In Git, `git add` stages changes in your working directory for the next commit, while `git commit` saves those staged changes to the repository's history; together, they allow you to manage and track your project's evolution efficiently.
git add . # Stages all changes
git commit -m "Your commit message here" # Commits the staged changes with a descriptive message
Understanding Git Basics
What is Git?
Git is a powerful version control system that enables developers to manage changes to code over time. It allows multiple users to collaborate on projects efficiently, providing a way to track and merge changes seamlessly. One of the core benefits of using Git is its ability to maintain a history of all modifications, which is crucial for debugging and understanding the evolution of a codebase.
The Workflow of Git
To fully grasp how to use git add and git commit, it's essential to understand Git's three main components: the Working Directory, the Staging Area, and the Repository.
- Working Directory: This is the local directory on your machine where your project files reside. You make changes to files here.
- Staging Area: This is a temporary space to hold changes before they are committed. Think of it as a place to prepare changes for the final save to the repository.
- Repository: This is where the committed changes are stored. Once changes are committed, they become part of the project's history, which can be revisited and reverted if necessary.
git add moves changes from the Working Directory to the Staging Area, while git commit takes those staged changes and saves them to the Repository.
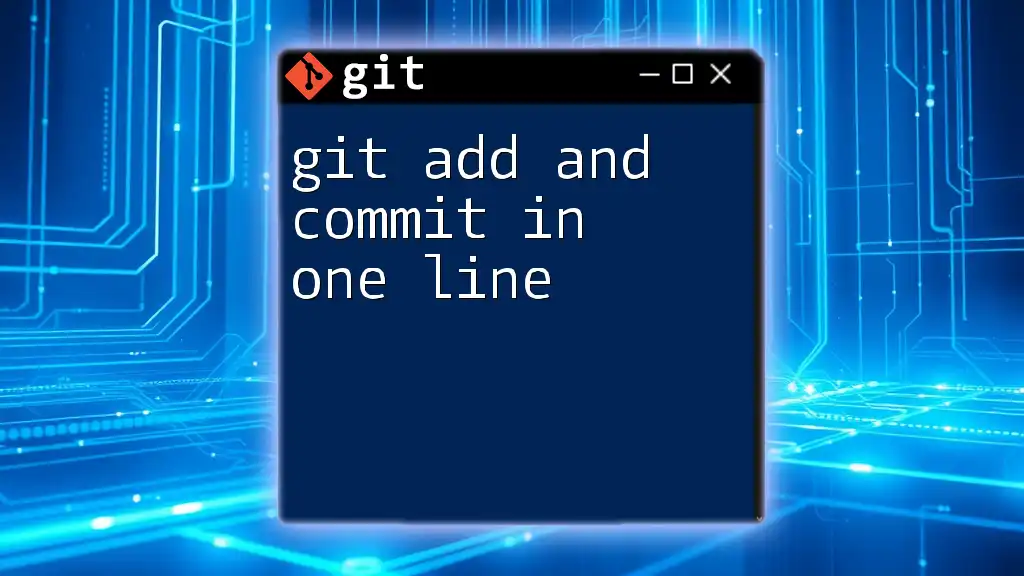
The Command: git add
What Does git add Do?
The git add command plays a crucial role in the Git workflow. It allows you to specify which changes you want to include in your next commit, effectively staging them. This step is critical because it gives you control over what changes are saved at any given time.
The Syntax of git add
The basic command structure for git add is as follows:
git add <file_path>
Staging Changes
When you want to stage a single file, you simply provide the path to that file. For instance, if you've modified a file named `myfile.txt`, you would stage it using:
git add myfile.txt
Staging Multiple Files
You can also stage multiple files at once by listing them one after the other:
git add file1.txt file2.txt
This is particularly handy when you want to group several changes into a single commit.
Staging All Changes
If you want to stage all modified files in your working directory, use the following command:
git add .
This command stages all new, modified, and deleted files, making it an efficient option for preparing for a commit.
Options and Variants
Several options enhance the functionality of git add. One useful option is `-A`, which stages all changes (including file deletions):
git add -A
Another option is `-u`, which stages modified and deleted files, but not new files:
git add -u
Using these options effectively can streamline your workflow and save time.
