Git is a powerful version control system that allows multiple developers to collaborate on projects efficiently, track changes, and manage code revisions.
Here's a simple command to initialize a new Git repository:
git init
What is Git?
Git is a distributed version control system that tracks changes in files, enabling multiple users to collaborate on a project simultaneously without overriding each other's work. Unlike traditional version control systems, Git allows users to maintain a complete history of changes, making it easier to revisit and manage previous versions of code.
Definition of Git
Git stands out due to its fundamental design principles. It's highly efficient in managing large codebases, and its branching model makes it both flexible and powerful. Key features of Git include:
- Branching: Users can create separate branches of code to develop features or patches, isolating their work until it's ready to be merged back into the main codebase.
- Merging: Git allows users to combine changes from different branches seamlessly.
- Committing: Users can save changes in smaller increments with descriptive messages, creating a clear history of the project’s evolution.
History of Git
Created by Linus Torvalds in 2005 as a response to the need for a better version control system for the Linux kernel, Git has since gained widespread adoption in software development and beyond. Its decentralized nature means that every developer has a complete copy of the repository, allowing for better collaboration and more robust project management.
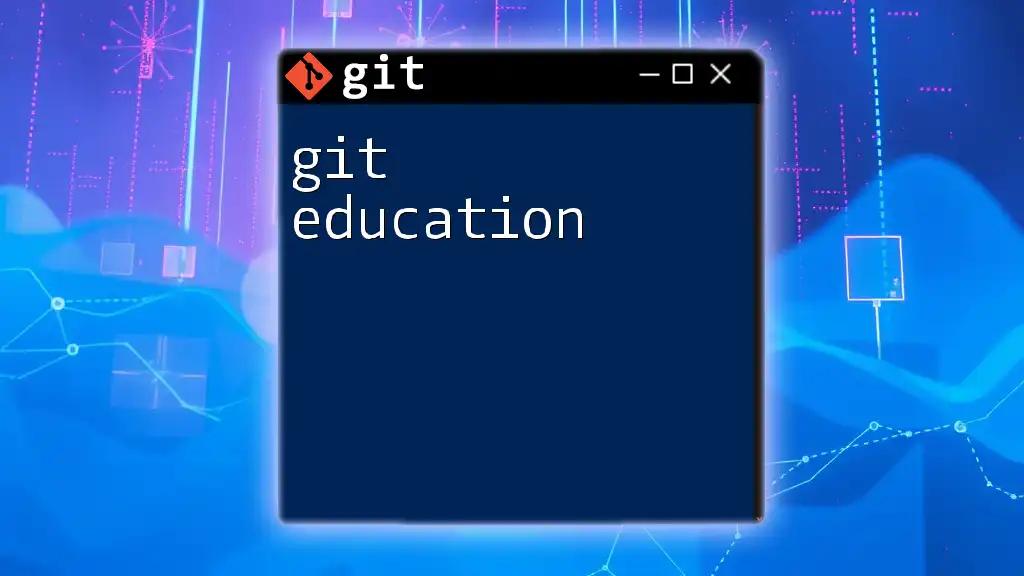
Why Use Git?
Benefits of Using Git
The importance of version control cannot be understated in software development, and Git provides several advantages:
- Collaboration: Git allows multiple developers to work on the same project without overwriting each other’s changes. Each user can work on their branch and then merge it back into the main project when ready.
- Version Control: With Git, every change is recorded. This facilitates easy tracking of what changes were made, who made them, and why.
- Backup: The repository is stored locally on each user's machine, providing an instant backup. If something goes wrong, restoring the previous state is straightforward.
Use Cases
Git is utilized across various industries and projects, such as software development, web development, and even documentation. Open-source projects thrive with Git since it allows contributors to work independently and compile their efforts into a cohesive product.
