When you encounter a "git push stuck after writing objects" issue, it typically indicates that the process is hanging during the data transfer, often due to network issues or large files; you can resolve it by checking your internet connection or using the following command to configure Git to use a more aggressive transfer strategy.
Here's a code snippet to increase the push timeout:
git config --global http.postBuffer 524288000
Understanding Git Push
What is `git push`?
The `git push` command is essential in the Git workflow, allowing users to transfer commits from a local repository to a remote repository. It not only uploads changes but also ensures that the version control remains synchronized between local and remote sources. When you issue a `git push`, you're essentially saying, "I want to share my changes with others in this repository." The command works hand-in-hand with other Git commands, such as `git pull`, to manage and synchronize development work efficiently.
Common Errors During Git Push
Just as powerful as it is useful, `git push` comes with its set of challenges. Various errors can interrupt your workflow, causing frustration—one of the most common being when it gets stuck after writing objects. Understanding why this happens will pave the way for quick resolutions.
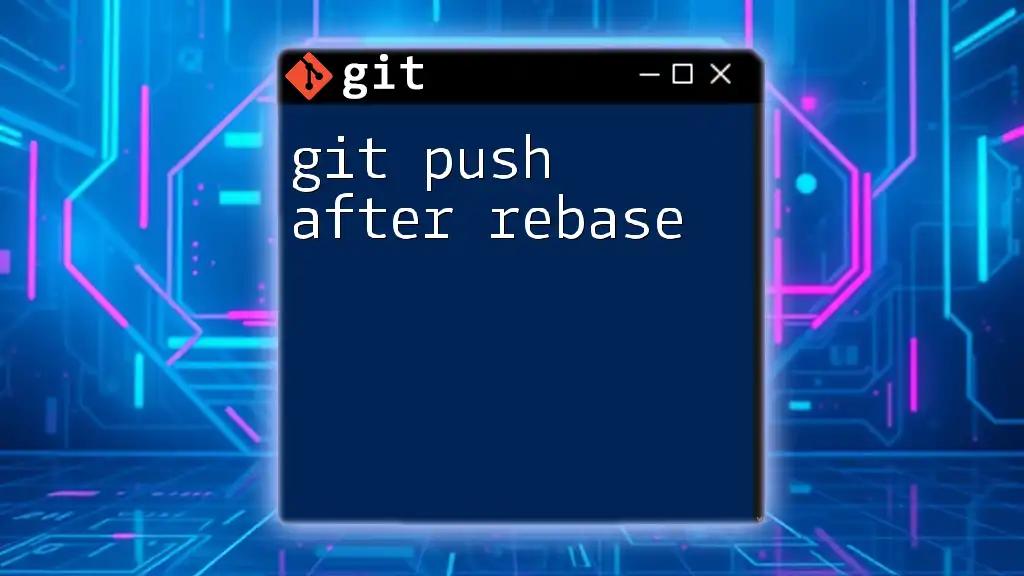
Diagnosing the "Stuck After Writing Objects" Error
Symptoms
When a `git push` freezes, users may notice that the command line hangs indefinitely after displaying a message indicating that objects are being written. For instance, you might see output similar to this:
Counting objects: 100% (50/50), done.
Writing objects: 100% (40/40), 35.78 KiB, done.
At this point, it seems that the process is completed; however, it doesn’t finalize, leaving you stuck.
Potential Causes
Network Issues
Network connectivity problems are often the first culprits behind a stuck push. A weak or intermittent internet connection can lead to timeouts or lost packets, hence preventing your local changes from reaching the remote repository.
To troubleshoot network issues:
- Test your connectivity by running a ping check or using online services to assess your internet speed.
- If on Wi-Fi, consider switching to a wired connection to eliminate drops and improve consistency.
Large Object Sizes
Another significant reason could be large files that exceed limitations set by the server or even your local system. If you're pushing large multimedia files, they could lead to delays or timeouts.
To identify large files in your repository, you can use the following command:
git rev-list --objects --all | git cat-file --batch-check='%(objecttype) %(objectname) %(rest)' | grep '^blob' | awk '{ print $3 " " $4 }' | sort -n -r | head -n 10
Review the output to check for any unusually large files that may be causing the issue.
