The `git push -m` command allows you to push changes to a remote repository along with a message describing the push; however, note that the `-m` flag is typically used in `git commit` rather than `git push`, so you should use `git push` without `-m` when syncing changes.
Here’s how you normally push changes:
git push origin main
If you intend to include a message with your commits prior to pushing, you would use:
git commit -m "Your commit message here"
Understanding Git's Push Mechanism
What is `git push`?
The `git push` command is a fundamental part of using Git for version control. In essence, it allows you to send your committed changes in a local repository to a remote repository. This step is crucial for keeping your team members up-to-date with the latest code changes. When you push your changes, you make them accessible to others, contributing to a collaborative environment.
The Role of Commit Messages
Commit messages serve as a historical record of what changes were made and why. A well-crafted commit message can significantly enhance collaboration among team members. It acts as a guide for anyone reading the repository’s history, helping them comprehend the rationale behind each change.
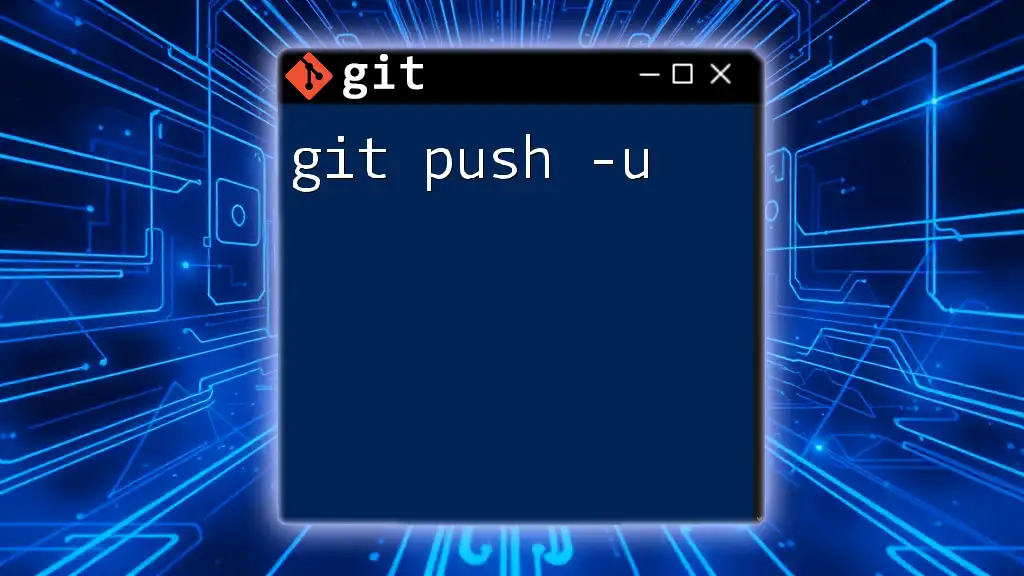
The `-m` Option with `git push`
What Does `-m` Stand For?
The `-m` option stands for "message" and allows you to include a commit message directly within the `git push` command. This feature is useful for providing context about the changes being pushed, but it is typically used in conjunction with the `git commit` command to streamline your workflow.
Syntax for Using `git push -m`
The general syntax for using `git push` with the `-m` option is:
git push [<options>] [<repository> [<refspec>...]] -m "<commit message>"
- `<options>` represents any additional options you might want to include.
- `<repository>` is the remote repository to which you are pushing.
- `<refspec>` specifies which branch or tag to push.
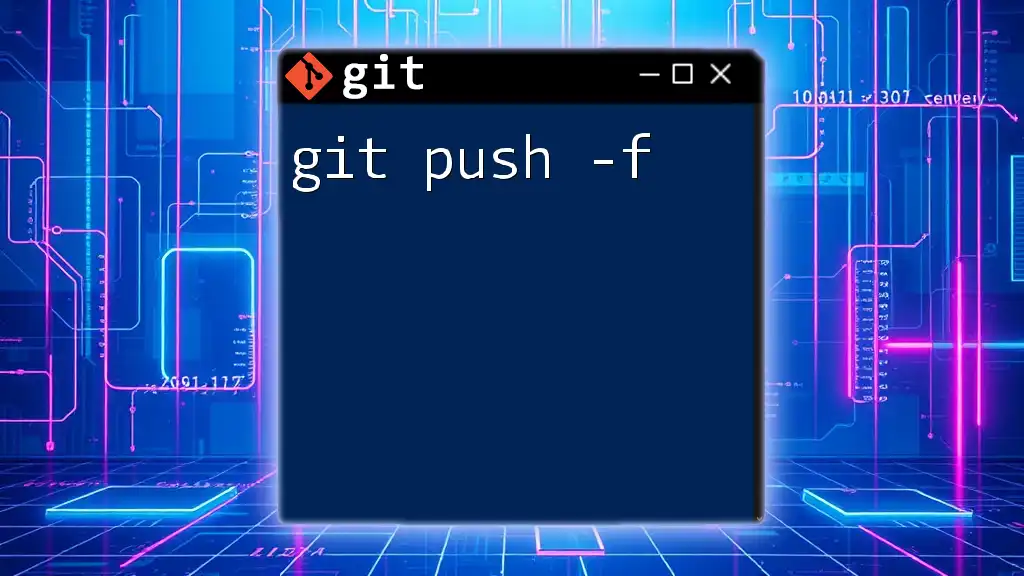
Setting Up Your Repository
Initializing a Git Repository
Before you can use the `git push -m` command, you need a Git repository. Here’s how to create a new Git repository from scratch:
git init my-repo
cd my-repo
This creates a new directory called `my-repo` and initializes it as a Git repository.
