"Git masters are skilled individuals who seamlessly navigate version control systems using powerful commands to manage and collaborate on software projects efficiently."
Here's a simple git command to clone a repository:
git clone https://github.com/username/repository.git
What is a Git Master?
A Git Master is an individual who has mastered the intricacies of the Git version control system, enabling them to effectively manage code, collaborate in teams, and maintain a clean and efficient workflow. In today’s development environment, understanding Git not only boosts personal productivity but also enhances collaboration with team members.
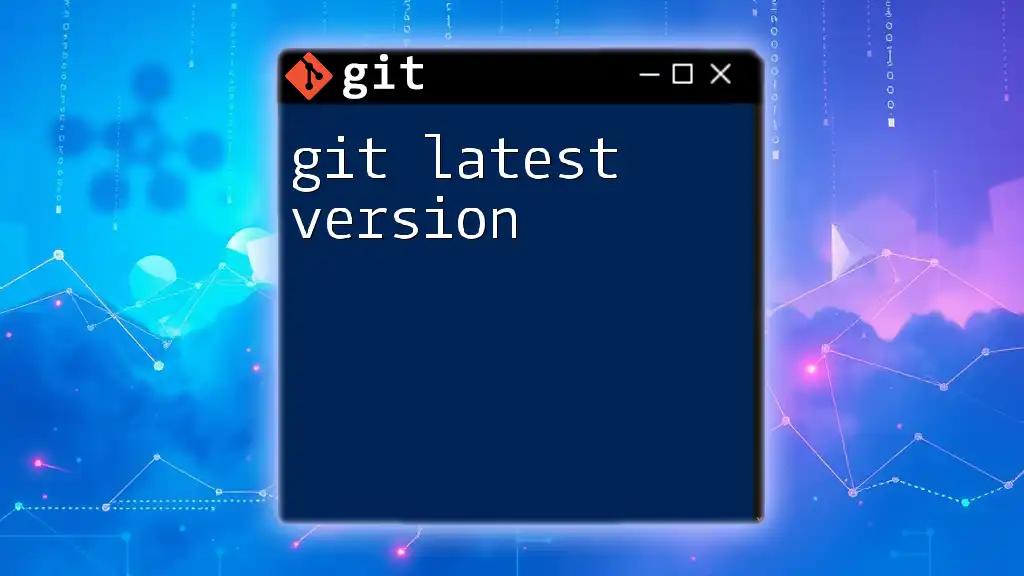
Why Strive to Become a Git Master?
Mastering Git signifies more than knowing commands; it means being proficient at using Git as a powerful tool for collaboration and version control. Git Masters can tackle complex development tasks effortlessly, resolve conflicts swiftly, and maintain a clear history of every code contribution. This expertise can elevate an entire team’s performance and facilitate seamless project management.
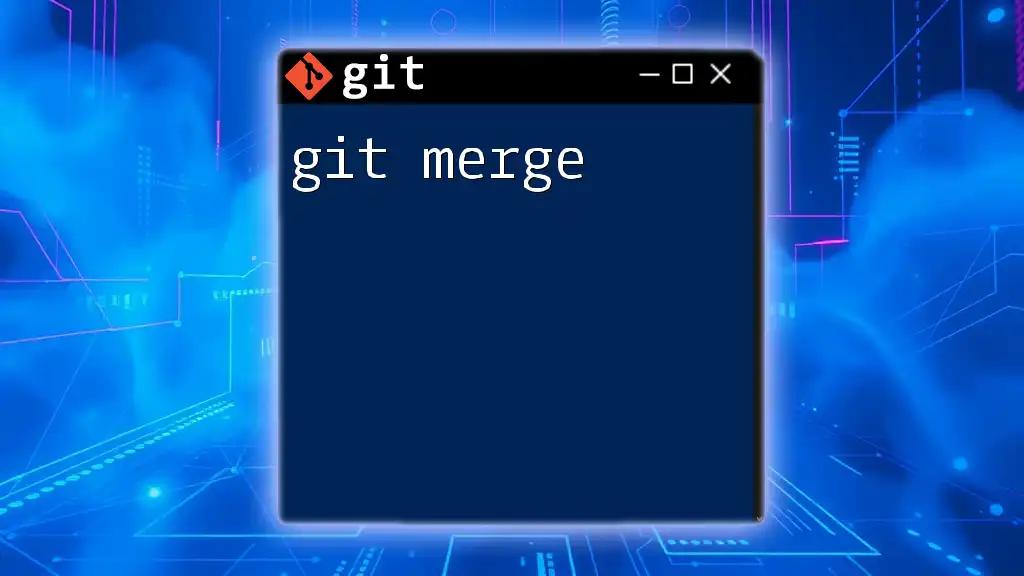
Understanding Git Fundamentals
What is Git?
