Git SSH is a secure method for accessing remote Git repositories using SSH (Secure Shell) for authentication and communication, allowing you to push and pull changes without repeatedly entering your username and password.
Here’s how to set up an SSH key and add it to your Git configuration:
ssh-keygen -t rsa -b 4096 -C "your_email@example.com"
eval "$(ssh-agent -s)"
ssh-add ~/.ssh/id_rsa
cat ~/.ssh/id_rsa.pub
What is SSH?
Understanding SSH
SSH, or Secure Shell, is a protocol used to securely access and manage devices over an unsecured network. Initially developed to replace older protocols that transmitted data in cleartext, SSH encrypts the data sent between a client and server, providing a secure channel over which sensitive information can be transferred without risk of interception.
One of the main benefits of using SSH is that it provides a secure way to authenticate users and devices, making it a vital tool for developers and system administrators working with remote servers and applications.
How SSH Works
SSH operates using a client-server model where a client initiates a connection to a server. This connection is secured through a combination of cryptographic algorithms and protocols that provide:
- Encryption: This protects the data being sent from eavesdroppers.
- Authentication: This verifies that the communication is only between legitimate parties.
- Integrity: This ensures that the data sent has not been tampered with during transmission.
At the core of SSH is the concept of public/private key pairs. The public key can be shared with anyone while the private key must remain confidential. When a user tries to connect, the server verifies the client's identity by checking its public key against the stored public keys. If they match and the user can present the private key, the connection is established.
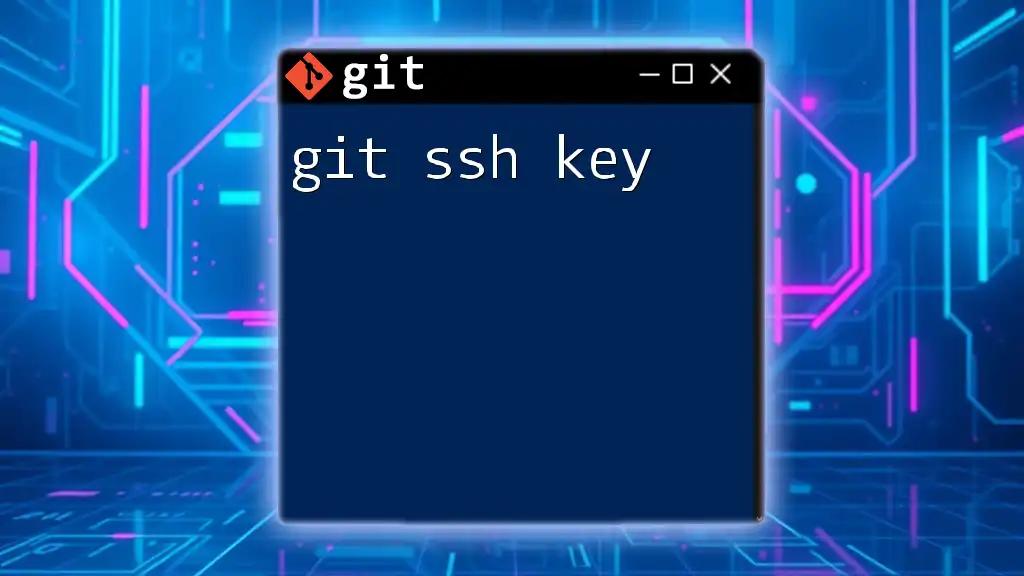
Setting Up SSH for Git
Generating SSH Keys
To begin using `git ssh`, you'll first need to generate an SSH key pair for secure communication. This can be done easily from the command line.
To generate your SSH key pair, use the following command:
ssh-keygen -t rsa -b 4096 -C "your_email@example.com"
- The `-t` flag specifies the type of key to create; RSA is commonly used.
- The `-b` flag sets the key length, with 4096 bits being highly secure.
- The `-C` option allows you to add a label or comment to your key, usually your email for identification purposes.
