The command `git push -u -f` forces a push to the remote repository, setting the upstream for the current branch while potentially overwriting changes that exist on the remote counterpart.
git push -u -f origin your-branch-name
Understanding the Basics of `git push`
What is `git push`?
`git push` is a fundamental command in Git used to transfer local repository content to a remote repository. The purpose of this command is not only to upload changes but also to synchronize your local branches with the branches on a remote server, ensuring that other collaborators can access your latest work.
Common Options Used with `git push`
`-u` (or `--set-upstream`)
The `-u` option, or `--set-upstream`, is used to link your local branch to a remote branch. By using `-u`, you essentially tell Git to remember the relationship between the local and remote branch, allowing you to omit the remote and branch name in future `git push` and `git pull` commands.
Example: Setting Upstream Branch
git checkout -b new-feature
git add .
git commit -m "Initial commit for new feature"
git push -u origin new-feature
Explanation: In this scenario, a new feature branch is created, committed, and pushed to the remote repository. By using `-u`, this branch will now be linked to the remote `new-feature` branch. In subsequent pushes, you can simply use `git push`, without specifying the remote and branch.
`-f` (or `--force`)
The `-f` option, or `--force`, is used to overwrite changes on the remote branch. This is particularly useful in scenarios where you need to push changes that may not be in sync with the remote version, like after a rebase or when you've rewritten commit history.
Caution: While force pushing can be incredibly useful, it comes with significant risks. Always be aware that force pushing may lead to data loss on the remote repository if changes made by others are removed inadvertently.
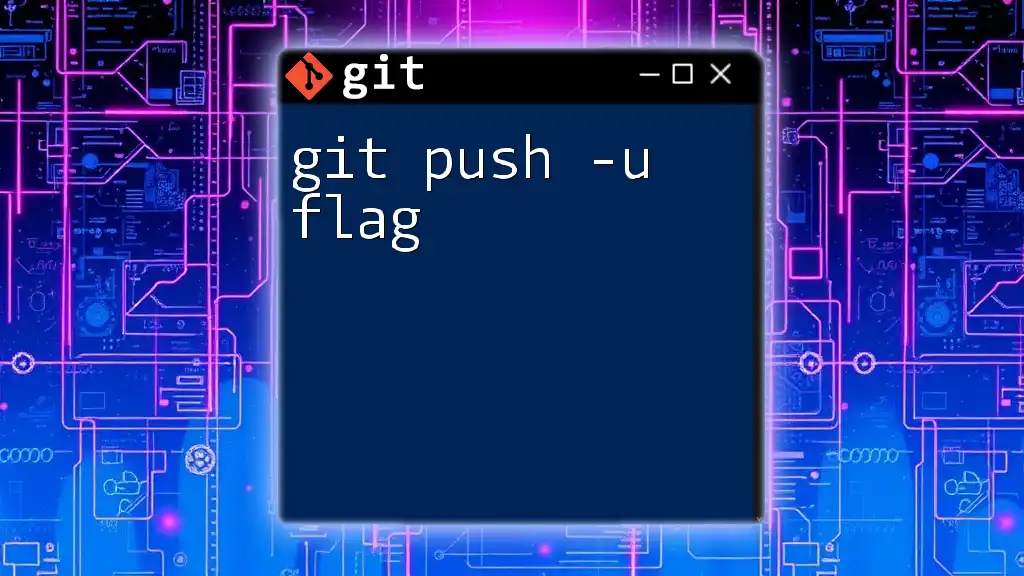
In-Depth Exploration of `git push -u -f`
Combining Options: `-u` and `-f`
When you use `git push -u -f`, you are combining the capabilities of both commands. This action sets your local branch while also allowing you to overwrite any conflicting changes on the remote branch. This is especially relevant when you are sure that your changes are the correct ones and you either want to discard or replace the remote history.