Making Changes and Committing
Once your repository is set up, you can start making changes. To commit your changes, follow these steps:
-
Create a new file or modify an existing one:
echo "Hello, World!" > hello.txt
-
Stage your changes for commit:
git add hello.txt
-
Commit your changes with a meaningful message:
git commit -m "Add hello.txt file"
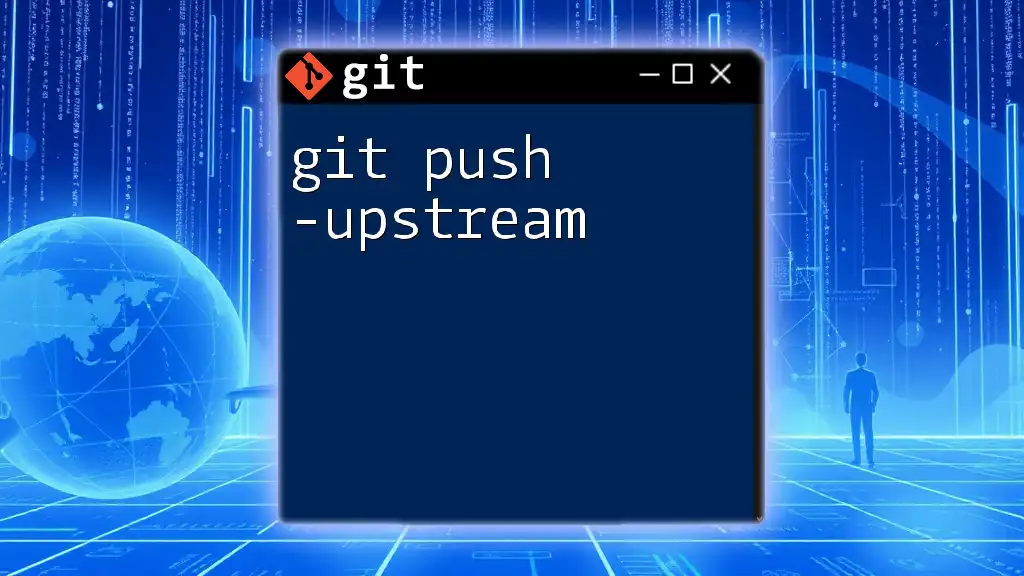
Using `git push -m`
How to Push Changes with a Message
To push your local changes to a remote repository, use the `git push` command along with the `-m` option. Here’s how you would typically do it:
git push origin main -m "Pushing changes to main branch"
In this command:
- `origin` refers to the name of your remote repository.
- `main` is the branch you are pushing to.
- The `-m` option is followed by the message detailing the push.
Understanding Output Messages
When you run the `git push -m` command, you will see output messages indicating the success or failure of your push. Successful pushes will generally include confirmation of the branch that was updated. If there’s an issue, Git will provide error messages that can guide you in troubleshooting.
Alternative to `git push -m`
Using `git commit` Separately
It's important to note that using `git push -m` is not the only way to push changes. In fact, it’s often better to commit your changes using `git commit -m` and then push separately. This practice can simplify your workflow and make the history clearer. Here’s how it looks:
git commit -m "Update README"
git push origin main
In this case, the push is clearly delineated from the commit, allowing for a more organized workflow.
