The `git pull --rebase` command fetches changes from the remote repository and applies your local commits on top of them, allowing you to incorporate upstream changes while maintaining a cleaner project history.
Here's a code snippet illustrating the command:
git pull --rebase origin main
What is `git pull`?
Definition and Purpose
`git pull` is a command that facilitates the process of synchronizing your local repository with a remote one. It is a composite command that performs two tasks: it fetches the changes from the specified remote branch, and then it merges those changes into your current working branch. This is essential for maintaining an up-to-date local repository, especially when multiple developers are collaborating on a project.
Difference Between `git pull` and `git fetch`
While both commands are pivotal in the synchronization workflow, they serve distinct purposes. `git fetch` retrieves changes from the remote repository without integrating them into your current branch. This allows you to review changes before incorporating them. On the other hand, `git pull` automatically merges these changes, making it more of a hands-off approach.
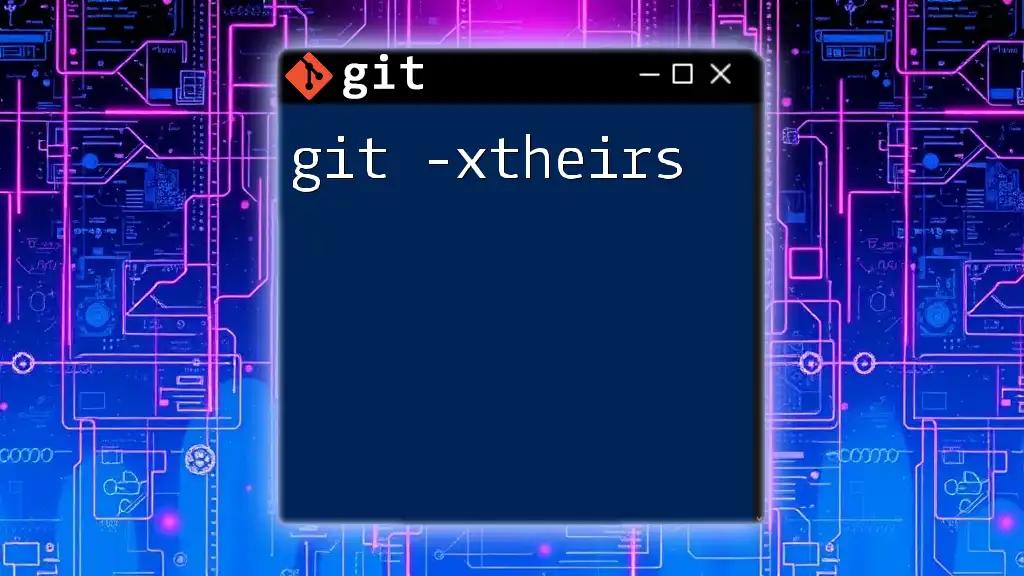
The Concept of "Theirs"
Understanding Merge Conflicts
A merge conflict occurs when two branches have competing changes that Git cannot automatically reconcile. This typically happens when two developers modify the same line of code in a file, create a new file with the same name, or modify the same file in similar locations. Identifying these conflicts is crucial as they require intervention to resolve.
The "Theirs" Strategy
In Git, the “theirs” strategy refers to prioritizing the changes from the branch being merged into your current branch during a conflict. Choosing “theirs” allows you to keep the updates from the remote branch instead of your local changes. This strategy can be particularly beneficial when you want to overwrite local changes in favor of collaborative updates that are considered more reliable or relevant.
