Git education empowers individuals to efficiently manage version control through concise commands and practical examples. Here's a basic command to get started with Git:
git init
Understanding Version Control
What is Version Control?
Version control systems (VCS) are essential tools in modern software development, allowing teams to manage changes to code over time. A brief history reveals that early systems were centralized, with all changes stored in one location. As collaboration became more critical in development processes, the need for a more flexible system gave birth to distributed version control systems (DVCS).
The significance of using a VCS lies in its ability to track changes, allowing developers to revert to previous versions, collaborate without overwriting each other’s work, and maintain a record of their development history. This functionality ensures that software projects remain organized and efficient.
Types of Version Control Systems
In the landscape of version control, we typically encounter two main types:
-
Centralized Version Control Systems (CVCS): With CVCS, like Subversion (SVN), the repository is stored on a single server. Developers commit their changes to this central repository, which simplifies some aspects of project management but introduces risks with reliance on a single point of failure.
-
Distributed Version Control Systems (DVCS): Git excels as a DVCS because each developer has a full copy of the repository, including its history. This setup allows for offline work, increased resilience, and easier branching and merging processes.
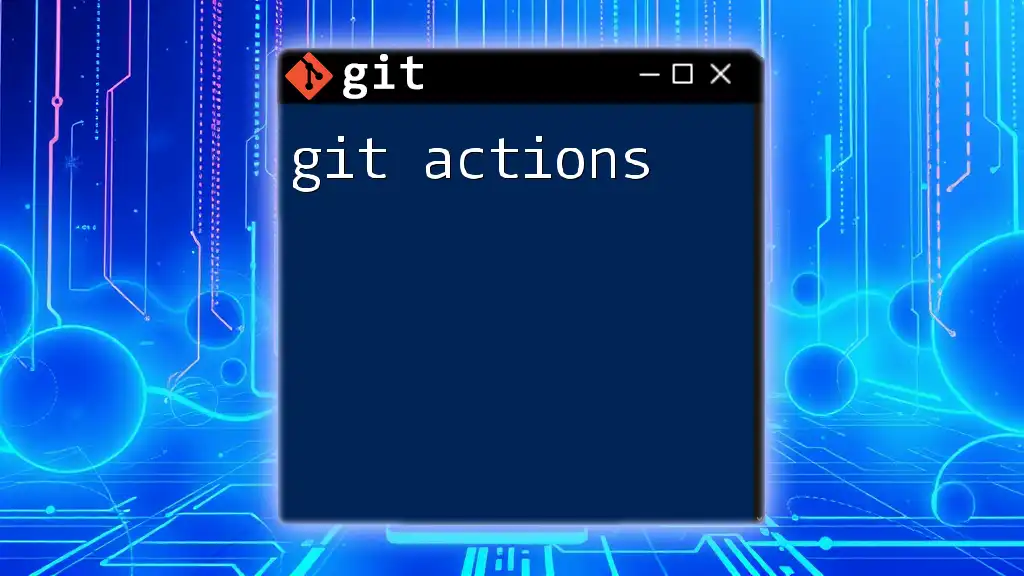
Introduction to Git
What is Git?
Git is a powerful and widely-used version control system that provides robust functionality for tracking changes in source code. It is designed to handle projects of any size and has features that enhance performance and scalability. Key advantages of using Git include:
- Speed: Git commands are designed for fast execution, offering quick access to commit history and file versions.
- Branching and Merging: Git encourages a branching workflow, which allows developers to separate work on different features, bugs, or experiments without affecting the main codebase.
- Collaboration: As a distributed system, Git makes collaboration more manageable, facilitating contributions from multiple developers seamlessly.
Git Terminology for Beginners
Understanding Git involves familiarizing yourself with essential terms:
-
Repository (Repo): A repository is a storage location where your project files, including its version history, reside. Repositories can be local (on your machine) or remote (hosted online, such as on GitHub).
-
Commit: A commit represents a snapshot of your project at a specific point in time. It's used to save changes to the repository. For example:
git commit -m "Initial commit with project setup"
-
Branch: A branch allows you to diverge from the main line of development and work on different features or fixes independently. This feature provides a way to experiment without disturbing the live codebase.
