The `git config` command is used to set configuration options for Git, enabling you to customize your environment and control various aspects of its behavior.
git config --global user.name "Your Name"
Understanding `git config`
What is `git config`?
`git config` is a powerful command used in Git to configure user and repository settings. By modifying configuration options, you tailor your Git environment to suit your workflow and enhance your development experience. Essentially, `git config` allows you to establish preferences and behaviors that Git will adhere to while managing your code.
Scope of Configuration
Git configurations can be set at three levels, each carrying different scopes:
- Local: Configuration that only affects a single repository. This is useful for project-specific settings.
- Global: Configuration that applies to the user across all repositories on the machine. Ideal for settings you want to maintain consistently.
- System: Configuration that applies to all users on the system. This is often used for institutional defaults.
Understanding these scopes helps you make the most of your configurations and avoid conflicts.
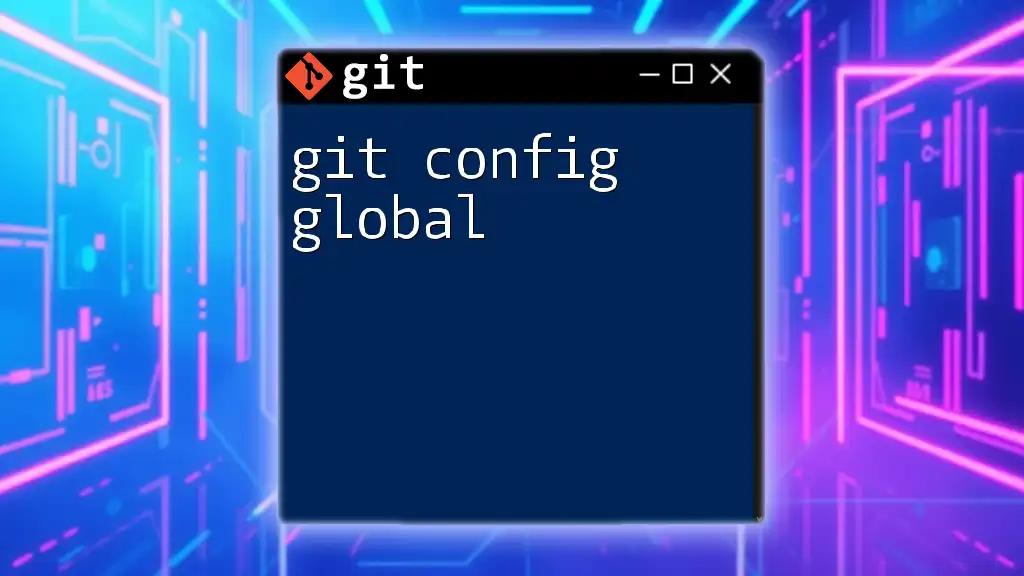
Setting Up `git config`
Installing Git
Before utilizing `git config`, you need to have Git installed on your system. The installation process varies based on your operating system:
- For Windows, download the installer from the official Git website and follow the setup instructions.
- For macOS, you can install it via Homebrew by running `brew install git`.
- On Linux, you can usually install it using your package manager, for example, `sudo apt-get install git` for Ubuntu.
Make sure to verify the installation by running `git --version` in your terminal.
Initializing Configuration
Once Git is installed, it’s time to start configuring it. You can begin by setting your user information and editor preferences. Run the following commands to initialize your configuration:
git config --global user.name "Your Name"
git config --global user.email "you@example.com"
By initializing these settings, you ensure that your commits are accurately attributed to you.
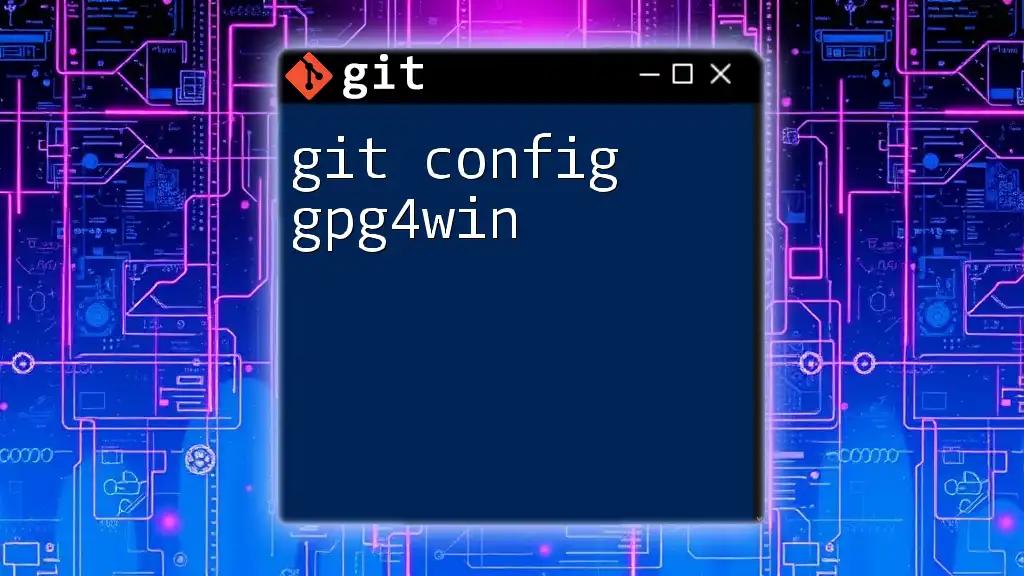
Common Git Config Commands
Setting User Information
One of the first things to configure in Git is your user information. This includes your username and email address. These details are essential because they identify you as the author of your commits. To set this information globally, use:
git config --global user.name "Your Name"
git config --global user.email "you@example.com"
Always ensure that the email matches the one associated with your Git hosting service (e.g., GitHub, GitLab) to ensure proper linkage.
