To pull a specific branch from the origin remote repository, you can use the following command:
git pull origin branch_name
Replace `branch_name` with the name of the branch you wish to pull.
Understanding Remote Repositories
What is a Remote Repository?
A remote repository is essentially a version of your project that is hosted on the internet or another network. It allows multiple collaborators to work on the same project simultaneously, facilitating teamwork and centralized storage for the codebase. Common platforms for hosting remote repositories include GitHub, GitLab, and Bitbucket.
The Concept of Origin
When working with Git, the term origin typically refers to the default name given to the remote repository from where you cloned your project. This serves as a convenient shortcut in your Git commands, allowing you to reference the remote repository without needing to type its entire URL.
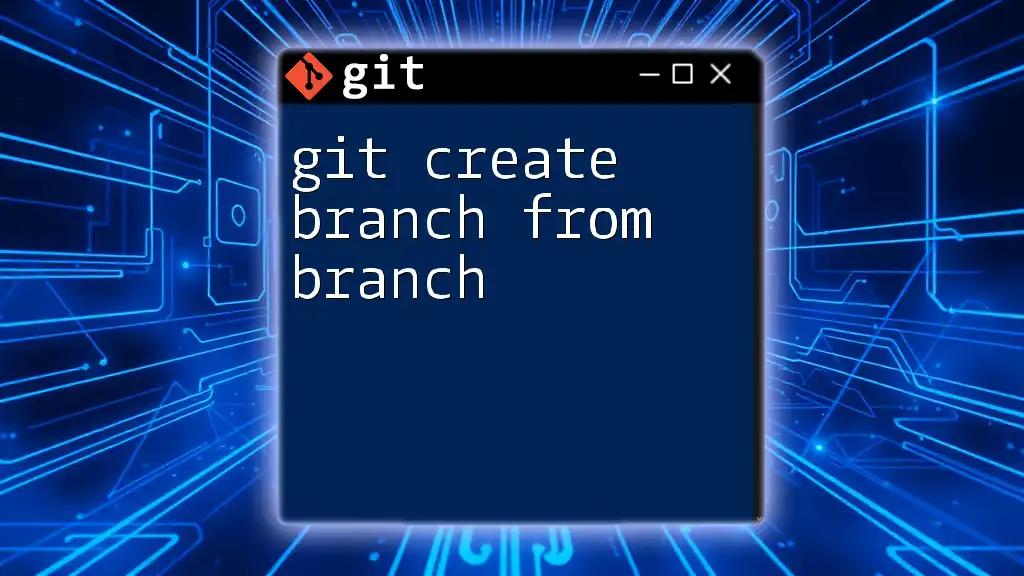
The Basics of the `git pull` Command
What Does `git pull` Do?
The `git pull` command is primarily a two-step process. It first fetches the changes from the specified remote repository (like `origin`), and then it merges those changes into your current branch. This command is crucial for ensuring your local work stays synchronized with the collaborative efforts happening on the remote repository.
Syntax of `git pull`
The basic syntax for the `git pull` command is:
git pull <remote> <branch>
- `<remote>`: This usually refers to `origin` but can be any remote repository you have configured.
- `<branch>`: This is the name of the branch you want to pull the latest changes from.
