Git authentication is the process of validating your identity to allow access to a Git repository, commonly achieved using SSH keys or HTTPS credentials.
Here's an example of how to set up authentication with an SSH key:
ssh-keygen -t rsa -b 4096 -C "your_email@example.com"
eval "$(ssh-agent -s)"
ssh-add ~/.ssh/id_rsa
Make sure to add the generated SSH key to your Git hosting service (like GitHub or GitLab) to complete the setup.
Understanding Git Authentication
What is Git Authentication?
Git authentication is the process of verifying a user's identity when interacting with Git repositories. This verification is crucial for maintaining the integrity and security of codebases, especially in collaborative environments. Proper authentication ensures that only authorized users can access, modify, or deploy code, which safeguards against unauthorized changes and potential security breaches.
Types of Authentication Methods
There are several common methods of authentication when using Git:
- Username and Password
- SSH Keys
- Personal Access Tokens (PATs)
Each method has its own advantages and security implications, allowing users to choose the one that best fits their workflow.
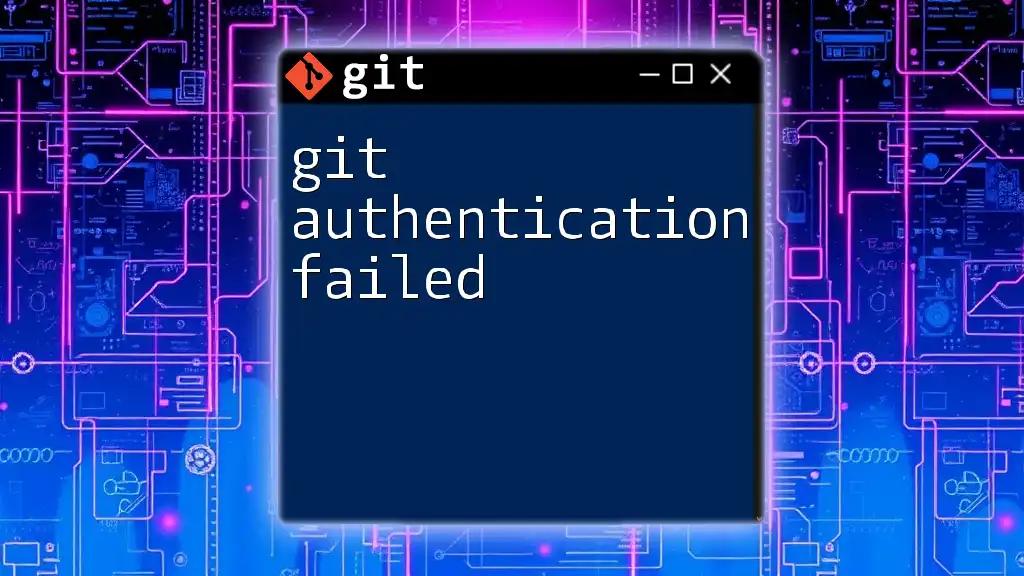
Setting Up Authentication
Username and Password Authentication
When using username and password authentication, you simply provide your Git hosting service credentials upon attempting to clone, push, or pull from a repository.
Basic Commands:
To authenticate with your username and password, you can use the following command:
git clone https://username@repository-url.git
In this command, replace `username` with your actual username and `repository-url` with the URL of your Git repository.
Security Implications: Keep in mind that storing passwords in plain text can lead to security risks. Many platforms, including GitHub, recommend switching to more secure authentication methods like SSH keys or Personal Access Tokens, especially for long-term use.
SSH Key Authentication
What are SSH Keys?
SSH keys are a pair of cryptographic keys that provide a more secure way of logging into your Git repository than traditional username and password methods. This system uses a private key that remains on your machine and a public key that gets added to your Git hosting service.
Generating SSH Keys
To generate your SSH keys, follow these simple steps:
- Open your terminal.
