"Git for Visual Studio Code allows developers to manage their repositories efficiently using integrated version control features directly within the editor."
Here's a simple command to clone a repository in Git:
git clone https://github.com/username/repository.git
Setting Up Git in Visual Studio Code
Installing Git
To start using Git with Visual Studio Code, you first need to install Git on your machine. Git is available for various operating systems. Here’s how to install it depending on your environment:
-
Windows: Download the Git installer from the [official Git website](https://git-scm.com/). Run the installer and follow the prompts. Make sure to choose the option to add Git to your system PATH, which will allow you to access Git commands from the command prompt or within Visual Studio Code.
-
macOS: You can install Git using Homebrew, a popular package manager for macOS. Open your terminal and run:
brew install git
Alternatively, you can install Git by downloading Xcode Command Line Tools. Just type:
git --version
in your terminal, and it will prompt you to install the tools if you don't have them.
-
Linux: Depending on your distribution, you can install Git using your package manager. For Ubuntu, for example, you can run:
sudo apt update sudo apt install git
Configuring Git in Visual Studio Code
Once Git is installed, it’s essential to configure it before you start using it. This configuration is crucial for ensuring that your commits and contributions are accurately tracked.
-
Setting Global Username and Email: Open your terminal and run the following commands to set your global username and email. This information will show in your commit history.
git config --global user.name "Your Name" git config --global user.email "you@example.com"
-
Verify Your Configuration: To check if your configuration is correct, run:
git config --list
This command will list your current Git configuration, allowing you to confirm that your settings are correctly applied.
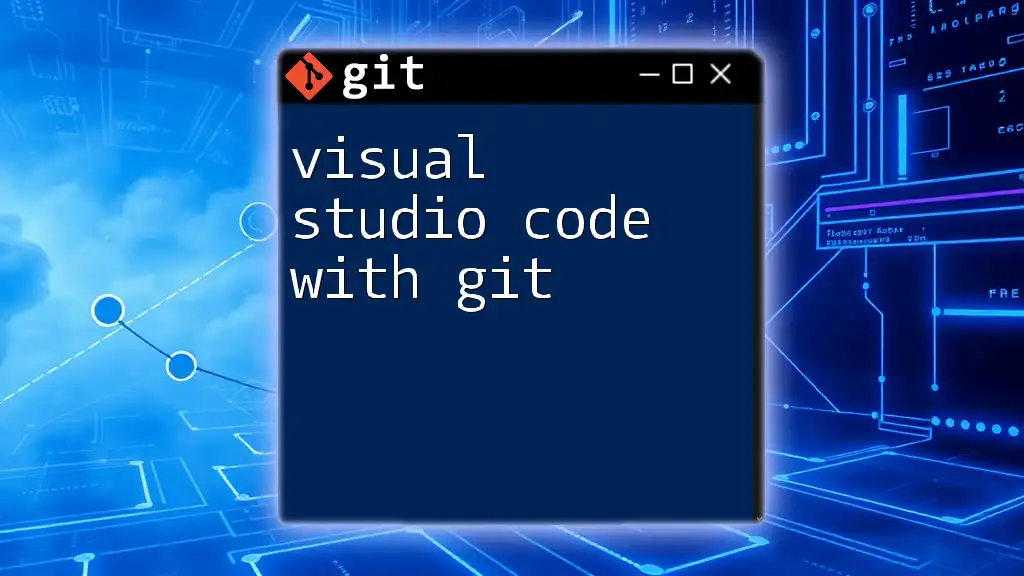
Integrating Git with Visual Studio Code
Enabling the Git Extension
Visual Studio Code comes with built-in Git integration, which simplifies daily workflows. To access the Source Control panel, click on the Source Control icon in the Activity Bar on the left side of the window. This panel lets you view changed files, stage changes, and commit.
The Source Control panel provides key features such as:
- Viewing diffs for modified files.
- Staging individual files or changes within files.
- Managing branches and remote repositories.
Working with Repositories
Cloning a Repository
Cloning a repository allows you to create a local copy on your machine. From the Source Control panel, you can easily clone a repo from platforms like GitHub.
For example, to clone a repository, use the terminal within VS Code:
git clone https://github.com/username/repository.git
After cloning, you can open the repository by navigating to its folder in Visual Studio Code.
Creating a New Repository
To start a new project, you can create a new Git repository directly from Visual Studio Code. Here’s how:
- Open the Command Palette (press `Ctrl + Shift + P` or `Cmd + Shift + P` on macOS).
