Git maintenance refers to the regular tasks you perform to ensure your repository remains efficient and organized, such as cleaning up unnecessary files and optimizing the history.
Here's a code snippet to remove unused branches and clean up your repository:
git branch --merged | grep -v '\*' | xargs -n 1 git branch -d
Understanding the Git Workflow
Overview of Git Commands
To effectively perform git maintenance, it's crucial to have a solid understanding of basic Git commands. Popular commands include `git commit`, `git push`, and `git pull`, but maintenance-specific commands like `git branch`, `git gc`, and `git stash` play a key role in ensuring your repository's health.
Having a good grasp of these commands enables you to manage your code effectively and streamline your development workflow.
The Git Directory Structure
Understanding the Git directory structure is essential for efficient maintenance. The `.git` folder located at the root of your repository contains all the necessary information for your project, including:
- Branches: Your project's different lines of development.
- Configuration: Settings for Git repository specifics.
- Hooks: Scripts that run on specific commits or actions.
- Objects: All versions of your files, represented in a compressed format.
Being familiar with this structure helps diagnose issues and enrich your Git maintenance practices.
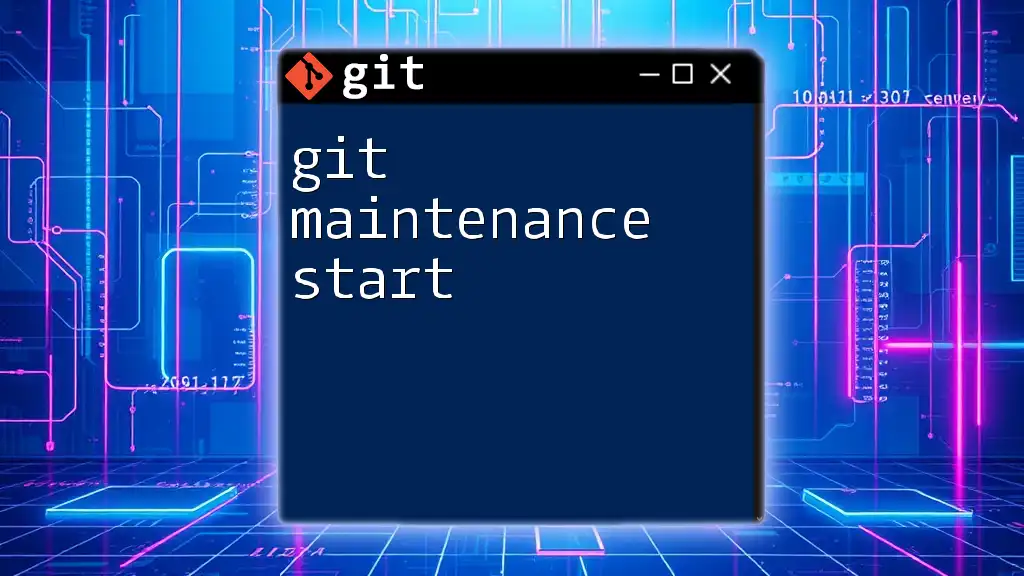
Regular Git Maintenance Tasks
Cleaning Up Unused Branches
Old branches can clutter your repository, making it challenging to track active development. Regularly cleaning up these branches is an important aspect of git maintenance.
To view all branches in your repository, use the following command:
git branch --list
To delete a branch that is no longer in use, you can execute:
git branch -d branch-name
Replace `branch-name` with the actual name of the branch you wish to delete. This streamlines your branch list and clarifies which branches are relevant.
Pruning Remote Tracking Branches
As your team pushes and removes branches, your local repository can accumulate stale remote tracking branches. Pruning these can help maintain clarity in your development environment.
You can prune all stale remote-tracking branches using:
git remote prune origin
This command cleans up your local view of the remote repository and ensures you only see active branches.
Stashing Uncommitted Changes
Sometimes you may find yourself needing to work on a different task without completing your current one. This is where stashing becomes invaluable.
To stash your current changes, simply run:
git stash
Once stashed, your workspace is clean. You can easily return to your changes later with:
git stash apply
This is particularly useful when juggling multiple tasks in parallel.
