To update your current branch with the latest changes from the main branch, use the following command:
git pull origin main
What is Git Pull?
Definition of Git Pull
The `git pull` command serves as a vital tool in your Git toolkit, allowing you to synchronize your local repository with changes made in a remote repository. In simple terms, it fetches changes from a specified branch in the remote repository and merges them into your current branch. By doing so, developers can collaborate effectively, ensuring everyone's work is aligned.
Why You Might Need to Pull from Main
Keeping your feature branches updated with the latest changes from the main branch is crucial for maintaining project integrity. Frequent pulls can prevent potential merge conflicts later in the development process and ensure that the feature you’re working on is developed based on the most current codebase. In a collaborative environment, this also allows different team members to integrate their work more effectively.
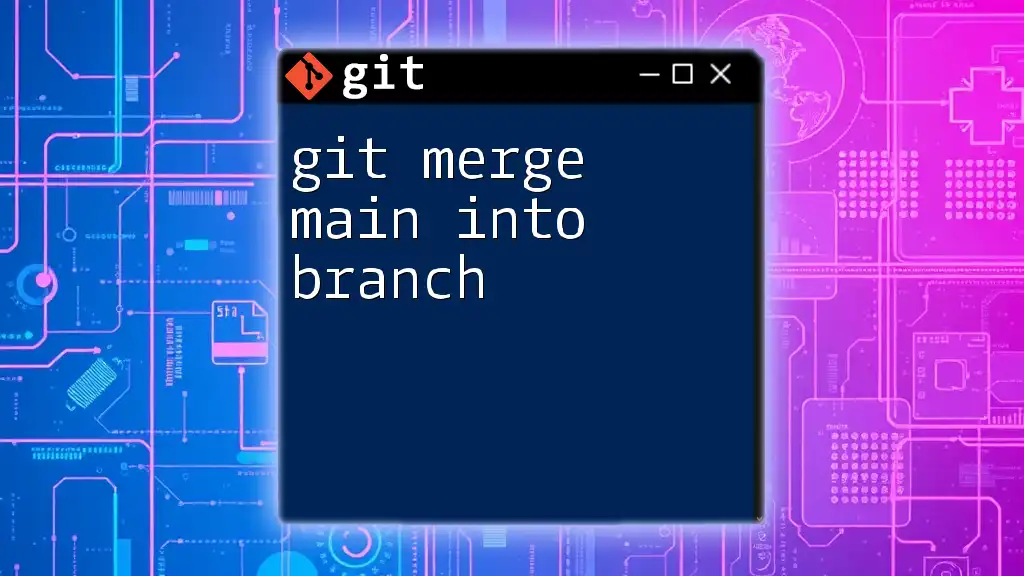
Understanding Branches in Git
What is a Branch?
A branch in Git represents a separate line of development. By creating branches, developers can work on new features, fix bugs, or experiment without affecting the main codebase directly. This enhances productivity, as multiple features can be developed simultaneously in isolation.
Common Branching Strategies
Several branching strategies exist to streamline project organization. Two popular methods include:
- Feature Branching: Each new feature is developed in its dedicated branch, which is later merged back into the main branch when complete.
- Git Flow: This strategy employs multiple branches for development, staging, and production, allowing for a structured development process.
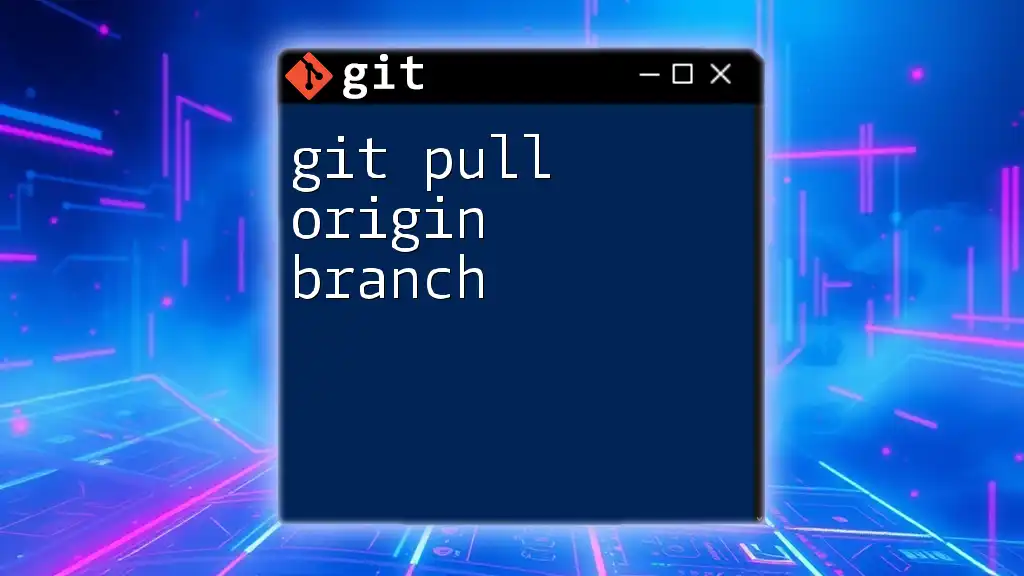
The Main Branch
What is the Main Branch?