- Type and select "Git: Initialize Repository".
- Choose an empty folder or create a new one. Now, you have initialized a new Git repository.
The command line alternative is:
git init
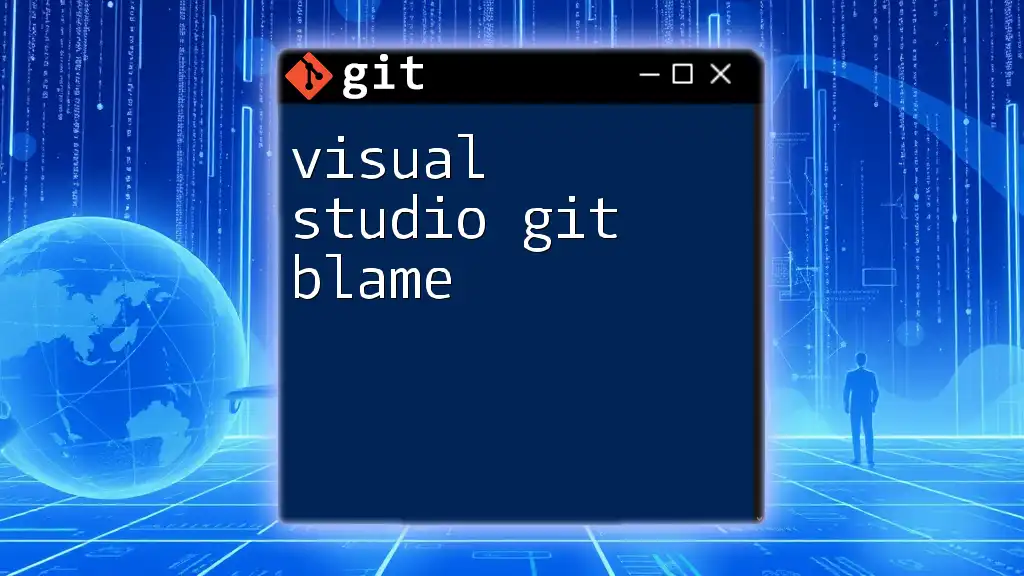
Basic Git Commands within Visual Studio Code
Staging Changes
Staging is a crucial part of the Git workflow; it allows you to prepare changes before committing them. In the Source Control panel, you can see the list of changed files. Hover over a file and click the plus (+) icon to stage it. Alternatively, to stage all changes using the terminal:
git add .
This command stages all modified and new files.
Committing Changes
Once changes are staged, it's time to commit them. A commit represents a snapshot of your project at a specific point in time, making meaningful commit messages important for your project’s history.
In Visual Studio Code, after staging your changes, type your commit message in the input box located above the staged changes. You can commit by clicking the checkmark icon.
Using the terminal, you can commit with:
git commit -m "Your commit message"
Pushing Changes to a Remote Repository
Pushing is how you transfer your commits from your local repository to a remote one. This is essential for collaboration.
To push your changes, ensure you've set the remote repository. You can push changes using the Source Control panel or the terminal:
git push origin main
Replace `main` with your branch name if necessary.
Pulling Changes from a Remote Repository
Pulling is essential for keeping your local repository up-to-date with the remote version. Within Visual Studio Code, you can pull changes from the Source Control panel effortlessly.
To pull via the terminal:
git pull origin main
This command fetches the changes from the remote repository and merges them into your local branch.
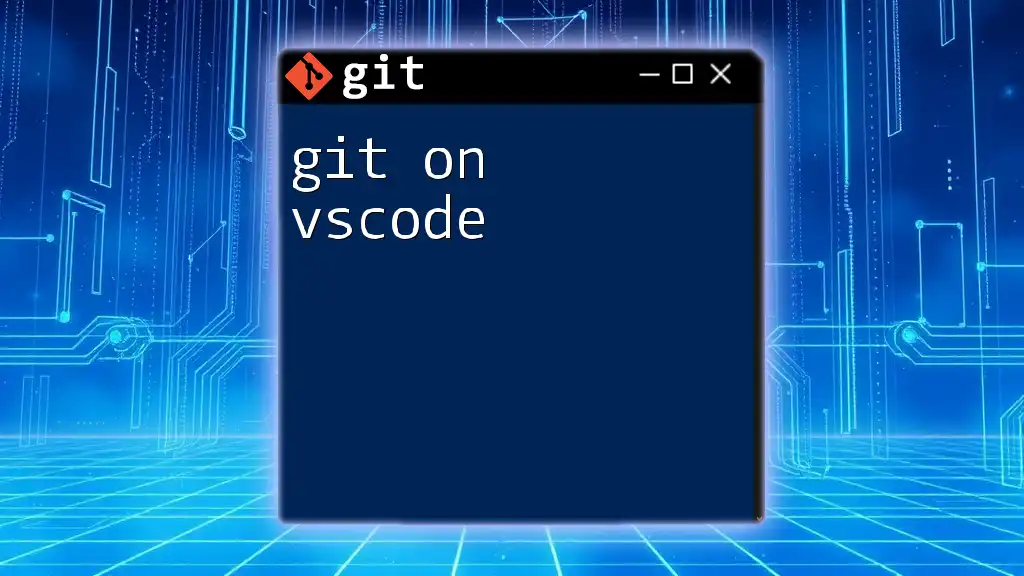
Branching and Merging in Visual Studio Code
Understanding Branches
Branches in Git allow you to work on features or fixes in isolation. This means that you can develop new features without affecting the stable version of your codebase.
