The `git pull origin branch` command is used to fetch and integrate updates from a specified branch in a remote repository into your current local branch.
git pull origin branch
Understanding `git pull origin branch`
What is `git pull`?
`git pull` is a command used in Git that allows you to update your local repository with changes from a remote repository. It is a higher-level command that combines two important Git actions: fetching and merging. When you use `git pull`, you are asking Git to first retrieve any new commits from the specified branch of the remote repository and then merge those changes into your current local branch.
It's crucial to highlight the difference between `git fetch` and `git pull`. While `git fetch` only retrieves changes from the remote repository without merging them, `git pull` not only fetches those changes but also attempts to automatically merge them into your working branch. This makes `git pull` a more comprehensive command when you want to keep your local repository up to date with changes made by others.
Breaking Down the Command
Let's break down the components of the command `git pull origin branch` to understand its syntax:
-
git: This is the command-line interface for the Git version control system.
-
pull: This action instructs Git to fetch and merge changes from the remote repository.
-
origin: This term refers to the default name for the remote repository. It is the version of the repository hosted on platforms like GitHub or Bitbucket.
-
branch: This specifies the name of the branch in the remote repository from which you want to pull updates. For instance, you may want to pull changes from the `main` or `develop` branch.
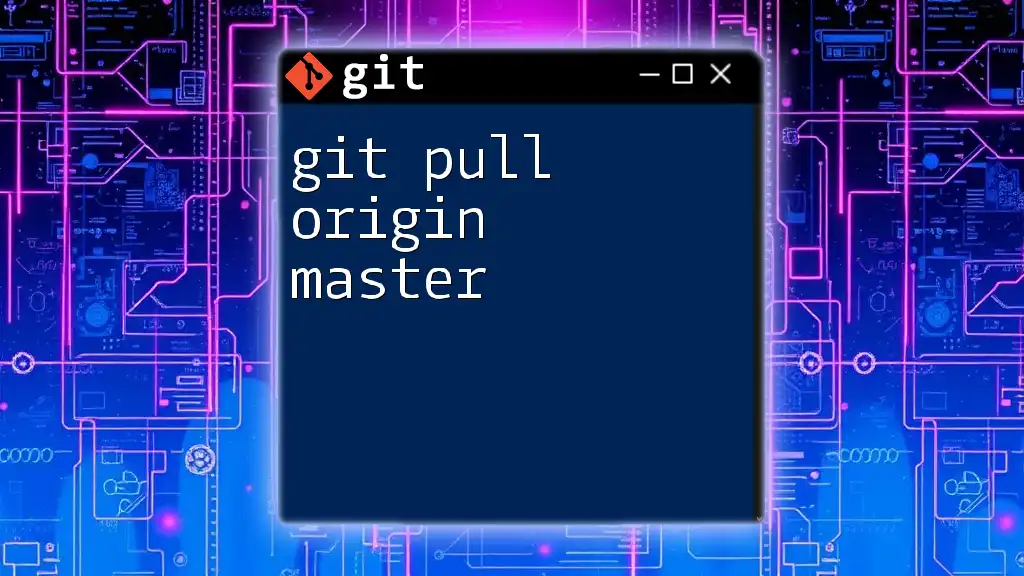
The Workflow of `git pull origin branch`
Step-by-step Process of Using `git pull origin branch`
When you use the command `git pull origin branch`, you're essentially requesting Git to follow a two-step process that involves fetching and merging:
-
Fetching Updates: This first part of the command retrieves updates from the remote repository to your local machine. You can view it as a way of synchronizing your local branch with the remote one, providing you access to the latest commits.
To see what’s happening, the command would be:
git fetch origin
This command downloads the latest changes from the `origin` remote but does not apply them to your current working branch yet.
-
Merging Updates: After fetching, the next step is to merge the changes into your local branch.
This occurs automatically when you execute:
git pull origin branch
If there are no conflicts, Git will seamlessly combine the changes. However, if there are discrepancies with the existing code, you might have to resolve conflicts manually.
Common Scenarios for Using `git pull origin branch`
It's important to understand when to use `git pull origin branch`. Here are some common scenarios:
-
Collaborating on Team Projects: If you're working on a team, using this command helps ensure that you always have the latest version of the code, making collaboration smoother.
-
Keeping Up With Changes: If changes are made frequently in your project, using the command regularly helps keep you updated and less likely to face merge conflicts during collaboration.
-
Avoiding Merge Conflicts: By pulling changes frequently, you reduce the risk of conflicts which arise when multiple people modify the same lines of code.