The main branch, often named `main` or historically `master`, serves as the central repository of a project's stable and production-ready code. This branch is where completed features and verified code reside, making it critical for the team’s overall success.
Best Practices for the Main Branch
To maintain the main branch's reliability, follow these best practices:
- Ensure that the main branch is always in a deployable state.
- Implement strict review processes to prevent untested code from being merged.
- Utilize continuous integration (CI) tools to automate testing and deployment.
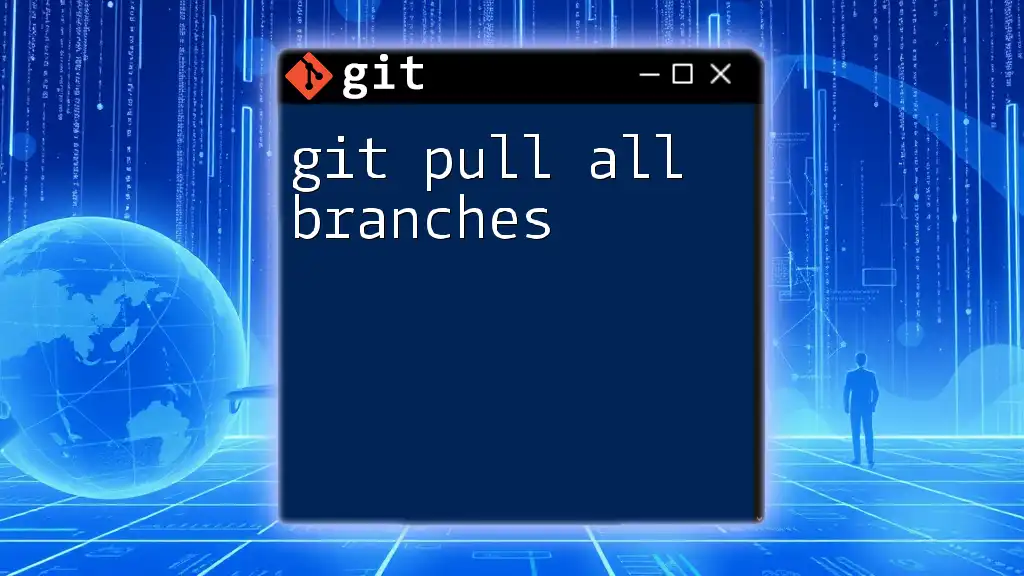
The Command: `git pull main into branch`
The Mechanics of the Command
To integrate changes from the main branch into your current working branch, you essentially execute the `git pull` command directed at your remote repository's main branch. The general structure looks like this:
git pull origin main
This command tells Git to fetch the latest updates from the `main` branch of the `origin` remote, and subsequently merge those changes into your current branch.
Typical Usage
Imagine you're in a feature branch (`feature-branch`) and need to update it with the latest from the main branch:
git checkout feature-branch
git pull origin main
This sequence first switches you to the `feature-branch` and then pulls updates from `main`, ensuring your feature development is based on the latest codebase.
Key Considerations
When using `git pull`, you might encounter merge commits or fast-forward merges. A merge commit occurs when there are changes in both branches, requiring a combined history, while a fast-forward merge happens when your branch is behind the main branch but has no diverging changes.