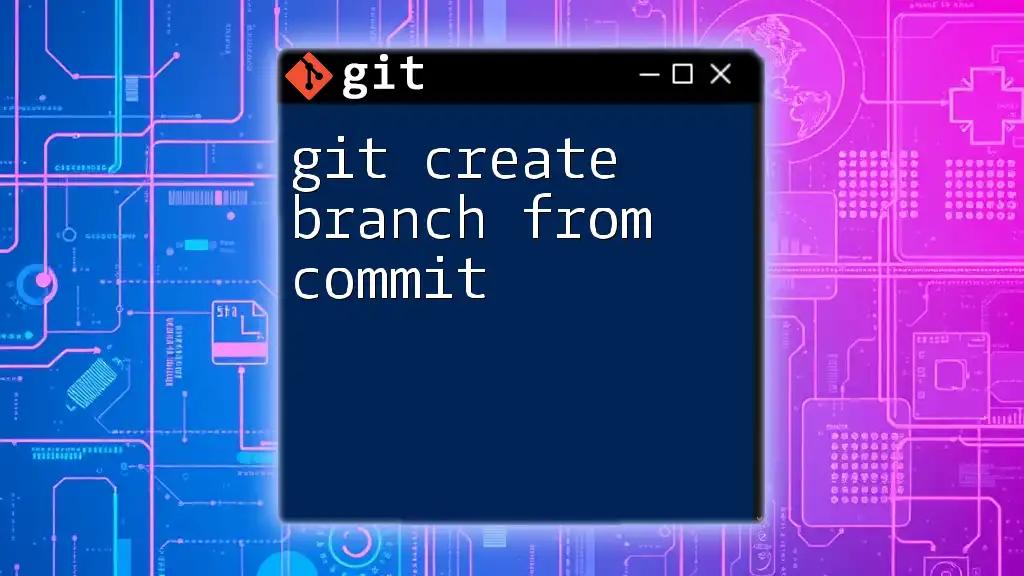
How to Pull a Branch from Origin
Step-by-Step Guide
Configuring Your Local Repository
Before you pull a branch, you need to ensure that your local repository is properly set up. If you haven't already cloned the repository, you can do so using the following command:
git clone <repository-url>
This command not only downloads all the files from the remote repository but also configures the `origin` remote automatically.
Fetching the Latest Changes
It’s a good practice to fetch the latest changes before pulling to make sure you are aware of the ongoing developments. You can do this by running:
git fetch origin
Using the `fetch` command retrieves the latest changes from the remote without merging them into your current branch just yet. This gives you a chance to review what has changed.
Checking Out the Desired Branch
Before you pull a branch, you need to check out the branch that you want to update or pull from. To do this, use the following command:
git checkout <branch-name>
Replacing `<branch-name>` with the name of the branch you wish to work on. This command will switch your working directory to that branch, setting you up properly for the pull.
Executing the Pull Command
Once you’re on the correct branch and have fetched the latest changes, you can now execute the pull command:
git pull origin <branch-name>
When you run this command, Git will pull changes from the specified branch of the `origin`. On successful execution, you will see output similar to “Already up to date” or a summary of the changes that have been pulled in.

Handling Merge Conflicts
Common Causes of Merge Conflicts
Merge conflicts can arise during a pull when changes made in the local branch and the remote branch diverge. For example, if two contributors have made changes to the same line in a file, Git won’t know which change to keep.
Resolving Merge Conflicts
When a merge conflict occurs, Git will notify you, and you can see the files with conflicts using the following command:
git status
This will show you the files that need attention. To resolve conflicts, open the conflicted files and manually merge the changes. After resolving, you add the resolved files to the staging area:
git add <file-name>
Then, you can commit your changes with a message indicating that you’ve resolved the conflicts:
git commit -m "Resolved merge conflict"
Resolving merge conflicts is an essential skill in collaborative environments to ensure that all changes are properly incorporated.
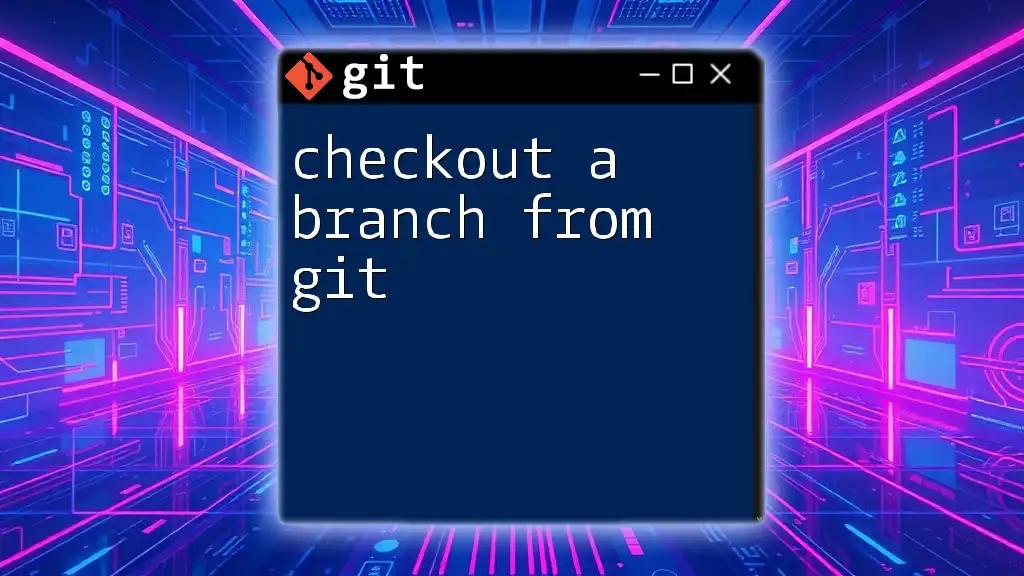
Best Practices for Pulling from Origin
Always Be on the Right Branch
Before pulling, ensure that you are on the correct branch where you want to merge changes. Pulling changes into the wrong branch can lead to confusion and complications in the project.
