To fetch and check out a specific tag from a remote repository in Git, use the following command:
git fetch origin tag_name && git checkout tag_name
Replace `tag_name` with the actual name of the tag you wish to pull.
Understanding Git Tags
What are Git Tags?
In Git, tags are a powerful feature that allows developers to create point-in-time markers on commits. You can think of tags as bookmarks — when you tag a commit, you’re essentially marking it with a human-readable label for easy reference later.
There are two primary types of tags:
- Lightweight Tags: These are essentially pointers to a specific commit and are similar to a branch that doesn't change. They are quite swift to create and do not carry any additional information.
- Annotated Tags: These are full objects in the Git database, which contain metadata like the tagger’s name, email, date, and an optional message. This makes them more suitable for releases or key milestones in a project.
Utilizing tags efficiently enables teams to keep track of important releases and versions throughout the project's lifecycle.
How Tags Work in Git
Tags in Git are stored in the repository alongside commits. Each tag points to a specific commit, allowing not only for easy access but also precise identification of important points in the development history. When retrieving a commit associated with a tag, you can quickly correlate those moments in time when releases or major changes occurred, giving a historical perspective on project development.
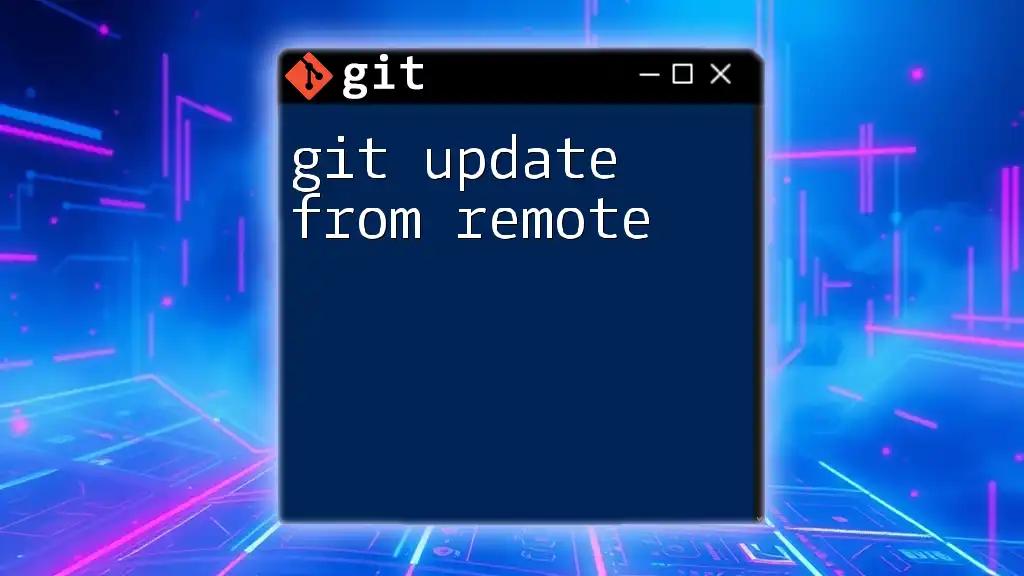
The Purpose of Pulling Tags from Remote
Why Pull Tags?
Pulling tags from a remote repository is essential for keeping your local repository updated with the latest markers set by your team or other collaborators. Tags ensure that everyone involved in the project is working with the same context, avoiding confusion over which release or version is being used.
When to Pull Tags
It's crucial to pull tags especially when:
- Entering a new project or branch where tags might indicate stable versions or important releases.
- Prior to branching off from a project to ensure you’re based on the correct version.
- Collaborating with different teams where multiple releases might be managed.
