`git bisect` is a powerful debugging tool that helps you efficiently identify the commit that introduced a bug by performing a binary search through your project's history.
git bisect start # Start the bisect session
git bisect bad # Mark the current commit as bad
git bisect good <commit> # Mark a known good commit
# Test and then run one of the following based on the result
git bisect good # Mark the current commit as good
git bisect bad # Mark the current commit as bad
Overview of Git Bisect
Git bisect is a powerful command used to identify the commit that introduced a bug or issue in your code. It employs a binary search algorithm to efficiently narrow down the culprit, minimizing the number of tests needed to find the offending commit. This feature is especially useful in complex projects where the commit history can span numerous changes. Knowing when to employ `git bisect` will save you time and frustration when debugging.
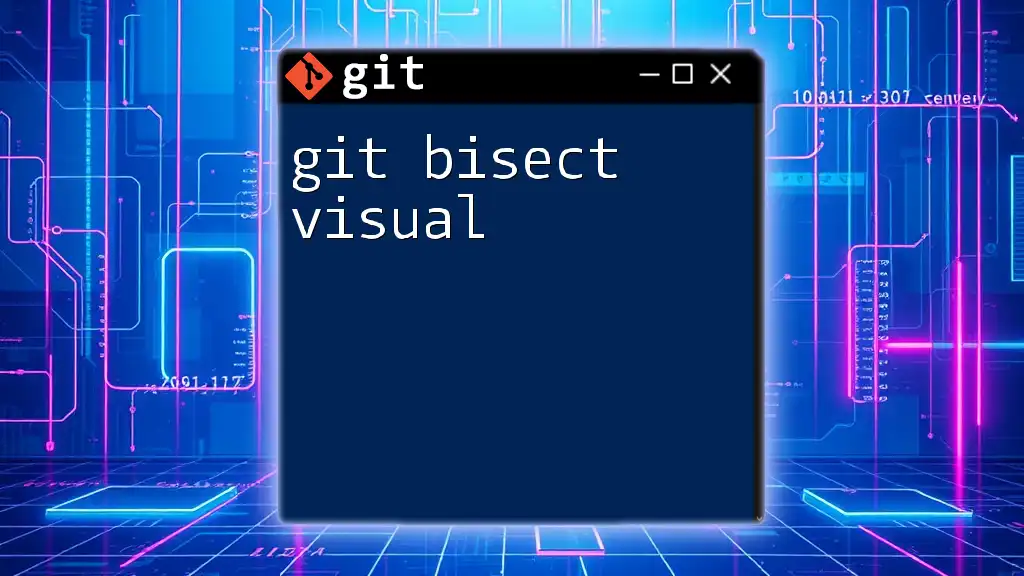
How Git Bisect Works
The bisect process works by taking advantage of the binary search algorithm. Instead of testing every single commit to locate a bug, Git divides the range of commits in half, asking you to determine whether the current commit is "good" (the feature functions correctly) or "bad" (the feature has the bug). This method dramatically reduces the number of iterations needed to find the bad commit.
Visualizing the commit history can help in understanding how `git bisect` works. Imagine a scenario where you have ten commits: testing each one sequentially would take too long. Instead, with each test, you eliminate approximately half of the commits, honing in on the bad commit in significantly fewer steps.
