The `git version` command displays the current version of Git installed on your system.
Here's how to use it:
git --version
Understanding Git Version
What is Git Version?
Git versioning refers to the ability of Git to manage changes to files over time. This system allows developers to save multiple snapshots of their project, making it easier to track the history of changes and collaborate effectively. Understanding how Git handles versioning is crucial for any developer, as it not only helps in organizing the development process but also provides a safety net for undoing mistakes and reverting to prior stages of the project.
The Role of Versioning in Git
Version control is a core component of modern software development. It allows multiple developers to work on the same project without overwriting each other's changes or losing work. Git versioning achieves this through systematic management of the changes made to code and project files, enabling teams to develop features individually and integrate them seamlessly.
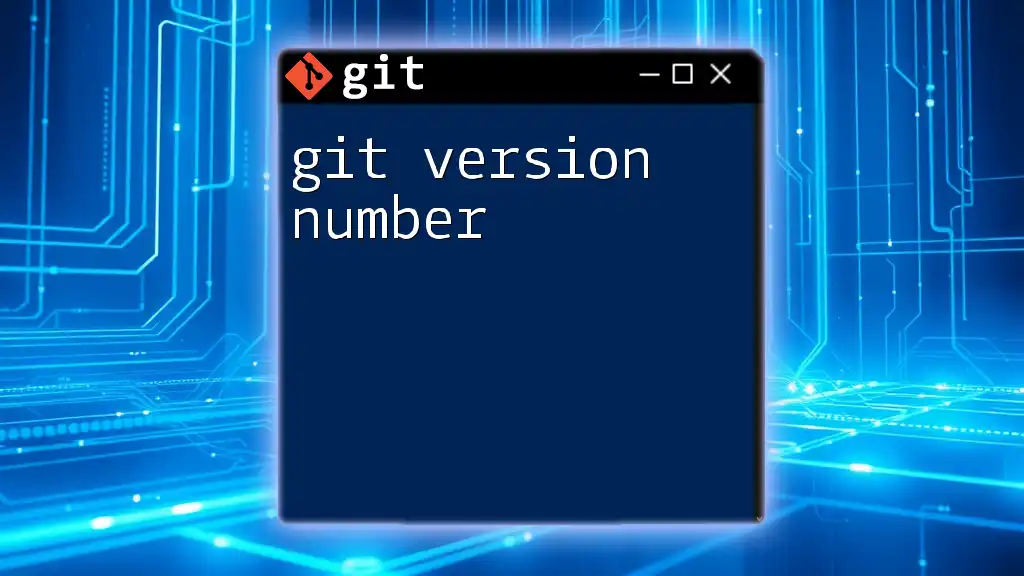
Key Concepts in Git Versioning
Commits
In Git, a commit represents a snapshot of your project at a particular point in time. Think of a commit as a save point in a video game; you can return to it at any time. Each commit is accompanied by a message that describes the changes made, which is vital for maintaining clarity in the project’s history.
Good commit messages generally follow the structure of:
- A brief summary of the changes (usually 50 characters or fewer)
- A deeper explanation, if necessary, provided in the body of the message (72 characters per line)
Example of Good vs. Bad Commit Messages:
- Bad: "fixed stuff"
- Good: "Fixed issue with user login handling"
To create a commit, you use the following command:
git commit -m "Describe your changes here"
Branches
Branches in Git allow you to diverge from the main line of development, enabling you to work on features or fixes in isolation. This way, you can experiment or enhance your project without impacting the live version.
To create a new branch and switch to it, use:
git checkout -b new-feature
Utilizing branches is critical in collaborative environments where multiple developers may be working on different aspects of the project simultaneously. It allows for efficient merging of features once they are completed and tested.
Tags
Tags in Git signify specific points in your project’s history, usually marking release versions. They serve as identifiers for significant milestones in the project.
There are two types of tags:
- Lightweight tags: Simplified markers that only refer to a commit.
- Annotated tags: Full objects in the Git database including the tagger's name, email, and date, along with a tagging message.