- Enter the following command to create a new SSH key:
ssh-keygen -t rsa -b 4096 -C "your_email@example.com"
This command generates a new SSH key using the provided email as a label.
You'll be prompted to choose a location to save the key (default is `~/.ssh/id_rsa`). You can also set a passphrase for an extra layer of security.
Adding SSH Keys to SSH Agent
Next, you need to ensure your SSH key is added to the SSH agent, enabling it to manage your keys automatically. Use these commands:
eval "$(ssh-agent -s)"
ssh-add ~/.ssh/id_rsa
This will add your private SSH key to the agent, allowing you to authenticate without needing to re-enter your passphrase repeatedly.
Adding Your SSH Key to Your Git Hosting Service
After generating your SSH key, you'll need to add the public key to your Git hosting service. This process can vary between services, but generally includes:
- Copying your public key with:
cat ~/.ssh/id_rsa.pub
- Navigating to the SSH settings on your Git provider's website (such as GitHub, GitLab, or Bitbucket).
- Pasting the public key into the designated area.
By doing this, you authorize your machine to communicate with your Git repository securely.
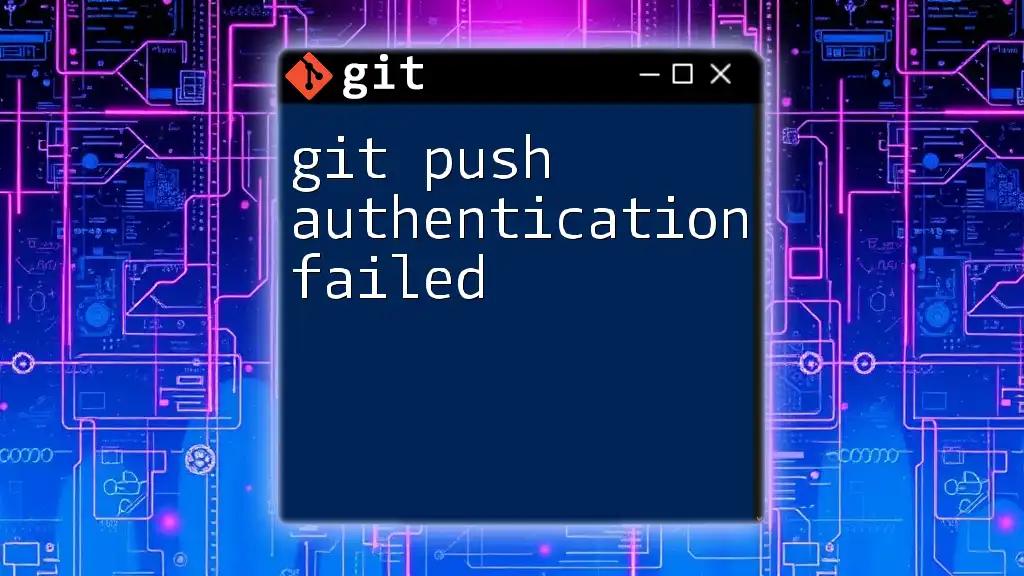
Using Personal Access Tokens (PATs)
What are Personal Access Tokens?
Personal Access Tokens (PATs) are an alternative to using passwords for Git operations. They provide a way to authenticate with Git hosting services without exposing your actual password, adding a layer of security.
Generating a Personal Access Token
Creating a PAT is straightforward. Here’s how to do it:
- Log into your Git hosting service account.
- Navigate to the settings or developer settings section.
- Look for the Personal Access Tokens option and click on it.
- Select the scopes (permissions) you want the token to have based on your needs.
- Generate the token and make sure to copy it, as you won’t be able to retrieve it later.
Using PAT in Git Commands:
You can use the following command to access your repository with a PAT:
git clone https://username:token@repository-url.git
Replace `username` with your Git username, `token` with your personal access token, and `repository-url` with your repository address.
When to Use Personal Access Tokens
Personal Access Tokens are especially useful in scenarios where:
- Your organization requires enhanced security practices.