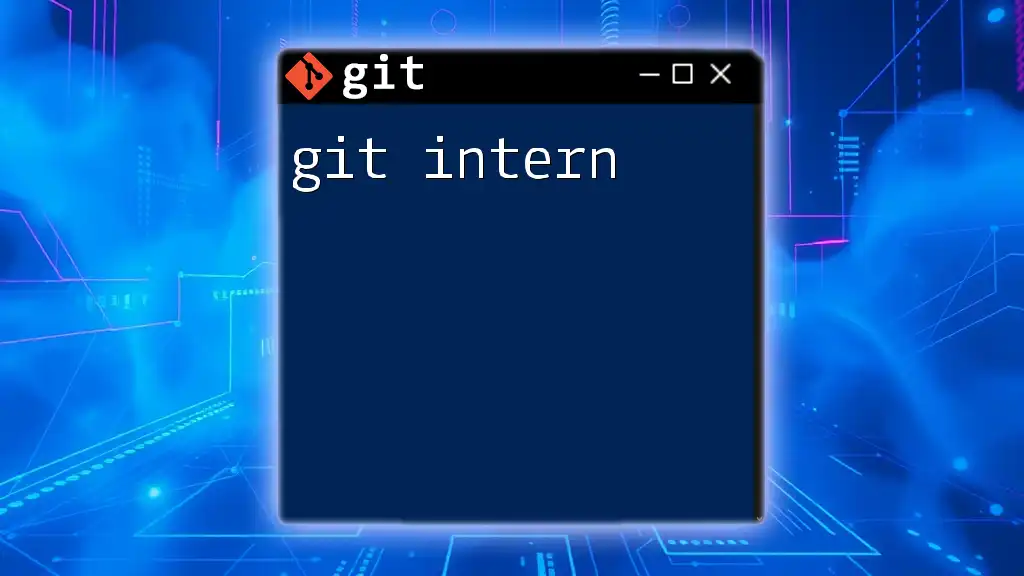
Optimizing Git Repository Performance
Garbage Collection
Over time, your Git repository can accumulate unnecessary files and data, leading to decreased performance. Garbage collection helps clean up these unreferenced objects, boosting efficiency. To run garbage collection, use:
git gc
This command should be executed periodically to keep your repository organized and performant, especially if it has been heavily modified.
Repackaging Loose Objects
Git stores files as loose objects when they are first created. Over time, these can become unwieldy. Repackaging them into a single, packed file can improve speed and efficiency.
To repack your repository, run:
git repack
This command is highly beneficial for larger repositories or those experiencing slow performance due to numerous loose objects.
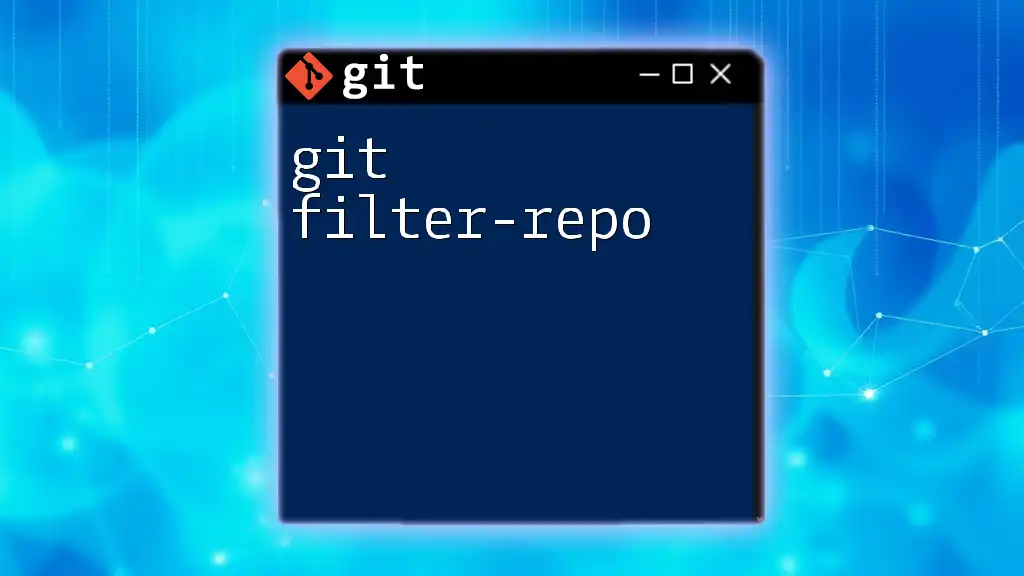
Keeping Git History Clean
Using Commit Messages Effectively
Well-structured commit messages not only help you understand changes but are also crucial for team collaboration. Adopting a consistent format, such as the conventional commits approach, can significantly enhance your project history's clarity.
