To revert multiple commits in Git, you can use the `git revert` command followed by a range of commits to create new commits that undo the changes made by the specified commits.
Here's the code snippet:
git revert HEAD~3..HEAD
Understanding Git Revert
What is Git Revert?
`git revert` is a powerful command used in Git to undo changes made by previous commits while preserving the project’s commit history. Unlike `git reset`, which can erase commits entirely from the history, `git revert` creates a new commit that reverses the effects of a specified commit. This is crucial when working in collaborative environments where altering the history could lead to inconsistencies for other team members.
When to Use Git Revert
You might find yourself needing to use `git revert` in several scenarios. For instance, if a committed change introduces a bug or you realize that a previously made decision was incorrect, reverting that specific change can be beneficial. However, it’s essential to remember that using `git reset` could have adverse effects in a team environment, as it alters the commit history and can cause synchronization issues.
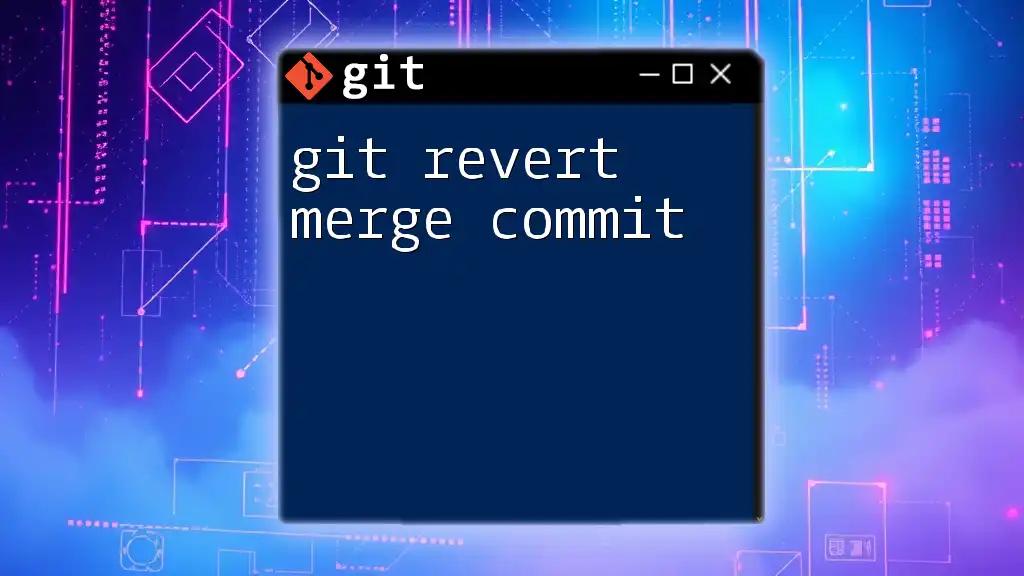
The Syntax of Git Revert
Basic Syntax of Git Revert
The basic syntax of the `git revert` command can be expressed as follows:
git revert <commit>
In this line, `<commit>` refers to the commit identifier (SHA-1 hash) you want to revert.
Understanding Commit References
Commit references can come from different sources:
- SHA-1 Hash: Every commit in Git has a unique SHA-1 hash that identifies it.
- Branch Names: You can also revert based on branch names, particularly if you want to revert the latest commit on a specific branch.
To find commit hashes, use:
git log
This command will display a history of commits, providing the hashes necessary for reverting.