Configuring Default Editor
Customizing the default text editor can significantly enhance your efficiency when writing commit messages. You can easily set the default editor using:
git config --global core.editor "nano"
You can replace `"nano"` with your preferred editor, such as `vim`, `code` (for Visual Studio Code), or `notepad` on Windows. This configuration ensures that when you invoke the commit command without the `-m` flag, the specified editor opens automatically.
Adjusting Line Ending Preferences
Line ending discrepancies can lead to problems, especially in cross-platform development environments. You can manage this by configuring Git to handle line endings properly:
git config --global core.autocrlf true
Setting `core.autocrlf` to `true` ensures that line endings are converted to `LF` when you commit the file and to `CRLF` when checking out code on Windows. Alternatively, you can set it to `input` on Unix systems to maintain `LF` endings throughout.
Setting Up Aliases for Common Commands
Creating aliases for frequently used Git commands can streamline your workflow considerably. To create an alias, use the following syntax:
git config --global alias.co checkout
This command allows you to replace `git checkout` with `git co`, making your command line interactions faster and more efficient. Other popular aliases include:
git config --global alias.st status
git config --global alias.br branch
Customizing Colors and Output
Enabling color output can significantly improve readability by marking different elements of the output. To enable colored output, run:
git config --global color.ui auto
This command makes it easy to differentiate between different types of information by highlighting them in different colors, enhancing your interaction with Git.
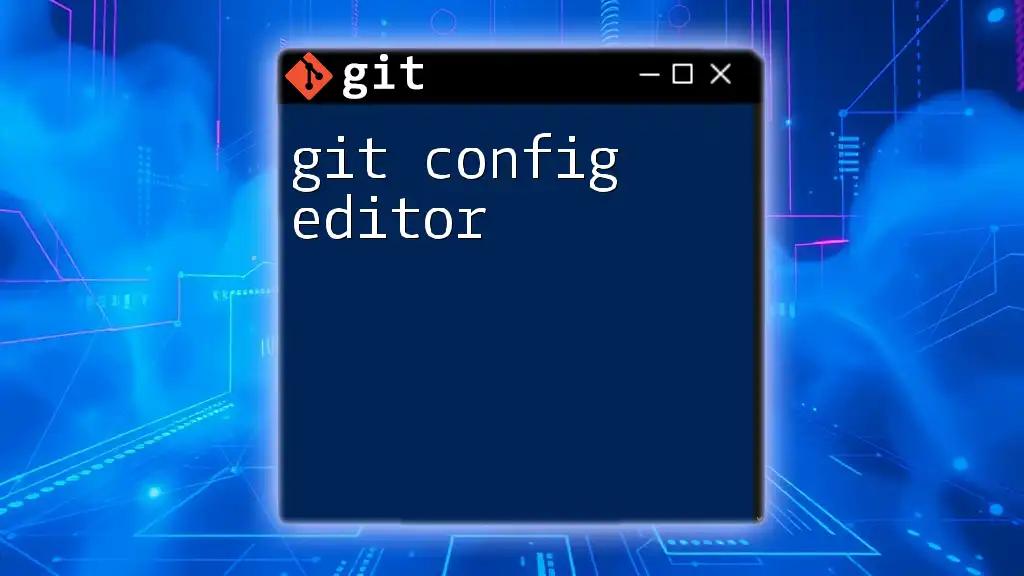
Viewing and Editing Configuration
Checking Current Configuration
To check which configurations are currently set, run:
git config --list
This command outputs all configurations Git is aware of, along with their current values. Understanding what settings are active can help you troubleshoot issues or optimize your Git environment.