Git is a distributed version control system designed for tracking changes in source code during software development. It allows multiple developers to work on the same project simultaneously without overwriting one another's contributions.
Core Concepts of Git
- Repository: A repository (repo) is where all the project files, including their revision history, reside.
- Commit: A commit records changes made to the repository. It serves as a snapshot of the project's state at a specific moment.
- Branch: Branches allow developers to work on different versions or features of a project in isolation from the main branch.
- Merge: Merging combines changes from different branches. This process is essential for integrating features developed in isolation.
- Remote: A remote repository is a version of your project that is hosted on the internet or another network. It can be accessed by multiple developers.
The Role of Git in Software Development
Using Git significantly enhances collaboration among team members and keeps track of every change made in the codebase. This facilitates not only version tracking but also revisiting earlier code versions when needed, ensuring that developers can work efficiently and flexibly.
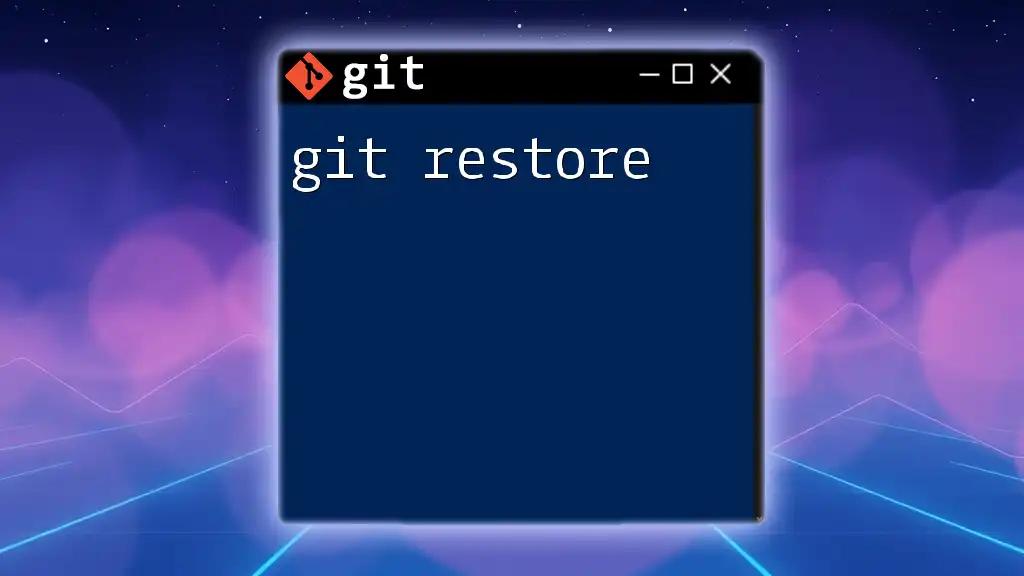
Essential Git Commands Every Master Should Know
Navigating the Git Terrain
To start with Git, you first need to create a repository. This is done using the command:
git init my-new-repo
This command initializes a new Git repository in the my-new-repo directory, setting the stage for tracking changes.
Tracking Changes
Once you have your repository set up, tracking changes involves adding and committing:
git add file.txt
git commit -m "Initial commit"
With `git add`, you stage changes, while `git commit` saves those changes to the repository with a descriptive message explaining what was altered.
Working with Branches
Branches are crucial for managing features or fixes without affecting the main codebase.
Creating and Managing Branches
To create a new branch, you can use:
git branch new-feature
This command creates a new branch called new-feature.
Switching Branches
To work on this new branch without merging it to the main branch, switch to it using:
git checkout new-feature
Merging and Resolving Conflicts
When you've finished developing a feature in a separate branch, it's time to integrate it back into the main branch.