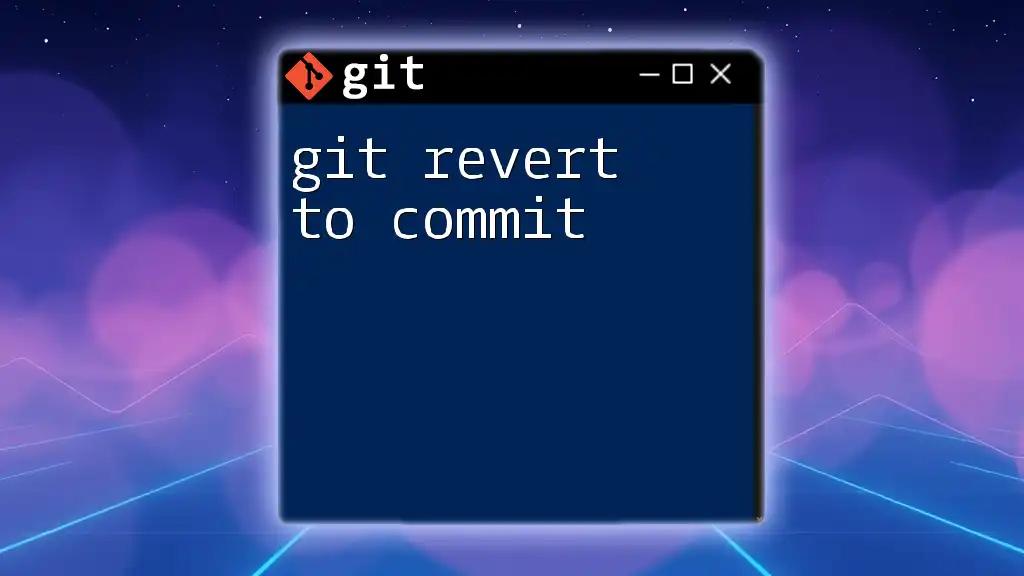
Reverting Multiple Commits
Reverting Multiple Commits in One Command
Reverting multiple commits in a single command can streamline your workflow. To do this, you may use a range of commits in your command:
git revert HEAD~3..HEAD
In this example, `HEAD~3` indicates the commit three places before the most recent commit (HEAD), and `HEAD` refers to the latest commit. This command effectively creates new commits that revert the last three changes.
Reverting Non-Sequential Commits
There are times when you may want to revert specific commits that are not in a continuous sequence. You can achieve this using multiple commands in one line:
git revert <commit1> <commit2>
By specifying the individual commit hashes, Git will revert each commit you list. Ensure you’re aware of the order because the effects of the earlier commits can affect the later ones.
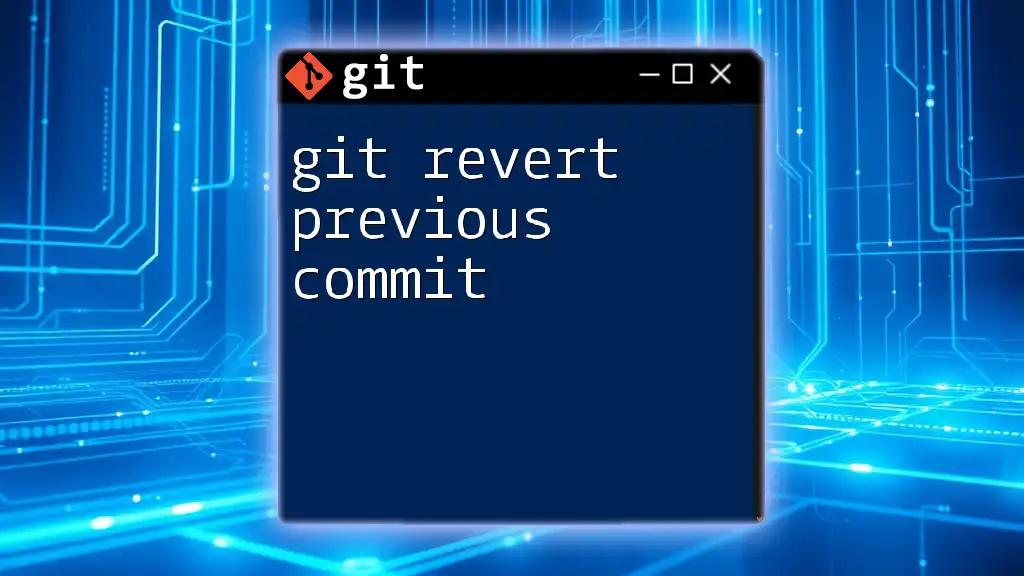
Handling Merge Commits
The Challenge of Reverting Merge Commits
Reverting a merge commit is more complex because it combines two branches into one. When performing a revert on a merge commit directly, you cannot apply a straightforward revert, as Git needs to know which parent to revert to.