-
Merge: Merging combines changes from different branches. It's an essential part of collaborative work and allows you to integrate your changes with those made by others.
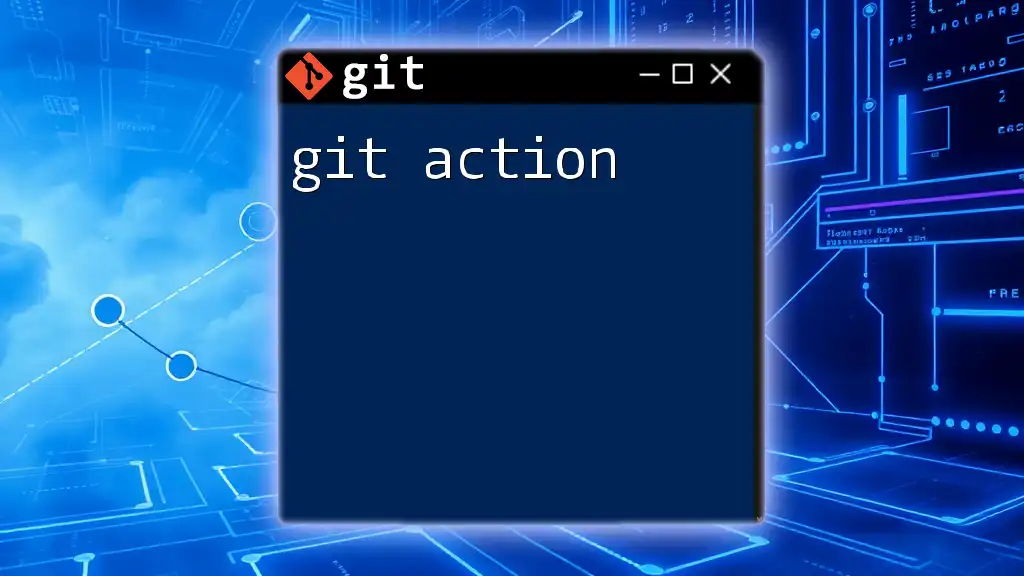
Installation and Setup
How to Install Git
Installing Git is straightforward and can be done on various operating systems:
- Windows: Download the Git installer from the official Git website and run it. Follow the installation prompts, choosing your preferred options (like enabling Git Bash).
- macOS: You can install Git using Homebrew by running:
brew install git
- Linux: Use your package manager to install Git, e.g., for Debian-based systems:
sudo apt-get install git
After installation, verify that Git is installed correctly by executing:
git --version
Configuring Git
Once installed, setting up Git involves configuring a few key settings:
- To set your user name and email, use the following commands:
Note: Using `--global` applies these settings to all repositories on your machine, while omitting it applies them to the current repository only.git config --global user.name "Your Name" git config --global user.email "you@example.com"
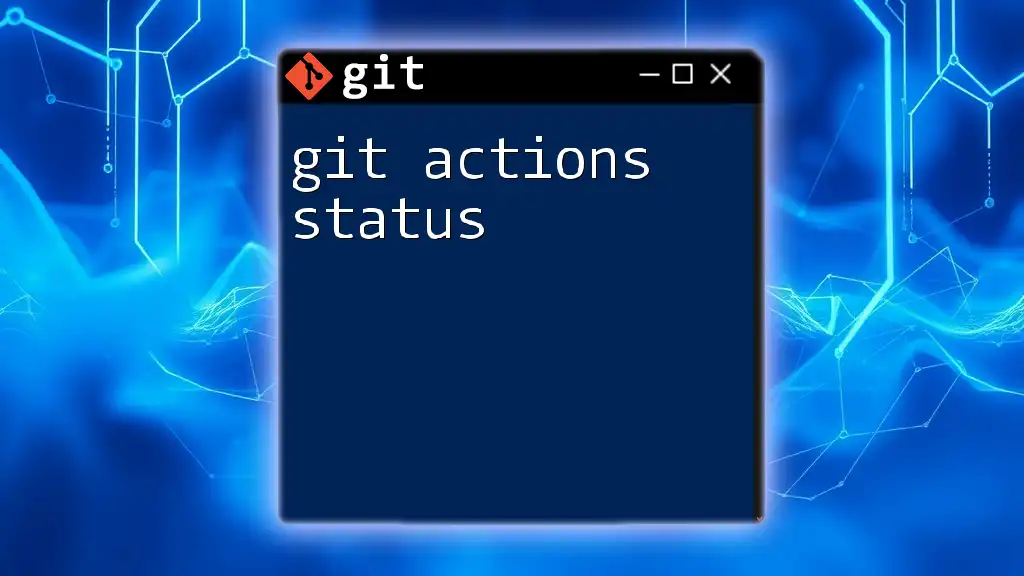
Basic Git Commands
Creating a Repository
To create a new repository, navigate to your project folder and run:
git init my-repo
This command initializes a new Git repository, allowing you to start tracking your project.