Merging Branches
To merge your newly created feature into the main branch, first switch to the main branch, then execute:
git checkout main
git merge new-feature
Handling Merge Conflicts
Sometimes, changes made in different branches conflict. Git will prompt you to resolve these conflicts manually. Reviewing the conflicting files, you can either choose which changes to keep or use a merge tool with:
git mergetool
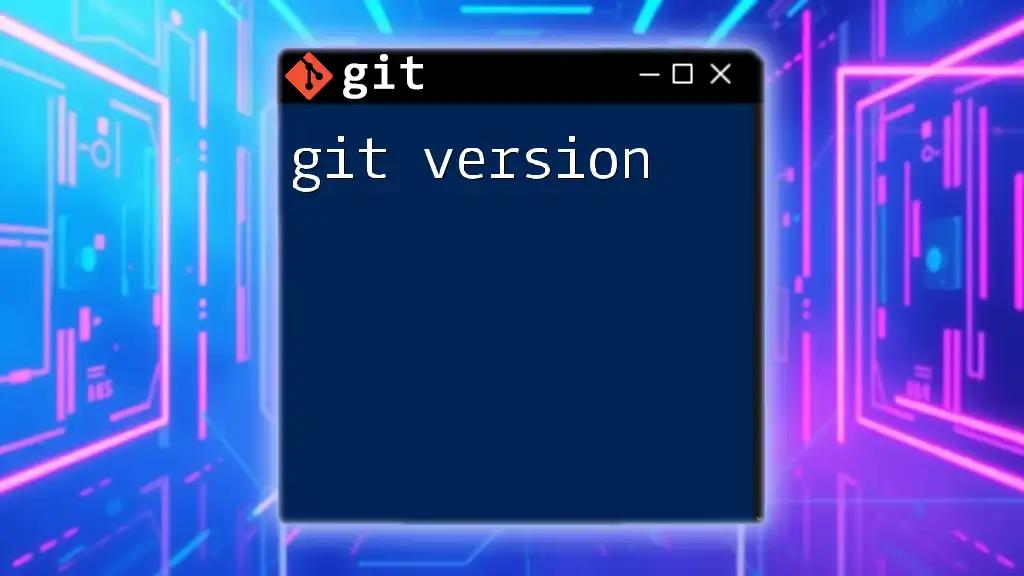
Remote Repositories and Collaboration
Understanding Remotes
Remote repositories are essential for collaboration, allowing multiple developers to push and pull code changes to shared locations.
Adding a Remote Repository
To link your local repository with a remote one, use:
git remote add origin https://github.com/user/repo.git
This command connects your local repository with a remote GitHub repository.
Pushing and Pulling Changes
Pushing to Remote Repositories
When you're ready to share your changes with others, upload your commits using:
git push origin main
This command sends your local changes to the main branch of the remote repository.
Pulling Changes from Remote
To integrate changes made by others into your branch, use:
git pull origin main
This command fetches changes from the remote repository and merges them into your local branch.
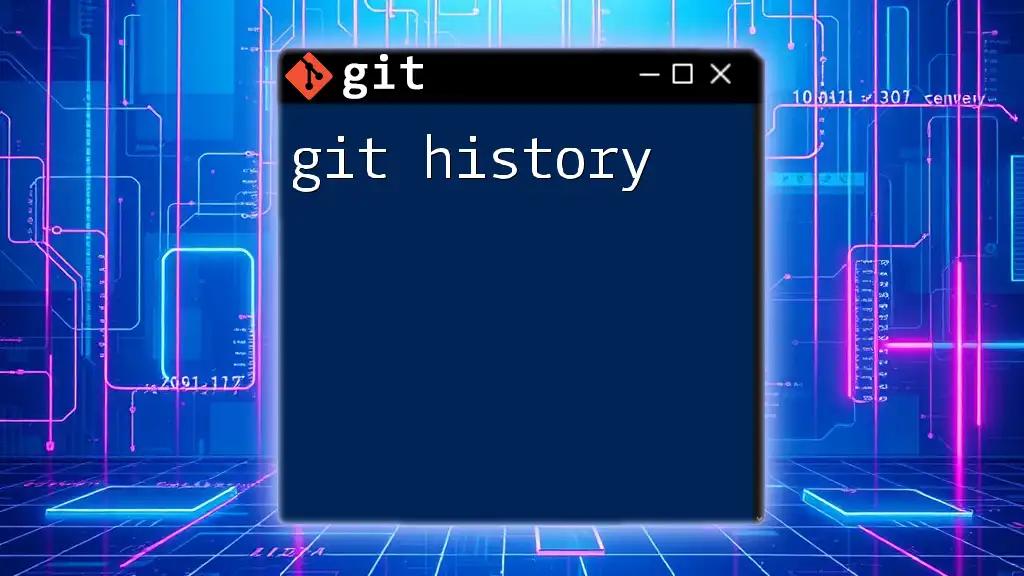
Advanced Git Techniques for Masters
Rebasing vs. Merging
Rebasing and merging are two methods for integrating changes from one branch to another. Rebasing rewrites commit history to create a linear progression, while merging preserves the branch structure.
When rebasing, you might run:
git rebase main
This effectively replays your commits on top of the latest commits from the main branch.
Using Git Stash for Better Workflow
At times, you may need to switch branches before completing your current work. For such scenarios, Git stash lets you save your unsaved changes temporarily:
git stash
You can retrieve these stashed changes later with:
git stash pop
Interactive Rebase
Interactive rebasing allows you to edit your commit history, enabling you to squash or reorder commits.
To start an interactive rebase, use:
git rebase -i HEAD~3
This command allows you to modify the last three commits, providing greater control over your commit history.