To create an annotated tag, you can use:
git tag -a v1.0 -m "Version 1.0 Release"
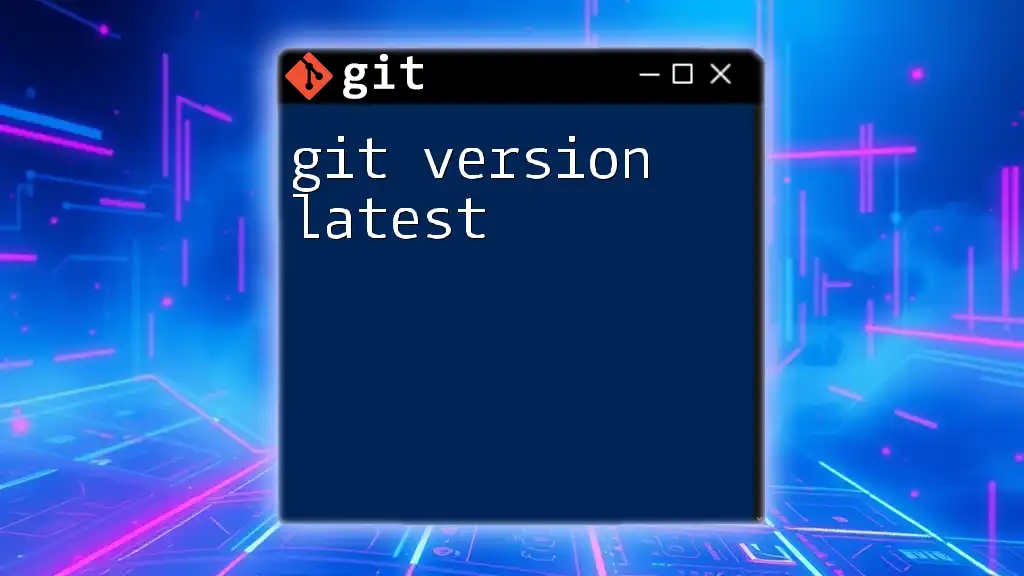
Managing Versions with Git
Viewing Version History
You can view the complete history of commits in your repository with:
git log
This command presents a chronological view of your project's commits, including each commit’s hash, author, date, and message. Understanding this output is key to navigating your project’s development history.
Comparing Versions
Comparing different commits is crucial for evaluating changes and ensuring quality. The `git diff` command allows you to see differences between commits, branches, or the working directory versus the last commit.
For example, to compare two specific commits:
git diff commit1 commit2
This command outputs the changes between the two versions, facilitating a thorough review process.
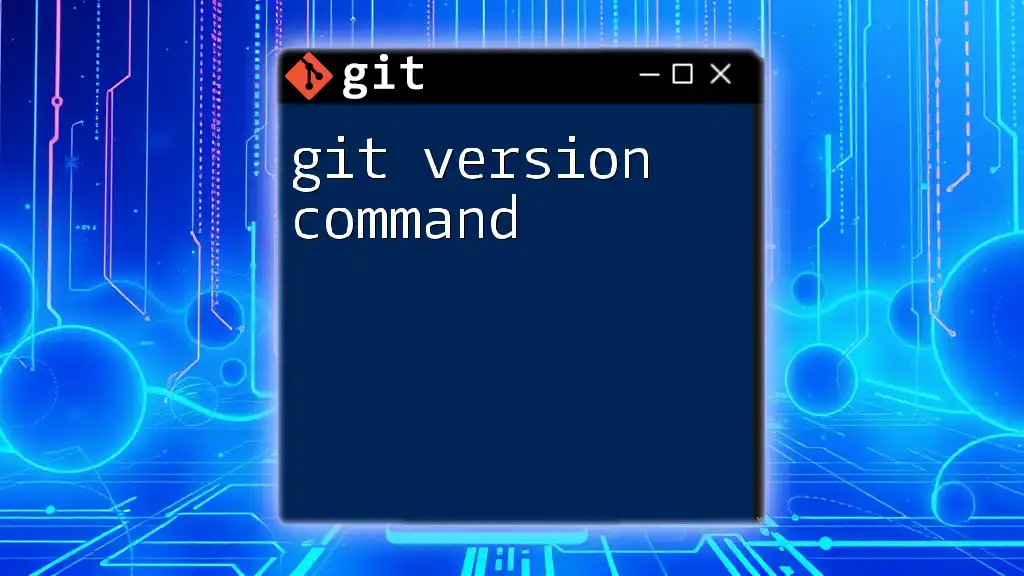
Best Practices for Versioning in Git
Commit Frequently
One of the best practices in Git is to commit often. Frequent commits maintain granular control over your project history, making it easier to pinpoint where issues arose. Moreover, small, manageable commits allow for straightforward rollbacks and reviews.