Consider including the following elements in your commit messages:
- Type of change: (e.g., feat, fix, docs)
- Scope: Where the change is applied (e.g., "UI", "backend")
- Short description: Briefly describe the change.
An example of a clear commit message could be:
feat(UI): improve button responsiveness
Squashing Commits
Squashing commits condenses multiple related commits into a single logical change, cleaning up your history. To squash the last n commits, use:
git rebase -i HEAD~n
This opens an interactive rebase editor where you can choose which commits to squash. This technique helps to maintain a cleaner history that’s easier to understand.
Performing Interactive Rebase
Interactive rebasing is a powerful way to edit, delete, or squash commits. By executing:
git rebase -i HEAD~n
You can modify your commit logs freely. This method fosters stronger repository mechanics, as it allows you to polish your commit history before pushing it to shared repositories.
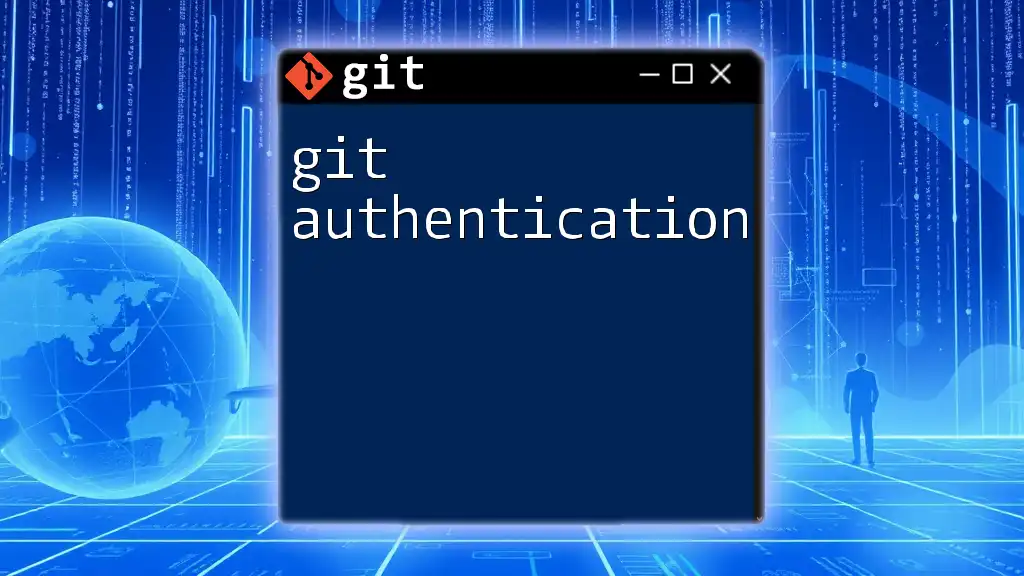
Advanced Maintenance Tasks
Managing Merge Conflicts
Merge conflicts can arise for numerous reasons, such as simultaneous changes in the same line of a file. Understanding how to resolve these conflicts is crucial for a smooth workflow.
First, identify the conflicting files – Git will indicate these during a merge operation. You resolve conflicts directly in your editor, then mark them as resolved with:
git add conflicted-file
This approach minimizes disruptions to your development flow and keeps your repository in good health.
Monitoring Repository Health
To ensure optimal performance, regularly gauge your repository's health. Use the `git fsck` command to check for integrity issues:
git fsck
This command scans the repository for errors and missing objects, allowing you to address potential problems before they escalate.