Editing Configuration Files Manually
If you prefer direct manipulation, you can edit Git configuration files manually. The global configuration file is usually located in your home directory as `.gitconfig`, while local configuration resides within the `.git/config` of each repository. Open these files with your preferred text editor, and modify them as needed. Ensure that you maintain the correct syntax to prevent any errors.
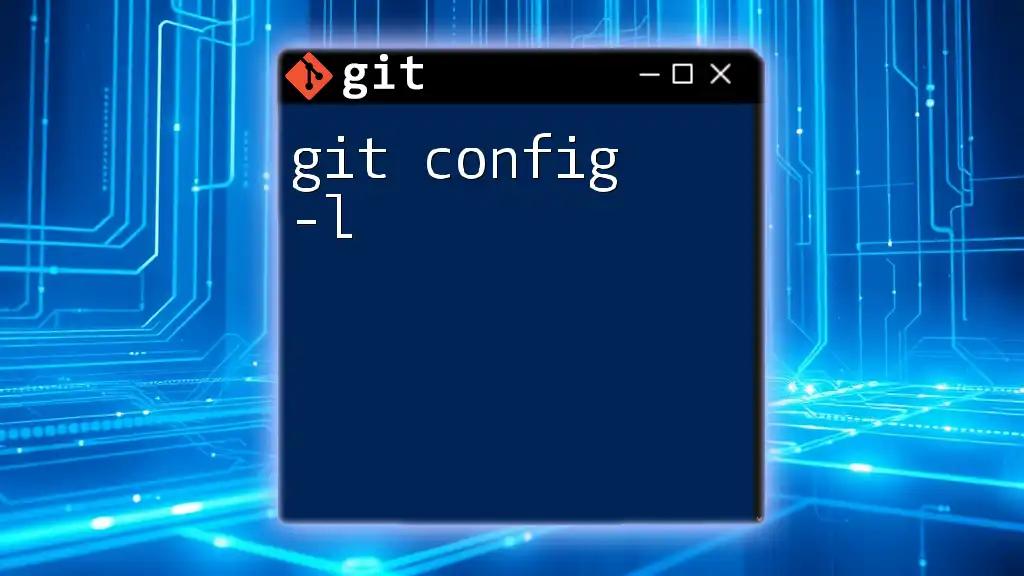
Best Practices for Git Configuration
Keeping Configuration Consistent
Maintain consistency across your data by ensuring uniform settings within your global configuration. Doing so can reduce confusion and streamline your workflow, especially when working across multiple repositories or collaborating with others.
Security Considerations
Avoid putting sensitive information, such as passwords or any form of personal tokens, in your Git configurations. Instead, use credential helpers or other secure methods to manage sensitive data.
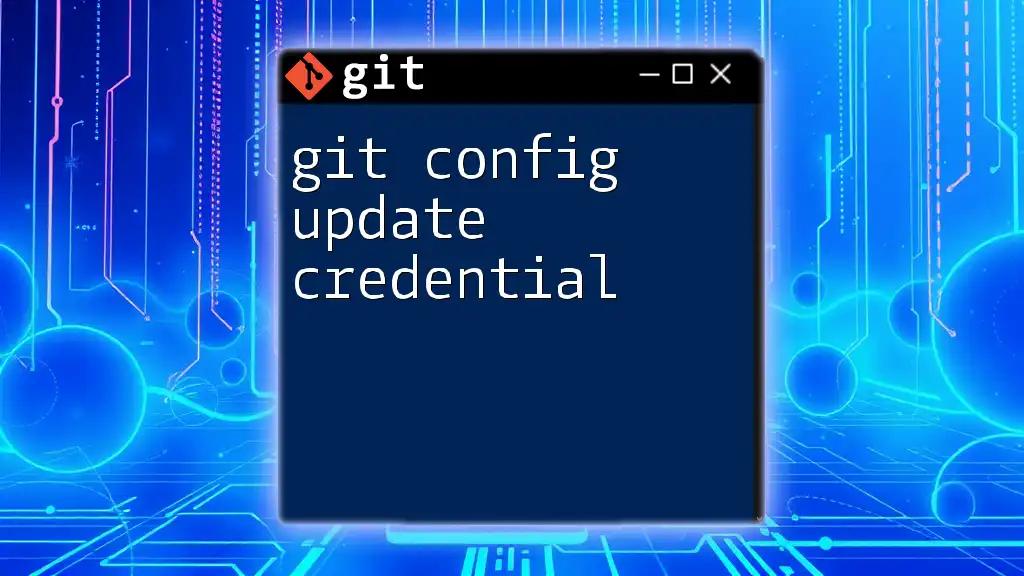
Troubleshooting Common `git config` Issues
Common Errors and Fixes
Errors may arise from conflicts between local and global settings. When updating configurations, always identify the scope first. Use `git config --list --show-origin` to see where each setting comes from, helping you resolve discrepancies effectively.