- You want to automate processes using scripts without hardcoding your password.
- You wish to grant limited access to third-party applications.
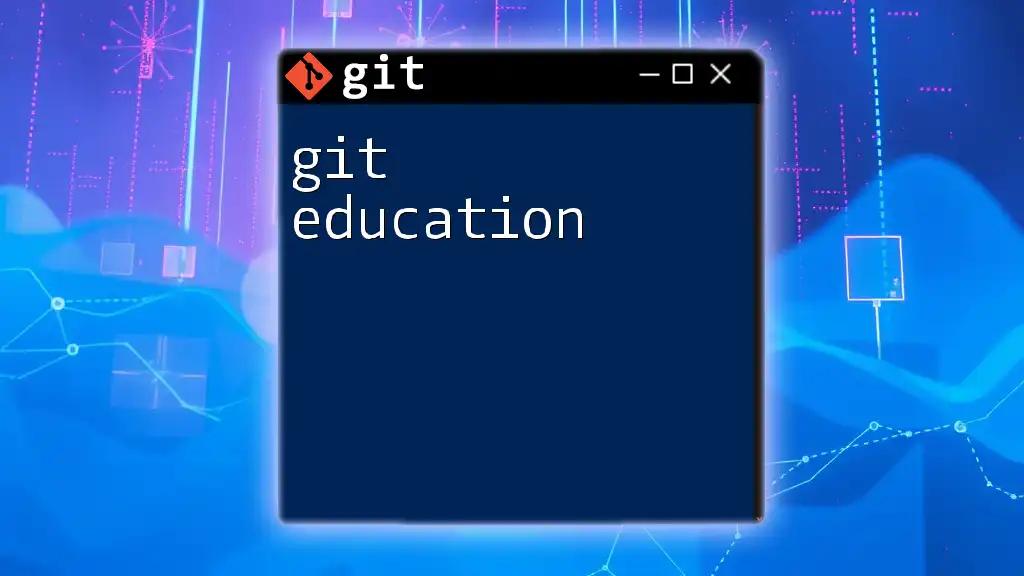
Best Practices for Git Authentication
Keeping Your Credentials Secure
Storing credentials securely is vital. Here are a few recommended practices:
- Use credential managers provided by your operating system or Git client to save your credentials securely.
- Avoid saving your credentials in plain-text files or scripts.
Regularly Rotating Authentication Keys and Tokens
Rotating your keys and tokens periodically minimizes the risk of unauthorized accesses. If you suspect that your keys have been compromised, revoke them immediately and regenerate new keys or tokens.
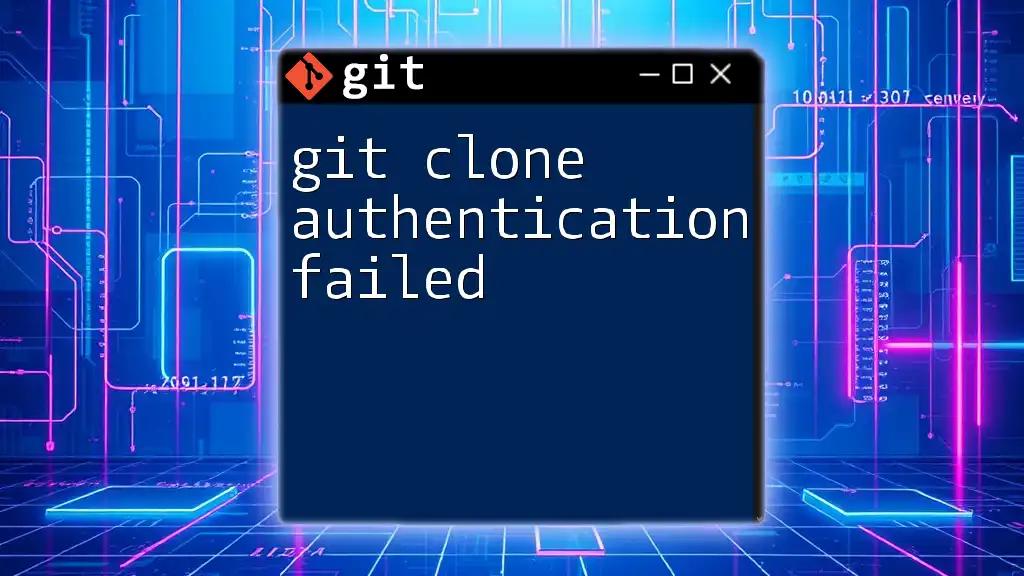
Troubleshooting Common Authentication Issues
Common Errors and Solutions
Even with proper setup, you may encounter various authentication problems. Here are some common issues and their solutions:
- Authentication failed errors: Often caused by incorrect credentials or lack of proper permissions on the repository.
