The `-u` option in the `git push` command sets the upstream tracking reference for the current branch to the specified remote branch, making future pushes and pulls easier by allowing you to skip the remote specification.
git push -u origin main
What is `git push`?
The `git push` command is a fundamental part of Git, primarily used to upload local branch commits to a remote repository. It serves as a way to share changes you've made in your local environment with collaborators or deploy updates to a live system.
Basic Syntax
git push <remote> <branch>
Here, `<remote>` typically refers to the name of the remote repository (most commonly `origin`), and `<branch>` specifies the branch you are pushing.
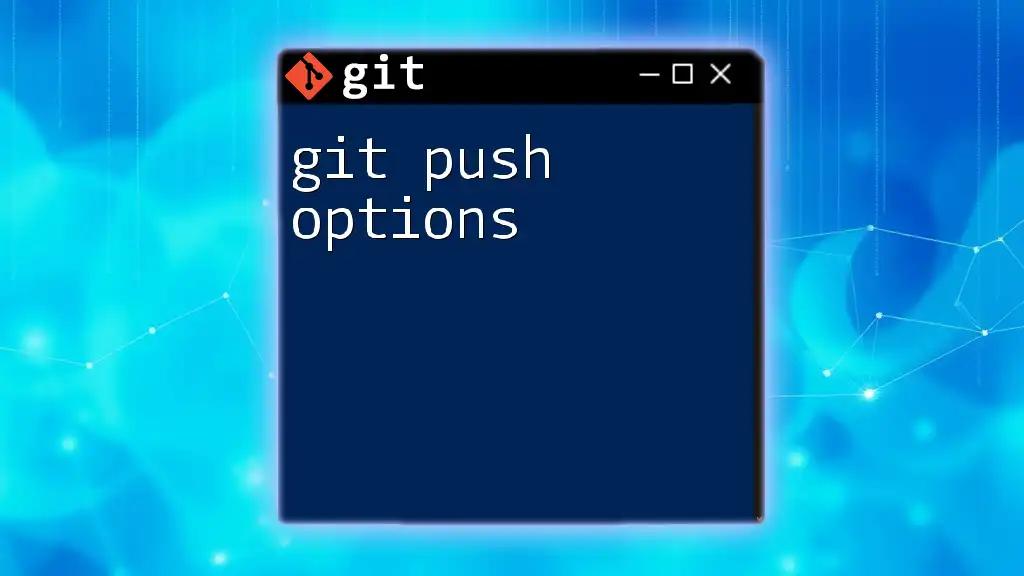
Understanding the `-u` Option
The `-u` option, short for `--set-upstream`, plays a crucial role in simplifying your Git workflow. By using this option, you create a default tracking relationship between your local branch and the specified remote branch.
When to Use `-u`
Using the `-u` option is particularly beneficial in the following scenarios:
- First-time Push of a New Branch: When you're pushing a new branch to a remote for the first time, utilizing the `-u` option streamlines the process.
- Collaboration: In a multi-developer environment, setting the upstream helps in reducing confusion when pulling and pushing changes.