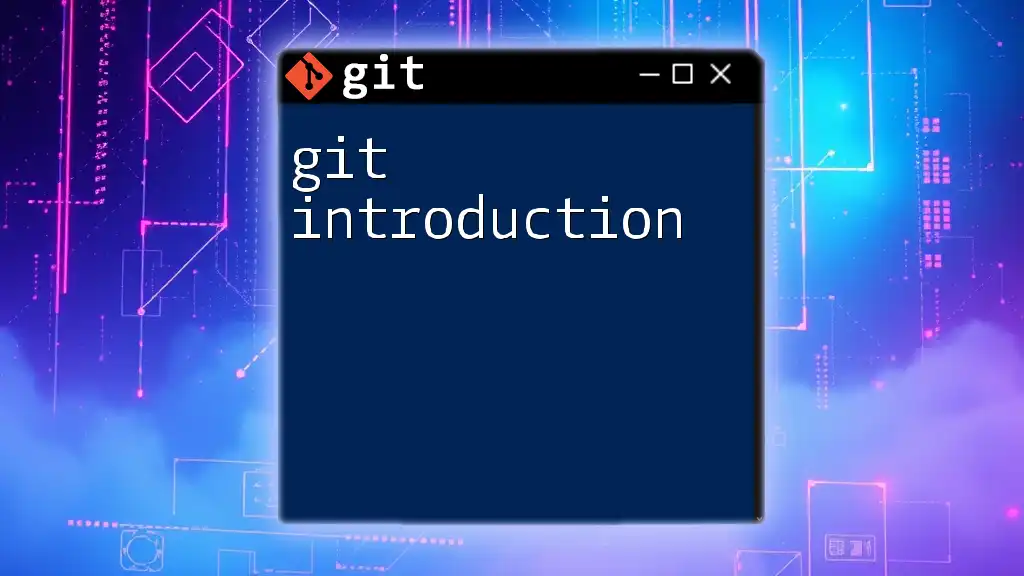
Conclusion
Regular git maintenance is indispensable for optimal performance and a clean development environment. By implementing the practices outlined above, such as cleaning up unused branches, effectively managing commits, and monitoring repository health, you enhance both individual and team productivity.
Incorporating git maintenance into your routine not only improves repository management but also fosters a culture of professionalism and efficiency within the development team.
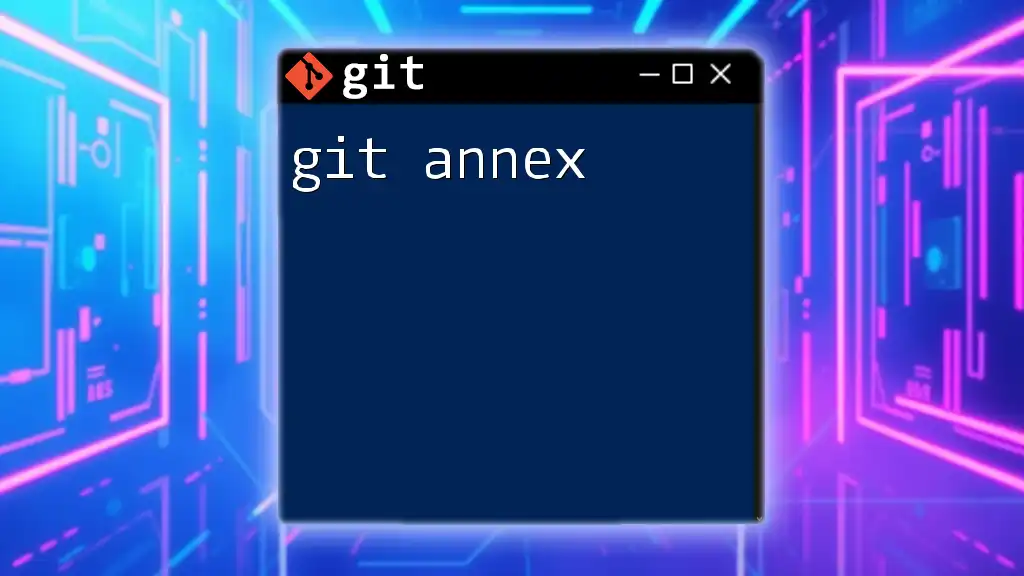
Additional Resources
For further reading, consider exploring the official Git documentation, which offers a wealth of information on advanced concepts and commands. Additionally, seeking out community forums where Git users share experiences and solutions can greatly enrich your understanding.
Call to Action
Embrace git maintenance as a regular part of your workflow. The healthier your repository, the smoother your development process will be. Subscribe to stay updated on best practices and tips for mastering Git commands!