The `git pull` command fetches changes from a remote repository and merges them into the current branch, allowing you to update your local repository with the latest commits.
git pull origin main
The Mechanics of `git pull`
What Happens When You Run `git pull`?
When you execute the `git pull` command, it performs a two-step process that is crucial for synchronizing your local repository with the remote repository. This process consists of:
- Fetching: This retrieves the latest changes from the specified remote repository without merging them into your working branch.
- Merging: After fetching, Git attempts to automatically merge the new changes into your current branch.
It's important to differentiate `git pull` from `git fetch`. While `git pull` fetches and merges in one convenient command, `git fetch` allows you to see what changes are available on the remote without altering your local files. Knowing when to use each command can significantly impact your workflow.
Syntax of the `git pull` Command
The basic syntax of the `git pull option` is:
git pull [options] [<repository> [<refspec>...]]
This syntax gives you the flexibility to specify various options, choose a repository, and determine the specific reference specifications you want to pull.
Common Options for `git pull`
`--rebase`
The `--rebase` option is a powerful command that allows you to reapply your changes on top of the upstream changes. This can be particularly useful in maintaining a cleaner project history by avoiding unnecessary merge commits.
Scenario to Use Rebase: If you're collaborating on a shared branch, you can use the following command to rebase your current changes onto the latest changes from the remote:
git pull --rebase origin main
Using rebase helps in creating a linear history, which can make the project history easier to read.
`--no-commit` and `--commit`
Another important aspect of the `git pull option` is choosing whether or not to create a new commit automatically during the merging process. The `--no-commit` option allows you to prevent Git from creating a merge commit, giving you the chance to review changes first.
To use this option, you might run:
git pull --no-commit origin develop
If you later decide that the changes should be committed, you can manually commit them using:
git commit -m "Merged changes from develop"
Handling Conflicts with `git pull`
What Are Merge Conflicts?
Merge conflicts happen when Git cannot automatically combine changes from different branches. This situation arises typically when two contributors modify the same line in a file or when one contributor deletes a file that another contributor is trying to edit.
Understanding how to handle these conflicts is essential for any Git user.
Steps to Resolve Conflicts
- Run `git pull` and if you encounter a conflict, Git will inform you with a message.
- Check Conflicted Files: Use the command below to determine which files are in conflict.
git status
- Manual Resolution: Open the conflicted files in your text editor. Look for `<<<<<`, `=====`, and `>>>>>` markers which indicate the conflicting sections. Edit the file to resolve the conflicts.
- Add Resolved Files: Once you've resolved all conflicts, stage the files:
git add <file_with_conflict>
- Continue the Merge: To complete the merging process after resolving conflicts, run:
git merge --continue
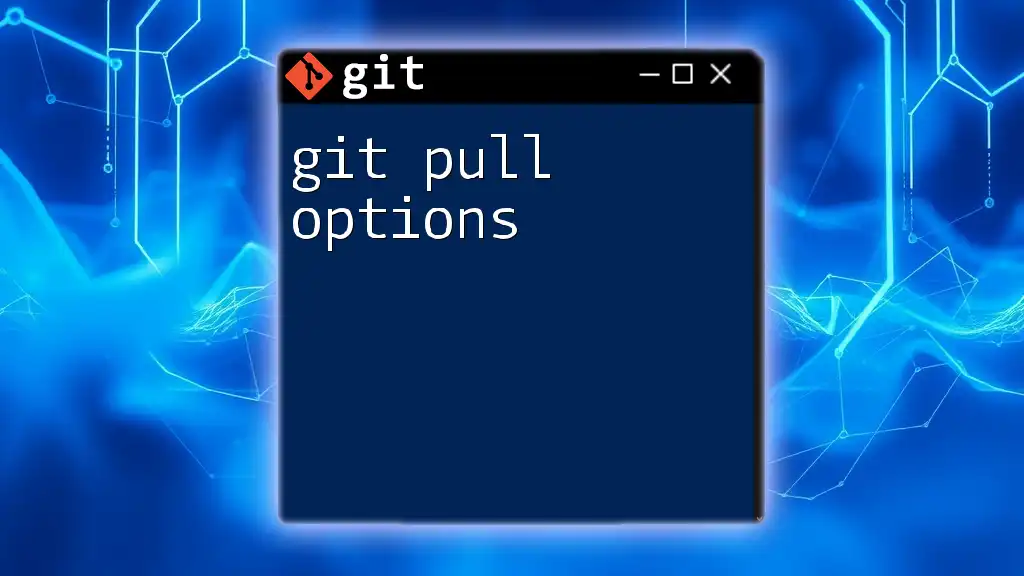
Practical Examples of Using `git pull`
Example 1: Pulling from the Default Remote
When you are working in a local branch that tracks a remote branch, you can simply run:
git pull
This command will fetch and merge changes from the default remote repository into your current branch.
