Git actions refer to the fundamental commands used for version control, allowing users to manage and manipulate their code repositories effectively.
Here’s an example of a common Git action that initializes a new repository:
git init
Understanding Git Actions
Git actions are fundamental commands that allow users to manage their repositories, enabling effective version control, collaboration, and project management. By understanding and mastering these actions, users can efficiently navigate their development workflows.
Defining Git Actions
At its core, a Git action is any command that interacts with a Git repository, modifying its state, structure, or content. These actions can range from the simplest commands like checking out a branch to more complex tasks like resolving merge conflicts. Each action plays a pivotal role in maintaining the integrity and organization of a project.
Why Git Actions Matter
Mastering Git actions is crucial for anyone involved in software development or collaborative projects. Proficiency in Git commands enhances productivity, enabling team members to streamline workflows and minimize errors. Using Git actions effectively can significantly reduce the time spent on version control tasks, allowing developers to focus on writing code and delivering features.
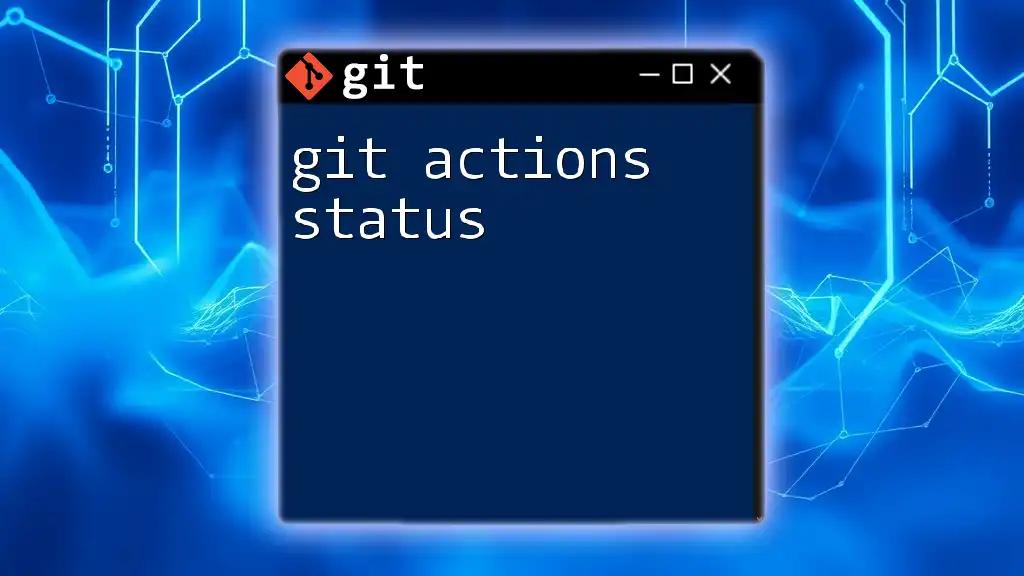
Essential Git Actions
Cloning a Repository
Cloning is one of the first steps a developer takes when they want to contribute to a project. The command `git clone` creates a local copy of a remote repository, enabling users to start working on it right away.
Code Snippet:
git clone <repository-url>
For example, if you want to clone the popular open-source project hosted on GitHub, the command would look like this:
git clone https://github.com/username/repository.git
Cloning not only downloads the files but also sets up the remote origin, establishing a connection between your local repository and the remote one.
Committing Changes
Once you’ve made changes to your local files, the next step is to save these changes to the repository. This is done using the `git commit` command. A critical aspect of committing is writing meaningful commit messages that explain what changes were made and why.
Code Snippet:
git commit -m "Your commit message here"
An effective commit message could be:
git commit -m "Fix bug in user login feature"
This gives context to your team, improving collaboration.
Pushing Changes to Remote
After committing changes, you'll want to share them with your team by pushing to the remote repository. This is accomplished through the `git push` command.
Code Snippet:
git push origin <branch-name>
For instance, if you are on the `feature-branch`, you would use:
git push origin feature-branch
This updates the remote repository with your commits, making them available for others.
Pulling Updates from Remote
Staying up-to-date with changes made by other collaborators is vital. The `git pull` command allows you to fetch and integrate changes from the remote repository into your current branch.
Code Snippet:
git pull origin <branch-name>
For example:
git pull origin main
This command ensures that your local branch reflects the latest updates, helping to avoid conflicts later.