You will be prompted to specify a filename for your new key. You can press Enter to use the default location (`~/.ssh/id_rsa`). Optionally, you can create a custom name for your key if you plan to generate multiple keys. You will then be asked to enter a passphrase for added security. Choosing a strong passphrase is highly recommended.
Adding SSH Keys to the SSH Agent
An SSH agent is a program that runs in the background and manages your SSH keys, enabling you to work without the hassle of repeatedly entering your passphrase. To ensure your SSH key is added to the agent, follow these steps:
First, start the SSH agent in the background with the command:
eval "$(ssh-agent -s)"
After starting the agent, add your SSH private key to it:
ssh-add ~/.ssh/id_rsa
Make sure to replace `id_rsa` with your key's filename if you customized it in the previous step.
Adding SSH Key to Git Hosting Service
If you use Git hosting services like GitHub, GitLab, or Bitbucket, you need to add the public key associated with your SSH to your account for authentication.
To copy your public SSH key to the clipboard, use:
cat ~/.ssh/id_rsa.pub
Now, you can add it to your preferred Git hosting service:
-
GitHub: Navigate to Settings > SSH and GPG keys > New SSH key, and paste the copied key.
-
GitLab: Go to Preferences > SSH Keys, then paste the public key, and click Add key.
-
Bitbucket: Select Personal settings > SSH keys, then click Add key and paste your public key.
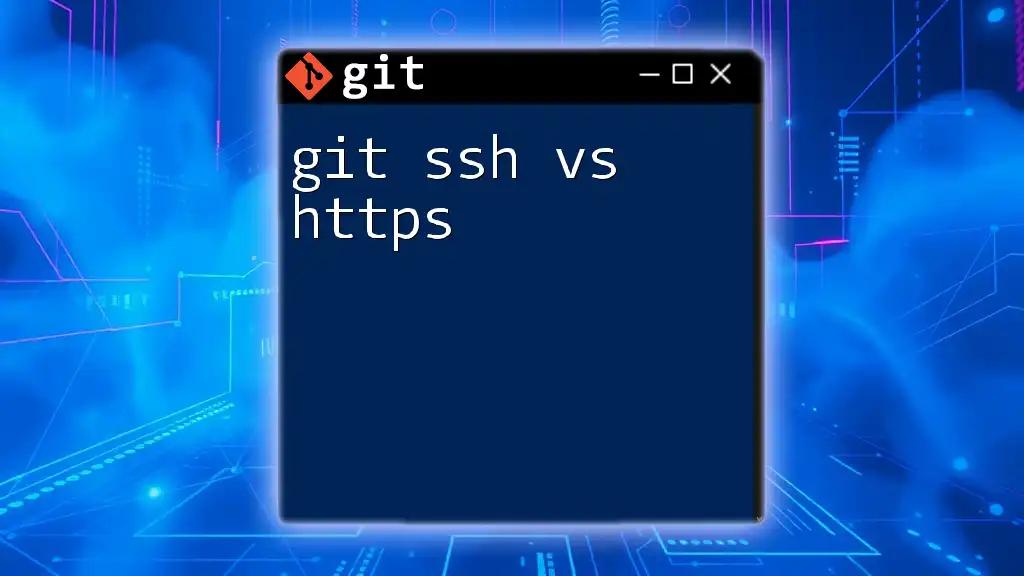
Configuring Git to Use SSH
Verifying SSH Configuration
To check if everything is set up correctly, attempt to connect to your hosting service with the following command:
ssh -T git@github.com
You should receive a success message confirming that your identity is recognized.
Configuring Git Remote URLs
It's important to configure your Git repositories to use SSH URLs instead of HTTPS for secure operations. You can easily change your remote URL with this command:
git remote set-url origin git@github.com:username/repo.git
Replace `username` with your GitHub username and `repo` with your repository name. This change allows you to push and pull without entering your username and password each time.
