The "initial commit" in Git marks the creation of a new repository and signifies the first recorded change to the codebase.
Here’s how you can make an initial commit:
git init # Initializes a new Git repository
git add . # Stages all files for the commit
git commit -m "Initial commit" # Creates the initial commit with a message
What is an Initial Commit?
An initial commit is the first recorded change in a Git repository, marking the beginning of version control for a project. This pivotal moment not only establishes the project’s timeline but also lays down the initial structure for all subsequent development.
Purpose of the Initial Commit
The purpose of the initial commit extends beyond setting up version control; it serves several foundational objectives:
- Establishing a Base: It provides a source point for all future changes and milestones in the project.
- Documenting Intent: The commit message often reflects the intention of the project, helping convey its goals to contributors over time.
- Version Control Readiness: By making an initial commit, you make the repository ready for tracking changes, collaborating with others, or simply managing your own iterations of the project.
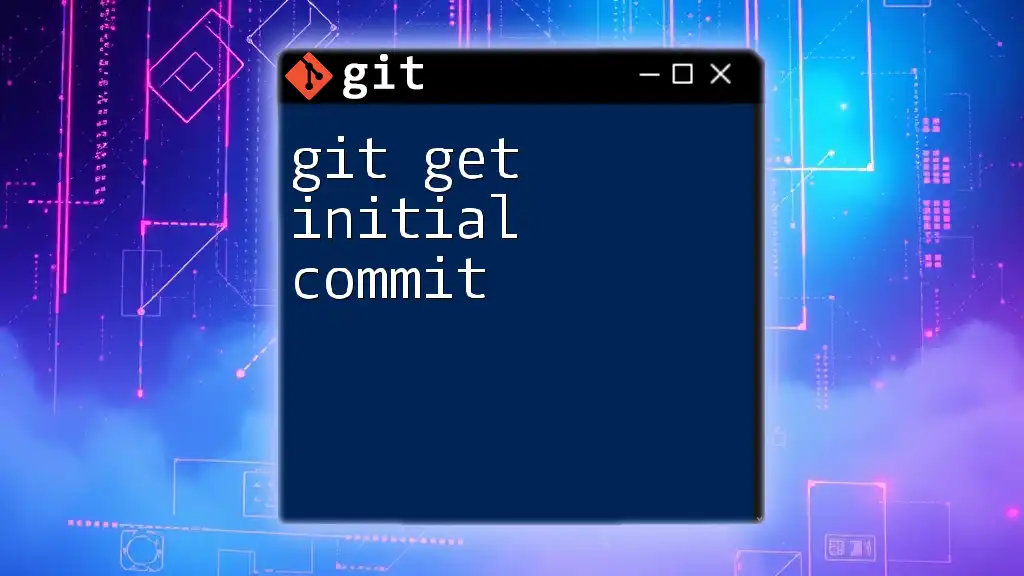
How to Prepare for Your Initial Commit
Before making your initial commit, it's essential to set up your Git environment correctly.
Setting Up Your Git Environment
Installing Git
Installing Git depends on your operating system:
- Windows: Download the Git installer from the official site and follow the instructions.
- macOS: Install via Homebrew with `brew install git` or download the installer from the official site.
- Linux: Use your package manager, for example, `sudo apt install git` for Debian-based systems.
Configuring Your Git
Setting up your Git identity ensures that commits are tagged with the correct author information. Use the following commands to configure it:
git config --global user.name "Your Name"
git config --global user.email "youremail@example.com"
Creating a New Directory for Your Project
To create and navigate into a new project directory, execute the following commands. This becomes the new workspace for your project.
mkdir my-awesome-project
cd my-awesome-project
Initializing a Git Repository
Now that your project directory is created, you need to initialize it as a Git repository. This is done using the `git init` command:
git init
This command sets up a new Git repository by creating a special `.git` directory in your project folder, which will track all your commits and configurations.
