The `git push` command is used to upload local repository changes to a remote repository, with various options available to customize its behavior, such as setting the upstream branch or pushing only specific branches or tags.
git push origin main --set-upstream
Understanding `git push`
The `git push` command is a crucial element of using Git, as it enables developers to upload their local repository content to a remote repository. Essentially, it facilitates collaboration in version-controlled projects by synchronizing changes that you, your team, or others have made. When you run this command, you inform Git to take your commits from a local branch and transfer them to a remote branch, keeping everything updated.
Basic `git push` Syntax
The syntax of the `git push` command is straightforward but packed with significance. The command typically follows this structure:
git push <remote> <branch>
- git: This invokes the Git version control system.
- push: This specifies the action you’re taking—pushing your commits.
- <remote>: This denotes the name of the remote repository (typically `origin`).
- <branch>: This identifies the branch you want to push to.
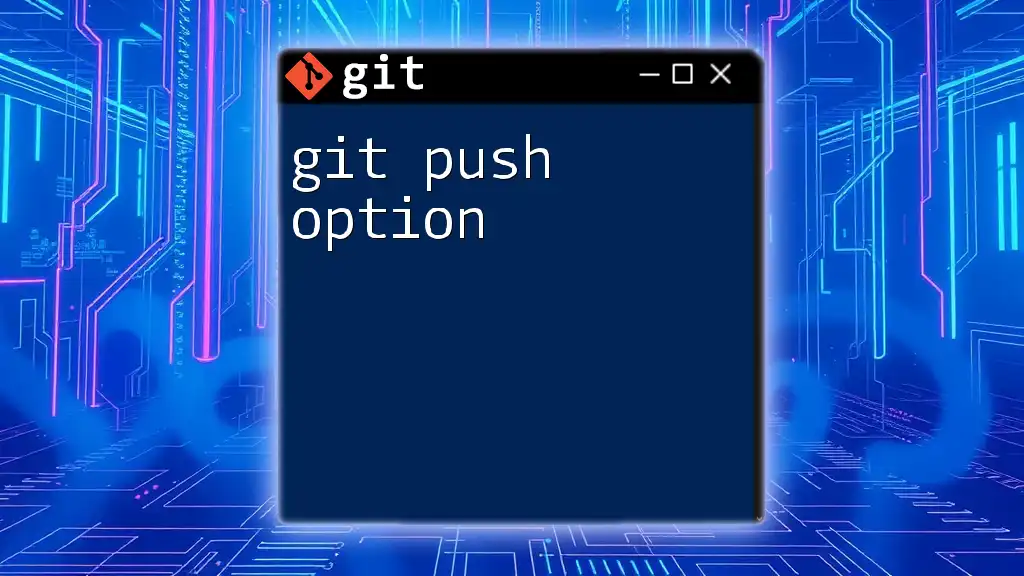
Common `git push` Options and Their Usage
`--all`
The `--all` option is a powerful feature that allows users to push all local branches to the remote repository. This can be particularly useful when you want to share all your developments at once.
Code Example
git push --all origin
In this case, all the branches in your local repository are pushed to the `origin` remote reference, ensuring that your teammates have all the latest updates across branches.
`--tags`
With the `--tags` option, you can push all your local tags to the remote repository. Tags are essential for marking specific points in your repository's history, often used for releases.
Code Example
git push --tags
Utilizing this feature is important when you want to ensure that all version tags are accessible in the remote, making it easier to manage and track releases.
`--force` and `--force-with-lease`
The `--force` option tells Git to overwrite the remote changes with your local changes, regardless of the state of the remote repository. While this can be useful, it carries risks, as it may delete commits in the remote repository that others have contributed.
Code Example
git push --force origin feature-branch
On the other hand, `--force-with-lease` is a safer alternative. It allows you to push changes only if the remote branch hasn't been updated since the last time you fetched it. This way, you mitigate the risk of unintentionally overwriting someone else's work.
`--dry-run`
If you want to see what would happen if you executed a push without actually performing it, the `--dry-run` option is most beneficial. This simulates the push process, providing insight into what will occur.
Code Example
git push --dry-run
This option is particularly useful for verifying your actions ahead of time, ensuring that you're aware of what changes might be pushed to the remote repository.
`--set-upstream` and `-u`
The `--set-upstream` (or `-u`) option is essential for setting the upstream tracking branch, allowing your local branch to reference which remote branch it should sync with. This can save time for subsequent pushes and pulls.
Code Example
git push -u origin new-feature
By using this option, you link your local `new-feature` branch to the corresponding remote branch on `origin`. Future commands will automatically apply to this remote branch, streamlining your workflow.