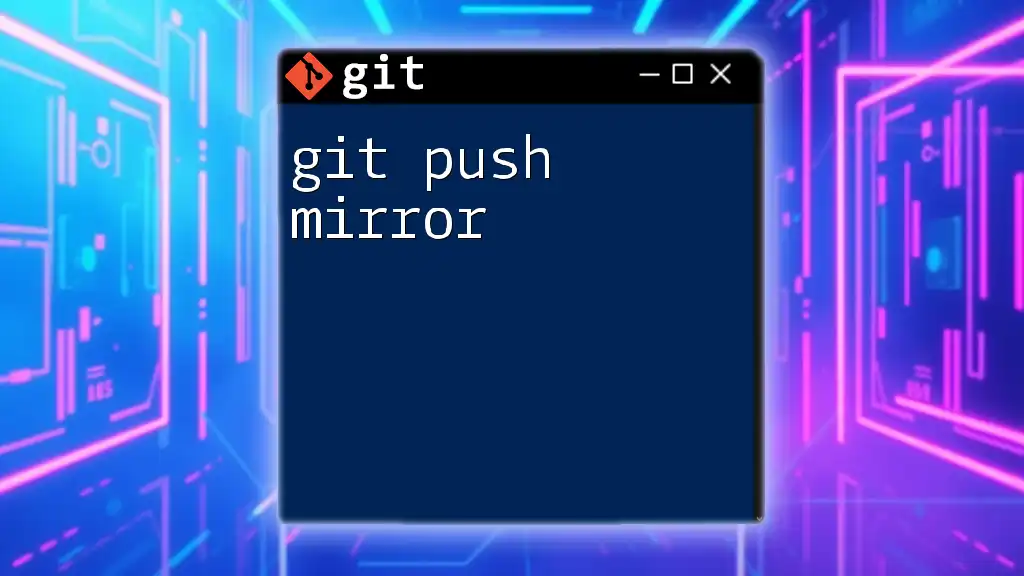
Common Scenarios and Troubleshooting
Scenario 1: Pushing to a Remote Branch
When you want to push to a branch other than the one you are currently on, you can specify the branch directly:
git push origin feature-branch -m "Merge feature branch"
This command pushes your local changes in `feature-branch` to the remote repository.
Scenario 2: Handling Merge Conflicts
Sometimes, the push may fail if there are merge conflicts. In this case, you’ll need to pull the latest changes from the remote branch, resolve any conflicts, and attempt the push again.
Scenario 3: Pushing with Authentication
When pushing changes to a remote repository, you might need to authenticate your connection, especially if you use SSH or HTTPS. Here’s a sample command for adding a remote using SSH:
git remote add origin ssh://user@host/repo.git
Understanding authentication methods will help you avoid issues during the push process.
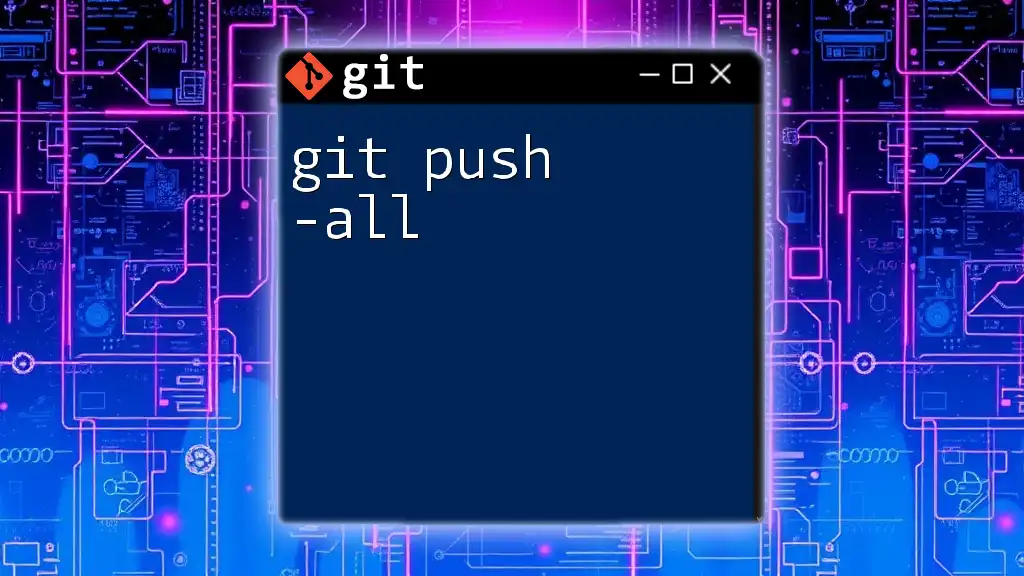
Best Practices for Commit Messages
Importance of Clear and Concise Messages
A good commit message is critical for collaboration and project management. It provides insight into the history of your project and helps team members understand what has been accomplished at a glance.
Structure of a Good Commit Message
A best practice for commit messages is to follow a simple structure:
- Title: Short description (50 characters or less).