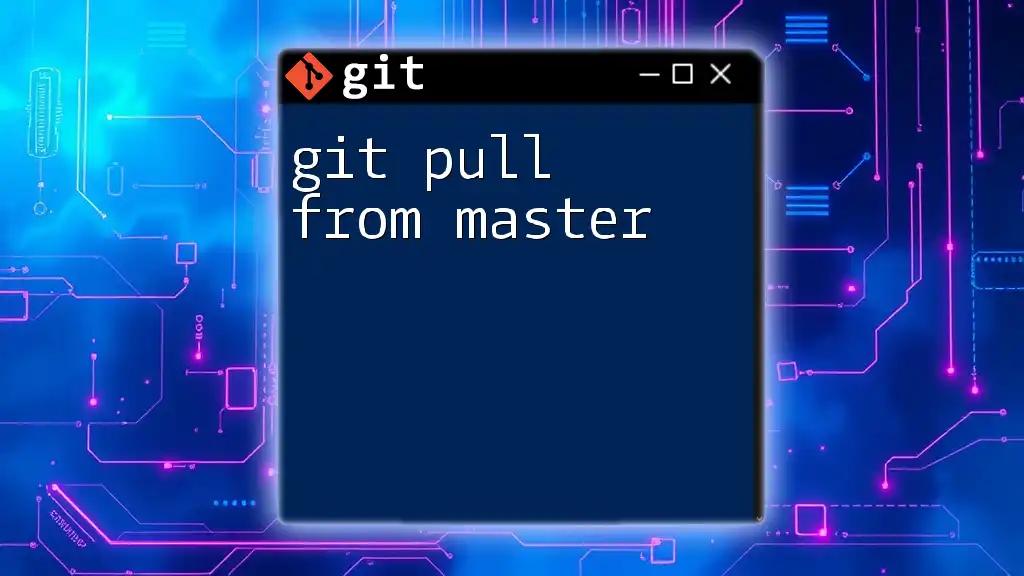
How to Pull Tags from a Remote Repository
Step-by-Step Guide to Pull Tags
Before you can pull tags, ensure you have a proper environment with a local repository that is linked to a remote repository. You can pull tags using the Git command:
git fetch --tags
This command retrieves all tags from the remote repository without modifying any of your local branches. It captures both new tags and updates to existing ones.
Confirming Successfully Pulled Tags
After executing the command to pull tags, you should verify that they were retrieved correctly. Use the following command to list all local tags:
git tag
This command outputs a list of all tags in your local repository, confirming that the pull was successful. If you see the tags you expect, you've successfully synced your local repository with the remote.
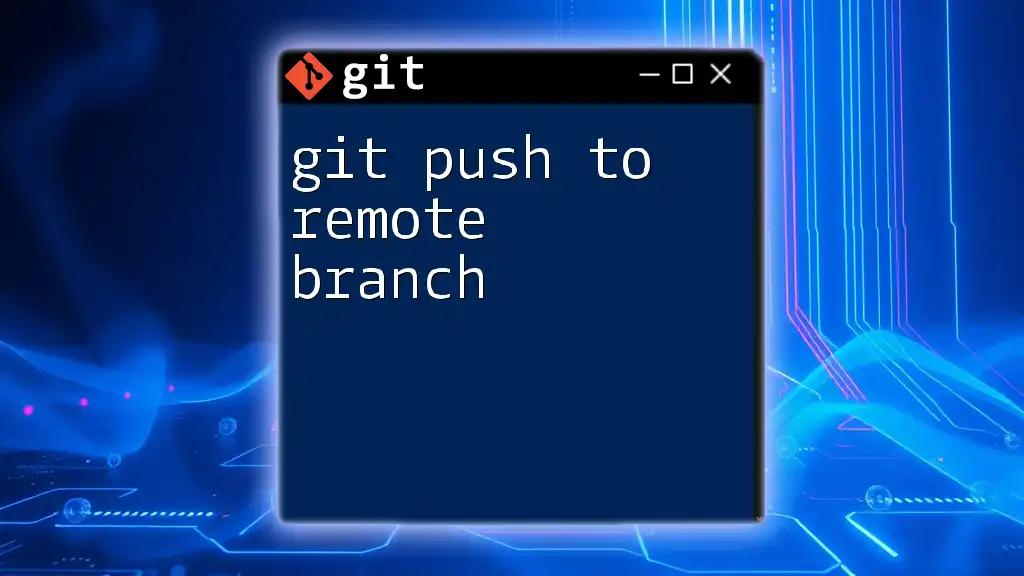
Working with Pulled Tags
Checking Out a Tag
Once you have retrieved tags, you may want to check out a specific tag to inspect the project state at that point in the history:
git checkout <tag-name>
This command will place your working directory in a “detached HEAD” state, meaning you’re not currently on a branch, but rather at a specific point in the history. While in this state, you can review files, run tests, or investigate issues, but keep in mind that any new commits will not be associated with your master branch unless you create a new branch from this state.
Deleting a Remote Tag
If you find that a tag needs to be removed from a remote repository (perhaps due to a versioning mistake), you can delete it with:
git push --delete <remote-name> <tag-name>
This command will remove the specified tag from the remote repository, helping to maintain a clean, accurate tagging system.
Pushing Tags to Remote
When you create tags locally and want to share them with your team, you'll need to push them to the remote. Use:
git push <remote-name> <tag-name>
This command pushes a specific tag to the specified remote, ensuring everyone has access to the latest version markers.
