To update a single file in your local repository from a remote repository using Git, you can use the following command to fetch that specific file without merging the entire branch.
git fetch origin main -- path/to/your/file && git checkout FETCH_HEAD -- path/to/your/file
What is Git?
Git is a distributed version control system that allows multiple developers to work on a project simultaneously without overwriting each other's changes. It keeps track of changes made to files and folders over time, enabling users to revert to earlier versions and understand modifications. The ability to manage different versions of a project efficiently is crucial for both individual and collaborative efforts.
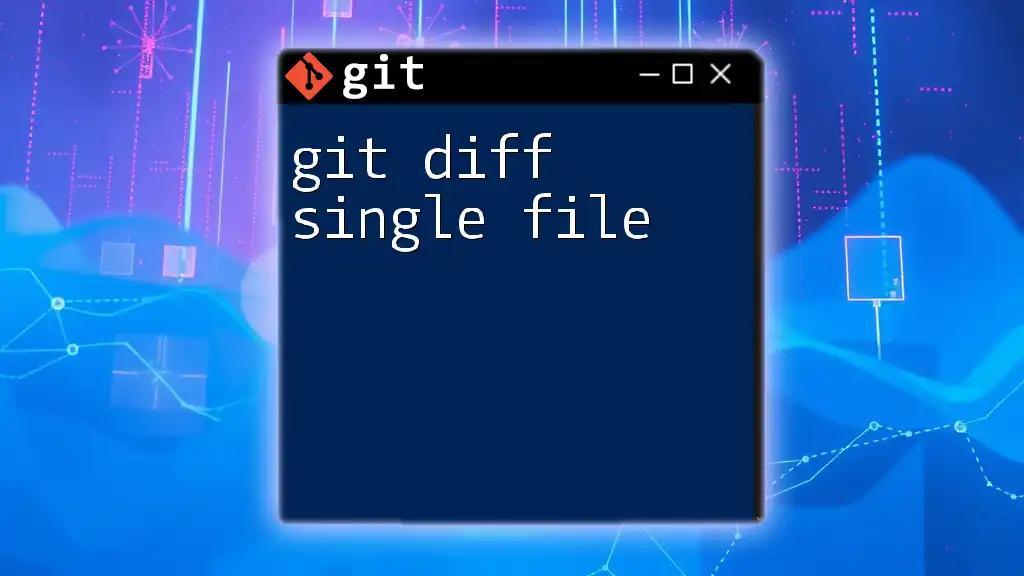
Understanding Git Pull
The `git pull` command is a vital tool in the Git ecosystem used to synchronize your local repository with a remote one. Essentially, it fetches changes from the specified remote branch and merges them into your current branch. Importantly, `git pull` combines two commands: `git fetch`, which retrieves updates, and `git merge`, which applies them.
It's essential to recognize the difference between `git pull` and `git fetch`. Using `git fetch` only pulls changes without applying them, allowing you to review changes before merging them into your working branch. In contrast, `git pull` automatically merges changes, which can sometimes lead to conflicts.