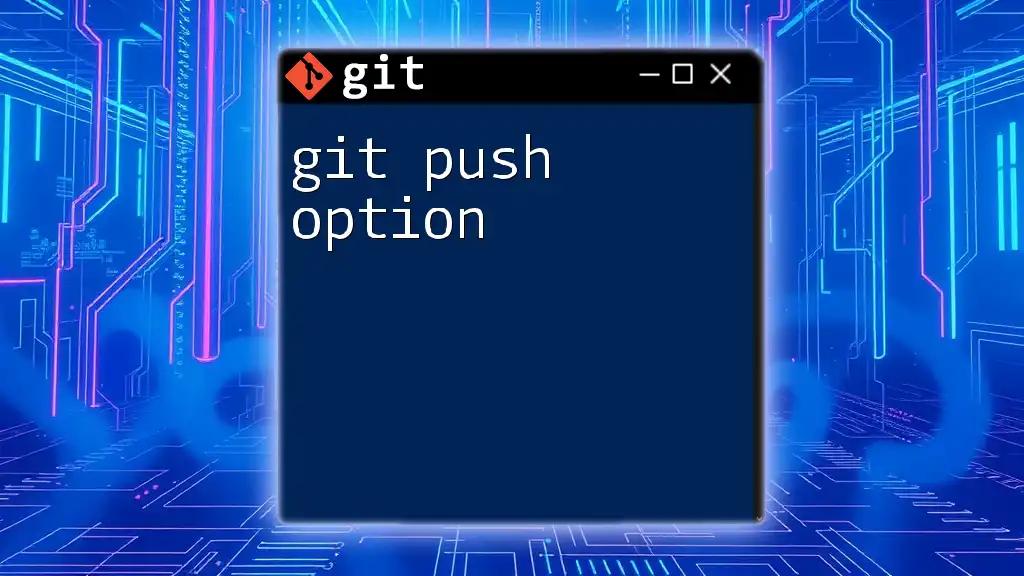
How to Use `git push -u`
The basic command structure for using the `git push -u` option is straightforward:
git push -u <remote> <branch>
Example Usage
Let’s consider a practical example: you're developing a new feature and have created a branch named `feature/new-feature`. The first time you want to push this branch to the remote repository, you can do so by executing:
git push -u origin feature/new-feature
Explanation of the Example
When you run this command, Git does two things:
- It pushes all your commits from the `feature/new-feature` branch to the remote repository named `origin`.
- It sets the branch `feature/new-feature` to track the remote branch of the same name. This means that in future pushes or pulls, you can simply use `git push` or `git pull` without having to specify the remote and branch, simplifying your workflow.
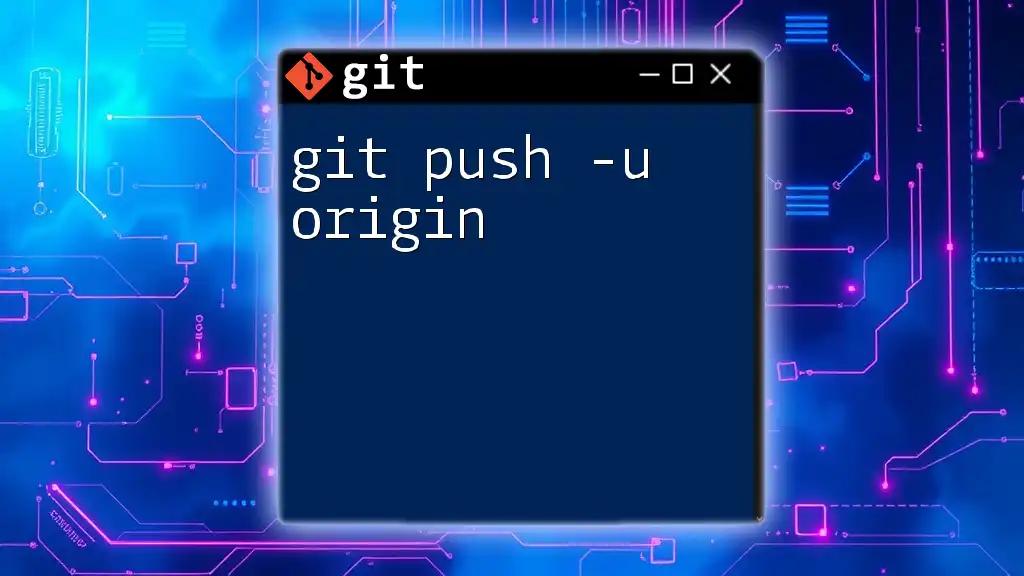
Benefits of Using `git push -u`
Simplifying Future Pushes
One of the most significant advantages of using the `-u` option is that it eliminates the need to specify the remote and branch name during future pushes. This means once you've set up tracking with `-u`, you can just type:
git push
This streamlined command is a huge time-saver, especially when collaborating on various branches.
Enhanced Collaboration
In a multi-developer environment, using `git push -u` makes managing branches much more straightforward. It allows all developers on a team to see which branch you are working on by simply running:
git branch -vv
This enhances transparency and helps facilitate pull requests, making the collaborative coding process smoother.
