`git bisect visual` is a command that helps you visually track and fix bugs by performing a binary search through your project's commit history to isolate the commit that introduced the problem.
Here's a code snippet to illustrate how to use `git bisect`:
# Start the bisect session, specify known good and bad commits
git bisect start
git bisect bad # mark the current commit as bad
git bisect good <commit_hash> # mark a known good commit
# Git will now checkout a commit for you to test
# After testing the commit, run:
git bisect good # if the commit is good
git bisect bad # if the commit is bad
# Repeat, and when finished:
git bisect reset # to end the bisect session
What is Git Bisect?
Git bisect is a powerful command that helps developers find the specific commit that introduced a bug or caused a regression in their codebase. By employing a binary search strategy, Git bisect allows users to efficiently narrow down the range of commits that could contain the issue. Instead of sifting through numerous commits manually, developers can quickly ascertain the point at which their code began to misbehave.
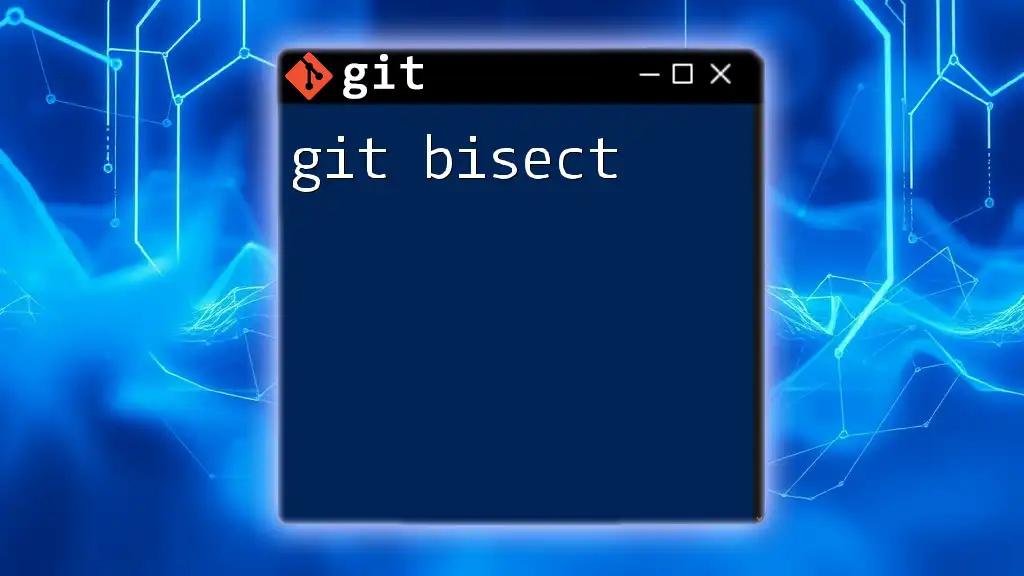
Importance of Visualizing Git Bisect
Visualizing the bisect process is crucial for several reasons. First, it enhances comprehension by allowing users to see the commit history graphically, helping identify patterns and relationships. Second, it aids in debugging by providing a clear view of which commits are functioning correctly and which ones are not. Various tools exist that can help in this visualization, transforming the often linear output of Git commands into more digestible graphs or diagrams.
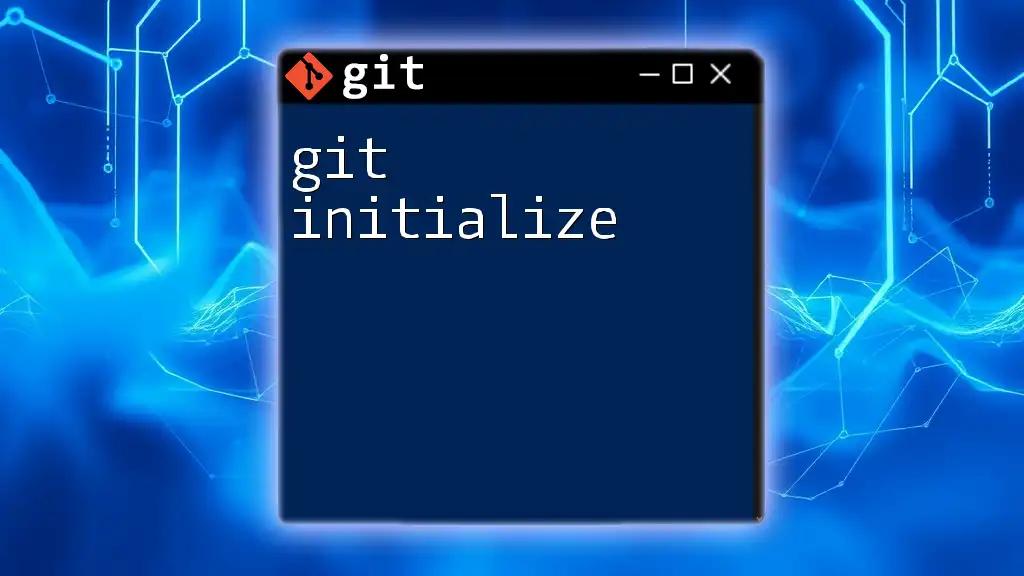
Understanding Git Bisect
Why Use Git Bisect?
