To discard a commit in Git while preserving your changes in the working directory, use the `git reset` command with the `--soft` or `--mixed` option followed by the commit identifier or `HEAD~1` to refer to the last commit.
git reset --soft HEAD~1
Understanding Commits
What is a Commit?
In Git, a commit serves as a snapshot of your project's history. Each commit is essentially a saved state of your files at a specific point in time. When you commit changes, you not only store those changes but also write a commit message that explains what was changed. This context is crucial, especially when collaborating with others or retracing your steps later.
Examples of commit messages can range from simple descriptions like "fix typo in README" to more elaborate explanations detailing the impact of changes.
Scenarios for Discarding a Commit
There are several scenarios where you might find the need to discard a commit:
- You accidentally committed changes and need to remove them.
- Sensitive information, like passwords or API keys, was included in a commit.
- You realize that the logic or code in the commit is fundamentally incorrect.
Understanding the context for needing to discard a commit is important because it influences the method you choose to roll back those changes.
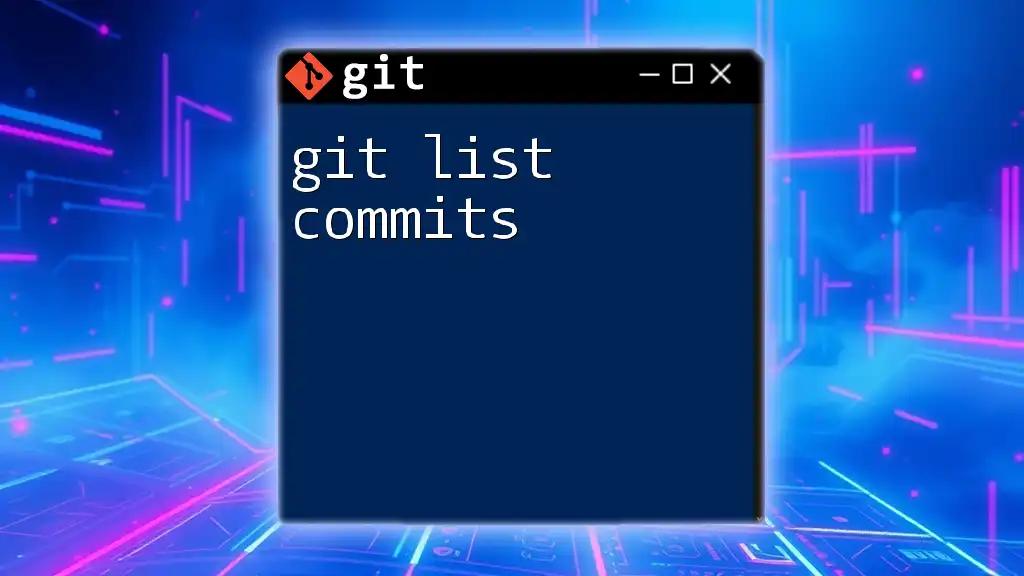
Discarding the Last Commit
Resetting the Last Commit
One of the most straightforward ways to discard your last commit is by using the `git reset` command. This command allows you to undo commits and can alter the state of your working directory depending on the option you choose.
-
`--soft`: This option undoes the last commit but keeps your changes staged. It's useful if you realize you need to make further modifications to your changes before recommitting.
git reset --soft HEAD~1
-
`--mixed`: This is the default option if you don't specify one. It undoes the last commit and unstages your changes, keeping them in your working directory. This is the best choice if you want to edit the changes further before committing again.
git reset --mixed HEAD~1
-
`--hard`: Caution is advised here. This option discards all changes permanently—both the commit and the associated changes in your working directory. Use this when you are sure you won’t need the changes again.
git reset --hard HEAD~1
Making the right choice among these methods is key; consider the consequences of using `--hard`, as it will erase your changes forever.
Reverting the Last Commit
If you wish to discard a commit but keep a record of your history, `git revert` is your best option. It creates a new commit that reverses the changes made by the commit you choose to discard. This method is non-destructive and maintains a clear history.
Use the following command to revert the last commit:
git revert HEAD
This command will prompt you to edit the default commit message for the new commit that undoes your last changes. Using `git revert` is particularly beneficial in collaborative environments where you want to preserve a clear record of what occurred.