Cloning an Existing Repository
When you want to work on an existing project, you can clone it using:
git clone https://github.com/user/repo.git
This command creates a local copy of the repository, along with its complete history.
Adding Files
To begin tracking changes to files, use the `git add` command:
git add filename.txt
This stages the specified file, preparing it to be committed. You can also add all changes at once with:
git add .
Committing Changes
Committing changes is fundamental to Git. After staging your changes, you need to commit them:
git commit -m "Add a new feature"
Important: Write clear, concise commit messages to convey what changes were made.
Viewing Status and Logs
To check the current status of your repository, utilize:
git status
This command informs you of which files have been modified, added, or deleted.
When you want to see your commit history, run:
git log
This command displays a list of commits along with their messages, authors, and timestamps.
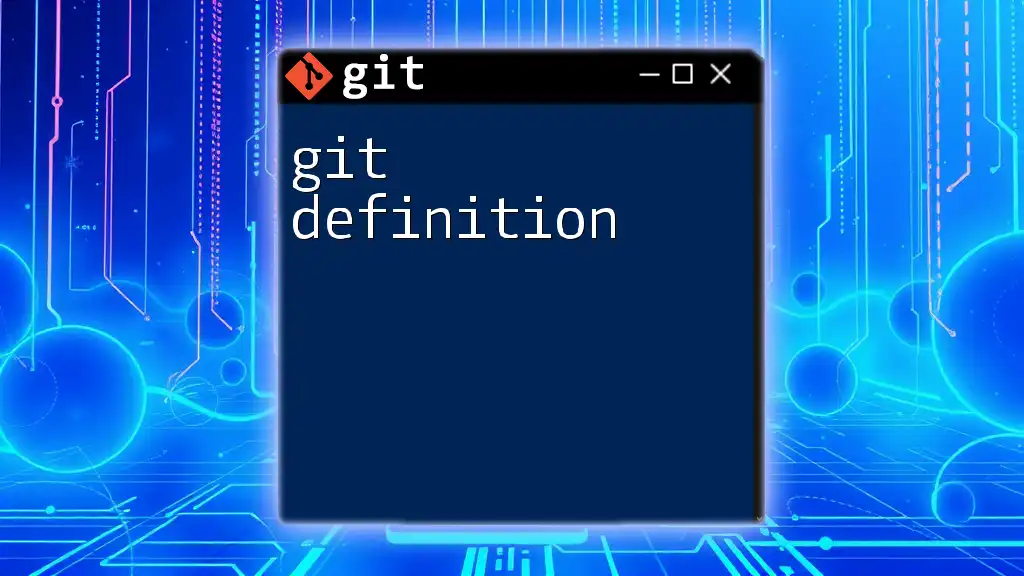
Branching and Merging
Understanding Branches
Branches are a core concept in Git, providing developers with the flexibility to work on multiple tasks simultaneously. They allow you to isolate your feature development without interfering with the main codebase.
