The `git update-index --assume-unchanged` command allows you to tell Git to ignore changes in a specified file in your working directory, effectively treating it as unmodified for future commits.
git update-index --assume-unchanged path/to/your/file
Understanding `git assume-unchanged`
What is `git assume-unchanged`?
The `git assume-unchanged` command is a powerful tool used in Git that signals to the version control system that the specified file should be treated as unchanged, even if local modifications exist. This allows developers to temporarily ignore changes to certain files that may not be ready to commit or are not relevant to the current work.
When to Use `git assume-unchanged`
Common scenarios for using `git assume-unchanged` include:
- Configuration Files: When you have local configuration files that differ from your repository's version, and you want to keep those local changes without them being tracked.
- Temporary Debugging: During debug sessions, you might want to ignore minor changes in files that are not relevant to the bug you're fixing.
The primary benefit of using this command is that it helps keep your working directory clean and organized by allowing you to focus on relevant changes without the distraction of unrelated modifications.

How to Use `git assume-unchanged`
Basic Syntax
The general command structure for marking a file as "assume unchanged" is as follows:
git update-index --assume-unchanged <file_path>
It is essential to recognize that this command modifies the index. However, it does not revert or modify the actual content of the file.
Example: Using `git assume-unchanged`
To illustrate how to effectively use `git assume-unchanged`, let's go through a step-by-step example.
Step 1: Identify the File
Before using the command, you need to identify the file you want to mark. You can do this by checking the status of your Git repository to see which files have been modified.
git status
This command will show you a list of modified files, allowing you to identify which one needs the `assume-unchanged` flag.
Step 2: Mark the File as "Assume Unchanged"
Once you have identified the file you want to ignore, you can mark it using the command:
git update-index --assume-unchanged <file_path>
Replace `<file_path>` with the actual path of the file. This command tells Git to stop tracking changes for this file in your local repository.
Step 3: Confirm the Change
To ensure that the file status has been updated correctly, you should check the current state of the index. Use the following command:
git check-ignore -v <file_path>
This will help you confirm whether the specified file is indeed being ignored.
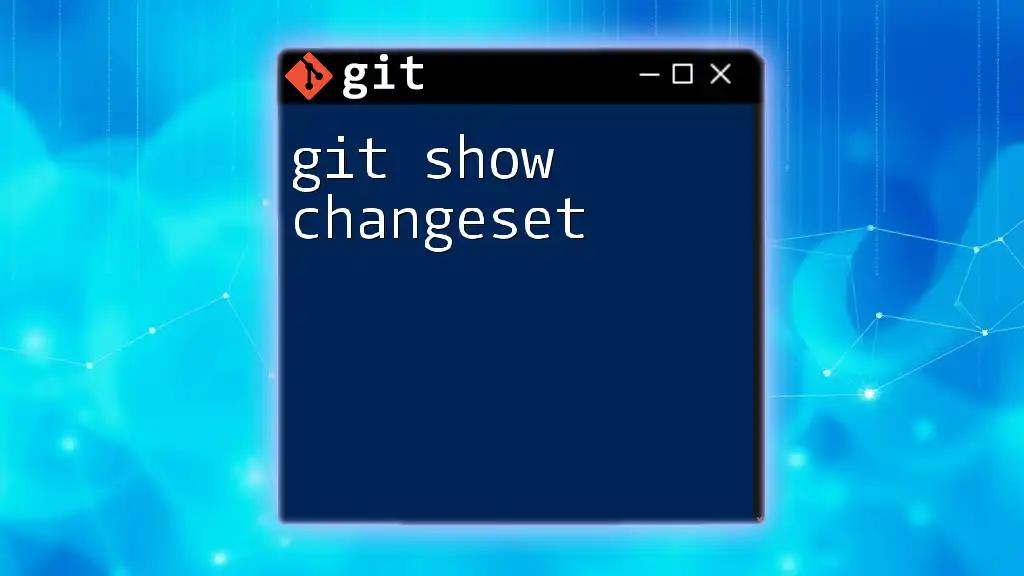
Limitations of `git assume-unchanged`
Persistent File Tracking
While `git assume-unchanged` prevents Git from tracking changes to the specified file, it is crucial to note that any changes made to the file are still present in your working directory. If you later revert or remove the assumption, those changes will be staged for commit.
Difference with `.gitignore`
It’s important to understand that `git assume-unchanged` is not the same as `.gitignore`. The `.gitignore` file is used to tell Git to completely ignore specific files or directories, while `git assume-unchanged` instructs Git to temporarily overlook modifications to a tracked file. Use `.gitignore` for files that should never be tracked, and `git assume-unchanged` for temporary local changes to files that are already part of the repository.