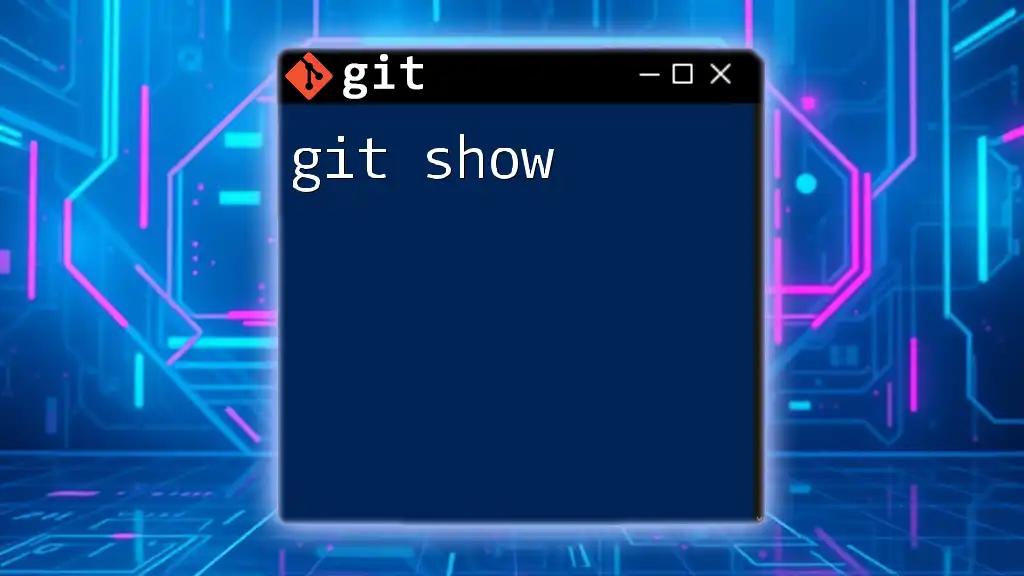
Managing Multiple SSH Keys
Use Cases for Multiple SSH Keys
Sometimes, developers need different SSH keys for various environments or roles, such as personal usage versus work-related projects. This ensures that keys remain compartmentalized and protect against unauthorized access.
Configuring Multiple SSH Keys
To handle multiple SSH keys, generate additional keys similar to the first step but with unique filenames, such as `id_rsa_work` and `id_rsa_personal`.
Next, you can configure your SSH client to use these keys based on the host by editing your `~/.ssh/config` file. Example configuration would look like this:
Host github.com
HostName github.com
User git
IdentityFile ~/.ssh/id_rsa_github
Host gitlab.com
HostName gitlab.com
User git
IdentityFile ~/.ssh/id_rsa_gitlab
Each `Host` directive corresponds to a specific service, ensuring that the correct SSH key is used automatically.
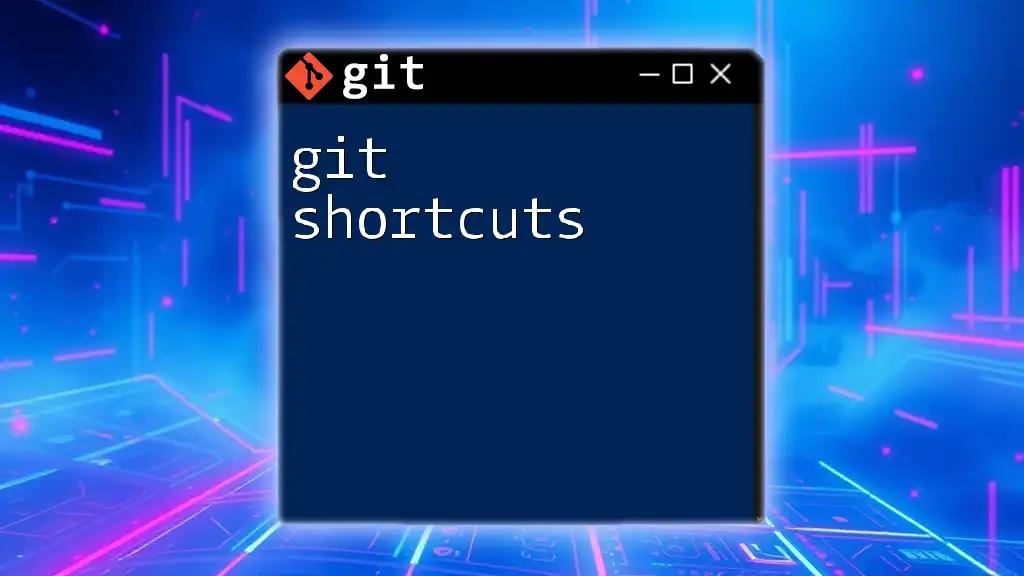
Best Practices for SSH Security
Keeping Your SSH Keys Secure
Securing your private SSH keys is crucial. Always store them in the `.ssh` directory and never share them. Use strong passphrases to encrypt your private key files. Consider limiting access to your SSH keys with permission settings:
chmod 600 ~/.ssh/id_rsa
Rotating SSH Keys Regularly
It’s a good practice to rotate your SSH keys regularly, especially if you suspect that your keys may have been compromised. To safely rotate your keys, generate a new key pair, update the services with the new public key, and then remove the old key.