Imagine a situation where a seamless feature is suddenly broken in your code. Instead of combing through every single commit made since the last known good version, you can leverage Git bisect to isolate the problem efficiently. This approach saves time and effort, making it invaluable for development teams under tight deadlines.
How Bisect Works
Git bisect operates by marking two commits: one that is "bad" (where the issue occurs) and another that is "good" (where everything works as expected). By keeping these two points in mind, Git can isolate the culprit commit in logarithmic time.
Basic Git Bisect Commands
Here are the essential commands to initiate the bisect process:
-
Starting Bisect: To begin a bisect session, you’ll first need to run:
git bisect start
-
Marking Bad Commits: To signify the problematic commit, use:
git bisect bad HEAD
-
Marking Good Commits: Define a known good commit with:
git bisect good v1.0
Example: Basic Workflow
When starting a bisect session, your commands will look like this:
git bisect start
git bisect bad HEAD
git bisect good v1.0
This command sequence informs Git about your starting points and kicks off the bisecting process.
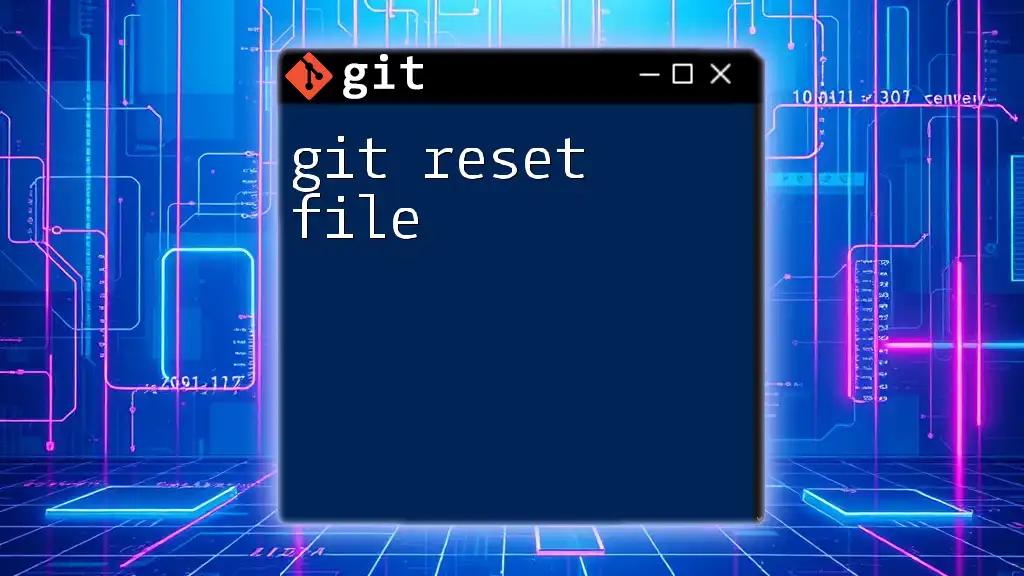
Visualizing Git Bisect
Using Git UI Tools
Many graphical user interfaces (GUIs) provide straightforward visualizations of your repositories. Software like GitKraken and SourceTree enhances usability by transforming command-line history into stunning graphs. Utilizing GUIs allows developers to visually track their commits and easily define "good" and "bad" commits without wrestling with command-line syntax.
Using Terminal Visualizers
For those who prefer the terminal, commands like `git log --graph` can effectively visualize commit history. This command generates a visual representation of commit branches and merges, making it easier to identify where things went wrong.