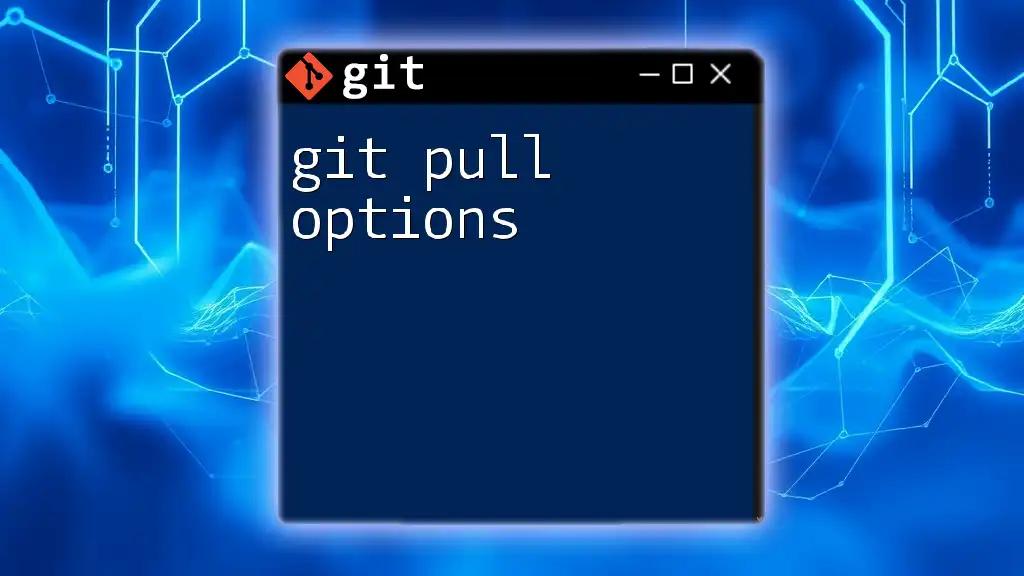
How to Verify Upstream Tracking
To check which upstream branch is currently set for your local branches, you can use:
git branch -vv
This command will display a list of all local branches, their tracking status, and the latest commits, making it clear which branches are properly set up.
Interpreting the Output
The output will look something like this:
* feature/new-feature d5ae2d3 [origin/feature/new-feature] Added new feature with initial structure.
In this example, the asterisk (*) indicates the current branch, and the bracket shows the upstream branch it's tracking.
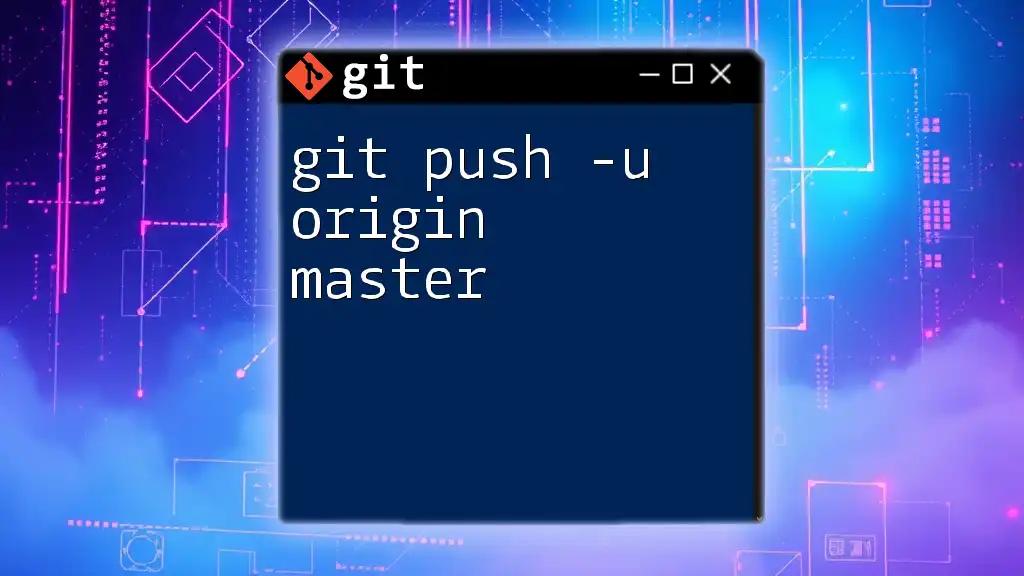
Changing the Upstream Branch
If you need to change the upstream branch for your local branch, you can use the `--set-upstream-to` option. The syntax is:
git branch --set-upstream-to=<remote>/<branch>
Example Scenario
Suppose you originally set the upstream for `feature/new-feature` but now want it to track a different branch, say `origin/feature/another-feature`. Here’s how you would do it:
git branch --set-upstream-to=origin/feature/another-feature
This command updates your local branch's tracking information to the newly specified remote branch.