Example 2: Pulling a Specific Branch
If you need to pull from a specific branch on a remote repository, the command is:
git pull origin feature-branch
This is particularly useful when you are collaborating on multiple features simultaneously.
Example 3: Combining `git pull` with Other Commands
Sometimes, you may want to update your branch while keeping it clean with a rebase. You can achieve this with:
git pull --rebase origin main
This command pulls the latest changes from the `main` branch and reapplies your changes on top, thus maintaining a cleaner commit history.
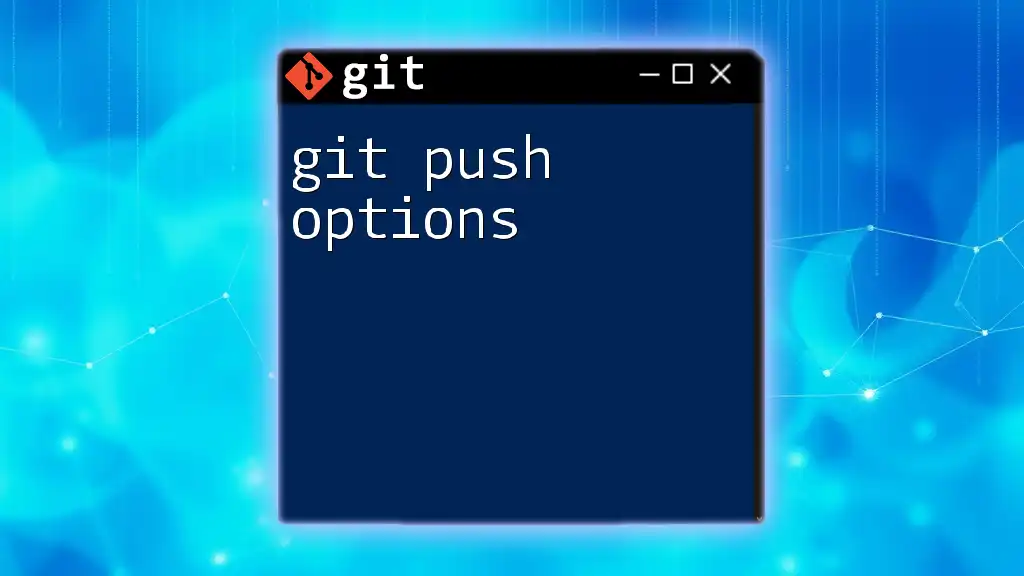
Best Practices for Using `git pull`
Regular Pulling: It is advisable to pull frequently, especially if you're working in a team environment. By doing so, you minimize the risk of encountering large merge conflicts and ensure your local repository is up-to-date.
Using `git fetch` First: For more cautious developers, it is often recommended to fetch changes before pulling. This way, you can examine incoming changes using:
git fetch
By reviewing the updates, you gain insight into what changes are coming, reducing the likelihood of merge conflicts.
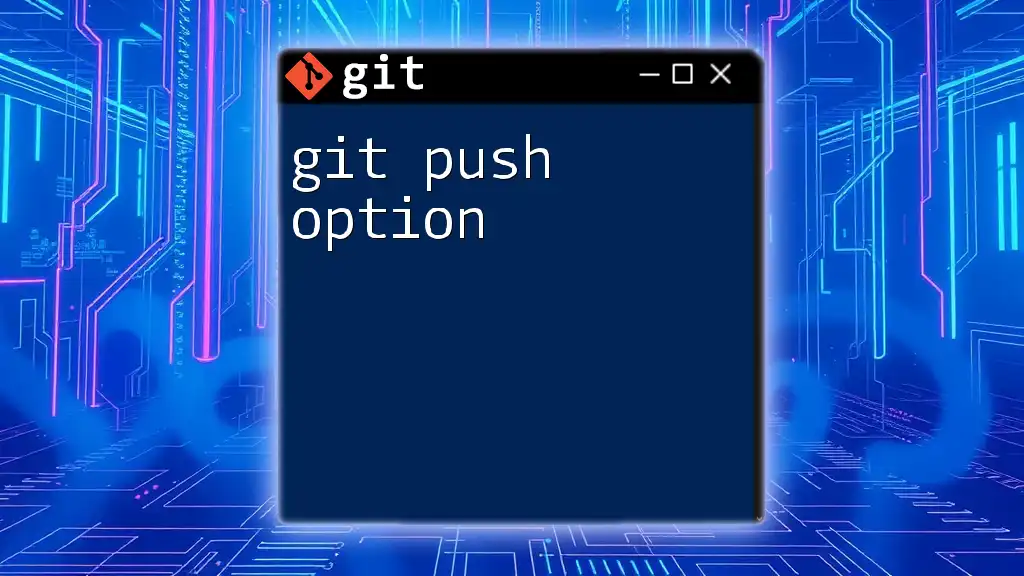
Troubleshooting `git pull`
Common Errors and Solutions
One of the most common errors encountered during a `git pull` is:
Error: "Your local changes to the following files would be overwritten by merge."