Creating and Switching Branches
To create a new branch:
- Open the Command Palette and type "Git: Create Branch".
- Enter the name of your new branch.
You can also create and switch to a new branch in one command using:
git checkout -b new-feature
Merging Branches
Once your feature is complete, it's time to merge it back into your main branch. Switch to the branch you want to merge into (e.g., `main`):
git checkout main
Then merge your changes with:
git merge new-feature
If there are conflicts, Visual Studio Code will visually indicate them, allowing for conflict resolution directly in the editor.
Deleting Branches
After merging, you can clean up by deleting the old branch. You can delete a branch both through the UI and the terminal:
git branch -d branch_name
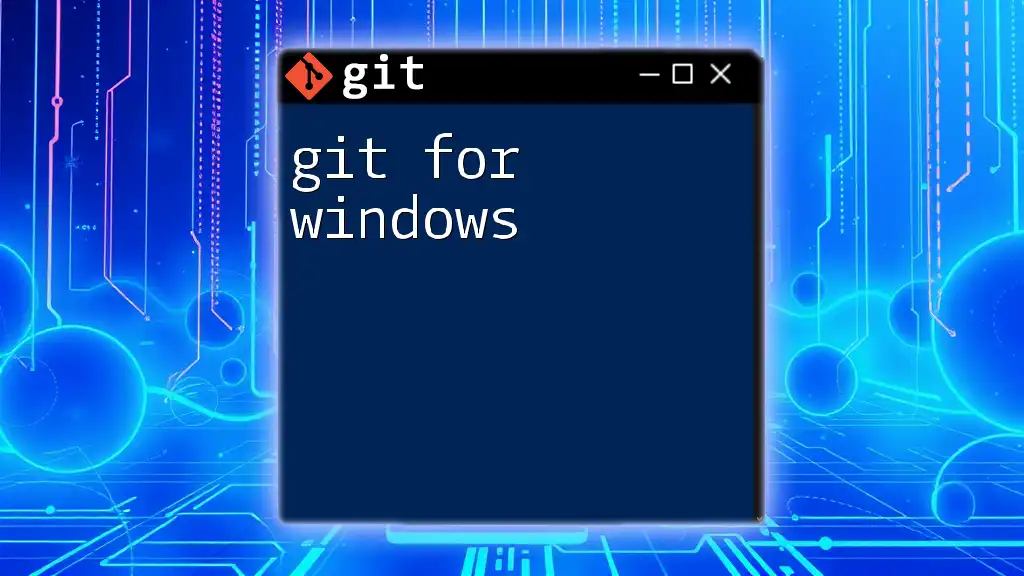
Resolving Git Conflicts
Understanding Merge Conflicts
Merge conflicts occur when two branches have changes in the same place. It’s crucial to resolve them manually to ensure code integrity.
Resolving Conflicts using Visual Studio Code
Visual Studio Code makes it easy to manage merge conflicts:
- When conflicts arise, the affected files will be highlighted. Open these files to see conflicting sections clearly marked.
- Choose "Accept Incoming Change", "Accept Current Change," or "Accept Both Changes" based on your needs.
Finalize the process by staging the resolved files and committing the changes.