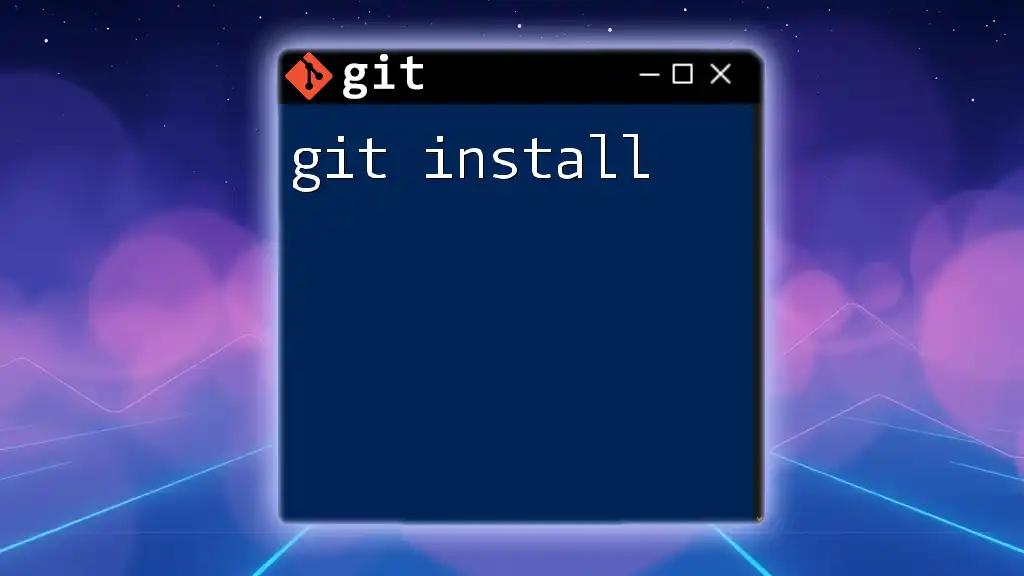
Intermediate Git Actions
Branching in Git
Branches are a fundamental concept in Git, allowing developers to create multiple lines of development within a project. This isolates features and bug fixes, facilitating experimentation without affecting the main codebase.
Creating a New Branch: To create a new branch, you would use:
git checkout -b <branch-name>
For example:
git checkout -b feature-new-login
Switching Branches: You can switch between branches easily with:
git checkout <branch-name>
If you want to switch to `main`, you'd use:
git checkout main
Merging Branches
When you're ready to integrate features or fixes from one branch into another, you'll need to merge them. The `git merge` command combines the changes from different branches into a single branch.
Code Snippet:
git merge <branch-name>
It’s often recommended to merge from your feature branch into `main`, as follows:
git merge feature-new-login
This will bring the changes from `feature-new-login` into your current branch.
Resolving Merge Conflicts
Merge conflicts can occur when two branches have changes to the same line of a file or when one branch has deleted a file that the other branch has modified. You'll need to resolve these conflicts manually.
Typically, Git will highlight areas of conflict within the files. You must then edit the file to reconcile the differences and mark it as resolved:
git add <resolved-file>
git commit -m "Resolved merge conflict in <file-name>"
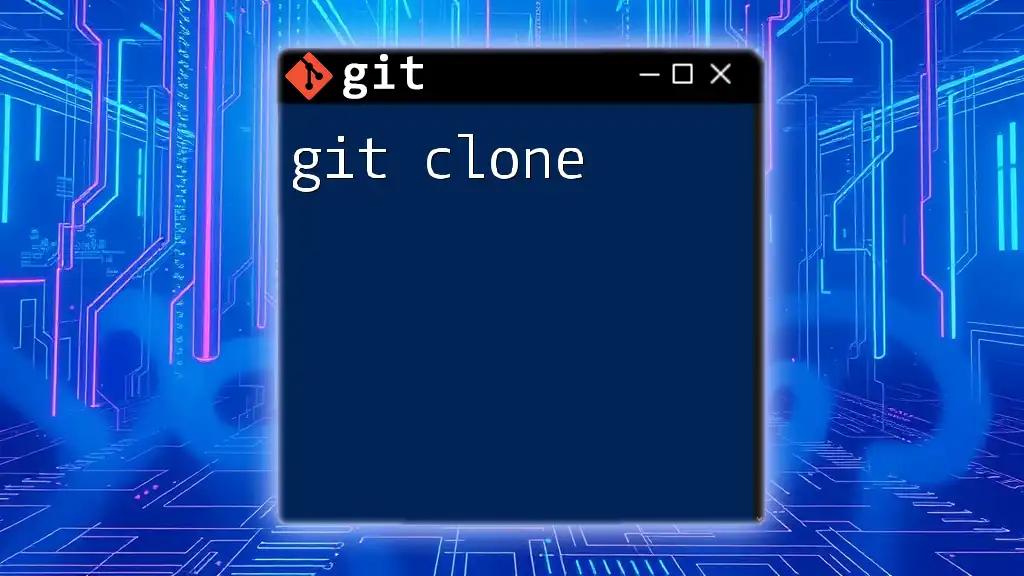
Advanced Git Actions
Stashing Changes
Stashing allows you to temporarily save uncommitted changes, letting you switch contexts without having to make a commit. This is particularly useful when you need to perform a quick task without disturbing your current work.
