"Git issues refer to the challenges or problems you may encounter while using Git, such as conflicts during merges or problems with remote repositories, which can often be resolved through various commands."
Here’s a command snippet to check the status of your Git repository and identify any issues:
git status
Understanding Git Issues
What Are Git Issues?
Git issues refer to the challenges or problems encountered while using Git, a version control system widely adopted in software development. These issues can range from simple scenarios like forgetting to stage changes to more complex situations such as merge conflicts or a detached HEAD state. Understanding and effectively managing these git issues is crucial in maintaining seamless collaboration and ensuring a smooth development workflow.
The Git Issue Workflow
A well-defined Git issue workflow can significantly enhance your projects. The workflow typically involves identifying an issue, troubleshooting it, applying a resolution, and communicating with your team about the changes made. This process ties closely with Git branches and commits, ensuring that your project history remains clean and understandable.
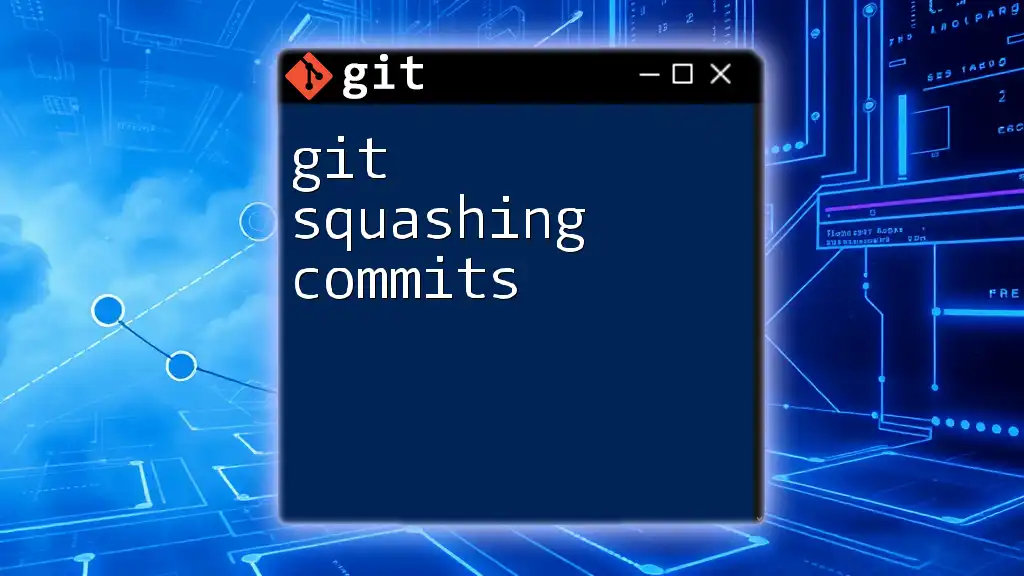
Common Issues in Git
Merge Conflicts
Merge conflicts occur when Git is unable to automatically resolve differences between two branches that are being merged. This usually happens when two or more contributors have made changes to the same line in a file.
Example: Imagine two developers, Alice and Bob, are working on the same file in separate branches. When Alice merges her branch into the main branch, she inadvertently alters a line that Bob has also changed in his branch.
Code Snippet: The conflicting section in the code might look like this:
<<<<<<< HEAD
print("Alice's changes")
=======
print("Bob's changes")
>>>>>>> bob-branch
To resolve merge conflicts, follow these steps:
-
Identify conflicting files using:
git status
-
Open the affected files and look for conflict markers (`<<<<<<<`, `=======`, `>>>>>>>`).
-
Carefully modify the code, choosing how to merge changes.
-
After resolving the conflicts, stage the changes:
git add <filename>
-
Finally, commit the resolved changes:
git commit -m "Resolved merge conflict"
To avoid merge conflicts, it’s best to communicate with your teammates about ongoing changes and to use shorter-lived branches for features.