Common Issues with `git push -u`
Even with its advantages, using the `-u` option can lead to some common issues that are important to be aware of.
Error Messages Explained
One of the most frequent error messages you might encounter is:
fatal: The upstream branch does not exist
This typically occurs when you attempt to push a branch for the first time without specifying the tracking option.
Troubleshooting Steps
To fix this error, always ensure that you're using the `-u` option on your first push of a new branch. If you accidentally set an incorrect upstream, you can use the `--set-upstream-to` command highlighted above to correct it.
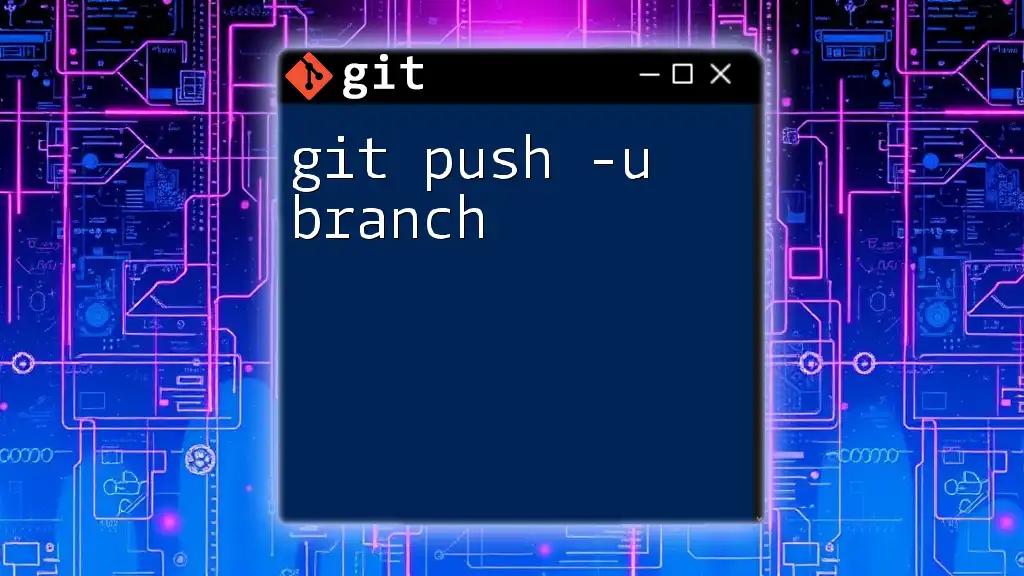
Best Practices for Using `git push -u`
When to Use `-u`
Utilize the `-u` option whenever you’re pushing a new branch for the first time. It’s a best practice to establish this tracking relationship upfront to avoid confusion later on.
Avoiding Confusion
Managing multiple branches can lead to confusion, especially in collaborative environments. To avoid this:
- Always keep your branch names clear and descriptive.
- Regularly check the tracking status with `git branch -vv` to ensure you know where your branches stand.