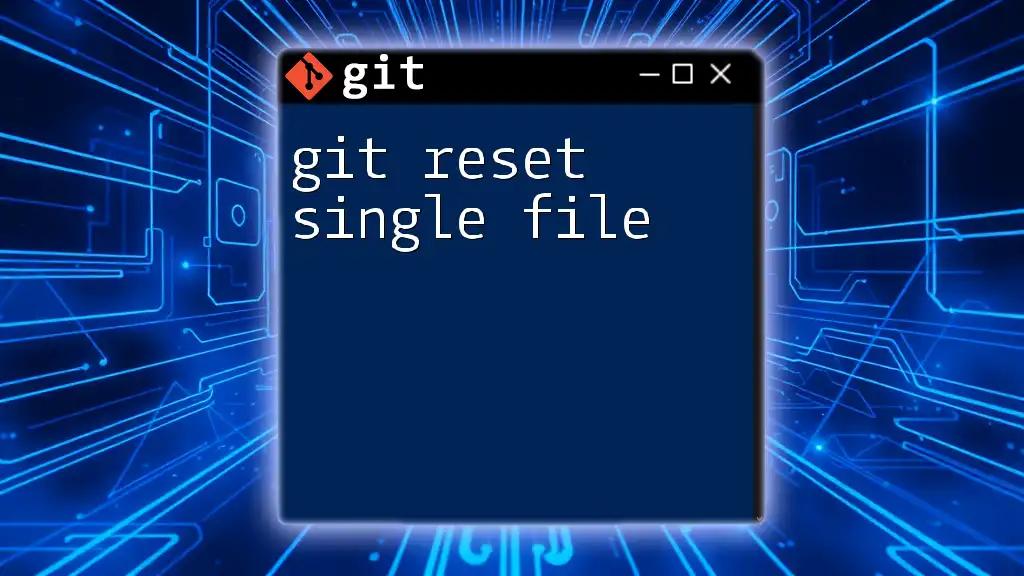
Why Pulling a Single File Can Be Useful
Pulling a single file instead of an entire branch or repository can be beneficial in various scenarios. For instance, if a colleague has made changes to a specific file that you need without altering the entire project, pulling just that file can save you significant time and bandwidth. Additionally, it avoids potential merge conflicts for unrelated files, allowing for smoother collaboration in busy projects.
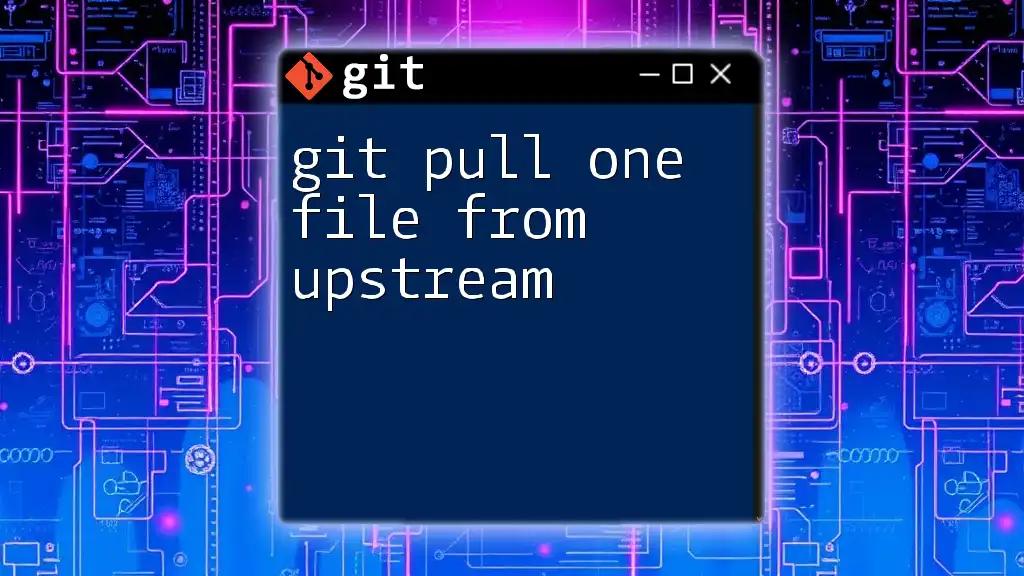
Setting Up Your Git Environment
Installing Git
To start using Git, you'll need to install it on your machine. This process may vary depending on your operating system, but you can typically download Git from the official [Git website](https://git-scm.com/). After installation, configure your user name and email with the command:
git config --global user.name "Your Name"
git config --global user.email "your.email@example.com"
This configuration sets your identity and is crucial for commit history.
Initializing a Git Repository
To begin your version control journey, you can either create a new repository using `git init` or clone an existing remote one via `git clone`. For example:
git clone https://github.com/username/repo.git
This command creates a local copy of the specified remote repository on your machine.