Drawing the Commit Graph
Creating a mental or drawn map of your commit graph during a bisect process can help you visualize the search process better. You can see how many steps it takes to converge on the problematic commit, providing insight into your repository's structure and commit history.
Understanding the Output of Git Bisect
As you go through each commit, Git will provide output that tells you whether each commit is "good" or "bad." Familiarizing yourself with this output can enhance your understanding of the process and the commitment involved.
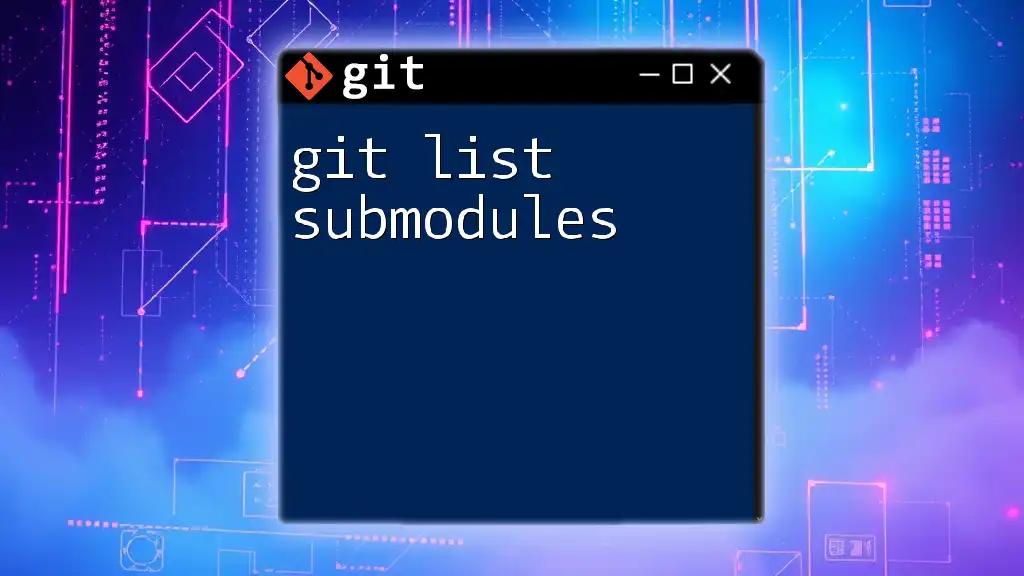
Step-by-Step Git Bisect Process
Preparing Your Environment
Setting Up a Demo Repository
Before diving into the bisecting, creating a sample repository is essential for practice. A demo repo with a clear history of good and bad commits will provide a safe space for experimentation without risking your main project.
Running Git Bisect Visually
When conducting a visual bisect, you narrow down your search through commits, marking them as either "good" or "bad." Both the scenarios can unfold as follows:
git bisect good commit_1
git bisect bad commit_2
Each time you run a test, Git will check out the next commit based on your prior marks.
Using Scripts to Automate Testing
Automating your tests within the bisect context can greatly improve efficiency. You can create a simple test script that Git will run to evaluate the current commit's status. For instance:
#!/bin/bash
make test # Run your tests here
By executing `git bisect run ./test-script.sh`, Git automatically tests each commit until it finds the one that broke your code.