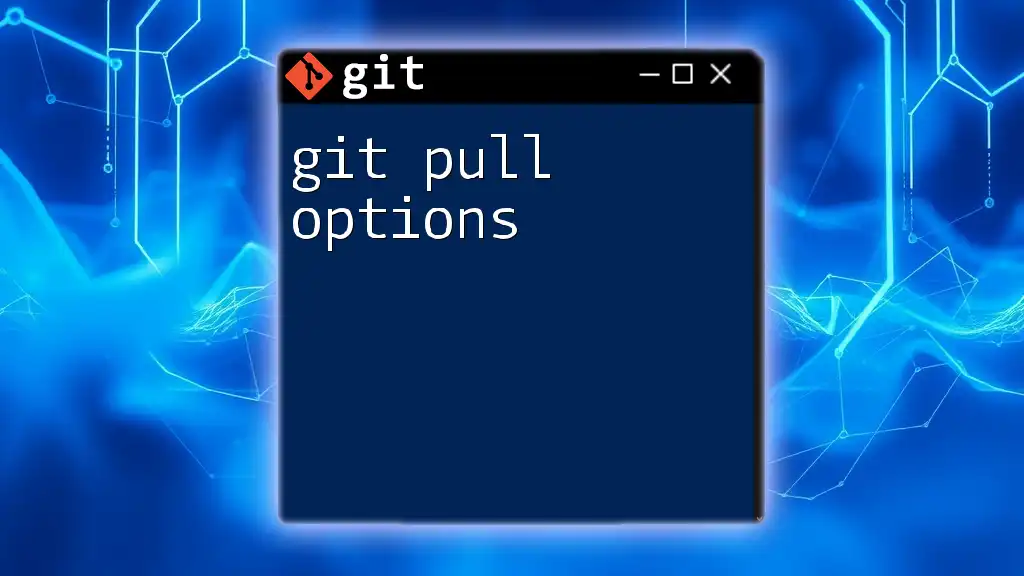
Best Practices for Using `git push`
When using `git push`, it's crucial to understand the current state of your branches before pushing. Here are some essential best practices to keep in mind:
-
Before pushing, always make sure your local branch is up-to-date with its corresponding remote branch. This helps prevent merge conflicts and ensures that you are not inadvertently overwriting new changes made by others.
-
Use `--force` sparingly: Only use this option when absolutely necessary, and preferably after communicating with your team. Understand the risks of losing others’ valuable contributions.
-
Keep your local repository clean by removing or organizing branches that are no longer in use. This minimizes clutter and simplifies the push process.
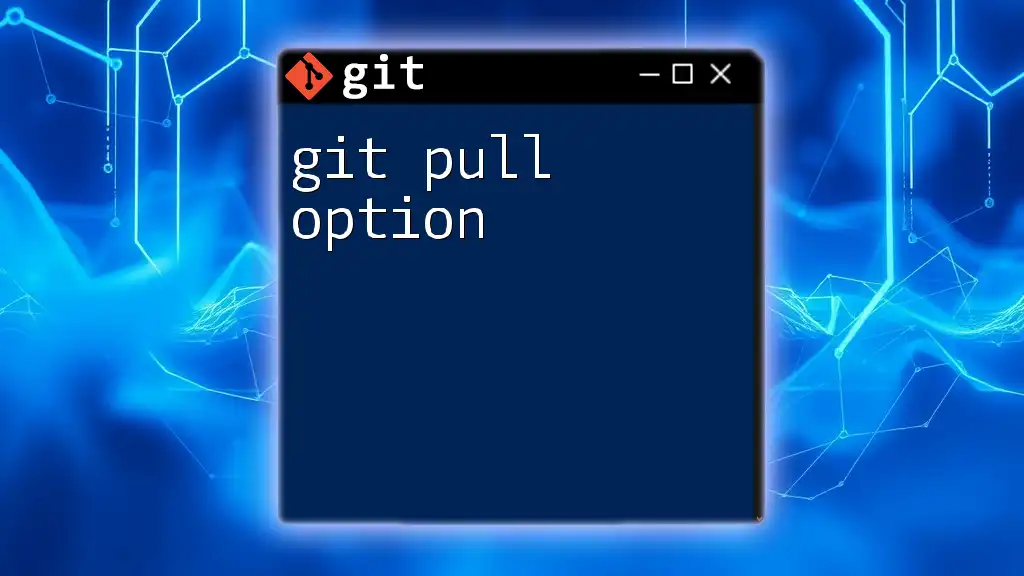
Dealing with Common Push Errors
Error: ‘failed to push some refs to’
Encountering the error message “failed to push some refs to” usually means that the remote branch has changes that are not present in your local branch. This commonly occurs when someone else has pushed changes to the same remote branch.
Code Example
To resolve this, you may need to pull the latest changes and merge them into your branch:
git pull --rebase origin master
git push origin master
This approach fetches the latest updates from the remote, rebases your local changes on top, and then allows you to push them successfully.