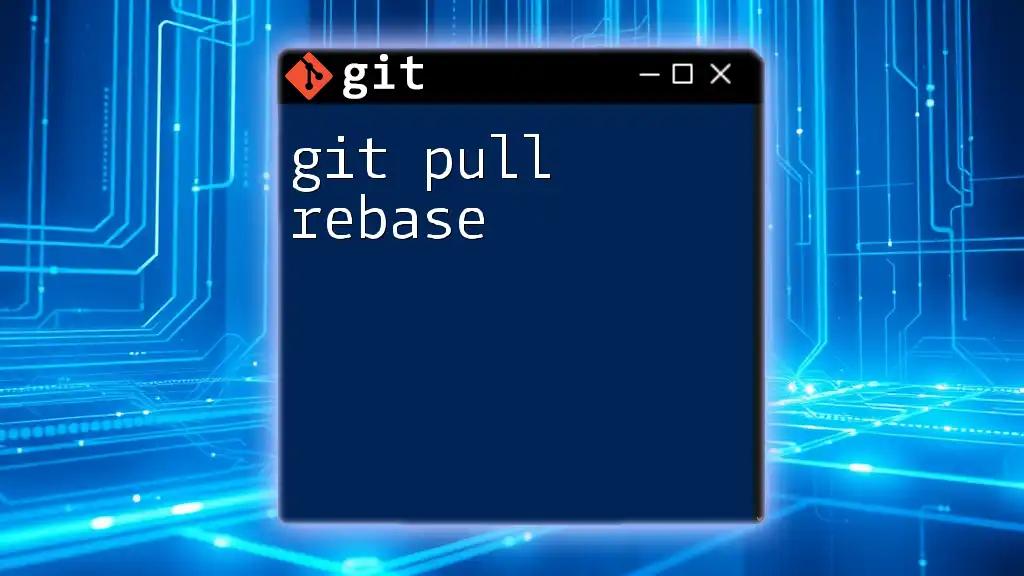
How to Use `git pull theirs`
Step-by-Step Workflow
Step 1: Identifying Conflicts
Before using `git pull theirs`, it's crucial to know if conflicts exist. You can check the status of your branch by executing:
git status
This command will alert you to any unmerged paths, enabling you to see where the conflicts occur.
Step 2: Initiating a Pull
To start the process, you need to pull the latest changes from the remote repository. The following command retrieves the changes from the `main` branch of the origin:
git pull origin main
During this, if there are any conflicting changes, Git will notify you, indicating the files that need resolution.
Step 3: Resolving Conflicts with "Theirs"
When a conflict arises, you can resolve it using the “theirs” strategy. By default, Git features a mechanism to allow you to favor "theirs" over your own local changes. You can specify this in your merge command. Use:
git checkout --theirs <filename>
This command retrieves the version of the file from the branch you are pulling from and overwrites your local changes.
Step 4: Finalizing the Merge
After resolving the conflicts using the “theirs” strategy, the next step is to add the resolved files to the staging area and commit the changes:
git add <filename>
git commit -m "Resolved conflict using theirs strategy"
This finalizes the merge, and you can now continue working in your repository with the latest updates from your team.
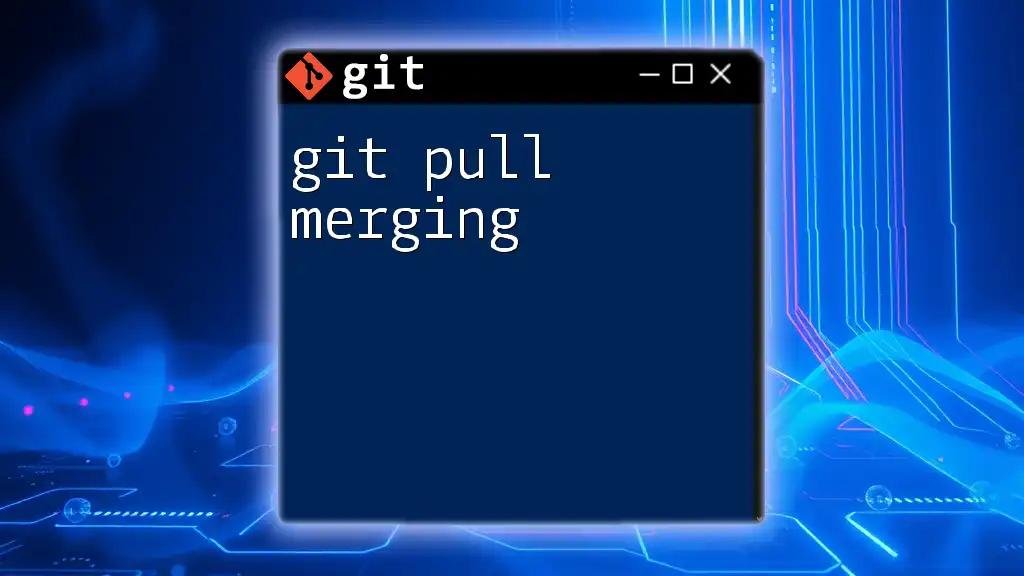
Practical Examples of `git pull theirs`
Scenario 1: Working in a Team
Consider a scenario with a team of developers all collaborating on the same project. Each developer works on different features but occasionally overlaps when modifying core files. Suppose you are working in a file called `app.js`, and your teammate has made updates that conflict with your local changes.