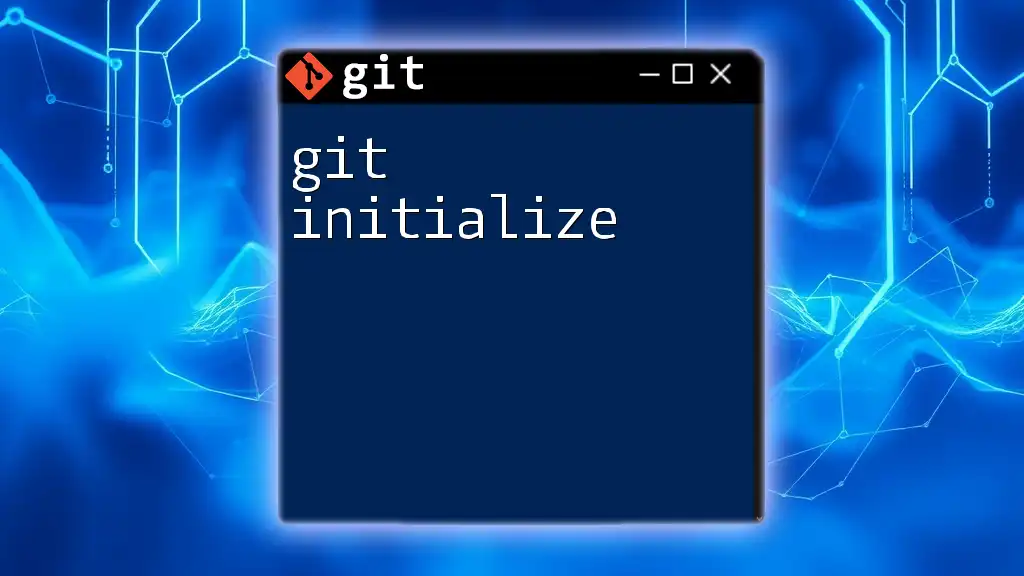
Making Your Initial Commit
With your environment prepared and the repository initialized, it’s time to make your initial commit.
Adding Files to Your Project
Start by creating a sample file, such as `README.md`, which often describes your project. You can create it with the following command:
echo "# My Awesome Project" >> README.md
This command creates a `README.md` file and adds a title to it.
Staging Your Changes
Before you can commit your changes, you need to add files to the staging area. This temporary space holds your changes until you are ready to commit them.
To stage the newly created file, use:
git add README.md
You can also stage all files in the directory simultaneously with:
git add .
This command stages every new or modified file for the next commit.
Committing Your Changes
With your files staged, you are ready to make your initial commit using the command:
git commit -m "Initial commit"
The `-m` flag allows you to include a message that explains the nature of the changes. It’s essential to write clear, descriptive commit messages to help anyone looking at the history understand the context of each commit.
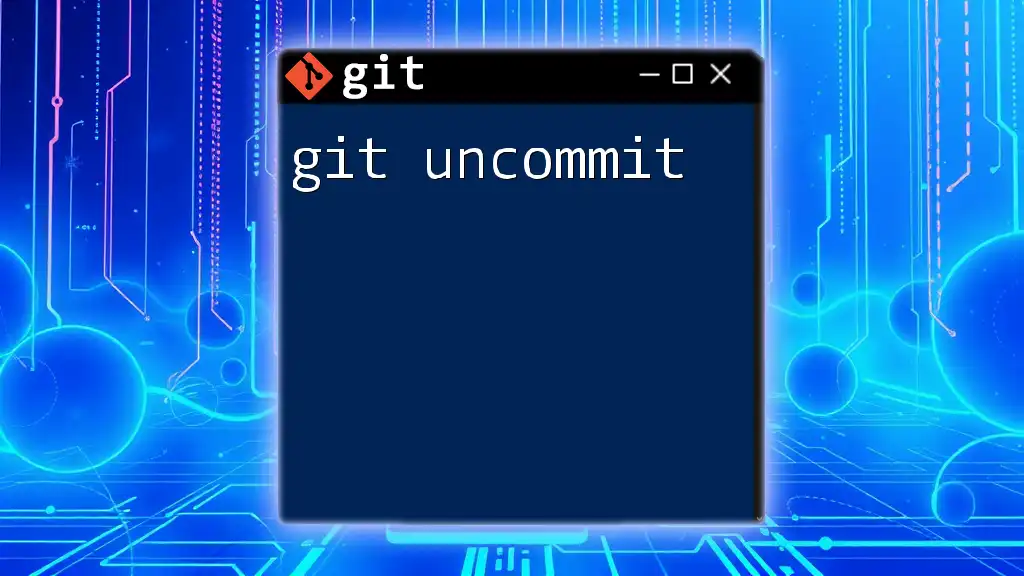
Understanding Git Status and Log
Once you have committed your changes, you might want to check the state of your repository.
Checking the Status of Your Repository
You can use the `git status` command to verify which files are staged, unstaged, or untracked. This command provides crucial information on the state of your repository before making any further commits.
git status
Viewing Your Commit History
To view the commit history, use the `git log` command, which shows a list of all commits in the current branch:
git log
This command provides detailed information about each commit, including the commit hash, author, date, and commit message. It's a window into your project’s evolution.