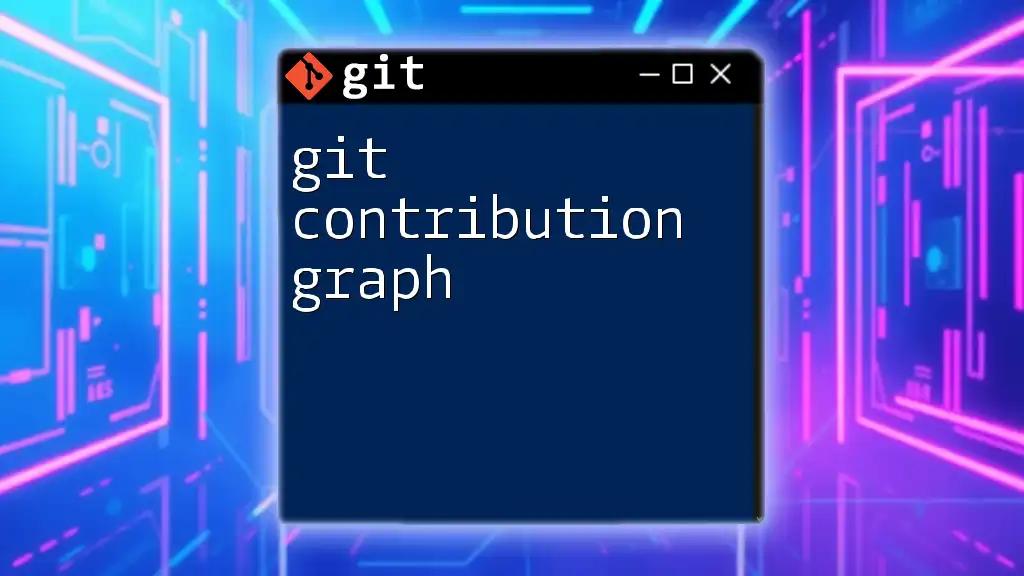
Getting Started with Git
Installing Git
To use Git, you first need to install it on your machine. The installation process differs based on the operating system:
Step-by-Step Guide:
-
For macOS: Open your terminal and run:
brew install git
-
For Windows: Use a package manager like Chocolatey:
choco install git
-
For Ubuntu: In the terminal, execute:
sudo apt-get install git
Configuring Git
Setting Up Your User Information
Configuring your user information is crucial because Git associates your commits with this data. Set up your username and email with the following commands:
git config --global user.name "Your Name"
git config --global user.email "your.email@example.com"
Configuring Default Text Editor
Set your preferred text editor, as Git will use it for commit messages. For example, if you prefer nano, you can set it as follows:
git config --global core.editor "nano"
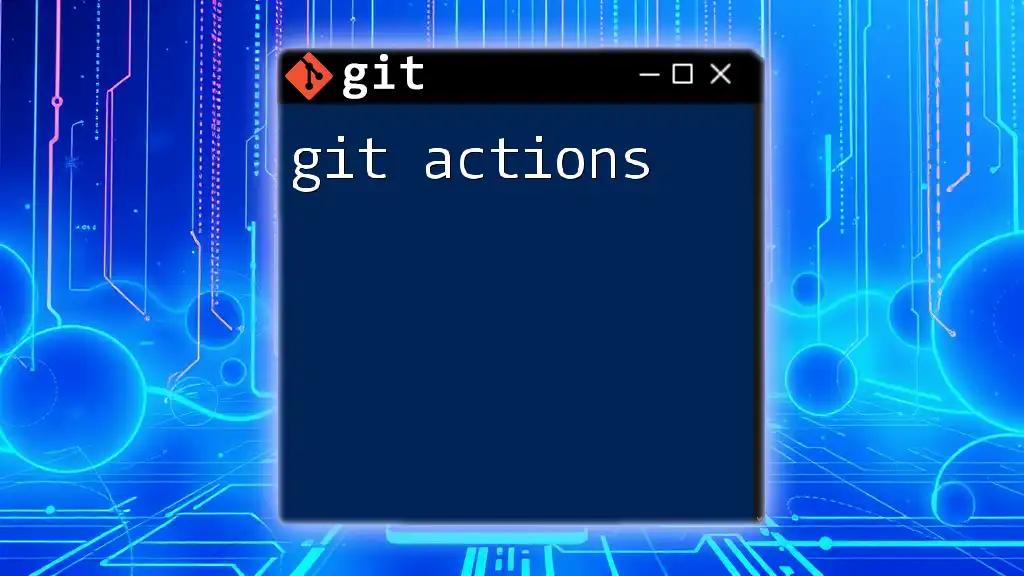
Basic Git Commands
Understanding the Command Line Interface
While Git also offers graphical user interface (GUI) applications, using the command line interface (CLI) is essential for mastering its functionalities. Familiarity with terminal commands can significantly enhance your efficiency when using Git.
Essential Commands
Creating Repositories
A repository is a storage space for your project and its version history. You can create one using:
git init my-repo
This command initializes a new Git repository in a folder called `my-repo`.
Cloning a Repository
To copy an existing repository, use the clone command. This allows you to work on a project that already has a history:
git clone https://github.com/user/repo.git
Staging Changes
Before committing changes, you need to stage them. This means telling Git which changes you want to include in your next commit:
git add filename
Committing Changes
After staging your changes, you can save them to the repository with a commit. Always include a meaningful commit message:
git commit -m "Initial commit"
Viewing Changes
Checking Status
The `git status` command shows the current state of the repository, including staged, unstaged, and untracked files:
git status
Viewing Commit History
To view the history of commits, the `git log` command provides a detailed list:
git log
This command displays each commit's hash, author, date, and message, enabling you to track the evolution of the project.