- Body: Detailed explanation (if necessary), covering the why and how of the changes.
- Footer: Optional to include issues being closed or further references.
An effective commit message looks like this:
Short description of changes
Detailed explanation of why those changes are necessary and any relevant context.
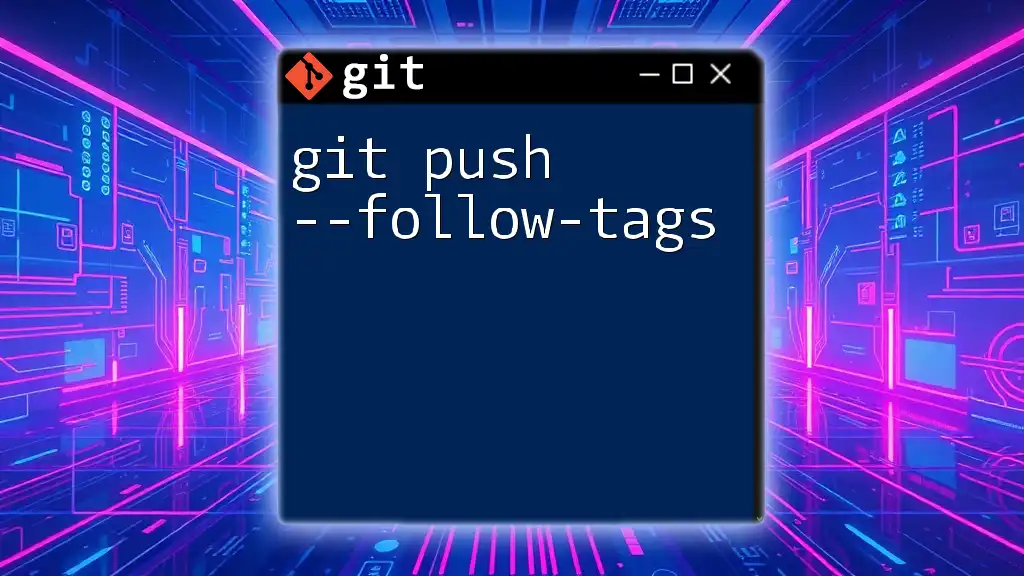
Conclusion
The `git push -m` command is a powerful tool in your Git arsenal, facilitating the process of pushing code changes and providing context through commit messages. Familiarizing yourself with its nuances not only strengthens your own workflow but also promotes better collaboration with others. Don’t hesitate to practice these commands in your projects; the more comfortable you become, the smoother your Git experience will be.
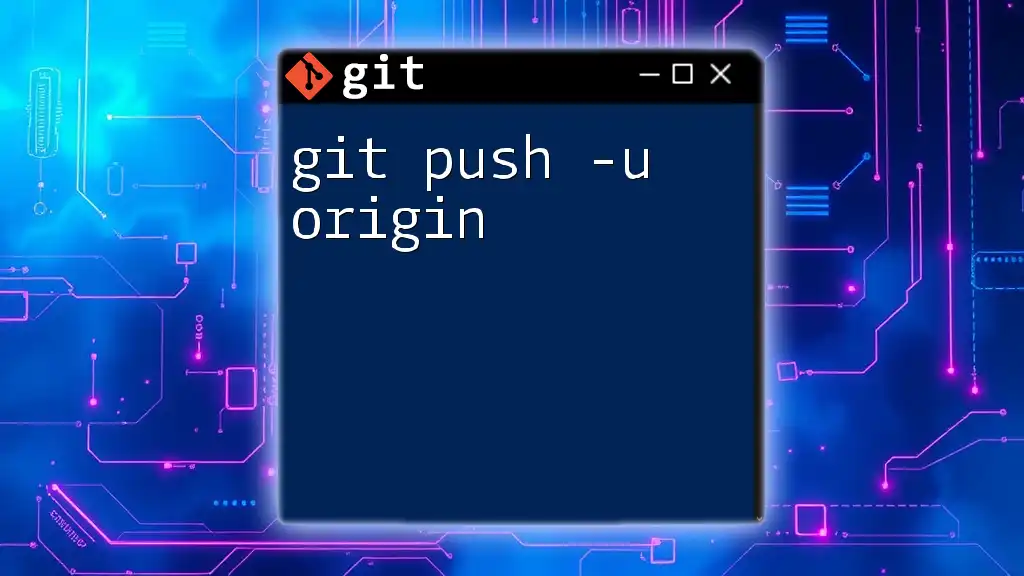
Additional Resources
For further reading and to enhance your Git skills, consider exploring Git documentation, reputable tutorials, and community forums. Learning from various resources can bring new insights and techniques to your version control practices.