When you rebase your local branch and want to push those changes to a remote repository, you should use the `git push --force` command to overwrite the remote history with your newly rebased commit history.
git push --force
Understanding Git Rebase
What is Git Rebase?
Git rebase is a powerful feature in Git that allows you to integrate changes from one branch into another. Rather than creating a new merge commit, rebase "rewrites" commit history by applying commits from your current branch on top of another branch (often `master` or `main`). This results in a linear sequence of commits that can simplify your project's history.
Comparing rebase with merge highlights its advantages: while merge preserves the complete history, rebasing offers a cleaner, more understandable log by eliminating unnecessary merge commits. When collaborating in a team, keeping a tidy commit history helps everyone comprehend the project’s evolution more clearly.
Types of Rebase
Interactive Rebase
An interactive rebase allows you to alter commit history by reorganizing, rewriting, or squashing commits. It’s particularly useful for cleaning up your commits before merging them into the main branch.
To initiate an interactive rebase, you can use the following command:
git rebase -i HEAD~n
Here, `n` is the number of commits you want to rebase interactively. When you run this command, a text editor opens, enabling you to choose which commits to edit, squash, or revert.
Automatic Rebase
An automatic rebase, on the other hand, applies all the commits from one branch onto another branch without additional prompts for user input. An example command would be:
git rebase master
This command will apply all changes from the `master` branch onto your current branch, ensuring your work incorporates the latest changes from `master`.
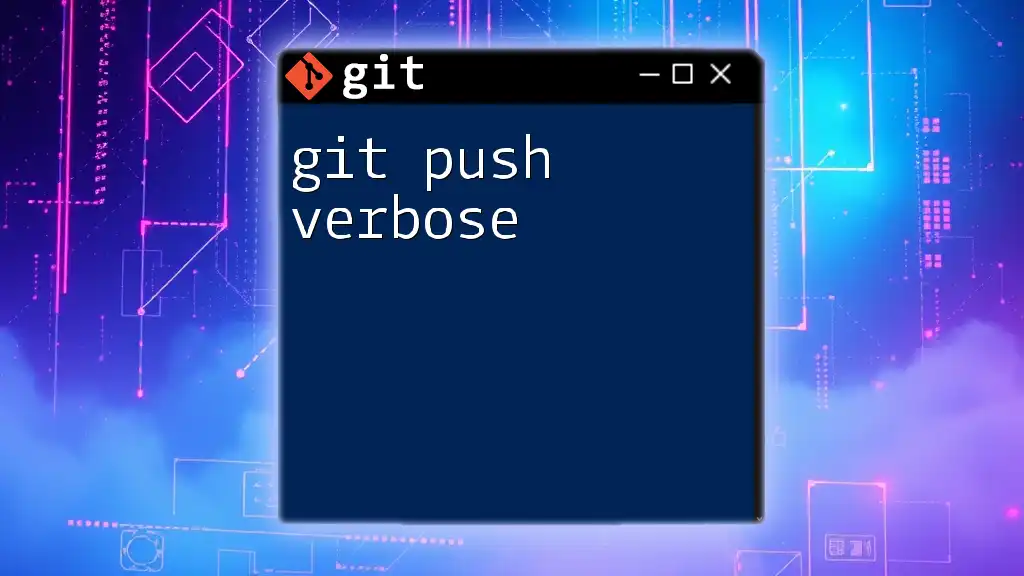
The Workflow of Rebasing
Steps Before Rebasing
Before starting a rebase, it’s essential to ensure that your branch is up to date with the target branch. Begin by fetching the latest changes and pulling them to your local repository:
git fetch origin
git pull origin master
This process ensures you have the latest changes integrated into your local branch before applying your own commits.
Executing a Rebase
Once you’ve confirmed your branch is up to date, you can execute a rebase. For example, if you want to rebase your current branch with `master`, use the following command:
git rebase master
Git will then attempt to apply your commits on top of the latest commit in the `master` branch.
Handling Conflicts During Rebase
During a rebase, conflicts might arise if changes in your commits overlap with changes in the target branch. Git will pause and alert you to resolve these conflicts. You can check the status of the conflicted files with:
git status
After resolving conflicts manually, mark the files as resolved:
git add <resolved-file>
Then, continue the rebase process with:
git rebase --continue
If at any point you feel overwhelmed by conflicts, you have the option to abort the rebase using:
git rebase --abort
This command will restore your branch to its original state before the rebase attempt.