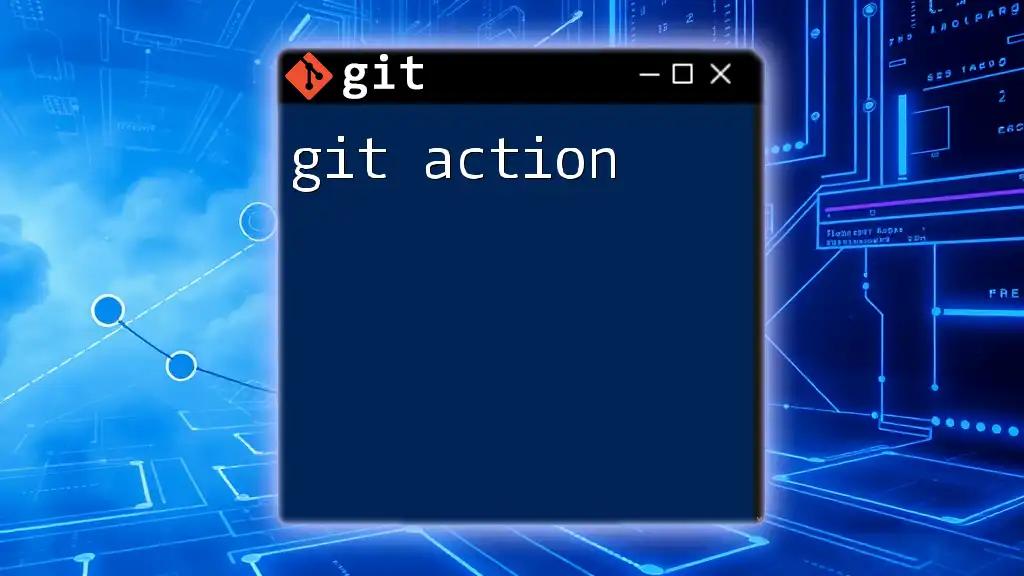
Branching and Merging
What are Branches?
Branches are an essential part of Git. They allow you to work on new features or fixes without affecting the main code—commonly referred to as the `main` branch.
Creating and Switching Branches
You can create a new branch using the following command:
git branch new-branch
To switch to the newly created branch, use:
git checkout new-branch
Merging Branches
Once you've finished making changes in a branch, merging them back into the main branch is straightforward:
git checkout main
git merge new-branch
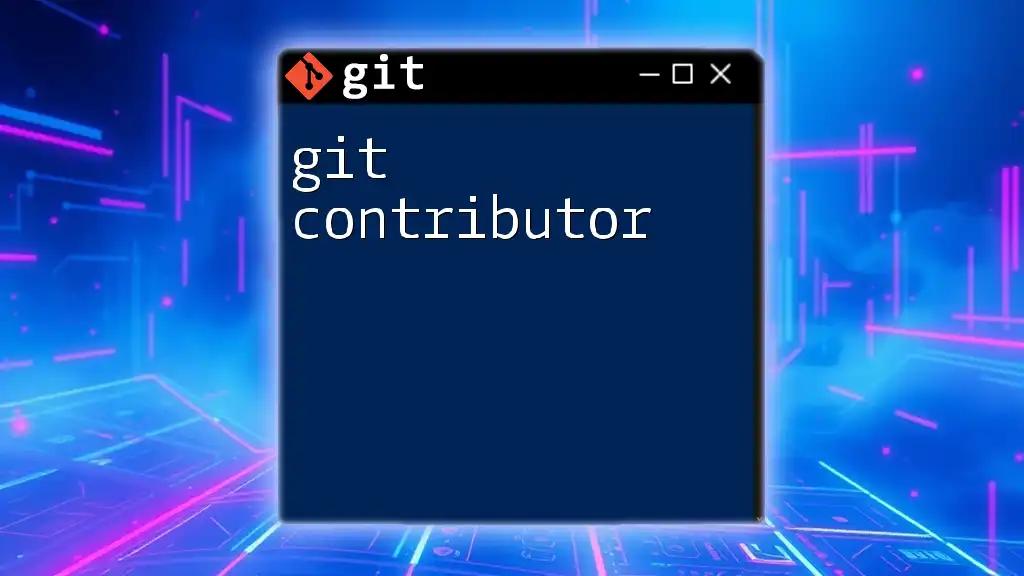
Resolving Conflicts
Understanding Merge Conflicts
Merge conflicts occur when two branches have competing changes in the same part of a file. Git will prompt you to resolve the conflict, ensuring that both sets of changes are taken into account.