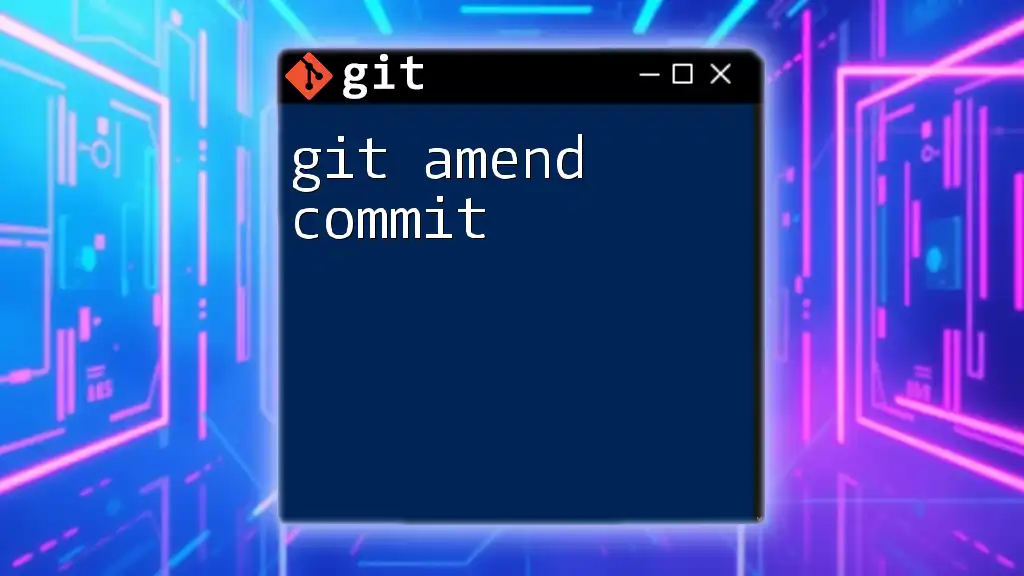
The Command: git commit
What Does git commit Do?
The git commit command is used to save your staged changes to the repository. Commits represent a point in the project's history, allowing you to track changes over time. Every commit requires a commit message, which should summarize the changes being made.
The Syntax of git commit
The command structure for git commit is straightforward:
git commit -m "<commit_message>"
Writing Meaningful Commit Messages
Writing effective commit messages is crucial for maintaining a clear project history. A good commit message should be concise yet descriptive. For instance:
git commit -m "Add feature to improve performance"
This message is clear and indicates the purpose of the commit. Always strive to use the imperative mood—this convention makes it easier to read the logs later.
Committing Staged Changes
Once you have staged your changes with git add, committing them is just a matter of executing the command:
git commit -m "Fix bug in user authentication"
This saves the changes to the repository, making them part of the project's history.
Amending Commits
In case you realize that you made a mistake or need to adjust your last commit, you can easily amend it with:
git commit --amend -m "Update commit message"
This command lets you modify the last commit’s message or changes.
Multi-Line Commit Messages
To write multi-line commit messages for more complex changes, use:
git commit -m "Initial commit" -m "This commit includes the basic project structure"
Providing additional context in a multi-line format can help explain the reasoning behind changes more thoroughly.