Code Snippet:
git stash
Later, you can retrieve your stashed changes with:
git stash apply
Reverting Changes
If you need to undo changes that have already been committed, you can use the `git revert` command. This creates a new commit that undoes the changes made by the specified commit.
Code Snippet:
git revert <commit-hash>
For example:
git revert abc1234
This is a safe way to reverse changes while preserving the project's history.
Resetting Changes
The `git reset` command is powerful and can be risky if not used carefully. It can modify the history of commits. There are several types of resets:
- Soft Reset: Moves HEAD but keeps changes in the working directory.
- Mixed Reset: Moves HEAD and indexes changes back to the working area.
- Hard Reset: Moves HEAD and discards all changes in the working directory.
Code Snippet for Hard Reset:
git reset --hard <commit-hash>
Be cautious with this command, as it can lead to irreversible data loss.
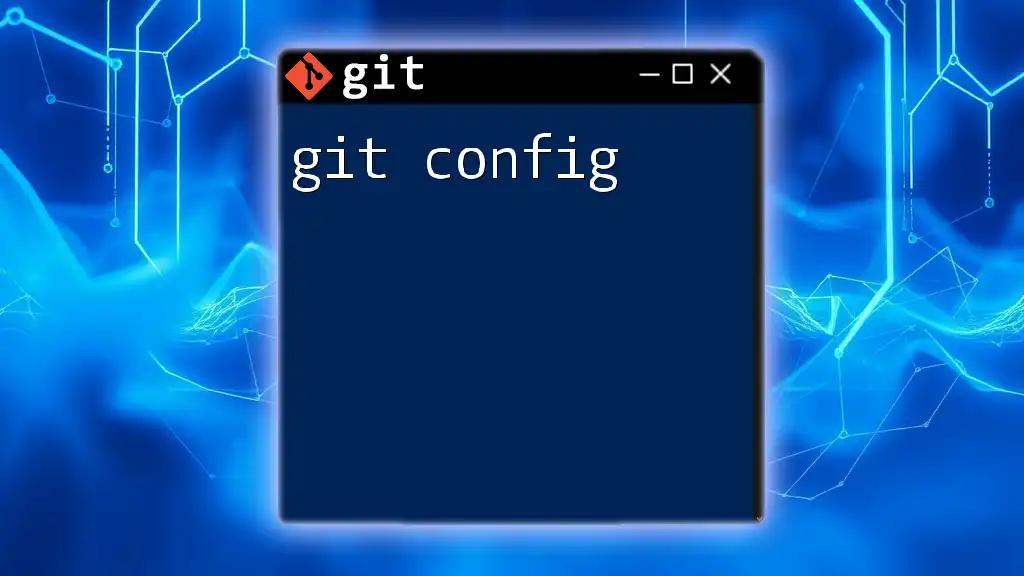
Additional Git Actions
Viewing History and Logs
To review the repository's history, you can use the `git log` command. This shows a list of all commits along with their messages, authors, and dates.
Code Snippet:
git log --oneline
This command will present a brief view of the commit history, making it easier to browse through significant changes.
Checking the Status of Your Repository
The `git status` command provides vital information on your current branch and any uncommitted changes.
Code Snippet:
git status
This will inform you of any modified, staged, or untracked files, helping you manage your repository effectively.
Tagging in Git
Tags are crucial for marking specific points in the repository’s history, often used for releases. Creating a tag helps you identify particular versions of your project.
Code Snippet:
git tag -a <tag-name> -m "Tag message"
For example:
git tag -a v1.0 -m "Version 1.0 release"
Tags provide a way to reference important states of your project easily.