Strategies for Reverting Merge Commits
To revert a merge commit, you can utilize the `-m` option, which specifies the parent number. Here’s how to go about it:
- Identify the Merge Commit: Use `git log` to locate the merge commit's SHA-1 hash.
- Perform the Revert: Execute the revert command with the `-m` option:
git revert -m <parent-number> <merge-commit>
In this command, `<parent-number>` is either `1` or `2`, depending on which branch you want to keep, and `<merge-commit>` is the SHA-1 of the merge commit.
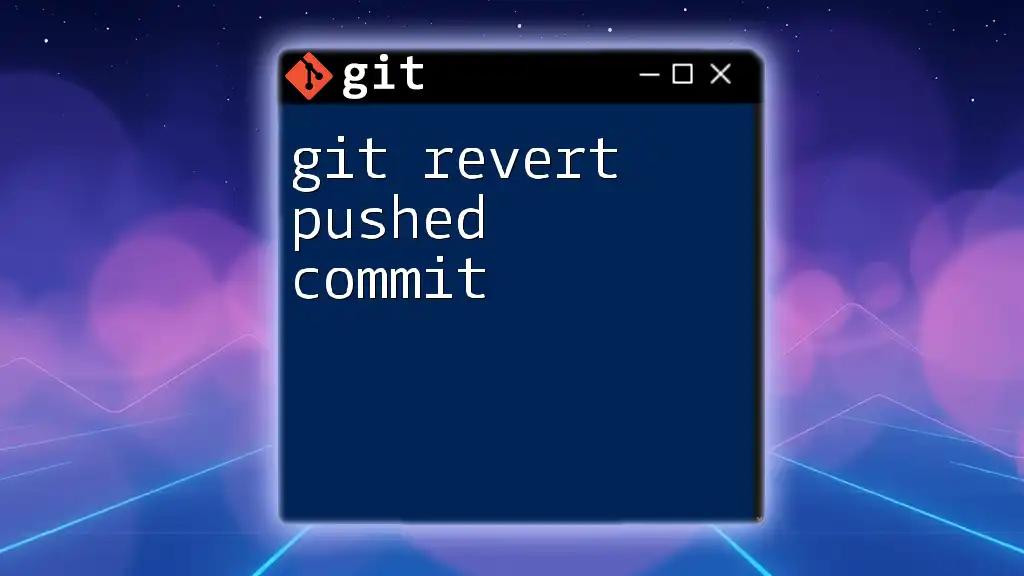
Finalizing the Reversion Process
Committing the Revert
Once you’ve performed a revert, it’s crucial to commit the revert changes. This creates a new commit in your history that explicitly states which changes have been reverted, preserving the clarity of the project’s development:
git commit -m "Reverted commit <commit-hash>"
Clear and descriptive commit messages are vital for tracking changes over time.
Verifying Your Changes
After reverting, you should verify that the changes were applied correctly. Use the following commands to confirm:
git log
git status
The `git log` command will show you the history, including your revert commits, while `git status` will provide the current state of your working directory and staging area.