- Permission denied (publickey): Indicates that your public key isn’t recognized by the server, potentially due to it not being added to your Git hosting service.
- Invalid credentials: Check for any typos in your username and token. Make sure the token is not expired.
Helpful Git Commands for Debugging
For troubleshooting potential issues, you can run the following commands:
- To check if your SSH configuration is working correctly:
ssh -T git@github.com
This should respond with a message confirming your successful authentication.
- To clear any cached credentials:
git credential-cache exit
This command will help resolve any issues stemming from cached credentials.
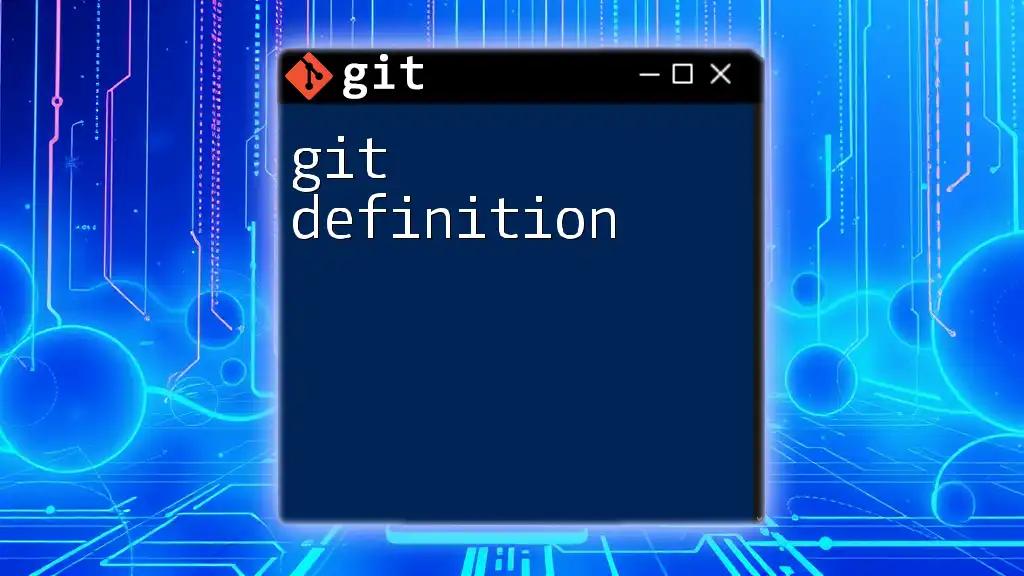
Conclusion
Recap of Key Points
We’ve explored Git authentication, highlighting the various methods including username/password, SSH keys, and Personal Access Tokens. Each method serves unique security and convenience purposes, allowing users to choose what fits their workflow best.
Encouragement for Best Practices
Maintaining robust security practices surrounding your Git authentication will go a long way in preserving the integrity of your projects. Regularly review and update your methods to ensure you protect your codebases effectively.
Additional Resources
To continue learning about Git authentication, consult the official documentation for your preferred Git hosting service and consider exploring online resources and tutorials that delve deeper into Git practices.