Creating and Switching Branches
To create a new branch and switch to it, use:
git checkout -b new-feature
This command creates a branch named `new-feature` and switches your working directory to this new branch, making it easy to begin work without altering the main branch.
Merging Branches
After you've completed work on a branch, you can merge it into another branch (usually `main`) with:
git checkout main
git merge new-feature
It's crucial to validate that no merge conflicts arise. If conflicts occur, Git prompts you to resolve them manually.
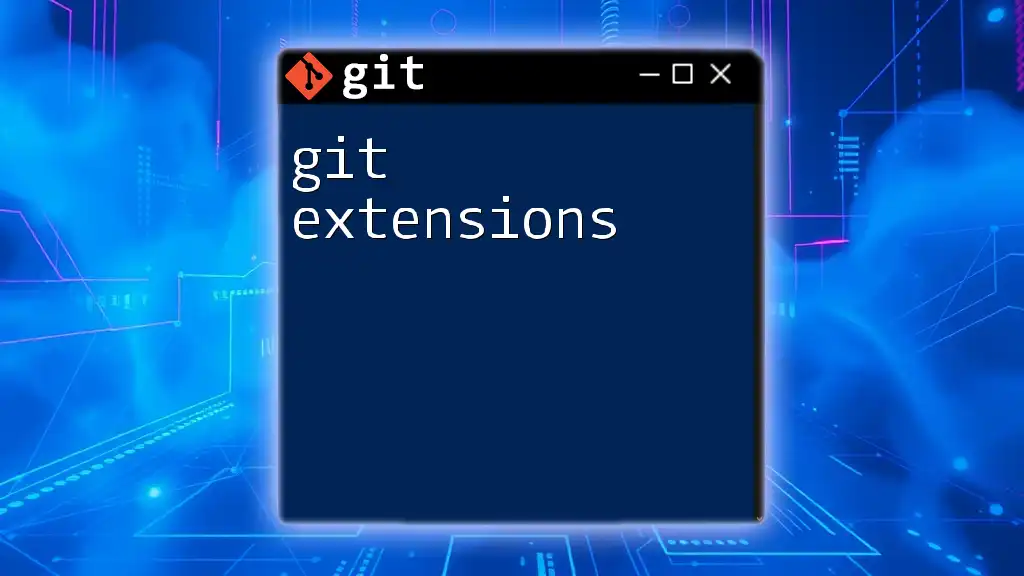
Remote Repositories
Adding a Remote Repository
To collaborate with others, you'll often need to work with a remote repository. Add a remote repository with:
git remote add origin https://github.com/user/repo.git
By doing this, you link your local repository to the remote location.
Pushing Changes
Modifying code locally is essential, but you must share these changes using:
git push origin main
This command uploads your committed changes to the specified remote branch.
Pulling Changes
To keep your local repository up to date with remote changes, utilize:
git pull origin main
This command fetches changes from the remote repository and merges them into your local branch.
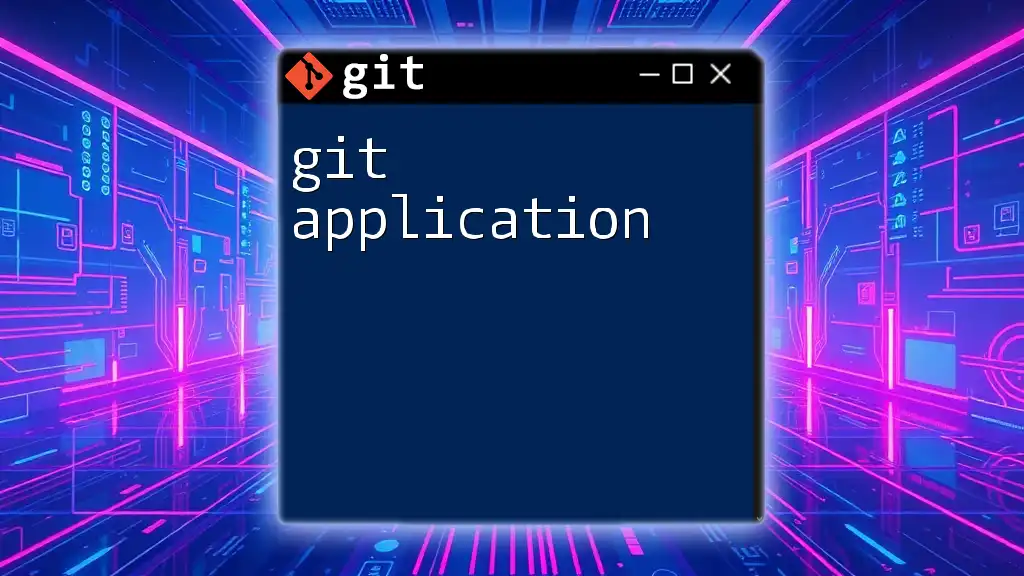
Advanced Git Commands and Techniques
Git Stashing
When you need to switch contexts but aren't ready to commit your changes, Git offers a feature called stashing. Save your current changes and revert to a clean working directory with:
git stash
You can later restore your stashed changes with:
git stash pop
Git Tags
Tags are used to mark specific points in your repository's history as significant, often for releases. Create a tag with:
git tag v1.0
This command marks your current commit with a version number, which is useful for software releases.