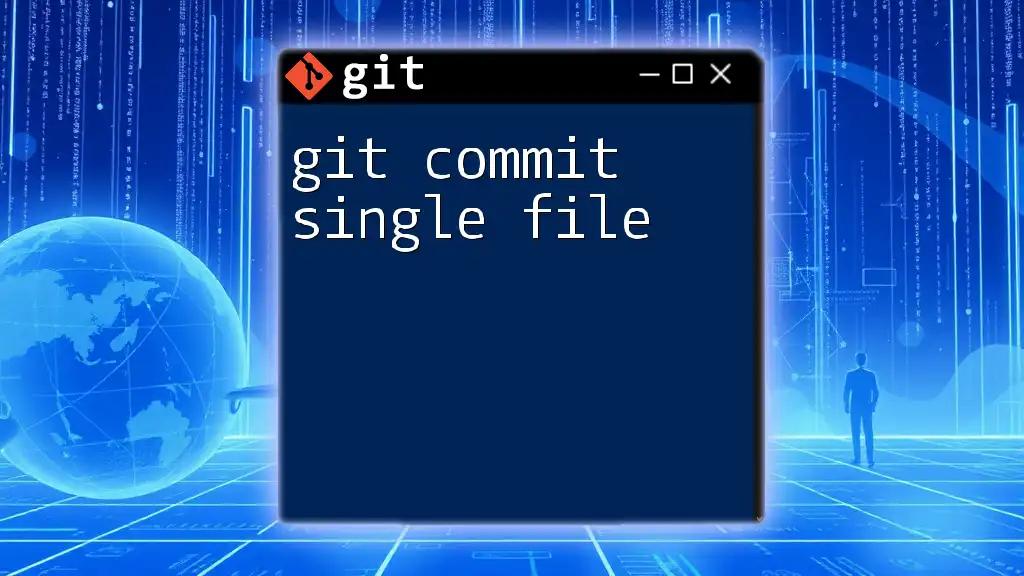
How to Pull a Single File with Git
Basic Syntax of Git Commands
When working with Git commands, it’s essential to understand their structure. Most commands follow a straightforward format:
git <command> [options] [arguments]
For pulling a single file, the command structure is crucial for successfully executing the operation.
Step-by-Step Guide to Pulling a Single File
Fetching the Latest Changes
Before pulling a specific file, it’s often a good practice to fetch the latest changes from the remote repository. You can do this using:
git fetch origin
This command retrieves updates from the remote without merging them. By doing so, you can view changes before applying them to your local files.
Checking Out a Specific File
Once you've fetched the changes, you can pull a specific file from the remote branch using the `git checkout` command:
git checkout origin/main -- path/to/your/file.txt
In this command:
- `origin` refers to the remote repository,
- `main` specifies the branch where the file exists,
- `path/to/your/file.txt` is the path to the file you wish to pull.
This command extracts the latest version of the specified file from the remote branch and replaces your local version.
Understanding the Impact of Pulling Files
When you pull a file, it directly replaces the local version with the one from the remote branch. If you have made local changes to that file, it's crucial to be aware that these changes will be overwritten. For safer practices, ensure you commit or stash any work on the file you’re pulling to avoid losing your local changes.
Additionally, while pulling a single file minimizes the chance of encountering merge conflicts, it doesn’t eliminate them entirely. If there are circumstances where the file has local modifications since the last pull, Git will notify you of a merge conflict that needs resolution.