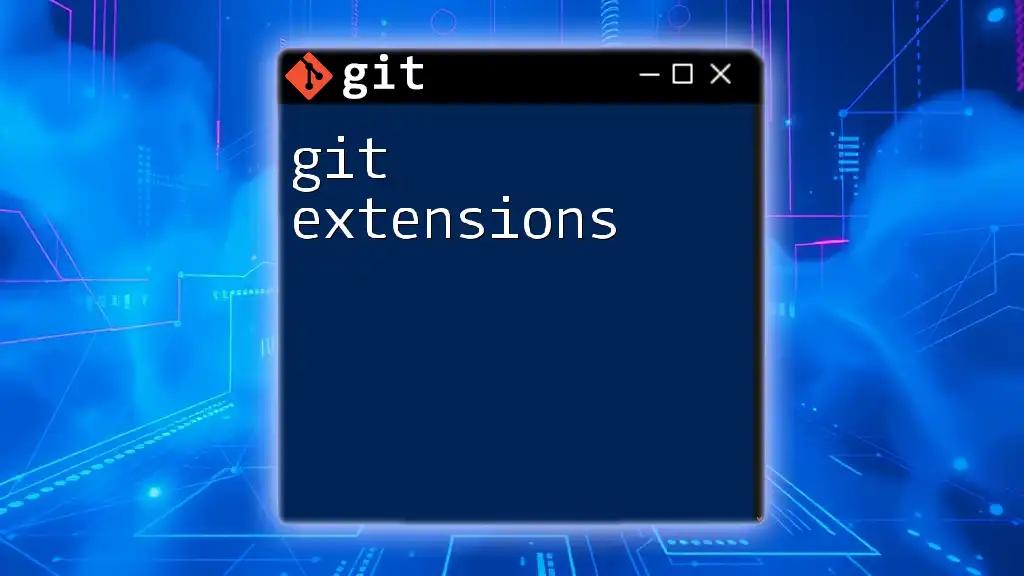
Git Workflows
Common Git Workflows
Understanding different Git workflows can greatly enhance collaboration. Two popular workflows are feature branching and GitFlow. Feature branching allows developers to create a separate branch for each feature, promoting independent development. GitFlow incorporates more structured management of releases and hotfixes.
Best Practices for Using Git Actions
To maximize the effectiveness of Git actions, here are a few recommended practices:
- Commit Frequently: Small, incremental commits allow for easier tracking of changes.
- Write Clear Commit Messages: Clarity in commit messages fosters better collaboration.
- Keep Your Branches Organized: Delete unnecessary branches to avoid clutter.
By avoiding common pitfalls like committing large changes without context, you will maintain a clean and navigable Git history.
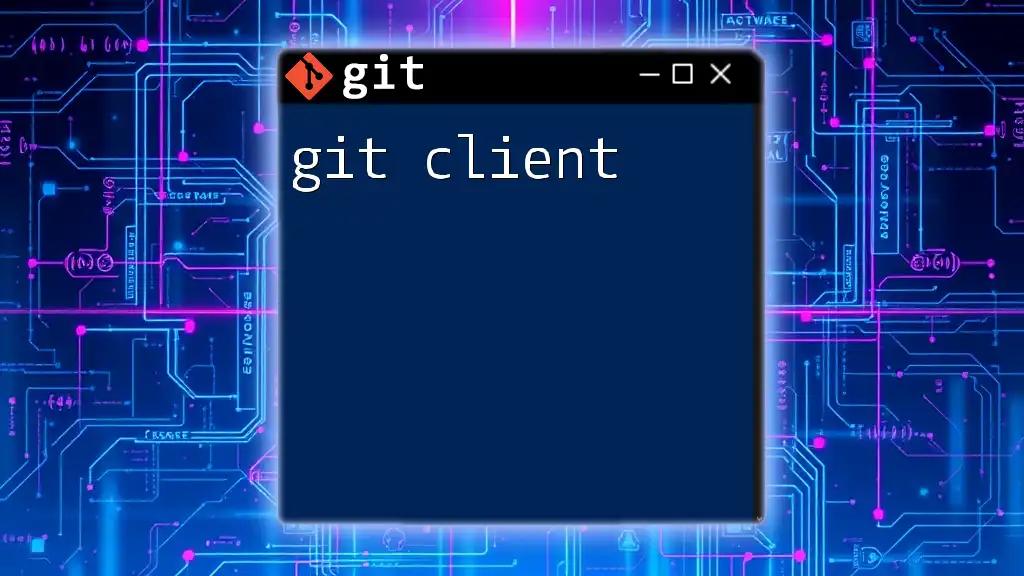
Conclusion
Understanding and utilizing Git actions is essential for anyone involved in software development. By mastering commands for cloning, committing, pushing, pulling, merging, and more, you can efficiently manage your codebase and collaborate effectively with others.