Using `git config` Help
When in doubt, the Git command line offers an intuitive help system. You can access it by running:
git help config
This command provides information on all aspects of `git config`, from syntax to available options, helping you gain a deeper understanding of the command.
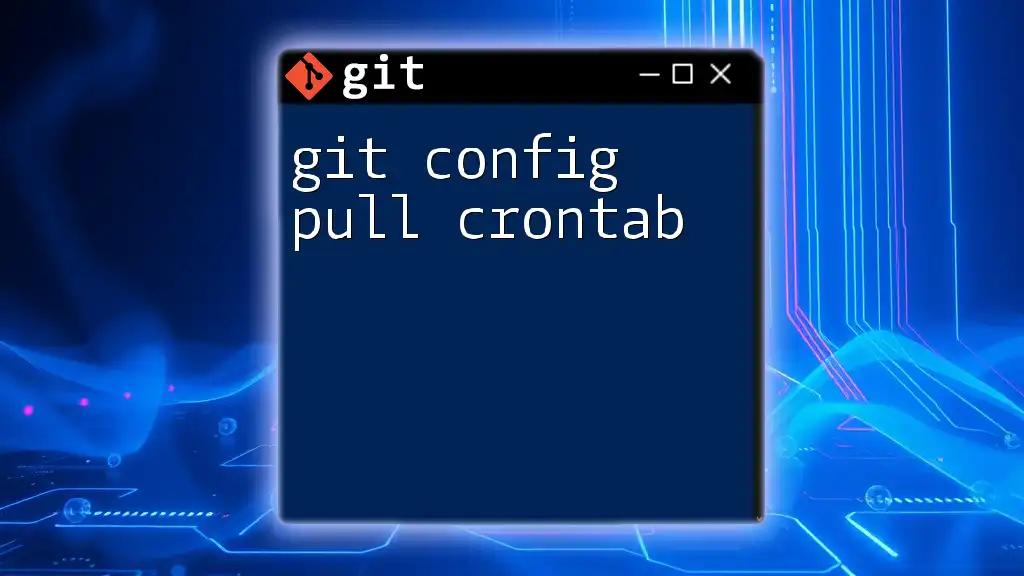
Conclusion
By mastering `git config`, you set the stage for a more efficient, organized, and enjoyable experience with Git. Proper configuration is not just about personal preference; it’s about improving collaboration, accountability, and security in your version control environment. Feel free to explore the settings available and fine-tune them to enhance your workflow!
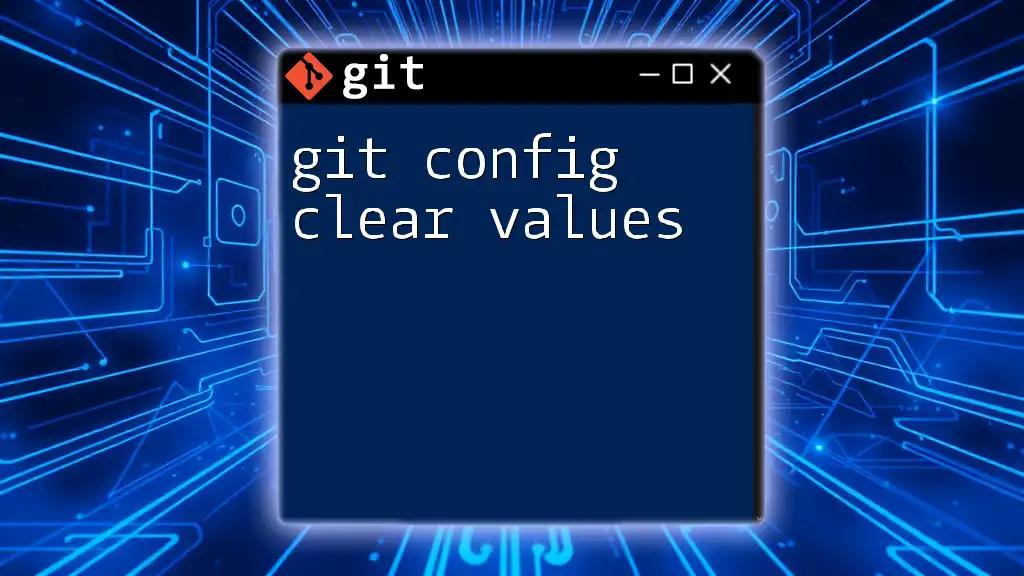
Additional Resources
For further learning, refer to the official Git documentation and explore community forums or tutorials that focus on Git and version control best practices. Engaging with additional resources will expand your knowledge and improve your Git proficiency.