Pull Regularly
Frequent pulling from the remote repository helps to keep your local branch updated with the latest developments. This reduces the chances of facing significant merge conflicts down the road, making it easier to collaborate effectively.
Always Pull Before Pushing
It is recommended to pull changes before pushing your own commits to the remote repository. This helps you incorporate the latest updates from your collaborators and resolve any potential issues before your changes are made public.
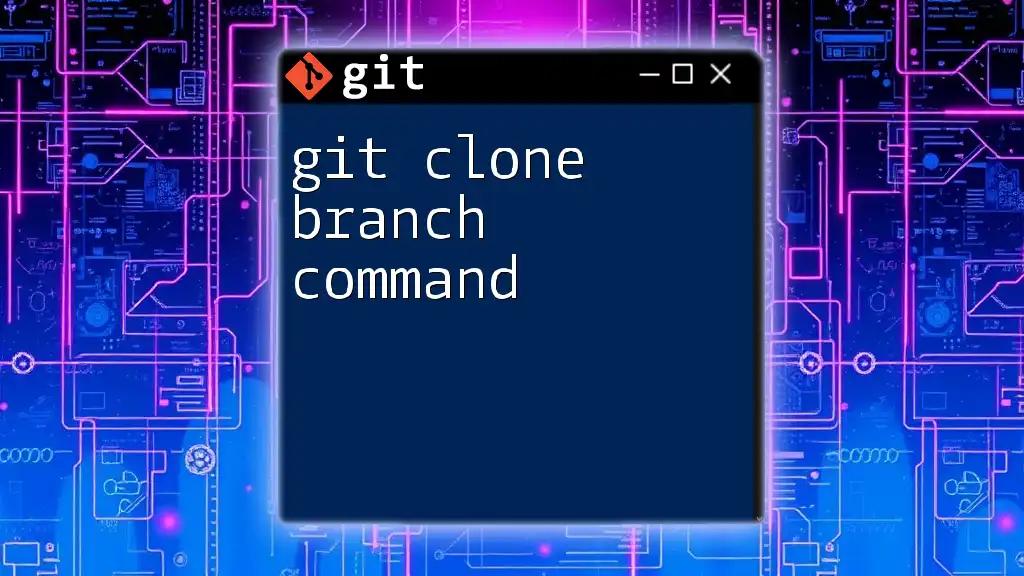
Conclusion
Pulling a branch from origin is a fundamental skill every developer should master when working with Git. Following the steps outlined above, you can ensure that your local repository stays updated and conflicts are managed effectively. Regular practice will enhance your proficiency in using Git commands and improve your collaborative development experience.