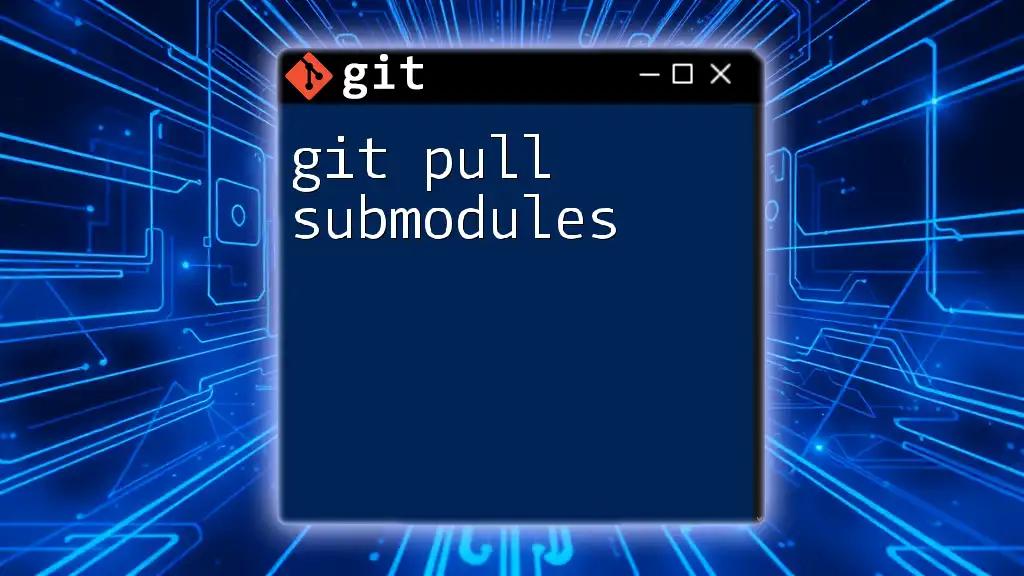
Dealing with Merge Conflicts
Identifying Merge Conflicts
In scenarios where you attempt to pull a file that has been changed both locally and remotely, Git faces a conflict it cannot automatically resolve. You’ll typically see a message indicating that there are conflicts, along with the conflicted file's name. This serves as a prompt to address and resolve the conflict manually.
Resolving Merge Conflicts
To resolve conflicts effectively, follow these steps:
- Open the conflicted file in your text editor. Git will mark conflicting sections with `<<<`, `===`, and `>>>`, revealing changes made in both branches.
- Review the changes to determine which parts to keep or modify.
- After resolving the conflicts, save the file and then mark it as resolved with:
git add path/to/your/file.txt
- Finally, commit your resolution using:
git commit -m "Resolved merge conflict on path/to/your/file.txt"
Best Practices
To minimize the likelihood of merge conflicts, consider employing the following strategies:
- Communicate frequently with team members about ongoing work to avoid overlapping changes on the same files.
- Pull changes regularly to ensure your local copy matches the remote repository, reducing the chances of conflicts when pulling specific files later.
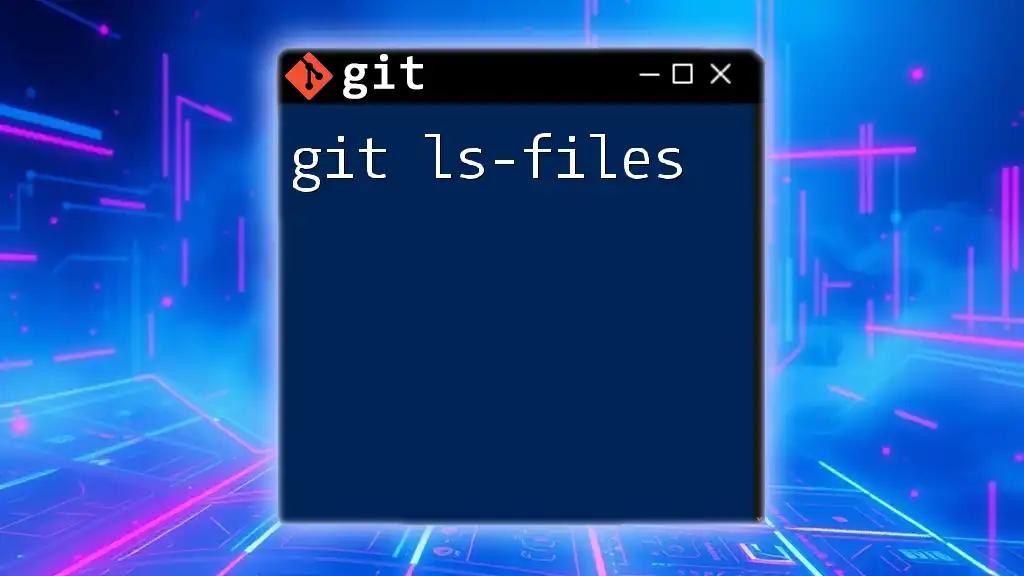
Alternatives to Pulling a Single File
Using Stash
If you're in a situation where local changes prevent you from pulling a file, consider temporarily saving your work with `git stash`. This command stores your changes and allows you to revert to the latest version from the remote before retrieving the specific file:
git stash
git fetch origin
git checkout origin/main -- path/to/your/file.txt
git stash pop
Checkout for Non-Tracked Files
If you need to access a file that hasn’t been tracked for version control, `git checkout` can retrieve a specific version from another branch. However, be cautious: using `git checkout` on non-tracked files can result in lost changes if the local file hasn’t been committed.