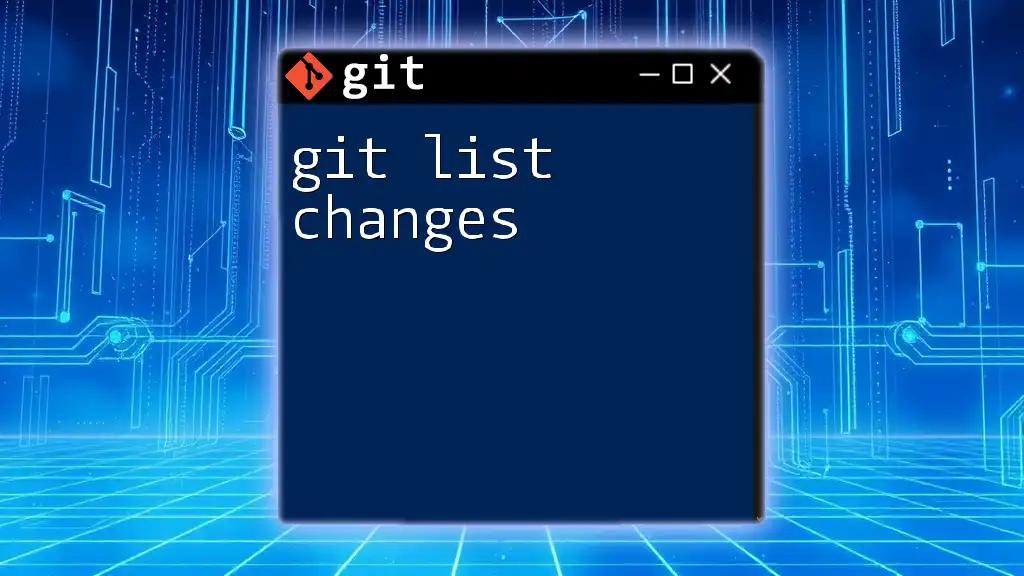
Managing Files with `git assume-unchanged`
Listing Files Marked as "Assume Unchanged"
To manage files that have been marked with `git assume-unchanged`, you can list all files currently in this state by running:
git ls-files -v | grep '^[[:lower:]]'
This command will display all files in the "assume unchanged" state, helping you keep track of them.
Reversing `git assume-unchanged`
If you decide that you want to stop ignoring changes to a file, you can revert the `assume-unchanged` status with the following command:
git update-index --no-assume-unchanged <file_path>
This will mark the file to be tracked again, and any changes you made will now be monitored by Git.
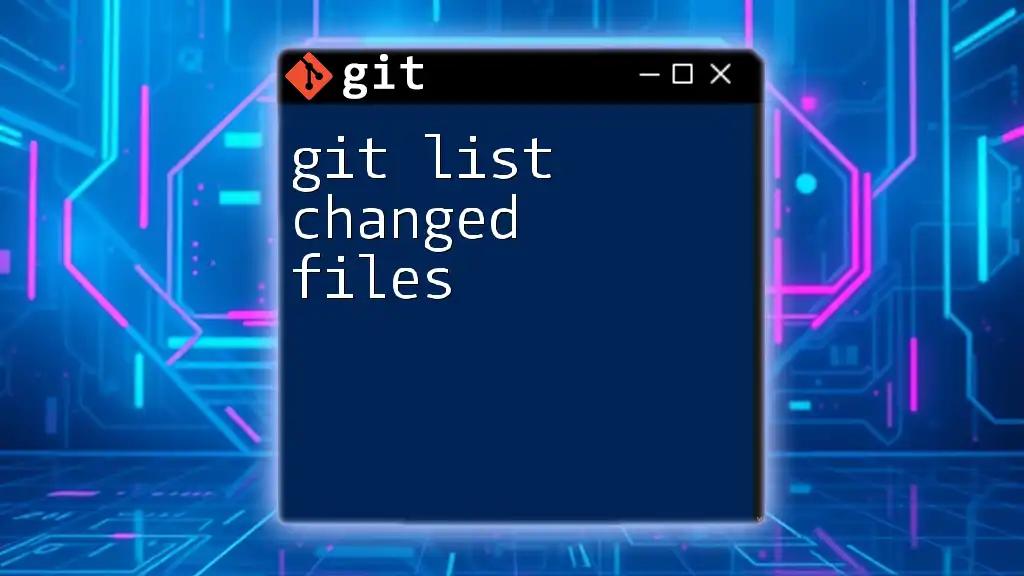
Best Practices for Using `git assume-unchanged`
Consider Version Control Integrity
Maintaining project integrity is essential when using `git assume-unchanged`. Always be mindful of which files you are marking in this way. Avoid marking files that are crucial for the project's functionality, as this could lead to discrepancies between local and shared codebases.
Use Sparingly
It is recommended to use `git assume-unchanged` sparingly. Overusing this command can lead to situations where important changes are overlooked, potentially leading to bugs or merge conflicts in future commits.