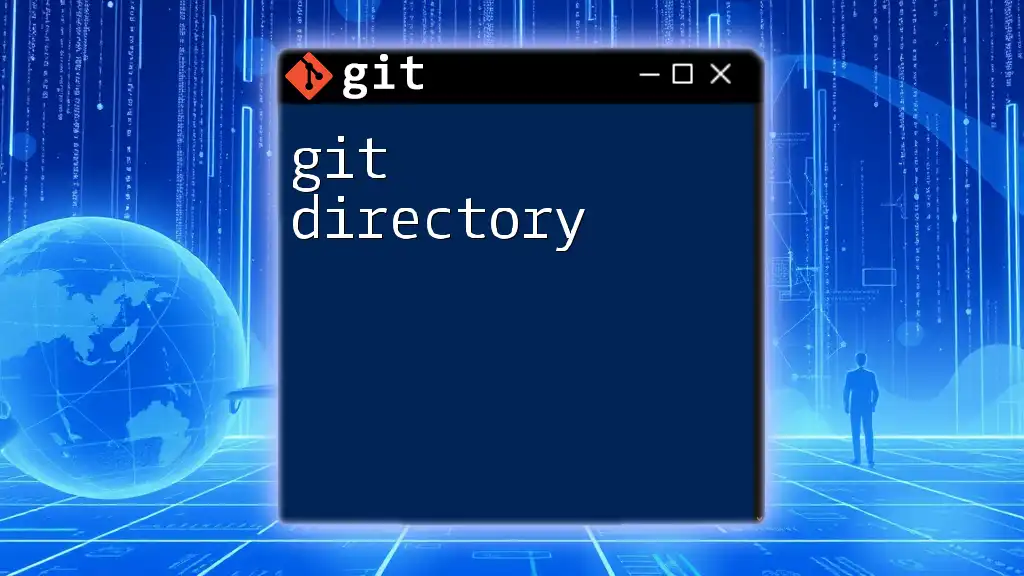
Setting Up for a Bisect
Before starting a bisect session, ensure that your repository is in the correct state. The following steps are essential:
Prerequisites for Using Git Bisect
Make sure to check the state of your repository. It’s crucial that you don't have any uncommitted changes in your working directory; a clean state allows `git bisect` to function correctly and ensures that you won't be testing code that hasn't been saved.
Marking Good and Bad Commits
To effectively utilize `git bisect`, you need to identify your starting points:
- Good Commit: This is a commit where the feature or code works correctly. You should ideally select the last known working commit.
- Bad Commit: This is the commit where the bug manifests itself. It could be the most recent commit if the bug was introduced after recent changes.
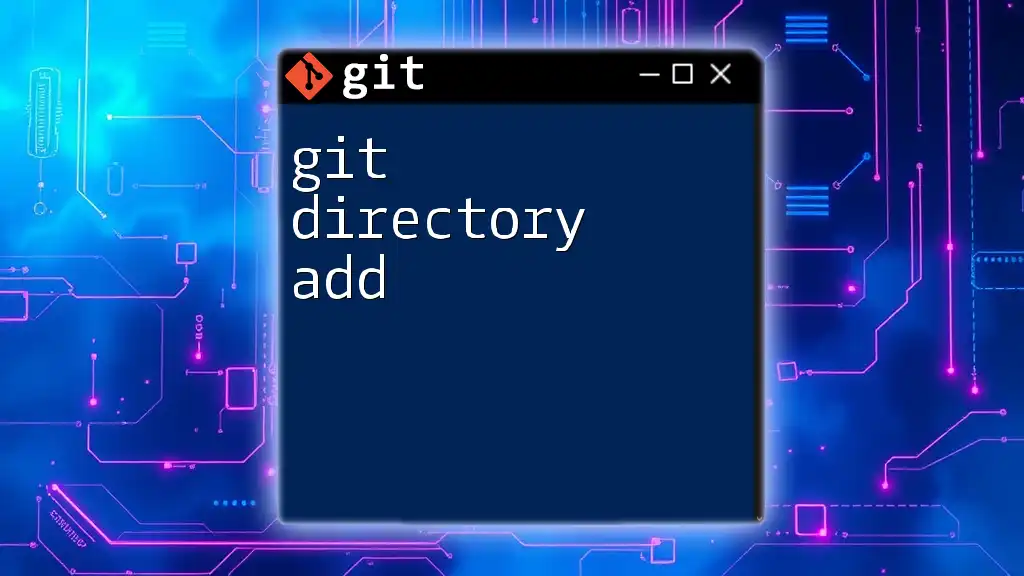
Executing Git Bisect
Now that you've prepared, it's time to start the bisect session.