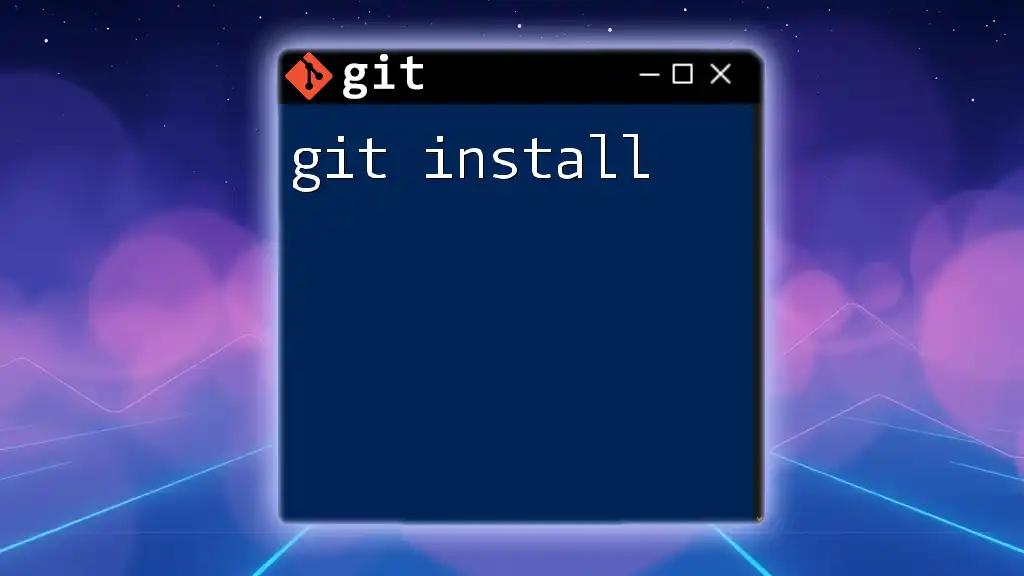
Interpreting Results
Finding the Culprit Commit
Once you've narrowed down the search, Git will report back with the exact commit that introduced the bug. This output can often look like:
found: <commit_hash>
This statement indicates the offending commit, allowing you to delve into its changes for further examination.
Resetting Your Bisect Session
After identifying the problem, you'll want to exit the bisect session and return to your original branch. This can be easily accomplished with:
git bisect reset
This command allows you to clean up after your debug session and continue your work unencumbered.
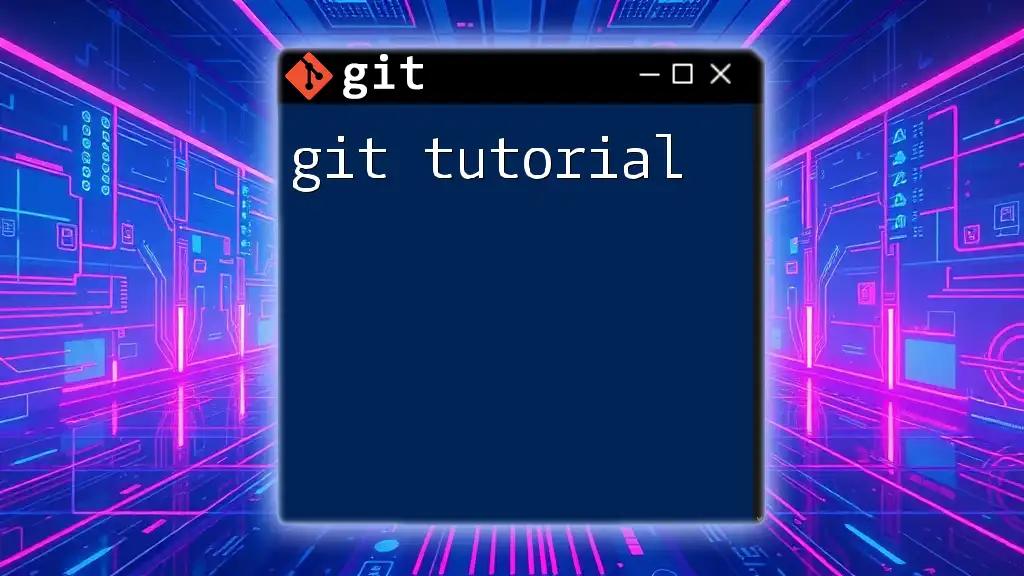
Case Studies and Examples
Example: Debugging a Feature Breakage
Suppose your feature that previously filled a shopping cart is now broken due to recent commits. By employing Git bisect, you can quickly determine the last known good commit. After marking your good and bad commits, you would navigate through a series of tests that lead you to the specific commit responsible for this disruption. This method is not only efficient but also provides a definitive answer to complex questions regarding code functionality.
Common Pitfalls and Troubleshooting
Tips for Using Git Bisect
Using Git bisect effectively comes with its own set of guidelines. Ensure that your commits are well-commented and that you maintain stable versions to clarify the "good" states in your code.
Troubleshooting Tips
During your bisect journey, you might encounter challenges, such as conflicting merges or a non-linear commit history. Familiarizing yourself with resolving merge conflicts and understanding how Git logs branches can facilitate a smoother bisect experience.