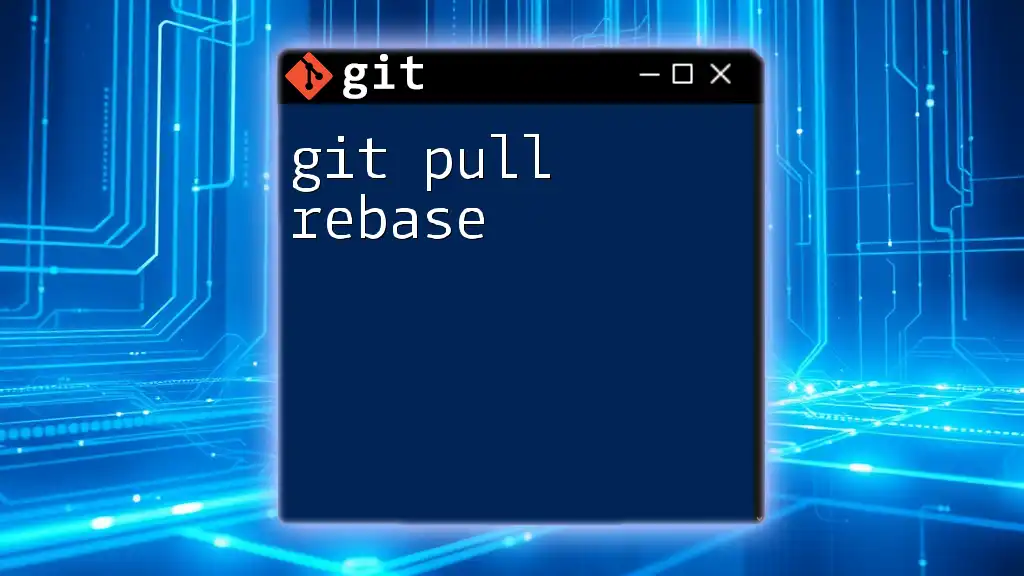
Understanding Git Push
What is Git Push?
After completing your rebase, the next logical step is to push your changes. The `git push` command uploads your local commits to the remote repository, ensuring your changes are shared with others. This becomes crucial after rebasing because Git’s history has been rewritten.
Different Push Options
- Standard Push
The standard `push` command simply updates the remote branch with changes from your local branch. For example, if your branch name is `feature-branch`, you would push using:
git push origin feature-branch
This command syncs your changes to the remote repository, providing access to all collaborators.
- Force Push
If you find that your push is rejected because the remote branch has been updated (e.g., "non-fast-forward"), you may need to use a force push. A standard force push looks like this:
git push --force origin feature-branch
Caution: Force pushing can overwrite changes made by others, potentially leading to data loss. It’s often safer to use `--force-with-lease`, which checks that the remote branch is in sync with what you last pulled.
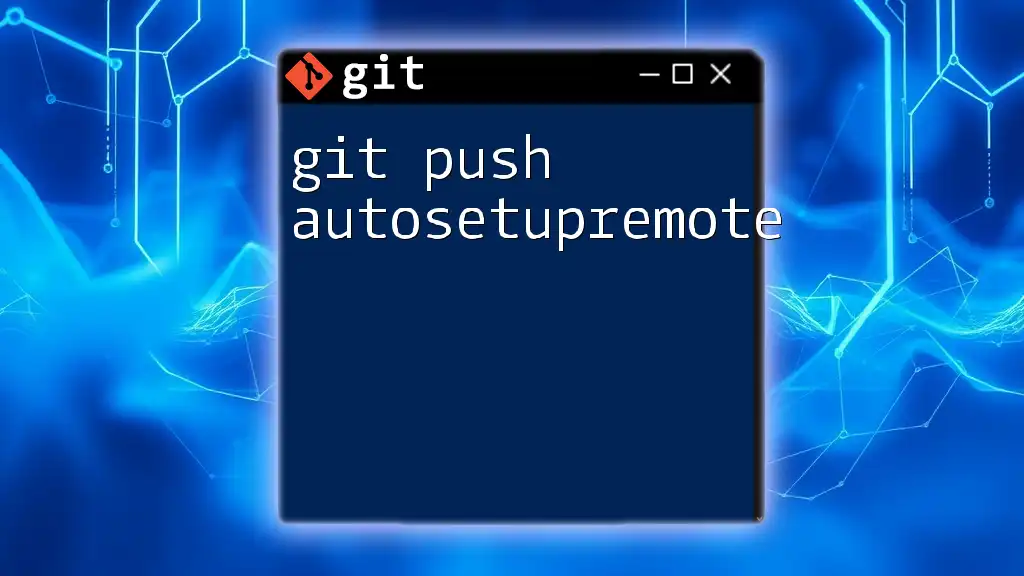
Git Push After Rebase
Why You Need to Push After a Rebase
Pushing after a rebase is crucial because it updates the remote repository with your newly rewritten commit history. If you skip this step and let your local changes linger, it can lead to confusion for your team members, who may see a conflicting or outdated history when trying to sync their own branches.