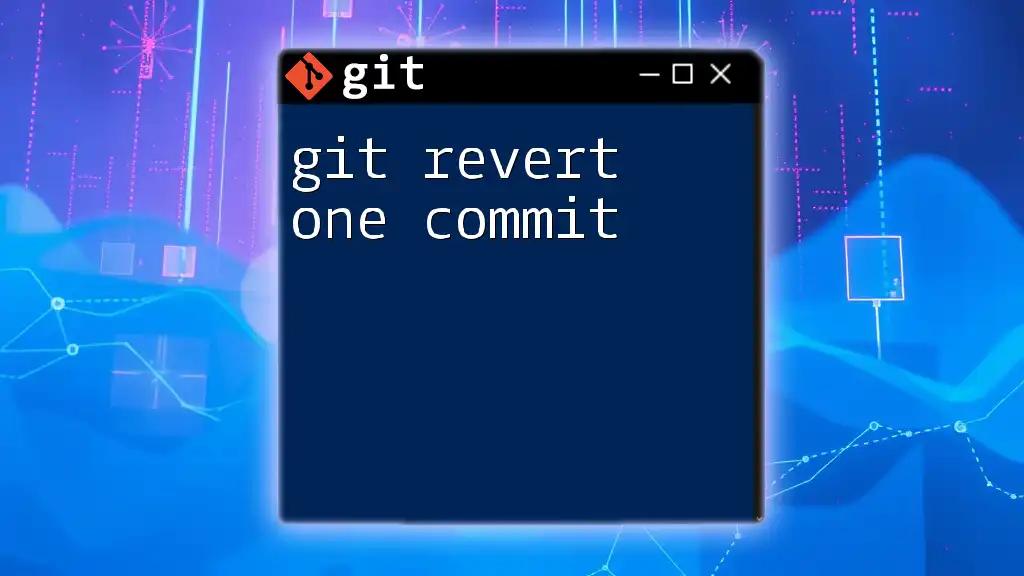
Best Practices for Reverting Commits
When to Revert vs. Other Solutions
It’s essential to understand when to use `git revert` versus other options like `git reset` and `git checkout`. While `git revert` is appropriate for maintaining history in a collaboration scenario, `git reset` might be acceptable in personal projects with no shared branches. Consider creating a new branch for extreme alterations to minimize potential disruption.
Documenting Your Revert Actions
Proper documentation of your revert actions is vital. Always include clear and informative commit messages in your `git revert` commands. Keeping a record of reverted commits in your project documentation can help team members understand the evolution of the project and the reasoning behind decisions made along the way.
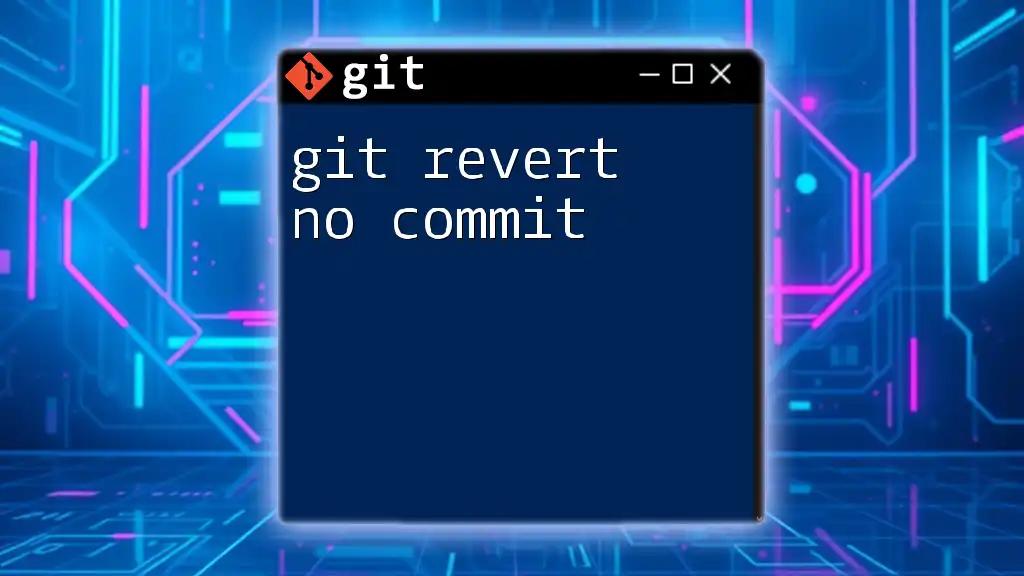
Conclusion
In summary, mastering the `git revert` command, especially in the context of "git revert multiple commits," is key to maintaining a clean and meaningful project history. Understanding when and how to apply these techniques will empower you to keep your codebase healthy and maintainable. Embrace these strategies, and don't hesitate to explore further through practice and study of advanced Git concepts.