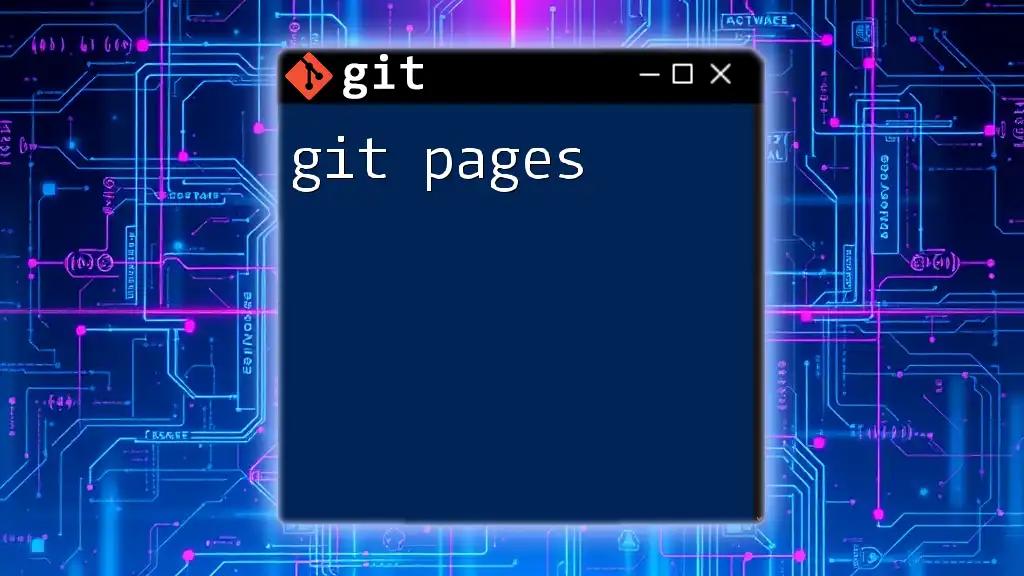
Best Practices for Git Masters
Commit Messages: Crafting the Perfect Message
A good commit message should be concise yet descriptive. It should convey what changes were made and why. A well-written commit history is invaluable for anyone reviewing the project later.
Branch Naming Conventions
Effective branch names clarify the purpose of the branch. Using a naming scheme like feature/your-feature-name or bugfix/your-bug-name helps maintain organization and clarity.
Code Review Strategies
Git is intimately connected to the code review process. Utilize platforms like GitHub and GitLab to facilitate pull requests, allowing team members to review code before it merges into the main branch. This fosters discussions around code quality, encourages feedback, and ultimately leads to better software.
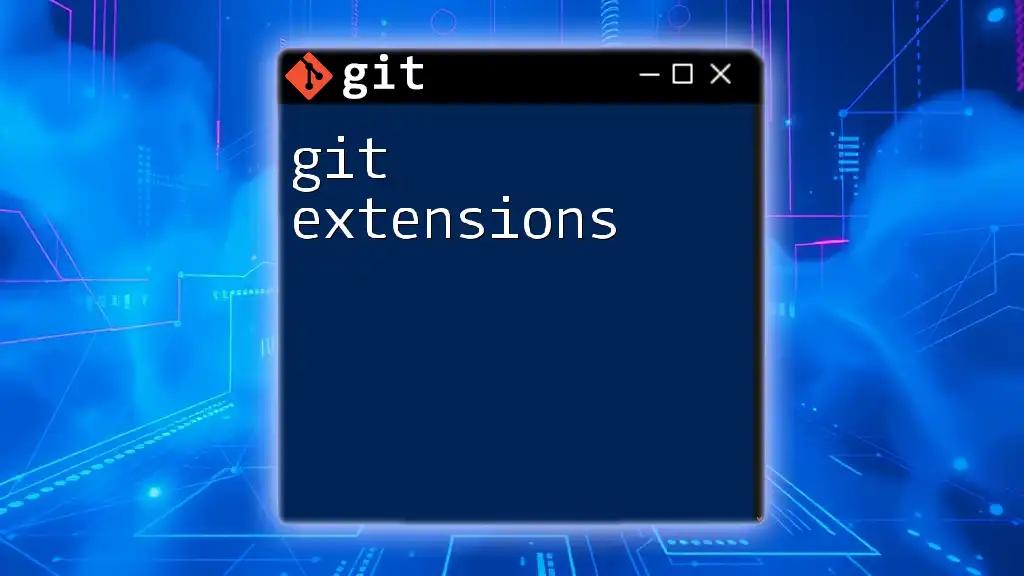
Conclusion
Becoming a Git Master transcends simply knowing the commands; it involves adopting a philosophy of collaboration, cleanliness, and predictive problem-solving. Mastery of Git equips developers with an invaluable toolkit for navigating complexities in team environments, ensuring smoother collaboration and better version control.

Additional Resources
For continued growth in Git proficiency, look for recommended books and online courses covering both foundational and advanced topics, alongside useful Git tools and plugins available for enhancing your workflow.