A "git request pull" typically refers to creating a pull request in a collaborative Git workflow, where a contributor asks to merge their changes into a project repository.
Here's an example of how to create a pull request using the Git command line tool:
git push origin feature-branch
To create the actual pull request, you would typically do this through your Git hosting service's web interface, such as GitHub or GitLab, after pushing your branch.
What is a Pull Request?
A Pull Request (PR) is a method of submitting contributions to a project within platforms that use Git for version control, such as GitHub, GitLab, and Bitbucket. The primary purpose of a PR is to enable code collaboration amongst developers, allowing them to propose changes and discuss potential alterations before integrating them into the main codebase.
In the context of using Git, branching is a core concept that allows multiple developers to work on different features simultaneously without interfering with each other's code. Commits are individual changes that you make in your branch, while repositories store all the versions and changes of your project.
When to Use a Pull Request
A PR is essential in several scenarios:
- Collaboration: When multiple team members work together, a PR facilitates collective code review and discussion, ensuring that all changes are scrutinized before being merged into the main branch.
- Feature Implementation: When developing new features, it becomes necessary to propose these changes formally, allowing other team members to provide feedback.
- Bug Fixes: PRs are also ideal for addressing bugs, enabling developers to present their fixes for review before merging them into production.
In comparison to direct merges, Pull Requests are invaluable for maintaining code quality and ensuring that all team members have visibility into the evolving project.
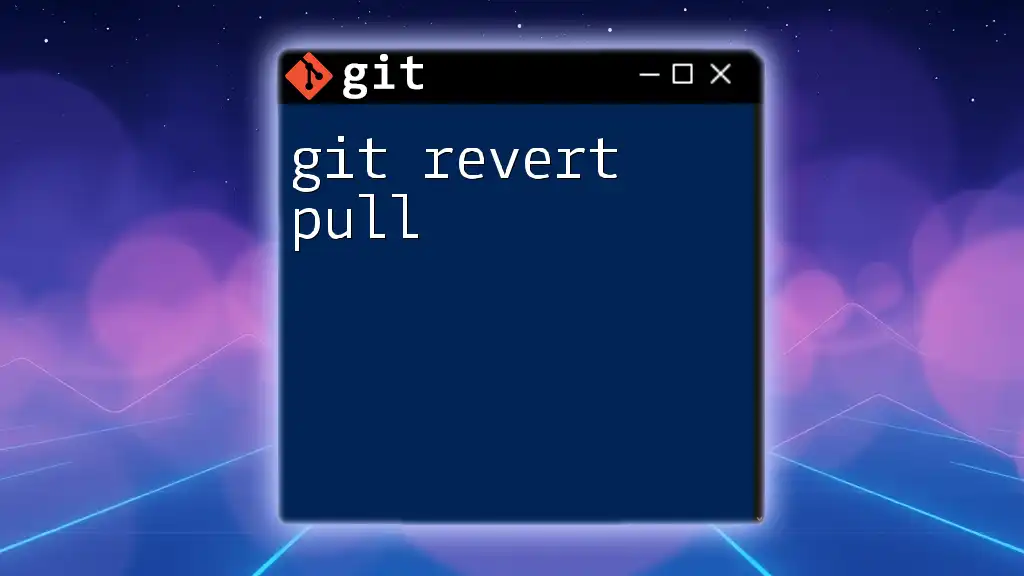
How to Create a Pull Request
Setting Up Your Repository
To create a Pull Request, you first need to set up your working environment. Begin with forking the original repository (if contributing to a public project) and cloning it to your local machine. Next, create a new branch distinct from the `main` or `develop` branch:
git checkout -b my-feature-branch
This new branch is where you will make your changes.
Committing Your Changes
When making changes, it’s imperative to follow the practice of atomic commits—each commit should encapsulate one logical change. This not only streamlines the review process but also maintains a clean project history. After making your modifications, stage your changes using:
git add .
Once staged, you can commit your changes with a clear and concise message:
git commit -m "Add new feature"
Pushing Changes to Remote
After committing your changes, push your branch to the remote repository. This process makes your updates available to others on the team:
git push origin my-feature-branch
Opening a Pull Request
To open a Pull Request, navigate to the repository on your chosen platform (like GitHub). Here’s a general outline of the process:
- Locate the "Pull Requests" tab.
- Click on the "New Pull Request" button.
- Select your newly pushed branch as the source and the base branch (usually `main` or `develop`) as the destination.