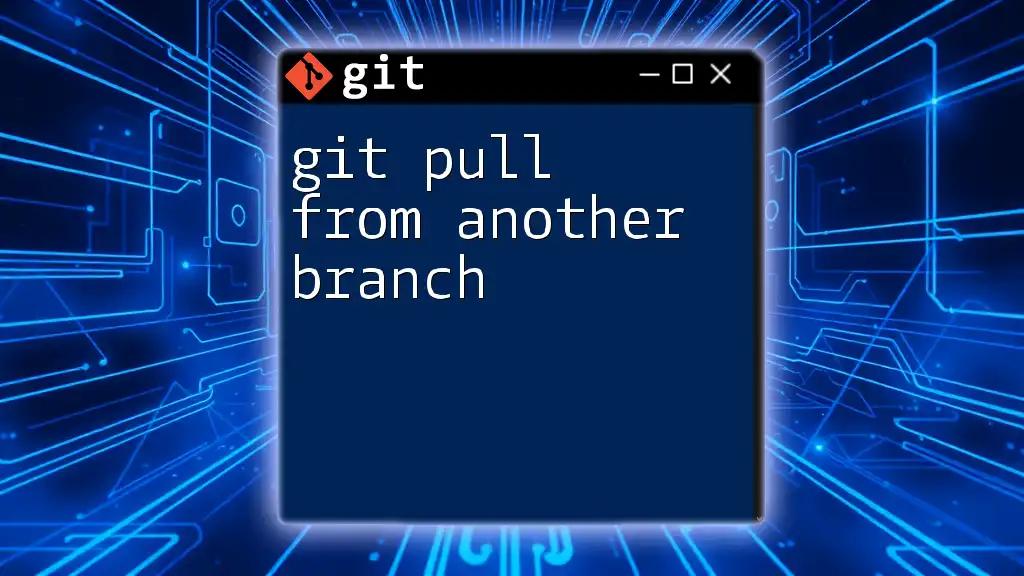
Best Practices for Using Git Tags
Tagging Conventions
It's beneficial to follow a consistent naming convention for tags, especially in collaborative environments. Using semantic versioning (like `v1.0.0`, `v2.1.3`) helps communicate the nature of changes effectively — whether they are major changes, minor updates, or patches.
Keeping Tags Organized
Organizing tags is vital for maintaining a clean project history. Pulling tags regularly ensures that your local environment remains current. Set intervals (e.g., weekly) to fetch tags, and encourage team members to do the same to minimize the chances of version discrepancies.
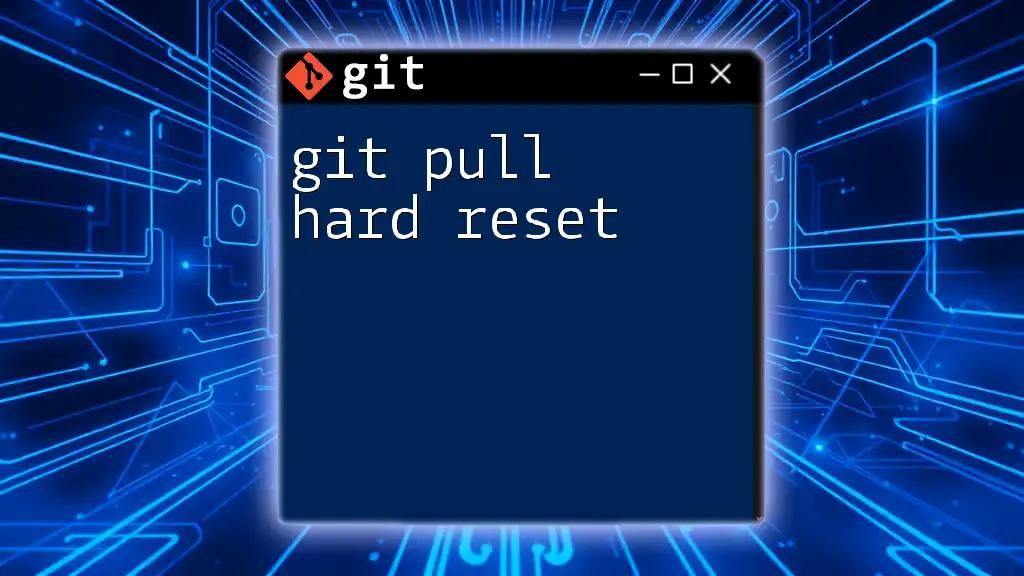
Troubleshooting Common Issues
Common Errors When Pulling Tags
While pulling tags is usually straightforward, you may encounter issues such as:
- Nothing to fetch: This indicates that there are no new tags on the remote repository. It might mean you’ve already synced with the latest tags.
