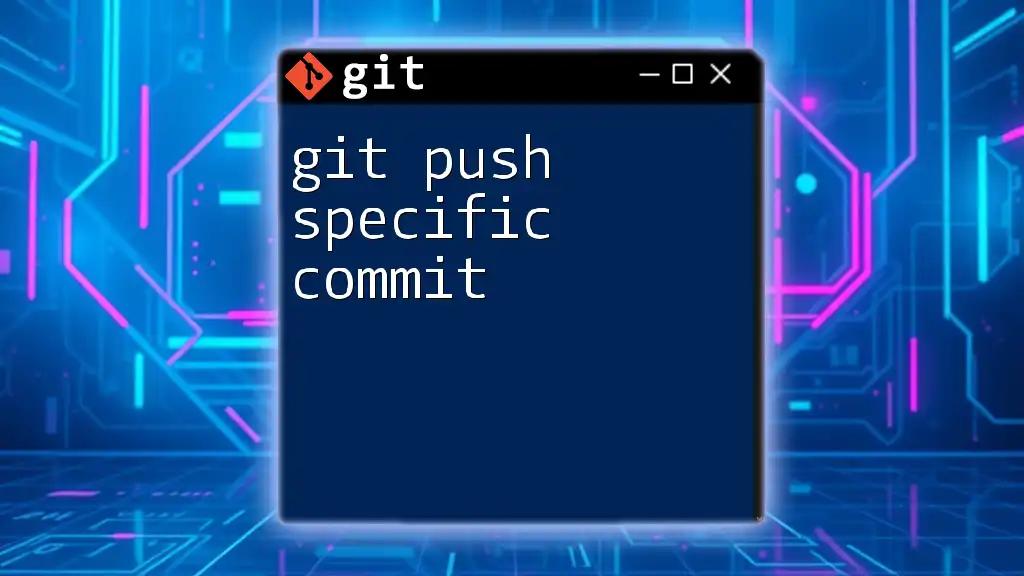
When Does a Push Get Stuck?
Several scenarios can lead to a stuck push. Most commonly, issues arise from:
- Network problems: Interruption in your internet connection can cause the push to stall.
- Large files: Pushing large files or binaries can exceed timeout limitations or lead to network bottlenecks.
- Permission errors: Changes in repository access settings or lack of proper credentials can prevent a successful push.
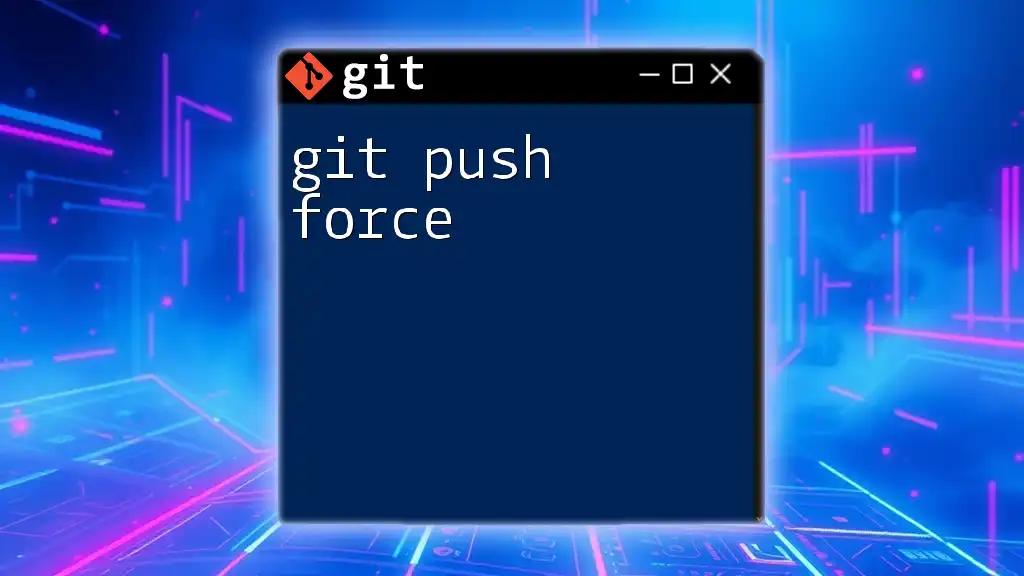
Common Causes of a Stuck `git push`
Network Issues
When your network connection is unstable or interrupted, it can cause your push to become stuck. Signs of network problems during a push include:
- Slow performance in your terminal or command line interface.
- Error messages related to connectivity, such as timeouts or refusal messages.
Troubleshooting Steps
To diagnose and fix network-related issues, consider taking the following steps:
- Check Your Internet Connection: Ensure that you are connected to the internet and that there are no ongoing disruptions.