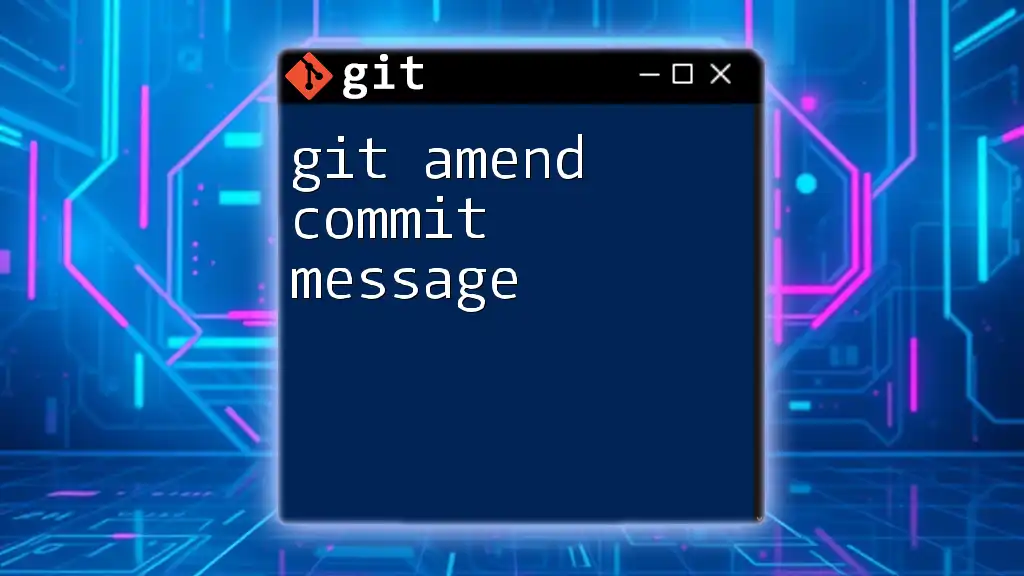
Best Practices for Using git add and git commit
When to Use git add
Understanding when to stage changes is vital. It is essential only to stage relevant changes for a specific task or fix. Over-staging can lead to including unintended modifications in commits, making your project's history confusing.
Commit Frequency
Regularly committing changes is another best practice. Aim to commit often but ensure each commit is meaningful. Smaller, incremental commits make it easier to identify changes and roll back if necessary.
Consistency in Commit Messages
Maintaining a consistent style in your commit messages fosters clarity. Teams should agree on a format or guidelines to follow, so everyone can read and understand the project history with ease.
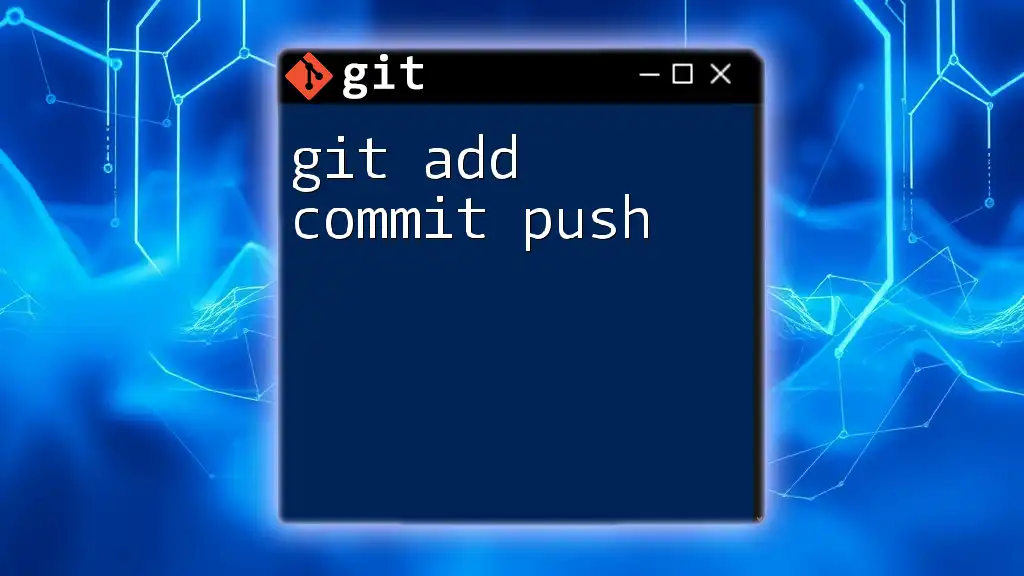
Troubleshooting Common Issues
What to Do When You Forget to Add Changes
If you overlook staging changes, use git status to check the state of your working directory. After identifying any untracked files, quickly stage and commit them:
git add forgotten_file.txt
git commit -m "Include forgotten file in past commit"
Handling Mistakes in Commits
If you realize that you made a mistake in your commit, Git provides options to correct it. You can undo the last commit and keep the changes in staging with:
git reset --soft HEAD~1
This command can save the day when you need to refine your last commit.