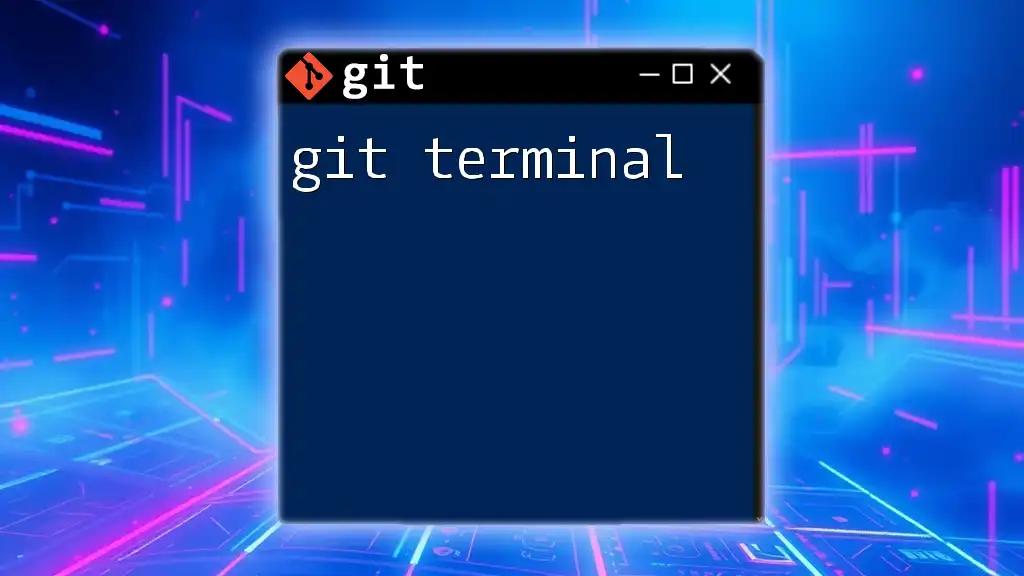
Conclusion
In summary, Git bisect is an essential command for pinpointing troublesome commits, and visualizing the process further enhances its efficiency and comprehensibility. By understanding how to leverage both command-line and visual tools, developers can streamline their debugging processes.
Integrating good practices surrounding Git bisect into your workflow can ultimately improve your coding and debugging skills. The more you use Git bisect, the more intuitive it will become, providing you with a robust defense against complex software issues.
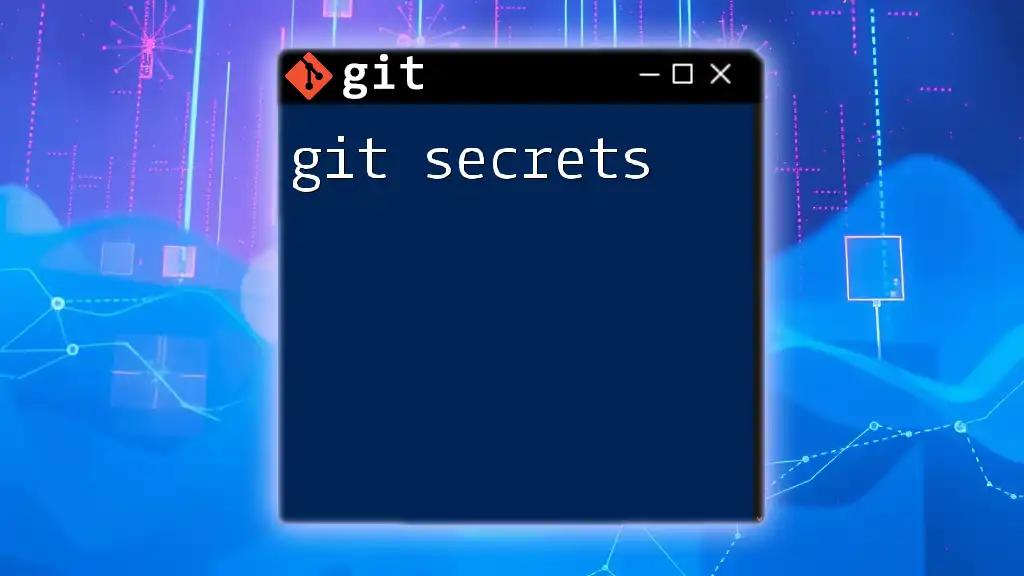
Call to Action
If you're looking to deepen your understanding of Git commands, consider enrolling in one of our specialized training courses. Join us in mastering Git and version control to take your development capabilities to the next level! Don't forget to subscribe for more insights on Git best practices and advanced techniques!