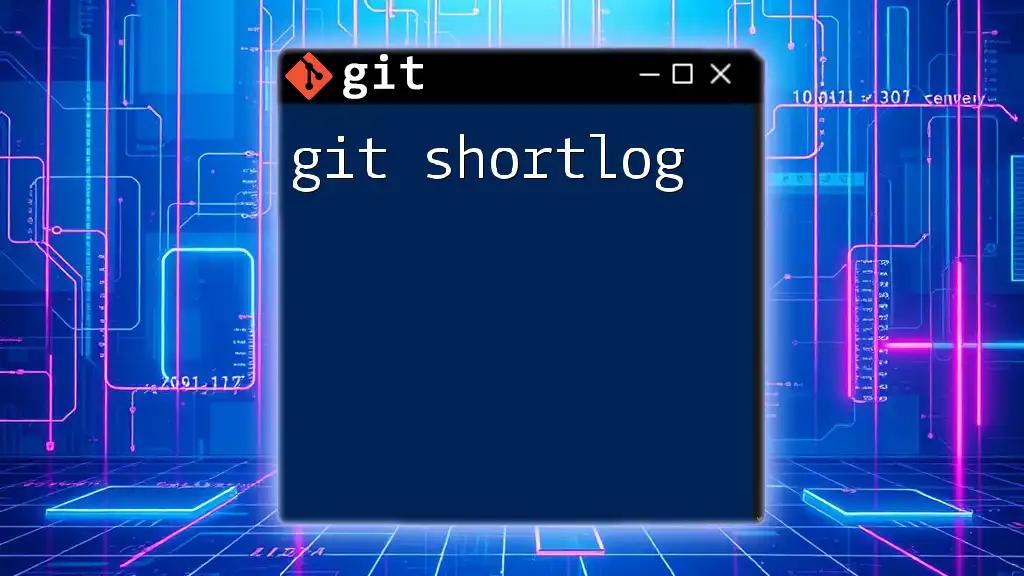
Common Troubleshooting Tips
Connecting Issues
If you encounter any connection issues, you may see errors such as “Permission denied (public key).” This generally means your public key is not correctly set in the Git hosting service or the private key permissions are incorrect.
Verifying SSH Key Permissions
Ensure that your private key has the correct permissions. If they are too permissive, you can adjust them using:
chmod 600 ~/.ssh/id_rsa
This command restricts access to the file, allowing only the owner to read and write, which prevents unauthorized users from accessing your private keys.