Detached HEAD State
The detached HEAD state occurs when you check out a commit that is not the tip of a branch. This situation can make it tricky to return to your ongoing work because you are not on a branch.
Example: You might end up in a detached HEAD state when you run a command like:
git checkout <commit-hash>
If you need to recover from a detached HEAD state, follow these steps:
-
Identify where you are by checking the status:
git status
-
Reattach your HEAD to an existing branch:
git checkout <branch-name>
-
If you made changes while in detached HEAD, create a new branch to preserve your work:
git checkout -b new-branch
Using descriptive branch names can help you avoid confusion and detachment in the future.
Unstaged Changes
Unstaged changes refer to modifications made to files that Git has not yet staged for commit. It's easy to lose track of which changes are ready to be committed and which are not.
Code Snippet: You can check the status of your working directory with:
git status
If you see files listed as "modified," it indicates unstaged changes. To fix this, you can stage the desired changes using:
git add <filename> # This stages a specific file
or use:
git add . # This stages all modified files in the current directory
Fixing Unstaged Changes
To remove unstaged changes, if they are not needed, you can revert them back to the last committed state with:
git checkout -- <filename>
Alternatively, if you want to stage the changes correctly, implement a consistent practice of checking your `git status` frequently to maintain awareness of your working directory’s state.
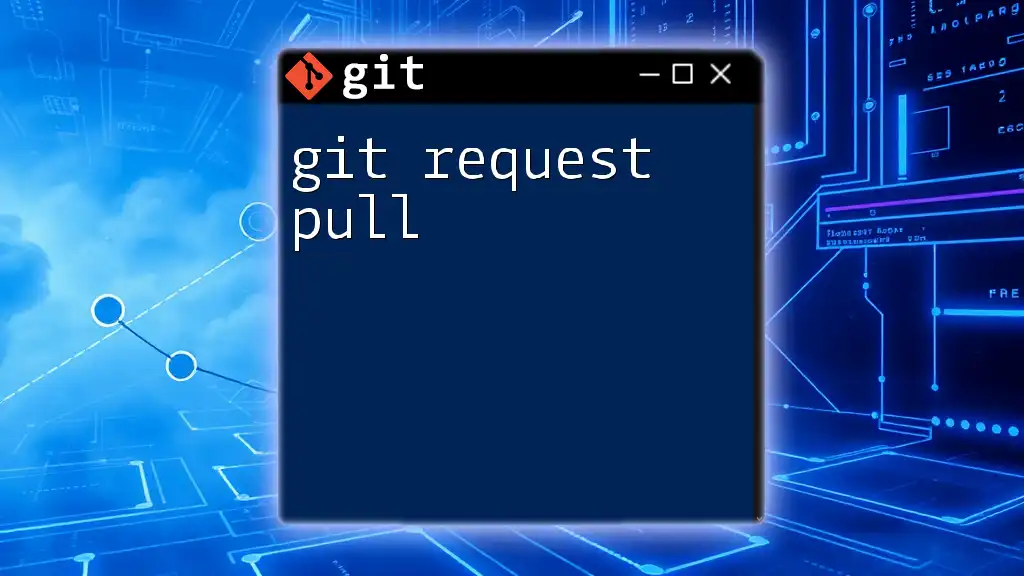
Identifying Issues with Git Commands
Using `git status`
The `git status` command is among the most crucial tools for identifying and understanding git issues. It provides a summary of which files have been modified, staged, or are untracked in your current repository state.
Code Snippet:
git status
The output typically includes sections such as:
- Changes to be committed
- Changes not staged for commit
- Untracked files
Regular use of `git status` can help prevent many issues by ensuring that you remain informed about your changes.
Using `git log`
Another vital command for tracking down issues is `git log`, which displays the commit history, including authors, commit messages, and timestamps.
Code Snippet:
git log
You can customize the output for better clarity, for instance:
git log --oneline --graph --decorate
This provides a visual representation of commits, making it easier to identify where issues may have originated.