Rebasing
Rebasing offers a way to integrate changes from one branch into another more cleanly than merging. Use it to maintain a linear project history:
git rebase branch-name
While rebasing can simplify history, be careful to use it on local branches that haven't been pushed to a remote yet to avoid complicating collaboration.
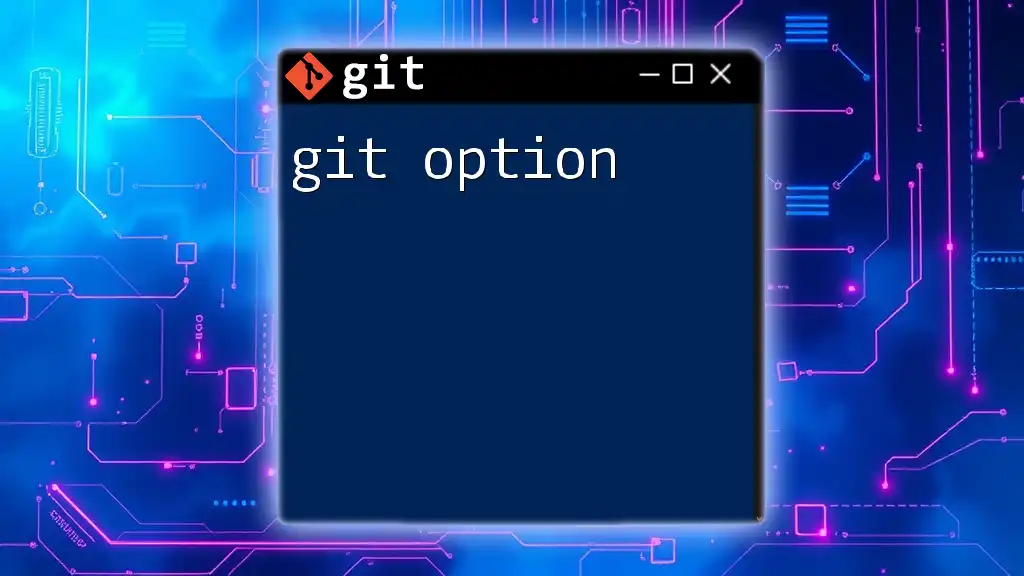
Best Practices for Using Git
To ensure efficient use of Git, developers should:
- Write clear and meaningful commit messages summarizing what changes were made.
- Commit code regularly to keep the history manageable and avoid large, complicated commits.
- Organize branches logically, adopting naming conventions that reflect the purpose of the work (e.g., feature branches, bugfix branches).