- Could not read from remote repository: This error often suggests issues with your internet connection, Git credentials, or repository configurations. Troubleshooting steps involve checking your remote URL and ensuring you have the necessary permissions.
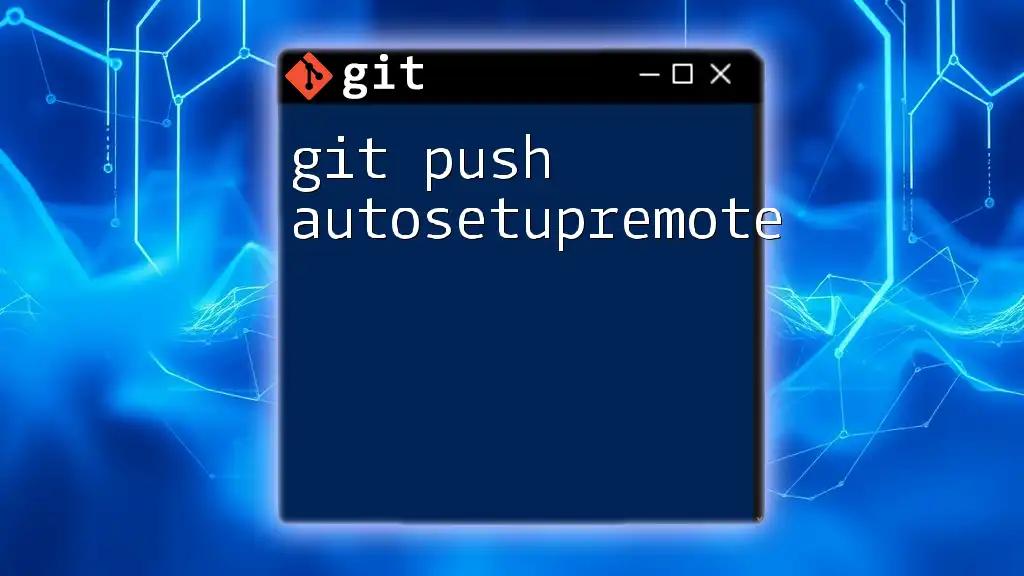
Conclusion
In summary, knowing how to git pull tag from remote is essential for effective version control and collaboration in software development. Tags serve as powerful markers that can simplify navigation through project history and enhance communication among team members. By mastering tag usage in Git, developers can improve their workflows and maintain better project organization.
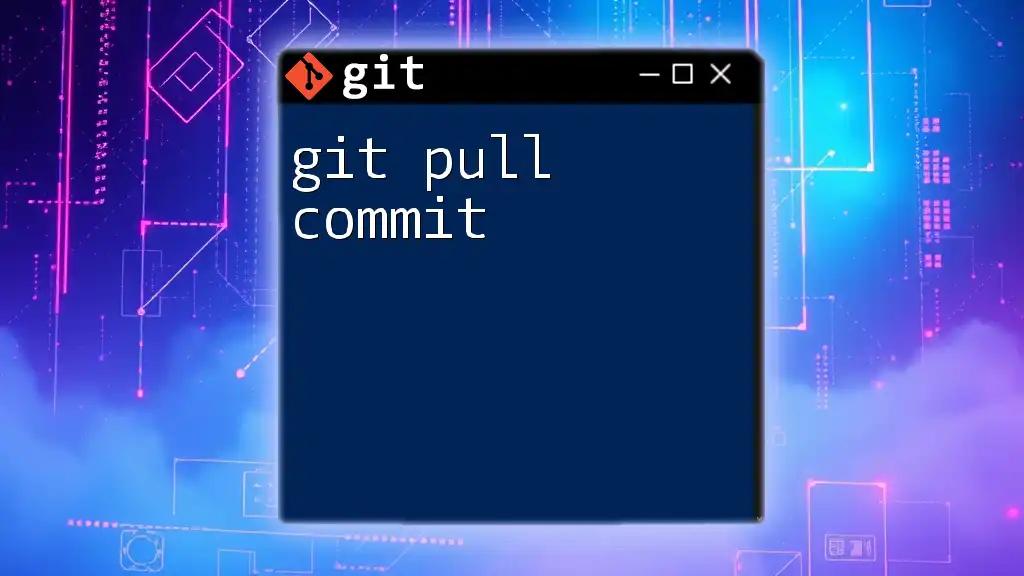
Additional Resources
For further learning, consider checking out the official Git documentation or joining community forums to deepen your understanding of version control and Git operations. This knowledge will empower you to utilize Git commands effectively and enhance your development practices.