Performing the Push
Once your rebase is successfully completed, perform the push to sync your changes with the remote repository:
git push origin feature-branch
Handling Common Errors
It’s possible to encounter push errors after a rebase. If your push is rejected due to a non-fast-forward update, this means the remote branch has commits that your local branch does not. In such a case, you can either:
- Pull the latest changes, rebase again, and push.
- Use a force push if you’re confident that you want to overwrite the remote commits:
git push --force-with-lease origin feature-branch
This command forces your local branch updates to the remote while ensuring others’ changes remain intact by checking for remote updates before pushing.
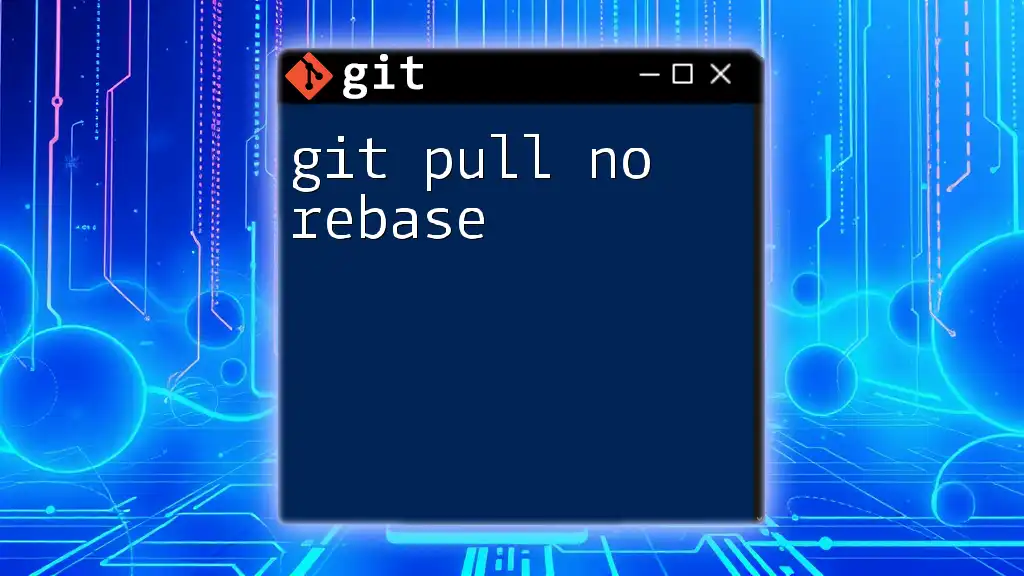
Best Practices for Rebasing and Pushing
Frequency of Rebasing
To maintain a clean and understandable commit history, it’s recommended to rebase often, especially before merging your code into the main branch. Regularly rebasing allows you to incorporate changes from your team effortlessly and minimizes extensive merge conflicts.
Communicating with Your Team
Clear communication with your team members about the state of your branches and ongoing work is essential during the rebasing process. Collaborating effectively can prevent potential conflicts and misunderstandings, particularly in larger teams where multiple people might be working on the same files.
Backing Up Before Rebasing
Always consider backing up your branch before rebasing, particularly if you're unsure of the outcomes. You can create a backup branch like this:
git branch backup-branch
This command saves your current state, allowing you to revert if necessary without losing any work.