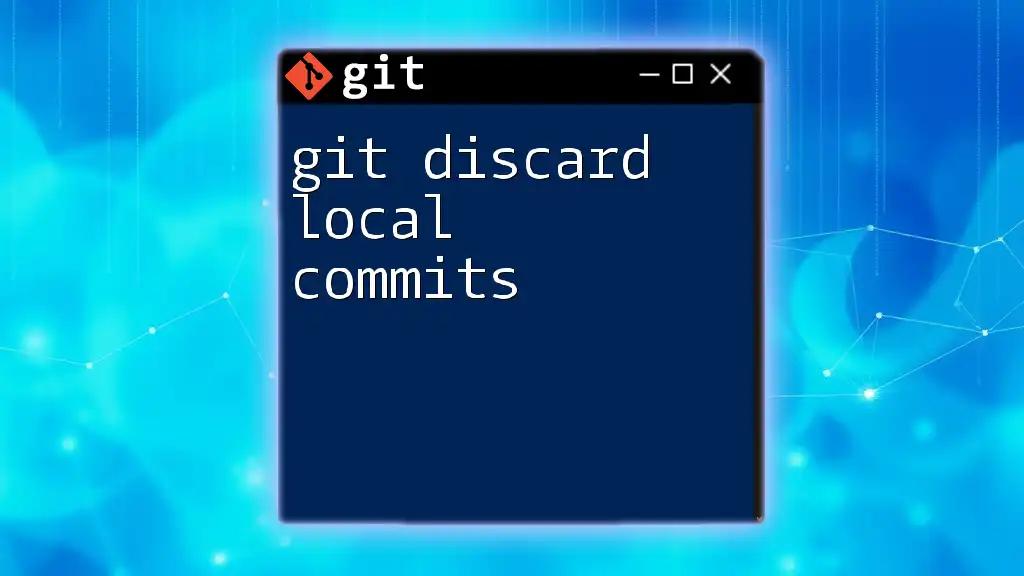
Discarding Specific Commits
Using Git Rebase
When you want to discard a specific commit (not just the last one), `git rebase` is an excellent choice. This command allows you to reorder commits and discard selected ones during the process.
Start by using the following command to initiate an interactive rebase:
git rebase -i HEAD~n # Replace n with the number of commits to consider
Your text editor will open a list of the last `n` commits, where you can choose to remove a commit by deleting its line or changing the word `pick` to `drop`. After saving the file, Git will proceed to reapply the remaining commits on top of the previous state. Be prepared to resolve any conflicts that may arise during this process.
Cherry Picking and Reverting Specific Commits
In some cases, you may want to include a specific commit while removing another. The `git cherry-pick` command allows you to apply the changes from a specific commit to your current branch.
To include a commit while also removing an unwanted one, you can use:
git cherry-pick -n <commit_hash>
This applies the changes from the specified commit but does not create a new commit automatically, allowing you to modify the changes before finalizing them.
If you want to remove a specific commit altogether, you can do so with:
git revert <commit_hash>
This will safely remove the changes made in that commit while keeping a history of it.
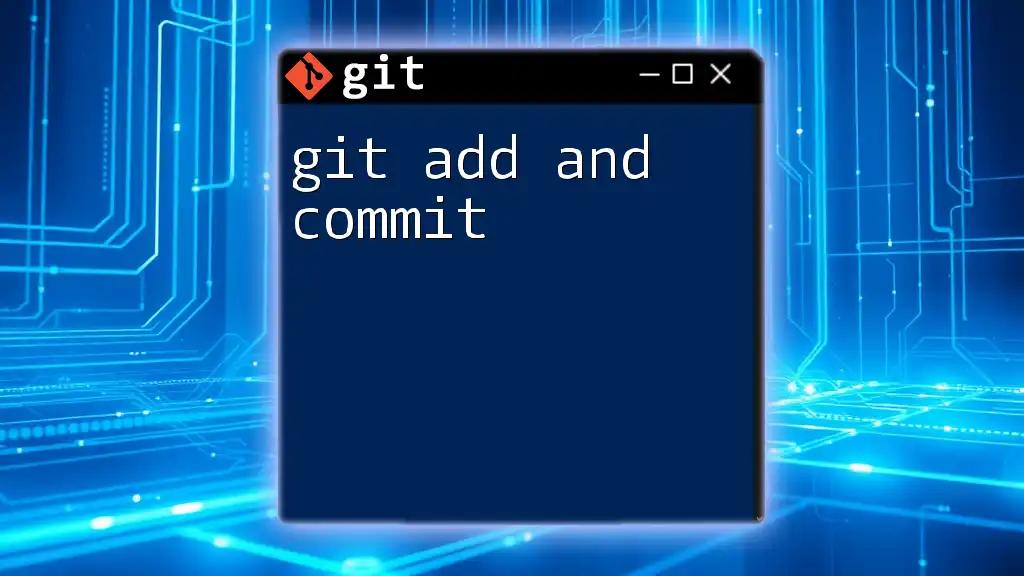
Recovering Discarded Commits
Finding Lost Commits
If you’ve accidentally lost a commit (especially after a `git reset --hard`), you can often find it using `git reflog`. This command logs all actions related to your Git repository, even those that affect the commit history.
git reflog
By running this command, you'll see a list of reference logs with commit hashes corresponding to actions you've taken, from commits to merges.
Restoring a Lost Commit
To restore a discarded commit, first identify the hash of the lost commit from the reflog output. Then, you can use `git checkout` to create a new branch starting from that commit or even reset the current branch to that specific state:
git checkout <commit_hash>
This command allows you to see your repository as it existed at that commit, providing you an opportunity to recover any lost work.