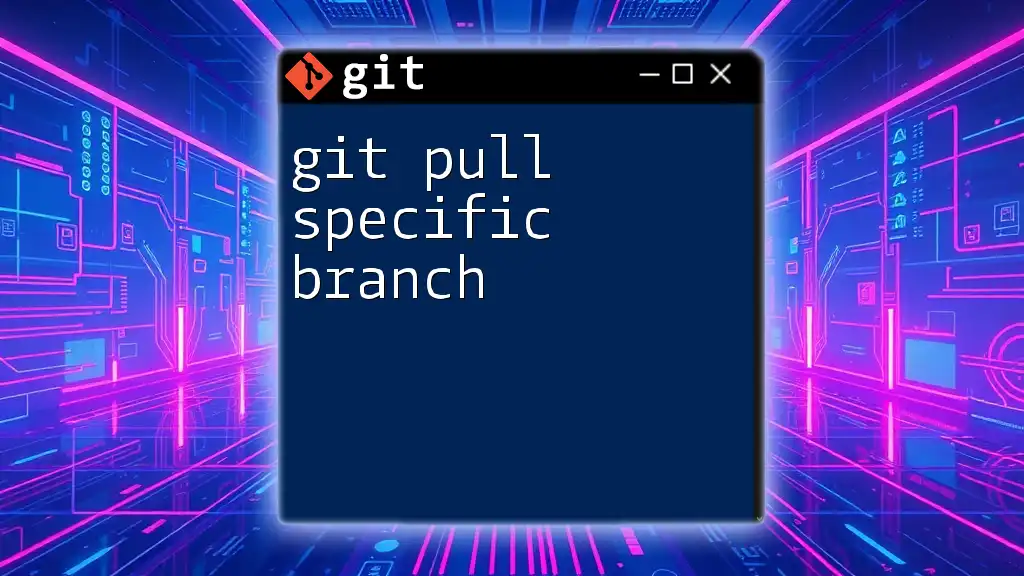
How to Pull from Main into Your Branch
Step-by-Step Guide
Step 1: Checkout Your Branch
To begin, you need to switch to your feature branch where you want to apply the updates from main:
git checkout feature-branch
This command sets your working directory to work on the specified feature branch.
Step 2: Pull from Main
Now that you're on the feature branch, execute the following command to pull the changes from the main branch:
git pull origin main
This command merges the latest changes from the main branch into your current branch.
Example Code Snippet
Here’s the complete sequence of commands for clarity:
git checkout feature-branch
git pull origin main
After running these commands, you will have the most recent changes from the main branch integrated into your feature branch.
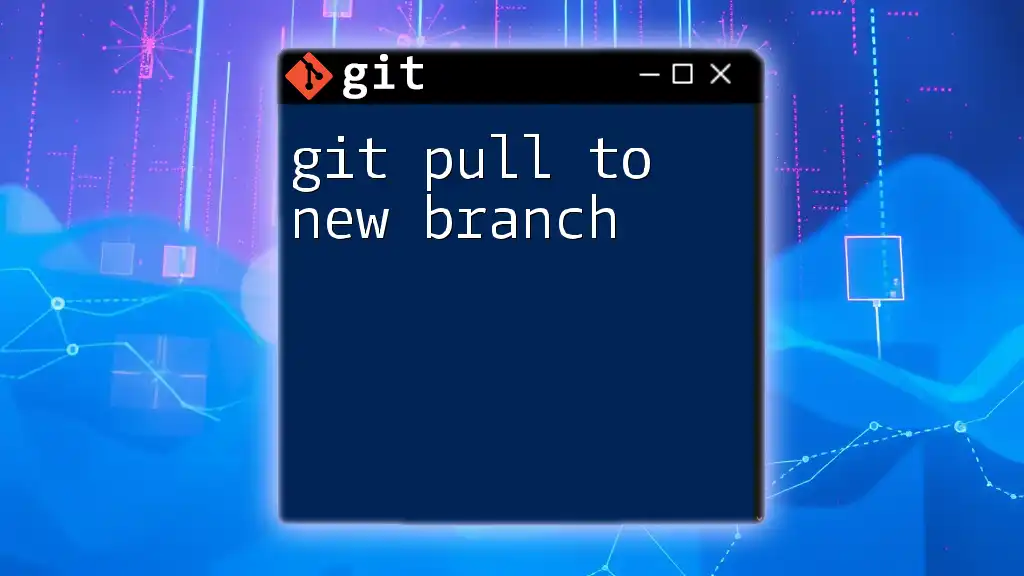
Handling Merge Conflicts
What are Merge Conflicts?