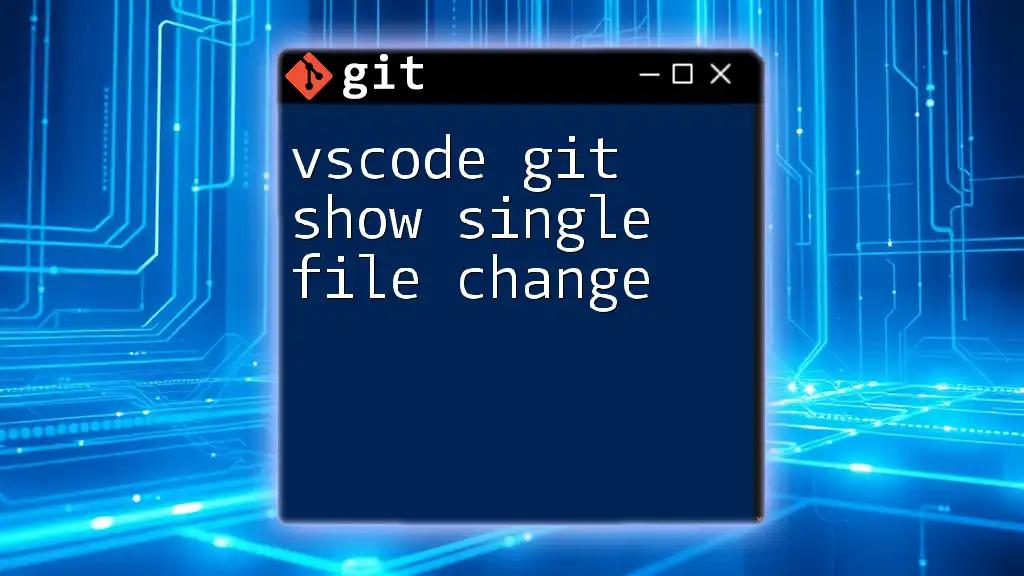
Conclusion
In summary, understanding how to utilize the `git pull single file` command can greatly enhance your workflow efficiency in Git. By focusing on precise file updates rather than full branch merges, you’ll save time and prevent unnecessary conflicts. Practice these commands and principles to become adept at managing your version control processes.