During a `git pull`, you realize there are conflicts. By opting for “theirs,” you might run:
git pull origin feature-branch
git checkout --theirs app.js
git add app.js
git commit -m "Resolved app.js conflict; favored teammates changes"
This way, you ensure that your team’s verified changes are maintained over your local, possibly untested modifications.
Scenario 2: Simple Feature Branch Merge
Imagine you have a feature branch that you’ve been working on, and when you attempt to merge this branch back into `main`, conflicts arise. To ensure that the more stable updates in `main` are preserved, you can use:
git pull origin main
git checkout --theirs <conflicted_file>
git add <conflicted_file>
git commit -m "Merged feature branch into main, resolved conflicts favoring theirs"
This reinforces the reliability of the code in the main branch and helps mitigate issues that can result from untested or less reliable feature branch changes.
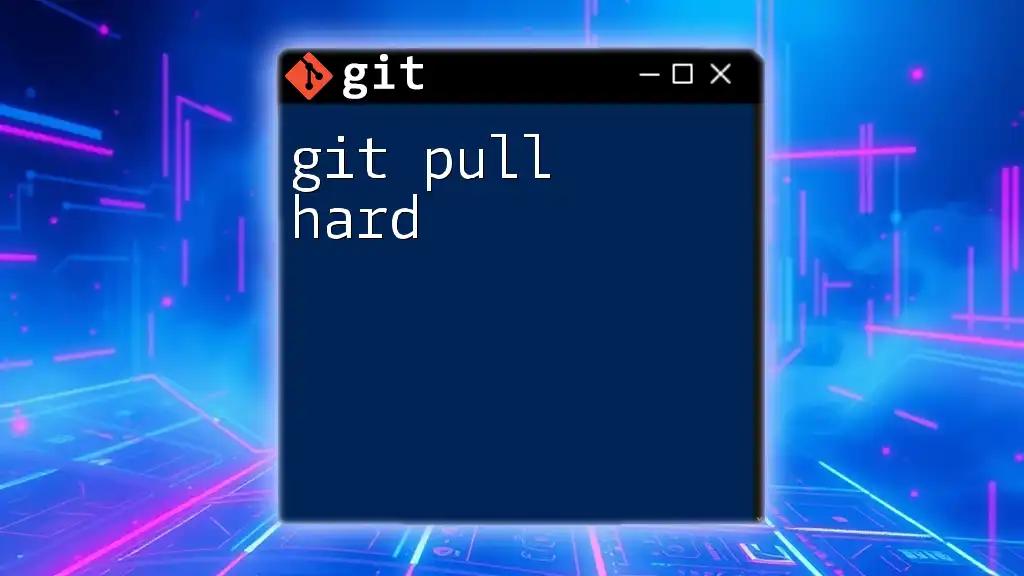
Best Practices for Using `git pull theirs`
When to Use "Theirs"
Using the “theirs” strategy can be beneficial in various scenarios, especially when you're new to the project and want to rely on more established changes made by other collaborators. However, it’s crucial to ensure that you really want to discard your local changes and that the remote changes are indeed safe and valid.
Cautions on Its Usage and Potential Risks
Though “theirs” can resolve conflicts efficiently, there’s a significant risk of losing code that might contain valuable updates. Always double-check that the changes from the remote repository align with project goals and coding standards before overwriting your work.
Alternatives to "Theirs"
Other merge strategies exist in Git, such as the “ours” strategy, where you keep your local changes over remote updates. Manual resolution is also an option if you wish to review both changes and select lines from each version.
It’s critical to understand the context of your changes and the overall impacts on the project when deciding the appropriate method for conflict resolution.