Starting a Bisect Session
Begin by launching the session with the following command:
git bisect start
This command prepares Git to start tracking your forthcoming tests.
Marking Commits as Good or Bad
Tell Git which commit is bad and which is good.
To mark the bad commit, run:
git bisect bad
Next, to specify the good commit, execute:
git bisect good <commit-hash>
Replace `<commit-hash>` with the hash of the commit you verified as good.
Automating the Testing Process
If you have a way to run tests—perhaps through a specific script—you can automate the bisect process. Create a script that returns a status of success (0) or failure (1). For instance, a simple test script might look like this:
#!/bin/bash
# Replace the following command with your actual test
./run_tests.sh
Make sure the script is executable with:
chmod +x your_script.sh
Now you can utilize this script within the bisect session:
git bisect run ./your_script.sh
Git will then automatically mark each commit as good or bad depending on the script's output.
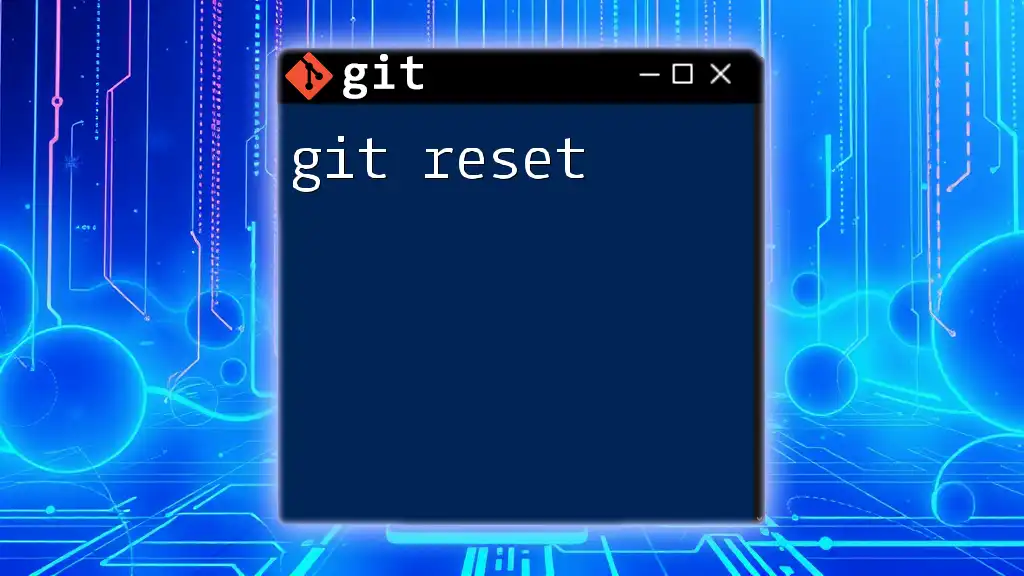
Analyzing the Results
After executing `git bisect`, you’ll start receiving output from your tests.
Understanding the Output of Git Bisect
Pay attention to the responses after each test execution. Git will narrow down the commits, showing you the path it’s taking towards identifying the bug. Each response from your testing script helps Git decide whether to move up or down the commit history, effectively cutting down the list of possible commits.
Finalizing the Bisect Process
Once you have identified the bad commit, it’s essential to reset the bisect session. Use the following command:
git bisect reset
This command brings you back to the original state before you began the bisecting process, ensuring your workspace remains organized.