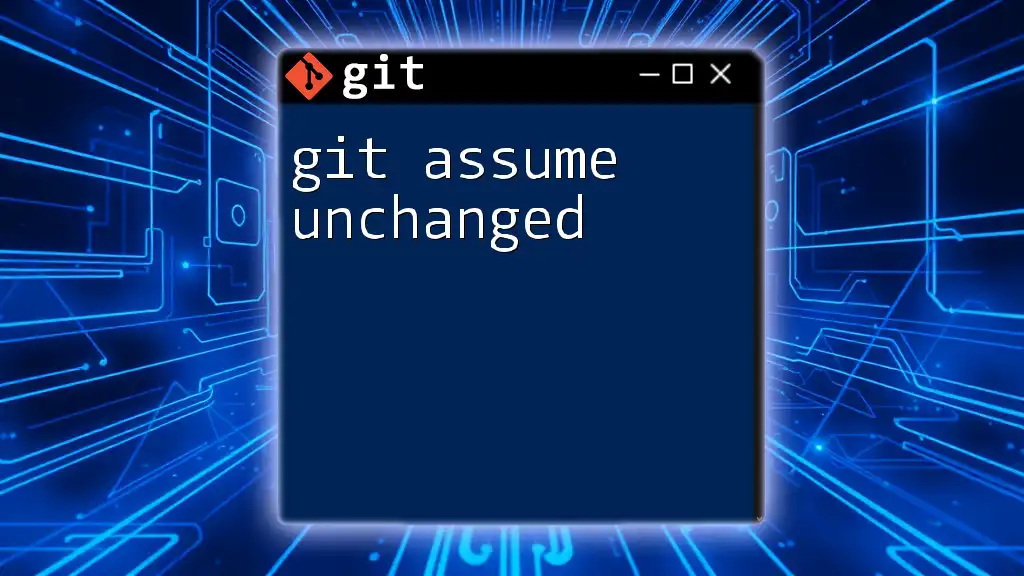
Best Practices for Managing Git Issues
Regular Commits
Making frequent commits is a fundamental practice that keeps your changes manageable and your project history clear. Meaningful commit messages that describe what has been altered can prevent confusion over changes made in previous commits.
Branching Strategies
Adopting a strategic branching model helps avoid git issues. Common strategies include:
- Feature Branching: Create a new branch for each new feature, and merge back into the main branch once the feature is complete and tested.
- Hotfix: Use a dedicated branch for urgent fixes directly on production.
These strategies minimize the risk of conflicts and keep your main branch stable.
Example: Suppose you’re developing a new feature on a separate branch, and by the time you merge it into the main branch, you discover several issues exist. Because you’ve isolated your changes, fixing them won't disrupt the stability of your main branch.
Documentation and Communication
Documenting issues and solutions, as well as maintaining open lines of communication within your team, ensures everyone is on the same page. Use GitHub Issues or a project management tool to maintain clarity about resolved problems and ongoing tasks.