- Use Diagnostic Commands: Tools like `ping` can help check the status of your connection. For example:
ping google.com
If you identify that network issues are indeed the problem, consider switching to a different network or using a VPN as a backup.
Large Files and Repositories
Large files can hinder your push, particularly if they exceed size limits imposed by your hosting provider. Pushing bulky files can lead to timeout errors or a complete inability to push altogether.
Solutions for Large Files
If you're regularly working with large files, consider using Git LFS (Large File Storage). Git LFS is designed to handle large files more efficiently. To set it up, follow these steps:
-
Install Git LFS:
git lfs install
-
Track Your Large Files: For example, if you want to track `.largefileextension` files, you would execute:
git lfs track "*.largefileextension"
-
Add Your Changes: After setting up LFS, ensure you update your `.gitattributes` file:
git add .gitattributes
By implementing Git LFS, you can effectively manage large files and minimize the likelihood of encountering a stuck push due to file size issues.
Permission Errors
Another common reason for a git push stuck situation is permission errors. Understanding and troubleshooting permission-related issues is critical when dealing with Git repositories.
How to Diagnose and Fix Permissions
When pushing to a remote repository, make sure you have the proper access rights. Common causes of permission issues include:
- Changes in user access control settings on Git hosting platforms.
- Misconfigured credentials on your local machine.
To troubleshoot:
-
Check Your Remote Repository Access: Confirm that you have write permissions for the repository to which you're trying to push.
-
Update Your Credentials: Use the `git config` commands to verify your Git username and email. For example:
git config --global user.name "Your Name" git config --global user.email "your.email@example.com"
-
Re-authenticate with Your Remote Provider: Depending on your setup, you may need to re-enter your credentials. For GitHub, for instance:
git config --global credential.helper cache
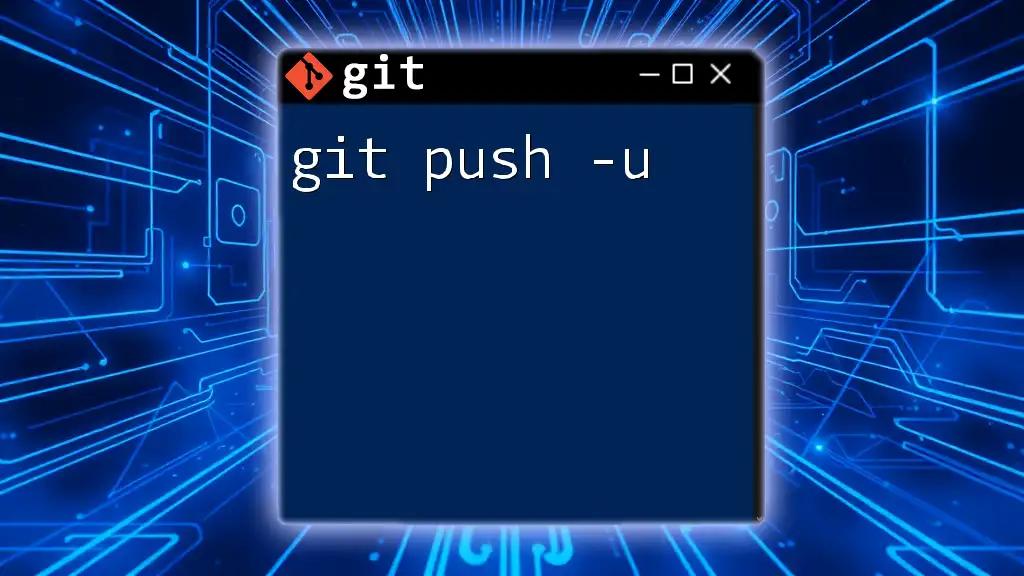
How to Resolve a Stuck `git push`
Checking Push Status
When faced with a stuck push, first check the status of your local repository. Use the following commands to understand what is happening:
git status
And to review the commit history:
git log
These commands provide insight into any pending changes or commits that may be causing the hang-up during the push.
Canceling a Stuck Push
If you realize that your push is truly stuck, you can safely abort the operation. This is typically done using keyboard shortcuts specific to your terminal or command interface, such as `Ctrl + C`. However, if needed, you can also close the terminal window.
Retrying the Push
Once you've addressed any underlying issues, you can attempt to push your changes again. Before doing so, ensure that your local changes are ready for synchronization. Once verified, simply execute:
git push origin main
By regularly checking for updates and verifying local changes, you can minimize the chances of encountering a stuck push.