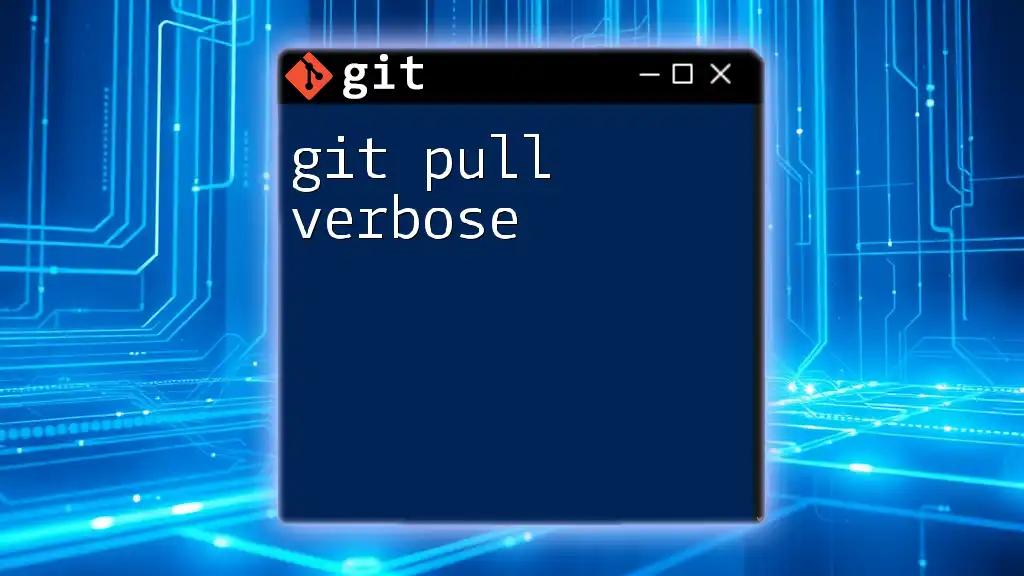
Efficient Workflow Tips
Regularly Fetching and Pulling Changes
To maintain a smooth workflow, it’s vital to regularly fetch and pull changes from the remote repository. This helps to minimize conflicts by keeping your local branch in sync with the ongoing updates from your colleagues. A simple command to keep your repository updated is:
git fetch origin
Communication in Teams
Effective communication amongst team members is paramount when working on shared projects. Utilize project management tools and regular meetings to align your objectives, which can significantly reduce the chances of conflicting changes.
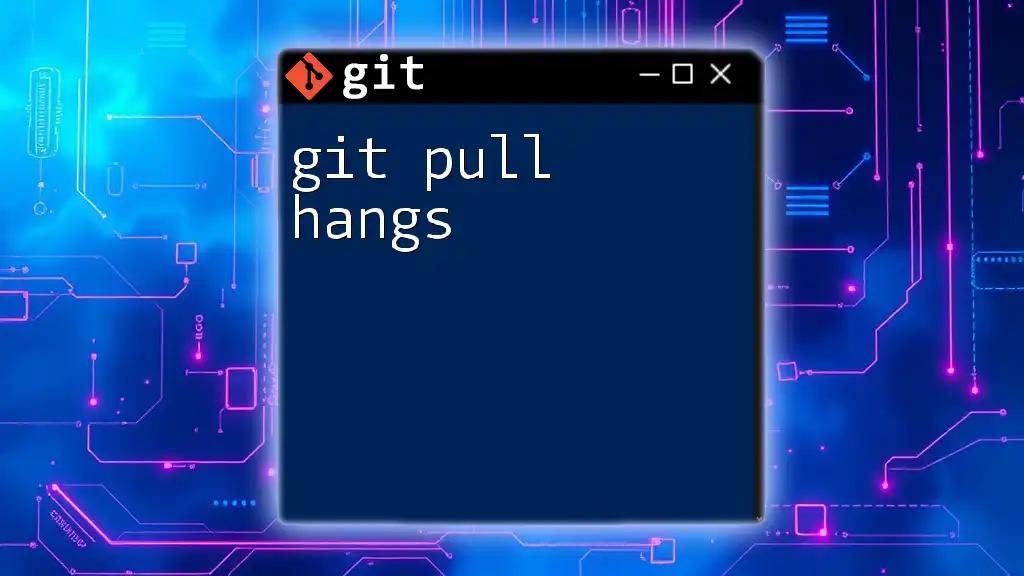
Conclusion
Mastering `git pull theirs` is an invaluable skill for any developer who collaborates in a team environment. It streamlines the conflict resolution process, ensuring that you can work more efficiently with your teammates. Continue learning and practicing your Git commands, as this will enhance your proficiency and confidence in version control systems.