- Fill in the PR title and description, providing context for your changes. This step is crucial for reviewers to understand the motivation behind your feature.
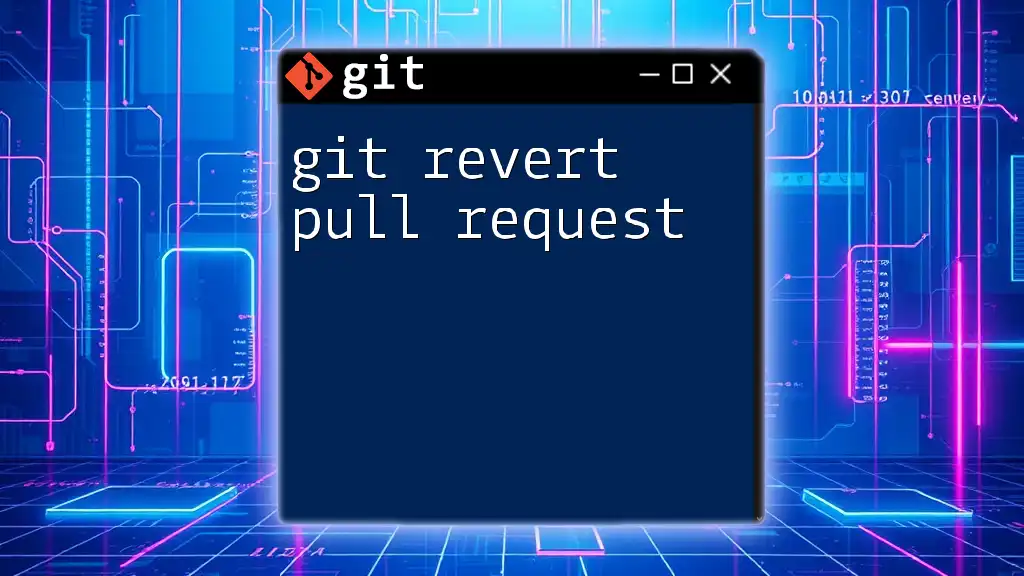
Understanding the Pull Request Process
Code Review
Once you've opened a PR, the next step is the code review process. Here, your teammates will assess the quality of your code, test its functionality, and provide feedback. The importance of thoughtful feedback cannot be overstated as it enhances code quality and fosters collaboration.
Common review practices may include suggesting ways to improve code performance or highlighting potential bug areas. It’s essential to view reviews as constructive, aiming to elevate the project's quality.
Merging a Pull Request
After the PR has received approvals and all comments have been addressed, it’s time to merge the changes into the base branch. During this process, you have several merge strategies to choose from:
- Merge Commit: Maintains the history of all branches, creating a new commit for merging.
- Squash and Merge: Combines all your commits from the feature branch into a single commit in the main branch, providing a cleaner project history.
- Rebase and Merge: Moves your commits to the tip of the base branch, preserving a linear commit history.
Each strategy has its pros and cons, so choose one that fits your team's workflow and project requirements.
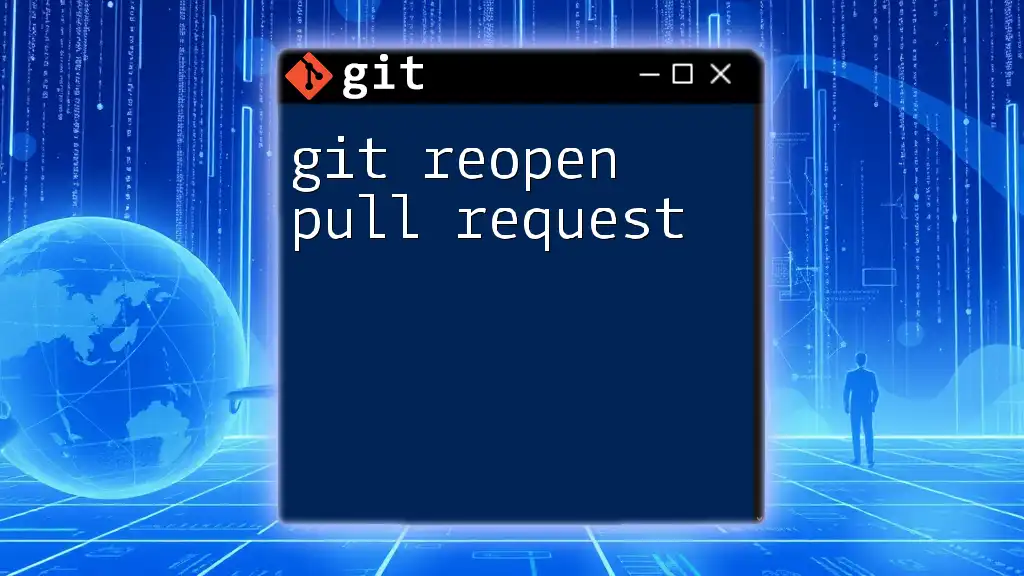
Best Practices for Pull Requests
Writing Effective PR Descriptions
A well-structured PR description aids reviewers and increases the likelihood of swift feedback. Begin with a clear, descriptive title and continue with a body that includes:
- The motivation behind the changes.