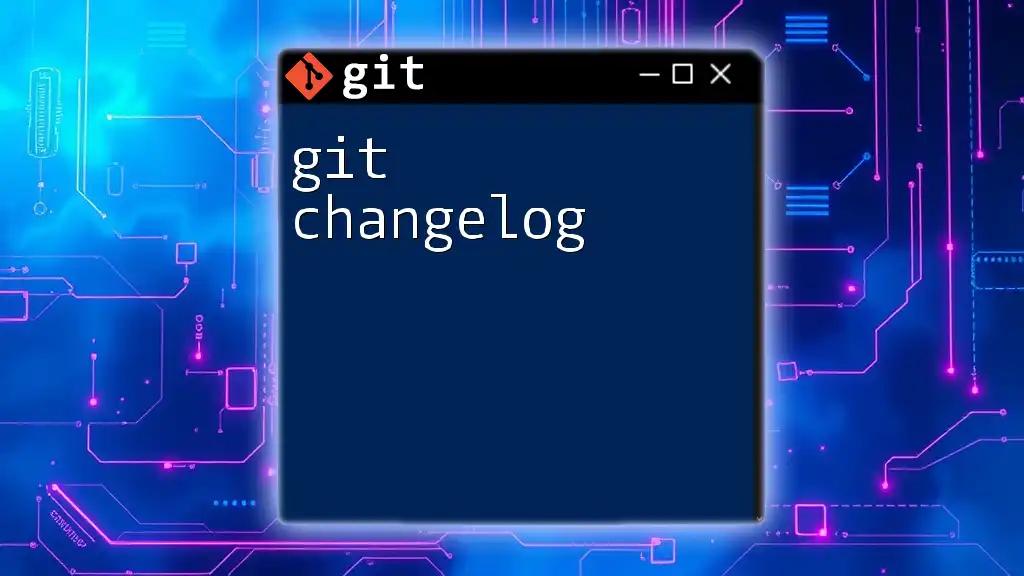
Alternative Methods
Using Stashing
If you have local changes that you want to set aside without affecting the current branch, consider using `git stash`. This allows you to save your changes temporarily and apply them later when needed. For example:
git stash push -m "My temporary changes"
This command stores your changes away cleanly, allowing you to switch branches or reset your state without losing your work.
Branching Strategies
Another method to manage changes without cluttering your working directory is through branching. If you know that you need to make divergent changes, create a new branch for those changes instead of marking files as "assume unchanged." This approach keeps your project organized and makes it easier to manage various development streams.
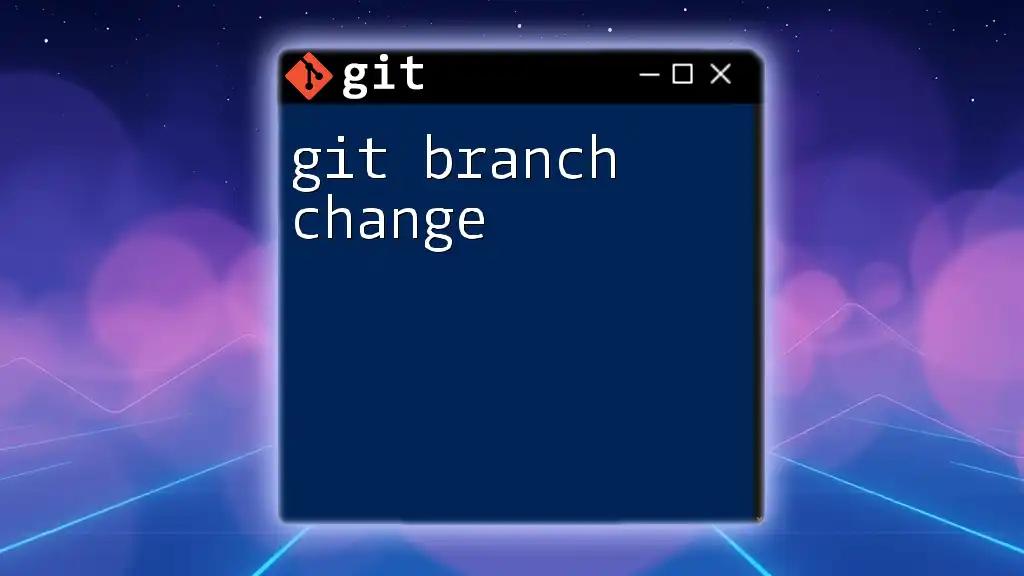
Conclusion
In summary, the `git assume-unchanged` command is a handy tool for developers who need to temporarily ignore changes to certain files. However, it should be used judiciously to avoid complications in version control. By understanding when and how to apply this command, you can maintain a cleaner workflow and focus on what truly matters in your development process. As always, be sure to explore other Git features and commands to enhance your productivity and version control strategies.