Remote Repository Limitations
Remote server configurations or limitations can also lead to this situation. Instances such as exceeding the server’s file size limit or lack of configuration pertaining to data upload can hinder completing a `git push`.
Ensure that you have correct permissions for writing to the remote repository, as sometimes read-only settings can cause this error to arise.
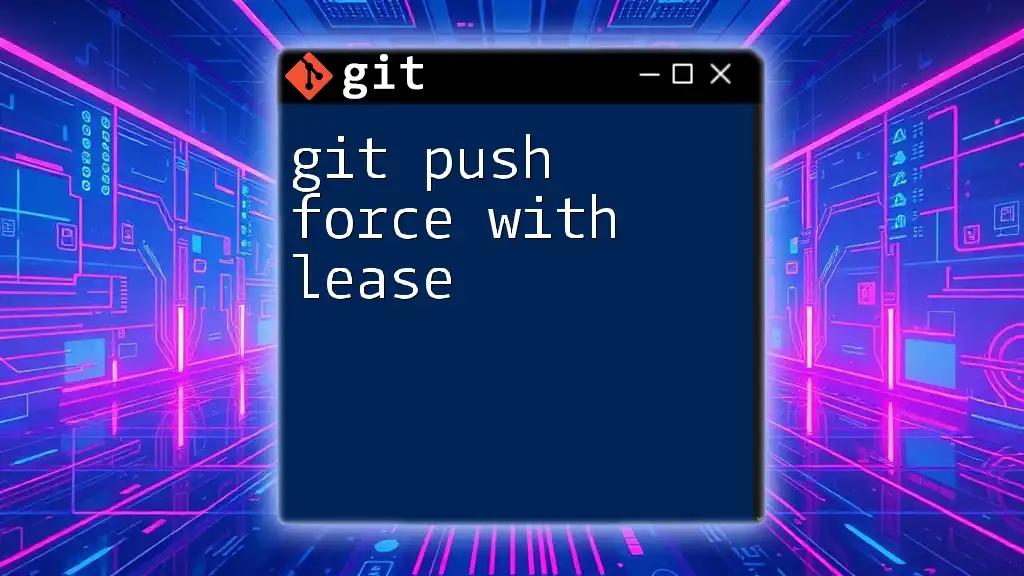
Resolving the Push Issue
General Troubleshooting Steps
Check Your Internet Connection: Before taking any further steps, ensure that your internet connection is stable. Use online services or ping commands to check for latency issues.
Review Large Files and History: If large files are the problem, consider utilizing Git LFS (Large File Storage) to manage them efficiently. Here’s how to migrate large files to Git LFS:
git lfs track "*.large_file_extension"
git add .gitattributes
git add <large_file>
git commit -m "Migrated large file to Git LFS"
This will offload the management of large files and reduce the chances of your push stalling due to file sizes.
Increase Git Buffer Size: Sometimes, the configurations set for Git's buffer size may be too restrictive. Increasing it allows more data to be sent in each batch:
git config http.postBuffer 524288000
This command increases the buffer size to 500 MB, which can be useful for large pushes.
Alternative Commands
If you still find that the `git push` command stalls, consider using alternatives such as `git push --force`, but be cautious with this option as it can overwrite changes on the remote repository. Moreover, you might try exploring specific options like:
git push origin <branch_name> --follow-tags
This ensures that your tags and branches are pushed accurately even when the command encounters obstacles.