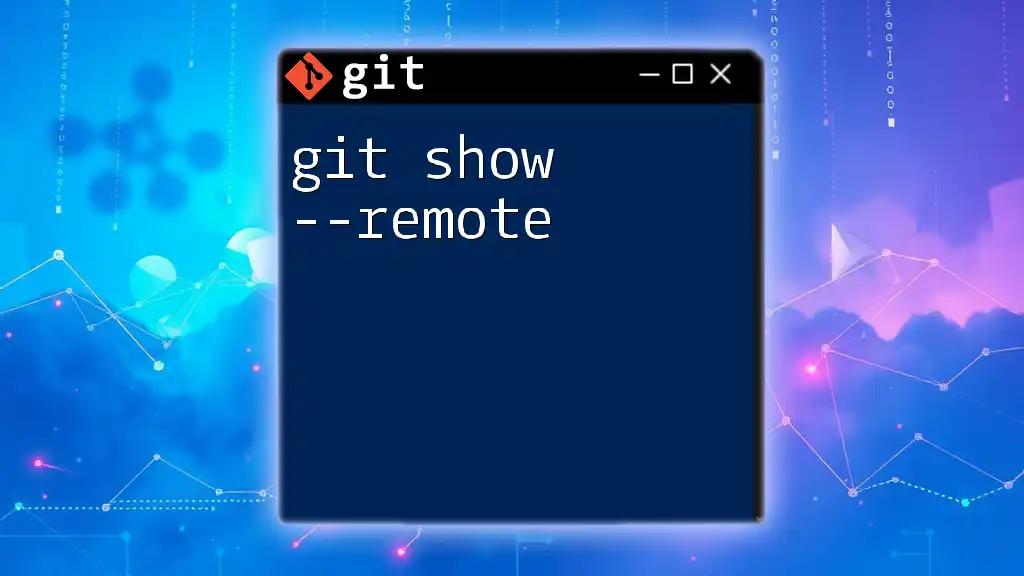
Conclusion
Using `git ssh` for version control is not only a secure way to handle your repositories but also simplifies authentication while working with remote servers. By following the steps outlined in this guide, you can seamlessly set up and manage SSH keys to improve your workflow and protect your code. Embrace SSH for your Git operations and enjoy the enhanced security it provides!
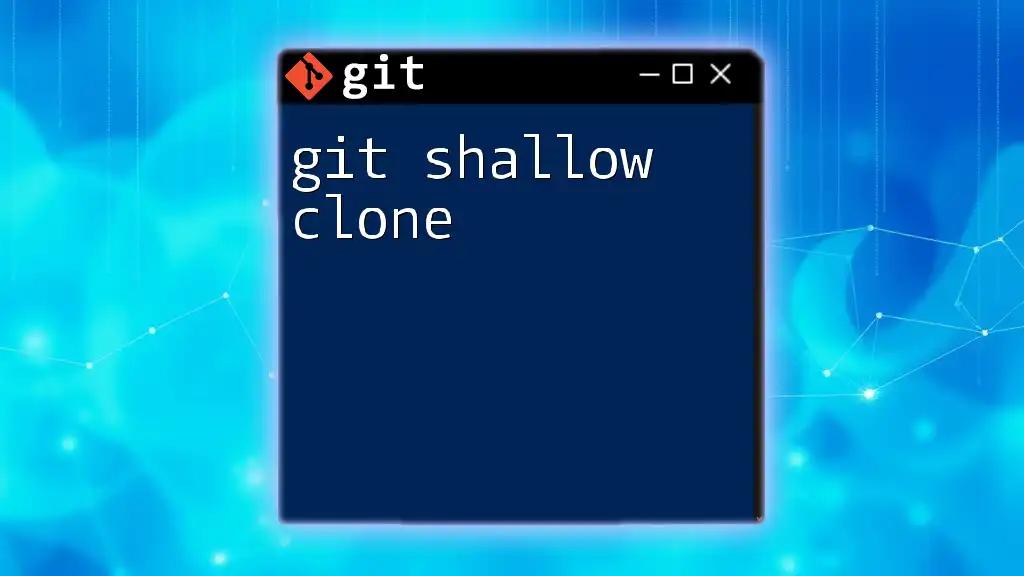
Additional Resources
To further your knowledge, refer to the official SSH documentation and Git documentation for additional details and advanced configurations. You'll find many resources to help deepen your understanding of these essential tools for modern software development.