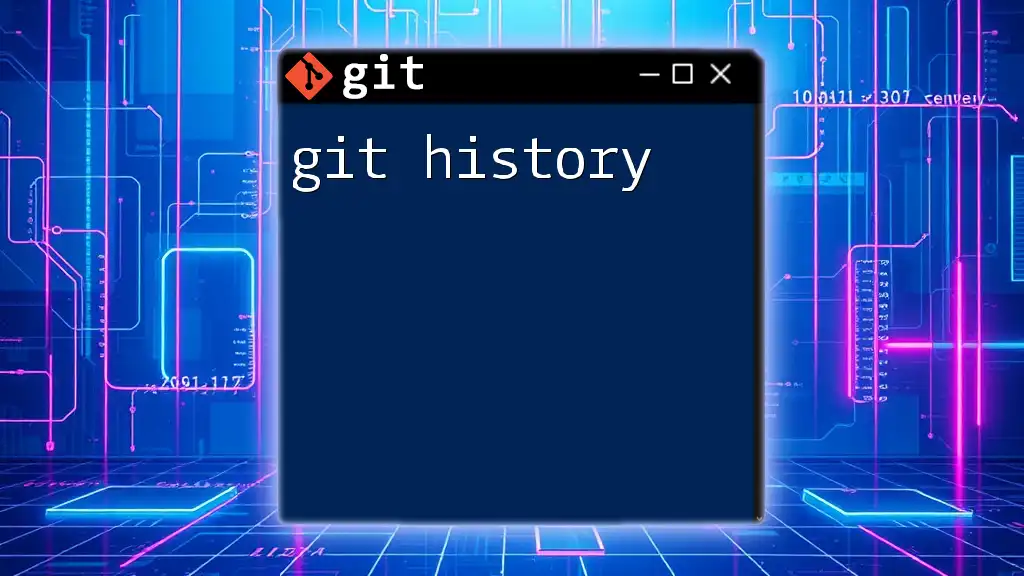
Real-Life Example of Git Bisect in Action
Imagine your team shipped a feature, but soon after, a bug appeared that caused a section of the application to crash. By utilizing git bisect, you can identify which commit introduced this bug systematically.
- Locate the last commit where the bug was absent (the good commit).
- Find the newest commit where the bug occurs (the bad commit).
- Execute the bisect commands as previously described.
With each test, Git narrows down the list. At the end of the process, it may reveal something like:
d8f7a0d is the first bad commit
Now you know the commit that introduced the bug!
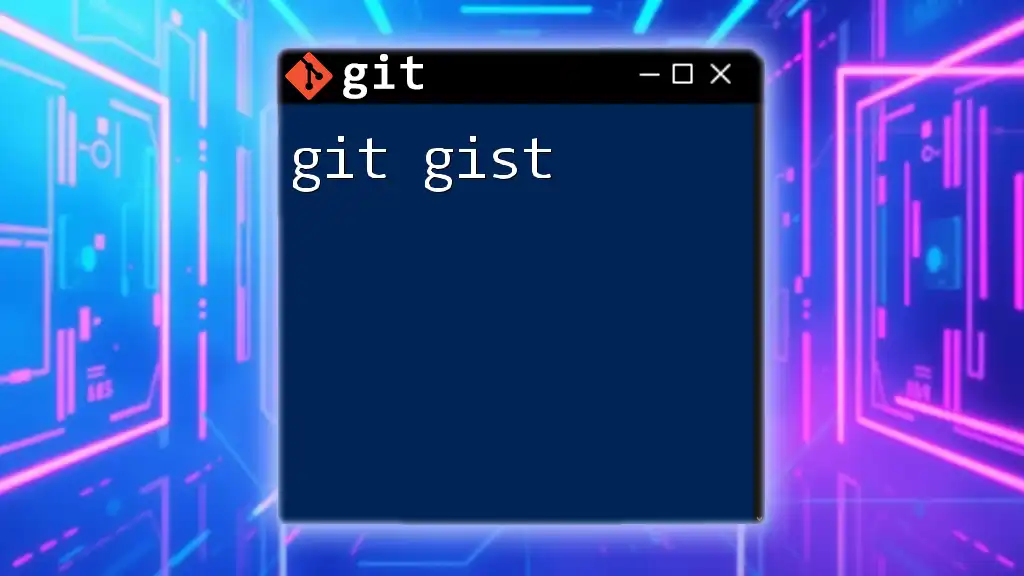
Advanced Usage of Git Bisect
Git bisect can handle more complexities than basic scenarios.