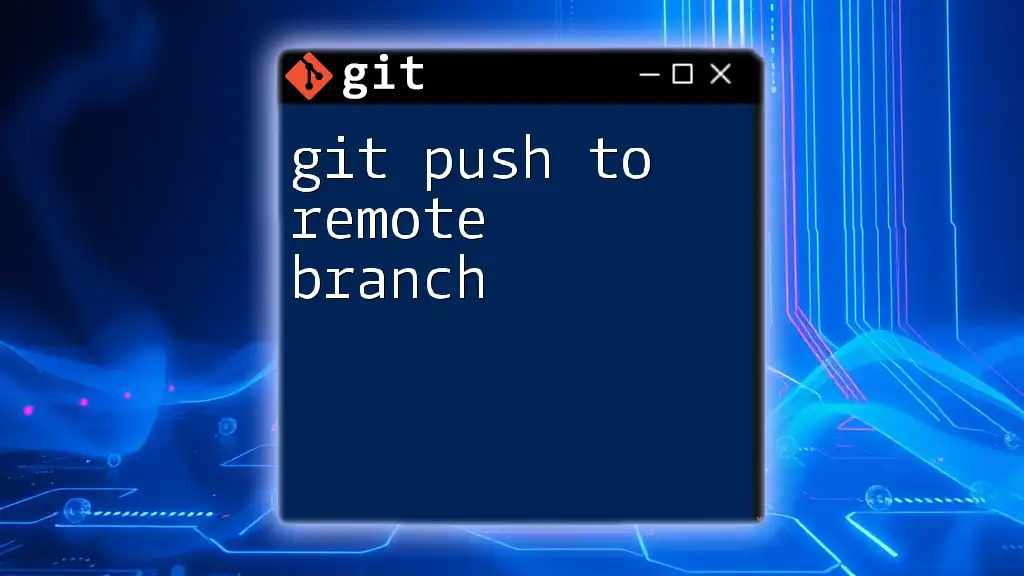
Conclusion
Understanding git push after rebase is vital for smooth collaboration within teams and maintaining a clean project history. By grasping the nuances of rebase and how it intertwines with the push command, you’re positioned to navigate Git more effectively. We encourage you to explore these concepts further and practice executing them as you dive deeper into using Git, enhancing your development workflow and collaboration skills. Explore the Git courses we offer to sharpen your command of these essential tools!
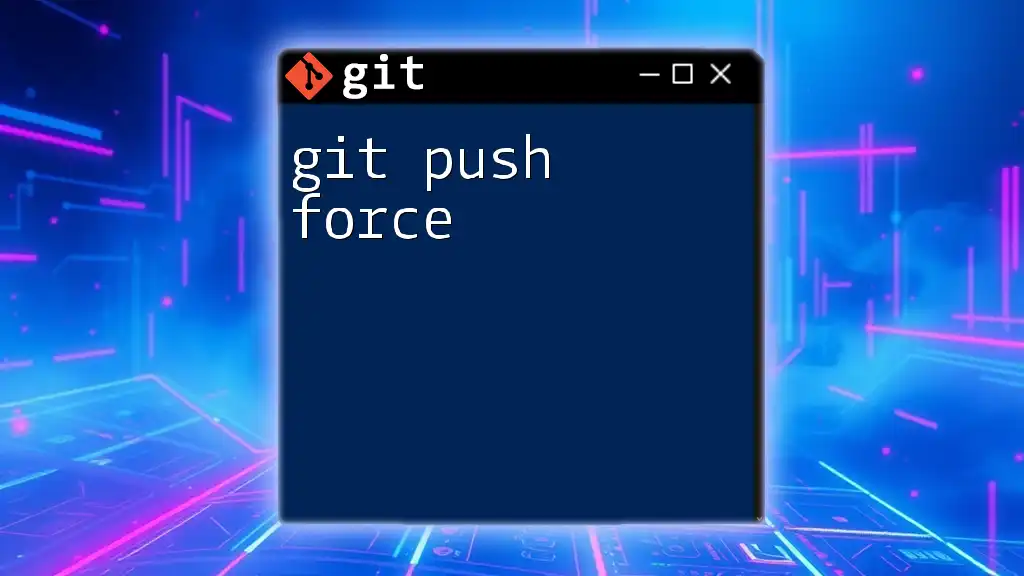
Additional Resources
For those eager to expand their knowledge, consider delving into official Git documentation and guides on GitHub or GitLab. This will help reinforce and enhance your understanding of Git commands. Don’t hesitate to access our company’s resources dedicated to teaching Git commands in a concise and efficient manner.