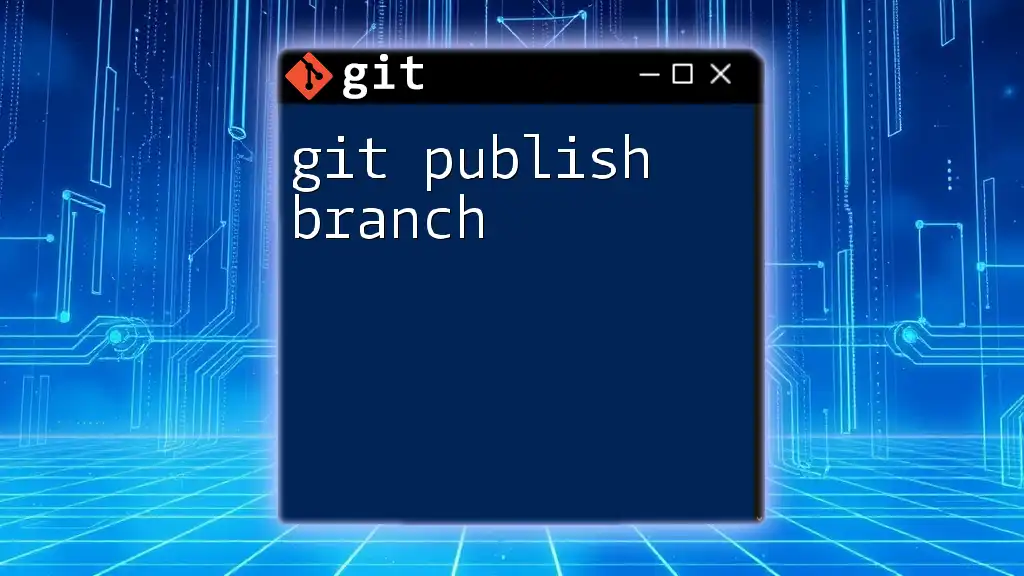
Examples of Using `git pull origin branch`
Example 1: Simple Pull
Consider an instance when you need to pull the latest updates from the `main` branch:
git pull origin main
This command effectively synchronizes your local workspace with the most recent updates from the `main` branch of the origin remote.
Example 2: Pulling Updates from a Feature Branch
When working on a feature branch named `feature-login`, you can pull the latest updates with:
git pull origin feature-login
This command is particularly useful in team workflows where multiple developers might be working on different branches simultaneously. By keeping your feature branch updated, you ensure compatibility with ongoing changes in the existing codebase.
Example 3: Dealing with Merge Conflicts
Sometimes, your pull attempt may lead to merge conflicts, which occur when changes from the remote branch cannot be automatically merged. In such cases, Git will notify you of the conflicting files. For example, you may see:
CONFLICT (content): Merge conflict in <file_name>
Resolving these conflicts requires careful attention. You'll typically need to:
- Open the conflict files.
- Manually edit the sections marked by Git.
- Once resolved, add the resolved files.
- Finally, commit the changes to complete the merge.
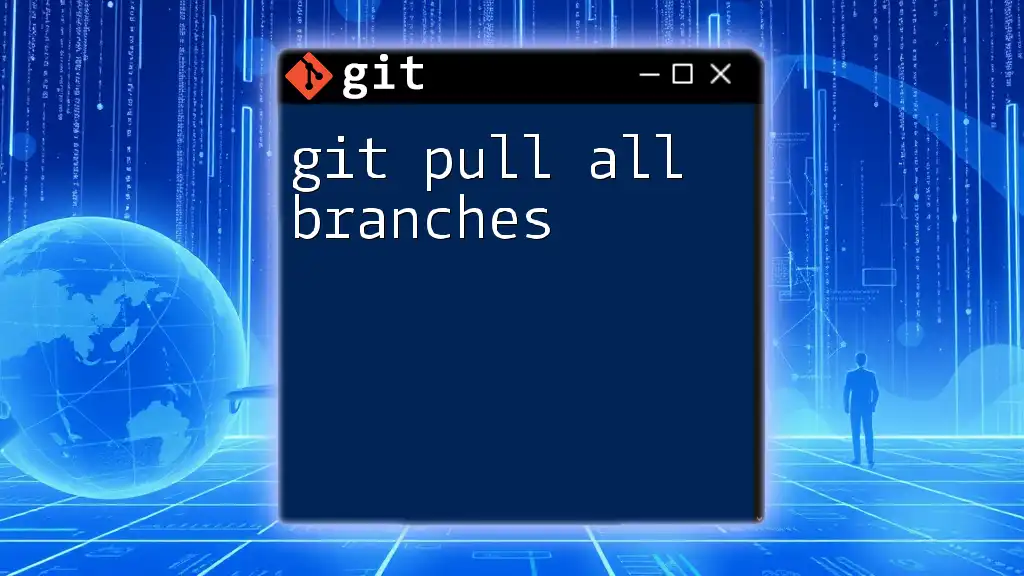
Best Practices for Using `git pull origin branch`
To minimize issues while navigating Git, consider the following best practices:
-
Always Check the Status Before Pulling: Before executing a pull, it’s a good idea to check your current branch status. This can help you identify any uncommitted changes that might interfere with the merge. Run:
git status
-
Pull Frequently: Regularly using `git pull` helps you avoid large and complicated merges down the line. Keeping your work synced with the remote repository is essential for maintaining order in collaborative scenarios.
-
Read Commit Messages Before Pulling: Understanding the history of the changes will prepare you for any potential issues and help you understand the context of the updates.