Using Git Bisect with Merge Commits
When working with merge commits, special considerations are necessary. Marking a merge commit as normal could lead to confusing outcomes since both parent commits might be good or bad. You may need to test the commits that were merged together individually.
Integrating Git Bisect with Continuous Integration (CI)
Employing git bisect within a CI process can be a game-changer. Automate testing by embedding the bisect logic in your CI/CD pipelines. This script can be triggered upon detecting a bug, saving time and effort while ensuring your commits remain stable.
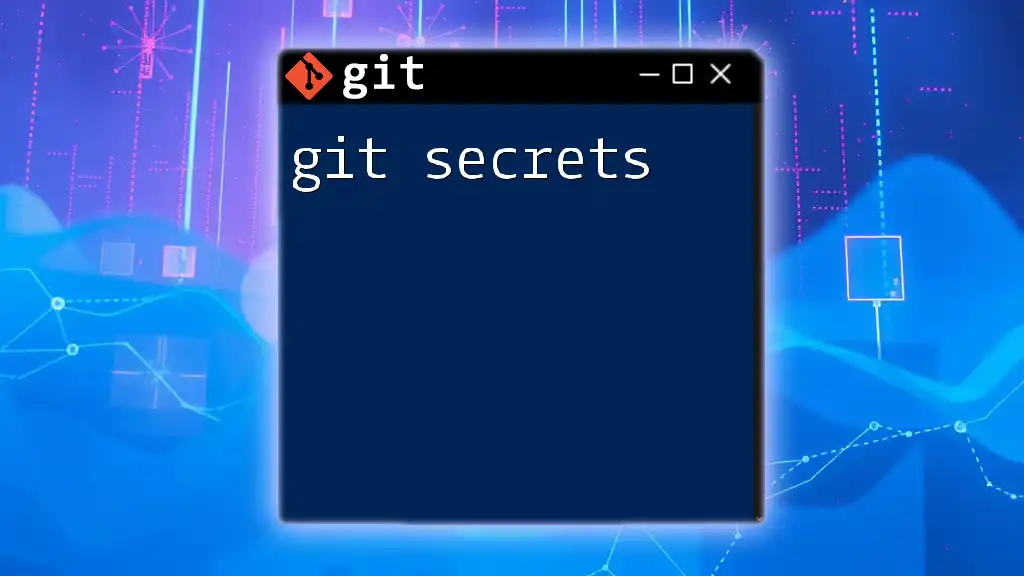
Common Pitfalls and Troubleshooting
Though `git bisect` is a valuable tool, several common mistakes can hinder its effectiveness.
Common Mistakes While Using Git Bisect
Omitting to mark a commit as good or bad can lead to inaccurate results. Always double-check your indications before proceeding.
Troubleshooting Bisect Sessions
When you encounter conflicts during testing, it might signify that two commits are intertwined. Ensure that you handle merges appropriately.
Tips for efficient testing include keeping your testing scripts simple and focusing on the essential parts that could lead to failure.