To fix this, you need to stash your changes first:
git stash
git pull
git stash pop
This sequence stashes your modifications, pulls the latest changes, and then re-applies your work.
Another common issue is:
Error: "Merge conflict."
To effectively address this situation, you can follow the conflict resolution steps mentioned previously.
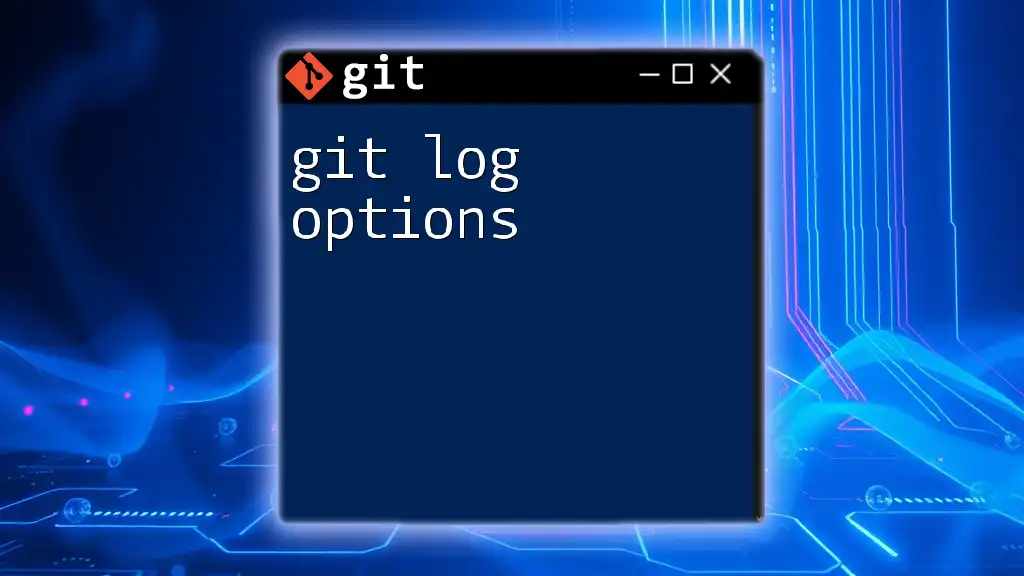
Conclusion
Understanding the `git pull option` is essential for efficient collaboration in any software development project. This command serves as a bridge to keep your local environment synchronized with the team's progress. The more familiar you become with the nuances of `git pull`, the more seamlessly you can contribute to team efforts. Regular practice and mindful use of this command will enhance your skills and make working with Git a more rewarding experience.
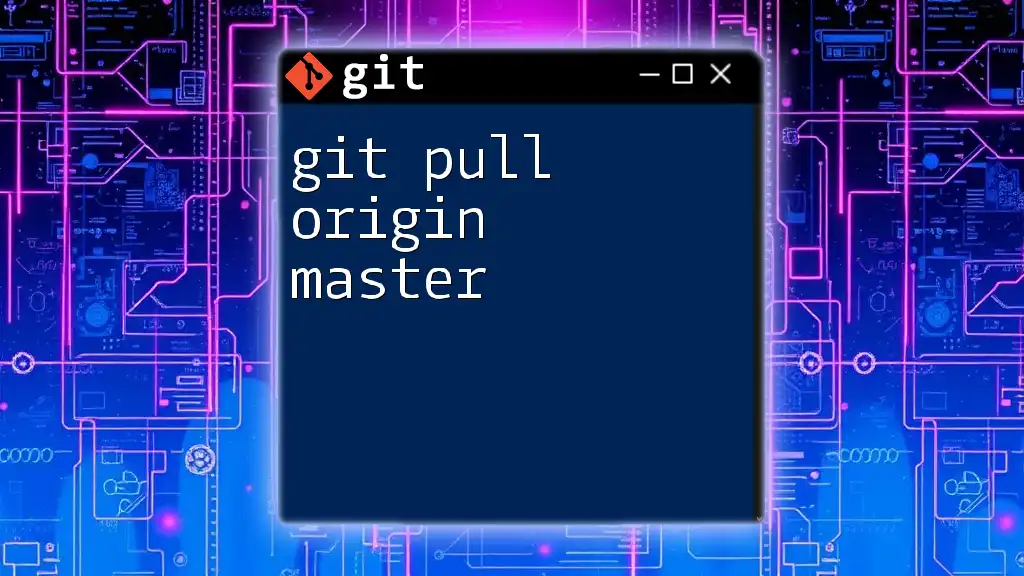
Additional Resources
For further learning, refer to the official Git documentation and explore recommended tutorials and courses focused on mastering Git commands.