Keep History Clean
A clean Git history is essential for understanding project evolution. Maintaining this cleanliness involves avoiding overly verbose commits and using strategies like interactive rebase to squash related commits before merging them into the main branch.
Consistent Branching Strategy
Implementing a structured branching strategy enhances clarity and efficiency in collaborative settings. Common models, such as Git Flow or feature branching, help in organizing work and maintain focus, ensuring that each feature is developed independently before integration.
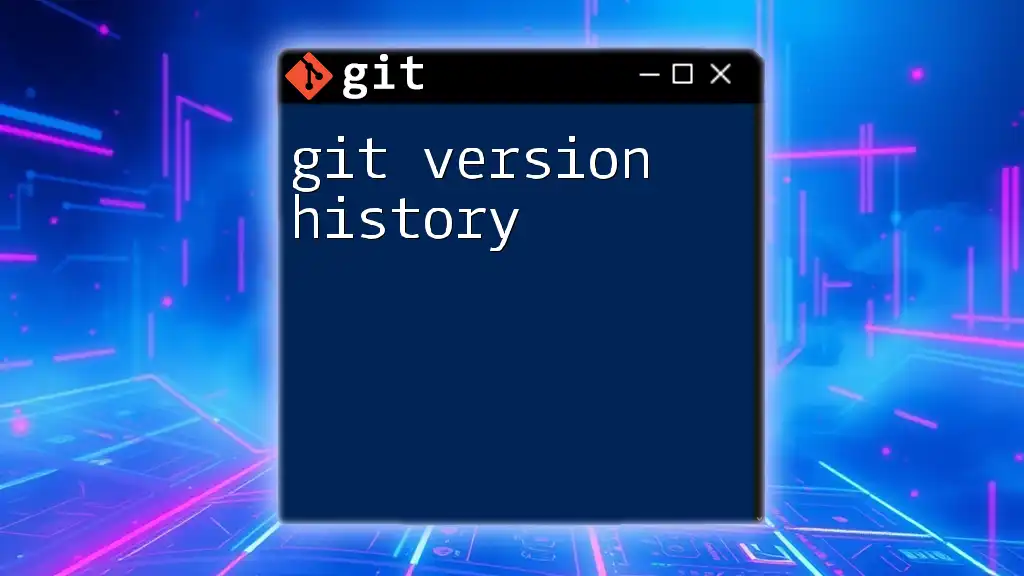
Advanced Versioning Techniques
Reverting Changes
If a particular commit resulted in issues or regressions, you can easily revert it using the following command:
git revert commit_id
This creates a new commit that undoes the changes made by the specified commit, preserving the commit history for future reference.
Undoing Mistakes
Mistakes happen. Whether you've committed the wrong changes or need to reset to an earlier state, Git provides commands to help revert to previous stages effectively. You can undo commits using:
git reset --hard HEAD~1 # Revert to the previous commit
This command discards the last commit along with any changes made, restoring your working directory to the state it was in before that commit.