- A summary of the modifications.
- Instructions for testing if necessary.
Example of a good PR description:
Title: Add user authentication
Body:
- Implemented login and registration features
- Included validations for user input
- Added tests for the new features
Keeping Pull Requests Small
Aim to keep PRs small and focused. Smaller PRs are easier to review and reduce the chances of introducing significant bugs. If your work involves a large feature, consider breaking it down into smaller, more manageable parts. This practice not only benefits you but also simplifies the review process for your teammates.
Handling Conflicts
Identifying Merge Conflicts
When working collaboratively, merge conflicts can occur if two or more branches have modifications to the same lines in a file. You'll typically receive notification of conflicts when attempting to merge a PR, indicating that manual intervention is required.
Resolving Merge Conflicts
To resolve conflicts, follow these steps:
- Pull the latest changes from the base branch:
git pull origin main
- Identify and resolve conflicts in your working files. Git will mark the conflicting areas, allowing you to choose which changes to keep.
- Once resolved, stage the modified files:
git add resolved-file
- Finally, commit the resolution:
git commit -m "Resolved merge conflicts"
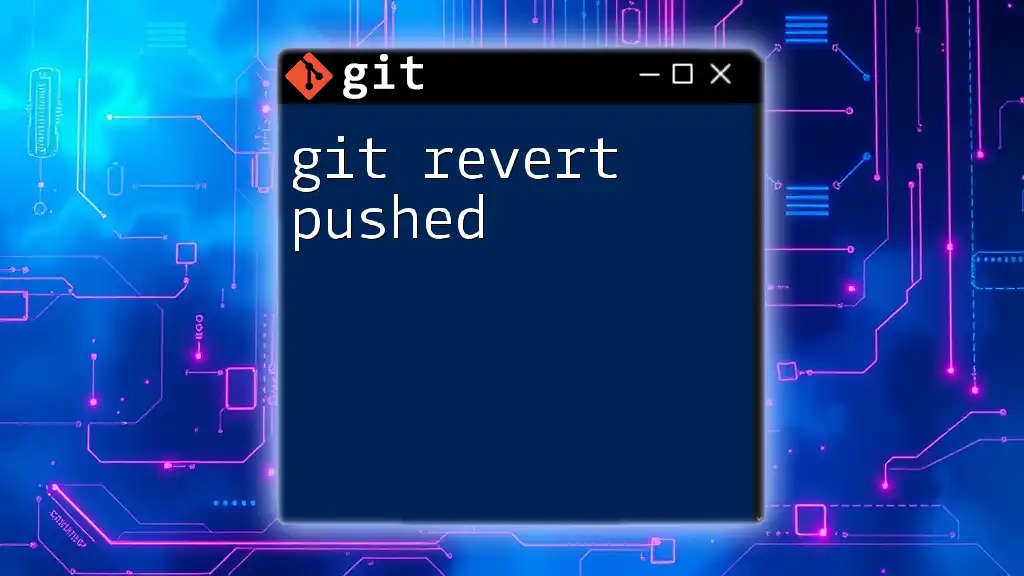
Best Practices for Reviewing Pull Requests
Components of a Good Review
As a reviewer, it's essential to comprehensively assess the changes:
- Code Quality: Check for readability, maintainability, and adherence to coding standards.
- Testing: Ensure that appropriate tests exist for the new features or bug fixes.
- Documentation: Verify that code changes are adequately documented.
Tools and Integrations
Utilize tools that can assist in reviewing PRs effectively, such as linters to enforce coding styles and Continuous Integration (CI) tools to automate testing. These integrations not only streamline the review process but also help catch issues early.