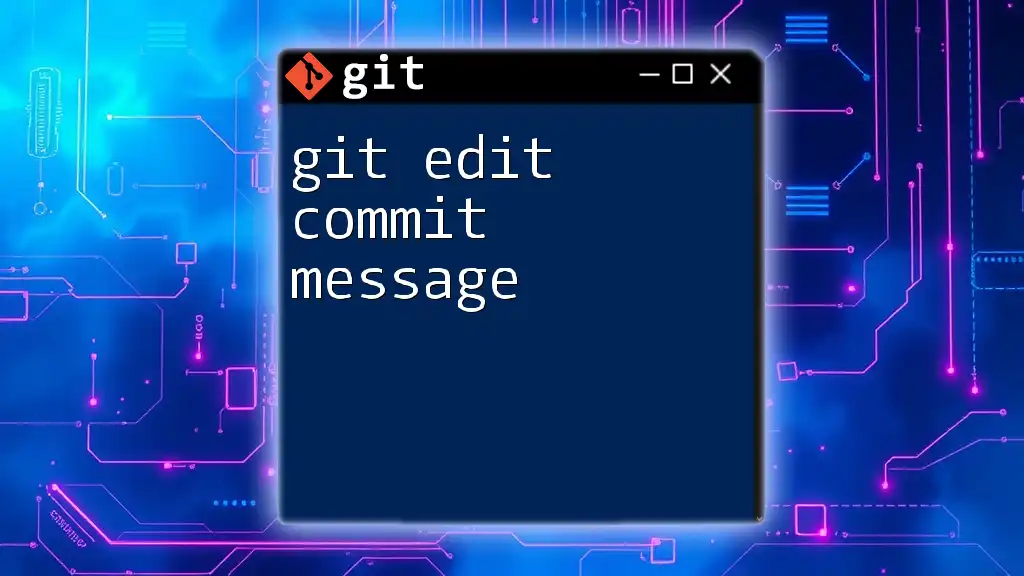
Customizing Your Initial Commit (Optional)
While the initial commit can be straightforward, there are opportunities for customization that can help structure your project better.
Adding Additional Files
Feel free to include any additional files or directories in your initial commit. For instance, if you wanted to include a LICENSE file, create it and stage it similarly.
Using .gitignore
Including a `.gitignore` file at the outset can save you from tracking files you don’t want to include, such as temporary files or libraries. This file tells Git what files or directories to ignore in your project. To create a `.gitignore` file, use:
touch .gitignore
echo "node_modules/" >> .gitignore
The example above ensures that the `node_modules` directory won't be tracked, which is especially useful in JavaScript projects.
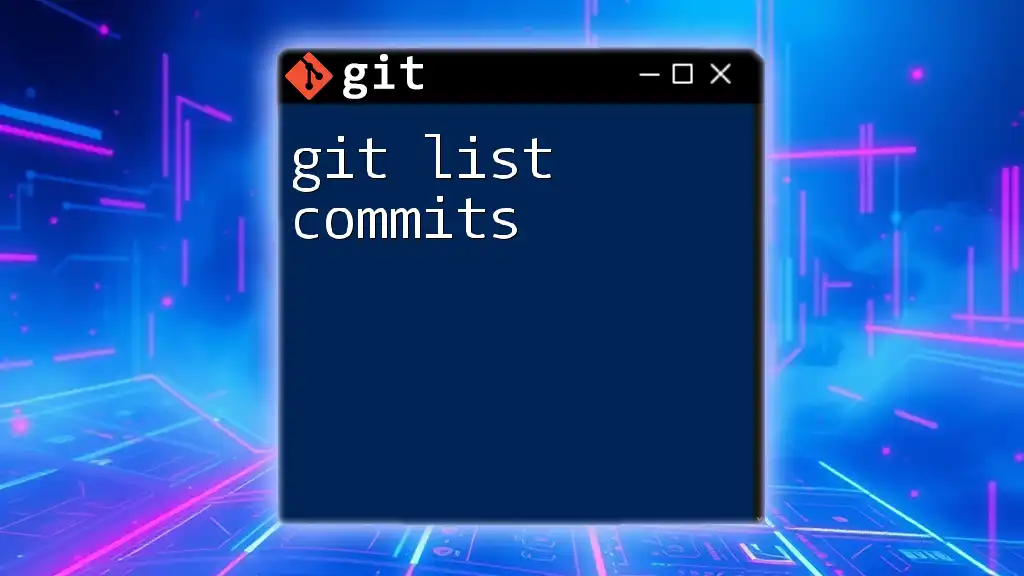
Common Mistakes to Avoid
While making your initial commit, be aware of potential pitfalls that could lead to confusion or issues later on.
Forgetting to Stage Files
A common error is forgetting to stage files before executing the commit command. This oversight means that changes won’t be included in the commit, which can lead to gaps in your project’s history.