Encouragement to Practice
Now that you have a comprehensive guide to Git actions, it’s time to put this knowledge into practice. Experiment with your own repositories or contribute to existing projects to solidify your understanding of these essential commands.
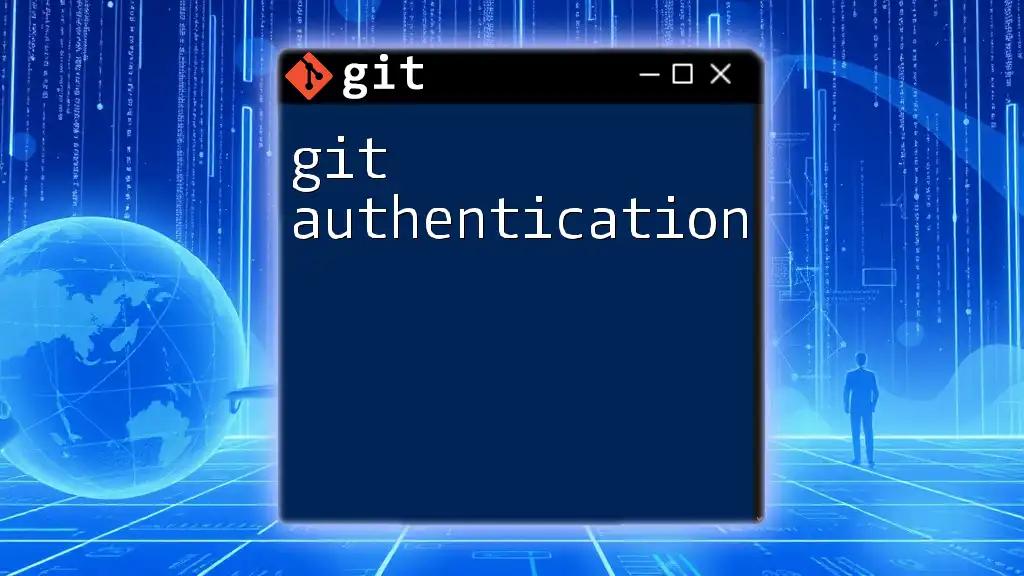
Resources and Further Reading
Recommended Tools
Explore various Git GUI clients and integrations that can simplify your interactions with Git.
Helpful Documentation Links
Take advantage of official Git documentation and guides for deeper insights into the tool.
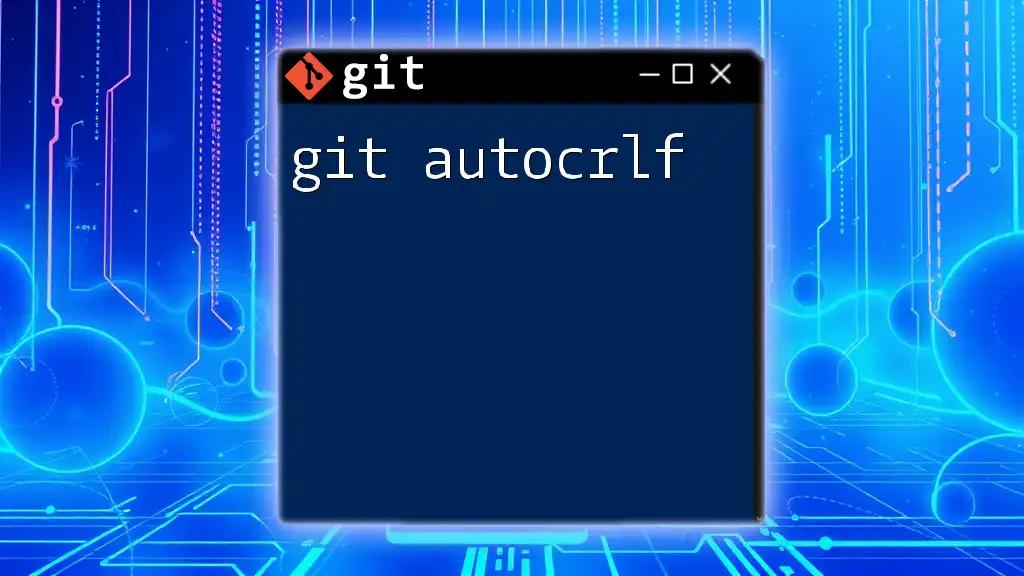
Call to Action
Join Our Git Course! If you’re looking to enhance your Git skills and learn how to use Git commands quickly and effectively, consider enrolling in our specialized courses today. Let’s master Git together!