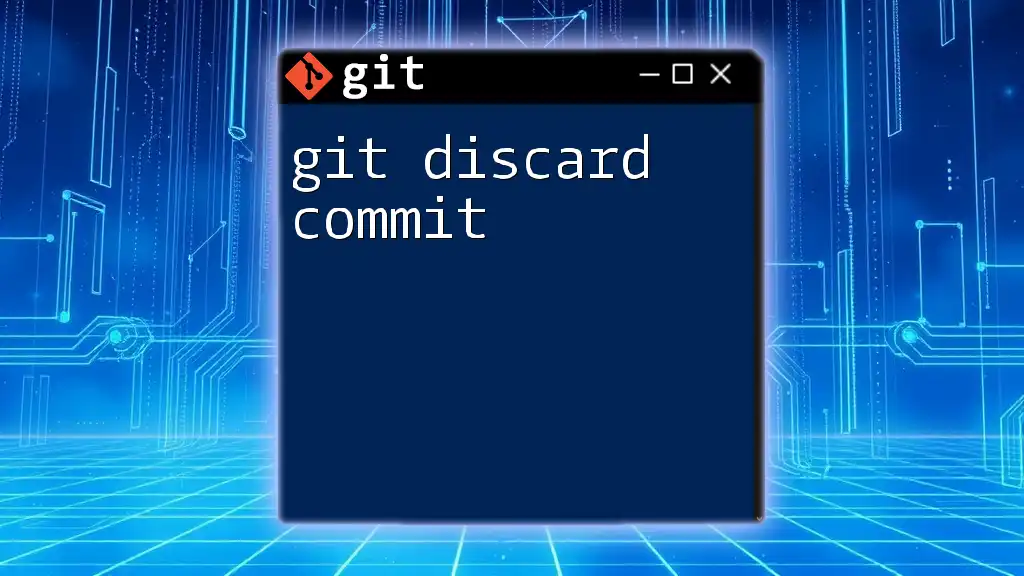
Advanced Git Features in Visual Studio Code
Using Git Graph Extension
To visualize your commit history, consider installing the Git Graph extension. This powerful tool provides a graphical representation of branches, merges, and commits, helping you navigate complex commit histories more intuitively.
Rebasing and Cherry-Picking
For more advanced workflows, understand rebasing and cherry-picking. Rebasing allows you to move your entire branch to a new base commit, maintaining a clean project history. You can initiate a rebase with:
git rebase main
Cherry-picking enables you to apply individual commits from one branch to another, providing more control over your changes.
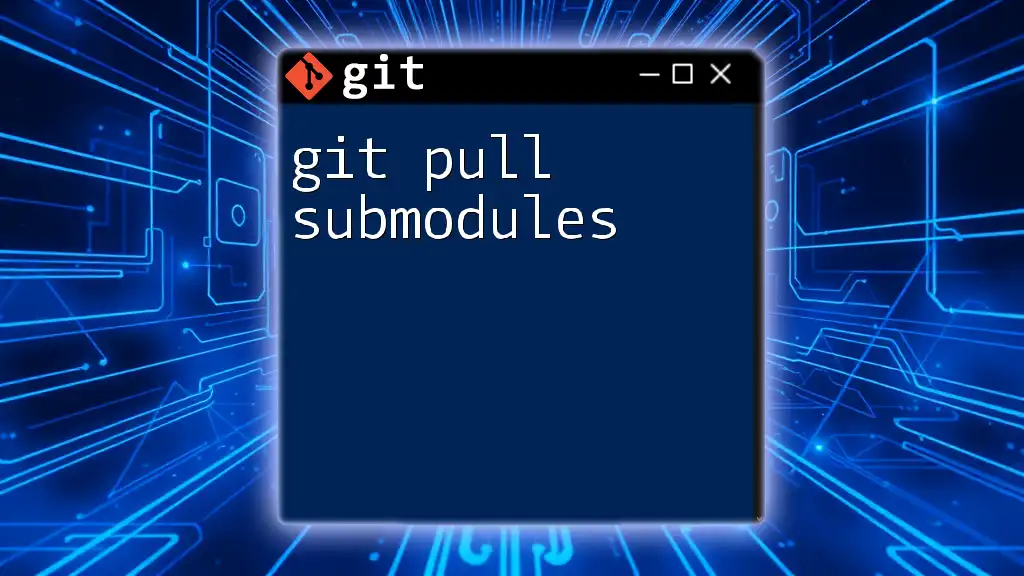
Best Practices for Using Git in Visual Studio Code
To maximize your efficiency with Git in Visual Studio Code, remember these best practices:
- Commit often: Frequent commits make it easier to manage changes.
- Write meaningful commit messages: They provide context for future developers (or yourself).
- Use branching effectively: Keep features and fixes separate to streamline your workflow.
- Regularly pull changes: This helps in minimizing conflicts and keeping your local repository up-to-date.