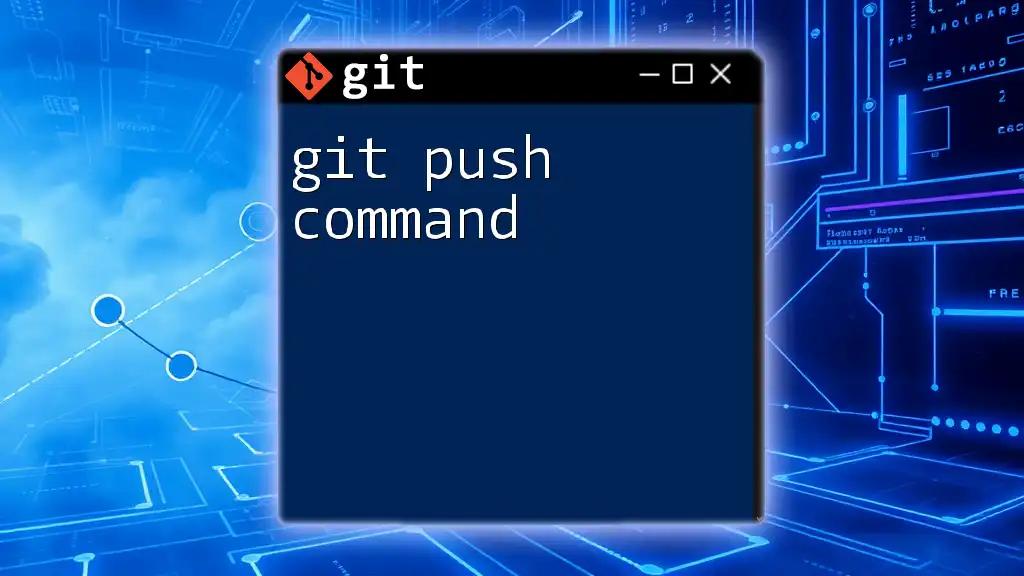
Tools and Resources for Managing Git Pushes
Useful Git Commands
Familiarize yourself with these essential commands to help troubleshoot and manage your Git operations:
- `git remote -v`: Displays the remote repository URLs.
- `git fetch`: Updates your local copy of remote branches.
- `git push --force-with-lease`: Allows you to force a push while ensuring you don't overwrite someone else's work.
Recommended Git GUI Applications
For those who prefer graphical user interfaces (GUIs), consider using applications such as:
- GitKraken
- Sourcetree
- GitHub Desktop
These tools simplify many Git operations, reducing the complexity that may lead to a stuck push.
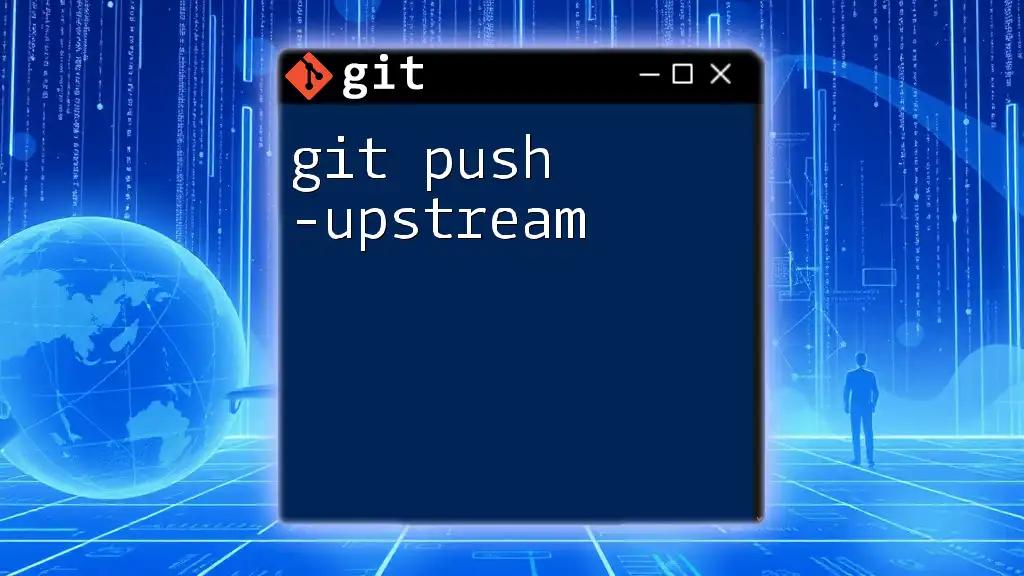
Preventing Future Push Issues
Best Practices for Using Git
To avoid getting stuck during future pushes, adhere to these best practices:
- Make frequent commits and push regularly to keep your changes synced.
- Perform checks and balances before pushing (like verifying remote branches).