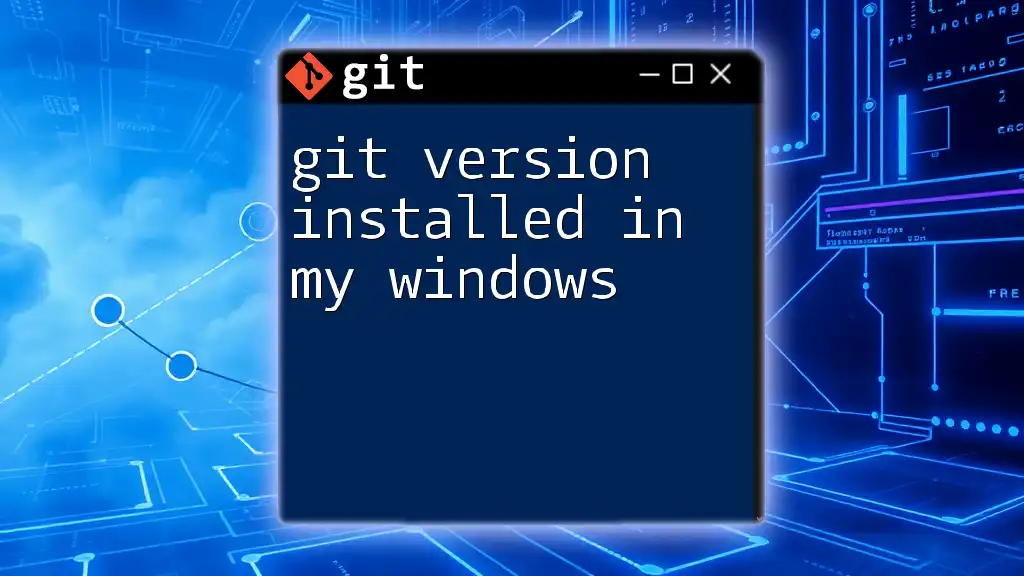
Conclusion
Understanding Git versioning is a fundamental skill for developers working in today’s collaborative environments. By mastering the concepts of commits, branches, and tags, and by adhering to best practices, you'll enhance your workflow and productivity significantly. Embrace the power of Git to improve your version control skills and manage your projects effectively.
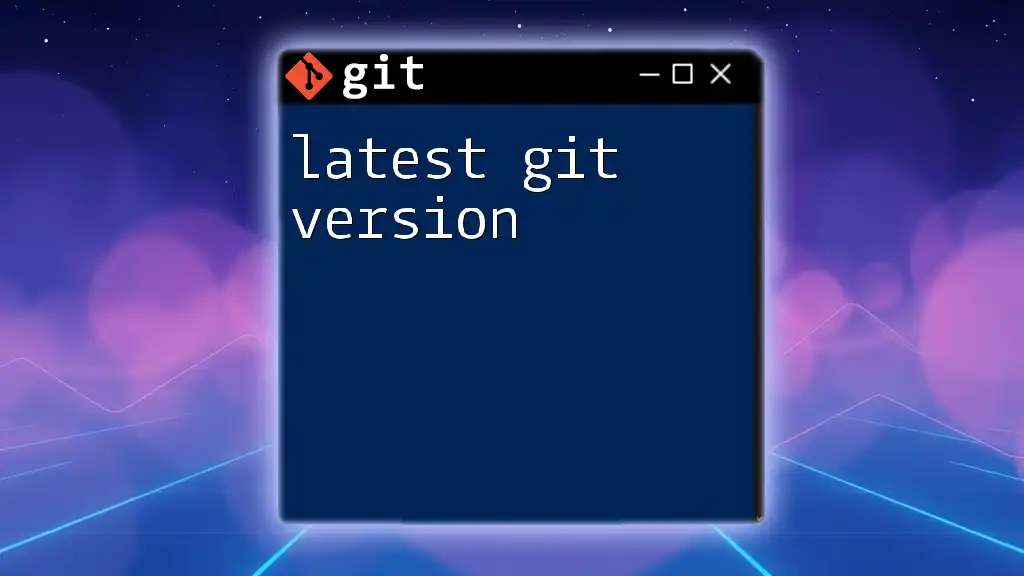
Additional Resources
- For further exploration of Git functionalities, refer to the [official Git documentation](https://git-scm.com/doc).
- Recommended readings include books like "Pro Git" by Scott Chacon and Ben Straub. Online platforms such as Coursera and Udacity also provide comprehensive courses on Git and version control.