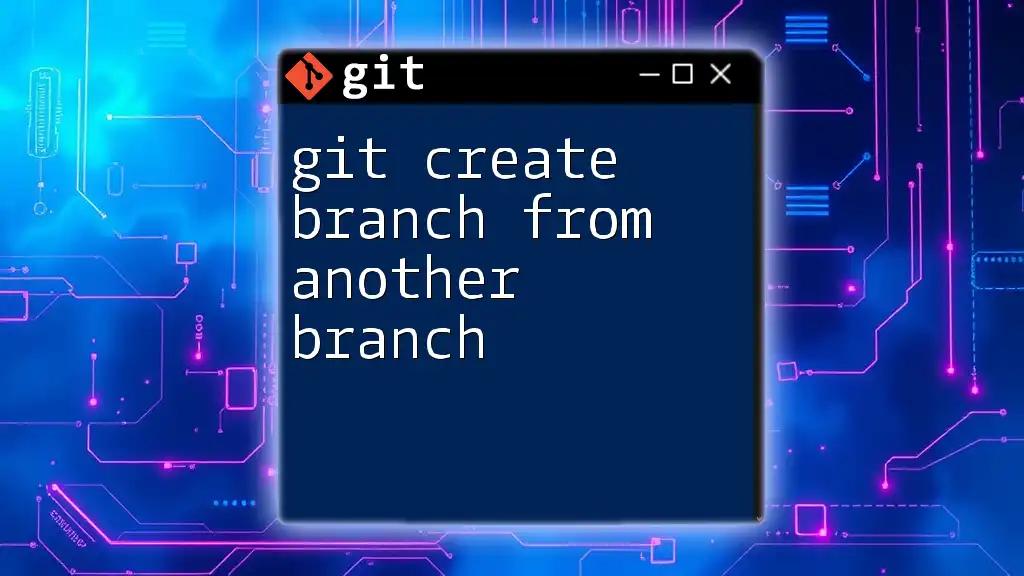
FAQs about Pulling a Branch from Origin
-
Can I pull multiple branches at once? You cannot pull multiple branches simultaneously. You must check out each branch you wish to pull individually.
-
What happens if I pull from a branch that doesn’t exist on the remote? Git will notify you that the branch does not exist on the remote, and the pull command will fail.
-
How do I undo a pull if something goes wrong? If you need to undo a pull due to unexpected changes, you can use the `git reset --hard` command to revert your branch to the state it was in before the pull, but use with caution, as it will discard all uncommitted changes.
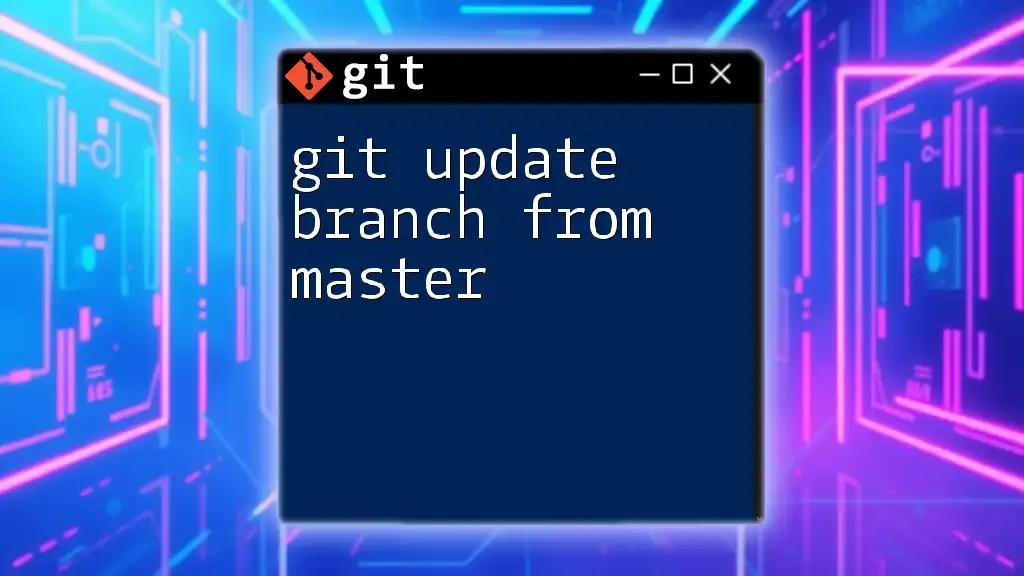
Additional Resources
To deepen your understanding of Git and its commands, consider exploring comprehensive guides, videos, and official documentation. These resources will help you enhance your capabilities and navigate through the nuances of version control effectively.