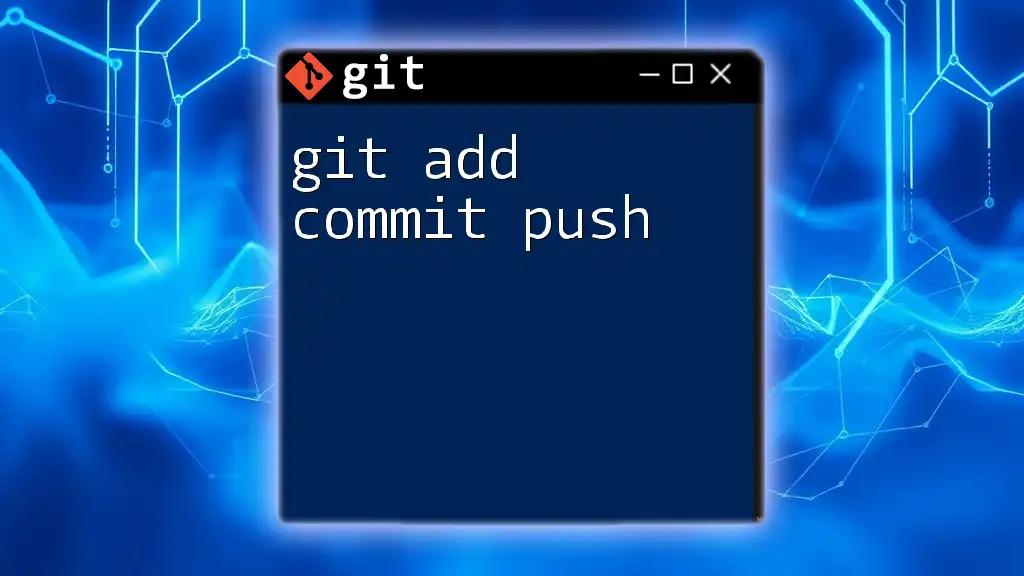
Conclusion
Understanding how to git discard commit operations are vital to effectively manage your projects. Whether you need to remove accidental commits, revert specific changes, or recover lost work, having a firm grasp of these commands helps maintain the integrity of your project while providing flexibility in handling changes.
Practicing these commands in a safe environment, like a sandbox repository, allows you to become familiar with their effects without risking important work.

Additional Resources
For further learning, refer to the [official Git documentation](https://git-scm.com/doc) and explore recommended readings on version control best practices. Utilizing tools designed to facilitate Git management can also enhance your workflow significantly.
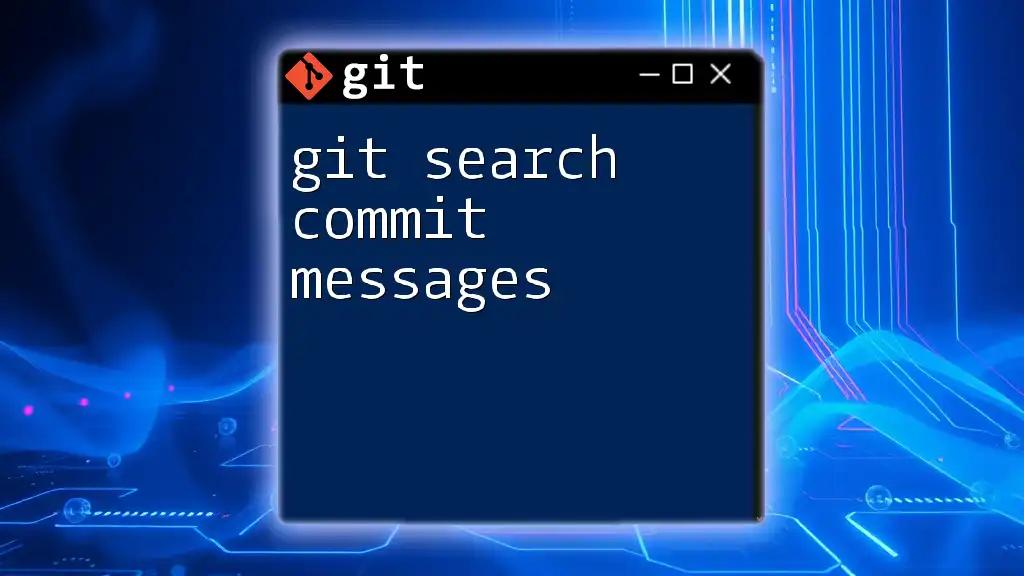
FAQ Section
-
What happens to the changes after a hard reset? A hard reset permanently removes the committed changes from your local history as well as from your working directory.
-
Can I recover files after a hard reset? Recovering files after a hard reset can be challenging, but utilizing `git reflog` may help you find lost commits.
-
What's the difference between reset, revert, and checkout? `git reset` alters your commit history, `git revert` safely creates a new commit to undo changes, and `git checkout` allows you to switch to a different commit or branch without changing the history.
With this guide, you should feel equipped to confidently manage commits in Git, ensuring that your version control processes are efficient and effective.