Merge conflicts arise when the same lines in a file have been modified differently in your feature branch and the main branch. This scenario prevents Git from automatically merging changes, necessitating manual intervention to resolve the conflict.
Resolving Merge Conflicts
When you encounter a merge conflict, Git will notify you, and you will see conflict markers in the affected files. Here’s a simplified approach to resolve these conflicts:
-
Identify Conflicted Files: Use `git status` to see which files are affected.
-
Open Affected Files: Look for conflict markers `<<<<<<<`, `=======`, and `>>>>>>>`.
-
Edit the File: Resolve the conflict by choosing which changes to keep or by merging the changes manually.
-
Mark as Resolved: Once resolved, add the file to staging:
git add <filename>
-
Complete the Merge: Finally, commit the resolved changes:
git commit -m "Resolved merge conflict"
Useful Tools for Conflict Resolution
To simplify conflict management, various tools can assist, such as GitKraken, SourceTree, or the built-in tools in code editors like VSCode. These tools provide visual interfaces that make resolving conflicts more intuitive.
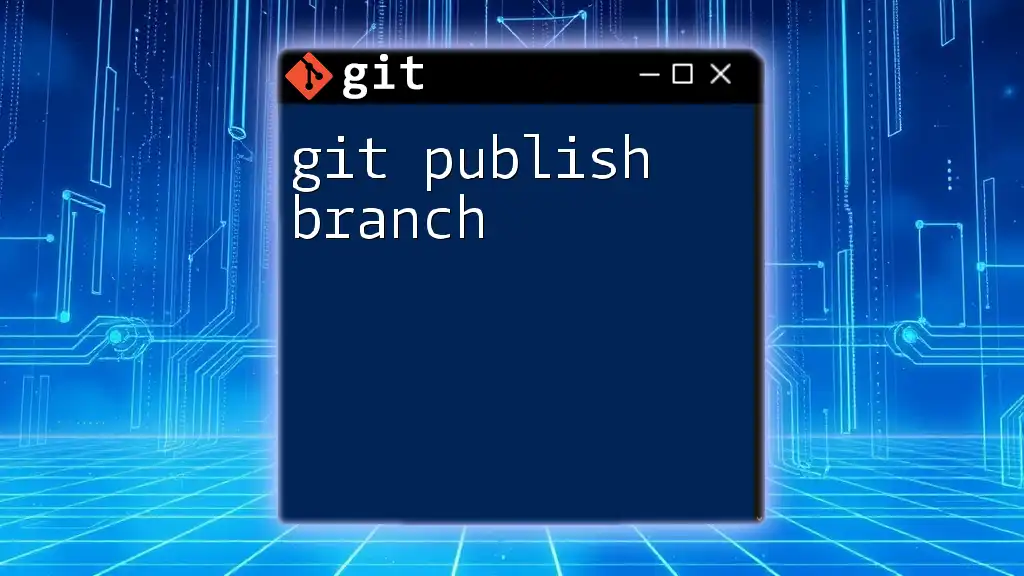
Verifying Changes After Pulling
Review the Changes
After executing `git pull`, it's vital to assess the changes that have merged. Use the following commands to review:
git log
git diff
The `git log` command shows the commit history, while `git diff` displays the differences between your current changes and the previous state.
Testing and Validation
Testing is essential after integrating changes. Ensure that your feature branch maintains functionality by running unit tests or performing manual tests on the affected features. It guarantees that the integration has not introduced any regression issues.