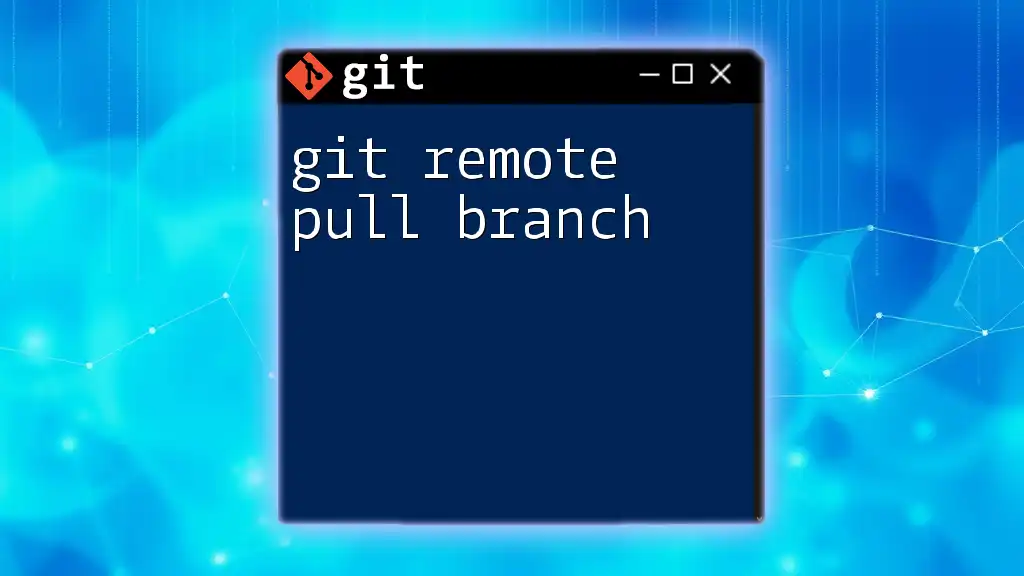
Conclusion
In summary, a git request pull is central to collaborative programming, promoting a structured way to introduce and discuss changes before merging them into the main codebase. By leveraging Pull Requests, developers can ensure higher code quality, facilitate communication, and maintain a healthy development workflow. Embrace the power of PRs in your projects, and take the first step towards an organized and efficient version control practice.
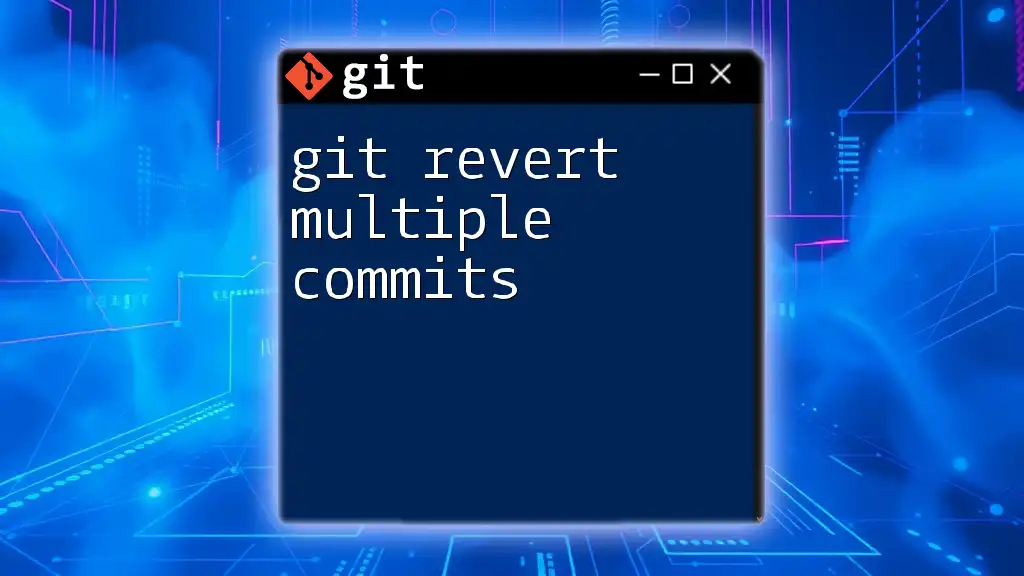
Further Reading and Resources
For those eager to deepen their knowledge, consider exploring a variety of materials:
- Official Git documentation
- Online courses focusing on Git techniques
- Best practices in collaborative coding environments
By incorporating the above practices into your workflow, you will not only enhance your own development experience but also contribute positively to your team's success in mastering Git.