For further learning, refer to the [official Git documentation](https://git-scm.com/doc) and participate in community forums to learn tips and tricks from other developers.
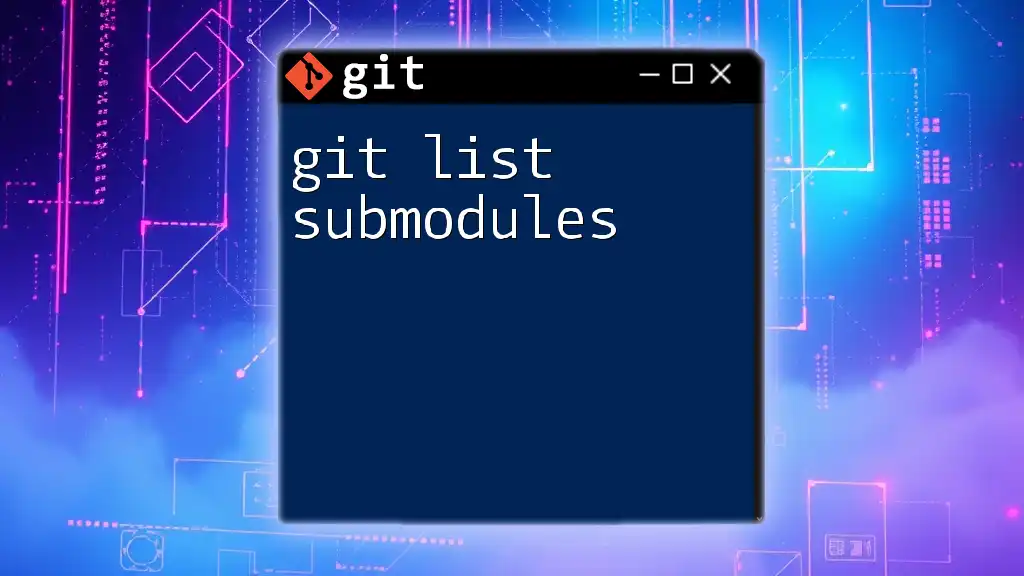
Conclusion
Using Git effectively with Visual Studio Code streamlines your development process. With the steps outlined in this guide, you can easily navigate version control and collaborate efficiently with your team. Embrace these practices to enhance your productivity and safeguard your projects.
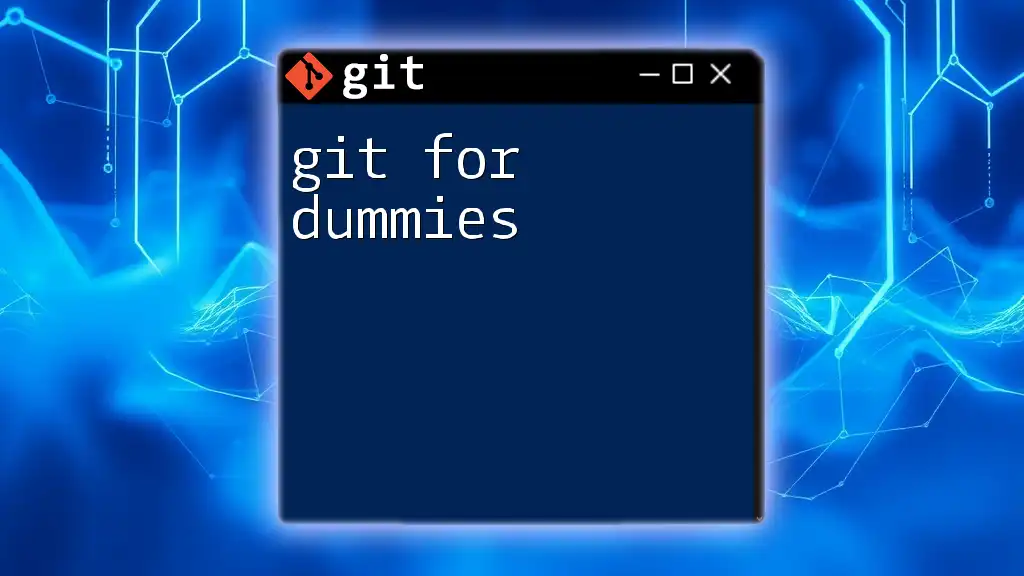
Call to Action
Have you started using Git in Visual Studio Code? Share your experiences or ask any questions you might have in the comments section below! Don’t forget to follow our company for more tutorials, guides, and tips on mastering Git and Visual Studio Code.