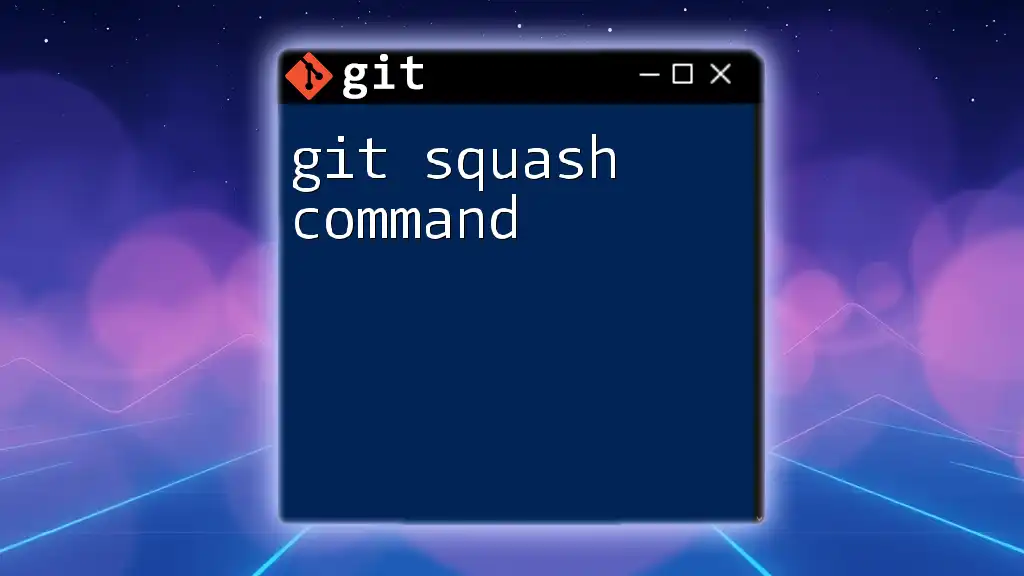
Tools and Resources for Issue Tracking
GitHub Issues
GitHub Issues is a built-in feature for tracking task progress, bugs, and feature requests. It allows users to create issue tickets that can be labeled, assigned, and linked to commits and pull requests.
To create a new issue:
- Navigate to the "Issues" tab in your GitHub repository.
- Click "New Issue," fill out the template with relevant details, and submit.
Alternatives to GitHub Issues
While GitHub Issues is popular, other platforms like JIRA, GitLab Issues, and Trello also provide issue tracking. Each comes with unique features; for instance, JIRA includes agile project management tools, while GitLab integrates issues directly within the CI/CD pipeline.
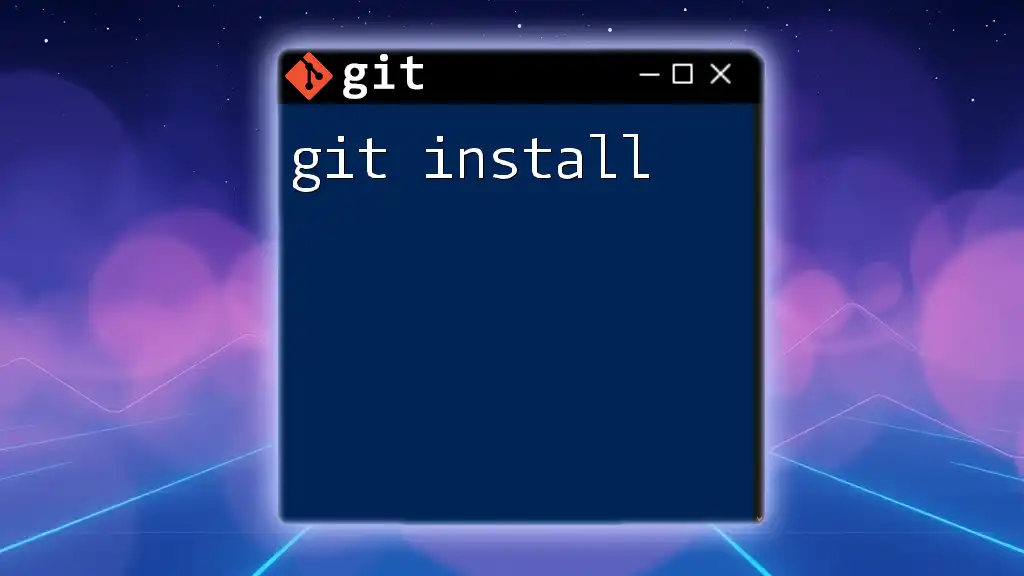
Final Thoughts
Importance of Issue Tracking in Development
Understanding and effectively managing git issues is paramount to successful collaboration and project completion in software development. By applying practices outlined in this guide, developers can resolve challenges swiftly and maintain the integrity of their work.
Call to Action
Dive into practicing Git commands today to gain confidence in handling issues! Explore resources such as tutorials and official documentation to further enhance your understanding and skillset in Git. Happy coding!