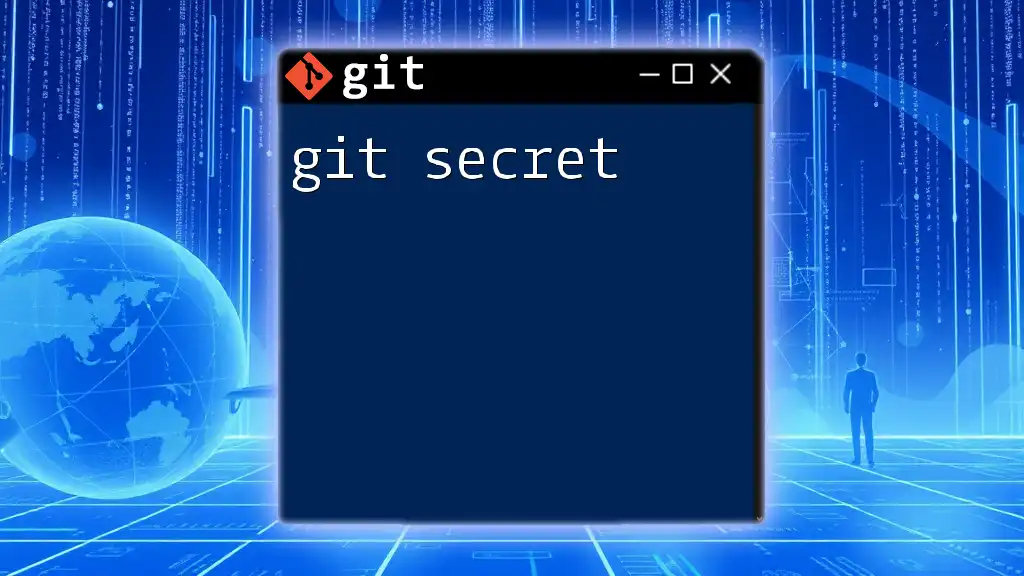
Conclusion
Git bisect is an indispensable tool in a developer's toolbox for quickly locating problematic commits. Its ability to efficiently narrow down potential issues can save hours of frustration.
To truly master `git bisect`, practice is essential. Using it on real projects will deepen your understanding and prepare you for any debugging challenges you may face.
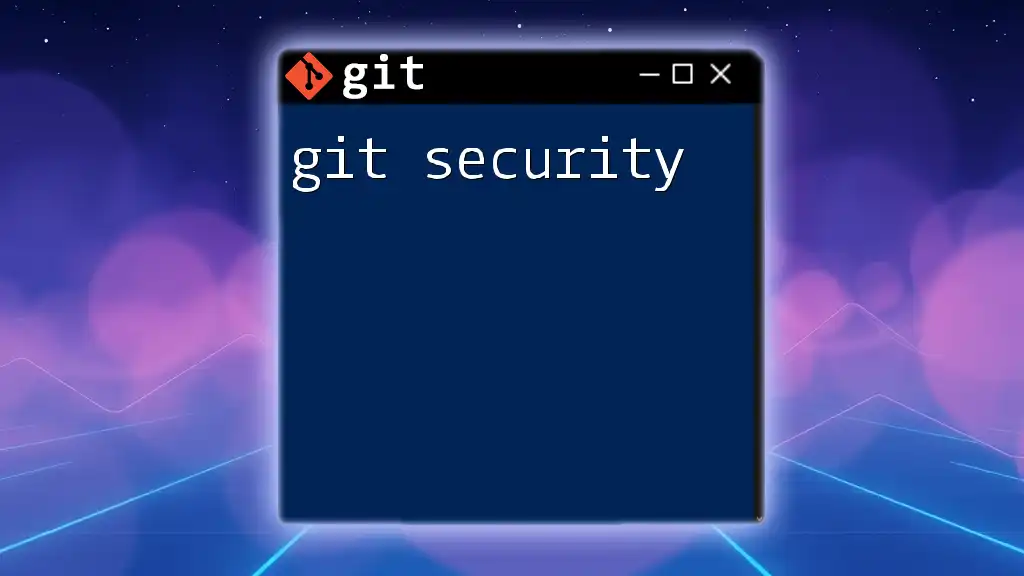
Additional Resources
For further learning, refer to the official Git documentation and consider creating your own Git bisect cheat sheet to enhance your productivity! Embrace the power of `git bisect` in your workflow, and watch your debugging process improve significantly.