Writing Poor Commit Messages
Another frequent mistake is failing to write clear and informative commit messages. A good commit message provides context and details about what changes were made and why, aiding in better project management and collaboration in the future.
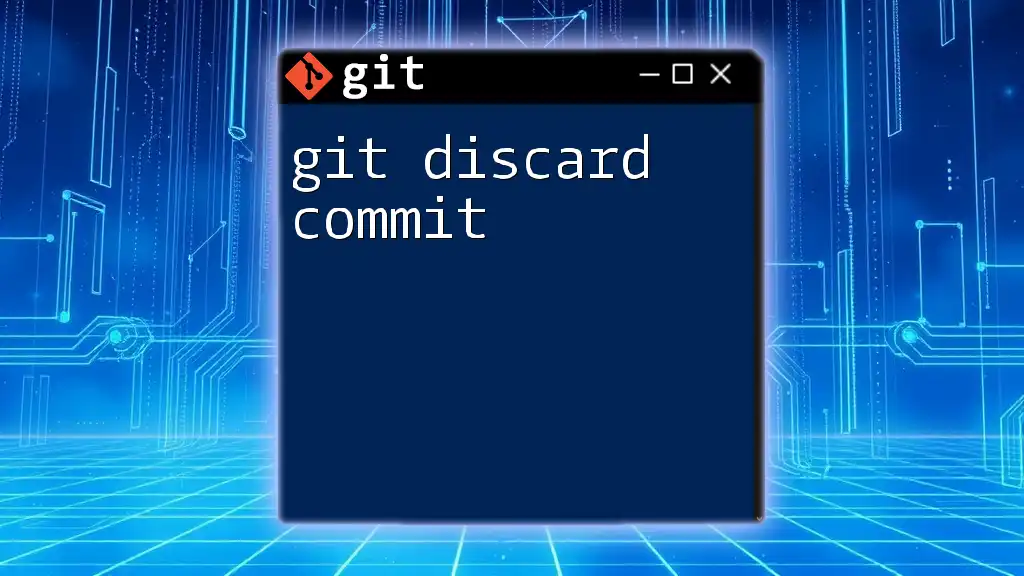
Conclusion
In summary, establishing a git initial commit is a vital first step in any Git-based project. Following the outlined steps ensures you set a solid foundation for tracking changes and collaboration. By practicing these procedures, you'll gain familiarity with Git commands that will serve you well throughout your development journey.
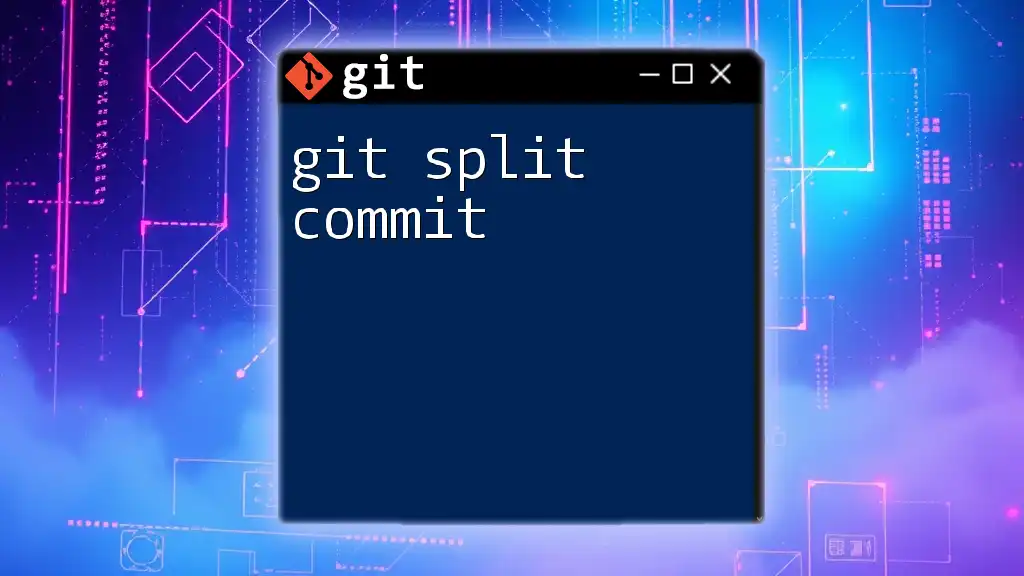
Additional Resources
For further reading and exploration, consider diving into the [official Git documentation](https://git-scm.com/doc) or check out comprehensive courses on platforms like Codecademy, Udacity, or free tutorials available online. These resources can deepen your understanding of Git beyond the initial commit.