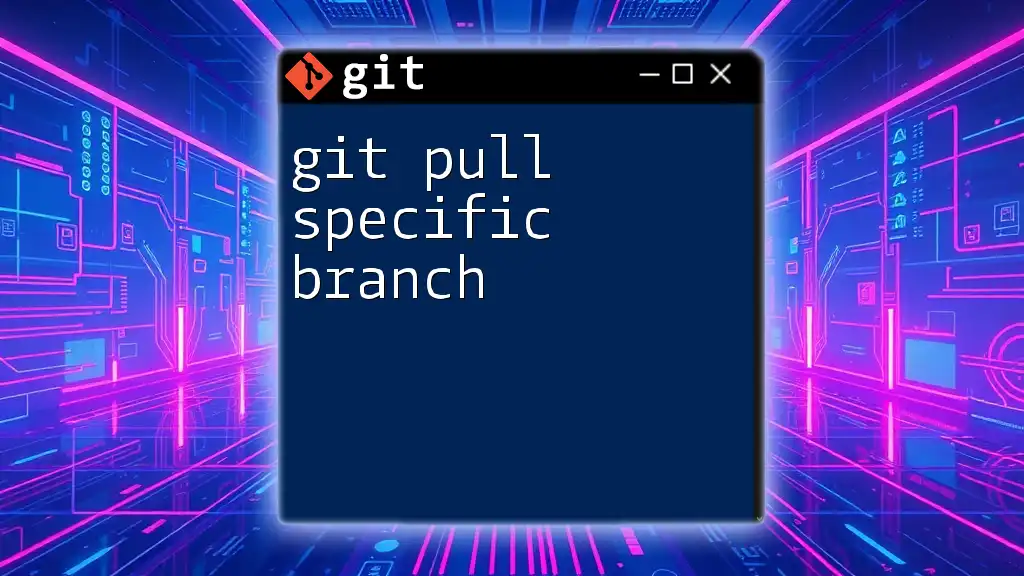
Troubleshooting `git pull origin branch`
Common Issues and Solutions
While using `git pull origin branch`, you may encounter some common issues. Understanding how to tackle them is vital for effective workflow management.
-
Understanding Error Messages: You might see messages indicating that your branch is ahead of `origin/branch` by a certain number of commits. This generally means you have local changes that haven’t been pushed yet.
To push your changes to the remote branch, simply run:
git push origin branch
-
Handling Upstream Changes: If your branch has diverged (especially if someone else has pushed updates to the same branch), consider checking the status and fetching the changes again.
Useful Commands Related to `git pull`
There are several commands that you might find useful in conjunction with `git pull`:
git remote -v
This command displays the remote repositories linked to your local repository.
Another useful command is:
git diff
This allows you to review changes between your current branch and the desired commit.
Lastly, if you want to see the history of your commits, you can use:
git log
This provides a log of all your commits, which can help in understanding the changes to be merged.
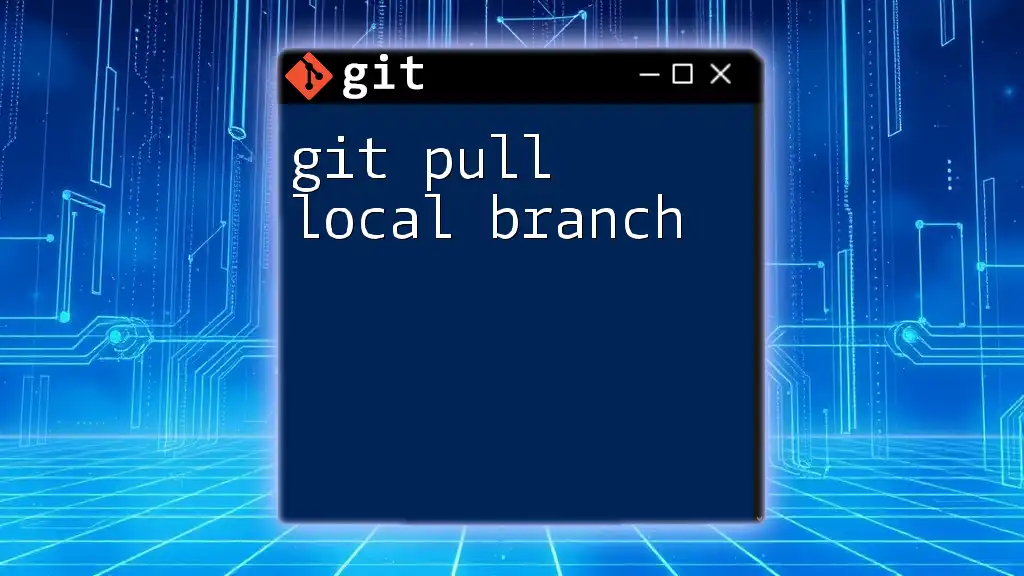
Conclusion
In summary, understanding the command `git pull origin branch` is essential for effective collaboration and version control when working with Git. Regularly practicing this command and applying the best practices discussed can significantly enhance your development workflow. Don't hesitate to explore advanced features of Git as you become more comfortable with these fundamental commands.