Examples and Code Snippets
Example 1: Setting Upstream Branch
Let's look at an example where we create a new branch and push it:
git checkout -b new-feature
git add .
git commit -m "Initial commit for new feature"
git push -u origin new-feature
In this case, the `-u` option links the local `new-feature` branch to `origin/new-feature`, allowing for easier future synchronization.
Example 2: Force Pushing Changes
Consider a scenario where you want to overwrite the remote branch after rebasing:
git rebase main
git push -u -f origin new-feature
Here, the rebased local branch is pushed to the remote, effectively replacing its content with the new linear history. This command is potent when you are confident about the changes you are applying.
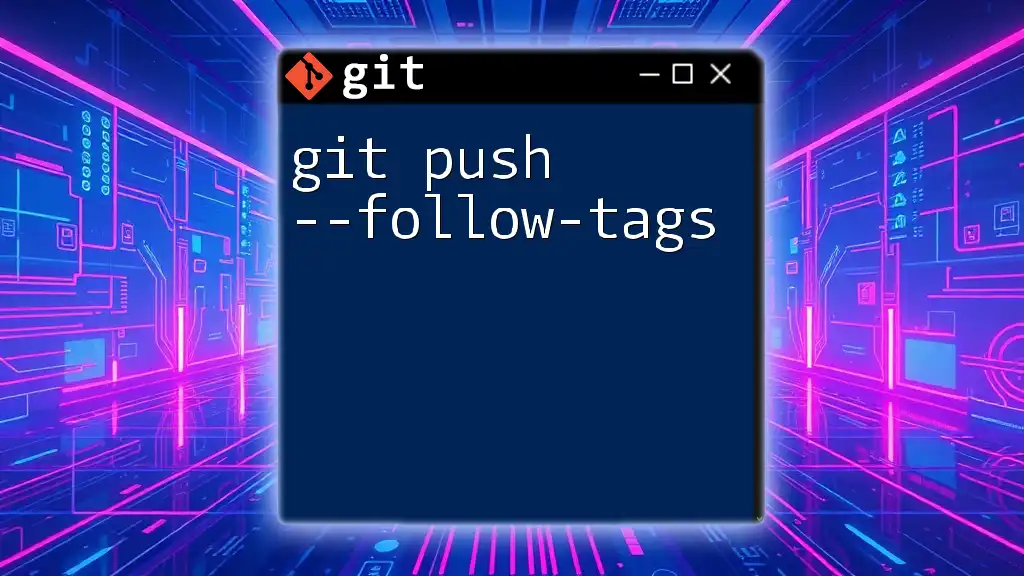
Potential Risks of Using `-f`
Understanding the Consequences of Force Pushing
Using `git push -u -f` can lead to unintended consequences. When you force push, any commits that exist on the remote branch but not in your local branch will be lost. This could disrupt your collaborations and cause frustration for your co-workers.
When you perform this action, the remote branch history is rewritten entirely based on your local branch. This often renders shared work obsolete, which could negatively impact project timelines and result in lost effort.
Best Practices to Avoid Mistakes
To use the force push responsibly, consider adhering to the following best practices:
- Before executing a force push, communicate with your team to ensure that there are no conflicting changes.
- Consider using `--force-with-lease` instead of `-f`. This option is safer as it prevents you from overwriting changes unless your local branch is updated with the latest remote changes.
By practicing caution and ensuring clear communication, you can mitigate many of the risks associated with force pushing.