Error: Push is rejected due to non-fast-forward update
A non-fast-forward update happens when the state of the remote repository has diverged from your local repository. To address this, you can resolve the divergence by either merging or rebasing your changes against the latest updates from the remote.
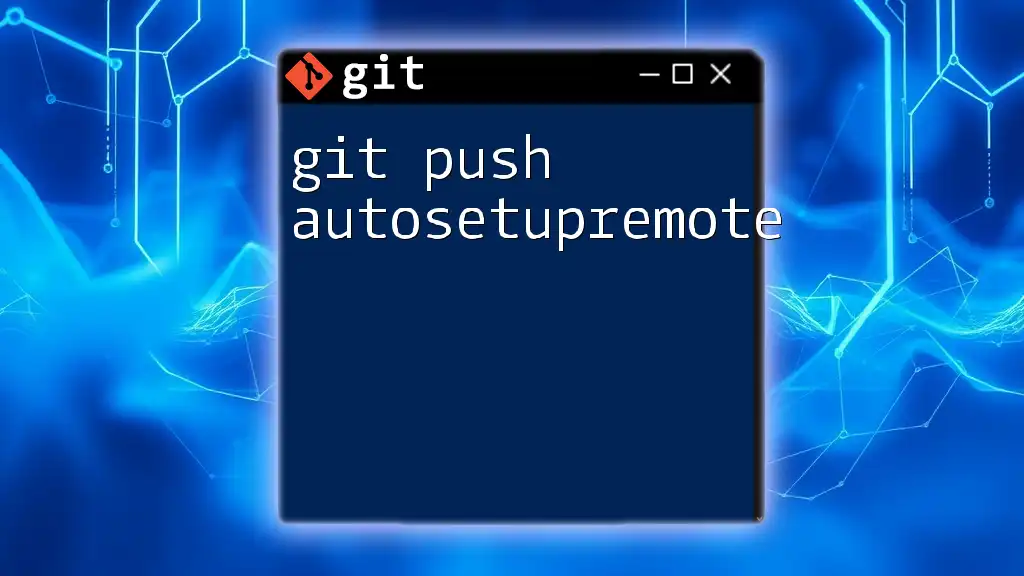
Summary
Understanding the different git push options is crucial for any developer looking to streamline their workflow and enhance collaboration with their team. Each option serves a specific purpose and can be invaluable when used appropriately.
Practice using these options regularly to become more proficient and confident in managing your repositories. As you continue to explore Git, remember that grasping how to properly use `git push` is a fundamental skill that can greatly enhance your development experience.
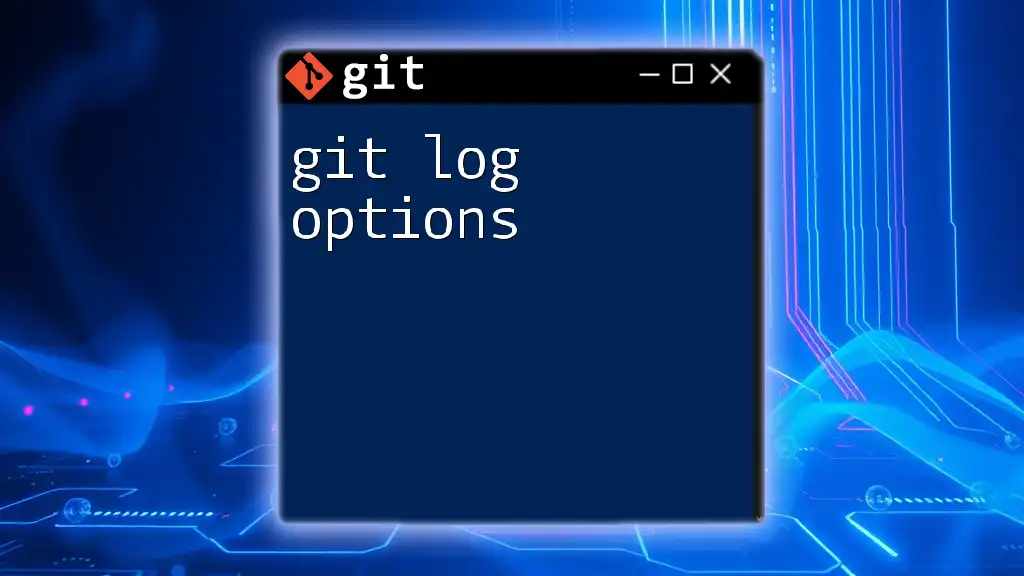
Additional Resources
To further your knowledge of Git, consider exploring more advanced Git commands and best practices. Numerous resources are available, including the official Git documentation, online courses, and community forums. Additionally, feel free to check out our services for tailored Git training sessions that can help you or your team master these essential skills.