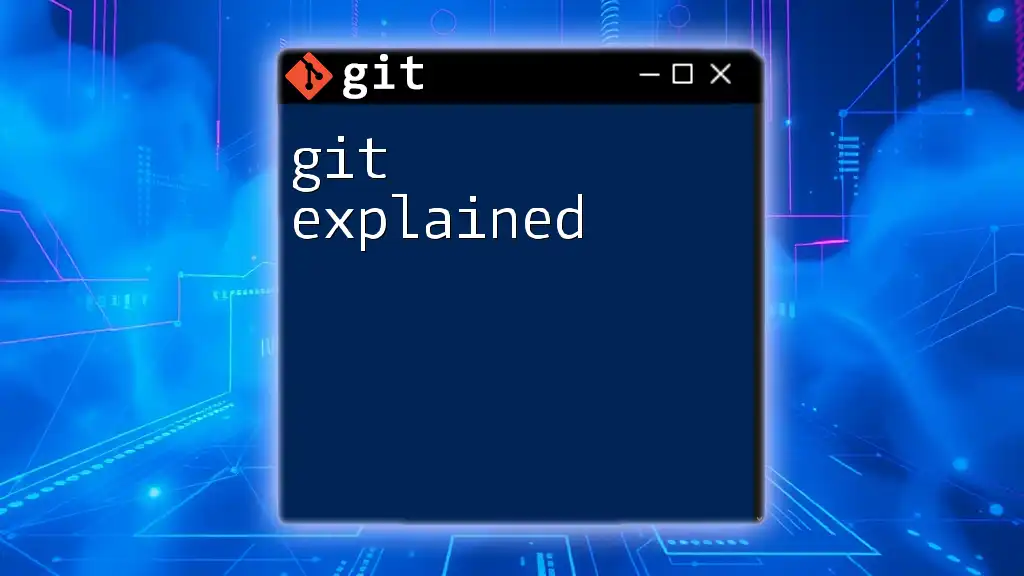
Conclusion
Git education is vital for anyone involved in software development. Understanding Git not only enhances your coding skills but also equips you to collaborate more effectively with others. By mastering the commands and concepts outlined in this guide, you'll be well on your way to leveraging Git to enhance your projects.
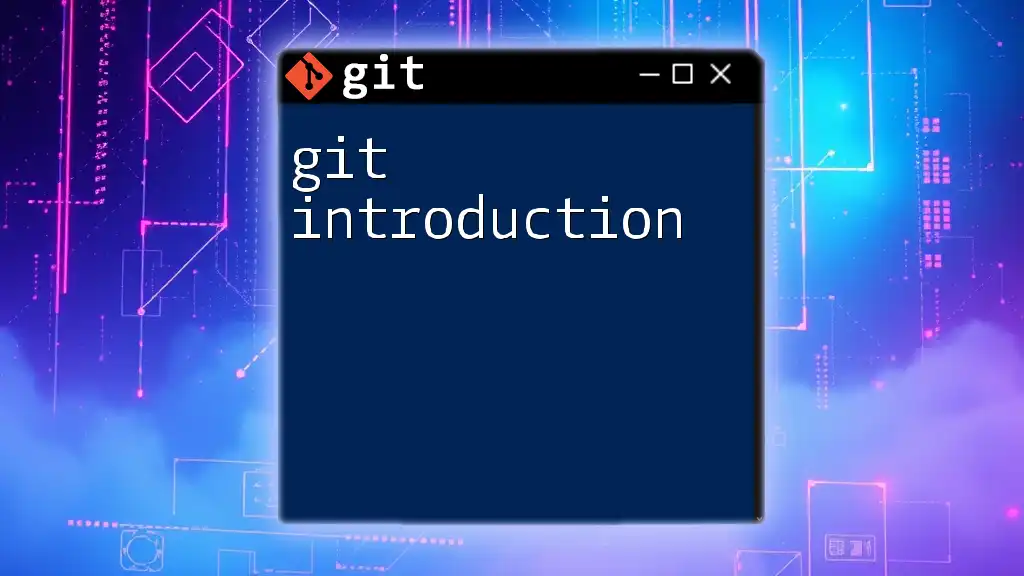
Additional Resources
For further learning, consider exploring the official Git documentation, reputable online courses, or open-source communities. Engaging with these resources will deepen your understanding of Git and its numerous capabilities.