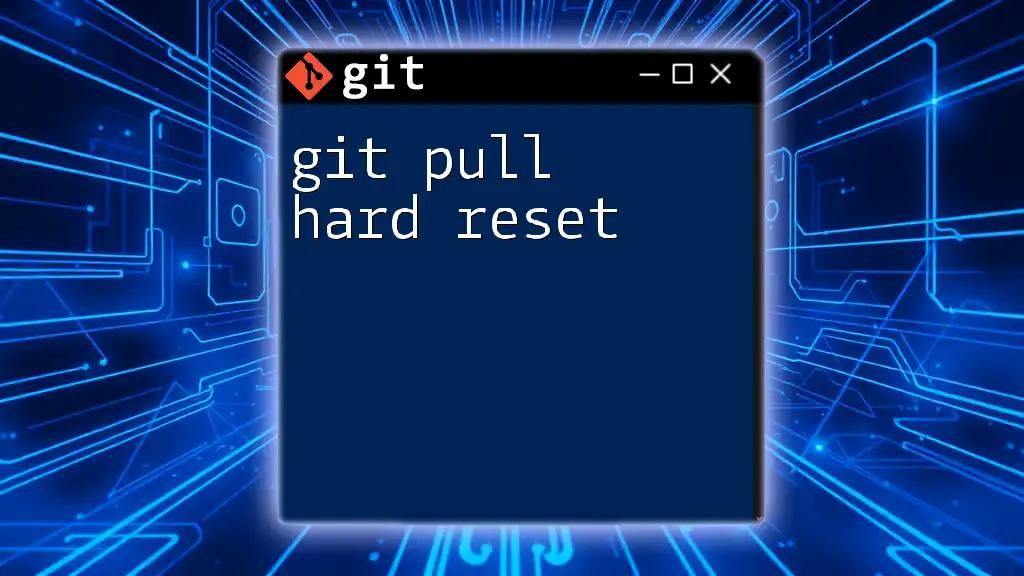
Additional Resources
To gain a deeper understanding of Git and its functionalities, consider exploring these invaluable resources:
- Official Git Documentation: Comprehensive reference for all commands and strategies.
- Recommended Tutorials and Courses: A plethora of online courses that cater to various skill levels.
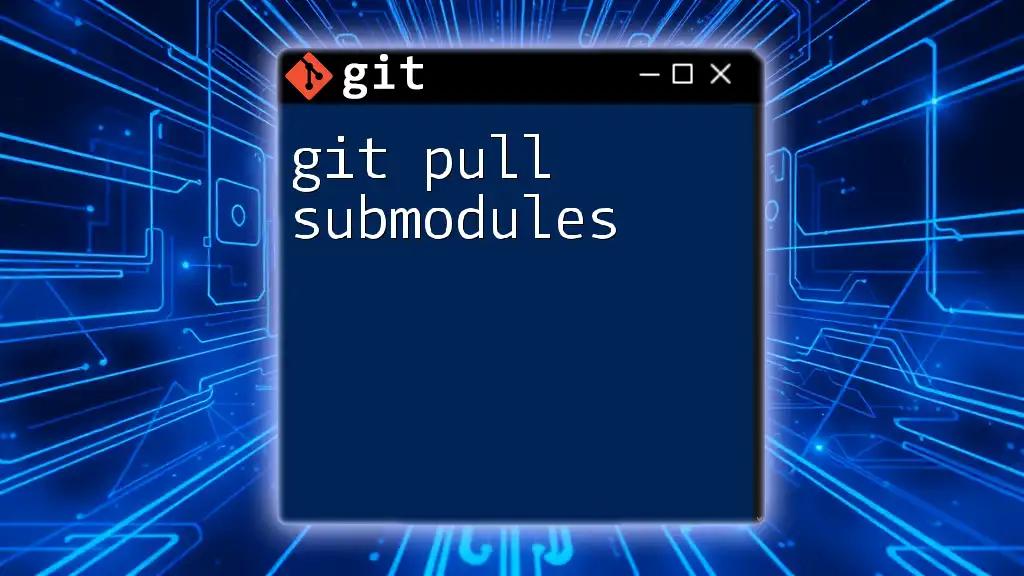
Call to Action
We invite you to share your experiences using the `git pull theirs` command! If you found this article useful, consider signing up for more concise tutorials and courses on Git commands to enhance your skills further.