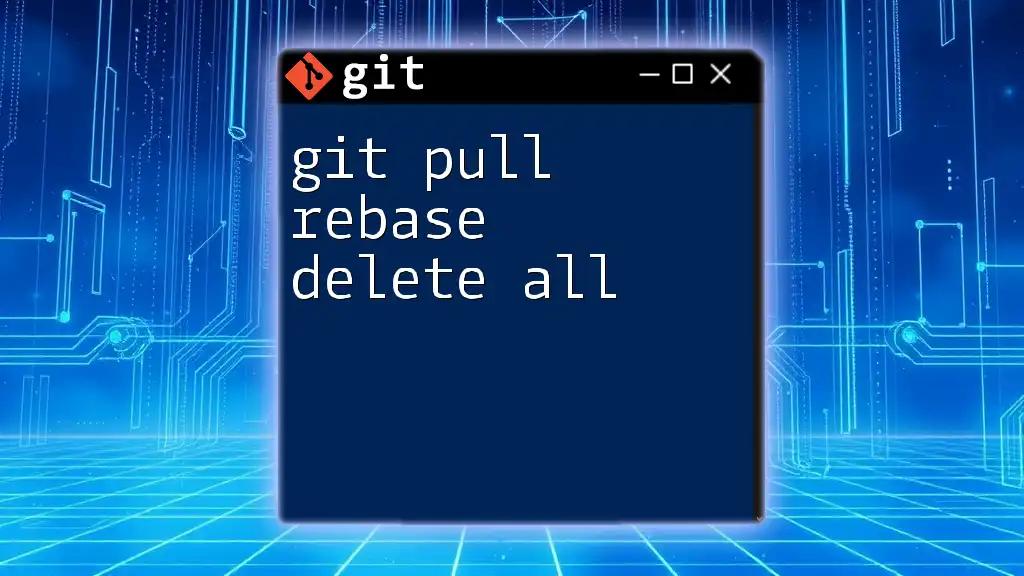
Additional Resources
For further reading and elaboration on Git commands, consider exploring [Git's official documentation](https://git-scm.com/doc) and reputable tutorials that delve deeper into Git's functionality and best practices.
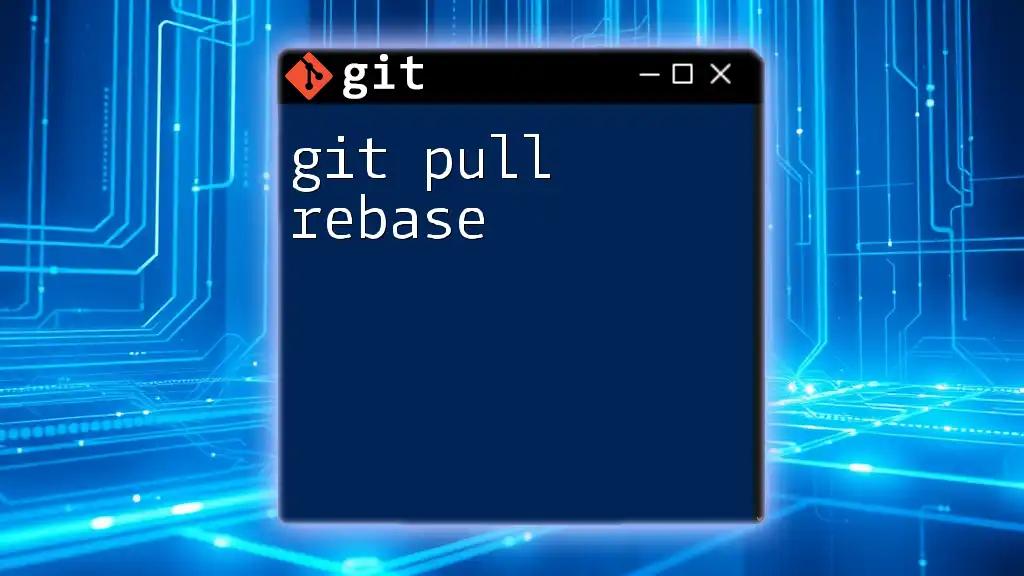
FAQs
Can I Pull Multiple Files at Once?
While Git does not have a direct command to pull multiple files in one operation as with `git pull`, you can specify several files in the `git checkout` command by listing them:
git checkout origin/main -- path/to/file1.txt path/to/file2.txt
What Happens to My Local Changes?
Before pulling a file, it’s essential to commit or stash any local changes to avoid overwriting them. Git will prompt you if you try to pull changes on a modified file.
Is Pulling a Single File in Separate Branches Possible?
Yes, you can pull a specific file from another branch by indicating the branch name in your checkout command:
git checkout other-branch -- path/to/your/file.txt
This allows you to selectively update files from different branches without merging them entirely.