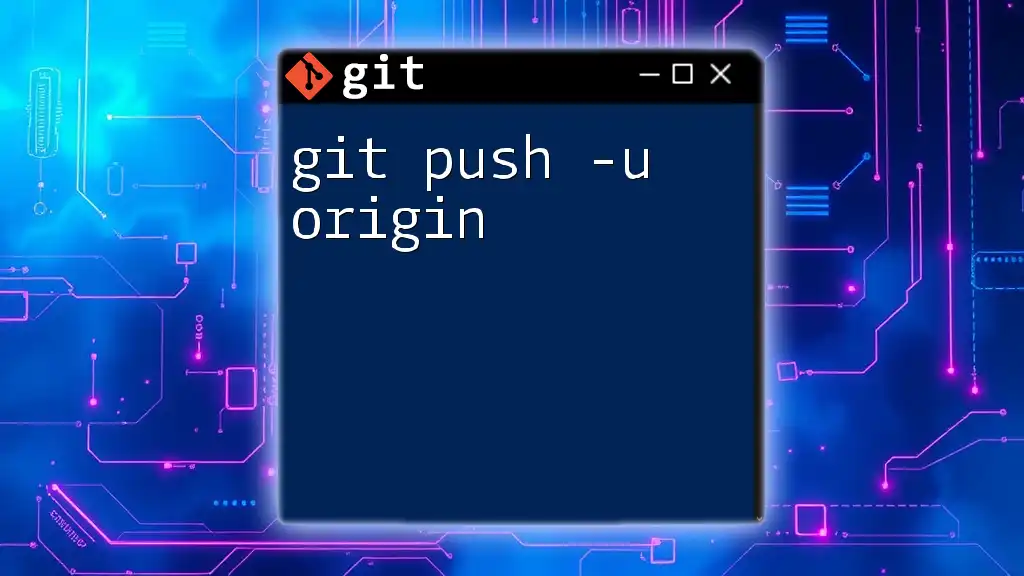
Conclusion
Understanding `git push -u -f` is essential for effectively managing your workflows in Git. While this command provides powerful options to control the relationship between your local and remote branches, it also carries significant risks that should not be underestimated.
As you practice with examples and scenarios, you will become more adept at using Git to its fullest potential. Remember to approach powerful commands like this one with caution and consideration for your collaborative environment.
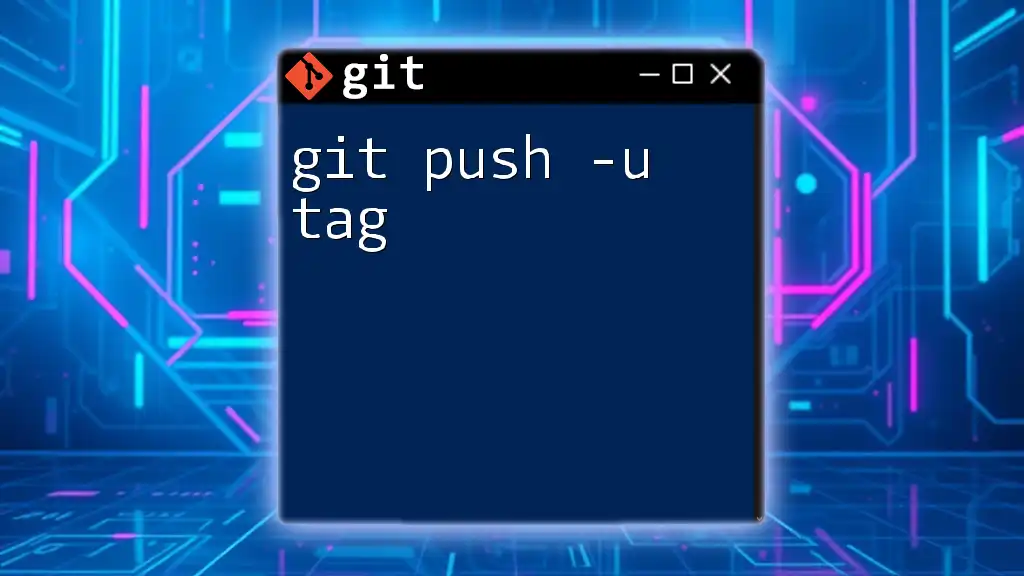
Additional Resources
Further Reading
For more comprehensive instructions and best practices, consult the official [Git documentation](https://git-scm.com/doc).
Community and Support
Join online Git communities and forums where you can seek advice and share experiences with other developers to further enhance your understanding and expertise in using Git commands.