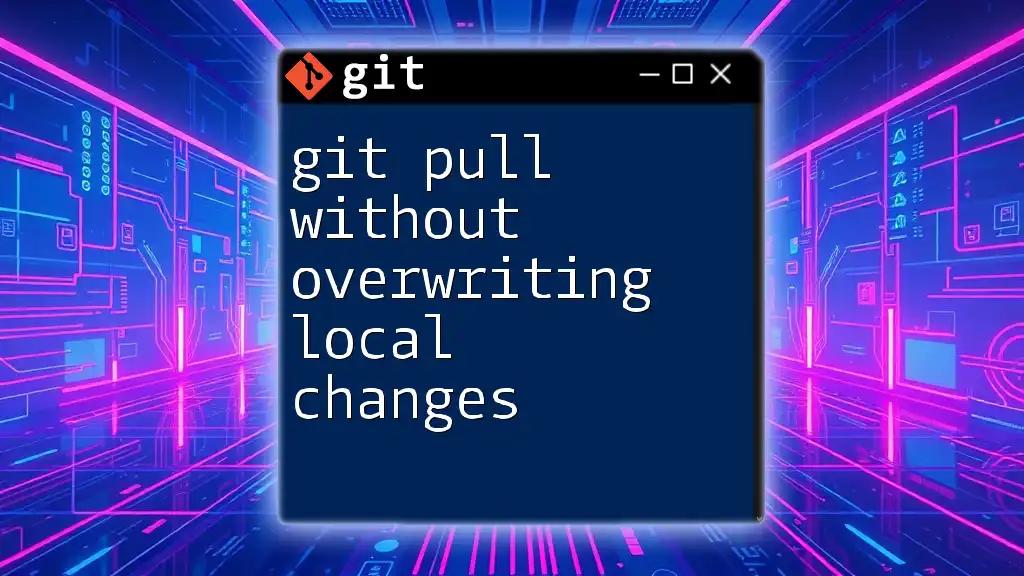
Advanced Solutions
Checking for Locks
Stale locks can hinder your pushing ability as well. If you suspect that a lock file may be in place, you can clear it using:
rm -f .git/index.lock
Removing this file can often solve permission-related issues that arise from temporary locks.
Remote Repository Settings
Don’t overlook the importance of ensuring that your remote repository settings are appropriately configured. Double-check that you possess the required permissions to push updates. If you don’t have write permissions, reach out to your repository administrator.
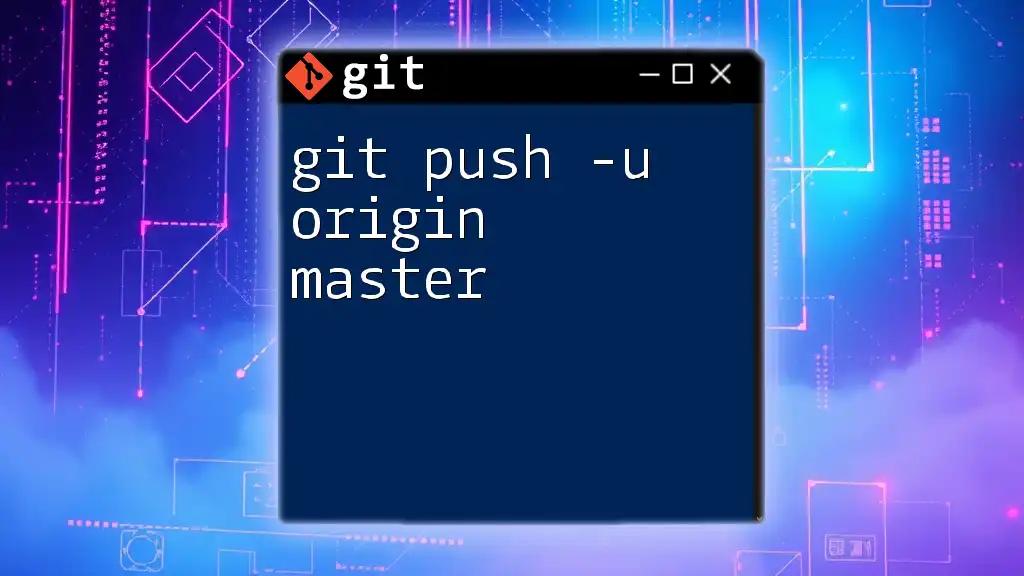
Preventive Measures
Best Practices for Git Usage
To minimize future issues with `git push`, adhere to these best practices:
- Always pull the latest changes from the remote repository before pushing.
- Keep a clean commit history by avoiding unnecessary commits and squashing commits when possible.
- Use branches effectively to segregate features and fixes.
Monitoring Git Performance
Be proactive by monitoring your Git performance, especially if working in large teams or on significant projects. Utilize built-in Git commands or third-party tools that can provide analytics on repository performance and collaboration.

Conclusion
The "git push stuck after writing objects" error can be a frustrating encounter, but understanding its potential causes and resolutions enables you to tackle it effectively. Adhering to best practices and proactively handling large files and network issues will ensure a smoother workflow and enhance your experience with Git. Should you face further challenges or have additional insights, sharing experiences or queries in the comments can foster a supportive community environment.