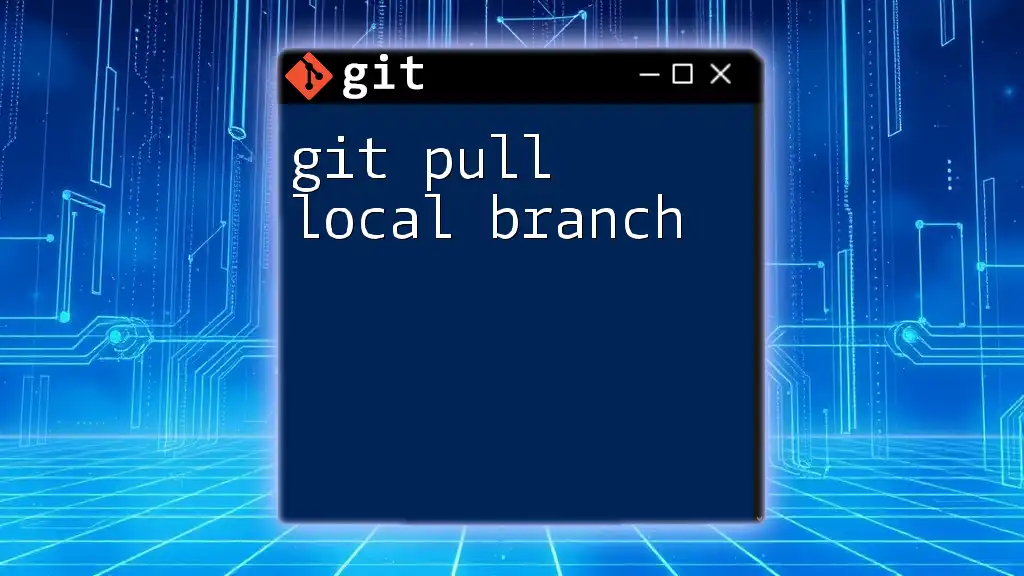
Conclusion
Pulling changes from the main branch into your feature branch is a fundamental practice in version control using Git. It facilitates keeping your development work in sync and significantly minimizes issues during collaboration. Implementing this practice not only streamlines your workflow but also enhances code quality.
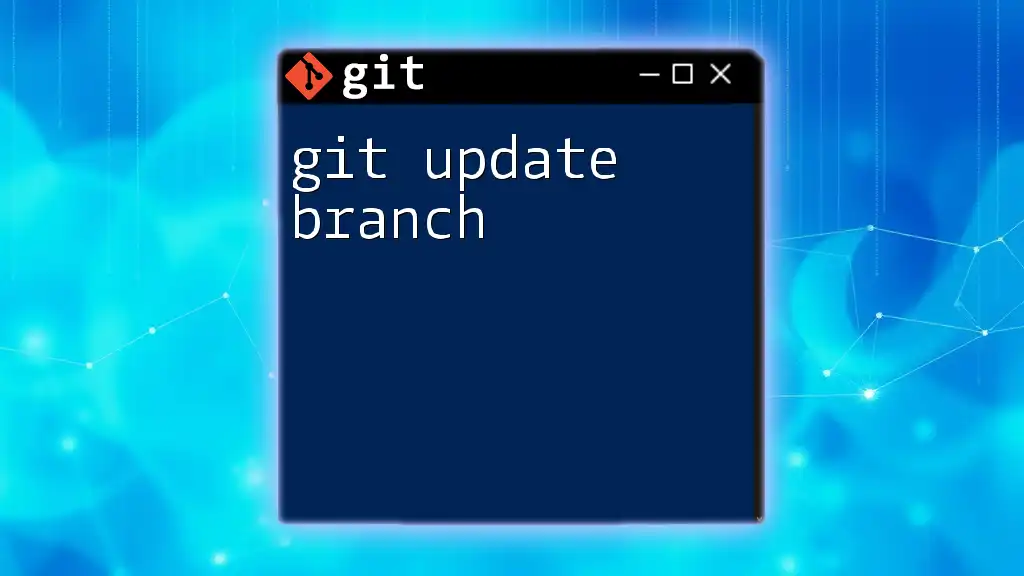
FAQ Section
Common Questions
-
What if I have uncommitted changes?
To integrate changes safely, consider stashing your work first using `git stash`, then perform the pull before applying your stash. -
Can I pull the main branch into multiple feature branches at once?
While you cannot pull into multiple branches simultaneously using a single command, you can repeat the pull command for each feature branch. -
How do I revert a pull if something goes wrong?
You can reset your branch to a previous state using `git reset --hard HEAD~1` (this will remove the latest pull). Use this command cautiously, as it will discard any changes made since the last commit.
Additional Resources
For more insights, consider reviewing the [official Git documentation](https://git-scm.com/doc) or exploring tutorials on effective Git command usage. With practice, you'll quickly become proficient in merging and integrating code across branches, ensuring a seamless collaboration experience.