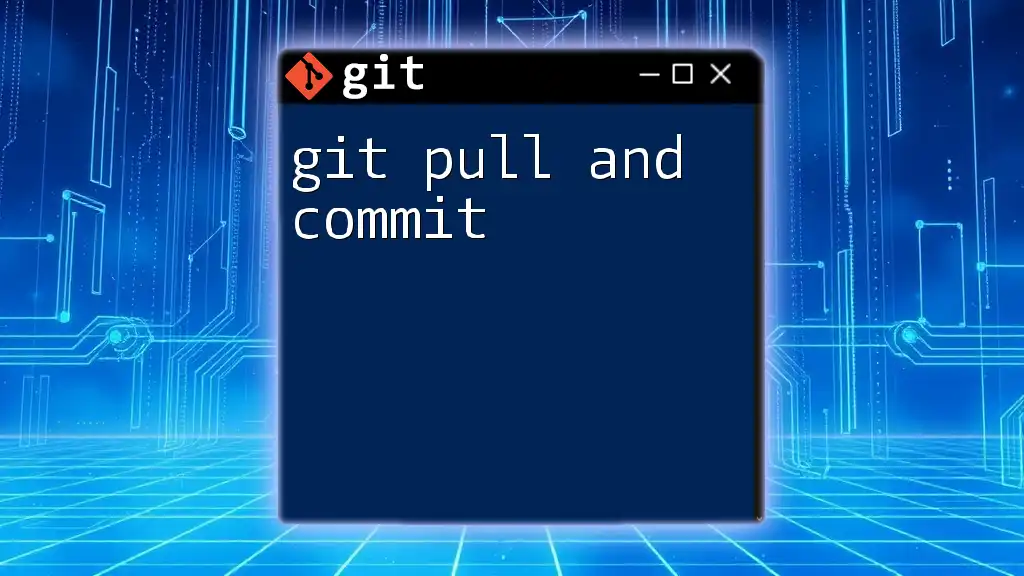
Conclusion
Mastering git add and commit is essential for anyone working with Git. These commands are cornerstones of the Git workflow, enabling effective tracking and management of changes. Regular practice will not only boost your confidence but also enhance your coding projects. Remember to always adopt the best practices mentioned above, and you'll find your version control process becomes ever more seamless and efficient.
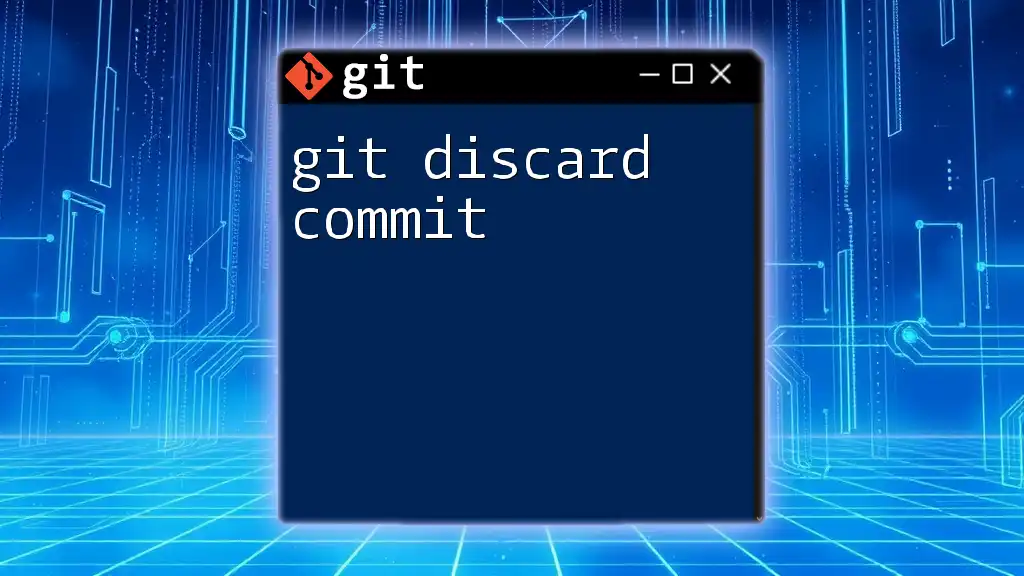
Call to Action
If you're eager to dive deeper into Git and improve your skills, consider subscribing to our updates for more insightful tutorials. You can also grab our downloadable cheat sheet for quick reference on essential git commands!