How to Resolve Conflicts
To resolve conflicts, open the conflicting file. Git marks the conflicting sections, allowing you to choose which changes to keep. After making your choices, stage the resolved changes:
git add filename
And then commit the resolved changes:
git commit -m "Resolved merge conflict"
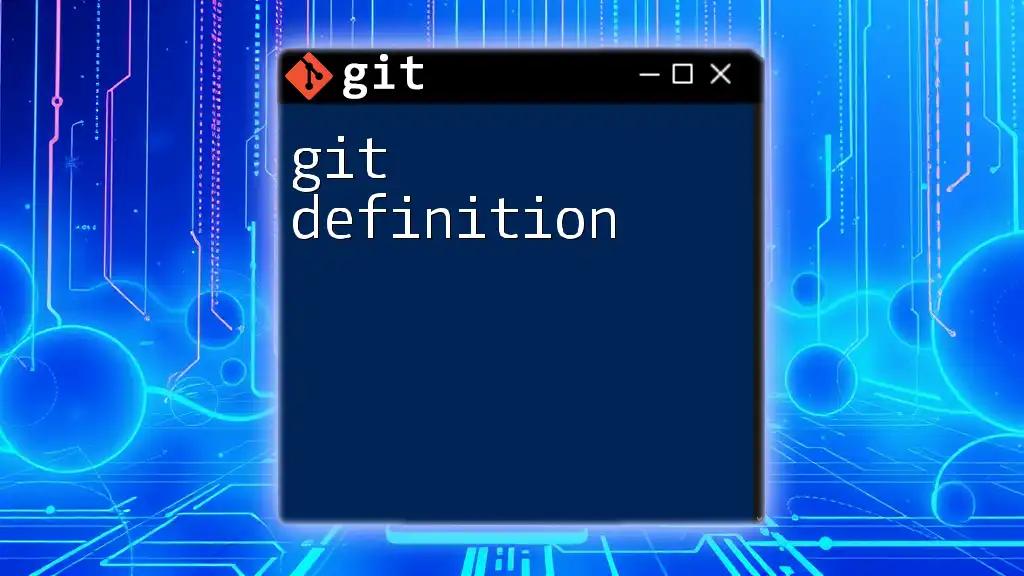
Best Practices for Using Git
Writing Meaningful Commit Messages
Crafting meaningful commit messages is vital for documenting your work. A good commit message should describe what changed and why, providing context for your future self and collaborators.
Regular Commits
Regularly committing your work creates a reliable history and helps prevent data loss. Make a habit of committing small, incremental changes rather than massive updates all at once.
Branch Management
Organize your branches effectively. Use descriptive names and delete branches that are no longer needed to keep your repository tidy.
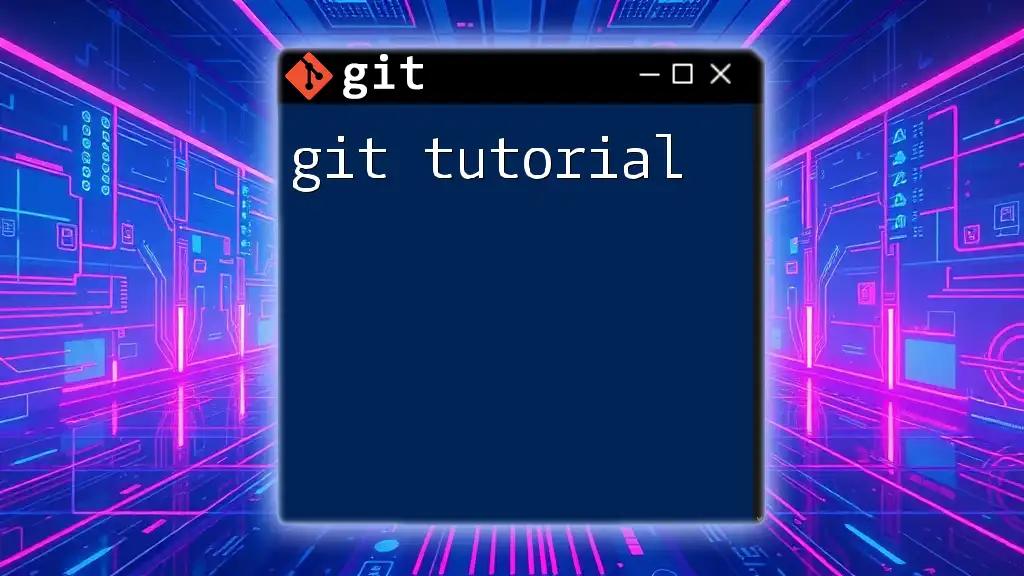
Conclusion
In summary, Git is a powerful tool that facilitates collaboration, tracks changes, and provides a robust backup system. By understanding its functionalities—like committing, branching, and merging—you can streamline your development process.
As you continue learning, don't hesitate to explore advanced topics such as Git workflows and integrating Git with platforms like GitHub. Getting started with Git will not only enhance your project development skills but also make collaborative coding a breeze. Take the step today to harness the full power of version control in your coding journey!