Regularly Monitoring Repository Health
Regularly monitoring your repository is vital for its overall health. Use GitHub Actions, CI/CD tools, or simple scripts to automate checks and audits of your repository.
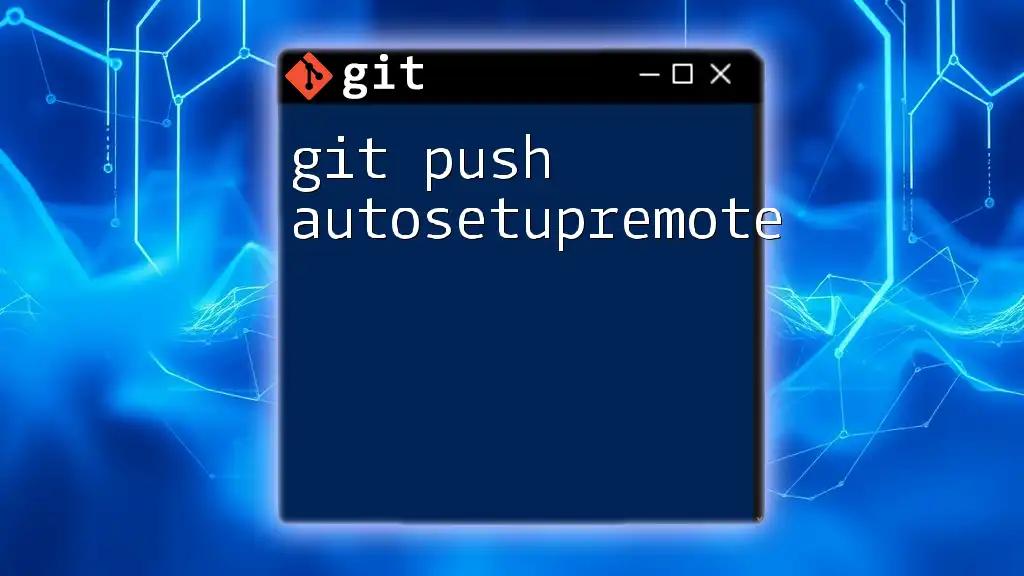
Conclusion
Encountering a "git push stuck" situation can be frustrating, but understanding the underlying causes and how to address them can help streamline your Git operations. By implementing best practices, employing tools like Git LFS for large files, and staying aware of your repository's health, you can ensure that your pushing process runs smoothly. Don’t hesitate to reach out for further assistance, as the Git community is always available to help troubleshoot any challenges you face.
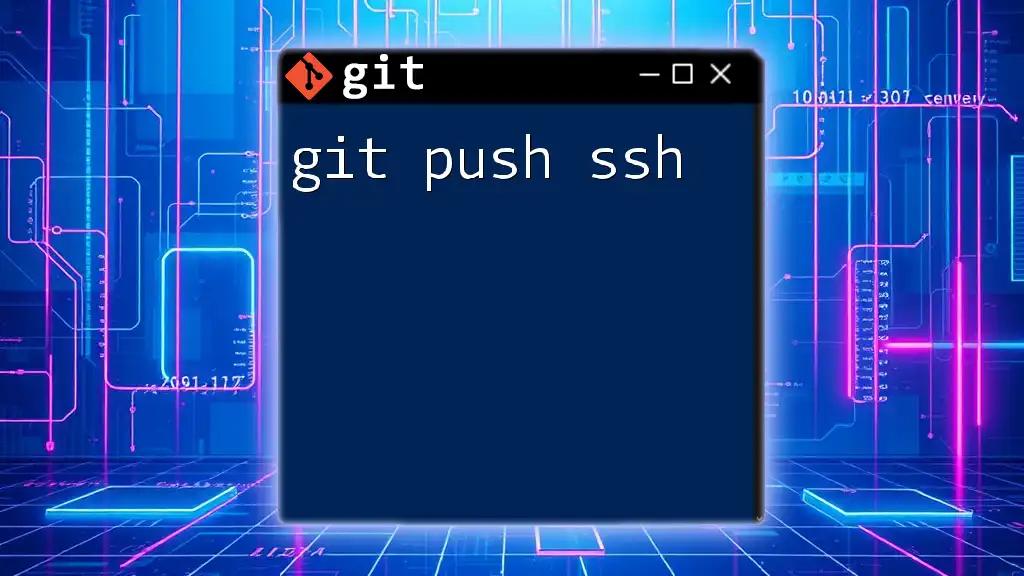
Additional Resources
For those looking to deepen their understanding, several resources are available:
- Official [Git documentation](https://git-scm.com/doc)
- Online tutorials and courses on platforms like Udemy or Coursera
- Community forums such as Stack Overflow for real-time help from fellow developers