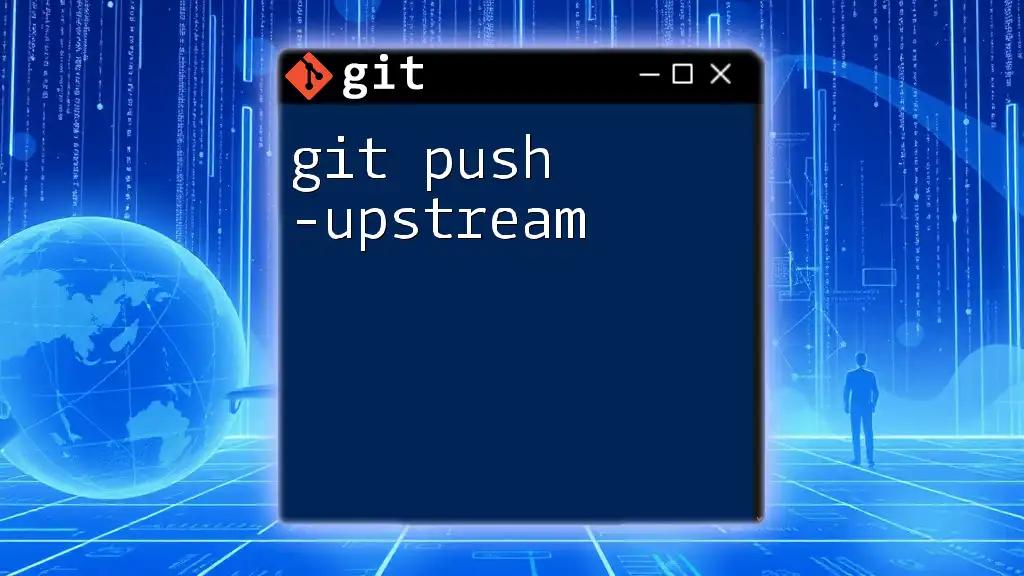
Conclusion
The `git push -u` option is a powerful tool that streamlines your Git workflow significantly. By establishing a tracking relationship between local and remote branches, you make your collaboration with others more efficient and reduce the complexity of managing multiple branches. Incorporating the `-u` option into your daily usage of Git will undoubtedly enhance your overall development experience.
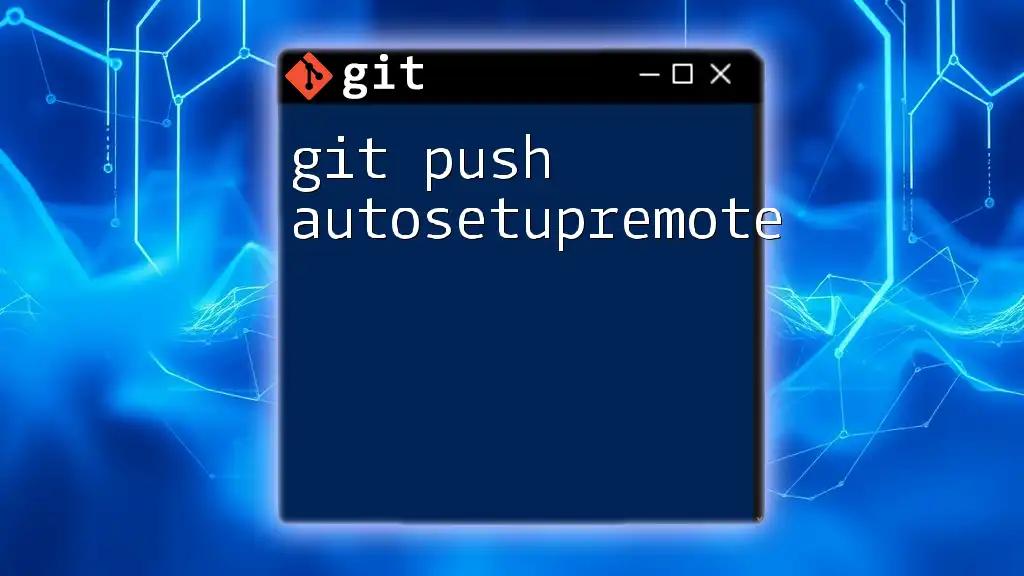
Additional Resources
Explore the official Git documentation for in-depth coverage of the `git push` command and consider engaging with learning platforms for enhanced understanding. Joining forums and FAQs can also provide support for any lingering questions you may have.